{
"cells": [
{
"cell_type": "markdown",
"id": "c89ded84-826c-47a2-b200-ba7b028b2d5e",
"metadata": {
"jp-MarkdownHeadingCollapsed": true
},
"source": [
"#### M4W Series\n",
"\n",
"* [Table of Contents](M4WTOC.ipynb)\n",
"*
\n",
"* [](https://nbviewer.org/github/4dsolutions/m4w/blob/main/Polyhedrons.ipynb)"
]
},
{
"cell_type": "markdown",
"id": "1c719788-6ea7-4843-b3ee-c5ba21a4b2e7",
"metadata": {},
"source": [
"# Polyhedrons Я Objects\n",
"\n",
"
\n",
"\n",
"One of our signature moves is to present object-oriented thinking (as in computer science), with polyhedrons as paradigm objects. With the Polyhedron type come its methods for rotating and scaling itself about the origin.\n",
"\n",
"We start with a canonical arrangement of polyhedrons known to students of *Synergetics* as the concentric and or cosmic hierarchy. The accompanying volumes table centers around the unit edge tetrahedron as unit volume concept, and comes with a consistent vector algebra akin to XYZ's: [Quadrays](https://github.com/4dsolutions/Python5/blob/master/Polyhedrons.ipynb).\n",
"\n",
"Each polyhedron in the hierarchy (Tetrahedron, Cube, Octahedron...) is a subclass of Polyhedron, but with specific faces, tuples of vertices, hard-coded or selected from a database.\n",
"\n",
"Verboten Math (Martian Math) Volumes Table:
\n",
"
\n",
"\n",
"From *Synergetics*:
\n",
"
\n",
"\n",
"Rendered in Blender:
\n",
"
\n",
"\n",
"Think Kepler"
]
},
{
"cell_type": "markdown",
"id": "adfa45a5-5268-485c-b4e3-855ccb54ad2f",
"metadata": {},
"source": [
"## Supermarket Math\n",
"\n",
"To get the full experience of a database behind a web-based API, we store our [Polyhedrons](https://github.com/4dsolutions/Python5/blob/master/Polyhedrons%20101.ipynb) as related SQL tables and serve them out in response to HTTP requests. \n",
"\n",
"Starting with [SQLite](https://www.sqlite.org/index.html) inside of Python makes it easy, as no separate database engine need be started up or maintained. [`sqlite3`](https://docs.python.org/3/library/sqlite3.html) is already included in the [Python Standard Library](https://docs.python.org/3/tutorial/stdlib.html).\n",
"\n",
"A database is where we keep track of inventory, save stuff, bank.\n",
"\n",
"A typical table structure: a main table has one line per polyhedron, which points one-to-many to its faces, which in turn point one-to-many to the vertex vectors each face has, going around. Edges distill from faces, e.g. if we have face ('A', 'B', 'C') then we have edges 'AB', 'BC', 'AC'. To keep edge names unique, we tend to alphabetize them, such that 'CA' and 'AC' don't register separately. Just use 'AC'.\n",
"\n",
"* Main --> each Polyhedron (name, number of vertices, edges, faces)\n",
"* Faces --> Edges distill on the fly from Faces\n",
"* Vertexes --> XYZ and/or Quadray coordinates, canonical positions\n",
"\n",
"See my [Digital Mathematics from the Silicon Forest](https://wikieducator.org/Digital_Math) for more context (with Supermarket, Martian, Neolithic and Casino Math).\n",
"\n",
""
]
},
{
"cell_type": "markdown",
"id": "73b0692c-2793-4b14-8dbc-d3a1b7d59de3",
"metadata": {},
"source": [
"## Python Warmup\n",
"\n",
"About `*` and `**` as \"splat apart\" vs \"smash together\" operators respectively"
]
},
{
"cell_type": "code",
"execution_count": 1,
"id": "48a990da-fcef-44c3-beb4-bc5e69eaba11",
"metadata": {},
"outputs": [
{
"data": {
"text/plain": [
"([1, 2], [3, 4])"
]
},
"execution_count": 1,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"f = lambda *x: x # combine as a tuple\n",
"\n",
"arg = ([1, 2], [3,4]) # two lists inside a tuple\n",
"\n",
"f(*arg) # splat apart tuple then smash lists together"
]
},
{
"cell_type": "markdown",
"id": "19c2d047-73ad-4682-ae38-c4b0a0b0c3ac",
"metadata": {},
"source": [
"## Polyhedron Play\n",
"\n",
"In this section we're doing a closed loop transformation involving what David Koski and I have dubbed the \"S factor\", meaning the volumetric ratio of the S to E modules.\n",
"\n",
"Another way to compute the same sfactor is to ratio the cuboctahedron with the icosahedron of same edges, the two being related by the Jitterbug Transformation.\n",
"\n",
"The sfactor, applied to the cuboctahedron (CO) of volume 2.5 (half the edges of the edge 2R CO, R = IVM ball radius) may be modeled as the beginning of a twisting action, akin to the Jitterbug, but wherein edges are allowed to lengthen. \n",
"\n",
"The trianglar faces twist in a way that keeps them flush with a containing octahedron of volume 4. Two applications of the sfactor take us to the icosahedron with eight of its faces flush to said Octahedron. \n",
"\n",
"
\n",
"\n",
"**From: RBF, Synergetics, Fig. 988.00 Polyhedral Evolution: S Quanta Module: Comparisons of skew polyhedra.**\n",
"\n",
"This relationship of the two polys (octa and icosa) begets the S modules in the first place: 24 of them (12L and 12R) brick in the difference. 24 S modules, applied to the icosahedron, build its containing volume 4 octahedron.\n",
"\n",
"We call this faces-flush icosahedron the IcosaWithin. The 2.5 CO morphs into the IcosaWithin with two applications of the sfactor i.e. one application of the 2nd power of the sfactor.\n",
"\n",
"This IcosaWithin has edges equal to the sfactor. Multiplying by the reciprocal of the sfactor therefore models shrinking the IcosaWithin to one of edges 1R, i.e. 1/8th the volume of the Jitterbug Icosa, the one with edges equal the volume 20 CO's.\n",
"\n",
"But those edges 1 match the those of the 2.5 volumed CO. One more application of the sfactor will therefore bring us full circle back to 2.5. Then we may start the whole cycle again: 2.5 CO times sfactor times sfactor brings us to IcosaWithin, which times 1/sfactor brings us to 1R edged Icosa, times the sfactor again brings us back to the 2.5 volumed CO. "
]
},
{
"cell_type": "code",
"execution_count": 2,
"id": "fae50931-1748-4b95-9faa-d3324d43e02c",
"metadata": {},
"outputs": [],
"source": [
"import sympy as sy\n",
"from sympy import Symbol, symbols"
]
},
{
"cell_type": "markdown",
"id": "74875af6-c9e3-47a1-b536-fd8cc490e9d4",
"metadata": {},
"source": [
"Fuller wanted to make a fresh start with Synergetics, free of much cultural baggage, or at least that's one explanation for why he expelled $\\phi$ from the proceedings. However, bringing $\\phi$ back considerably simplifies the symbolic expressions, $\\phi$ being a symbol for the golden mean.\n",
"\n",
"$$\n",
"\\phi:1 :: (1 + \\phi) : \\phi\n",
"$$\n",
"\n",
"$$\n",
"\\phi = (\\sqrt{5} + 1)/2\n",
"$$"
]
},
{
"cell_type": "code",
"execution_count": 3,
"id": "ac78f153-9027-4bde-ab3f-713888cfd132",
"metadata": {},
"outputs": [
{
"data": {
"text/plain": [
"[1/2 - sqrt(5)/2, 1/2 + sqrt(5)/2]"
]
},
"execution_count": 3,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"φ = Symbol('φ')\n",
"sy.solve('φ - (1 + φ)/φ')"
]
},
{
"cell_type": "code",
"execution_count": 4,
"id": "13e67eac-d1f8-4648-844b-c7af3f166f9a",
"metadata": {},
"outputs": [],
"source": [
"phi = (sy.sqrt(5)+1)/2"
]
},
{
"cell_type": "code",
"execution_count": 5,
"id": "35b6690b-ccdd-41d1-9c56-5cbba1b27c5c",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 1.6180339887498948482045868343656381177203091798058$"
],
"text/plain": [
"1.6180339887498948482045868343656381177203091798058"
]
},
"execution_count": 5,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"phi.evalf(50)"
]
},
{
"cell_type": "markdown",
"id": "6aca9a97-bb6f-4164-920e-25561fee9e16",
"metadata": {},
"source": [
"
\n"
]
},
{
"cell_type": "code",
"execution_count": 6,
"id": "51a4a527-c8e7-45cd-8877-b872f72e82ae",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 18.5122958682192$"
],
"text/plain": [
"18.5122958682192"
]
},
"execution_count": 6,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"icosavol = 5 * sy.sqrt(2) * phi**2 # icosa of edges 2R\n",
"icosavol.evalf()"
]
},
{
"cell_type": "code",
"execution_count": 7,
"id": "196ee950-ea1f-4587-aac7-83c76bfb1e35",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle - \\sqrt{10} + 3 \\sqrt{2}$"
],
"text/plain": [
"-sqrt(10) + 3*sqrt(2)"
]
},
"execution_count": 7,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"sfactor = sy.Integer(20)/icosavol # CO:Icosa ratio = S:E ratio\n",
"sfactor = sfactor.simplify()\n",
"sfactor"
]
},
{
"cell_type": "code",
"execution_count": 8,
"id": "792922cc-fe4f-4e61-bf64-3b0f98419e8f",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 1.08036302695091$"
],
"text/plain": [
"1.08036302695091"
]
},
"execution_count": 8,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"sfactor.evalf()"
]
},
{
"cell_type": "markdown",
"id": "412e0f91-cc19-4283-bdef-d2533af83521",
"metadata": {},
"source": [
"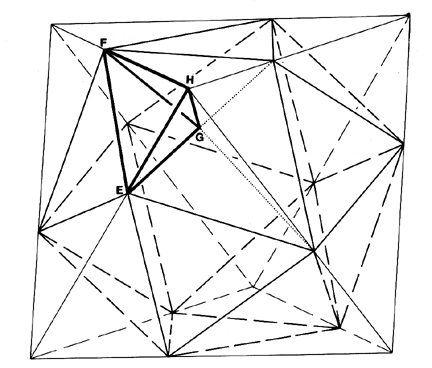\n",
"\n",
"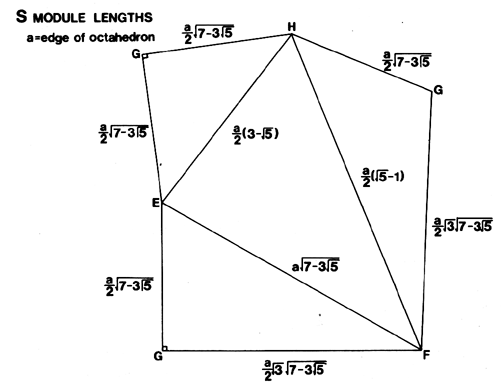"
]
},
{
"cell_type": "code",
"execution_count": 9,
"id": "d0f1474d-29de-4f07-b570-8cd2d8218ce5",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 1.08036302695091$"
],
"text/plain": [
"1.08036302695091"
]
},
"execution_count": 9,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"a = Symbol('a')\n",
"a = 2\n",
"FE = a * sy.sqrt(7 - 3 * sy.sqrt(5))\n",
"FE.evalf() # also sfactor"
]
},
{
"cell_type": "markdown",
"id": "f7fa51c1-c106-4364-bacd-f85e7c9ea867",
"metadata": {},
"source": [
"$$\n",
"S Factor = 2\\sqrt{7 - 3\\sqrt{5}}\n",
"$$"
]
},
{
"cell_type": "code",
"execution_count": 10,
"id": "3c1cf700-a1b6-4c2e-9b96-c1c73bcb63ba",
"metadata": {},
"outputs": [],
"source": [
"icosa_xyz = a**3 * (5/12)*(3 + sy.sqrt(5)) # textbook XYZ volume"
]
},
{
"cell_type": "code",
"execution_count": 11,
"id": "ec0620af-3385-44bc-bb36-cf78e5591018",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 17.4535599249993$"
],
"text/plain": [
"17.4535599249993"
]
},
"execution_count": 11,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"icosa_xyz.evalf()"
]
},
{
"cell_type": "code",
"execution_count": 12,
"id": "ac0cab19-99c7-44b4-8e6b-90aa78fb024c",
"metadata": {},
"outputs": [],
"source": [
"S3 = sy.sqrt((9/8)) # Synergetics constant"
]
},
{
"cell_type": "code",
"execution_count": 13,
"id": "9537b762-9ec0-4699-8d1b-d011d41241b2",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 1.06066017177982$"
],
"text/plain": [
"1.06066017177982"
]
},
"execution_count": 13,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"S3"
]
},
{
"cell_type": "code",
"execution_count": 14,
"id": "e1b2fd91-a8fb-4632-a1be-1d74d6893563",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 18.5122958682192$"
],
"text/plain": [
"18.5122958682192"
]
},
"execution_count": 14,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"S3 * icosa_xyz.evalf() # convert to tetravolumes"
]
},
{
"cell_type": "code",
"execution_count": 15,
"id": "556872e2-e92b-462f-a0d6-fb76fa53da5f",
"metadata": {},
"outputs": [],
"source": [
"IcosaWithin = sy.Rational(5,2) * sfactor**2 # 2.5 CO to IcosaWithin\n",
"Smod = (4 - IcosaWithin)/24\n",
"Smod = Smod.simplify()"
]
},
{
"cell_type": "code",
"execution_count": 16,
"id": "034448c2-7e31-47f9-a235-a1733bb9c01d",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle - \\frac{11}{4} + \\frac{5 \\sqrt{5}}{4}$"
],
"text/plain": [
"-11/4 + 5*sqrt(5)/4"
]
},
"execution_count": 16,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"Smod"
]
},
{
"cell_type": "code",
"execution_count": 17,
"id": "ff13548d-67a5-4470-bec8-f6d15a148c60",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 4.0$"
],
"text/plain": [
"4.00000000000000"
]
},
"execution_count": 17,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"(24 * Smod + IcosaWithin).evalf()"
]
},
{
"cell_type": "code",
"execution_count": 18,
"id": "46afccf7-b640-4e03-b8c9-5c2ad587d16e",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 1.08036302695091$"
],
"text/plain": [
"1.08036302695091"
]
},
"execution_count": 18,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"sfactor.evalf()"
]
},
{
"cell_type": "code",
"execution_count": 19,
"id": "bc264eaf-a9bb-4d75-8f7f-5d51d7d449a0",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 2.314036983527395149501237411581816490446$"
],
"text/plain": [
"2.314036983527395149501237411581816490446"
]
},
"execution_count": 19,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"tinyme = (icosavol/8).evalf(40) # 1/8th icosa of edges 2R, edges R\n",
"tinyme"
]
},
{
"cell_type": "markdown",
"id": "dab47fb2-9713-4af0-b75a-f27029ffb9c8",
"metadata": {},
"source": [
"Volume changes as a 3rd power of the scale factor as applied to edges. For example if we apply 1/sfactor then the corresponding volume changes by a 3rd power of 1/sfactor. This is only if we assume the shape is held constant, in terms of surface and central angles."
]
},
{
"cell_type": "code",
"execution_count": 20,
"id": "ae350949-9383-49e2-b279-183fa4629669",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 18.5122958682192$"
],
"text/plain": [
"18.5122958682192"
]
},
"execution_count": 20,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"(8 * IcosaWithin * (1/sfactor**3)).evalf() # check"
]
},
{
"cell_type": "code",
"execution_count": 21,
"id": "c2277f8e-9080-49cf-acd0-5463763e7520",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 2.314036983527395149501237411581816490446$"
],
"text/plain": [
"2.314036983527395149501237411581816490446"
]
},
"execution_count": 21,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"(IcosaWithin * 1/sfactor**3).evalf(40) # same as tinyme"
]
},
{
"cell_type": "code",
"execution_count": 22,
"id": "7e13258f-553f-47ec-9fd4-a78b653fa3c2",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 2.5$"
],
"text/plain": [
"2.500000000000000000000000000000000000000"
]
},
"execution_count": 22,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"(tinyme * sfactor).evalf(40) # Jitterbug from icosa to CO, back to 2.5"
]
},
{
"cell_type": "markdown",
"id": "a3101600-f909-463a-9a3b-3c25c86fcf32",
"metadata": {},
"source": [
"When we apply the sfactor to the 2.5 CO of edges 1R, we might imagine it going, right there and then, to a CO of edges sfactor and volume 2.5 times the sfactor to the 3rd power.\n",
"\n",
"This very CO may then be shrunk to IcosaWithin with same length edges, and embedded in the Octa 4, by the application of 1/sfactor."
]
},
{
"cell_type": "code",
"execution_count": 23,
"id": "6dd1dc1e-be0c-48b3-8597-b6b245da7de4",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 2.91796067500631$"
],
"text/plain": [
"2.91796067500631"
]
},
"execution_count": 23,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"(2.5 * sfactor**3 * 1/sfactor).evalf() # IcosaWithin"
]
},
{
"cell_type": "markdown",
"id": "b00bc218-ea0e-4ce4-ae78-2d2c8c54de95",
"metadata": {},
"source": [
"David Koski: Too cool Icosawithin is 60S + 20s3\n",
"\n",
"What does he mean? The S module scaled up by phi in linear dimensions, has its volume impacted by phi to the 3rd power. S3 (capital S) means stretch S mod legs by phi, and so scale up volume by phi to the 3rd. s3 (lowercase s) means shrink S mod legs by 1/phi, and so scale down volume by (1/phi) to the 3rd."
]
},
{
"cell_type": "code",
"execution_count": 24,
"id": "7e0bdd25-ec79-4b34-8e3e-2346416d1a7e",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 2.917960675006309107724789938061712936781$"
],
"text/plain": [
"2.917960675006309107724789938061712936781"
]
},
"execution_count": 24,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"(60 * Smod + 20 * Smod * (1/phi**3)).evalf(40)"
]
},
{
"cell_type": "code",
"execution_count": 25,
"id": "51d54bde-b84c-4093-ad12-c400a4419d34",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle \\frac{5 \\left(- \\sqrt{10} + 3 \\sqrt{2}\\right)^{2}}{2}$"
],
"text/plain": [
"5*(-sqrt(10) + 3*sqrt(2))**2/2"
]
},
"execution_count": 25,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"IcosaWithin"
]
},
{
"cell_type": "code",
"execution_count": 26,
"id": "a909db0e-13a5-498c-8bd6-0f22750ee5d8",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 2.917960675006309107724789938061712936781$"
],
"text/plain": [
"2.917960675006309107724789938061712936781"
]
},
"execution_count": 26,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"IcosaWithin.evalf(40)"
]
},
{
"cell_type": "markdown",
"id": "6c5fee72-e3fa-4db9-ab90-30f163f322ad",
"metadata": {},
"source": [
"## Fun with Classes\n",
"\n",
"Now it's time to do some actual object oriented programming by defining a poly type that may be hit with either a volume scaler, or an edge scalar. These two have a consistent power relationship meaning to rescale volume by X is to rescale edges by ${X}^{1/3}$. To rescale edges by X is to rescale volume by $X^{3}$.\n",
"\n",
"\n",
"David Koski:\n",
"\n",
"Fundamental to E->S is phi scaling:\n",
"* E = (4) e3 + (1) e6\n",
"* S = (4) s3 + (1) s6"
]
},
{
"cell_type": "code",
"execution_count": 27,
"id": "ddf7332b-c680-4450-823d-ce108ff05209",
"metadata": {},
"outputs": [],
"source": [
"class Poly:\n",
"\n",
" def __init__(self, spine, vol):\n",
" \"\"\"\n",
" spine may be any linear dimension we care about\n",
" volume is shape volume\n",
" \"\"\"\n",
" self.edge = spine\n",
" self.volume = vol\n",
"\n",
" def __mul__(self, scale_v):\n",
" \"\"\"\n",
" scale_v resizes volume, and edges by a 3rd root of scale_v\n",
" \"\"\"\n",
" return type(self)(self.edge * scale_v**(1/3), self.volume * scale_v)\n",
"\n",
" def __call__(self, scale_e):\n",
" \"\"\"\n",
" scale_e resizes edges, and volume by a 3rd power of scale_e\n",
" \"\"\"\n",
" return type(self)(self.edge * scale_e, self.volume * scale_e**3)"
]
},
{
"cell_type": "code",
"execution_count": 28,
"id": "989841e1-98b6-47af-b6cf-c7717f30b6fd",
"metadata": {},
"outputs": [],
"source": [
"rt2 = sy.sqrt # map sympy sqrt to rt2 for 2nd root\n",
"φ = (rt2(5)+1)/2 # golden ratio -- same as phi above\n",
"\n",
"E = Poly(1, (rt2(2) / 8) * (φ**-3)) # home base Emod\n",
"e3 = E * φ**-3 # small e, phi down\n",
"e6 = e3 * φ**-3 # small e, phi down again\n",
"E3 = E * φ**3 # capital E, phi up\n",
"E6 = E3 * φ**3 # phi up yet again\n",
"\n",
"S = Poly(sfactor, (φ**-5) / 2) # home base Smod\n",
"s3 = S * φ**-3 # small s, phi down\n",
"s6 = s3 * φ**-3 # small s, phi down again\n",
"S3 = S * φ**3 # capital s, phi up\n",
"S6 = S3 * φ**3 # phi up yet again"
]
},
{
"cell_type": "code",
"execution_count": 29,
"id": "6c371a09-bbf6-416d-aa4d-29aed33d95a0",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle \\frac{\\sqrt{2}}{8 \\left(\\frac{1}{2} + \\frac{\\sqrt{5}}{2}\\right)^{9}} + \\frac{\\sqrt{2}}{2 \\left(\\frac{1}{2} + \\frac{\\sqrt{5}}{2}\\right)^{6}}$"
],
"text/plain": [
"sqrt(2)/(8*(1/2 + sqrt(5)/2)**9) + sqrt(2)/(2*(1/2 + sqrt(5)/2)**6)"
]
},
"execution_count": 29,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"(4 * e3.volume + e6.volume)"
]
},
{
"cell_type": "code",
"execution_count": 30,
"id": "fbca030f-aafb-4416-a2a3-b28eb8687ef8",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle \\frac{\\sqrt{2}}{8 \\left(\\frac{1}{2} + \\frac{\\sqrt{5}}{2}\\right)^{9}} + \\frac{\\sqrt{2}}{2 \\left(\\frac{1}{2} + \\frac{\\sqrt{5}}{2}\\right)^{6}}$"
],
"text/plain": [
"sqrt(2)/(8*(1/2 + sqrt(5)/2)**9) + sqrt(2)/(2*(1/2 + sqrt(5)/2)**6)"
]
},
"execution_count": 30,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"rt2(2)/(8*φ**9) + rt2(2)/(2*φ**6)"
]
},
{
"cell_type": "code",
"execution_count": 31,
"id": "9c1bbc97-cfa9-4406-a8f3-2a5bcaa7edb3",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 0.04173131692777365429943951200166529707253$"
],
"text/plain": [
"0.04173131692777365429943951200166529707253"
]
},
"execution_count": 31,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"(4 * e3.volume + e6.volume).evalf(40)"
]
},
{
"cell_type": "code",
"execution_count": 32,
"id": "9dc46cc5-f84b-4314-8bb6-60ba1655163a",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 0.04173131692777365429943951200166529707253$"
],
"text/plain": [
"0.04173131692777365429943951200166529707253"
]
},
"execution_count": 32,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"E.volume.evalf(40)"
]
},
{
"cell_type": "code",
"execution_count": 33,
"id": "52fc4819-ff52-4278-876a-1eb463d31c9b",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle \\frac{2 \\sqrt{2}}{\\left(\\frac{1}{2} + \\frac{\\sqrt{5}}{2}\\right)^{2}}$"
],
"text/plain": [
"2*sqrt(2)/(1/2 + sqrt(5)/2)**2"
]
},
"execution_count": 33,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"S.volume/E.volume"
]
},
{
"cell_type": "code",
"execution_count": 34,
"id": "d83f5ef2-c6e4-485b-84d5-635c2a666ca3",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle \\frac{2 \\sqrt{2}}{\\left(\\frac{1}{2} + \\frac{\\sqrt{5}}{2}\\right)^{2}}$"
],
"text/plain": [
"2*sqrt(2)/(1/2 + sqrt(5)/2)**2"
]
},
"execution_count": 34,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"(2 * rt2(2))/φ**2"
]
},
{
"cell_type": "code",
"execution_count": 35,
"id": "fb374bc0-3f0b-4415-9fb0-1511c1b1ef1d",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 1.080363026950905814406172628196375701989$"
],
"text/plain": [
"1.080363026950905814406172628196375701989"
]
},
"execution_count": 35,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"((2 * rt2(2))/φ**2).evalf(40)"
]
},
{
"cell_type": "markdown",
"id": "0ea8d9af-eaa5-4acf-9f5f-5059d0b22f6e",
"metadata": {},
"source": [
"The expression below is what we find in the pages of Synergetics, plane net for the S. It's the same number, but purged of $\\phi$."
]
},
{
"cell_type": "code",
"execution_count": 36,
"id": "fdca0c93-78ac-4ce2-a681-3203ac2a9703",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 2 \\sqrt{7 - 3 \\sqrt{5}}$"
],
"text/plain": [
"2*sqrt(7 - 3*sqrt(5))"
]
},
"execution_count": 36,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"2 * rt2(7 - 3 * rt2(5))"
]
},
{
"cell_type": "code",
"execution_count": 37,
"id": "b08ef909-02e3-4990-97cc-faae9f1fea0a",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 1.080363026950905814406172628196375701989$"
],
"text/plain": [
"1.080363026950905814406172628196375701989"
]
},
"execution_count": 37,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"2 * rt2(7 - 3 * rt2(5)).evalf(40)"
]
},
{
"cell_type": "markdown",
"id": "65787c9d-dc73-44de-92d6-37aaba5a045d",
"metadata": {},
"source": [
"Yes, we may scale a volume while preserving its shape, giving that simple 3rd power change relationship, between the linear and volumetric. Double the edges, eight-fold the volume. However, we may also grow or shrink a volume by transforming it to another shape. We think of the Jitterbug Transformation, the cuboctahedron (CO) to icosahedron transformation, from volume 20 to 18.51 as \"shape shifting\". In this case, the edges didn't resize at all, yet the volume shrank by a factor of 1/sfactor. \n",
"\n",
"Application of 1/sfactor in this case is not meant to preserve the CO shape.\n",
"\n",
"What we show below is a closed loop where we start from the 2.5 volumed CO(R) embedded in Octa(D). Two applications of the sfactor (i.e. 2nd power) stretches its legs as the VE twist-opens into Icosa(sfactor), faces embedded in Octa(D) all along the way.\n",
"\n",
"An additional shape shifting application of sfactor turns Icosa(sfactor) back into a CO with sfactor edges (i.e. unchanged -- the usual Jitterbug action).\n",
"\n",
"Now that we're at this larger CO(sfactor) of volume about 3.15, one application of 1/sfactor, strictly as a shape-preserving scaling factor this time, will fast track us back, in one step, back to the CO(R) of 2.5.\n",
"\n",
"It's like an express train versus a local. The local scenic route goes from CO(R) through some other intermediate step along the Octa(D) edges rail line -- what the CO(R)'s vertices follow when opening to IcosaWithin (volume ~2.92). \n",
"\n",
"Yet a 3rd application of sfactor, as a shape shifter, does the classic Icosa --> Cubocta Jitterbug while preserving edge lengths. We're now at our destination: CO(sfactor) i.e. a cuboctahedron with edges (and radii) equal sfactor (S:E).\n",
"\n",
"The express route is CO(sfactor) back to CO(R) direct, with 1/sfactor turning edges of sfactor (~1.08) back to edges of 1R, back to where we started in one step.\n",
"\n",
"In sum: CO(R) -> E2S -> E2S = IcosaWithin -> E2S = CO of edges E2S. CO -> S2E goes right back to CO(R) if S2E is applied as a resizer, not as a reshaper. That's after importing from a namespace developed below (E2S is the S factor, i.e. E * E2S -> S where E2S reshapes E to S)."
