{
"cells": [
{
"cell_type": "code",
"execution_count": 1,
"metadata": {
"collapsed": false
},
"outputs": [
{
"data": {
"text/html": [
"\n",
"\n",
"\n",
"\n",
"\n",
"\n"
],
"text/plain": [
""
]
},
"execution_count": 1,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"from IPython.core.display import HTML\n",
"css_file = './custom.css'\n",
"HTML(open(css_file, \"r\").read())"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"###### Content provided under a Creative Commons Attribution license, CC-BY 4.0; code under MIT License. (c)2014 [David I. Ketcheson](http://davidketcheson.info)"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"##### version 0.1 - May 2014"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"# Hyperbolic Conservation Laws"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"\\begin{equation*}\n",
"\\newcommand{Dx}{\\Delta x}\n",
"\\newcommand{Dt}{\\Delta t}\n",
"\\newcommand{imh}{{i-1/2}}\n",
"\\newcommand{iph}{{i+1/2}}\n",
"\\end{equation*}\n",
"Many models of wave phenomena are governed by *hyperbolic conservation laws*. In this short course, we will learn about hyperbolic conservation laws and their numerical solution."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"## Conservation of mass"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Imagine a fluid flowing in a narrow tube. We'll use $q$ to indicate the density of the fluid and $u$ to indicate its velocity. Both of these are functions of space and time: $q = q(x,t)$; $u=u(x,t)$. The total mass in the section of tube $[x_1,x_2]$ is\n",
"\n",
"\\begin{equation}\n",
"\\int_{x_1}^{x_2} q(x,t) dx.\n",
"\\end{equation}\n",
"\n",
"This total mass can change in time due to fluid flowing in or out of this section of the tube. We call the rate of flow the *flux*, and represent it with the function $f(q)$. Thus the net rate of flow of mass into (or out of) the interval $[x_1,x_2]$ at time $t$ is\n",
"\n",
"$$f(q(x_1,t)) - f(q(x_2,t)).$$\n",
"\n",
"We just said that this rate of flow must equal the time rate of change of total mass; i.e.\n",
"\n",
"$$\\frac{d}{dt} \\int_{x_1}^{x_2} q(x,t) dx = f(q(x_1,t)) - f(q(x_2,t)).$$\n",
"\n",
"Now since $\\int_{x_1}^{x_2} \\frac{\\partial}{\\partial x} f(q) dx = f(q(x_2,t)) - f(q(x_1,t))$, we can rewrite this as\n",
"\n",
"$$\\frac{d}{dt} \\int_{x_1}^{x_2} q(x,t) dx = -\\int_{x_1}^{x_2} \\frac{\\partial}{\\partial x} f(q) dx.$$\n",
"\n",
"Under certain smoothness assumptions on $q$, we can move the time derivative inside the integral. We'll also put everything on the left side, to obtain\n",
"\n",
"$$\\int_{x_1}^{x_2} \\left(\\frac{\\partial}{\\partial t}q(x,t) + \\frac{\\partial}{\\partial x} f(q)\\right) dx = 0.$$\n",
"\n",
"Since this integral is zero for *any* choice of $x_1,x_2$, it must be that the integrand (the expression in parentheses) is actually zero *everywhere*! Therefore we can write the **differential conservation law**\n",
"\n",
"$$q_t + f_x = 0.$$\n",
"\n",
"Here and throughout the course, we use subscripts to denote partial derivatives.\n",
"This equation expresses the fact that the total mass is conserved -- since locally the mass can change only due to a net inflow or outflow."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"## Advection"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"In order to solve the conservation law above, we need an expression for the flux, $f$. The rate of flow is just mass times velocity: $f=u q$. Thus we obtain the **continuity equation**\n",
"\n",
"$$q_t + (uq)_x = 0.$$\n",
"\n",
"In general, we need another equation to determine the velocity $u(x,t)$. In [Lesson 4](Lesson_04_Fluid_dynamics.ipynb) we'll look at the full equations of fluid dynamics, but for now let's consider the simplest case, in which all of the fluid flows at a single, constant velocity $u(x,t)=a$. Then the continuity equation becomes the **advection equation**\n",
"\n",
"$$q_t + a q_x = 0.$$\n",
"\n",
"This equation has a very simple solution. If we are given the density $q(x,0)=q_0(x)$ at time zero, then the solution is just\n",
"\n",
"$$q(x,t) = q_0(x-at).$$"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Let's plot the solution of the advection equation on the interval $[0,1]$ for the initial condition\n",
"$$q_0(x) = e^{-2(x-1/2)^2}.$$"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"First, let's import all the modules we'll need."
]
},
{
"cell_type": "code",
"execution_count": 2,
"metadata": {
"collapsed": false
},
"outputs": [],
"source": [
"%matplotlib inline\n",
"import numpy as np\n",
"import matplotlib.pyplot as plt\n",
"from matplotlib import animation\n",
"from clawpack.visclaw.JSAnimation import IPython_display"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Next, we'll set up a grid and the initial condition:"
]
},
{
"cell_type": "code",
"execution_count": 3,
"metadata": {
"collapsed": false
},
"outputs": [],
"source": [
"x = np.linspace(0,1,1000) # Spatial grid\n",
"t = np.linspace(0,1) # Temporal grid\n",
"a = 1.0 # Advection speed\n",
"\n",
"def q_0(x): # Initial condition\n",
" return np.exp(-200.*(x-0.2)**2)"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Finally, let's make an animation of the solution. It will take a few moments to run this code. For now, you don't need to worry about understanding all of the plotting code below. Just play with the animation until you have a feel for how the solution behaves."
