{
"cells": [
{
"cell_type": "markdown",
"metadata": {},
"source": [
"# **An Introduction Into Python**\n",
"\n",
"---"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Table of contents:\n",
"1. [Environment Setup](#Environment-Setup)\n",
"1. [Why Python?](#Why-Python?)\n",
"1. [Syntax](#Syntax)\n",
"1. [Variables and Datatypes](#Variables-and-Datatypes)\n",
"1. [Operators](#Operators)\n",
"1. [Collection Data-Types](#Collection-Data-Types)\n",
"1. [The if statement](#The-if-statement)\n",
"1. [For Loops](#For-Loops)\n",
"1. [Nested Loops](#Nested-Loops)\n",
"1. [While Loops](#While-Loops)\n",
"1. [continue and break](#continue-and-break)"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"## Environment Setup"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"The most recent major version of Python is Python 3, which we shall be using in this tutorial. However, Python 2, although not being updated with anything other than security updates, is still quite popular.\n",
"\n",
"[The Official Python Website](https://www.python.org/about/)"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"[Download Python](https://www.python.org/downloads/)"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"With over 6 million users, the open source [Anaconda Distribution](https://www.anaconda.com/download/) is the fastest and easiest way to do Python and R data science and machine learning on Linux, Windows, and Mac OS X. It's the industry standard for developing, testing, and training on a single machine."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Anaconda gives you preinstalled tools such as [Jupyter Notebooks](https://jupyter.org/), [Spyder](https://www.spyder-ide.org/) and [RStudio](https://www.rstudio.com/). It is the one thing you will need to set up your development environment."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Please follow this tutorial to install Anaconda : [Installing Anaconda on Windows](https://www.datacamp.com/community/tutorials/installing-anaconda-windows)"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"## Why Python?"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"- Python is an easy to learn, easy to use programing language.\n",
"- It is intuitive, a lot of the syntax looks like English Language.\n",
"- No data type declaration for a variable.\n",
"- Support for inbuilt structures like Lists and Dictionaries make it great to work with.\n",
"- Most ML libraries are available in Python.\n",
"- More reasons will become clearer as you learn about Python.\n"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Suppose we were to write a program to read 10 strings, and merge the ones that started with a \"Hi\". How big would the program be?"
]
},
{
"cell_type": "code",
"execution_count": 1,
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"Hey\n",
"Hi\n",
"Hiya\n",
"Hira\n",
"h\n",
"f\n",
"s\n",
"w\n",
"e\n",
"r\n",
"HiHiyaHira\n"
]
}
],
"source": [
"inp_list = [input() for i in range(10)]\n",
"inp_list_2 = [i for i in inp_list if str.lower(i[:2])==\"hi\"]\n",
"print(\"\".join(inp_list_2))"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"See how easy this is? Note that the syntax is pretty intuitive as well.\n",
"\n",
"Don't worry if you can't understand anything here, soon you will be able to follow along as well. Let's dive into the basics."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"### How To Launch Python"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"- We can launch Python by opening terminal and typing \"python3\". This opens up an interactive terminal, where you can type the commands.\n",
"\n",
"- Another option is to open up a text editor, type the program, save the file with a '.py' extension (instead of .docx or .txt). The name of the file will be prog_name.py, so to execute it, we go to terminal and type \"python3 prog_name.py\"."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"### Errors"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Errors are key to identifying the mistakes in your program. They will show up for various reasons like syntax errors and logical errors."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"In languages like C, errors in syntax are brought up whether or not those statements are executed ([conditional statements](#The-if-statement)). In Python, unless a particular block of code is executed, even syntax errors will not show up for that block of code.\n",
"\n",
"This may seem counterproductive, but as you will later see, it is the basis for how Python functions."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"# Let's Begin!\n",
"---"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"## Syntax"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Syntax is nothing but the format you need to follow. They are the rules you need to adhere to."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"The legendary Hello World is here."
]
},
{
"cell_type": "code",
"execution_count": 2,
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"Hello World!\n"
]
}
],
"source": [
"print(\"Hello World!\")"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"To print something, we write print followed by the thing we want to."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"We enclose strings (i.e. text) in quotes. In python, we can use single quotes (' '), double quotes(\" \") or triple quotes(''' ''') to do so. Triple quotes is an exclusive Python added functionality."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"- Python is Case-Sensitive."
]
},
{
"cell_type": "code",
"execution_count": 3,
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"5\n",
"10\n"
]
}
],
"source": [
"a = 5\n",
"A = 10\n",
"print(a)\n",
"print(A)"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"**Did you notice** - *There's no semi-colon!* Python statements do not require an ending semi-colon."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"P.S.: Semicolons can be used though, if you have multiple statements on one line."
]
},
{
"cell_type": "code",
"execution_count": 4,
"metadata": {},
"outputs": [],
"source": [
"a=6;A=10"
]
},
{
"cell_type": "code",
"execution_count": 5,
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"6\n",
"10\n"
]
}
],
"source": [
"print(a)\n",
"print(A)"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"What are variables? These are containers to store temporary values. Anything you need to store, so that you can access it later in the program, is done by using a variable.\n",
"\n",
"In the above code segment, *a* and *A* are variables."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"A few syntax rules for variables are listed below:\n",
"- Variables names must start with a letter or an underscore\n",
"- The remainder of your variable name may consist of letters, numbers and underscores\n",
"- Variable names cannot be keywords.\n",
"- As mentioned earlier, Python is case sensitive.\n",
"\n",
"#### What are keywords?\n",
"Words that have a predefined meaning in the language, like print, input, list, str, int, and a lot more. \n",
"As and when you find out more about the language, you will know which words add to this list."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"*Other important syntax rules will be covered as and when we encounter the relevant concepts.*"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"## Variables and Datatypes"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"As we saw earlier, a variable is used to store a value in memory. Just like we need to remember things before an exam in order to be able to answer questions, a computer needs to store values in order to work with them.\n",
"\n",
"The concept of datatypes is crucial to programming.\n",
"\n",
"*What is a datatype?*\n",
"\n",
"It is used by languages to ensure that operations on these values are legitimate. And also because adding 2 integers is different to adding 2 strings. The process is different altogether.\n",
"\n",
"*What is a legitimate operation?*\n",
"\n",
"Consider you want to add 2 things. The only things that we consider adding are numbers, right? We can't add a character and a number. So, to ensure that what things we want to do actually make sense, programming languages use the concept of datatypes. Thus errors are detected easily. \n",
"\n",
"Most languages require you to declare what kind of value will a given variable store.\n",
"\n",
"*The kind of values that can be stored can be categorized into these broad headings:*\n",
"- Numerical\n",
"- Textual\n",
"- Boolean (True/False)\n",
"- There sometimes may be further categorization as well."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"The most annoying thing that other languages like C have is datatype declaration in the code for a variable. Once a variable is of a particular type, it can't be changed to store another type. **Python does not require this!**"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Does this mean the problem of checking datatypes does not exist in Python? **No.** Instead, Python does this checking just before execution.\n",
"\n",
"*How?*\n",
"\n",
"Python does not only store the variable value, but it also stores the type of the value stored with it."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"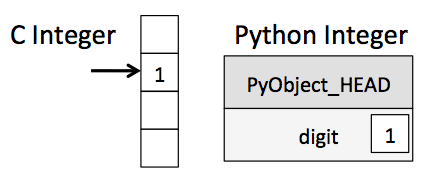"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Thus, we generally do *NOT* need to care about variable type, as we can reassign a variable which held an integer to now hold a string."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Now, for some examples on using variables."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Tell me which of the following are valid variable names:\n",
"\n",
"```python\n",
"my_var_1 = 10\n",
"my-var-1 = 10\n",
"print = 200\n",
"```"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"The first one is right.\n",
"\n",
"The second one uses a **'-'**, which is not allowed.\n",
"\n",
"The third one tries to assign a value to a keyword, which is also not allowed."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Let's dive into Datatypes now, as it will help give you some clarity into what is all this Integer Float Double and whatnot."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"#### The type() function gives us the datatype of a variable. We will look at functions in the next lecture."
]
},
{
"cell_type": "code",
"execution_count": 6,
"metadata": {},
"outputs": [],
"source": [
"an_int = 10"
]
},
{
"cell_type": "code",
"execution_count": 7,
"metadata": {},
"outputs": [
{
"data": {
"text/plain": [
"int"
]
},
"execution_count": 7,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"type(an_int)"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"An integer is used to store basic integer values (the mathematical set of integers, I/Z). "
]
},
{
"cell_type": "code",
"execution_count": 8,
"metadata": {},
"outputs": [],
"source": [
"a_float = 10.0"
]
},
{
"cell_type": "code",
"execution_count": 9,
"metadata": {},
"outputs": [
{
"data": {
"text/plain": [
"float"
]
},
"execution_count": 9,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"type(a_float)"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"A float is used to store decimal values(also called floating point numbers)."
]
},
{
"cell_type": "code",
"execution_count": 10,
"metadata": {},
"outputs": [],
"source": [
"an_str = 'a'"
]
},
{
"cell_type": "code",
"execution_count": 11,
"metadata": {},
"outputs": [
{
"data": {
"text/plain": [
"str"
]
},
"execution_count": 11,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"type(an_str)"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"A string can store text. It can consist of anything, we will look at them in depth [later](#Strings)."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"To take input from the user, we use input(). This returns the user input in the form of a string, we can store it in a variable."