]
},
{
"cell_type": "code",
"execution_count": 38,
"id": "ab7e22b6-dd8f-4408-9fce-cab7c1557771",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 2.91796067500631$"
],
"text/plain": [
"2.91796067500631"
]
},
"execution_count": 38,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"CO = Poly(1, 2.5)\n",
"IcosaWithin = CO * sfactor * sfactor\n",
"IcosaWithin.volume.evalf()"
]
},
{
"cell_type": "code",
"execution_count": 39,
"id": "4f119cdc-bc0e-4df4-8349-cb017a7eeedf",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 3.15245682737352$"
],
"text/plain": [
"3.15245682737352"
]
},
"execution_count": 39,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"COmax = IcosaWithin * sfactor\n",
"COmax.volume.evalf()"
]
},
{
"cell_type": "code",
"execution_count": 40,
"id": "e3306f61-11d7-48a8-9099-52824a96b453",
"metadata": {},
"outputs": [
{
"data": {
"text/plain": [
"<__main__.Poly at 0x7fb4c9b17c40>"
]
},
"execution_count": 40,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"COmax"
]
},
{
"cell_type": "code",
"execution_count": 41,
"id": "d09dfa2e-e96b-44f0-b12d-050dc3042c55",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 2.5$"
],
"text/plain": [
"2.50000000000000"
]
},
"execution_count": 41,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"(COmax(1/sfactor)).volume.evalf()"
]
},
{
"cell_type": "code",
"execution_count": 42,
"id": "92458cf1-80b0-493c-8890-56c0669c6680",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 2.91796067500631$"
],
"text/plain": [
"2.91796067500631"
]
},
"execution_count": 42,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"(CO * sfactor * sfactor).volume.evalf()"
]
},
{
"cell_type": "code",
"execution_count": 43,
"id": "7f22afe5-a5d7-4924-aded-e940b8a99fda",
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"2.70090756737726\n"
]
}
],
"source": [
"print((CO * sfactor).volume.evalf()) # somewhere along the Octa(D) rail system"
]
},
{
"cell_type": "code",
"execution_count": 44,
"id": "ab94e922-3361-4816-919e-967ec0d927ac",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 18.5122958682192$"
],
"text/plain": [
"18.5122958682192"
]
},
"execution_count": 44,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"(8 * (CO.volume * 1/sfactor)).evalf()"
]
},
{
"cell_type": "markdown",
"id": "ed92016b-f4c0-45a0-9ac0-a197f6dc2950",
"metadata": {},
"source": [
"## BEAST modules\n",
"\n",
"
\n",
"\n",
"
\n",
"\n",
"
\n",
"\n",
"Here's a quick primer if you do not remember or have no yet encountered the BEAST modules:\n",
"\n",
"[More about BEAST (Synergetics 101)](https://groups.io/g/synergeo/message/2495) Synergeo listserv Feb 13, 2024\n",
"\n",
"Here's a video review as well (about 30 mins):"
]
},
{
"cell_type": "code",
"execution_count": 45,
"id": "64233640-16e3-44e0-84e4-b7e35825db4f",
"metadata": {},
"outputs": [
{
"data": {
"image/jpeg": "/9j/4AAQSkZJRgABAQAAAQABAAD/2wCEABALDBoYFhsaGBodHRsfIC0mIiIiIygnKCUlLigxMC0nLTI2PVBCNThLOS0tRmFFS1NWW1xbMkFlbWRYbFBZW1cBERISGRYZMBsbMFdCN0NXV1dXXV1dV1dXV1dXV11XXVdYXVdXV1dXV19XV11XWFdXV1dXV1dXV1dYV1dXV1dXV//AABEIAWgB4AMBIgACEQEDEQH/xAAbAAEAAgMBAQAAAAAAAAAAAAAAAQUDBAYCB//EAEsQAAIBAgIECgcGBAMGBgMAAAABAgMEBRESITFRBhMWQVRhcZGS0RQiMoGhsfAzNEJScsEjU2LhNWTxByRzgqKyFSVEg6OzQ2OT/8QAGgEBAAMBAQEAAAAAAAAAAAAAAAECAwQFBv/EADMRAAIBAgMFBwQCAgMBAAAAAAABAgMREiExE0FRcbEEMmGBkaHRIsHh8CNCM2JScvE0/9oADAMBAAIRAxEAPwD5+AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAD1oPcNBk2ZNmeQetBkaLFmLMgE6LGixZixAJ0WNFizIsQCdFjRYsxYgE6LGixZixAJ0WNFizFiATosaLFmLEAnQZOgxZix5BOgydBizFjyD1oMcW9wwvgTZnkHrQY0GML4EWPIPWgxoMYXwFjyD06b3DQZOF8BY8g9aDGgyML4Cx5B60GNBjC+AseQelTe4mVGSeTWsnBLWxNmeAeuLe4jQZGF8BYgE6LJ0GLMix5B60GNBjCxY8gnRZOgxZix5BOixosWYIBOixosWYIBOQyFmCATkMhYEAnRYyFmLEAZAgAEqOZAAAJyAIBOiyeLe4mzJszK2C65JYh0Wfih5kcksQ6LPxQ8zS6NLopQXXJLEOiz8UPMcksQ6LPxQ8xdEXRSAu+SOIdFn4oeY5I4h0Wfih5i6FykBd8kcQ6LLxQ8xyRxDo0/FDzF0RcpCS65I4h0WXih5jkjiHRZeKHmLoXKUgvI8EcQ57aS/5oeZHJLEOiy8UPMtdElISmXXJHEOiy8UPMckcQ6LLxQ8xdEXKUF1ySxDo0vFDzHJLEOiy8UPMm6FymBdLgliHRpeKHmOSWIdFl4oeYuibopQXXJLEOiy8UPMjkliHRZeKHmTiRFymBdcksQ6LLxQ8xySxDo0vFDzJxIXKUkueSWIdGl4oeY5JYh0aXih5jEuIuUoLrkliHRZeKHmOSWIdFl4oeYxIXKUF1ySxDosvFDzHJLEOjS8UPMYlxFylDLrkliHRpeKHmOSWIdGl4oeYxIXRSkvYXPJLEOjS8UPMcksQ6NLxQ8xiQuUqJbLnkliHRpeKHmOSWIdGl4oeZOJcRcp9JtZHll1ySxDo0vFDzHJLEOjS8UPMhyT3i5SAuuSWIdGl4oeY5I4h0aXih5lboXKbIhl1yRxDosvFDzHJHEOiy8UPMYkLlKe3JaOWWveW/JHEOiy8UPMcksQ6LLxQ8wpJEplIQXfJHEOiy8UPMckcQ6LLxQ8yt0VKQF3yRxDosvFDzHJHEOiy8UPMi6BSZEF5yRxHosvFDzI5IYj0WXih5i6BSBF3yQxDosvFDzHJDEOiy8UPMi6IKXS1ZEZF5yQxDosvFDzJ5JYhll6LLxQ8xkSUALzkfiPRZeKHmOR+I9Fl4oeZUqUhBecj8R6LLxQ8yVwQxDosvFDzIJKPIIvpcEcQz1Ws/FDzPPJDEeiy8UPMtkSynpyyaPdaab1LIteSOI9Fl4oeY5I4j0WXih5mm0+nCTiPsIJBgUIBIAIBIAIBIAIBIAIBIAIJAAAAAIBIAIJAABBIAIJAAAAAAAAAAABBIAIBIAIJAAIJAAAAAIBIAIJAAAAABBIAAAAIBIAAAABBIAIBIAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAABBp4piVO1pOpU7Ix55PcinwLhJKrV4q5ioSnrpvLJNPZHX8HzlXJJ2No0Kk4OaWSOlBBJYxAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAABr3t5ChTlUqPKMfj1LrMletGnCU5tRjFZts4bGL6d1/GkmqWlo29PnnLY5Nc+Xkt5ScsKOns9Dayz0FtxmK3qlPVShra5ow5o9r89xc8LcIVSiq1OOU6S5ueC5vdt7yxwDDFa26i/tJetN/wBW73Fk0VjD6c95tV7VaqnT7scl++JznBfhBx64ms/4sVqf515nRnCYphCt7vRg+LjUelQnsUZ/lb3Z6urNdZ0mBYw66dKstC5p6pxerPL8SEJPRjtVGL/lpaPPl++xcAgk1OAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAHmclFNt5JLNt8yJOZvq88RrO2oNxt4P+LVX4v6Vv8Ap9sN2NadPG88ktWY61SWJVXm3CxovOUtnGNft8u3Z6wSh6Xcu6cdGhS9ShDm1c+X1rfUesZebpYbapRzy08vww26/m/7nQ2ltGjTjTgsoxWSM0rs66lTBTyyvp4LjzfQykgGp55X41hquqEqb1S2we6S+sjnaFOd1T4yDcL+29WW+aWzPr5v9TsTm8coytbiF9SXqtqNaK509Wfy96RnNbzt7NUb/j37ufDkyzwTFo3VPPLRqx1VIPbF+RZHO4nZPON/Za55aUorZUhlu35fWaLXCsSp3VJVKb/VHni9zLRe5mNWmrY4adHw+DdABYwAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAABBJoY5cOlaV5p5NQeT3N6l8WGTFXdirxa+qXVV2Vo/8AjVOaC519dm/Lfqyo4daPRWUYrUuec3v62crwaw1VdOnOtXo1clPKMslKLSafx+Jkt8I9Lup0o1q07eltnKWectmUeb/QwxPW2Z6kqNNfS5fTHN5a+fQveDNhKMZXNb7avrefNHmXv29xeFDyY/zdz4xyY/zdz4y6ulaxzVNnUk5Ofsy+BQ8mf83deMcmP83deMm8uBns6X/P2ZfHivRjUhKE1nGSaa6mUnJj/N3PjHJj/N3PjF3wChSX9/ZmHAa0rW4nY1Xmvaot86evL650ycUsp2dZ3lqm4v7ektjX5kvrfvNfFeC8o0nVp161SrBZpTlnqW3LnzMFhZU6to7iV5cpRT4xaeyS5vfzdpnnpY7bQk9rGWuTVtX+ep1dleQr0o1KbzjLvT50+s2DiuBU5U7mpSkpKNSmqkE9yeSfc/gdqaxd1c4e0UlSqOK0AALGAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAOe4a1H6Fxa21akYpe/P8AZHQnMcKq0fSbKE2lBTdSXZHJ/systDbs6vUXr6GvwsjGh6PKlJxrxi4LR2umo5a/rnZd8HLenTtKfFPSUlpOW+T292z3FdgVu7qvUvq0dTzjSi+aOxv5rvJwuTsruVpJ/wAKr69FvmfPH63dZmtcXE7KmdPYp5xzfjxXl8nSAgk2PNMVesqcXKXuS2tvYl1swYTeu4tqdZx0dOOeWeeWvZmZ7i3hVi4zipLrSeT36+cxYbYQtqMaNLPQjszyz9+8A2gAAQcFi1OlTv5UVUcbepOLrJbE888v36s+o6/GcRVrQlUeuWyC3yewrbHg+pWU41vtq/rzk9sZbV3fuzOaxZI7uyzVJOctHl+fLqY8QSo4rZzjqjOm6erZks8v+5HSnz+7vpRpUY1tVazrpPe4b+vYl3HfomDvcz7TTcMN+X75MkAFzlAINHFsYoWcNOtPLdFa5S7EWjFydorMG+DjLPhy+Okrig6dLNJSSbcM9mn2/WZ19CvCpBTpyUovWmnmmaVaFSl30QmmZAQSYkgAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAEHB8KpQqYio1JOMIRjFvLPJa5Npb9Z3pUY5gVO7jnqhWXsz/aW9FKkW1kdXZKsaVTFL/wwUeE1jCEYQm1GKSS0JakvcV+PYzZ3NH1KklVg9Km9CS9Zc2eXP5GxhF9BVPRbyjThXWpScY5VFze9/Ev/QqX8qn4UVzktTVunRnfC766rP2KSz4W27pQdaTjUy9ZKLaz6sjPyss/5kvBLyNG9t4WV9Gq4R9Hr+rJOKyhPet2/vOhVlR/lU/DElYtLkVVQVpKLs/H203FZyss/wCZLwS8hyss/wCZLwS8i09DpfyqfhQ9DpfyqfhRNpcTLFQ/4v1XwVfKuz/mS8EvIcrLP+ZLwS8iz9Co/wAqn4UU/COcKdONGjShx9d6McorUnqbIeJK9y9NUZyUVF+q+CquMbt7i9hOrJq3orOC0W9Ke9r62Fzyss/5kvBLyNzD8IpUaMKehCTS1ycU23zs84lVtbak6lWFNLmSjHOT3IhKSV7lpzo1JKMYt2yWf4OR4UXdrcuNWhJup7Mk4tZrmfadphNbjLWjN7ZU459uWs5+wwiV7UVxcU406K+zpRWWkuZvq+fYdVGKSSSSS2Jcwgndtk9qqQwRpx1Xn5Hohs1MRxKja0+MrTUVzLnk9yXOc2vTMWf4rayfjqLy+HaddOi5LE8o8fjiee2beI8JnOp6Ph8OPrbHL8EOtvn+XyMmE8GVCfpF3P0i5evOWuMX/SvrqyLXDcMo2tPi6MFFc755Pe3zmzUqRhFyk1GKWbbeSSLyrJLBSVlx3v8AeAtxOXwOhGpiGKQqRUoSlFOMlmn7RFfBbmwm62HSc6Tec7eTz7XH6z7TBwZxOjLEr7KpH+NJcXnq08nLZ3nZG1epOlUs1k0sno8kQldFTgvCCjeLKPqVV7VKWqSy25b0WxTY1wcpXT4yLdK4jrjVhqefNnv+ZXWmP17OaoYnHLPVCvFZxl+r67VzmTpRqZ0vTf5ceovbU6sHmnNSSlFpxazTTzTR6OUsAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAQVt7fzpSaaWWayeXM+d6zKrVjTV5FoxcnZFmDQVS4/Kvh5jTuPyL4eZXb/6v0JweKN8Ghxlx+RfDzGncflXw8xt/9X6DB4o3waGncfkXw8xxlx+RfDzG3/1foMHijfBocZcfkXw8xp3H5F8PMbf/AFfoMHijfINHTuPyL4eZ5q168IuUoxSW16vMjbpf1foMHiicXwmld09GospL2ZrbF+XUVWH4rVtaitb7/wButzSXW/3795b2F1Oq9aWjlnsyab2LuMmIWFO5punVjmuZ86e9MtCSqRxwNozw/wAdTNdOQxGyjcUJ0pbJLU9z5mV3Bq9lKErat9tQei8+ePM+v/TeaNteVcNqKhctztpPKnV/L1Py7tWzNjtN0qlLEKHraOSqZa9OD5/rq3Fr7zWNPLZN3Tzi/H86M6MGO3rRqQjODzjJJp9TMhocLVjxWqxhCU5PKMU23uSKDAaUrmvUvqiyz9WinzRWrP66xj9WVzWp2NJtaXrVmuaC5vrqNjE8Vp2cIUKMdOq0o06cdeW7P61mbeeeiOyEJRhhj3pey/PQ2cXxenaQzl605exBbZPyKzDcIqXFRXV9rl/+OlzRXNmv27zPhGCyU/Sbt8ZcS1pbVDqXX8i8JtfNlHONJYaeu9/ZeHUHP4vwmUJ+j2cPSLl80dcYfqf115GLh7eVKNkuLm4udRRbWpuOjJtfBFpgeG0LehHiIZKcU3J65SzWetnZCMIQVSed9Fy4nL4FbhvBpyqek4hPj6+1R/BDqS5/l8zoyJSSTbaSWtt7EjmrvhBVuZu3wyOnL8Vd+xDs3v61kfyV3d6LySGSLTGMco2cf4j0pv2acdcpPs/cp6eF3WItVL5ulQ2xt4vJvc5v6fYWOEcHadvLjqjde5euVWetp/07i5J2kaWVPXj8cOeotfU4bDMCt7i8xCjKGjGEo8W46nD2thvxv7rDGoXadxa7I14rOUF/Wvrtew9cG/8AEsS/XH5yOnlFNNNJp6mnsaNq9ZqeGeasui04EJGK0u6demqlKanB7Gibq1p1oOnVgpwe1NHPXXB+razdfDJ6D2zoS1wn2bvrWjdwfhFTuJOlUi6NxHVKlPU2/wCnf8zCVLLHSd17rn8k34lXUw27wxupZN17bW5UJbY/p/t3MvMHxyheQzpSykvahLVKPu3dZYnHcOLSlbwhd0c6Vy6iipQeWlqbba9xpCS7Q1Cfeej+fkjQ7EkxW09KnCT1txT70ZTjLAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA1MQt9OGzNr4rnX1uNsgpOCnFxe8mLad0UGH30qDUKuuk3lGW7+xfp57CruqEYzcZrOlPb1dm7Lb72YaNadnNU6rcqL9mW763dxy0ZygsM92XL8M6JxU8469fyXQEZJpNPNMk7TmIBo4xeyt6KqRjGXrwi821qnOMc14jfAIAPFarGEXKTySIbtmxqTVqKEXKTySKG4uZ3E00sqefqr8z2L4/uZvWum6lTONCOxfm+t/uRtYfT0pOo1klqguZc2rs2d5w1W6zUFo+nH4OmKVPPebdrQ4uCjte1ve+dmUEnckoqyOZu5hubaFWDp1IqUJbUzmakKmGtwqJ1rCpmnzunnzfW3qe3rDxUpqUXGSUotZNPWmiHG5tTq4MnmuH7vOc4OXao1ZWjmpU5evQn+aL15dv7pl3id9G2oTqy/CtS3vmRyWOYFUtJKvbOTpQlpZbXTe/rj9Mx399WxSrSpUItKKTlnsUueTe5bF7zPE4q287nQhWkqqf0/28vkz2d5OimoR42/uXnL/wDXF7E92/Lm59hfYLgioN1asuMuJ65TevLqj5mXB8Hp2sNXrVJe3Ue2T/ZFkXjHic1evibUNHr4/jgiCQC5yHIf7Rs3bUYrW3V2f8r8zYwnhDQo4XbVKs/W4vRUFrlJwejqXu2kcMNdbD4b7hZ9mcfM5jg3TlapXsrbj6GbWa1ypZP2kvf/AHR6dOkqlGN91/O709ijdmdHCxu8Tandt29rtjRi/Wmv639dnOdLaWlOhTVOlBQgtiR4w/EKVzTVSjNSj8U9zXMbJxVakn9LVktxZIkgx3NxClBzqSUILa28kcrWxi6xGTpYfF06OydxLV4d3z7BToyqZ6LjuDdjNwb/AMTxL9cfnI6g5KpwPnQjGrZXE43Mc83J6qme1dXvz/c2sL4UJ1PR76Ho9wtWv2JPqfN8us3rU1VeOk72S55K17cCFlqdGVuMYHRvI/xFo1F7NSOqUX285ZA5IzcHeLzLHLU8VucOap36dWhsjcRWbW5TX0+0qv8AaDfxqq1jSkpwalPOLzXMl+50uO47QoLiZR4+rNZKjFaTef5txwtTDp0a1dVacacpWs6kacXmoJvZ26mel2eGKSqtWfXxt+oo+B9Iwmela0Jb6UH/ANKNwreDss7C1/4MF3RSLI86orSa8S6AAKAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAw3NFVIOPPzPczVttGrTdGos8tWT3eaf7FgaF7Bwmqsd+tfW/Z3HNWWF7RefL8fJpB3+k1KdSdnNQnnKhJ+rL8vUXEZJpNPNPY0Y5RhWp5NZxkthVQnOymoyzlbyfqy54vcWT2f/Xp+C3+T/t1/JY4hYxuKfFzlJR0oy9VpPOLUltW9J+42Usltz6yITUknFpp601znivXjTi5SeSRs2krmVtxNatGnFyk8kiqpUp3ktOpnGivZj+b+3WKNGV3NVKqaor2Y/m6+z59m2zuKqpwb1bkjnbxrFLKK9zXuZLXoal29KUaNPVvy5kvJfsb9OCjFRWpJZI1MPo5J1Je1PftS+tZuFqMXnOWr9luRSb/AKokEBs3KEg1a1/Sh7U1nuWtmrLFXLPiqU5Le9SMpVoLK5jKvTjlf7lm0YLWypUU1SpxgpPN6KyzZo0cWnoqU6MnF/ijsNqjidGf4snulqIjXpy3iHaacsk9fI2ySFJPY8wbGxIAAOV4Vv8A33DlunOXdov9jPwEj/5dDrlP/uNThTL/AMxs+qFR/wDS/I2+CFaFLCqU6klGK0223kl68j0Z/wDzRXLrIpvJxDg241HcWE/R6+1xX2c+po05cNHRjKncUJK7hktCPsyb50+b4ivjVziE3Sw6LhSWqVxLV4d3z7DdteB9pCjKFSLqzn7VSXtZ747vrPMfTGKXac3uW9c/jXkORqW+A3F9NV8SllFa4W8Xkl2/WfXzHUUaMacVCEVGMVkklkkjl87zC9uld2a//pTX7r4dh0OHYlRuqfGUZqS596e5rmMe0Y5K+sd1tF8PmSjTwnG1dTahGOg4uUXppyyU9FZx69uf7mziuE0bunoVoZ7pLVKPWmYsPwSnb1FOEpPRjKEIvLKEZT05LUtevfzIxYxwhpWzVOKda4l7NKGt59e4wpKbksGpLKV1rzCH/EzubLPJS/HTWepfWrsM8sauMRk6WHri6S1Trz2rqivr3GS3wKteTVbEpZpa4W8X6sf1b39Z8wxHgw4VPSMOnxFZbYbIT6subs2dm07sdFv6rY+P9b+PzoVzLLB8Bo2ibinOq/aqz1zee3XzI57hdH/fX/VY1F3aTLPCeFClP0e8h6PcLVlLVGT6nzfWTZo8LY53tD+q2rr/AOOXmVpKpGv/ACa2fQO1si74Kyzw+2/Rl3Notii4Fyzwyh/z/wD2SLw5K6tVkvF9Sy0JBBJkSAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAADzOCknF609p6IANCzm6c3Slv1P637e3M3atKM4uMknF7UzWxCjnHTj7Ud27+20zW1bTgnz8/ac1L6G6T8uX4NJZ/Uiq0p2MsnnK3k9T54P67+3b7oUJXUlVrLKkvYhvW99Xz7DJwk+5Vf8Al/74m9a/ZU/0L5EqmsWHcs7F3P6cW/iZciun/HraP4Ibevq97+C6zPiFfRjkvalq1bfrmMlnQ4uCX4nrl2kT/kng3LN/ZfcovpVz3VrwgvXko9rNKpjFPPKClN9SMGI0FUuacG2k4vZ7z1Cxr0fsZxktzWRWVSq5NRWS82edOrVcmorJZcWeuOuqns0401vlt+vcSsLnP7atKXUtSIWJzhqrUZR61rRtUL+lU9maz3PUxHZydpNt+OXtkI7KbtKTb4PL2yFHDqMPZgs971v4mat7Ev0v5Hs8VvYl+l/I6MKjGyR1YYxjaKsauD/d4e/5sz1rSnP2oJ9fP3mDB/u8Pf8ANm6UpJOnFPgjOjFSpRTW5Fc8JUddKpOD7c0R/vdP8tVdzNytd04e1NLq5zSljGk8qNOVR/AykqUNHZ+HwYzjRg8nZ+Hx+D1HF0nlVhOD60blG6p1PYmn1Z6yvdG6re2404vm2v695g9CVG4opSbbbbbIVSrFq6y8cmVVWtFptXjlrk8/3gih4bXipX9GTTeVvPZvmpxRPB7g3O6oUZ3dRu3is6VGLyTT521s5+vrH+0q11W9dczdOXv1r5SIwfFbuwt6Lr0+Ns5xTjOO2mnzP+/fzH0cJuXZ0qWUtPHfp4nXvzO3oUYU4KFOKjGKySSySMhrWN/SuKaqUZqcXu5uprmZsHkyTTs9TQHP4jwbyqekWM/R6/Ol7E+qS+uw6EgtCpKDvEixyFPE8QvZStYQhbzp6q9VPPLPZorry/ui8wfAqFom6acqj9qpLXKXv5iv4P8A+I4n+un8pHRnR2idngjkrJ5eKuQgCSDkLGji2D0LyGjWhn+WS1Sj2M4TGbetYXFD0io61GMZqk/xaLjk08+1c52eNcIaFmtGT06r9mlHXJ7s9xwvC2d7UjSr3cVTjJyVOmtsVq29b1bd3Mep2LGu/wB3PXluKSOw4Cyzw2n1Smv+pv8Ac6AquC9pxFhbwayehpS7Zes/mWxwVpqdSUlvbLLQAAyJAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAIK+P8Ctl+CXw/0z7n1FiYLuhxkGufau0xrQclijqtPjzLxdsnoaXCT7nU7Y/96N22klRg3sUE/gVGKVtKxqRftRcVr3aa/wBPcbM6rlTpUobXGOfd+23uMtus5relbnnkaYHhS8X9jJaxdWq6ktkXkl1/2+bZYHmlTUIqK2JHo2pU8EbPXfzMpO7K26++Uex/uWRW3n3uh2MsiKXelz+yOWj3p8/sg0albDaM9sEnvjqNwGkoxlk0bShGStJXKr/w6rT+xrPskeZ3teEWqtLNNZaUewtjHc/Zz/S/kYujhX0Nroc8qCingbXT3KeyvaipRp0qTk1z8202PRLmr9pUUFuiZsF+7x7X8zeKUqWKCcm3kUoUcVOLk28llovY0aOEUY7U5vfLX8Nhuxikskkl1HoHTGEY91HVCnGGUVYFZd/fKHY/kyzKy4++0f0v5Mzr6Lmupl2jurmuph4WYbK6salOmtKospQW9p7O7M1OC+LUalCnaT9StTgoSp1Fk3ksnlnt7DoyrxnAaF4k5pxqL2akdUlu7UdlOULYZ6ceBuysvuDM6NR3GGz4qpz0vwS6urs2dhmwrhRGc+Iu4ej3C1ZS1Rk+pv67TVhil3hrUL5Ovb6lG4ita/V9Z9pbXljaYlQTejUi16tSPtR7HzdjOmbyW1zW6S/fZ5kci1JOP469wrVUzurNfiXt011/WXYdLh2JUbqnp0JqS596e5rmOapRcFiWceP7oSmU3B//ABHE/wBdP5SOjOc4P/4lif66fykbWNcJKNr/AA451a79mlDW8+bPd8zSrCU6mGKu7LoiFoW1evCnBzqSUYra28kjl6+O3N9N0cNg4088pXElkl+n6z7BQwK5vpqtiUnGG2NvB5Jfq+s+wtcRxa1w+lGDyTyyhSgtb7FzdpaMYwdorFL2Xz0Gpjwjg7QtM6s3xlbbKtU29bWezt2lDwirQxWvQt7WMqsadTOrUWqCi2k9fu8szdhh93iT07xu3ttTjQi8pS/V/fuR0tnZ06EFTowUILmXze9iclF4pPFP2X74ZAzRWSyWxEgHGWAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAABBIAKbGrLSTyeSnt7c8/jl8DZwq3yipvXmso/p3+/wAjenBSWTSa3Mk5o9nSq7T28eJo6jccJIBB0mZW333q395ZFdiH3i37X+xYmNPvT5/ZHPS78+f2RIANjoBiufs5/pfyMpiufs5/pfyIloysu6zVwX7vHtfzIli0Fcq3cKmcpOKlo+rmoKfblk9uzPUTgv3ePa/mYZ4TOV1Gu6uqM3Jer62i4aPFZ/kz9btM6H+OPJGfZ/8AFHki1ABqbArK332n+h/uWRXVPv0P0P8AcxraLmjnr6R5rqWJJBJsdB5nBSTUkmnqaetNHNXXByrb1HXwyfFyftUZexPqW762HTkGlOrKnp6bmQ1co8K4SU68uIuIOhcLU6c9Sl+lvb2GviPBdxqO4w+p6PX2uK9ifVlzfLqLXFsGoXkNGtDNr2ZrVKPY/wBijV1eYZ6tdO6tOaqvbpr+r+/fzHTTabvRdnwej5ceTIfiUuFvEK93d06ehRqTlHj5/kyzWUdu3Xs70dbhWB21hB1G055NzrVHr6+xHL4Xwho0bzEKsVKrx0ocVGK1zfrd21FtSwe5v5KpiEnTo7Y20Hl2aT+n2GtWUpr6/oj7u3u+iIR6uMfr3c5UcMhmlqlcS1Qj2fXuN7B+DdK3lxtRutcN5yqz1vP+lc3zLa3t4UoKFOKhBbElkjIcsq2WGmrL3fN/bQtbiCQDnJAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAABBIAK3Evtrd/wBT/YsStxX7S3/4nkbta5hTWc5JGEGlOd/Doc0Gozm3xXRGUFbLFXN5UKcpvfsQ9FuKv2lTQX5YE7ZPuK/T1LbdPuK/T1NuveU6ftSS6uc0quIzqRkqVKTjk85PUsjZoYZShr0dJ75azPcfZz/S/kRJVJLN25FZRqyTu7cvl/BUYdd1KdJfwnKnr9aO3uLGhiNKpslk9z1Mx4L93j2v5mevZU6ntQWe/YzOjGoqcXF3y3mdCNRU4uLvksn8ozgrXYVaf2NV5fllrRP/AIhUp/b0ml+aOtGu1t31boa7fD3017osStl9+X/DNuhe06nszWe56mav/rv/AGytSSkotPeiKslJRcXfNFiSQSdB0gAAAhrPaSACvs8EtaFSVWjRhCctrX7bvcb5IJbb1AABAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAANLErOVVR0JZSi81mVlOmqMs7ijKWv2880X4ZzzoKUsa19TmqdmjKWNa+q9DBbXNOa/hyXZs+BsGlXwulPWloy3x1GDQuaPstVY7ntJxzj3l6fBO0qQ78b+K+P/AEtDFcfZy/S/kalHFoN6NROnLdI2q006cmmmtF7OwuqkZp4WXVSE4vCzWwX7vHtfzN8r8G1W8e1/M9XGKUoas9KW6OspTnGFKLk9yM6U4woxcnbJG6Y61aEFnOSS6yv4y6rezFUo75bTJRwiCelUbqS69ncNpKXcXm8vyTtZz7kfN5fk0rmpSrNqjRcpZ+0vV7zawywqQnp1Ja9HJLPN95ZQgorJJJbkSRHs6xY5a+hWPZlixy19F+8wSAdB1AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAgkAGKtQhNZTin2mhUwlxz4mo4Z7U9aZaEGc6UJ6oynRhPvIqKGETcVGpUaivwxLC3sqdL2IJPftfebAIhRhDREU6FOnoiCQDU2AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAIB8Y5T3/SqveRyov8ApVXvAPtBJ8X5TX/SqveS+E1/0ur3k2JsfZwfF+U9/wBKq945T3/SqveLEWPtAPi/Ke/6VV7xynv+lVe8YQfaCD40uE1/0qr4iXwlvl/6ur3lsDJsfZAfGuU190qr3jlNf9Kq95GFix9mB8Z5TX/SqveOU1/0qr3k4GLH2Yg+Ncpb7pVXvJ5S33SqveMDFj7KD4zylvulVe8nlLfdKq94wMWPsoPjXKW+6VV7xylvulVe8nZsWPsoPjXKW+6VV7xylvulVe8bNix9kB8c5S33SqviHKW+6VV7ydkxY+xg+Ocpb7pVXxEcpb7pVXxEbJix9kB8cXCS+6VV8RL4S33SqviJ2TFj7ED45ylvulVe8cpb7pVXvGyYsfYwfHOUt90qr3jlLfdKq942TFj7GD45ylvulVe8cpb7pVXxEbNix9jB8c5S33SqveOUt90qr3jZsWPsYPjnKW+6VV7yOUt90qr3jZsWPsgPjnKW+6VV7yOUt90qr3jZsWPshJ8a5S33SqveOUt90qr3kYGLH2QHxzlLfdKq+IcpL7pVXvGBk4WfYwfG+U190qr4hymvulVe8YGMJ9kB8b5TX3SqveOU190qr4iMLGE+yA+N8pb7pVXvHKa+6VV8QwjCfZSD45ymvulVfERymvulVfEMIwn2QHxzlLfdKq+Icpb7pVXxDCycDPshB8c5S33SqviJ5S33SqviGFjAz7ED47ykvulVfESuEl90qr4hhZOzZ9hB8f5SX3SqviHKO+6VV8QwsbNlCEQCDM9uRDPOZOZNybnqOXOGeMwLi56B5zGYxEGTLUDxmMycSLXMieog8ZjSZOMXMmQMekxpMY0LmQlGLSY0mTjQuZCTFpMaTGNC5lBi0mNJjaIXMoMWkxpMnaIXM6RDMcKsk80yHUbebZO0jYm6MoMWmxpsbREXMoMWmyNJjaoXMwMOmxpMbRC5nisyaiSeowKo1sYdRvnG1RbErGUGHSZOm95G0RXEZAYtJjSY2iFzNmQYtJjSY2iGIzJkGLSY0mNoicRlGZi0mNJkY0MRnhlnr2CbWerYYNJjSYxonHlYyEmLSY0mRiRGJGXIg8abI0mRiQxIzZnkx6TGkxiGIygxaTGkxiGJGbIlGDTZOm94xFsaNqoo6svf2njIwcY944x7yXNNk7RGxkeoo1uNlvHGy3jEiyqxNrRJ0TV46W8cfLeMaJ2sTGADM5gAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAD/9k=\n",
"text/html": [
"\n",
" \n",
" "
],
"text/plain": [
""
]
},
"execution_count": 45,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"from IPython.display import YouTubeVideo\n",
"YouTubeVideo(\"OBuH2eAJT6Q\")"
]
},
{
"cell_type": "markdown",
"id": "1379d21a-05b9-4d6f-b8e3-f4518b3fcb98",
"metadata": {},
"source": [
"We have the shapes themselves, then we have scale factors taking us from one to another. However sometimes just a scale factor is not enough as we have strictly shape preserving, but also shape-shifting operations. The Jitterbug is a good example of shape shifting, whereas scaling an S or E module up and down by phi is strictly shape preserving.\n",
"\n",
"When we shape shift a T module of volume 1/24 into an S module, what does that look like in terms of a scale factor? What is the scale factor between any two shapes? Is that generally a meaningful question?\n",
"\n",
"We've already gotten a lot of mileage out of one such scale factor above, the S factor (S:E volume ratio). The S-factor expresses the edge length preserving yet shape shifting Jitterbug transformation from the Icosahedron to the Cuboctahedron. It also plays a role in a different non-edge-length-preserving cuboctahedron-to-icosahedron transformation, taken up as a topic in the Koski Identities section below.\n",
"\n",
"However another scale factor gets a lot of attention in Synergetics 2 especially and we might call it the T factor or T2E. The T & E modules differ by only a tiny relative volume, by a factor of about 0.9994 (E2T) or 1.0005 (T2E)."
]
},
{
"cell_type": "code",
"execution_count": 46,
"id": "537f748a-a5fb-4fa8-a2af-e68beab0bdf6",
"metadata": {},
"outputs": [],
"source": [
"A2B = B2A = T2A = A2B = T2B = B2T = 1"
]
},
{
"cell_type": "markdown",
"id": "43ca07ab-5598-474c-a7a8-12d844ed8b91",
"metadata": {},
"source": [
"
"
]
},
{
"cell_type": "code",
"execution_count": 47,
"id": "58bd0cb6-c0de-4a4d-8519-569f9578023e",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle - \\frac{\\sqrt[6]{2} \\cdot \\sqrt[3]{3}}{2} + \\frac{\\sqrt[6]{2} \\cdot \\sqrt[3]{3} \\sqrt{5}}{2}$"
],
"text/plain": [
"-2**(1/6)*3**(1/3)/2 + 2**(1/6)*3**(1/3)*sqrt(5)/2"
]
},
"execution_count": 47,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"T2E = sy.Rational(3,2) ** sy.Rational(1,3) * sy.sqrt(2) / φ\n",
"T2E.simplify()"
]
},
{
"cell_type": "code",
"execution_count": 48,
"id": "85760f0f-4b2d-43f5-a82b-6172f21623df",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 1.00051693482120114584792323435622229200028627527748$"
],
"text/plain": [
"1.00051693482120114584792323435622229200028627527748"
]
},
"execution_count": 48,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"T2E.evalf(51)"
]
},
{
"cell_type": "code",
"execution_count": 49,
"id": "b314fc87-7996-4693-b76e-de5d83adce5b",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle \\frac{2^{\\frac{5}{6}} \\cdot 3^{\\frac{2}{3}} \\cdot \\left(1 + \\sqrt{5}\\right)}{12}$"
],
"text/plain": [
"2**(5/6)*3**(2/3)*(1 + sqrt(5))/12"
]
},
"execution_count": 49,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"E2T = (1/T2E).simplify()\n",
"E2T"
]
},
{
"cell_type": "code",
"execution_count": 50,
"id": "15ba0b10-56ea-4153-90a8-2605529c5ce7",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 0.9994833322623434400464260276814215953818$"
],
"text/plain": [
"0.9994833322623434400464260276814215953818"
]
},
"execution_count": 50,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"(2**sy.Rational(5,6) * 3**sy.Rational(2,3) * φ / 6).simplify().evalf(40)"
]
},
{
"cell_type": "code",
"execution_count": 51,
"id": "789f58d0-69c6-485f-baec-24dc8c88cc06",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 0.9994833322623434400464260276814215953818$"
],
"text/plain": [
"0.9994833322623434400464260276814215953818"
]
},
"execution_count": 51,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"E2T.evalf(40)"
]
},
{
"cell_type": "markdown",
"id": "35f82adc-294a-4b6e-956d-c44f46806bb8",
"metadata": {},
"source": [
"David Koski:\n",
"\n",
"T->S is 12(phi**-5) = 1.082039324993691 factor which is 24S\n",
"Cleans up nicely"
]
},
{
"cell_type": "code",
"execution_count": 52,
"id": "81bd477f-231c-4295-9888-e21d69b0dde4",
"metadata": {},
"outputs": [],
"source": [
"T = sy.Rational(1, 24)"
]
},
{
"cell_type": "code",
"execution_count": 53,
"id": "44c5b802-6692-4693-bb7f-c80cf74c70aa",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle \\frac{1}{2 \\left(\\frac{1}{2} + \\frac{\\sqrt{5}}{2}\\right)^{5}}$"
],
"text/plain": [
"1/(2*(1/2 + sqrt(5)/2)**5)"
]
},
"execution_count": 53,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"S.volume"
]
},
{
"cell_type": "code",
"execution_count": 54,
"id": "e9955960-6a1e-4a93-9578-5978be37d658",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 1.08203932499369$"
],
"text/plain": [
"1.08203932499369"
]
},
"execution_count": 54,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"T2S = 12 * φ ** -5 # no, not the S-factor\n",
"T2S.evalf()"
]
},
{
"cell_type": "code",
"execution_count": 55,
"id": "3b1ddfe0-8c36-4a4a-a693-3f66e5b672e9",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 0.04508497187473712051146708591409529430077$"
],
"text/plain": [
"0.04508497187473712051146708591409529430077"
]
},
"execution_count": 55,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"(T * T2S).evalf(40)"
]
},
{
"cell_type": "code",
"execution_count": 56,
"id": "059a78b2-5b73-4fef-9448-0d9f025a9193",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 0.04508497187473712051146708591409529430077$"
],
"text/plain": [
"0.04508497187473712051146708591409529430077"
]
},
"execution_count": 56,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"S.volume.evalf(40)"
]
},
{
"cell_type": "code",
"execution_count": 57,
"id": "c8cf6d53-d219-4ff1-87c1-42da9c855e4e",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 1.082039324993690892275210061938287063219$"
],
"text/plain": [
"1.082039324993690892275210061938287063219"
]
},
"execution_count": 57,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"24*S.volume.evalf(40)"
]
},
{
"cell_type": "code",
"execution_count": 58,
"id": "32e61518-8df7-4dcb-9f6a-67203da06055",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 1.082039324993690892275210061938287063219$"
],
"text/plain": [
"1.082039324993690892275210061938287063219"
]
},
"execution_count": 58,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"T2S.evalf(40)"
]
},
{
"cell_type": "code",
"execution_count": 59,
"id": "4a0151b6-218a-4b92-91b0-11b38be20ec2",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle \\frac{2 \\sqrt{2}}{\\left(\\frac{1}{2} + \\frac{\\sqrt{5}}{2}\\right)^{2}}$"
],
"text/plain": [
"2*sqrt(2)/(1/2 + sqrt(5)/2)**2"
]
},
"execution_count": 59,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"E2S = S.volume/E.volume\n",
"E2S"
]
},
{
"cell_type": "code",
"execution_count": 60,
"id": "9716bc11-4acf-4f1f-9dd6-07b4b7a64b61",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 1.0803630269509058144$"
],
"text/plain": [
"1.0803630269509058144"
]
},
"execution_count": 60,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"E2S.evalf(20)"
]
},
{
"cell_type": "code",
"execution_count": 61,
"id": "11037e7e-19d2-4e08-99e9-1f57995f1ad1",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 1.0803630269509058144$"
],
"text/plain": [
"1.0803630269509058144"
]
},
"execution_count": 61,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"sfactor.evalf(20)"
]
},
{
"cell_type": "code",
"execution_count": 62,
"id": "ade9a892-5bb9-4272-952a-a55e3d08c722",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle \\frac{\\sqrt{2} \\left(\\frac{1}{2} + \\frac{\\sqrt{5}}{2}\\right)^{2}}{4}$"
],
"text/plain": [
"sqrt(2)*(1/2 + sqrt(5)/2)**2/4"
]
},
"execution_count": 62,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"S2E = 1/E2S\n",
"S2E"
]
},
{
"cell_type": "code",
"execution_count": 63,
"id": "bdbf5d76-e37f-416b-8063-99486396f7e5",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 0.9256147934109580598004949646327265961786$"
],
"text/plain": [
"0.9256147934109580598004949646327265961786"
]
},
"execution_count": 63,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"S2E.evalf(40)"
]
},
{
"cell_type": "markdown",
"id": "3a6e2304-b487-4c50-acb1-24b1a59f7e07",
"metadata": {},
"source": [
"Kirby Urner:\n",
"\n",
"CO(R) -> E2S -> E2S = IcosaWithin -> E2S = CO of edges E2S. CO -> S2E goes right back to CO(R) if S2E is applied as a resizer, not as a reshaper."