]
},
{
"cell_type": "code",
"execution_count": 4,
"metadata": {
"collapsed": false
},
"outputs": [
{
"data": {
"text/html": [
"\n",
"\n",
"\n",
"
\n",
"\n",
"\n",
"\n"
],
"text/plain": [
""
]
},
"execution_count": 4,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"fig = plt.figure(figsize=(8,4)) # Create an empty figure\n",
"ax = plt.axes()\n",
"line, = ax.plot([], [],linewidth=2) # Create an empty line plot\n",
"plt.axis((0,1,-0.1,1.1)) # Set the bounds of the plot\n",
"\n",
"def plot_q(t):\n",
" line.set_data(x,q_0(x-a*t)) # Replace the line plot with the solution at time t\n",
" \n",
"animation.FuncAnimation(fig, plot_q, frames=t) # Animate the solution"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"As you can see, the initial pulse just moves to the right at speed $a$ as time advances. This isn't very interesting, but it captures the most important feature of hyperbolic equations: waves travel at finite speed."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"## Characteristics"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Notice that the solution value is constant along the line $x-at=x_0$, in the $x-t$ plane, for each value of $x_0$. These lines are called **characteristics**; they are the trajectories along which solution information is transmitted. The value $a$ is referred to as the **characteristic velocity**. The code below plots some of these characteristics.\n",
"\n",
"When we learn about more complicated conservation laws, we'll see that information still travels along characteristics, but those characteristics aren't necessarily straight lines."
]
},
{
"cell_type": "code",
"execution_count": 5,
"metadata": {
"collapsed": false
},
"outputs": [
{
"data": {
"text/plain": [
"(0, 1)"
]
},
"execution_count": 5,
"metadata": {},
"output_type": "execute_result"
},
{
"data": {
"image/png": [
"iVBORw0KGgoAAAANSUhEUgAAAeYAAAEACAYAAABmjtVQAAAABHNCSVQICAgIfAhkiAAAAAlwSFlz\n",
"AAALEgAACxIB0t1+/AAAGTtJREFUeJzt3X+o5fdd5/Hna5JUpmTbrmToH8lI1JTZutBsFNNGNzjS\n",
"gmkFS+qSUn8sWqlhSUogSqMi2yDRbf5YcGtLKd2mhECaP3SL2aVMcdBBU5pq2CRNaxIyqWXzQ0p/\n",
"qBTpHw2+949zTr29uXPvuefHPe/v9zwf8IG5c78593O+ue/7nO85Z86kqpAkST0c2/QGJEnSvzLM\n",
"kiQ1YpglSWrEMEuS1IhhliSpEcMsSVIjB4Y5yT1JvprkiX2O+WCSZ5I8nuSa1W5RkqTtMc8V8yeA\n",
"Gy70ySRvA66qqtcBvw58ZEV7kyRp6xwY5qr6K+Af9jnk54B7p8d+HnhNkteuZnuSJG2XVTzHfDnw\n",
"3I6PnweuWMHtSpK0dS5e0e1k18ff8z6fSXzfT0nS1qmq3X080CqumF8ATu74+Irp70mSpENaxRXz\n",
"g8CtwANJ3gT8Y1V9da8DF/mTg+aX5M6qunPT+xg7z/P6eY7Xz3O8OklOAL8JvAf4JPAB4P8tensH\n",
"hjnJJ4GfAi5L8hzwfuASgKr6aFV9OsnbkpwH/hn41UU3I0nSUOwR5Kur6rnp5xa+3QPDXFXvmuOY\n",
"WxfegSRJA7JfkFfBd/4al3Ob3sCWOLfpDWyBc5vewBY4t+kNDE2SE0nuBp4GLmUS5FtWGWWAVK3/\n",
"BdOzV2X7HLMkaWj2eg75oBgv0z2vmCVJ2sNRXSHvZpglSdphU0GeMcySJLH5IM8YZknSVusS5BnD\n",
