{ "cells": [ { "cell_type": "markdown", "metadata": {}, "source": [ " 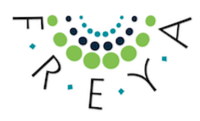 | [FREYA](https://www.project-freya.eu/en) WP2 [User Story3](https://www.pidforum.org/t/pid-graph-graphql-example-research-organization/929) | As an administrator for the University of Oxford I am interested in the reuse of research outputs from our university, so that I can help identify the most interesting research outputs.\n", ":------------- | :------------- | :-------------\n", "\n", "It is important for research organisations to measure quality and quantity of their outputs as well as their relevance to latest global research trends and to their own strategic science direction.
\n", "This notebook uses the [DataCite GraphQL API](https://api.datacite.org/graphql) to retrieve up to 100 outputs (e.g. publications or datasets) from [University of Oxford](https://ror.org/052gg0110) in order to quantify and visualise their reuse.\n", "\n", "**Goal**: By the end of this notebook, for a given organization, you should be able to display:\n", "- Counts of citations, views and downloads metrics, aggregated across all of the organization's outputs;\n", "- An interactive stacked bar plot showing how the metric counts of each of the following output characteristics contributes the corresponding aggregated metric counts: