{
"nbformat": 4,
"nbformat_minor": 0,
"metadata": {
"colab": {
"name": "3_T5_question_answering_and_Text_summarization.ipynb",
"provenance": [],
"collapsed_sections": [],
"toc_visible": true
},
"kernelspec": {
"name": "python3",
"display_name": "Python 3"
}
},
"cells": [
{
"cell_type": "markdown",
"metadata": {
"id": "CebbOFtOMv6X"
},
"source": [
"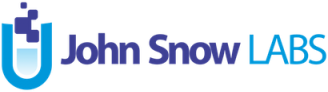\n",
"\n",
"\n",
"\n",
"[](https://colab.research.google.com/github/JohnSnowLabs/nlu/blob/master/examples/colab/component_examples/sequence2sequence/T5_question_answering.ipynb)\n",
"\n",
"# `Open book` and `Closed book` question answering with Google's T5 \n",
"With the latest NLU release and Google's T5 you can answer **general knowledge based questions given no context** and in addition answer **questions on text databases**. \n",
"These questions can be asked in natural human language and answerd in just 1 line with NLU!.\n",
"\n",
"\n",
"\n",
"\n",
"## What is a `open book question`? \n",
"You can imagine an `open book` question similar to an examen where you are allowed to bring in text documents or cheat sheets that help you answer questions in an examen. Kinda like bringing a history book to an history examen. \n",
"\n",
"In `T5's` terms, this means the model is given a `question` and an **additional piece of textual information** or so called `context`.\n",
"\n",
"This enables the `T5` model to answer questions on textual datasets like `medical records`,`newsarticles` , `wiki-databases` , `stories` and `movie scripts` , `product descriptions`, 'legal documents' and many more.\n",
"\n",
"You can answer `open book question` in 1 line of code, leveraging the latest NLU release and Google's T5. \n",
"All it takes is : \n",
"\n",
"```python\n",
"nlu.load('answer_question').predict(\"\"\"\n",
"Where did Jebe die?\n",
"context: Ghenkis Khan recalled Subtai back to Mongolia soon afterwards,\n",
" and Jebe died on the road back to Samarkand\"\"\")\n",
">>> Output: Samarkand\n",
"```\n",
"\n",
"Example for answering medical questions based on medical context\n",
"``` python\n",
"question ='''\n",
"What does increased oxygen concentrations in the patient’s lungs displace? \n",
"context: Hyperbaric (high-pressure) medicine uses special oxygen chambers to increase the partial pressure of O 2 around the patient and, when needed, the medical staff. \n",
"Carbon monoxide poisoning, gas gangrene, and decompression sickness (the ’bends’) are sometimes treated using these devices. Increased O 2 concentration in the lungs helps to displace carbon monoxide from the heme group of hemoglobin.\n",
" Oxygen gas is poisonous to the anaerobic bacteria that cause gas gangrene, so increasing its partial pressure helps kill them. Decompression sickness occurs in divers who decompress too quickly after a dive, resulting in bubbles of inert gas, mostly nitrogen and helium, forming in their blood. Increasing the pressure of O 2 as soon as possible is part of the treatment.\n",
"'''\n",
"\n",
"\n",
"#Predict on text data with T5\n",
"nlu.load('answer_question').predict(question)\n",
">>> Output: carbon monoxide\t\n",
"```\n",
"\n",
"Take a look at this example on a recent news article snippet : \n",
"```python\n",
"question1 = 'Who is Jack ma?'\n",
"question2 = 'Who is founder of Alibaba Group?'\n",
"question3 = 'When did Jack Ma re-appear?'\n",
"question4 = 'How did Alibaba stocks react?'\n",
"question5 = 'Whom did Jack Ma meet?'\n",
"question6 = 'Who did Jack Ma hide from?'\n",
"\n",
"# from https://www.bbc.com/news/business-55728338 \n",
"news_article_snippet = \"\"\" context:\n",
"Alibaba Group founder Jack Ma has made his first appearance since Chinese regulators cracked down on his business empire.\n",
