{ "cells": [ { "cell_type": "markdown", "metadata": {}, "source": [ " 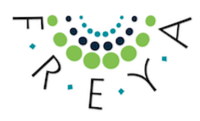 | [FREYA](https://www.project-freya.eu/en) WP2 [User Story 5](https://github.com/datacite/freya/issues/35) | As a student using the British Library's EThOS database, I want to be able to find all dissertations on a given topic. \n", " :------------- | :------------- | :-------------\n", "\n", "It is important for postgraduate students to identify easily existing dissertations on a research topic of interest.
\n", "This notebook uses the [DataCite GraphQL API](https://api.datacite.org/graphql) to retrieve all dissertations for three different queries: *Shakespeare*, *Machine learning* and *Ebola*. These queries illustrate trends in the number of dissertations created over time.\n", "\n", "**Goal**: By the end of this notebook you should be able to:\n", "- Retrieve all dissertations (across multiple repositories) matching a specific query; \n", "- For each query:\n", " - Display a bar plot of the number of dissertations per year, including a trend line, e.g.