# Gateway API Policies DCE 5.0 cloud native gateway supports several API policies, including load balancing, timeout, retries, and blacklisting/whitelisting. This article demonstrates how to configure these policies and their specific effects using a real microservice application. !!! note - For detailed configuration steps and parameter explanations for each policy, refer to [API Policy Configuration](../gateway/api/api-policy.md). - This article focuses on showcasing the effects of each policy and may omit some configuration steps. ## Prerequisites - Prepare a demo service, such as `my-otel-demo-adservice`. - Create a [gateway](../gateway/index.md), [domain](../gateway/domain/index.md), and [API](../gateway/api/index.md). - Connect the demo service to the gateway as explained in [Connecting Services to the Gateway](gateway01.md). ## Load Balancing 1. Scale the demo service to multiple replicas for load balancing demonstration. 2. Configure the load balancing policy following the instructions in [Load Balancing](../gateway/api/api-policy.md#_2). 3. Write a script to record the traffic received by each service replica. ??? note "Click to view the script content" ```python import requests def lb_pod_count(): pod1_ip = "10.244.1.135" # IP address of the service replica's node pod2_ip = "10.244.2.207" # IP address of the service replica's node pod3_ip = "10.244.3.193" # IP address of the service replica's node pod1_count = 0 pod2_count = 0 pod3_count =0 host = "http://10.6.222.24:30040/ip" # Service access address headers = {"Host": "ad.service.virtualhost"} for i in range(300): res_ip = requests.get(host, headers=headers).text[11:] if res_ip == pod1_ip: pod1_count += 1 elif res_ip == pod2_ip: pod2_count += 1 elif res_ip == pod3_ip: pod3_count += 1 print("Traffic directed to %s: %d" %(pod1_ip,pod1_count)) print("Traffic directed to %s: %d" %(pod2_ip,pod2_count)) print("Traffic directed to %s: %d" %(pod3_ip,pod3_count)) if __name__ == '__main__': lb_pod_count() ``` 4. The effect of random load balancing is as follows. Each replica receives traffic randomly, resulting in some replicas being under more load. 5. The effect of round-robin load balancing is as follows. Each replica receives traffic in a round-robin fashion, resulting in a similar total traffic load for each replica. ## Rewrite Path 1. In the initial state, accessing the root path of the service returns the content as shown in the image.  2. Configure the path rewriting policy to change the access from the root path to the `/test2` endpoint. 