"His absence had fuelled speculation over his whereabouts amid increasing official scrutiny of his businesses.\n",
"The billionaire met 100 rural teachers in China via a video meeting on Wednesday, according to local government media.\n",
"Alibaba shares surged 5% on Hong Kong's stock exchange on the news.\n",
"\"\"\"\n",
"# join question with context, works with Pandas DF aswell!\n",
"questions = [\n",
" question1+ news_article_snippet,\n",
" question2+ news_article_snippet,\n",
" question3+ news_article_snippet,\n",
" question4+ news_article_snippet,\n",
" question5+ news_article_snippet,\n",
" question6+ news_article_snippet,]\n",
"nlu.load('answer_question').predict(questions)\n",
"```\n",
"This will output a Pandas Dataframe similar to this : \n",
"\n",
"|Answer|Question|\n",
"|-----|---------|\n",
"Alibaba Group founder| \tWho is Jack ma? | \n",
"|Jack Ma\t|Who is founder of Alibaba Group? | \n",
"Wednesday\t| When did Jack Ma re-appear? | \n",
"surged 5%\t| How did Alibaba stocks react? | \n",
"100 rural teachers\t| Whom did Jack Ma meet? | \n",
"Chinese regulators\t|Who did Jack Ma hide from?|\n",
"\n",
"\n",
"\n",
"## What is a `closed book question`? \n",
"A `closed book question` is the exact opposite of a `open book question`. In an examen scenario, you are only allowed to use what you have memorized in your brain and nothing else. \n",
"In `T5's` terms this means that T5 can only use it's stored weights to answer a `question` and is given **no aditional context**. \n",
"`T5` was pre-trained on the [C4 dataset](https://commoncrawl.org/) which contains **petabytes of web crawling data** collected over the last 8 years, including Wikipedia in every language.\n",
"\n",
"\n",
"This gives `T5` the broad knowledge of the internet stored in it's weights to answer various `closed book questions` \n",
"\n",
"You can answer `closed book question` in 1 line of code, leveraging the latest NLU release and Google's T5. \n",
"You need to pass one string to NLU, which starts which a `question` and is followed by a `context:` tag and then the actual context contents. \n",
"All it takes is : \n",
"\n",
"\n",
"```python\n",
"nlu.load('en.t5').predict('Who is president of Nigeria?')\n",
">>> Muhammadu Buhari \n",
"```\n",
"\n",
"\n",
"```python\n",
"nlu.load('en.t5').predict('What is the most spoken language in India?')\n",
">>> Hindi\n",
"```\n",
"\n",
"\n",
"```python\n",
"nlu.load('en.t5').predict('What is the capital of Germany?')\n",
">>> Berlin\n",
"```\n",
"\n"
]
},
{
"cell_type": "code",
"metadata": {
"id": "s6p3BcAQYeBl"
},
"source": [
"import os\n",
"! apt-get update -qq > /dev/null \n",
"# Install java\n",
"! apt-get install -y openjdk-8-jdk-headless -qq > /dev/null\n",
"os.environ[\"JAVA_HOME\"] = \"/usr/lib/jvm/java-8-openjdk-amd64\"\n",
"os.environ[\"PATH\"] = os.environ[\"JAVA_HOME\"] + \"/bin:\" + os.environ[\"PATH\"]\n",
"! pip install nlu pyspark==2.4.7 > /dev/null \n",
"import nlu\n"
],
"execution_count": null,
"outputs": []
},
{
"cell_type": "markdown",
"metadata": {
"id": "CqI-ovPLjzH7"
},
"source": [
"# Closed book question answering example"
]
},
{
"cell_type": "code",
"metadata": {
"colab": {
"base_uri": "https://localhost:8080/"
},
"id": "FYZQHT4FYjlQ",
"outputId": "33390dc2-02f7-4f18-dd0f-2ffde860d787"
},
"source": [
"t5_closed_book = nlu.load('en.t5')"
],
"execution_count": null,
"outputs": [
{
"output_type": "stream",
"text": [
"google_t5_small_ssm_nq download started this may take some time.\n",
"Approximate size to download 139 MB\n",
"[OK!]\n"
],
"name": "stdout"
}
]
},
{
"cell_type": "code",
"metadata": {
"id": "uHK91QxwYn6y",
"colab": {
"base_uri": "https://localhost:8080/",
"height": 106
},
"outputId": "f8b3c8fe-1f6a-4482-ba46-c64a47cec5a5"
},
"source": [
"t5_closed_book.predict('What is the capital of Germany?')"