]
},
{
"cell_type": "code",
"execution_count": 12,
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"Enter something\n",
"\n"
]
}
],
"source": [
"print(\"Enter something\")\n",
"inp = input()"
]
},
{
"cell_type": "code",
"execution_count": 13,
"metadata": {},
"outputs": [
{
"data": {
"text/plain": [
"str"
]
},
"execution_count": 13,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"type(inp)"
]
},
{
"cell_type": "code",
"execution_count": 14,
"metadata": {},
"outputs": [],
"source": [
"a_complex = 1+3j"
]
},
{
"cell_type": "code",
"execution_count": 15,
"metadata": {},
"outputs": [
{
"data": {
"text/plain": [
"complex"
]
},
"execution_count": 15,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"type(a_complex)"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Python provides in-built support for the mathematical concept of a complex number.\n",
"\n",
"The format is a+bj, where a and b are real values and j is a character which is essential and non-replacable. It differentiates a complex number from a real number or from looking like an addition operation.\n",
"\n",
"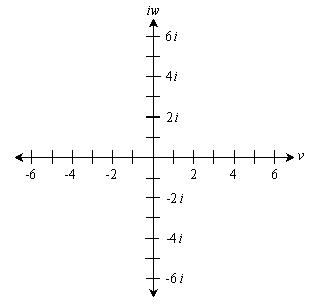\n",
"\n",
"\n",
"If we want to store an imaginary number that lies on the real axis, we can use code like so:\n",
"```python\n",
"a_complex = 2+0j\n",
"```\n"
]
},
{
"cell_type": "code",
"execution_count": 16,
"metadata": {},
"outputs": [],
"source": [
"a_boolean = True"
]
},
{
"cell_type": "code",
"execution_count": 17,
"metadata": {},
"outputs": [
{
"data": {
"text/plain": [
"bool"
]
},
"execution_count": 17,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"type(a_boolean)"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"A boolean is the most basic of datatypes, which only has 2 values: True or False."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"A very key concept in Python is that we can always use the same variable to store different types. Also, we may not know what type of data will the variable store, and the program will run smoothly unless you try to perform an illegitimate operation like add an integer and a string."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Now, let's move on to operators."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"## Operators"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"The base of programming lies in performing mathematical operations by using operators. We'll go over them one-by-one."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"- Arithmetic Operator\n",
"- Relational Operator\n",
"- Assignment Operator\n",
"- Logical Operator\n",
"- Membership Operator\n",
"- Identity Operator\n",
"- Bitwise Operator"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"---\n",
"Arithmetic Operators are used to perform arithmetic operations on variables. The major ones are listed below:"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"The Basic Ones:\n",
"```python\n",
"Addition = 3 + 4\n",
"Subtraction = 3 - 4\n",
"Multiplication = 3 * 4\n",
"Division = 3 / 4\n",
"Modulo = 3 % 4 #This gives the remainder of division\n",
"```"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"To perform $$x^y$$Python provides an operator."
]
},
{
"cell_type": "code",
"execution_count": 18,
"metadata": {},
"outputs": [
{
"data": {
"text/plain": [
"81"
]
},
"execution_count": 18,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"3**4"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"We can also perform division and truncate the part after the decimal to get an integer result instead of a float (NOT rounding off) using 1 operator."
]
},
{
"cell_type": "code",
"execution_count": 19,
"metadata": {},
"outputs": [
{
"data": {
"text/plain": [
"0"
]
},
"execution_count": 19,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"3//4"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"---\n",
"Relational Operators are used to perform comparisons, they always return boolean values."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"The Basic Ones:\n",
"```python\n",
"Lesser than = 3 < 4\n",
"Lesser than or equal to = 3 <= 4\n",
"Greater than = 3 > 4\n",
"greater than or equal to = 3 >= 4\n",
"```"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"To check for equality, we use =="
]
},
{
"cell_type": "code",
"execution_count": 20,
"metadata": {},
"outputs": [
{
"data": {
"text/plain": [
"False"
]
},
"execution_count": 20,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"3==4"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"*Note: a=b is an assignment operation, while a==b is to check wheter a is equal to b*"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"To check for inequality, we use !="
]
},
{
"cell_type": "code",
"execution_count": 21,
"metadata": {},
"outputs": [
{
"data": {
"text/plain": [
"True"
]
},
"execution_count": 21,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"3!=4"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"---\n",
"Assignment Operators and Shorthand Notation\n",
"\n",
"The statement\n",
"```python\n",
"a = 4\n",
"```\n",
"assigns a with the value 4. a is now a variable."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"a+=b is a shorthand for a = a + b.\n",
"\n",
"Similarly, a-=b, a*=b, a/=b, a**=b,a%=b,a//=b exist."
]
},
{
"cell_type": "code",
"execution_count": 22,
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"9\n"
]
}
],
"source": [
"a = 5\n",
"a+=4\n",
"print(a)"
]
},
{
"cell_type": "code",
"execution_count": 23,
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"1\n"
]
}
],
"source": [
"a = 5\n",
"a-=4\n",
"print(a)"
]
},
{
"cell_type": "code",
"execution_count": 24,
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"20\n"
]
}
],
"source": [
"a = 5\n",
"a*=4\n",
"print(a)"
]
},
{
"cell_type": "code",
"execution_count": 25,
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"1.25\n"
]
}
],
"source": [
"a = 5\n",
"a/=4\n",
"print(a)"
]
},
{
"cell_type": "code",
"execution_count": 26,
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"1\n"
]
}
],
"source": [
"a = 5\n",
"a%=4\n",
"print(a)"
]
},
{
"cell_type": "code",
"execution_count": 27,
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"625\n"
]
}
],
"source": [
"a = 5\n",
"a**=4\n",
"print(a)"
]
},
{
"cell_type": "code",
"execution_count": 28,
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"1\n"
]
}
],
"source": [
"a = 5\n",
"a//=4\n",
"print(a)"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"---\n",
"Logical Operators are used on boolean values. They are used to operate boolean logic."