3. The effect is shown in the image. From the returned content, it can be seen that the accessed endpoint has changed, and the returned data has also changed.  ## Configure Timeout 1. Enable the timeout configuration and set it to consider the request as failed if the response exceeds 3 seconds. 2. Use the dedicated test endpoint `/timeout` to make the request respond within 1 second. The expectation is that the request will succeed. The image below shows a successful access, and the interface returns the set request time.  3. Use the dedicated test endpoint `/timeout` to make the request respond within 4 seconds. The expectation is that the request will fail. The image below shows a failed access, and the interface returns an upstream request timeout message.  ## Retry Mechanism 1. Enable the retry configuration with a maximum of 6 retries when the request fails, and consider it a retry failure if the retry response time exceeds 1 second. 2. Use the dedicated test endpoint `/set-retry-count` to make the `/retry` endpoint of the service require 3 requests to succeed.  Access the `retry` endpoint. With 6 retries, it is expected to succeed. 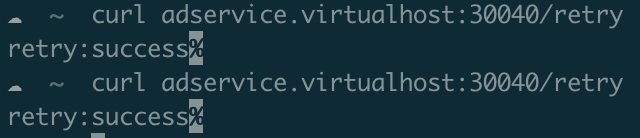 3. Use the dedicated test endpoint `/set-retry-count` to make the `/retry` endpoint of the service require 7 requests to succeed.  Access the `retry` endpoint. With 6 retries, it is expected to succeed as well. Because the initial successful request plus the 6 retries after failure adds up to the required 7 requests. 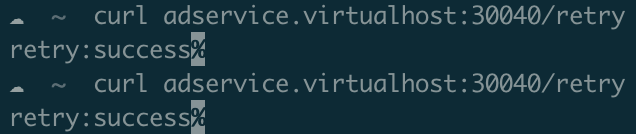 4. Use the dedicated test endpoint `/set-retry-count` to make the `/retry` endpoint of the service require 8 requests to succeed.  Access the `retry` endpoint. With 6 retries, the first attempt is expected to fail. Because the initial successful request plus the 6 retries after failure can only reach a maximum of 7 requests, which is not enough for the required 8 requests. However, manually accessing the endpoint again will show a successful access. At this point, the accumulated number of accesses has reached 8. 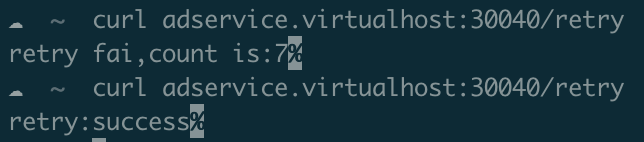 ## Rewrite Request Header 1. Enable the request header rewriting policy to remove the `user-agent` (case-insensitive) header and add `demo-req` when making requests to the service. 2. Use Postman tool to view the request headers present in the initial state. It can be seen that `user-agent` exists, but `demo-req` is not present. 