{
"cells": [
{
"cell_type": "markdown",
"metadata": {},
"source": [
"# Use Case: Matched Instances Input\n"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"## Install Dependencies"
]
},
{
"cell_type": "code",
"execution_count": 10,
"metadata": {},
"outputs": [],
"source": [
"!pip install panoptica auxiliary rich numpy > /dev/null"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"If you installed the packages and requirements on your own machine, you can skip this section and start from the import section.\n",
"\n",
"### Setup Colab environment (optional) \n",
"Otherwise you can follow and execute the tutorial on your browser.\n",
"In order to start working on the notebook, click on the following button, this will open this page in the Colab environment and you will be able to execute the code on your own (*Google account required*).\n",
"\n",
"\n",
" \n",
"\n",
"\n",
"Now that you are visualizing the notebook in Colab, run the next cell to install the packages we will use. There are few things you should follow in order to properly set the notebook up:\n",
"1. Warning: This notebook was not authored by Google. Click on 'Run anyway'.\n",
"1. When the installation commands are done, there might be \"Restart runtime\" button at the end of the output. Please, click it.\n",
"If you run the next cell in a Google Colab environment, it will **clone the 'tutorials' repository** in your google drive. This will create a **new folder** called \"tutorials\" in **your Google Drive**.\n",
"All generated file will be created/uploaded to your Google Drive respectively.\n",
"\n",
"After the first execution of the next cell, you might receive some warnings and notifications, please follow these instructions:\n",
" - 'Permit this notebook to access your Google Drive files?' Click on 'Yes', and select your account.\n",
" - Google Drive for desktop wants to access your Google Account. Click on 'Allow'.\n",
"\n",
"Afterwards the \"tutorials\" folder has been created. You can navigate it through the lefthand panel in Colab. You might also have received an email that informs you about the access on your Google Drive."
]
},
{
"cell_type": "code",
"execution_count": 11,
"metadata": {},
"outputs": [],
"source": [
"import sys\n",
"\n",
"# Check if we are in google colab currently\n",
"try:\n",
" import google.colab\n",
"\n",
" colabFlag = True\n",
"except ImportError as r:\n",
" colabFlag = False\n",
"\n",
"# Execute certain steps only if we are in a colab environment\n",
"if colabFlag:\n",
" # Create a folder in your Google Drive\n",
" from google.colab import drive\n",
"\n",
" drive.mount(\"/content/drive\")\n",
" # clone repository and set path\n",
" !git clone https://github.com/BrainLesion/tutorials.git /content/drive/MyDrive/tutorials\n",
" BASE_PATH = \"/content/drive/MyDrive/tutorials/panoptica\"\n",
" sys.path.insert(0, BASE_PATH)\n",
"\n",
"else: # normal jupyter notebook environment\n",
" BASE_PATH = \".\" # current working directory would be BraTs-Toolkit anyways if you are not in colab"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"## Setup Imports"
]
},
{
"cell_type": "code",
"execution_count": 12,
"metadata": {},
"outputs": [],
"source": [
"import numpy as np\n",
"from auxiliary.nifti.io import read_nifti\n",
"from rich import print as pprint\n",
"from panoptica import InputType, Panoptica_Evaluator\n",