]
},
{
"cell_type": "code",
"execution_count": 29,
"metadata": {},
"outputs": [
{
"data": {
"text/plain": [
"False"
]
},
"execution_count": 29,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"True and False"
]
},
{
"cell_type": "code",
"execution_count": 30,
"metadata": {},
"outputs": [
{
"data": {
"text/plain": [
"True"
]
},
"execution_count": 30,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"True or False"
]
},
{
"cell_type": "code",
"execution_count": 31,
"metadata": {},
"outputs": [
{
"data": {
"text/plain": [
"False"
]
},
"execution_count": 31,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"not True"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"---\n",
"Membership Operator will be covered in the next lecture.\n",
"\n",
"Identity Operator will be covered later."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"---\n",
"Bitwise Operators are used to perform binary operations on numeric values.\n",
"\n",
"Numbers are stored in binary format, hence bitwise operations can sometimes be useful."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"& performs bit by bit AND operation of 2 numbers."
]
},
{
"cell_type": "code",
"execution_count": 32,
"metadata": {},
"outputs": [
{
"data": {
"text/plain": [
"0"
]
},
"execution_count": 32,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"0&1"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"| performs bit by bit OR operation of 2 numbers."
]
},
{
"cell_type": "code",
"execution_count": 33,
"metadata": {},
"outputs": [
{
"data": {
"text/plain": [
"1"
]
},
"execution_count": 33,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"0|1"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"^ performs bit by bit XOR operation of 2 numbers."
]
},
{
"cell_type": "code",
"execution_count": 34,
"metadata": {},
"outputs": [
{
"data": {
"text/plain": [
"1"
]
},
"execution_count": 34,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"0^1"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"~ performs bit by bit 1's complement operation of a number."
]
},
{
"cell_type": "code",
"execution_count": 35,
"metadata": {},
"outputs": [
{
"data": {
"text/plain": [
"-2"
]
},
"execution_count": 35,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"~1"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"<< performs a left shift operation on a number."
]
},
{
"cell_type": "code",
"execution_count": 36,
"metadata": {},
"outputs": [
{
"data": {
"text/plain": [
"16"
]
},
"execution_count": 36,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"4<<2"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"\\>\\> performs a right shift operation on a number."
]
},
{
"cell_type": "code",
"execution_count": 37,
"metadata": {},
"outputs": [
{
"data": {
"text/plain": [
"1"
]
},
"execution_count": 37,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"4>>2"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Now, we move on to a few advanced Python data structures, which give Python the power it has."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"## Collection Data-Types"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"So far we have seen built-in types like: *int, float* and *bool*. int, float, and bool are considered to be simple or primitive data types because their values are not composed of any smaller parts. **They cannot be broken down.**\n",
"\n",
"On the other hand, *strings, lists, sets, dictionaries* and *tuples* are different from the others because they are made up of smaller pieces. In the case of strings, they are made up of smaller strings each containing one character.\n",
"\n",
"**Types that are comprised of smaller pieces are called collection data types.** Depending on what we are doing, we may want to treat a collection data type as a single entity (the whole), or we may want to access its parts. This ambiguity is useful."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"### Strings"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Strings are a collection of characters. They can store a line of text. Strings are arrays of bytes representing Unicode characters.\n",
"\n",
"Interestingly enough, Python does not have a character datatype. It stores characters as a string of length 1.\n",
"\n",
"Strings can be created by using:\n",
"- Single Quotes(' ')\n",
"- Double Quotes(\" \")\n",
"- Triple Quotes(''' ''')\n",
"\n",
"Why are there 3 ways? To answer that, let's look at another question: If we want to store a sentence containing single quotes and/or double quotes, how do we do that?\n",
"\n",
"The answer is we can use triple quotes to define the start and end of the string.\n",
"\n",
"But what is we want to use triple quotes as well? \n",
"\n",
"Thus we have something called an *Escape sequence.*\n",
"\n",
"For characters that have special meaning in a string, we can use an escape sequence, to render that character in the string.\n",
"\n",
"Didn't get a word I'm saying? I'll give you an example."
]
},
{
"cell_type": "code",
"execution_count": 38,
"metadata": {},
"outputs": [],
"source": [
"str_1 = \"Hi! 'I'm Here\""
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"This is valid"
]
},
{
"cell_type": "code",
"execution_count": 39,
"metadata": {},
"outputs": [],
"source": [
"str_2 = ''' Hi! \"Hello I'm here ''' "
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"This is valid as well."
]
},
{
"cell_type": "code",
"execution_count": 40,
"metadata": {},
"outputs": [
{
"ename": "SyntaxError",
"evalue": "invalid syntax (, line 1)",
"output_type": "error",
"traceback": [
"\u001b[1;36m File \u001b[1;32m\"\"\u001b[1;36m, line \u001b[1;32m1\u001b[0m\n\u001b[1;33m str_3 = ''' Hello! I'm \"Happy!\" These are triple ''' quotes'''\u001b[0m\n\u001b[1;37m ^\u001b[0m\n\u001b[1;31mSyntaxError\u001b[0m\u001b[1;31m:\u001b[0m invalid syntax\n"
]
}
],
"source": [
"str_3 = ''' Hello! I'm \"Happy!\" These are triple ''' quotes''' "
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"This is not valid."
]
},
{
"cell_type": "code",
"execution_count": 41,
"metadata": {},
"outputs": [],
"source": [
"str_3 = 'Hello! I\\'m \"Happy!\" These are triple \\'\\'\\' quotes'"
]
},
{
"cell_type": "code",
"execution_count": 42,
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"Hello! I'm \"Happy!\" These are triple ''' quotes\n"
]
}
],
"source": [
"print(str_3)"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Here, notice how single quotes preceded by a backslash(\\\\) are rendered as a single quote and do not cause the string to end prematurely."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"A few escape characters are mentioned below:\n",
"- Single Quote: \\'\n",
"- Double Quote: \\\"\n",
"- Backslash: \\\\\\"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"#### Indexing a String"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Since a string is a collection of characters, we can access characters individually as well from a string. To do so, we use the following syntax:\n",
"```python\n",
"str_1 = \"Hello\"\n",
"print(str_1[1])\n",
"```\n",
"This will output 'e'.\n",
"\n",
"Indexes in Python start at 0. Thus, for str_1,\n",
"- H is stored at index 0\n",
"- e is stored at index 1\n",
"- l is stored at index 2\n",
"- l is stored at index 3\n",
"- o is stored at index 4"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"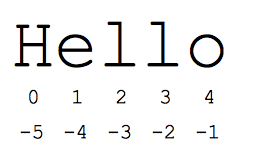"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Python supports negative indexing, that is the last character is stored at index -1, second last at -2 and so on."
]
},
{
"cell_type": "code",
"execution_count": 43,
"metadata": {},
"outputs": [],
"source": [
"str_1 = \"Hey! Hello! Hi!\""
]
},
{
"cell_type": "code",
"execution_count": 44,
"metadata": {},
"outputs": [],
"source": [
"str_1 = \"Hello\""
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"The value inside the square brackets is the index whose value you want."
]
},
{
"cell_type": "code",
"execution_count": 45,
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"e\n"
]
}
],
"source": [
"print(str_1[1])"
]
},
{
"cell_type": "code",
"execution_count": 46,
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"o\n"
]
}
],
"source": [
"print(str_1[-1])"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"We can get multiple items from a string (slicing) by using a semi-colon. The emi-colon can be thought of as meaning 'everything'.\n",
"\n",
"- If it is preceded by an integer, it means everything from the element at that index.\n",
"```python\n",
"str_1[2:]\n",
"```\n",
"\n",
"- If it is followed by an integer, it means everything upto but not including the element at that index.\n",
"```python\n",
"str_1[:4]\n",
"```\n",
"\n",
"- If it has both the above, it returns a slice of the string, starting at the element at the index preceding it, until the index following it.\n",
"```python\n",
"str_1[2:4]\n",
"```"
]
},
{
"cell_type": "code",
"execution_count": 47,
"metadata": {},
"outputs": [
{
"data": {
"text/plain": [
"'llo'"
]
},
"execution_count": 47,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"str_1[2:]"
]
},
{
"cell_type": "code",
"execution_count": 48,
"metadata": {},
"outputs": [
{
"data": {
"text/plain": [
"'Hello'"
]
},
"execution_count": 48,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"str_1[:5]"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"### Lists"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"The array substitute in Python with some added functionality is a List. A list is a type of container, which is used to store multiple data items at the same time. It is ordered (i.e. it can be indexed).\n",
"\n",
"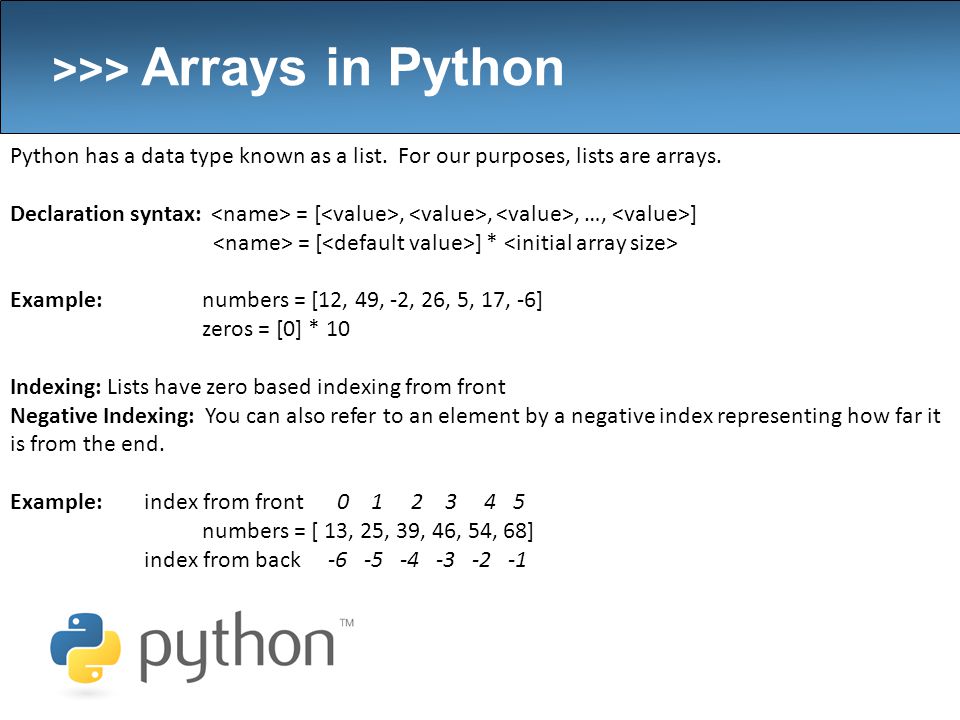"
]
},
{
"cell_type": "code",
"execution_count": 49,
"metadata": {},
"outputs": [],
"source": [
"lst = [2,3,'a','b',5.6,True, 1+3j]"
]
},
{
"cell_type": "code",
"execution_count": 50,
"metadata": {},
"outputs": [
{
"data": {
"text/plain": [
"list"
]
},
"execution_count": 50,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"type(lst)"
]
},
{
"cell_type": "code",
"execution_count": 51,
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"[2, 3, 'a', 'b', 5.6, True, (1+3j)]\n"
]
}
],
"source": [
"print(lst)"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"It can also contain another list within itself. This is how higher dimensional arrays can be stored."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Indexing is the same in lists as it is in strings. For a 2-D array (list of lists), we have to use indexing as shown below."