],
"execution_count": null,
"outputs": [
{
"output_type": "execute_result",
"data": {
"text/html": [
"
\n",
"\n",
"
\n",
" \n",
" \n",
" | \n",
" document | \n",
" T5 | \n",
"
\n",
" \n",
" origin_index | \n",
" | \n",
" | \n",
"
\n",
" \n",
" \n",
" \n",
" 0 | \n",
" What is the capital of Germany? | \n",
" Berlin | \n",
"
\n",
" \n",
"
\n",
"
"
],
"text/plain": [
" document T5\n",
"origin_index \n",
"0 What is the capital of Germany? Berlin"
]
},
"metadata": {
"tags": []
},
"execution_count": 3
}
]
},
{
"cell_type": "code",
"metadata": {
"id": "4IugHdKcZMTW",
"colab": {
"base_uri": "https://localhost:8080/",
"height": 106
},
"outputId": "18f851dc-036e-4cee-bd21-68e850856b85"
},
"source": [
"t5_closed_book.predict('Who is president of Nigeria?')"
],
"execution_count": null,
"outputs": [
{
"output_type": "execute_result",
"data": {
"text/html": [
"\n",
"\n",
"
\n",
" \n",
" \n",
" | \n",
" document | \n",
" T5 | \n",
"
\n",
" \n",
" origin_index | \n",
" | \n",
" | \n",
"
\n",
" \n",
" \n",
" \n",
" 0 | \n",
" Who is president of Nigeria? | \n",
" Muhammadu Buhari | \n",
"
\n",
" \n",
"
\n",
"
"
],
"text/plain": [
" document T5\n",
"origin_index \n",
"0 Who is president of Nigeria? Muhammadu Buhari"
]
},
"metadata": {
"tags": []
},
"execution_count": 4
}
]
},
{
"cell_type": "code",
"metadata": {
"id": "dZfMDsyXqvZ0",
"colab": {
"base_uri": "https://localhost:8080/",
"height": 106
},
"outputId": "813c4c72-747b-43f7-94ca-d6d421f92f29"
},
"source": [
"t5_closed_book.predict('What is the most spoken language in India?')\n"
],
"execution_count": null,
"outputs": [
{
"output_type": "execute_result",
"data": {
"text/html": [
"\n",
"\n",
"
\n",
" \n",
" \n",
" | \n",
" document | \n",
" T5 | \n",
"
\n",
" \n",
" origin_index | \n",
" | \n",
" | \n",
"
\n",
" \n",
" \n",
" \n",
" 0 | \n",
" What is the most spoken language in India? | \n",
" Hindi | \n",
"
\n",
" \n",
"
\n",
"
"
],
"text/plain": [
" document T5\n",
"origin_index \n",
"0 What is the most spoken language in India? Hindi"
]
},
"metadata": {
"tags": []
},
"execution_count": 5
}
]
},
{
"cell_type": "markdown",
"metadata": {
"id": "3Bu-Beo7ZNps"
},
"source": [
"# Open Book question examples\n",
"\n",
"**Your context must be prefixed with `context:`**\n"
]
},
{
"cell_type": "code",
"metadata": {
"id": "886fxf0iZO5A",
"colab": {
"base_uri": "https://localhost:8080/"
},
"outputId": "49df08e1-983d-4409-98dd-efe0e3fb6190"
},
"source": [
"t5_open_book = nlu.load('answer_question')\n"
],
"execution_count": null,
"outputs": [
{
"output_type": "stream",
"text": [
"t5_base download started this may take some time.\n",
"Approximate size to download 446 MB\n",
"[OK!]\n"
],
"name": "stdout"
}
]
},
{
"cell_type": "markdown",
"metadata": {
"id": "7SqPPK1mtmyz"
},
"source": [
"## The context : \n",
"`Ghenkis Khan recalled Subtai back to Mongolia soon afterwards, and Jebe died on the road back to Samarkand`"
]
},
{
"cell_type": "code",
"metadata": {
"id": "5koR8GOOZqUN",
"colab": {
"base_uri": "https://localhost:8080/",
"height": 106
},
"outputId": "204d5010-0fae-4b3a-8544-0d116b79e142"
},
