{
"cells": [
{
"cell_type": "markdown",
"metadata": {},
"source": [
"# \"Unit 3 Sections 14 and 15\"\n",
"> \"Libraries and Random Values\"\n",
" \n",
"- toc: true\n",
"- branch: master\n",
"- badges: true\n",
"- comments: true\n",
"- permalink: /jupyter/libraries\n",
"- categories: [lessons]"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
""
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"### wget https://raw.githubusercontent.com/aidenhuynh/CS_Swag/master/_notebooks/2022-11-30-randomvalues.ipynb\n",
"\n",
"# Libraries\n",
"\n",
"* A library is a collection of precompiled codes that can be used later on in a program for some specific well-defined operations. \n",
"* These precompiled codes can be referred to as modules. Each module contains bundles of code that can be used repeatedly in different programs.\n",
"* A library may also contain documentation, configuration data, message templates, classes, and values, etc.\n",
"\n",
"### Why are libraries important?\n",
"* Using Libraries makes Python Programming simpler and convenient for the programmer. \n",
"* One example would be through looping and iteration, as we don’t need to write the same code again and again for different programs. \n",
"* Python libraries play a very vital role in fields of Machine Learning, Data Science, Data Visualization, etc.\n",
"\n",
"### A few libraries that simplify coding processes:\n",
"* **Pillow** allows you to work with images.\n",
"* **Tensor Flow** helps with data automation and monitors performance.\n",
"* **Matplotlib** allows you to make 2D graphs and plots.\n",
"\n",
"The AP Exam Refrence Sheet itself is a library!\n",
"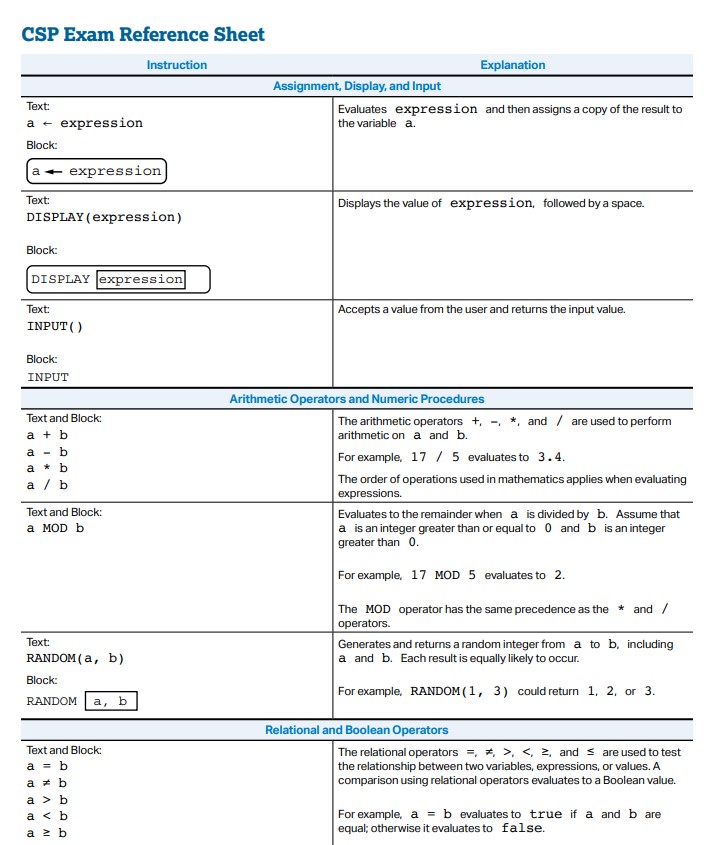\n",
"\n",
"## Hacks:\n",
"Research two other Python Libraries NOT DISCUSSED DURING LESSON and make a markdown post, explaining their function and how it helps programmers code.\n",
"\n",
"### API’s\n",
"* An Application Program Interface, or API, contains specific direction for how the procedures in a library behave and can be used. \n",
"* An API acts as a gateway for the imported procedures from a library to interact with the rest of your code.\n",
"\n",
"### Activity: Walkthrough with NumPy\n",
"* Install NumPy on VSCode:\n",
" 1. Open New Terminal In VSCode:\n",
" 2. pip3 install --upgrade pip\n",
" 3. pip install numpy \n",
" \n",
"REMEMBER: When running library code cells use Python Interpreter Conda (Version 3.9.12)\n",
" \n",
"### Example of using NumPy for arrays:"
]
},
{
"cell_type": "code",
"execution_count": null,
"metadata": {},
"outputs": [],
"source": [
"import numpy as np\n",
"new_matrix = np.array([[1, 2, 3],[4, 5, 6],[7, 8, 9]])\n",
" \n",
"print (new_matrix)"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"#### Example of using NumPy for derivatives:"
]
},
{
"cell_type": "code",
"execution_count": null,
"metadata": {},
"outputs": [],
"source": [
"import numpy as np\n",
" \n",