"from panoptica.metrics import Metric"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"## Load Data"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"To demonstrate we use a reference and predicition of spine a segmentation with matched instances.\n",
"\n",
"\n",
"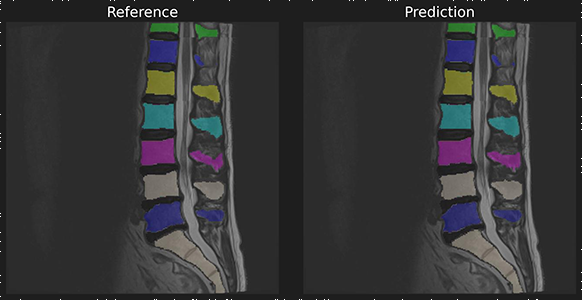"
]
},
{
"cell_type": "code",
"execution_count": 13,
"metadata": {},
"outputs": [
{
"data": {
"text/plain": [
"(array([ 0, 2, 3, 4, 5, 6, 7, 8, 26, 102, 103, 104, 105,\n",
" 106, 107, 108, 202, 203, 204, 205, 206, 207, 208], dtype=uint8),\n",
" array([ 0, 2, 3, 4, 5, 6, 7, 8, 26, 102, 103, 104, 105,\n",
" 106, 107, 108, 202, 203, 204, 205, 206, 207, 208], dtype=uint8))"
]
},
"execution_count": 13,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"ref_masks = read_nifti(f\"{BASE_PATH}/spine_seg/matched_instance/ref.nii.gz\")\n",
"pred_masks = read_nifti(f\"{BASE_PATH}/spine_seg/matched_instance/pred.nii.gz\")\n",
"\n",
"# labels are matching\n",
"np.unique(ref_masks), np.unique(pred_masks)"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"## Run Evaluation"
]
},
{
"cell_type": "code",
"execution_count": null,
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"Panoptic: Start Evaluation\n",
"-- Got MatchedInstancePair, will evaluate instances\n"
]
}
],
"source": [
"evaluator = Panoptica_Evaluator(\n",
" expected_input=InputType.MATCHED_INSTANCE,\n",
" decision_metric=Metric.IOU,\n",
" decision_threshold=0.5,\n",
")\n",
"\n",
"result = evaluator.evaluate(pred_masks, ref_masks)[\"ungrouped\"]"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"## Inspect Results\n",
"The results object allows access to individual metrics and provides helper methods for further processing"
]
},
{
"cell_type": "code",
"execution_count": 15,
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"\n",
"+++ MATCHING +++\n",
"Number of instances in reference (num_ref_instances): 22\n",
"Number of instances in prediction (num_pred_instances): 22\n",
"True Positives (tp): 22\n",
"False Positives (fp): 0\n",
"False Negatives (fn): 0\n",
"Recognition Quality / F1-Score (rq): 1.0\n",
"\n",
"+++ GLOBAL +++\n",
"Global Binary Dice (global_bin_dsc): 0.9744370224078394\n",
"\n",
"+++ INSTANCE +++\n",
"Segmentation Quality IoU (sq): 0.8328184295330796 +- 0.15186064004517466\n",
"Panoptic Quality IoU (pq): 0.8328184295330796\n",
"Segmentation Quality Dsc (sq_dsc): 0.900292616009954 +- 0.10253566174957332\n",
"Panoptic Quality Dsc (pq_dsc): 0.900292616009954\n",
"Segmentation Quality ASSD (sq_assd): 0.250331887879225 +- 0.07696680402317076\n",
"Segmentation Quality Relative Volume Difference (sq_rvd): 0.0028133049062930553 +- 0.034518928495505724\n",
"\n"
]
}
],
"source": [
"# print all results\n",
"print(result)"
]
},
{
"cell_type": "code",
"execution_count": 16,
"metadata": {},
"outputs": [
{
"data": {
"text/html": [
"
result.pq=0.8328184295330796\n", "\n" ], "text/plain": [ "result.\u001b[33mpq\u001b[0m=\u001b[1;36m0\u001b[0m\u001b[1;36m.8328184295330796\u001b[0m\n" ] }, "metadata": {}, "output_type": "display_data" } ], "source": [ "# get specific metric, e.g. pq\n", "pprint(f\"{result.pq=}\")" ] }, { "cell_type": "code", "execution_count": 17, "metadata": {}, "outputs": [ { "data": { "text/html": [ "
results dict: \n", "{\n", " 'num_ref_instances': 22,\n", " 'num_pred_instances': 22,\n", " 'tp': 22,\n", " 'fp': 0,\n", " 'fn': 0,\n", " 'prec': 1.0,\n", " 'rec': 1.0,\n", " 'rq': 1.0,\n", " 'sq': 0.8328184295330796,\n", " 'sq_std': 0.15186064004517466,\n", " 'pq': 0.8328184295330796,\n", " 'sq_dsc': 0.900292616009954,\n", " 'sq_dsc_std': 0.10253566174957332,\n", " 'pq_dsc': 0.900292616009954,\n", " 'sq_assd': 0.250331887879225,\n", " 'sq_assd_std': 0.07696680402317076,\n", " 'sq_rvd': 0.0028133049062930553,\n", " 'sq_rvd_std': 0.034518928495505724,\n", " 'global_bin_dsc': 0.9744370224078394\n", "}\n", "\n" ], "text/plain": [ "results dict: \n", "\u001b[1m{\u001b[0m\n", " \u001b[32m'num_ref_instances'\u001b[0m: \u001b[1;36m22\u001b[0m,\n", " \u001b[32m'num_pred_instances'\u001b[0m: \u001b[1;36m22\u001b[0m,\n", " \u001b[32m'tp'\u001b[0m: \u001b[1;36m22\u001b[0m,\n", " \u001b[32m'fp'\u001b[0m: \u001b[1;36m0\u001b[0m,\n", " \u001b[32m'fn'\u001b[0m: \u001b[1;36m0\u001b[0m,\n", " \u001b[32m'prec'\u001b[0m: \u001b[1;36m1.0\u001b[0m,\n", " \u001b[32m'rec'\u001b[0m: \u001b[1;36m1.0\u001b[0m,\n", " \u001b[32m'rq'\u001b[0m: \u001b[1;36m1.0\u001b[0m,\n", " \u001b[32m'sq'\u001b[0m: \u001b[1;36m0.8328184295330796\u001b[0m,\n", " \u001b[32m'sq_std'\u001b[0m: \u001b[1;36m0.15186064004517466\u001b[0m,\n", " \u001b[32m'pq'\u001b[0m: \u001b[1;36m0.8328184295330796\u001b[0m,\n", " \u001b[32m'sq_dsc'\u001b[0m: \u001b[1;36m0.900292616009954\u001b[0m,\n", " \u001b[32m'sq_dsc_std'\u001b[0m: \u001b[1;36m0.10253566174957332\u001b[0m,\n", " \u001b[32m'pq_dsc'\u001b[0m: \u001b[1;36m0.900292616009954\u001b[0m,\n", " \u001b[32m'sq_assd'\u001b[0m: \u001b[1;36m0.250331887879225\u001b[0m,\n", " \u001b[32m'sq_assd_std'\u001b[0m: \u001b[1;36m0.07696680402317076\u001b[0m,\n", " \u001b[32m'sq_rvd'\u001b[0m: \u001b[1;36m0.0028133049062930553\u001b[0m,\n", " \u001b[32m'sq_rvd_std'\u001b[0m: \u001b[1;36m0.034518928495505724\u001b[0m,\n", " \u001b[32m'global_bin_dsc'\u001b[0m: \u001b[1;36m0.9744370224078394\u001b[0m\n", "\u001b[1m}\u001b[0m\n" ] }, "metadata": {}, "output_type": "display_data" } ], "source": [ "# get dict for further processing, e.g. for pandas\n", "pprint(\"results dict: \", result.to_dict())" ] }, { "cell_type": "code", "execution_count": null, "metadata": {}, "outputs": [ { "name": "stdout", "output_type": "stream", "text": [ "key SEMANTIC not in intermediate steps, maybe the step was skipped?\n" ] } ], "source": [ "# To inspect different phases, just use the returned intermediate_steps_data object\n", "intermediate_steps_data = result.intermediate_steps_data\n", "intermediate_steps_data.original_prediction_arr # yields input prediction array\n", "intermediate_steps_data.original_reference_arr # yields input reference array\n", "\n", "intermediate_steps_data.prediction_arr(\n", " InputType.MATCHED_INSTANCE\n", ") # yields prediction array after instances have been matched\n", "intermediate_steps_data.reference_arr(\n", " InputType.MATCHED_INSTANCE\n", ") # yields reference array after instances have been matched\n", "\n", "# The other InputType do not work here, as the input was already a matched instance map, therefore the steps from instance approximation and matching have been skipped\n", "try:\n", " intermediate_steps_data.reference_arr(InputType.SEMANTIC)\n", "except AssertionError as e:\n", " print(e)\n", " # Error will indicate the problem" ] } ], "metadata": { "kernelspec": { "display_name": "helm", "language": "python", "name": "python3" }, "language_info": { "codemirror_mode": { "name": "ipython", "version": 3 }, "file_extension": ".py", "mimetype": "text/x-python", "name": "python", "nbconvert_exporter": "python", "pygments_lexer": "ipython3", "version": "3.10.14" } }, "nbformat": 4, "nbformat_minor": 2 }