{ "cells": [ { "cell_type": "markdown", "metadata": {}, "source": [ " 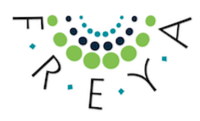 | [FREYA](https://www.project-freya.eu/en) WP2 [User Story 4](https://github.com/datacite/pidgraph-notebooks-python/issues/8) | As a funder I want to see how many of the research outputs funded by me have an open license enabling reuse, so that I am sure I properly support Open Science. \n", " :------------- | :------------- | :-------------\n", "\n", "Funders that support open research are interested in monitoring the extent of open access given to the outputs of grants they award - while the grant is active as well as retrospectively.
\n", "This notebook uses the [DataCite GraphQL API](https://api.datacite.org/graphql) to retrieve and report license types of outputs of the following funders to date:\n", " - [DFG (Deutsche Forschungsgemeinschaft, Germany)](https://doi.org/10.13039/501100001659)\n", " - [ANR (Agence Nationale de la Recherche, France)](https://doi.org/10.13039/501100001665)\n", " - [SNF (Schweizerischer Nationalfonds zur Förderung der Wissenschaftlichen Forschung, Switzerland)](https://doi.org/10.13039/501100001711)\n", "\n", "**Goal**: By the end of this notebook you should be able to:\n", "- Retrieve licenses across all output types for three different funders; \n", "- Plot interactive bar plots showing for each funder respectively the proportion of outputs:\n", " - issued under a given license type (including no license);\n", " - per output type (\"Dataset\" and \"Text\"), issued under a given license type (including no license).