]
},
{
"cell_type": "code",
"execution_count": 52,
"metadata": {},
"outputs": [
{
"data": {
"text/plain": [
"0"
]
},
"execution_count": 52,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"twod_arr = [[0,1],[2,3]]\n",
"twod_arr[0][0]"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"### Dictionaries"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"A dictionary stores pairs of key-value pairs. For those of you who know hashing, dictionaries are nothing but an implementation of a hashing function."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Basically, in lists, we used default indexes(0,1,2,etc). Here, we can define custom indices. They are known as keys. They can then store corresponding values. They are implemented using hash functions."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"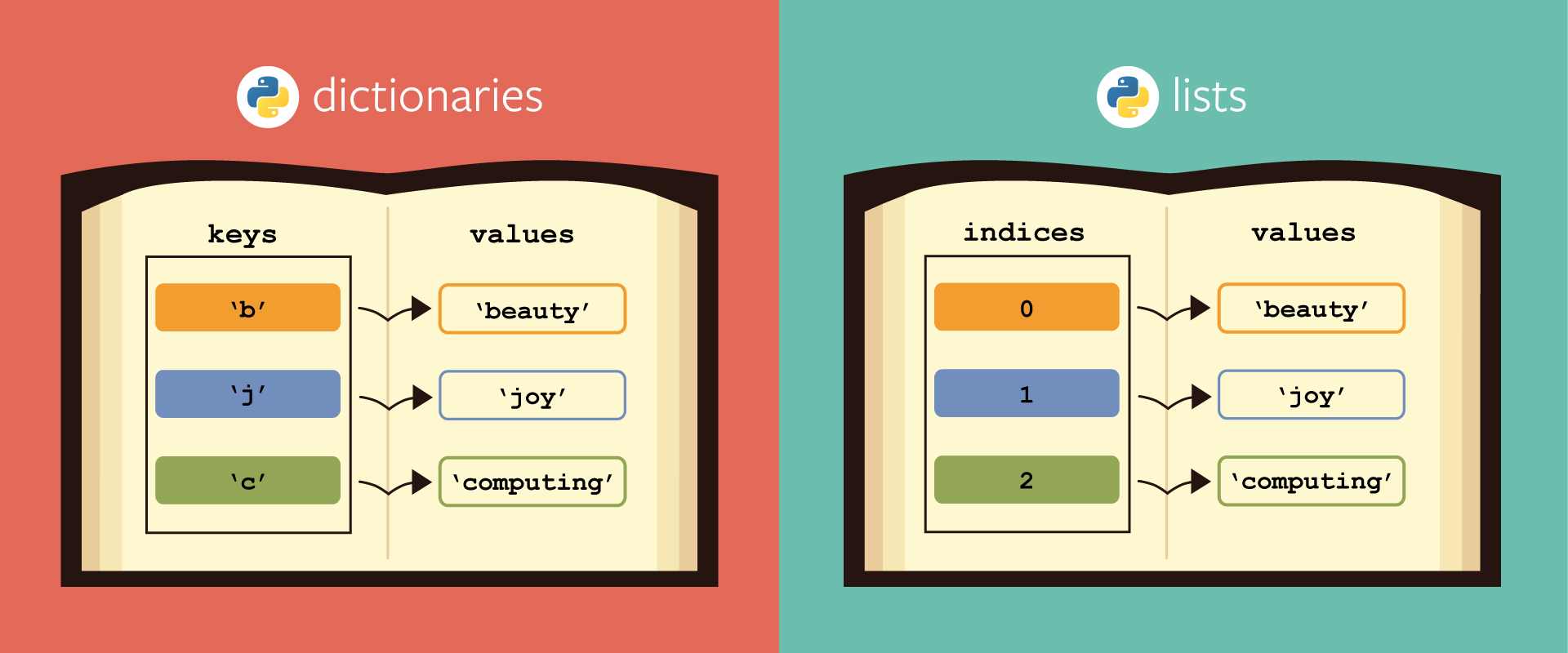"
]
},
{
"cell_type": "code",
"execution_count": 53,
"metadata": {},
"outputs": [],
"source": [
"my_dict = {'b':'beauty','j':\"joy\"}"
]
},
{
"cell_type": "code",
"execution_count": 54,
"metadata": {},
"outputs": [
{
"data": {
"text/plain": [
"'beauty'"
]
},
"execution_count": 54,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"my_dict['b']"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Thus, we can now have strings act as indices too!"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"### Sets"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"It is a collection of unordered items. It follows the properties of a mathematical set, where no duplicate elements are stored."