"LEnaSt2CPGOYJUlbpWuQZwyzJGkrdA/yjGGWJI3aUII8Y5glSaM0tCDPGGZJ0qgMNcgzhlmSNApD\n",
"D/KMYZYkDdpYgjxjmCVJgzS2IM8YZknSoIw1yDOGWZI0CGMP8oxhliS1ti1BnjHMkqSWti3IM4ZZ\n",
"ktTKtgZ5xjBLkloYS5CTXL/Mf2+YJUkbNaYgJzkL3LvM7RhmSdJGjDTI9wOnlrk9wyxJOlJjDnJV\n",
"3VNV31nmdg2zJOlIGOT5GGZJ0loZ5MMxzJKktTDIizHMkqSVMsjLMcySpJUwyKthmCVJSzHIq2WY\n",
"JUkLMcjrYZglSYdikNfLMEuS5mKQj4ZhliTtyyAfLcMsSdqTQd4MwyxJ+h4GebMODHOSG5I8leSZ\n",
"JHfs8fnLkpxJ8liSLyb5lbXsVJK0Vga5h33DnOQi4EPADcCPAO9K8vpdh90KPFpV/wE4Dfz3JBev\n",
"Ya+SpDUwyL0cdMV8LXC+qr4yvWMPAG/fdczfA6+a/vpVwDeq6qXVblOStGoGuaeDrmwvB3b+D3oe\n",
"eOOuYz4G/HmSF4F/A9y0uu1JklYtyQngN4H3AJ9kEuRBxRgmQQbeD/wQcBdw31BjvNNBYa45buN3\n",
"gMeq6nSSHwb+LMnVVfWt3QcmuXPHh+eq6tzcO5UkLcUgr1eS00ye0l3KQWF+ATi54+OTTK6ad/oJ\n",
"4PcBqurZJH8HnAIe2X1jVXXnwjuVJC3EIB+N6cXmOYAk71/0dg56jvkR4HVJrkzyCuCdwIO7jnkK\n",
"eMt0I69lEuUvL7ohSdJq+BzyMO17xVxVLyW5FfgMcBHw8ap6MsnN089/FPgD4BNJHmcS+vdV1TfX\n",
"vG9J0gV4hTxsqZrnaeQlv0hSAFWVtX8xSdpSewT5AwZ5M5bpnn/fWJIGzivkPpIcB359mdvwLTkl\n",
"aaB8DrmPJMeT3AY8y5KvzDbMkjQwBrmPPYL8s1V14zK36UPZkjQQPmTdx46HrO8APs8kyI+u4rYN\n",
"syQ1Z5D7WGeQZwyzJDVlkPs4iiDPGGZJasYg93GUQZ4xzJLUhEHuYxNBnjHMkrRhBrmPTQZ5xjBL\n",
"0oYY5D46BHnGMEvSETPIfXQK8oxhlqQjYpD76BjkGcMsSWtmkPvoHOQZwyxJa2KQ+xhCkGcMsySt\n",
"mEHuY0hBnjHMkrQiBrmPIQZ5xjBL0pIMch9DDvKMYZakBRnkPsYQ5BnDLEmHZJD7GFOQZwyzJM3J\n",
"IPcxxiDPGGZJOoBB7mPMQZ4xzJJ0AQa5j20I8oxhlqRdDHIf2xTkGcMsSVMGuY9tDPKMYZa09Qxy\n",
"H9sc5Jljm96AJG1KkhNJ7gaeBi5lEuRbhhblJNcnOQvcC9wPnKqqe4YU5STHk9wGPAucZhLkG4cW\n",
"5SSnkty3zG0YZklbxyD3McIgP8Tk+2phhlnS1jDIfYw4yFdV1V3L3KZhljR6BrmPsQe5qv5p2ds2\n",
"zJJGyyD3YZDnZ5gljY5B7sMgH55hljQaBrkPg7w4wyxp8AxyHwZ5eYZZ0mAZ5D4M8uoYZkmDY5D7\n",
"MMird2CYk9yQ5KkkzyS54wLHnE7yaJIvJjm38l1KEga5E4O8Pvu+V3aSi4APAW8BXgD+JsmDVfXk\n",
"jmNeA3wY+Jmqej7JZevcsKTt43tZ9zGW97JOcgr4XeAG4H8At24yxjsddMV8LXC+qr4y/eZ5AHj7\n",
"rmN+AfiTqnoeoKq+vvptStpGXiH34RXy0TkozJcDOwfg+env7fQ64PuT/EWSR5L88io3KGn7GOQ+\n",
"DPLRO+iffaw5buMS4EeBNwOvBD6X5OGqembZzUnaLj5k3YcPWW/OQWF+ATi54+OTTK6ad3oO+HpV\n",
"fRv4dpK/BK4GXhbmJHfu+PBcVZ077IYljY9B7sMgL/U1TzN5VGG526m68EVxkouZXPK/GXgR+Gvg\n",
"Xbte/PXvmLxA7GeA72PyP/KdVfW3O44pgKrKshuWNB57BPkDBnkz9gjy740kyH+0iSvkZbq37xVz\n",
"Vb2U5FbgM8BFwMer6skkN08//9GqeirJGeALwL8AH9sZZUnazSvkPrxC7mffK+aVfRGvmCXhFXIn\n",
"XiGv1zLd852/JK2dr7Luw1dZ92eYJa2NQe7DIA+HYZa0cga5D4M8PIZZ0soY5D4M8nAZZklLM8h9\n",
"GOThM8ySFmaQ+zDIfSRZqq2GWdKhGeQ+DHIfSY4luQl4YpnbMcyS5maQ+zDIfewK8u3Abyxzewe9\n",
"V7Yk+U5djfhOXX1MH7L+T0y+p77FJMifqapKFn8/LcMs6YIMch8GuY/9gryK2zfMkl7GIPdhkPtY\n",
"d5BnDLOk7zLIfRjkPo4qyDOGWZJBbsQg93HUQZ4xzNIWM8h9GOQ+NhXkGcMsbSGD3IdB7mPTQZ4x\n",
"zNIWMch9GOQ+ugR5xjBLW8Ag92GQ++gW5BnDLI2YQe7DIPfRNcgzhlkaIYPch0Huo3uQZwyzNCIG\n",
"uQ+D3MdQgjxjmKURMMh9GOQ+hhbkGcMsDZhB7sMg9zHUIM8YZmmADHIfBrmPoQd5xjBLA2KQ+zDI\n",
"fYwlyDOGWRoAg9yHQe5jbEGeMcxSYwa5D4Pcx1iDPGOYpYYMch8GuY+xB3nGMEuNGOQ+DHIf2xLk\n",