"source": [
"t5_open_book.predict(\"\"\"Where did Jebe die?\n",
"context: Ghenkis Khan recalled Subtai back to Mongolia soon afterwards, and Jebe died on the road back to Samarkand\"\"\" )"
],
"execution_count": null,
"outputs": [
{
"output_type": "execute_result",
"data": {
"text/html": [
"\n",
"\n",
"
\n",
" \n",
" \n",
" | \n",
" document | \n",
" T5 | \n",
"
\n",
" \n",
" origin_index | \n",
" | \n",
" | \n",
"
\n",
" \n",
" \n",
" \n",
" 0 | \n",
" Where did Jebe die? context: Ghenkis Khan reca... | \n",
" Samarkand | \n",
"
\n",
" \n",
"
\n",
"
"
],
"text/plain": [
" document T5\n",
"origin_index \n",
"0 Where did Jebe die? context: Ghenkis Khan reca... Samarkand"
]
},
"metadata": {
"tags": []
},
"execution_count": 7
}
]
},
{
"cell_type": "markdown",
"metadata": {
"id": "-9wDlwfLigQl"
},
"source": [
"## Todo Tesla Bitcoin News article!?"
]
},
{
"cell_type": "markdown",
"metadata": {
"id": "-OlZcBoGjPTS"
},
"source": [
"## Open book question example on news article"
]
},
{
"cell_type": "code",
"metadata": {
"id": "JSSQz8jxa4Bg",
"colab": {
"base_uri": "https://localhost:8080/",
"height": 254
},
"outputId": "9f547e41-3639-4aff-e6a6-746e3362c756"
},
"source": [
"question1 = 'Who is Jack ma?'\n",
"question2 = 'Who is founder of Alibaba Group?'\n",
"question3 = 'When did Jack Ma re-appear?'\n",
"question4 = 'How did Alibaba stocks react?'\n",
"question5 = 'Whom did Jack Ma meet?'\n",
"question6 = 'Who did Jack Ma hide from?'\n",
"\n",
"\n",
"# from https://www.bbc.com/news/business-55728338 \n",
"news_article_snippet = \"\"\" context:\n",
"Alibaba Group founder Jack Ma has made his first appearance since Chinese regulators cracked down on his business empire.\n",
"His absence had fuelled speculation over his whereabouts amid increasing official scrutiny of his businesses.\n",
"The billionaire met 100 rural teachers in China via a video meeting on Wednesday, according to local government media.\n",
"Alibaba shares surged 5% on Hong Kong's stock exchange on the news.\n",
"\"\"\"\n",
"\n",
"questions = [\n",
" question1+ news_article_snippet,\n",
" question2+ news_article_snippet,\n",
" question3+ news_article_snippet,\n",
" question4+ news_article_snippet,\n",
" question5+ news_article_snippet,\n",
" question6+ news_article_snippet,]\n",
"\n",
"\n",
"\n",
"t5_open_book.predict(questions)\n"
],
"execution_count": null,
"outputs": [
{
"output_type": "execute_result",
"data": {
"text/html": [
"\n",
"\n",
"
\n",
" \n",
" \n",
" | \n",
" document | \n",
" T5 | \n",
"
\n",
" \n",
" origin_index | \n",
" | \n",
" | \n",
"
\n",
" \n",
" \n",
" \n",
" 0 | \n",
" Who is Jack ma? context: Alibaba Group founder... | \n",
" Alibaba Group founder | \n",
"
\n",
" \n",
" 1 | \n",
" Who is founder of Alibaba Group? context: Alib... | \n",
" Jack Ma | \n",
"
\n",
" \n",
" 2 | \n",
" When did Jack Ma re-appear? context: Alibaba G... | \n",
" Wednesday | \n",
"
\n",
" \n",
" 3 | \n",
" How did Alibaba stocks react? context: Alibaba... | \n",
" surged 5% | \n",
"
\n",
" \n",
" 4 | \n",
" Whom did Jack Ma meet? context: Alibaba Group ... | \n",