"# defining polynomial function\n",
"var = np.poly1d([2, 0, 1])\n",
"print(\"Polynomial function, f(x):\\n\", var)\n",
" \n",
"# calculating the derivative\n",
"derivative = var.deriv()\n",
"print(\"Derivative, f(x)'=\", derivative)\n",
" \n",
"# calculates the derivative of after\n",
"# given value of x\n",
"print(\"When x=5 f(x)'=\", derivative(5))"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"## Random Values\n",
"\n",
"* Random number generation (RNG) produces a random number (crazy right?)\n",
" - This means that a procedure with RNG can return different values even if the parameters (inputs) do not change\n",
"* CollegeBoard uses RANDOM(A, B)
, to return an integer between integers A
and B
.\n",
" - RANDOM(1, 10) can output 1, 2, 3, 4, 5, 6, 7, 8, 9, or 10\n",
" - In Python, this would be random.randint(A, B)
, after importing Python's \"random\" library (import random
)\n",
" - JavaScript's works a little differently, with Math.random()
returning a value between 0 and 1.\n",
" - To match Python and CollegeBoard, you could make a procedure like [this](https://stackoverflow.com/a/7228322)\n",
"\n",
"#### CollegeBoard Example: What is the possible range of values for *answ3*\n",
"\n"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"#### Convert the following procedure to Python, then determine the range of outputs if n = 5.\n",
"
\n", "\n", "PROCEDURE Dice(n)\n", "\tsum ← 0\n", "\tREPEAT UNTIL n = 0\n", "\t\tsum ← sum + RANDOM(1, 6)\n", "\t\tn ← n - 1\n", "\tRETURN sum\n", "\n", "\n" ] }, { "cell_type": "code", "execution_count": null, "metadata": {}, "outputs": [], "source": [ "import _____ # Fill in the blank\n", "\n", "def Dice(n):\n", " # Code here\n", " \n", "Dice(5) # Will output a range of __ to __" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "## Homework\n", "1. Write a procedure that generates *n* random numbers, then sorts those numbers into lists of even and odd numbers (JS or Python, Python will be easier).\n", "\n", "2. Using NumPy and only coding in python cell, find the answer to the following questions:\n", " a. What is the derivative of 2x^5 - 6x^2 + 24x?\n", " b. What is the derivative of (13x^4 + 4x^2) / 2 when x = 9? \n", "\n", "3. Suppose you have a group of 10 dogs and 10 cats, and you want to create a random order for them. Show how random number generation could be used to create this random order." ] }, { "cell_type": "code", "execution_count": null, "metadata": {}, "outputs": [], "source": [ "# CODE CODE CODE" ] } ], "metadata": { "kernelspec": { "display_name": "Python 3.9.12 ('base')", "language": "python", "name": "python3" }, "language_info": { "file_extension": ".js", "mimetype": "application/javascript", "name": "python", "version": "3.9.12" }, "orig_nbformat": 4, "vscode": { "interpreter": { "hash": "ffc1763adbe1425c55f4d4f6bbc7c5400773fec8c10f143e4ec62144ce703445" } } }, "nbformat": 4, "nbformat_minor": 2 }