"GcMsNWCQ+zDIfWxbkGcMs7RBBrkPg9zHtgZ5xjBLG2CQ+zDIfYwlyNP5XtixVW1E0sGSnEhyN/A0\n",
"cCmTIN8ytCgnuT7JWeBe4H7gVFXdM6QoJzme5DbgWeA0kyDfOLQoJzmV5D7gISbfV1dV1V1DinKS\n",
"Y0luAp4AbmcS5Ouq6syQorxrvhdmmKUjYJD7MMh9jDTIlwJXL3N7PpQtrZEPWffhQ9Z9jOwh6z3n\n",
"O8nCt2uYpTUwyH0Y5D62IcirYJilFTLIfRjkPgzy4Rz4HHOSG5I8leSZJHfsc9yPJ3kpyTtWu0Wp\n",
"P59D7sPnkPsY83PI65zvfcOc5CLgQ0z+pPYjwLuSvP4Cx90NnAEWf2BdGhiD3IdB7sMgL+egK+Zr\n",
"gfNV9ZXpgD4AvH2P494L/DHwtRXvT2rJIPdhkPswyKtxUJgvB3Zu5Pnp731XksuZxPoj098azMmX\n",
"DmvTA7sqBrkPg9xHl/k+6MVf85zQPwR+q6oqk9eH+1C2RscXdfXhi7r68EVd63FQmF8ATu74+CST\n",
"q+adfgx4YPp3ti4D3prkO1X14O4bS3Lnjg/PVdW5w25YOkrdBnZRBrkPg9zHquc7yWkmj94sp6ou\n",
"uJiE+1ngSuAVwGPA6/c5/hPAO/b4/Zp8qQt/LZer0wJOMHlB4zeBDwMnN72nBe/H9cBZ4MvAu4FL\n",
"Nr2nBe7DceA24EXgU8A1m97TgvfjFHAfk9fi/C7w6k3vaYH7cAy4CfgS8DCTP1xk0/ta4H6sfb6X\n",
"6d6+V8xV9VKSW4HPABcBH6+qJ5PcPP38Rw/1pwCpOa+Q+/AKuQ+vkI9WjuK8Jpn88aHK55/V0h4D\n",
"+4GOA3uQkQb590YS5D8aQZDvZBxBXvt8L9M93/lLW20of4I+yEiD7BXyhniFvFmGWVtpqAO7m0Hu\n",
"wyD3MfT5NszaKkMf2BmD3IdB7mMs822YtRXGMrAGuQ+D3MdY5nvGMGvUxjKwBrkPg9zHWOZ7N8Os\n",
"URrLwBrkPgxyH2OZ7wsxzBqVsQysQe7DIPcxlvk+iGHWKIxlYA1yHwa5j7HM97wMswZtLANrkPsw\n",
"yH2MZb4PyzBrkMYysAa5D4Pcx1jme1GGWYMyloE1yH0Y5D5GNt8LM8wahJENrEFuwCD3MdL5Xtix\n",
"1WxHWo8kJ5LcDTwNXMpkYG8Z2tAmuT7JWeBe4H7gVFXdM6QoJzme5DYm/xTsaSZBvnFoUU5yKsl9\n",
"wENMvq+uqqq7hhTlJMeS3AQ8AdzOJMjXVdWZIUV5zPO9zO15xayWRvonaK+QN8gr5D62Yb6Txf8x\n",
"RcOsVrZhYIfCIPdhkHtZ93wbZrXgwPZhkPswyL0c1XwbZm2UA9uHQe7DIPdy1PNtmLURDmwfBrkP\n",
"g9zLpubbMOtIObB9GOQ+DHIvm55vw6wj4cD2YZD7MMi9dJlvw6y1cmD7MMh9GOReus23YdZaOLB9\n",
"GOQ+DHIvXefbMGulHNg+DHIfBrmX7vNtmLUSDmwfBrkPg9zLUObbMGspDmwfBrkPg9zL0ObbMGsh\n",
"DmwfBrkPg9zLUOfbMOtQHNg+DHIfBrmXoc+3YdZcHNg+DHIfBrmXMcw3GGYdwIHtwyD3YZB7GcN8\n",
"72SYtScHtg+D3IdB7mUM870Xw6zv4cD2YZD7MMi9jGG+92OYBTiwnRjkPgxyL2OY73kY5i3nwPZh\n",
"kPswyL2MYb4PwzBvKQe2D4Pch0HuZQzzvQjDvGUc2D4Mch8GuZcxzPcyDPOWcGD7MMh9GOReRjbf\n",
"Czs25xe6IclTSZ5Jcscen//FJI8n+UKSzyZ5wzKb0uokOZHkbuBp4FImA3vL0IY2yfVJzgL3AvcD\n",
"p6rqniENbZLjSW4DngVOMwnyjUOLcpJTSe4DHmLyfXVVVd01pCgnOZbkJuAJ4HYmQb6uqs4MKcrO\n",
"dx97zPfiqmrfBVwEnAeuBC4BHgNev+uY64BXT399A/Dwrs/X5Evt/7Vcq1vACeBu4JvAh4GTm97T\n",
"gvfjeuAs8GXg3cAlm97TAvfhOHAb8CLwKeCaTe9pwftxCrgP+BqTK+VXb3pPC9yHY8BNwJeAh6c/\n",
"r7LpfS1wP5zvJutC871M9+Z5KPta4HxVfQUgyQPA24End8T9czuO/zxwxRy3qzXwIa0+fMi6Dx+y\n",
"7sX53t88Yb4c2Pk//nngjfsc/2vAp5fZlA7Pge3DIPdhkHtxvuczT5jn/gZO8tNMHo74yQt8/s4d\n",
"H56rqnPz3rb25sD2YZD7MMi9bMt8JznNss8vw1zPMb8JOLPj498G7tjjuDcweS76qj0+53PMq39e\n",
"w+eYmix8DrnNwueQW61tnu9lujfPjV/M5FVmVwKvYO8Xf/3ANMpvWvUGXS87lw5sk2WQ+yyD3Gs5\n",
"32sO8/QLvJXJy/HPA789/b2bgZunv/6fwDeAR6frr1e1Qdd3z6ED22QZ5D7LIPdazvf33M56w7yC\n",
"O2qYFz93DmyTZZD7LIPcaznfe97eWv+6lDbAF3304Yu6+vBFXb043+thmJtxYPvoOLCLMMh9ON99\n",