" 100 rural teachers | \n",
"
\n",
" \n",
" 5 | \n",
" Who did Jack Ma hide from? context: Alibaba Gr... | \n",
" Chinese regulators | \n",
"
\n",
" \n",
"
\n",
"
"
],
"text/plain": [
" document T5\n",
"origin_index \n",
"0 Who is Jack ma? context: Alibaba Group founder... Alibaba Group founder\n",
"1 Who is founder of Alibaba Group? context: Alib... Jack Ma\n",
"2 When did Jack Ma re-appear? context: Alibaba G... Wednesday\n",
"3 How did Alibaba stocks react? context: Alibaba... surged 5%\n",
"4 Whom did Jack Ma meet? context: Alibaba Group ... 100 rural teachers\n",
"5 Who did Jack Ma hide from? context: Alibaba Gr... Chinese regulators"
]
},
"metadata": {
"tags": []
},
"execution_count": 8
}
]
},
{
"cell_type": "code",
"metadata": {
"id": "vlpHM1m8ixDL",
"colab": {
"base_uri": "https://localhost:8080/",
"height": 106
},
"outputId": "6e7b7334-3fe8-48e2-d777-e7ffcb431a74"
},
"source": [
"\n",
"\n",
"# define Data, add additional context tag between sentence\n",
"question ='''\n",
"What does increased oxygen concentrations in the patient’s lungs displace? \n",
"context: Hyperbaric (high-pressure) medicine uses special oxygen \n",
"chambers to increase the partial pressure of O 2 around the patient and,\n",
" when needed, the medical staff. Carbon monoxide poisoning, gas gangrene, \n",
" and decompression sickness (the ’bends’) are sometimes treated using these devices.\n",
" Increased O 2 concentration in the lungs helps to displace carbon monoxide from the\n",
" heme group of hemoglobin. Oxygen gas is poisonous to the anaerobic bacteria that cause gas gangrene, so increasing its partial pressure helps kill them. Decompression sickness occurs in divers who decompress too quickly after a dive, resulting in bubbles of inert gas, mostly nitrogen and helium, forming in their blood. Increasing the pressure of O 2 as soon as possible is part of the treatment.\n",
"'''\n",
"\n",
"\n",
"#Predict on text data with T5\n",
"t5_open_book.predict(question)"
],
"execution_count": null,
"outputs": [
{
"output_type": "execute_result",
"data": {
"text/html": [
"\n",
"\n",
"
\n",
" \n",
" \n",
" | \n",
" document | \n",
" T5 | \n",
"
\n",
" \n",
" origin_index | \n",
" | \n",
" | \n",
"
\n",
" \n",
" \n",
" \n",
" 0 | \n",
" What does increased oxygen concentrations in t... | \n",
" carbon monoxide | \n",
"
\n",
" \n",
"
\n",
"
"
],
"text/plain": [
" document T5\n",
"origin_index \n",
"0 What does increased oxygen concentrations in t... carbon monoxide"
]
},
"metadata": {
"tags": []
},
"execution_count": 9
}
]
},
{
"cell_type": "markdown",
"metadata": {
"id": "jbmPc4o5iSS-"
},
"source": [
"# Summarize"
]
},
{
"cell_type": "code",
"metadata": {
"colab": {
"base_uri": "https://localhost:8080/"
},
"id": "gHgQpl1Jc07e",
"outputId": "fa9b17a7-a466-4ced-b945-a11be7f835f2"
},
"source": [
"t5_sum = nlu.load('en.t5.base')"
],
"execution_count": null,
"outputs": [
{
"output_type": "stream",
"text": [
"t5_base download started this may take some time.\n",
"Approximate size to download 446 MB\n",
"[OK!]\n"
],
"name": "stdout"
}
]
},
{
"cell_type": "code",
"metadata": {