]
},
{
"cell_type": "code",
"execution_count": 55,
"metadata": {},
"outputs": [],
"source": [
"my_set = {\"DS\",\"AI\",\"DL\"}"
]
},
{
"cell_type": "code",
"execution_count": 56,
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"{'DS', 'DL', 'AI'}\n"
]
}
],
"source": [
"print(my_set)"
]
},
{
"cell_type": "code",
"execution_count": 57,
"metadata": {},
"outputs": [],
"source": [
"my_set_2 = {'a','a','b','b'}"
]
},
{
"cell_type": "code",
"execution_count": 58,
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"{'a', 'b'}\n"
]
}
],
"source": [
"print(my_set_2)"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"### Tuples"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"A tuple is a list, which cannot be changed/modified. Tuples are ordered, just like lists."
]
},
{
"cell_type": "code",
"execution_count": 59,
"metadata": {},
"outputs": [],
"source": [
"my_tuple = (\"a\",\"b\",\"c\")"
]
},
{
"cell_type": "code",
"execution_count": 60,
"metadata": {},
"outputs": [],
"source": [
"my_list = [\"a\",\"b\",\"c\"]"
]
},
{
"cell_type": "code",
"execution_count": 61,
"metadata": {},
"outputs": [
{
"ename": "TypeError",
"evalue": "'tuple' object does not support item assignment",
"output_type": "error",
"traceback": [
"\u001b[1;31m---------------------------------------------------------------------------\u001b[0m",
"\u001b[1;31mTypeError\u001b[0m Traceback (most recent call last)",
"\u001b[1;32m\u001b[0m in \u001b[0;36m\u001b[1;34m()\u001b[0m\n\u001b[1;32m----> 1\u001b[1;33m \u001b[0mmy_tuple\u001b[0m\u001b[1;33m[\u001b[0m\u001b[1;36m0\u001b[0m\u001b[1;33m]\u001b[0m \u001b[1;33m=\u001b[0m \u001b[1;34m'd'\u001b[0m\u001b[1;33m\u001b[0m\u001b[0m\n\u001b[0m",
"\u001b[1;31mTypeError\u001b[0m: 'tuple' object does not support item assignment"
]
}
],
"source": [
"my_tuple[0] = 'd'"
]
},
{
"cell_type": "code",
"execution_count": 62,
"metadata": {},
"outputs": [],
"source": [
"my_list[0] = 'd'"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"All datatypes have associated built-in methods which help in performing basic tasks on them. We will look at them once we look at functions, and understand objects. "
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"## The if statement"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"This is used to decide if a statement / a group of statements should be executed, based on a condition."
]
},
{
"cell_type": "code",
"execution_count": 63,
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"5\n",
"Yes!\n"
]
}
],
"source": [
"a = 5\n",
"b = 6\n",
"if a:\n",
" \n",
"```\n",
"- The statements after 'if' are executed if the expression evaluates to True.\n",
"- Notice that we do not have a bracket to denote the part of code which is to be executed if the condition is true.\n",
"- Instead, we use indentation to denote that block of statements. A constant indent tells Python that those statements belong to one code block."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"For a complicated condition(using logical operators and/or expressions), we enclose all of it in a bracket."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Now, we can also extend this to execute another block of statements if the condition evaluates to False."
]
},
{
"cell_type": "code",
"execution_count": 64,
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"6\n",
"No!\n"
]
}
],
"source": [
"a = 5\n",
"b = 6\n",
"if a>b:\n",
" print(a)\n",
" print(\"Yes!\")\n",
"else:\n",
" print(b)\n",
" print(\"No!\")"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Thus, the keywords 'if' and 'else' are used to execute statements based on a condition."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"What do you do if you want to have multiple condition based statement execution?\n",
"\n",
"For example, if a person is considered:\n",
"- Short if his height is less than 4 feet.\n",
"- Average if his height is between 4 and 5 feet.\n",
"- Tall if his height is more than 5 feet.\n",
"\n",
"We need another keyword here to make our lives easier, called 'elif'."
]
},
{
"cell_type": "code",
"execution_count": 65,
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"Average\n"
]
}
],
"source": [
"height = 4.5\n",
"if height<4:\n",
" print(\"Short\")\n",
"elif height<5:\n",
" print(\"Average\")\n",
"else:\n",
" print(\"Tall\")"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"The importance of indentation is paramount in Python. It replaces the cumbersome curly brackets that exist in C, and which if you forget to close, can lead to so many problems.\n",
"\n",
"Indentation makes it easy to trace which code block does which part of code belong to. When you have [nested loops](#Nested-Loops) (explained later), it aids in visual understanding."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"## For Loops"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"A for loop is used to repeat a block of instructions/statements multiple number of times(most common use case for beginners). In Python, it is used to iterate over a sequence of items as well (lists, sets, tuples and even strings)."