"dJ5vw9yEA9tH54E9DIPch/PdxxDm2zBvmAPbxxAGdh4GuQ/nu48hzbdh3hAHto8hDex+DHIfzncf\n",
"Q5xvw3zEHNg+hjiwezHIfTjffQx5vg3zEXFg+xjywO5kkPtwvvsYw3wb5jVzYPsYw8CCQe7E+e5j\n",
"LPMNhnltHNg+xjKwBrkP57uPscz3ToZ5xRzYPsYysAa5D+e7j7HM914M84o4sH2MZWANch/Odx9j\n",
"me/9GOYlObB9jGVgDXIfzncfY5nveRjmBTmwfYxlYA1yH853H2OZ78MwzIfkwPYxloE1yH04332M\n",
"Zb4XYZjn5MD2MZaBNch9ON99jGW+l2GYD+DA9jGWgTXIfTjffYxwvhd2bEV7GZ0kJ5LcDTwNXMpk\n",
"YG8Z2tAmuT7JWeBe4H7gVFXdM6ShTXI8yW3As8BpJgN749CGNsmpJPcBDzH5vrqqqu4aUpSTHEty\n",
"E/AEcDuTIF9XVWeGFGXnu48Rz/fiqmrtC6jJl1r/11rBXk8AdwPfBD4MnNz0nha8H9cDZ4EvA+8G\n",
"Ltn0nha4D8eB24AXgU8B12x6Twvej1PAfcDXmPxJ+tWb3tMC9+EYcBPwJeBhJlf72fS+FrgfzneT\n",
"Nfb5XqZ7R7Xx9mF2YPussQ/skJZB7rWc7z7roPk2zMvtzYFtsrZlYIewDHKv5Xz3WfPOt2FebE8O\n",
"bJO1bQPbeRnkXsv57rMOO9+G+XB7cWCbrG0d2I7LIPdaznefteh8G+b59uDANlnbPrCdlkHutZzv\n",
"PmvZ+TbM+39tB7bJcmD7LIPcaznffdaq5tsw7/01Hdgmy4Htswxyr+V891mrnm/D/L1fy4FtshzY\n",
"Pssg91rOd5+1rvk2zOXAdloObJ9lkHst57vPWvd8b3WYHdg+y4Htswxyr+V891lHNd9bGWYHts9y\n",
"YPssg9xrOd991lHP91aF2YHtsxzYPssg91rOd5+1qfneijA7sH2WA9tnGeRey/nuszY936MOswPb\n",
"ZzmwfZZB7rWc7z6ry3yPMswObJ/lwPZZBrnXcr77rG7zPaowO7B9lgPbZxnkXsv57rO6zvcowuzA\n",
"9lkObJ9lkHst57vP6j7faw3z9AfBU8AzwB0XOOaD088/vtf/5P026MD2WQ5sn2WQey3nu88aynyv\n",
"LczARcB54ErgEuAx4PW7jnkb8Onpr98IPDzPBh3Ytezl9IL/nQN7BOd5zts2yGs+x4fcR5v5XuI+\n",
"7DnfXc7xIe7HIIK8Y78Lh/kY+7sWOF9VX6mq7wAPAG/fdczPAfcy2cHngdckee2FbjDJiSR3A08D\n",
"lwJXV9UtVfXcAXtpJcn1Sc4yue/3A6eq6p7pedqU04c5OMnxJLcBz07/25+tqhur6tE17G1tkpxK\n",
"ch/wEJPvq6uq6q6q+qc1fcnTq77BJMeS3AQ8AdwO/AZwXVWdqemUD8EK5/v0yjd3CE3n+1DmmO/T\n",
"m9rbYWxgvjfuoDBfDuwcqOenv3fQMVfsdWMGuQeD3IdB7sX57mMM872o7Df7SX4euKGq3jP9+JeA\n",
"N1bVe3cc87+BD1TVZ6cfnwXeV1X/d8cxg/kBI0nSqlRVDvvfHHTF/AJwcsfHJ5lcEe93zBXT35Mk\n",
"SYd08QGffwR4XZIrmbxw4J3Au3Yd8yBwK/BAkjcB/1hVX915wCJ/YpAkaRvtG+aqeinJrcBnmLxC\n",
"++NV9WSSm6ef/2hVfTrJ25KcB/4Z+NW171qSpJHa9zlmSZJ0tA56jvnQktyQ5KkkzyS54wLHfHD6\n",
"+ceTXLPqPYzdQec4yS9Oz+0Xknw2yRs2sc8hm+f7eHrcjyd5Kck7jnJ/YzDnz4rTSR5N8sUk5454\n",
"i6Mwx8+Ly5KcSfLY9Dz/yga2OVhJ7kny1SRP7HPM4Zq34r9QvZI3JHEtfY6vY/qX75m8QYXneMXn\n",
"eMdxfw78H+DnN73vIa05v49fw+SNVq6YfnzZpvc9tDXneb4T+G+zcwx8A7h403sfymLyJjTXAE9c\n",
"4POHbt6qr5hX/oYkepkDz3FVfa7+9e/6fZ4L/L1yXdA838cA7wX+mMk7Eelw5jnHvwD8SVU9D1BV\n",
"Xz/iPY7BPOf574FXTX/9KuAbVfXSEe5x0Krqr4B/2OeQQzdv1WFe6RuSaE/znOOdfg349Fp3ND4H\n",
"nuMklzP5AfeR6W/5Yo3Dmef7+HXA9yf5iySPJPnlI9vdeMxznj8G/PskLzL59w5uO6K9bYtDN++g\n",
"vy51WPP+cNr916f8oTa/uc9Vkp9m8v6+P7m+7YzSPOf4D4HfqqpKEl7+Pa39zXOOLwF+FHgz8Erg\n",
"c0kerqpn1rqzcZnnPP8O8FhVnU7yw8CfJbm6qr615r1tk0M1b9Vh9g1J1m+ec8z0BV8fY/LObfs9\n",
"zKKXm+cc/xiTv7sPk+fl3prkO1X14NFscfDmOcfPAV+vqm8D307yl8DVTP4lO81nnvP8E8DvA1TV\n",
"s0n+jsk/GPHIkexw/A7dvFU/lP3dNyRJ8gomb0iy+wfVg8B/BrjQG5JoXwee4yQ/APwv4Jeq6vwG\n",
"9jh0B57jqvqhqvrBqvpBJs8z/xejfCjz/Kz4U+A/JrkoySuZvHDmb494n0M3z3l+CngLwPS5z1NM\n",
