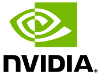 |
Jetson Inference
DNN Vision Library
|
Go to the documentation of this file.
23 #ifndef __URI_RESOURCE_H_
24 #define __URI_RESOURCE_H_
113 URI(
const char* uri );
119 bool Parse(
const char* uri );
124 void Print(
const char* prefix=
"" )
const;
129 inline const char*
c_str()
const {
return string.c_str(); }
134 operator const char* ()
const {
return string.c_str(); }
139 operator std::string ()
const {
return string; }
std::string protocol
Protocol string (e.g.
Definition: URI.h:159
const char * c_str() const
Cast to C-style string (const char*)
Definition: URI.h:129
std::string path
Path (for a network URI this comes after the port)
Definition: URI.h:164
std::string string
Full resource URI (what was originally parsed)
Definition: URI.h:154
std::string location
Path, IP address, or device name.
Definition: URI.h:174
void operator=(const char *uri)
Assignment operator (parse URI string)
Definition: URI.h:144
int port
IP port, camera port, ect.
Definition: URI.h:179
URI()
Default constructor.
Resource URI of a video device, IP stream, or file/directory.
Definition: URI.h:101
bool Parse(const char *uri)
Parse the URI from the given resource string.
operator std::string() const
Cast to std::string
Definition: URI.h:139
void Print(const char *prefix="") const
Log the URI, with an optional prefix label.
std::string extension
File extension (for files only, otherwise empty)
Definition: URI.h:169