]
},
{
"cell_type": "code",
"execution_count": 66,
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"0\n",
"1\n",
"2\n",
"3\n",
"4\n",
"5\n",
"6\n",
"7\n",
"8\n",
"9\n"
]
}
],
"source": [
"for i in range(10):\n",
" print(i)"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Here, the variable following for (in this case, i) is assigned values from the sequence of items serially which is referred to after the 'in' keyword. The range() function generates numbers from 0 to the number passed to it. This will become clearer when we study functions. For now, this is standard syntax when we want to generate numbers from 0 to n. \n",
"\n",
"Thus, the variable is assigned values from the sequence mentioned. Another example is given below:"
]
},
{
"cell_type": "code",
"execution_count": 67,
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"1\n",
"2\n",
"s\n",
"errve\n",
"0.4\n",
"True\n"
]
}
],
"source": [
"my_list = [1,2,'s','errve',0.4, True]\n",
"for i in my_list:\n",
" print(i)"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"We can loop through a string as well."
]
},
{
"cell_type": "code",
"execution_count": 68,
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"H\n",
"e\n",
"y\n",
" \n",
"t\n",
"h\n",
"e\n",
"r\n",
"e\n",
"!\n"
]
}
],
"source": [
"for i in \"Hey there!\":\n",
" print(i)"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"In short, **we can loop through any collective datatype**, fetching every item from the datatype and assigning it to the variable mentioned after the 'for' keyword."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Here, let us have a look at Nested Loops, which are extremely powerful."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"## Nested Loops"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"When we have a loop within a loop, we have Nested Loops. These can be used to generate various patterns."
]
},
{
"cell_type": "code",
"execution_count": 69,
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"0 0\n",
"0 1\n",
"0 2\n",
"\n",
"1 0\n",
"1 1\n",
"1 2\n",
"\n",
"2 0\n",
"2 1\n",
"2 2\n",
"\n"
]
}
],
"source": [
"for i in range(3):\n",
" for j in range(3):\n",
" print(i,j)\n",
" print()"
]
},
{
"cell_type": "code",
"execution_count": 70,
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"*\n",
"**\n",
"***\n",
"****\n",
"*****\n"
]
}
],
"source": [
"for i in range(5):\n",
" for j in range(i+1):\n",
" print('*',end='')\n",
" print()"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"range(n) goes from 0 to n-1"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Thus, using nested loops, you can set a variable inner loop dependent on the outer loop.\n",
"\n",
"Also notice how indentation now makes the code extremely readable."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"## While Loops"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Here, we can execute a set of statements as long as a condition is true."
]
},
{
"cell_type": "code",
"execution_count": 71,
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"10\n",
"9\n",
"8\n",
"7\n",
"6\n",
"5\n",
"4\n",
"3\n",
"2\n",
"1\n"
]
}
],
"source": [
"a = 10\n",
"while(a>0):\n",
" print(a)\n",
" a-=1"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Thus, in Python, for loop can iterate over a collective datatype, and a while loop executes until a statement evaluates to False."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"## continue and break"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"The continue statement is used in loops to skip all the following statements in the current code block, and proceed with the next iteration."
]
},
{
"cell_type": "code",
"execution_count": 72,
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"9\n",
"8\n",
"7\n",
"6\n",
"5\n",
"1\n",
"0\n"
]
}
],
"source": [
"a = 10\n",
"while(a>0):\n",
" a-=1\n",
" if(a<5 and a>1):\n",
" continue\n",
" print(a)"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"The break statement on the other hand, exits the loop being executed."
]
},
{
"cell_type": "code",
"execution_count": 73,
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"9\n",
"8\n",
"7\n",
"6\n",
"5\n"
]
}
],
"source": [
"a = 10\n",
"while(a>0):\n",
" a-=1\n",
" if(a<5 and a>1):\n",
" break\n",
" print(a)"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Time for a few exercises:\n",
"- Write a program that finds the units place digit of a number.\n",
"- Write a program that prints the following pattern:\n",
"\n",
"\\****\n",
"\n",
"\\*\\****\n",
"\n",
"\\*\\*\\****\n",
"\n",
"\\*\\****\n",
"\n",
"\\****\n",
"\n",
"- Write a program that reads a number from the user, and outputs it's factors."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"## In the Next Lecture, we will dive deeper into Python, and understand functions, and in-built methods. "
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"# Thank You!"
]
}
],
"metadata": {
"kernelspec": {
"display_name": "Python 3",
"language": "python",
"name": "python3"
},
"language_info": {
"codemirror_mode": {
"name": "ipython",
"version": 3
},
"file_extension": ".py",
"mimetype": "text/x-python",
"name": "python",
"nbconvert_exporter": "python",
"pygments_lexer": "ipython3",
"version": "3.6.5"
}
},
"nbformat": 4,
"nbformat_minor": 2
}