"/jUtrcahm7fSK+byDUnWbp5zDPxX4N8CH5le0X2nqq7d1J6HZs5zrCXM+bPiqSRngC8A/wJ8rKoM\n",
"8yHM+b38B8AnkjzO5GLtfVX1zY1temCSfBL4KeCyJM8B72fyNMzCzfMNRiRJamTlbzAiSZIWZ5gl\n",
"SWrEMEuS1IhhliSpEcMsSVIjhlmSpEYMsyRJjRhmSZIa+f8AlyF7zPx0nQAAAABJRU5ErkJggg==\n"
],
"text/plain": [
""
]
},
"metadata": {},
"output_type": "display_data"
}
],
"source": [
"fig = plt.figure(figsize=(8,4))\n",
"ax = plt.axes()\n",
"\n",
"for x_0 in np.linspace(0,1,10):\n",
" ax.plot(x,(x-x_0)/a,'-k')\n",
"plt.ylim(0,1)"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"## A finite volume method for advection"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"We can easily solve the advection equation exactly. But the advection equation is a prototype for more complicated conservation laws that we will only be able to solve approximately by using numerical methods. In order to better understand these methods, we will discuss them first in the context of the advection equation."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"For simplicity, we'll suppose that we wish to solve the advection equation on the interval $[0,1]$. We introduce a set of equally spaced *grid cells* of width $\\Dx$, and write $x_i$ to mean the center of cell $i$. Thus the first cell is the interval $[0,\\Dx]$ and $x_1=\\Dx/2$. We will also write $x_\\imh$ or $x_\\iph$ to denote the left or right boundary of cell $i$, respectively.\n",
"\n",
"We write $Q_i$ to denote the *average* value of the solution over cell $i$:\n",
"\n",
"$$Q_i = \\frac{1}{\\Dx} \\int_{x_\\imh}^{x_\\iph} q \\ dx.$$\n",
"\n",
"The simplest finite volume method is obtained by supposing that the solution is actually *equal* to $Q_i$ over all of cell $i$."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"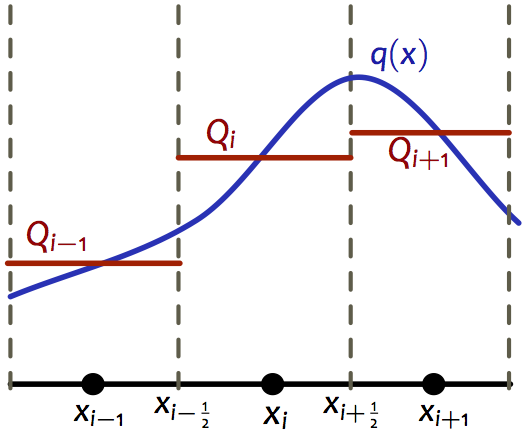"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Suppose $a>0$. Then the flux into cell $i$ from the left is $a Q_{i-1}$ and the flux out of cell $i$ to the right is $a Q_i$. Then our integral conservation law reads\n",
"\n",
"$$Q_i'(t) = -\\frac{a}{\\Dx}\\left(Q_i - Q_{i-1}\\right).$$\n",
"\n",
"Applying a forward difference in time we obtain the *upwind method*\n",
"\n",
"$$Q^{n+1}_i = Q^n_i -\\frac{a}{\\Dx}\\left(Q_i - Q_{i-1}\\right).$$\n",
"\n",
"We call this the upwind method because the solution behaves as if it were being blown by a wind to the right, and the method uses the value $Q_{i-1}$ from the upwind direction.\n",
"\n",
"Here is a bit of Python code to solve the advection equation using the upwind method."
]
},
{
"cell_type": "code",
"execution_count": 6,
"metadata": {
"collapsed": false
},
"outputs": [
{
"data": {
"image/png": [
"iVBORw0KGgoAAAANSUhEUgAAAXcAAAEKCAYAAADpfBXhAAAABHNCSVQICAgIfAhkiAAAAAlwSFlz\n",