]
},
{
"cell_type": "code",
"execution_count": 64,
"id": "c5dc9668-bf9d-4082-bec2-c4958f4c837c",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle \\frac{20}{\\left(\\frac{1}{2} + \\frac{\\sqrt{5}}{2}\\right)^{4}}$"
],
"text/plain": [
"20/(1/2 + sqrt(5)/2)**4"
]
},
"execution_count": 64,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"IW = sy.Rational(5, 2) * E2S * E2S\n",
"IW"
]
},
{
"cell_type": "code",
"execution_count": 65,
"id": "b14cd657-7a21-4e2c-9102-7b142b326f6b",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 3.15245682737352$"
],
"text/plain": [
"3.15245682737352"
]
},
"execution_count": 65,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"(IW * E2S).evalf() # CO edges E2S, same as IW"
]
},
{
"cell_type": "code",
"execution_count": 66,
"id": "0bee39f8-431d-4c05-9b2a-ebda9f984ac1",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 2.5$"
],
"text/plain": [
"2.50000000000000"
]
},
"execution_count": 66,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"((IW * E2S) * S2E**3).evalf() # back to CO"
]
},
{
"cell_type": "code",
"execution_count": 67,
"id": "8f47e202-d587-4acc-8050-610d39798c0c",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle \\frac{20}{\\left(\\frac{1}{2} + \\frac{\\sqrt{5}}{2}\\right)^{4}}$"
],
"text/plain": [
"20/(1/2 + sqrt(5)/2)**4"
]
},
"execution_count": 67,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"IW"
]
},
{
"cell_type": "markdown",
"id": "7a5974af-7d8a-4a74-b1ba-9ad761f80d2b",
"metadata": {},
"source": [
"David Koski:\n",
"\n",
"IcosaWithin * (phi**4)(1/sFactor) -> Icosa(D) of 18.51..."
]
},
{
"cell_type": "code",
"execution_count": 68,
"id": "0441d379-09c2-4740-b413-c04354c9930d",
"metadata": {},
"outputs": [],
"source": [
"IcosaWithin2IcosaD = S2E * φ**4"
]
},
{
"cell_type": "code",
"execution_count": 69,
"id": "babcf51e-b76f-475f-a25d-83e10592d0e2",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 18.51229586821916119600989929265453192357$"
],
"text/plain": [
"18.51229586821916119600989929265453192357"
]
},
"execution_count": 69,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"(IW * IcosaWithin2IcosaD).evalf(40)"
]
},
{
"cell_type": "code",
"execution_count": 70,
"id": "8d7dd061-8024-4325-b601-ac695fea5723",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 18.51229586821916119600989929265453192357$"
],
"text/plain": [
"18.51229586821916119600989929265453192357"
]
},
"execution_count": 70,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"(20 * S2E).evalf(40)"
]
},
{
"cell_type": "markdown",
"id": "8ea7aefb-f961-455a-897a-a7edf31f9b58",
"metadata": {},
"source": [
"T2E is applied edge-wise as a shape preserving increment. Volume changes as a 3rd power."
]
},
{
"cell_type": "code",
"execution_count": 71,
"id": "710413b5-612a-49e7-aadc-e596ed99fdcf",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle \\frac{\\sqrt{2}}{8 \\left(\\frac{1}{2} + \\frac{\\sqrt{5}}{2}\\right)^{3}}$"
],
"text/plain": [
"sqrt(2)/(8*(1/2 + sqrt(5)/2)**3)"
]
},
"execution_count": 71,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"(5 * T2E ** 3)/120"
]
},
{
"cell_type": "code",
"execution_count": 72,
"id": "84f7afc8-dba6-4671-842d-8acf67ed75c2",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 0.04173131692777365429943951200166529707253$"
],
"text/plain": [
"0.04173131692777365429943951200166529707253"
]
},
"execution_count": 72,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"((5 * T2E ** 3)/120).evalf(40)"
]
},
{
"cell_type": "code",
"execution_count": 73,
"id": "21769c13-a305-45c5-a108-c27d20014fd9",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 0.04173131692777365429943951200166529707253$"
],
"text/plain": [
"0.04173131692777365429943951200166529707253"
]
},
"execution_count": 73,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"E.volume.evalf(40)"
]
},
{
"cell_type": "code",
"execution_count": 74,
"id": "df7a56db-2789-403f-a0ce-d3ccd9a603c1",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 5.00775803133284$"
],
"text/plain": [
"5.00775803133284"
]
},
"execution_count": 74,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"(120 * E.volume).evalf()"
]
},
{
"cell_type": "code",
"execution_count": 75,
"id": "edfc9c74-d1f0-4d68-a368-38042ee2429a",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 21.21320343559642573202533086314547117855$"
],
"text/plain": [
"21.21320343559642573202533086314547117855"
]
},
"execution_count": 75,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"((120 * E.volume) * φ**3).evalf(40) # SuperRT"
]
},
{
"cell_type": "code",
"execution_count": 76,
"id": "c25ef230-9154-4a5e-9984-2a133ae65e66",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 1.060660171779821286601266543157273558927$"
],
"text/plain": [
"1.060660171779821286601266543157273558927"
]
},
"execution_count": 76,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"S3 = sy.sqrt(sy.Rational(9,8)) # S3 as in XYZ <-> IVM conversion constant\n",
"S3.evalf(40)"
]
},
{
"cell_type": "code",
"execution_count": 77,
"id": "43b6b16b-428d-4a97-951c-72485d750973",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 21.21320343559642573202533086314547117855$"
],
"text/plain": [
"21.21320343559642573202533086314547117855"
]
},
"execution_count": 77,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"(20 * S3).evalf(40) # SuperRT"
]
},
{
"cell_type": "markdown",
"id": "df710bf6-72e4-4ff8-885d-a2c2b06d6705",
"metadata": {},
"source": [
"## The Koski Identities\n",
"\n",
"Rendered in Blender:
\n",
"
\n",
"\n",
"The original two volumes, *Synergetics* and *Synergetics 2*, do not include phi-scaling the S and E modules, several notches \"up\" and \"down\" and then expressing concentric hierarchy volumes using whole number combinations of these.\n",
"\n",
"We go over this information below."
]
},
{
"cell_type": "code",
"execution_count": 78,
"id": "41bcc258-9c22-46ab-87bd-0edd621c06ee",
"metadata": {},
"outputs": [],
"source": [
"φ = (rt2(5) + 1)/2\n",
"S = (φ**-5) / 2\n",
"S3 = S * φ ** 3\n",
"S6 = S3 * φ ** 3 \n",
"S9 = S6 * φ ** 3\n",
"s3 = S * φ ** -3\n",
"s6 = s3 * φ ** -3\n",
"s9 = s6 * φ ** -3"
]
},
{
"cell_type": "code",
"execution_count": 79,
"id": "842e6df6-da04-4af0-b46b-ce967aac60b6",
"metadata": {},
"outputs": [],
"source": [
"E = (rt2(2) / 8) * (φ**-3)\n",
"E3 = E * φ ** 3\n",
"E6 = E3 * φ ** 3 \n",
"E9 = E6 * φ ** 3\n",
"e3 = E * φ ** -3\n",
"e6 = e3 * φ ** -3\n",
"e9 = e6 * φ ** -3"
]
},
{
"cell_type": "markdown",
"id": "a7707215-c027-4048-80c3-6c822e20b2a3",
"metadata": {},
"source": [
"The \"SkewIcosa\" in the table below is a regular icosahedron. The term \"skew\" in this case reminds us if how it's developed by skewing a cuboctahedron of volume 2.5, such that its edges stretch while its faces remain flush with an octahedron of volume 4. \n",
"\n",
"\n",
"
\n",
"\n",
"The resulting icosahedron, faces flush with the octahedron, serves as a basis for developing the S module.\n",
"\n",
"
\n",
"
"
]
},
{
"cell_type": "code",
"execution_count": 80,
"id": "cbf3b153-916d-4806-a26e-fae070300e02",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 4.0$"
],
"text/plain": [
"4.000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000"
]
},
"execution_count": 80,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"(24 * S + IcosaWithin.volume).evalf(100)"
]
},
{
"cell_type": "code",
"execution_count": 81,
"id": "9c6544b4-1d0d-43ea-91ca-d70f163754f8",
"metadata": {},
"outputs": [],
"source": [
"Tetrahedron = S6 + S3 # (volume = 1)\n",
"SkewIcosa = 60*S + 20*s3 # (volume = ~2.92)\n",
"Cube = 3*S6 + 3*S3 # (volume = 3)\n",
"Octahedron = 4*S6 + 4*S3 # (volume = 4)\n",
"RhTriac_T = 5*S6 + 5*S3 # (volume = 5) \n",
"RhTriac_E =120*E\n",
"RhDodeca = 6*S6 + 6*S3 # (volume = 6)\n",
"PentDodeca =348*E + 84*e3 # (volume = ~15.35)\n",
"Icosahedron =100*E3 + 20*E # (volume = ~18.51)\n",
"VE =420*S +100*s3 # (volume = 20)\n",
"SuperRT =480*E +120*e3 # (volume = ~21.21)"
]
},
{
"cell_type": "code",
"execution_count": 82,
"id": "d33f5419-8267-463b-9f04-0ce2ac34ac4c",
"metadata": {},
"outputs": [],
"source": [
"p = 50 # precision"
]
},
{
"cell_type": "code",
"execution_count": 83,
"id": "ebb914e6-44c1-48da-bea1-dbb2acb2aa8c",
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"\n",
"Tetrahedron = 1.000000000000000000000000000000000000000000000 = 1\n",
"SkewIcosa = 2.917960675006309107724789938061712936781449212 = 70 - 30*sqrt(5)\n",
"Cube = 3.000000000000000000000000000000000000000000000 = 3\n",
"Octahedron = 4.000000000000000000000000000000000000000000000 = 4\n",
"RhTriac_T = 5.000000000000000000000000000000000000000000000 = 5\n",
"RhTriac_E = 5.007758031332838515932741440199835648703170829 = -30*sqrt(2) + 15*sqrt(10)\n",
"RhDodeca = 6.000000000000000000000000000000000000000000000 = 6\n",
"PentDodeca = 15.350018208050781864011005748221813389851871774 = 3*sqrt(10)/2 + 15*sqrt(2)/2\n",
"Icosahedron = 18.512295868219161196009899292654531923571426914 = 5*sqrt(10)/2 + 15*sqrt(2)/2\n",
"VE = 20.000000000000000000000000000000000000000000000 = 20\n",
"SuperRT = 21.213203435596425732025330863145471178545078131 = 15*sqrt(2)\n",
"\n"
]
}
],
"source": [
"print(f\"\"\"\n",
"Tetrahedron = {Tetrahedron.evalf(p):50.45f} = {Tetrahedron.simplify()}\n",
"SkewIcosa = {SkewIcosa.evalf(p):50.45f} = {SkewIcosa.simplify()}\n",
"Cube = {Cube.evalf(p):50.45f} = {Cube.simplify()}\n",
"Octahedron = {Octahedron.evalf(p):50.45f} = {Octahedron.simplify()}\n",
"RhTriac_T = {RhTriac_T.evalf(p):50.45f} = {RhTriac_T.simplify()}\n",
"RhTriac_E = {RhTriac_E.evalf(p):50.45f} = {RhTriac_E.simplify()}\n",
"RhDodeca = {RhDodeca.evalf(p):50.45f} = {RhDodeca.simplify()}\n",
"PentDodeca = {PentDodeca.evalf(p):50.45f} = {PentDodeca.simplify()}\n",
"Icosahedron = {Icosahedron.evalf(p):50.45f} = {Icosahedron.simplify()}\n",
"VE = {VE.evalf(p):50.45f} = {VE.simplify()}\n",
"SuperRT = {SuperRT.evalf(p):50.45f} = {SuperRT.simplify()}\n",
"\"\"\")"
]
},
{
"cell_type": "code",
"execution_count": 84,
"id": "25e75857-96be-4ace-9e71-da68ec8b69a4",
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"18.512295868219161196009899292654531923571426913640\n"
]
}
],
"source": [
"print((8 * IcosaWithin.volume * (1/sfactor)**3).evalf(50)) # shrink to R-edge Icosa, inflate by 8 for D-edge Icosa"
]
},
{
"cell_type": "code",
"execution_count": 85,
"id": "2b91daa9-eaf5-4d91-8702-70f55376a852",
"metadata": {},
"outputs": [],
"source": [
"apex_e = S3 ** sy.Rational(1,3)"
]
},
{
"cell_type": "code",
"execution_count": 86,
"id": "5e85f668-399c-4b8f-aefd-ccdd63293ba0",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 0.575879441260916$"
],
"text/plain": [
"0.575879441260916"
]
},
"execution_count": 86,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"(apex_e).evalf()"
]
},
{
"cell_type": "code",
"execution_count": 87,
"id": "6f37c518-6712-4eaa-986c-f6d9a76e6f35",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 0.190983005625053$"
],
"text/plain": [
"0.190983005625053"
]
},
"execution_count": 87,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"S3.evalf()"
]
},
{
"cell_type": "code",
"execution_count": 88,
"id": "45640ae7-1a85-49d4-9999-ca504733221c",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 0.809016994374947$"
],
"text/plain": [
"0.809016994374947"
]
},
"execution_count": 88,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"S6.evalf()"
]
},
{
"cell_type": "code",
"execution_count": 89,
"id": "a580ed68-1572-4fe2-b0aa-ba12ff749aff",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 1.16718427000252$"
],
"text/plain": [
"1.16718427000252"
]
},
"execution_count": 89,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"(IW/2.5).evalf()"
]
},
{
"cell_type": "code",
"execution_count": 90,
"id": "8376f316-a484-4f3f-808c-9503a8a06ad6",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 1.16718427000252$"
],
"text/plain": [
"1.16718427000252"
]
},
"execution_count": 90,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"(sfactor**2).evalf()"
]
},
{
"cell_type": "markdown",
"id": "b09e026c-4a23-4048-abb0-1cfcef362976",
"metadata": {},
"source": [
"## Tessellating Tetrahedrons"
]
},
{
"cell_type": "markdown",
"id": "f6a3433f-3f94-4a25-b60a-a736a8f82a4f",
"metadata": {},
"source": [
"We start with the Goldberg paper submitting in 1972, published in 1974, before the first volume of Synergetics came out in 1975. \n",
"\n",
"The paper summarizes space-filling tetrahedrons already known, while adding a generalization allowing for an accordian-like expansion / contraction of RITEs and fractional RITEs (1/2 and 1/4) when stacking them in arbitrarily long equilateral prisms. \n",
"\n",
"The Mite and Bite (as they're known in Synergetics) stand outside of the infinite Rite families in this categorization, but may be lumped by other criteria, not taken up in the Goldberg paper, such as their composability from A and B modules (see above).\n",
"\n",
"
\n",
"\n",
"\n",
"[SLIDES](https://docs.google.com/presentation/d/13QLfgKo6kyX0j0K0RJ7W0uisB0ZyeBiK-_qbzytipck/edit?usp=sharing)"
]
},
{
"cell_type": "code",
"execution_count": 91,
"id": "44adbd19-b1b5-4695-a756-3e4fb05a0378",
"metadata": {},
"outputs": [],
"source": [
"from sympy import sqrt as rt2\n",
"from tetravolume import Tetrahedron"
]
},
{
"cell_type": "markdown",
"id": "38c025d4-d0f4-4cc9-9d44-c8c6df3d9e24",
"metadata": {},
"source": [
"The last two rows in each column (not counting the summation row) need to be swapped to stay compatible with our way of passing lengths to the Tetrahedron API, which expects edges of the base opposite the apex A to be traversed in the order BC, CD, BD, after AB, AC, AD in that order.\n",
"\n",
"The angles subcolumn in [the Goldberg Paper](https://www.sciencedirect.com/science/article/pii/0097316574900582?ref=cra_js_challenge&fr=RR-1), associated with each module, gives [dihedral angles](https://en.wikipedia.org/wiki/Dihedral_angle), the computation of which is included in the Tetrahedron type (as of May 6, 2024). \n",
"\n",
"I'm getting a mismatch in column 4 (465 vs 420 for the 1/4 Rite in question). Have I made an error somewhere? We agree on 435 in column 2."
]
},
{
"cell_type": "code",
"execution_count": 92,
"id": "e938b42d-d25a-4067-93a6-41372c9681ed",
"metadata": {},
"outputs": [],
"source": [
"column1 = (rt2(3), rt2(3), 2, 2, rt2(3), rt2(3))\n",
"column2 = (rt2(3), rt2(2), 2, 1, rt2(2), rt2(3))\n",
"column3 = (rt2(3), 2*rt2(2), 2, rt2(3), 2, rt2(3))\n",
"column4 = (rt2(3), rt2(3), rt2(5)/2, 2, rt2(5)/2, rt2(5)/2)\n",
"column5 = (rt2(3), rt2(3), rt2(11)/2, 2, rt2(3)/2, rt2(11)/2)"
]
},
{
"cell_type": "code",
"execution_count": 93,
"id": "56a78ff4-a65e-4fe2-b41a-86385e6fab2e",
"metadata": {},
"outputs": [],
"source": [
"rite = Tetrahedron(*column1)\n",
"mite = Tetrahedron(*column2)\n",
"bite = Tetrahedron(*column3)\n",
"qrite = Tetrahedron(*column4)\n",
"hrite = Tetrahedron(*column5)"
]
},
{
"cell_type": "code",
"execution_count": 94,
"id": "41a15dfc-eb99-42f8-be7c-e37a669eea8a",
"metadata": {},
"outputs": [],
"source": [
"k = rt2(2)/32 # constant of proportionality"
]
},
{
"cell_type": "code",
"execution_count": 95,
"id": "728e23a6-52b9-4198-9808-ff61005cce54",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle \\frac{1}{4}$"
],
"text/plain": [
"1/4"
]
},
"execution_count": 95,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"rite.ivm_volume() * k"
]
},
{
"cell_type": "code",
"execution_count": 96,
"id": "e0ded54e-aaea-42a2-9885-d058bca9d1ce",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle \\frac{1}{8}$"
],
"text/plain": [
"1/8"
]
},
"execution_count": 96,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"mite.ivm_volume() * k"
]
},
{
"cell_type": "code",
"execution_count": 97,
"id": "02f09a05-8f34-4bfb-8cb4-a1097c26e411",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle \\frac{1}{4}$"
],
"text/plain": [
"1/4"
]
},
"execution_count": 97,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"bite.ivm_volume() * k"
]
},
{
"cell_type": "code",
"execution_count": 98,
"id": "1dc0a52d-9991-4bb9-a093-b8bdd695d43f",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle \\frac{1}{16}$"
],
"text/plain": [
"1/16"
]
},
"execution_count": 98,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"qrite.ivm_volume() * k"
]
},
{
"cell_type": "code",
"execution_count": 99,
"id": "e0407ebd-53ef-4057-8a28-214e60a9e9b4",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle \\frac{1}{8}$"
],
"text/plain": [
"1/8"
]
},
"execution_count": 99,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"hrite.ivm_volume() * k"
]
},
{
"cell_type": "code",
"execution_count": 100,
"id": "2c2a95f9-e1bf-4e19-a4a8-537d30583aef",
"metadata": {},
"outputs": [],
"source": [
"from sympy import Symbol, Rational\n",
"k = Symbol('k')"
]
},
{
"cell_type": "markdown",
"id": "f3fbb0d0-1aa5-49de-ae8f-17aad6ddc19d",
"metadata": {},
"source": [
"Instead of using a volume changing scalar, lets get that number k's 3rd root and use that as a linear scale factor. This way, we should get all the edge lengths we need for the modules under scrutiny, and consistently with our volume requirements."
]
},
{
"cell_type": "code",
"execution_count": 101,
"id": "fcf7a4f9-0d49-4350-b603-a0c0755c6192",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle \\sqrt[3]{k}$"
],
"text/plain": [
"k**(1/3)"
]
},
"execution_count": 101,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"s = k**Rational(1,3) # 3rd root\n",
"s"
]
},
{
"cell_type": "markdown",
"id": "abef64af-def4-4227-901b-7394392b3b0d",
"metadata": {},
"source": [
"Every edge is to be individually rescaled by this 3rd root of k."
]
},
{
"cell_type": "code",
"execution_count": 102,
"id": "6f09a294-aafd-4d4d-aeb7-c416d00b1068",
"metadata": {},
"outputs": [],
"source": [
"column1 = [e*s for e in column1]\n",
"column2 = [e*s for e in column2]\n",
"column3 = [e*s for e in column3]\n",
"column4 = [e*s for e in column4]\n",
"column5 = [e*s for e in column5]"
]
},
{
"cell_type": "code",
"execution_count": 103,
"id": "5edc6cc6-caca-4c9e-97bf-00adfeb39012",
"metadata": {},
"outputs": [
{
"data": {
"text/plain": [
"[sqrt(3)*k**(1/3),\n",
" sqrt(3)*k**(1/3),\n",
" 2*k**(1/3),\n",
" 2*k**(1/3),\n",
" sqrt(3)*k**(1/3),\n",
" sqrt(3)*k**(1/3)]"
]
},
"execution_count": 103,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"column1"
]
},
{
"cell_type": "markdown",
"id": "2a44b5b0-c303-4c38-9fa3-abb71d08ec3d",
"metadata": {},
"source": [
"Now lets substitute in our expression for k and see how the overall lengths simplify."
]
},
{
"cell_type": "code",
"execution_count": 104,
"id": "13617192-aabb-4ec2-ad21-c7621189ba90",
"metadata": {},
"outputs": [],
"source": [
"rite = [e.subs({\"k\":rt2(2)/32}) for e in column1]\n",
"mite = [e.subs({\"k\":rt2(2)/32}) for e in column2]\n",
"bite = [e.subs({\"k\":rt2(2)/32}) for e in column3]\n",
"qrite = [e.subs({\"k\":rt2(2)/32}) for e in column4]\n",
"hrite = [e.subs({\"k\":rt2(2)/32}) for e in column5]"
]
},
{
"cell_type": "code",
"execution_count": 105,
"id": "d381c313-9942-41e9-9f2a-9cae82398874",
"metadata": {},
"outputs": [
{
"data": {
"text/plain": [
"[sqrt(6)/4, sqrt(6)/4, sqrt(2)/2, sqrt(2)/2, sqrt(6)/4, sqrt(6)/4]"
]
},
"execution_count": 105,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"rite # looking good"
]
},
{
"cell_type": "markdown",
"id": "15a54866-f491-4f41-974e-51b0a3a25d2e",
"metadata": {},
"source": [
"Now lets examine each module in turn, going left to right per the Goldberg Table (Table 1 in [the article](https://www.sciencedirect.com/science/article/pii/0097316574900582?ref=cra_js_challenge&fr=RR-1))."