"colab": {
"base_uri": "https://localhost:8080/",
"height": 136
},
"id": "zj-FbNaScofh",
"outputId": "681b92d0-ae5b-441b-df3a-58caf88f220b"
},
"source": [
"# Set the task on T5\n",
"t5_sum['t5'].setTask('summarize ') \n",
"\n",
"\n",
"\n",
"# define Data, add additional tags between sentences\n",
"data = [\n",
" '''\n",
" The belgian duo took to the dance floor on monday night with some friends . manchester united face newcastle in the premier league on wednesday . red devils will be looking for just their second league away win in seven . louis van gaal’s side currently sit two points clear of liverpool in fourth .\n",
" ''',\n",
" ''' Calculus, originally called infinitesimal calculus or \"the calculus of infinitesimals\", is the mathematical study of continuous change, in the same way that geometry is the study of shape and algebra is the study of generalizations of arithmetic operations. It has two major branches, differential calculus and integral calculus; the former concerns instantaneous rates of change, and the slopes of curves, while integral calculus concerns accumulation of quantities, and areas under or between curves. These two branches are related to each other by the fundamental theorem of calculus, and they make use of the fundamental notions of convergence of infinite sequences and infinite series to a well-defined limit.[1] Infinitesimal calculus was developed independently in the late 17th century by Isaac Newton and Gottfried Wilhelm Leibniz.[2][3] Today, calculus has widespread uses in science, engineering, and economics.[4] In mathematics education, calculus denotes courses of elementary mathematical analysis, which are mainly devoted to the study of functions and limits. The word calculus (plural calculi) is a Latin word, meaning originally \"small pebble\" (this meaning is kept in medicine – see Calculus (medicine)). Because such pebbles were used for calculation, the meaning of the word has evolved and today usually means a method of computation. It is therefore used for naming specific methods of calculation and related theories, such as propositional calculus, Ricci calculus, calculus of variations, lambda calculus, and process calculus.'''\n",
" ]\n",
"\n",
"\n",
"#Predict on text data with T5"
],
"execution_count": null,
"outputs": [
{
"output_type": "execute_result",
"data": {
"text/html": [
"\n",
"\n",
"
\n",
" \n",
" \n",
" | \n",
" document | \n",
" T5 | \n",
"
\n",
" \n",
" origin_index | \n",
" | \n",
" | \n",
"
\n",
" \n",
" \n",
" \n",
" 0 | \n",
" The belgian duo took to the dance floor on mon... | \n",
" manchester united face newcastle in the premie... | \n",
"
\n",
" \n",
" 1 | \n",
" Calculus, originally called infinitesimal calc... | \n",
" calculus, originally called infinitesimal calc... | \n",
"
\n",
" \n",
"
\n",
"
"
],
"text/plain": [
" document T5\n",
"origin_index \n",
"0 The belgian duo took to the dance floor on mon... manchester united face newcastle in the premie...\n",
"1 Calculus, originally called infinitesimal calc... calculus, originally called infinitesimal calc..."
]
},
"metadata": {
"tags": []
},
"execution_count": 16
}
]
},
{
"cell_type": "code",
"metadata": {
"colab": {
"base_uri": "https://localhost:8080/",
"height": 106
},
"id": "UH7PqsbPfLem",
"outputId": "95882722-fd21-4b9e-ab82-4bb5a719313e"
},
"source": [
"text = \"\"\"(Reuters) - Mastercard Inc said on Wednesday it was planning to offer support for some cryptocurrencies on its network this year, joining a string of big-ticket firms that have pledged similar support.\n",
"\n",
"The credit-card giant’s announcement comes days after Elon Musk’s Tesla Inc revealed it had purchased $1.5 billion of bitcoin and would soon accept it as a form of payment.\n",
"\n",
"Asset manager BlackRock Inc and payments companies Square and PayPal have also recently backed cryptocurrencies.\n",
"\n",
"Mastercard already offers customers cards that allow people to transact using their cryptocurrencies, although without going through its network.\n",
"\n",
"\"Doing this work will create a lot more possibilities for shoppers and merchants, allowing them to transact in an entirely new form of payment. This change may open merchants up to new customers who are already flocking to digital assets,\" Mastercard said. (mstr.cd/3tLaPZM)\n",
"\n",
"Mastercard specified that not all cryptocurrencies will be supported on its network, adding that many of the hundreds of digital assets in circulation still need to tighten their compliance measures.\n",
"\n",
"Many cryptocurrencies have struggled to win the trust of mainstream investors and the general public due to their speculative nature and potential for money laundering.\n",
"\"\"\"\n",
"short = t5_sum.predict(text)\n",
"short"
],
"execution_count": null,
"outputs": [
{
"output_type": "execute_result",
"data": {
"text/html": [
"\n",
"\n",
"
\n",
" \n",
" \n",
" | \n",
" document | \n",
" T5 | \n",
"
\n",
" \n",
" origin_index | \n",
" | \n",
" | \n",
"
\n",
" \n",
" \n",
" \n",
" 0 | \n",
" (Reuters) - Mastercard Inc said on Wednesday i... | \n",
" mastercard said on Wednesday it was planning t... | \n",
"
\n",
" \n",
"
\n",
"
"
],
"text/plain": [
" document T5\n",
"origin_index \n",
"0 (Reuters) - Mastercard Inc said on Wednesday i... mastercard said on Wednesday it was planning t..."
]
},
"metadata": {
"tags": []
},
"execution_count": 19
}
]
},
{
"cell_type": "code",
"metadata": {
"colab": {
"base_uri": "https://localhost:8080/",
"height": 69
},
"id": "ActCNiVClRcT",
"outputId": "91dbd046-29c7-4de8-e2ea-b19dff282dd3"
},
"source": [
"short.T5.iloc[0]"
],
"execution_count": null,
"outputs": [
{
"output_type": "execute_result",
"data": {
"application/vnd.google.colaboratory.intrinsic+json": {
"type": "string"
},
"text/plain": [
"'mastercard said on Wednesday it was planning to offer support for some cryptocurrencies on its network this year . the credit-card giant’s announcement comes days after Elon Musk’s Tesla Inc revealed it had purchased $1.5 billion of bitcoin . asset manager blackrock and payments companies Square and PayPal have also recently backed cryptocurrencies .'"
]
},
"metadata": {
"tags": []
},
"execution_count": 23
}
]
},
{
"cell_type": "code",
"metadata": {
"colab": {
"base_uri": "https://localhost:8080/"
},
"id": "vIDmj_JkmPmd",
"outputId": "ccdad8c7-a4e8-45e3-846d-2d32682c8063"
},
"source": [
"len('mastercard said on Wednesday it was planning to offer support for some cryptocurrencies on its network this year . the credit-card giant’s announcement comes days after Elon Musk’s Tesla Inc revealed it had purchased $1.5 billion of bitcoin . asset manager blackrock and payments companies Square and PayPal have also recently backed cryptocurrencies .')"
],
"execution_count": null,
"outputs": [
{
"output_type": "execute_result",
"data": {
"text/plain": [
"352"
]
},
"metadata": {
"tags": []
},
"execution_count": 24
}
]
},
{
"cell_type": "code",
"metadata": {
"colab": {
"base_uri": "https://localhost:8080/"
},
"id": "geQxsepZmTbS",
"outputId": "6728fb43-35f3-413e-811d-622e11b614f9"
},
"source": [
"len(text)"
],
"execution_count": null,
"outputs": [
{
"output_type": "execute_result",
"data": {
"text/plain": [
"1284"
]
},
"metadata": {
"tags": []
},
"execution_count": 25
}
]
},
{
"cell_type": "code",
"metadata": {
"id": "gkepJVvQiCod"
},
"source": [
""
],
"execution_count": null,
"outputs": []
}
]
}