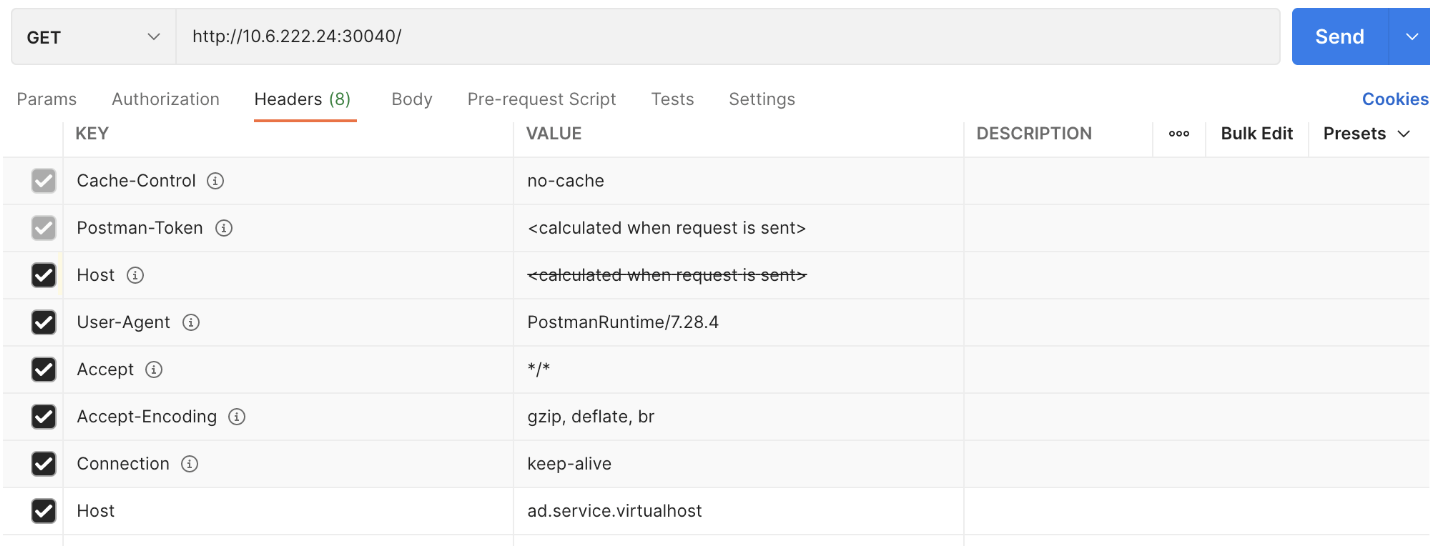 3. Use the dedicated test endpoint `/request-header` to check if the request includes the `user-agent` header. The returned value of `user-agent` is null, indicating that the header has been removed and the rewriting policy is effective. 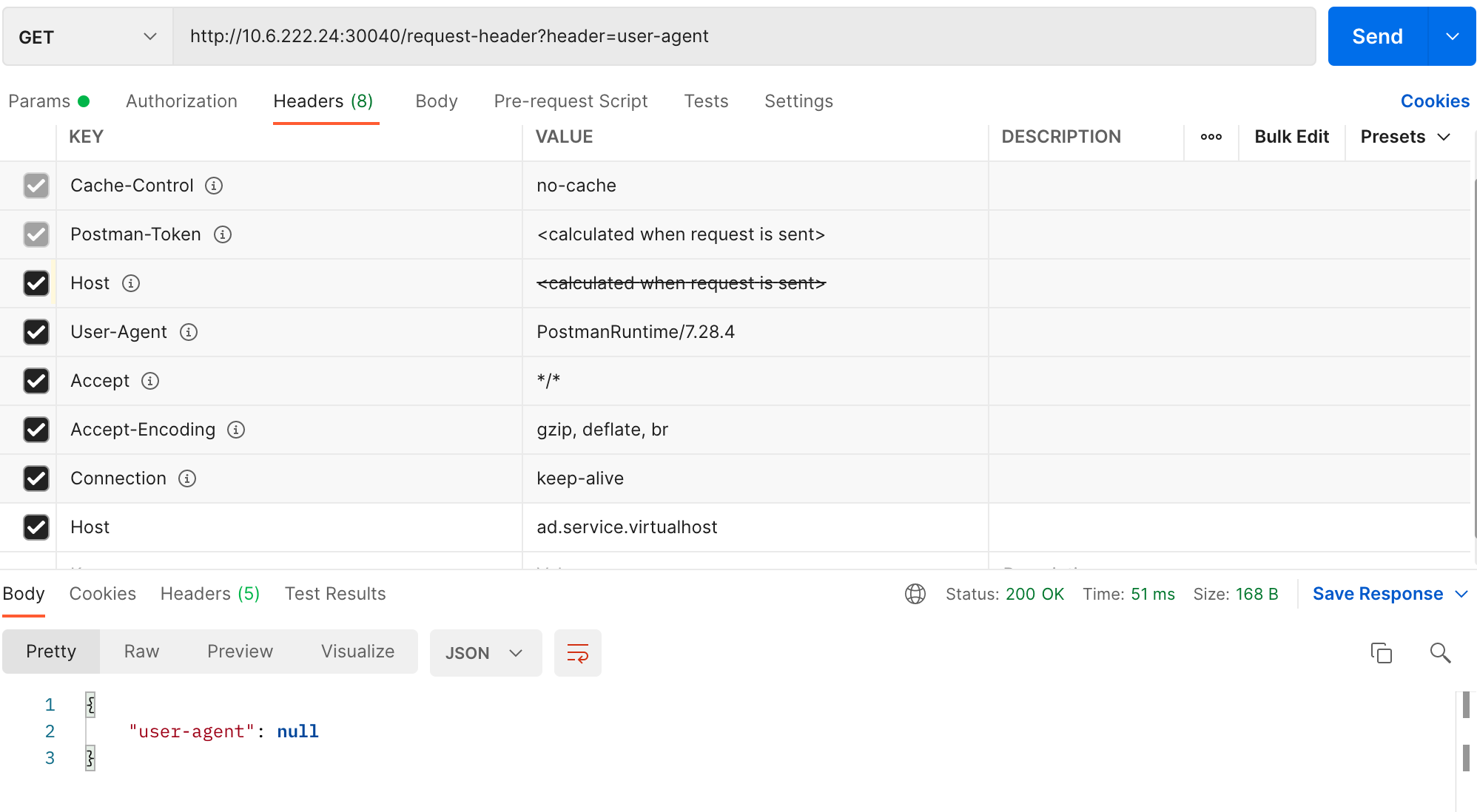 4. Use the dedicated test endpoint `/request-header` to check if the request includes the `user-agent` header. The expected value is returned, indicating that the header has been added and the rewriting policy is effective. 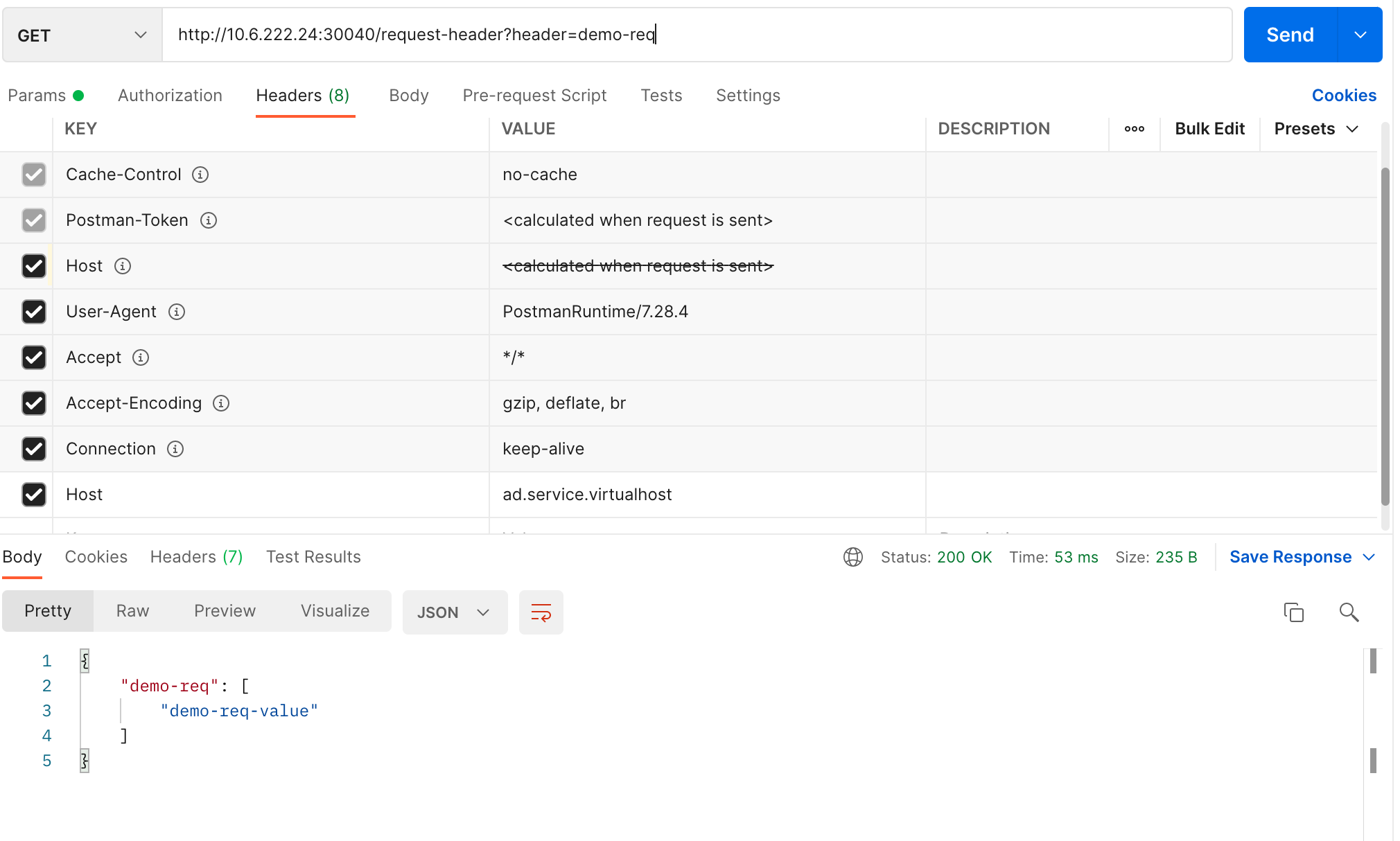 ## Rewrite Response Header 1. Enable the response header rewriting policy to remove the `x-envoy-upstream-service-time` (case-insensitive) response header and add `demo-res`. 2. Use Postman tool to view the response headers in the initial state. It can be seen that `x-envoy-upstream-service-time` exists, but `demo-res` is not present. 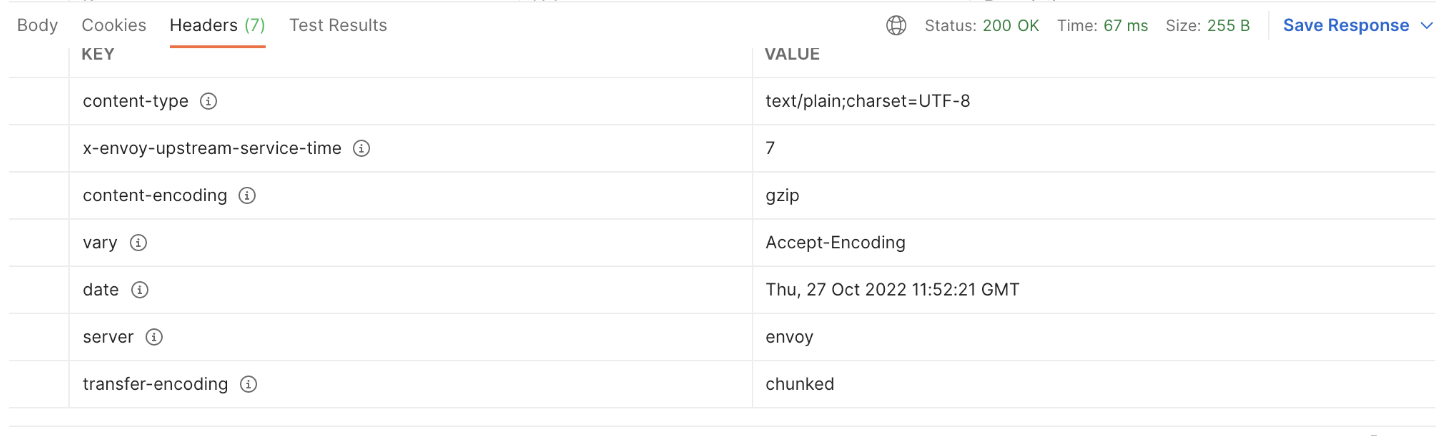 3. Access the service directly. The response headers do not include `x-envoy-upstream-service-time`, but `demo-res` is present, indicating that the rewriting policy is effective. 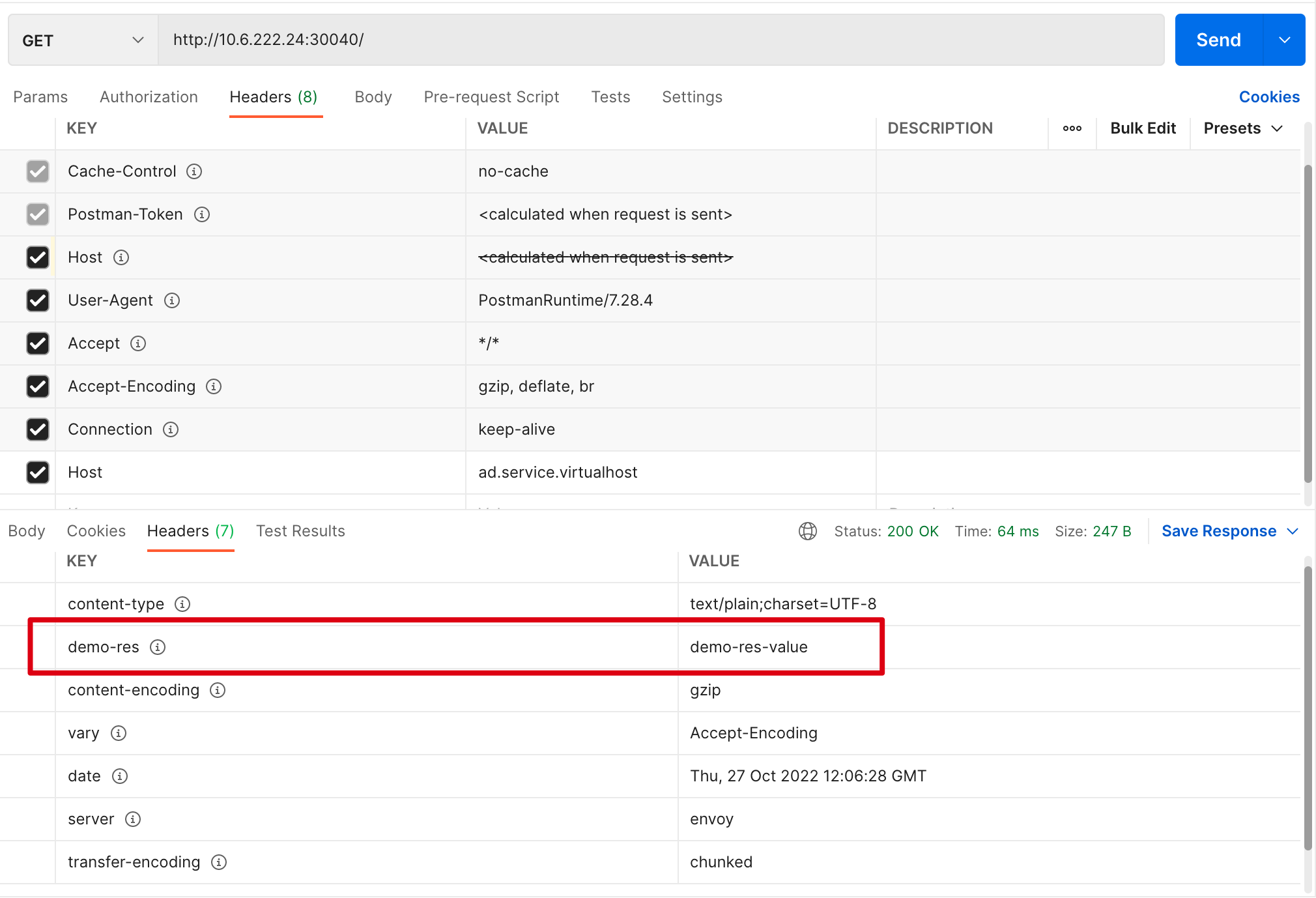 ## WebSocket 1. Write a script to test WebSocket connections. ??? note "Click to view the script" ```html