"AAALEgAACxIB0t1+/AAAIABJREFUeJzt3Xu0XGWd5vHvY8JF7iACGgIRCHdBUEKr4BwVMbhaUewZ\n",
"DDba2muMPSuK3Toijt1kVt/UYc1iFBtpmravY3paWo0jMWDr8cKAEG4iJJDILRfkfr8m8Js/3reS\n",
"onJO3Xftql3PZ62zdu2qfXb9dk7Oc95697vfrYjAzMyq5WVlF2BmZv3ncDczqyCHu5lZBTnczcwq\n",
"yOFuZlZBDnczswpyuJuZVZDD3YaWpLskva2gfZ8h6W5JT0r6tqTdW9TxtKQn8tcP6l7bR9JSSesl\n",
"vShpv4bvPU/S7ZIel7RS0plFHI9ZI4e7DbMA1O+dSjoC+DrwQWBv4Gngr1rU8dsRsXP+ml/32ovA\n",
"ZcD7p/neJ/P37gJ8GPhfkt7Y6zGYteJwt6Ek6R+B/YDv5dbyZ/q4+w8CSyPi5xHxFPDHwGmSdmxW\n",
"0lRPRsT9EfF1YMU0ry+OiNvz42uAnwEOdyucw92GUkScCdzDlhbzeY3bSNpP0iNNvj4wze4PB26q\n",
"e687gOeAg5uU9M+S7pe0XNJR3RyTpJcDxwG/6ub7zToxs+wCzLoVEfcA0/aVN7ET8FjDc48DO0+z\n",
"/RnA9aTG0FnAckmHRkTjPlr5OnBjRFze4feZdcwtdxtHTwK7Njy3K/DEVBtHxFUR8VxEPBMRXwQe\n",
"BU7s5A0l/Q/SJ4b/1EW9Zh1zuNswazplae6WeaLJ14JpvvUW4Oi6/RwIbAvc3o+6pqjzvwPvBE6O\n",
"iCc7+V6zbrlbxobZfcCBwI+mejF3y0zXldLMPwNXSToBuAH4U+DSfHL1JSTNJp3YvZbUGPoE8Arg\n",
"yrpttmfL79L2kraPiGfza+cAC4ATI+KRLmo164pb7jbM/hL4Qj45+kf92mlE3Ap8nBTy9wEvB/5L\n",
"7XVJF0q6MK/uTBom+TCwDjgZOKUhqJ8m9dkHsAqo/yPx58BsYE3dJ4rP9etYzKajVjfrkDQfOB+Y\n",
"AfxNRHyp4fU9gX8C9iG1Xs6LiL8rpFozM2tL03CXNAO4DTgJWE/6aLogIlbWbbMY2C4izslBfxuw\n",
"d0RsKrJwMzObXqtumXnAmoi4KyI2AkuAUxu2uRfYJT/eBXjIwW5mVq5WJ1RnAWvr1tcBxzdsczHw\n",
"I0kbSP2THuplZlayVuHezpCvz5MuzJjIQ8qukHR0RGweMyzJd+E2M+tSRHQ8x1Krbpn1pDP9NbNJ\n",
"rfd6bwL+NRfwa+BO4JBOCzEzs/5p1XJfAcyVNAfYAJxOGrNbbxXphOuVkvYmBfsdU+2sm78+o0LS\n",
"4ohYXHYdRany8VX52MDHN8p66fVoGu4RsUnSImA5aSjkJRGxUtLC/PpFwF8A35B0E+mTwGcj4uFu\n",
"CzIzs961vEI1IpYByxqeu6ju8YPAu/tfmpmZdctXqPbPZNkFFGyy7AIKNFl2AQWbLLuAgk2WXcAw\n",
"anmFal/eJPcbVbnP3cys33rJTrfczcwqyOFuZlZBDnczswpyuJuZVZDD3cysghzuZmYV5HA3M6sg\n",
"h7uZWQU53M3MKsjhbmaFkJgvcVTZdYwrh7uZ9Z3EW0gTDl4n8dGy6xlHDnczK8Kn8nImcInElyTn\n",
"zSD5H9vM+kriNcB7gY3AOcAm4LPAtyR2LLO2ceJwN7N+WwQIWBLBF4H5wGPA+4CfSry6zOLGhaf8\n",
"NbO+kdiZdJ/lXYA3RHBdfv5Q4PvAAaR7M785grtLK3REeMpfMxsWHyYF+89rwQ4QwSrgeOBqYBbw\n",
"n8spb3w43M2sL/IJ07Py6vmNr0fwIPBnefXtg6prXLUMd0nzJa2StFrS2VO8/hlJN+SvmyVtkrRb\n",
"MeWa2RB7F3AQcA/w3Wm2+SnwAnCcxC6DKmwcNQ13STOAC0gnRA4HFkg6rH6biDgvIo6JiGNIZ8Yn\n",
"I+LRogo2s6FVa7V/NYJNU20QwRPANcAM4C2DKmwctWq5zwPWRMRdEbERWAKc2mT7M4Bv9qs4MxsN\n",
"EkcCJwFPAZe02Pzf8/JthRY15lqF+yxgbd36uvzcViTtALwTuLQ/pZnZCPlkXv59BI+02PZHeel+\n",
"9wK1CvdOxkm+G/i5u2TMxovEnsCZefUrbXzLVcCzwFESexVW2Jib2eL19cDsuvXZpNb7VD5Aiy4Z\n",
"SYvrVicjYrLF+5vZ8PsYsD2wLILbWm0cwbMSV5Ja7hPA/ym2vNEiaYL079LbfppdxCRpJnAb6Yew\n",
"gXQiZEFErGzYblfgDmDfiHhmiv34IiazipK4DjgW+O0Ivt/m95wD/AXw1xEsLLK+UdZLdjZtuUfE\n",
"JkmLgOWks9uXRMRKSQvz6xflTd8LLJ8q2M2suiS2B44CXgR+0sG3+qRqwTz9gJl1TeK3SH3ot0Rw\n",
"ZAffNxN4iHQ16/4R3FNQiSPN0w+YWVmOy8trO/mmPA5+Mq+69V4Ah7uZ9aKrcM88JLJADncz60Ut\n",
"3K/p4ntr/e5vl3CXbZ+5z93MuiKxK/Ao8DywcwTPd/j9An4D7AUclmeOtDruczezMrw+L2/qNNgB\n",