]
},
{
"cell_type": "markdown",
"id": "482cd3a2-ea1f-4511-b3f8-fe439963061d",
"metadata": {},
"source": [
"### RITE"
]
},
{
"cell_type": "code",
"execution_count": 106,
"id": "05f56cb3-4f32-4177-98ba-d98dedb471e3",
"metadata": {},
"outputs": [
{
"data": {
"text/plain": [
"{'AB': sqrt(6)/4,\n",
" 'AC': sqrt(6)/4,\n",
" 'AD': sqrt(2)/2,\n",
" 'BC': sqrt(2)/2,\n",
" 'CD': sqrt(6)/4,\n",
" 'BD': sqrt(6)/4}"
]
},
"execution_count": 106,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"Rite = Tetrahedron(*rite)\n",
"Rite.edges()"
]
},
{
"cell_type": "code",
"execution_count": 107,
"id": "6bd964c0-7537-4123-8385-c5eb49f30362",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle \\frac{1}{4}$"
],
"text/plain": [
"1/4"
]
},
"execution_count": 107,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"Rite.ivm_volume() # check (2 Mites)"
]
},
{
"cell_type": "code",
"execution_count": 108,
"id": "a51930dc-39ee-4f22-84d9-3e26894c54bd",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 720.0$"
],
"text/plain": [
"720.000000000000"
]
},
"execution_count": 108,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"rite_angles = Rite.degrees(True)\n",
"sum(rite_angles.values())"
]
},
{
"cell_type": "code",
"execution_count": 109,
"id": "7f4ef1a9-e1c0-4b7e-bcf6-587e5e5c7341",
"metadata": {},
"outputs": [
{
"data": {
"text/plain": [
"{'BAC': 70.5287793655093,\n",
" 'CAD': 54.7356103172453,\n",
" 'BAD': 54.7356103172453,\n",
" 'ABC': 54.7356103172453,\n",
" 'CBD': 54.7356103172453,\n",
" 'ABD': 70.5287793655093,\n",
" 'ACB': 54.7356103172453,\n",
" 'ACD': 70.5287793655093,\n",
" 'BCD': 54.7356103172453,\n",
" 'ADC': 54.7356103172453,\n",
" 'BDC': 70.5287793655093,\n",
" 'ADB': 54.7356103172453}"
]
},
"execution_count": 109,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"rite_angles"
]
},
{
"cell_type": "code",
"execution_count": 110,
"id": "a74fbaa4-2dfa-4a1a-a5ee-0f177942ec75",
"metadata": {},
"outputs": [
{
"data": {
"text/plain": [
"{'AB': 60.0000000000000,\n",
" 'AC': 60.0000000000000,\n",
" 'AD': 90.0000000000000,\n",
" 'BC': 90.0000000000000,\n",
" 'CD': 60.0000000000000,\n",
" 'BD': 60.0000000000000}"
]
},
"execution_count": 110,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"rite_dihedrals = Rite.dihedrals(True, True)\n",
"rite_dihedrals"
]
},
{
"cell_type": "code",
"execution_count": 111,
"id": "1234fa90-cc2c-4204-a711-1984c04fa2a2",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 420.0$"
],
"text/plain": [
"420.000000000000"
]
},
"execution_count": 111,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"sum(rite_dihedrals.values())"
]
},
{
"cell_type": "markdown",
"id": "77041870-3281-4a10-93ba-c98e7c185e85",
"metadata": {},
"source": [
"### MITE"
]
},
{
"cell_type": "code",
"execution_count": 112,
"id": "83373760-e33c-49cf-8b96-3e2ce680c7e6",
"metadata": {},
"outputs": [
{
"data": {
"text/plain": [
"{'AB': sqrt(6)/4,\n",
" 'AC': 1/2,\n",
" 'AD': sqrt(2)/2,\n",
" 'BC': sqrt(2)/4,\n",
" 'CD': 1/2,\n",
" 'BD': sqrt(6)/4}"
]
},
"execution_count": 112,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"Mite = Tetrahedron(*mite)\n",
"Mite.edges()"
]
},
{
"cell_type": "code",
"execution_count": 113,
"id": "284b72ba-1822-4b8a-b3d1-9574bf63ccef",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle \\frac{1}{8}$"
],
"text/plain": [
"1/8"
]
},
"execution_count": 113,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"Mite.ivm_volume() # check"
]
},
{
"cell_type": "code",
"execution_count": 114,
"id": "827f3979-abdc-41ed-8867-7995b2ec085c",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 720.0$"
],
"text/plain": [
"720.000000000000"
]
},
"execution_count": 114,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"mite_angles = Mite.degrees(True)\n",
"sum(mite_angles.values())"
]
},
{
"cell_type": "code",
"execution_count": 115,
"id": "afbf3744-34d0-4c4f-88af-55e6f0944ecf",
"metadata": {},
"outputs": [
{
"data": {
"text/plain": [
"{'BAC': 35.2643896827547,\n",
" 'CAD': 45.0000000000000,\n",
" 'BAD': 54.7356103172453,\n",
" 'ABC': 54.7356103172453,\n",
" 'CBD': 54.7356103172453,\n",
" 'ABD': 70.5287793655093,\n",
" 'ACB': 90.0000000000000,\n",
" 'ACD': 90.0000000000000,\n",
" 'BCD': 90.0000000000000,\n",
" 'ADC': 45.0000000000000,\n",
" 'BDC': 35.2643896827547,\n",
" 'ADB': 54.7356103172453}"
]
},
"execution_count": 115,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"mite_angles"
]
},
{
"cell_type": "code",
"execution_count": 116,
"id": "2ec3c76c-34f4-4b4c-8b9c-d86a0539f1f9",
"metadata": {},
"outputs": [
{
"data": {
"text/plain": [
"{'AB': 60.0000000000000,\n",
" 'AC': 90.0000000000000,\n",
" 'AD': 45.0000000000000,\n",
" 'BC': 90.0000000000000,\n",
" 'CD': 90.0000000000000,\n",
" 'BD': 60.0000000000000}"
]
},
"execution_count": 116,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"mite_dihedrals = Mite.dihedrals(True, True)\n",
"mite_dihedrals"
]
},
{
"cell_type": "code",
"execution_count": 117,
"id": "0951fcb8-03ae-46e8-a202-e9d5f25d7c06",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 435.0$"
],
"text/plain": [
"435.000000000000"
]
},
"execution_count": 117,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"sum(mite_dihedrals.values())"
]
},
{
"cell_type": "markdown",
"id": "76218a5d-3d40-4b1b-a9a2-9e27c2eda14f",
"metadata": {},
"source": [
"### BITE"
]
},
{
"cell_type": "code",
"execution_count": 118,
"id": "206569c2-457d-4583-af93-c5e988da7a3b",
"metadata": {},
"outputs": [
{
"data": {
"text/plain": [
"{'AB': sqrt(6)/4,\n",
" 'AC': 1,\n",
" 'AD': sqrt(2)/2,\n",
" 'BC': sqrt(6)/4,\n",
" 'CD': sqrt(2)/2,\n",
" 'BD': sqrt(6)/4}"
]
},
"execution_count": 118,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"Bite = Tetrahedron(*bite)\n",
"Bite.edges()"
]
},
{
"cell_type": "code",
"execution_count": 119,
"id": "154737e0-71d5-477e-926e-f33a4feaeec2",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle \\frac{1}{4}$"
],
"text/plain": [
"1/4"
]
},
"execution_count": 119,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"Bite.ivm_volume() # check (2 Mites)"
]
},
{
"cell_type": "code",
"execution_count": 120,
"id": "2de000c9-ca7c-4e15-ad7c-0a2daa26e9eb",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 720.0$"
],
"text/plain": [
"720.000000000000"
]
},
"execution_count": 120,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"bite_angles = Bite.degrees(True)\n",
"sum(bite_angles.values())"
]
},
{
"cell_type": "code",
"execution_count": 121,
"id": "c25f7dfc-2866-4b73-a787-fe02c89ca150",
"metadata": {},
"outputs": [
{
"data": {
"text/plain": [
"{'BAC': 35.2643896827547,\n",
" 'CAD': 45.0000000000000,\n",
" 'BAD': 54.7356103172453,\n",
" 'ABC': 109.471220634491,\n",
" 'CBD': 70.5287793655093,\n",
" 'ABD': 70.5287793655093,\n",
" 'ACB': 35.2643896827547,\n",
" 'ACD': 45.0000000000000,\n",
" 'BCD': 54.7356103172453,\n",
" 'ADC': 90.0000000000000,\n",
" 'BDC': 54.7356103172453,\n",
" 'ADB': 54.7356103172453}"
]
},
"execution_count": 121,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"bite_angles"
]
},
{
"cell_type": "code",
"execution_count": 122,
"id": "ae5e1efb-a4ef-4f11-aef3-52cc228b0256",
"metadata": {},
"outputs": [
{
"data": {
"text/plain": [
"{'AB': 60.0000000000000,\n",
" 'AC': 90.0000000000000,\n",
" 'AD': 45.0000000000000,\n",
" 'BC': 60.0000000000000,\n",
" 'CD': 45.0000000000000,\n",
" 'BD': 120.000000000000}"
]
},
"execution_count": 122,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"bite_dihedrals = Bite.dihedrals(True, True)\n",
"bite_dihedrals"
]
},
{
"cell_type": "code",
"execution_count": 123,
"id": "209920d5-a3f3-4eb3-80b7-9c33a8efeb2d",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 420.0$"
],
"text/plain": [
"420.000000000000"
]
},
"execution_count": 123,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"sum(bite_dihedrals.values())"
]
},
{
"cell_type": "markdown",
"id": "9d14b28c-33a1-451c-b19a-9c48b00b89b5",
"metadata": {},
"source": [
"
\n",
"\n",
"[Figure 950.12](http://rwgrayprojects.com/synergetics/s09/figs/f5012.html)"
]
},
{
"cell_type": "markdown",
"id": "545888cd-152c-40de-904b-f77127b18bfd",
"metadata": {},
"source": [
"### 1/4 RITE"
]
},
{
"cell_type": "code",
"execution_count": 124,
"id": "fde4d9d0-8b4d-488d-ab65-acbbac3fdcbf",
"metadata": {},
"outputs": [
{
"data": {
"text/plain": [
"{'AB': sqrt(6)/4,\n",
" 'AC': sqrt(6)/4,\n",
" 'AD': sqrt(10)/8,\n",
" 'BC': sqrt(2)/2,\n",
" 'CD': sqrt(10)/8,\n",
" 'BD': sqrt(10)/8}"
]
},
"execution_count": 124,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"Qrite = Tetrahedron(*qrite)\n",
"Qrite.edges()"
]
},
{
"cell_type": "code",
"execution_count": 125,
"id": "d5133e8f-eb31-429b-bf2a-b723c71cd0a9",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle \\frac{1}{16}$"
],
"text/plain": [
"1/16"
]
},
"execution_count": 125,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"Qrite.ivm_volume() # Check (1/4 of 1/4)"
]
},
{
"cell_type": "code",
"execution_count": 126,
"id": "98392f31-286b-4c4c-9fe6-5949f0d4f632",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 720.0$"
],
"text/plain": [
"720.000000000000"
]
},
"execution_count": 126,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"qrite_angles = Qrite.degrees(True)\n",
"sum(qrite_angles.values())"
]
},
{
"cell_type": "code",
"execution_count": 127,
"id": "3d335806-4363-41a8-b064-95c01d241b3f",
"metadata": {},
"outputs": [
{
"data": {
"text/plain": [
"{'BAC': 70.5287793655093,\n",
" 'CAD': 39.2315204835923,\n",
" 'BAD': 39.2315204835923,\n",
" 'ABC': 54.7356103172453,\n",
" 'CBD': 26.5650511770780,\n",
" 'ABD': 39.2315204835923,\n",
" 'ACB': 54.7356103172453,\n",
" 'ACD': 39.2315204835923,\n",
" 'BCD': 26.5650511770780,\n",
" 'ADC': 101.536959032815,\n",
" 'BDC': 126.869897645844,\n",
" 'ADB': 101.536959032815}"
]
},
"execution_count": 127,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"qrite_angles"
]
},
{
"cell_type": "code",
"execution_count": 128,
"id": "b3d4511d-8374-4af6-b224-9b470061d371",
"metadata": {},
"outputs": [
{
"data": {
"text/plain": [
"{'AB': 30.0000000000000,\n",
" 'AC': 30.0000000000000,\n",
" 'AD': 131.810314895779,\n",
" 'BC': 45.0000000000000,\n",
" 'CD': 114.094842552111,\n",
" 'BD': 114.094842552111}"
]
},
"execution_count": 128,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"qrite_dihedrals = Qrite.dihedrals(True, True)\n",
"qrite_dihedrals"
]
},
{
"cell_type": "code",
"execution_count": 129,
"id": "38f73ebc-16fc-48e6-9deb-a8ba84fe31f2",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 465.0$"
],
"text/plain": [
"465.000000000000"
]
},
"execution_count": 129,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"sum(qrite_dihedrals.values()) # mismatch with column 4"
]
},
{
"cell_type": "markdown",
"id": "a93ba5f1-bc58-4e78-8c1f-db796efd9568",
"metadata": {},
"source": [
"### 1/2 RITE"
]
},
{
"cell_type": "code",
"execution_count": 130,
"id": "fb67b039-8327-4aa1-b699-cbff5b2b48c1",
"metadata": {},
"outputs": [
{
"data": {
"text/plain": [
"{'AB': sqrt(6)/4,\n",
" 'AC': sqrt(6)/4,\n",
" 'AD': sqrt(22)/8,\n",
" 'BC': sqrt(2)/2,\n",
" 'CD': sqrt(6)/8,\n",
" 'BD': sqrt(22)/8}"
]
},
"execution_count": 130,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"Hrite = Tetrahedron(*hrite)\n",
"Hrite.edges()"
]
},
{
"cell_type": "code",
"execution_count": 131,
"id": "d1ee890f-da36-4288-8d05-0bdfd3e6e8d6",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle \\frac{1}{8}$"
],
"text/plain": [
"1/8"
]
},
"execution_count": 131,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"Hrite.ivm_volume() # Check (1/2 of 1/4)"
]
},
{
"cell_type": "code",
"execution_count": 132,
"id": "3d8c32e4-e693-457d-96d1-9860ca6dc0b3",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 720.0$"
],
"text/plain": [
"720.000000000000"
]
},
"execution_count": 132,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"hrite_angles = Hrite.degrees(True)\n",
"sum(hrite_angles.values())"
]
},
{
"cell_type": "code",
"execution_count": 133,
"id": "ec992182-e4f9-4327-a9ad-7b3b97fa28fd",
"metadata": {},
"outputs": [
{
"data": {
"text/plain": [
"{'BAC': 70.5287793655093,\n",
" 'CAD': 29.4962084965664,\n",
" 'BAD': 58.5178458947061,\n",
" 'ABC': 54.7356103172453,\n",
" 'CBD': 25.2394018206789,\n",
" 'ABD': 58.5178458947061,\n",
" 'ACB': 54.7356103172453,\n",
" 'ACD': 70.5287793655093,\n",
" 'BCD': 54.7356103172453,\n",
" 'ADC': 79.9750121379243,\n",
" 'BDC': 100.024987862076,\n",
" 'ADB': 62.9643082105877}"
]
},
"execution_count": 133,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"hrite_angles"
]
},
{
"cell_type": "code",
"execution_count": 134,
"id": "62846e52-9462-4394-ac0d-4972526ef558",
"metadata": {},
"outputs": [
{
"data": {
"text/plain": [
"{'AB': 30.0000000000000,\n",
" 'AC': 60.0000000000000,\n",
" 'AD': 106.778654880960,\n",
" 'BC': 90.0000000000000,\n",
" 'CD': 60.0000000000000,\n",
" 'BD': 73.2213451190396}"
]
},
"execution_count": 134,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"hite_dihedrals = Hrite.dihedrals(True, True)\n",
"hite_dihedrals"
]
},
{
"cell_type": "code",
"execution_count": 135,
"id": "e91ea279-93b7-4c80-bc37-4a204671a9ab",
"metadata": {},
"outputs": [
{
"data": {
"text/latex": [
"$\\displaystyle 420.0$"
],
"text/plain": [
"420.000000000000"
]
},
"execution_count": 135,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"sum(hite_dihedrals.values())"
]
}
],
"metadata": {
"kernelspec": {
"display_name": "Python 3 (ipykernel)",
"language": "python",
"name": "python3"
},
"language_info": {
"codemirror_mode": {
"name": "ipython",
"version": 3
},
"file_extension": ".py",
"mimetype": "text/x-python",
"name": "python",
"nbconvert_exporter": "python",
"pygments_lexer": "ipython3",
"version": "3.9.15"
}
},
"nbformat": 4,
"nbformat_minor": 5
}