# Architecture Learning Journey In this learning journey you will learn about the Cinemax app architecture: its layers, key classes and the interactions between them. ## Goals and requirements The goals for the app architecture are: - Follow the [official architecture guidance](https://developer.android.com/jetpack/guide) as closely as possible. - Easy for developers to understand, nothing too experimental. - Support multiple developers working on the same codebase. - Facilitate local and instrumented tests, both on the developer’s machine and using Continuous Integration (CI). - Minimize build times. ## Architecture overview The app architecture has three layers: a [UI layer](https://developer.android.com/jetpack/guide/ui-layer), a [data layer](https://developer.android.com/jetpack/guide/data-layer) and a [domain layer](https://developer.android.com/jetpack/guide/domain-layer). 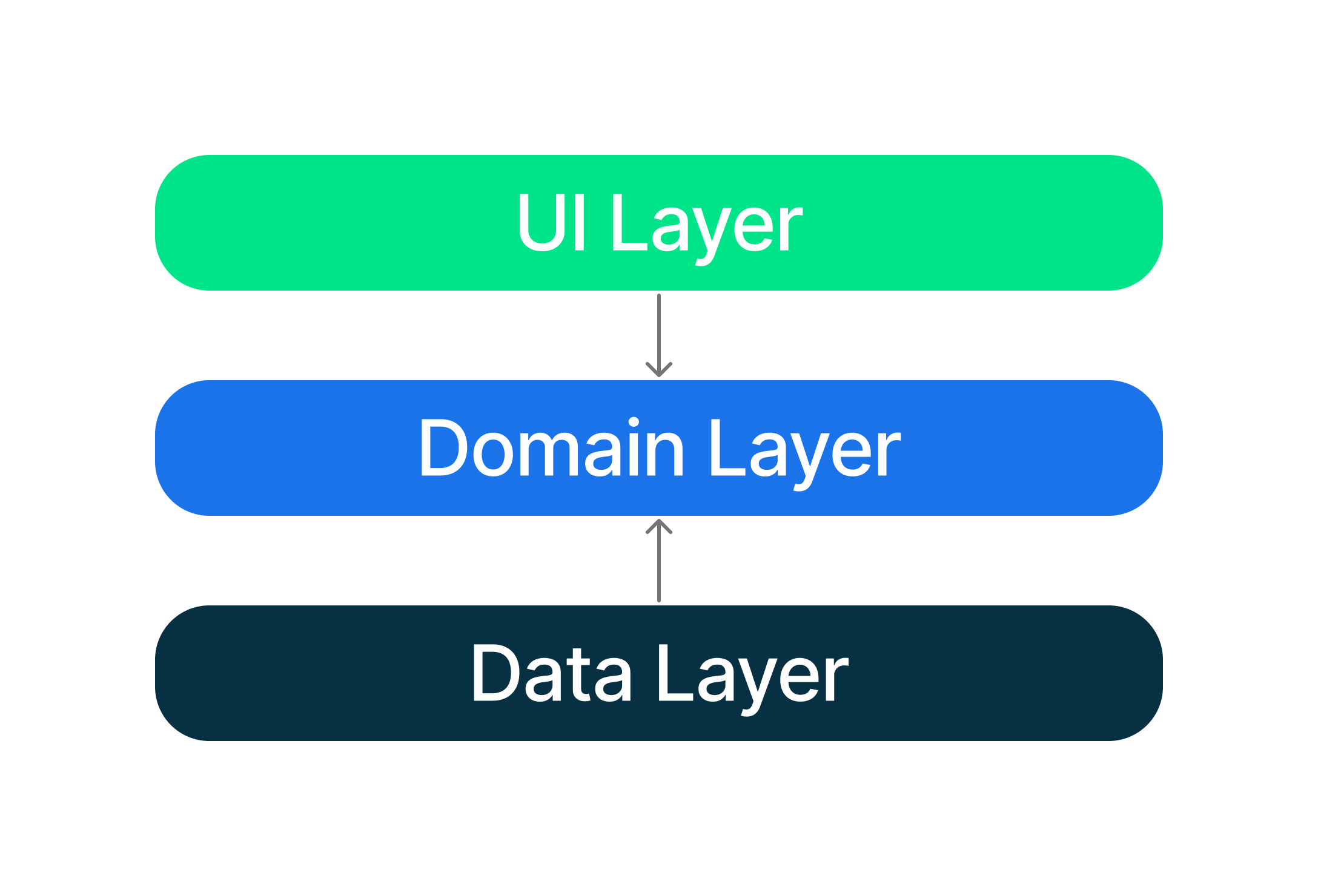 The architecture follows a reactive programming model with [unidirectional data flow](https://developer.android.com/jetpack/guide/ui-layer#udf). With the data layer at the bottom, the key concepts are: - Higher layers react to changes in lower layers. - Events flow down. - Data flows up. The data flow is achieved using streams, implemented using [Kotlin Flows](https://developer.android.com/kotlin/flow). ## Data layer The data layer is implemented as an offline-first source of app data and business logic. It is the source of truth for all data in the app. ### Reading data Data is exposed as data streams. This means each client of the repository must be prepared to react to data changes. ### Writing data To write data, the repository provides suspend functions. It is up to the caller to ensure that their execution is suitably scoped. ### Data sources A repository may depend on one or more data sources. For example, the `MovieRepositoryImpl` depends on the following data sources:
Name | Backed by | Purpose |
MovieDatabaseDataSource | Room/SQLite | Persistent relational data associated with Movies. |
MovieNetworkDataSource | Remote API accessed using Retrofit | Data for movies, provided through REST API endpoints as JSON. |