"IgjcNVMYh7uZdauX/vaaWrj7pGqfOdzNrFu99LfX1Prd3yoxo8d6rI7D3cy6NS8ve2m53wncDewO\n",
"vK7nimwzh7uZdUxib9JcU09C6/lkppP73X21agEc7mbWjVqXzHURvNDjvnxStQAOdzPrRq1Lppf+\n",
"9ppauJ/ofvf+cbibWTf6MVIGgAjuJU0lvgNwQK/7s8ThbmYdyRcf9S3cs1vy8og+7W/sOdzNrFNz\n",
"gFcAD5JGuvSDw73PHO5m1qnN/e15tEs/3JqXDvc+cbibWaf63SUDbrn3ncPdzDpVRLjXWu6H5ht5\n",
"WI8c7mbWtjxUsTZhWN/CPYLHgbXAtsCB/drvOHO4m1knDgN2BO6O4P4+79tdM33UMtwlzZe0StJq\n",
"SWdPs82EpBsk/UrSZN+rNLNhUUSXTI3DvY+a9m1JmgFcAJwErAeulbQ0IlbWbbMb8DXgnRGxTtKe\n",
"RRZsZqUaRLgfXsC+x06rlvs8YE1E3BURG4ElwKkN25wBXBoR6wAi4sH+l2lmQ6Kf0w40csu9j1qF\n",
"+yzSSY6adfm5enOBPST9WNIKSWf2s0AzGw4S2wFHAQFcV8Bb1EbMHOIRM71r9Q/YzgUK2wDHkmZ0\n",
"2wG4StLVEbG6cUNJi+tWJyNiss06zax8R5N+31dG8ES/dx7BkxJ3A/sDB8F43lNV0gQw0et+WoX7\n",
"etKczTWzSa33emuBByPiGeAZST8l/SfYKtwjYnH3pZpZyY7Oy+sLfI9bSOF+BGMa7rnROwkg6dxu\n",
"99OqW2YFMFfSHEnbAqcDSxu2+S5wgqQZknYAjmfLxyszq47D8vKWplv1xv3ufdK05R4RmyQtApYD\n",
"M4BLImKlpIX59YsiYpWkHwC/BF4ELo4Ih7tZ9dRGsaxsulVvHO59ooh+zfvT5E2kAIgIFf5mZlaI\n",
"3B++H3BoRPe31mvxHm8gDbO8JYIji3iPUdJLdjrczawliZ2AJ4CNwA4RbCrofXYk3Zd1I7BjBBuL\n",
"eJ9R0Ut2evoBM2vHIXl5e1HBDhDBU8BdpFE5BxX1PuPA4W5m7RhEf3uN+937wOFuZu2ojZQZxGAJ\n",
"h3sfONzNrB21cHfLfUQ43M2sHe6WGTEeLWNmTUlsCzwNCNgpgmcKfr8dSCNmXiCNmHm+yPcbZh4t\n",
"Y2ZFmku6iPHOooMdIIKngTtJF1nOLfr9qsrhbmatDLK/vcZdMz1yuJtZK7X+9kFOK+Jw75HD3cxa\n",
"KbPl7rsydcnhbmatuFtmBHm0jJlNS2IG8BSwHbBbBI8N6H1fThox8yJjPGLGo2XMrChzSMG+flDB\n",
"DpBH5dxBGjFz8KDet0oc7mbWTBldMjXumumBw93MmhnklamNHO49cLibWTODnDCsUe0PyiFNt7Ip\n",
"OdzNrJkyu2Vuz0v3uXfB4W5mU5IQ5Yb76rycm2uxDrQMd0nzJa2StFrS2VO8PiHpMUk35K8vFFOq\n",
"mQ3Yq4FdgIeABwb95hE8AjwI7Ai8atDvP+pmNntR0gzgAuAkYD1wraSlEdH4V/wnEfGegmo0s3Js\n",
"brVHUPwFMVO7HdiT1DWzoaQaRlKrlvs8YE1E3BURG4ElwKlTbOePTGbVU2aXTE2ta8b97h1qFe6z\n",
"gLV16+vyc/UCeJOkmyRdJslzQZhVQxkThjWqnVT11L8datotA219FLsemB0RT0s6BfgO0/yVlbS4\n",
"bnUyIibbKdLMSjEMLfexGzEjaQKY6HU/rcJ9PTC7bn02qfW+WUQ8Ufd4maS/krRHRDzcuLOIWNxD\n",
"rWY2WA73EuRG7ySApHO73U+rbpkVwFxJcyRtC5wOLK3fQNLekpQfzyNNRrZVsJvZ6JB4BbAXafKu\n",
"tS02L9KavDwwT2JmbWraco+ITZIWActJt9m6JCJWSlqYX78I+B3gDyRtIt1n8QMF12xmxau12leV\n",
"OFKGCJ6WWAfsC+xPmkzM2tCqW4aIWAYsa3juorrHXwO+1v/SzKxEw9AlU3M7KdwPxuHeNl+hamZT\n",
"KXNOmUYeDtkFh7uZTaXM2SAbeThkFxzuZjaVYeuWAbfcO+JwN7OXkNgR2A/YyHD0cbtbpgsOdzNr\n",
"VAvR1RFsKrWS5E7gBWB/ie3KLmZUONzNrNGhebmq1CqyfHPsO0lzWB1Ycjkjw+FuZo1q4T4M/e01\n",
"7prpkMPdzBoNVcs984iZDjnczazRMIe7W+5tcrib2WZ5/pbaDalvK7OWBu6W6ZDD3czq7Q9sB6yP\n",
"4IlWGw+QW+4dcribWb1h7JKBNDPlc8A+EjuXXcwocLibWb2hDPcIXmRL14xPqrbB4W5m9YYy3DP3\n",
"u3fA4W5m9YY53D0csgMOdzOrNwrh7pZ7GxzuZgZsvrXeK0m31ltfcjlTcbdMBxzuZlazudVe5q31\n",
"mtjccpdQqZWMAIe7mdUMc5cMwP3A48BuwCtKrmXotQx3SfMlrZK0WtLZTbY7TtImSaf1t0QzG5Ch\n",
"Dvf8acJdM21qGu6SZgAXAPNJt91aIOmwabb7EvAD8MclsxE11OGe+aRqm1q13OcBayLirojYCCwB\n",
"Tp1iu08A3wIe6HN9ZjY4oxTuHg7ZQqtwn0W67LdmXX5uM0mzSIF/YX5qGE/EmFkT+Q5HBwAvAmtK\n",
"LqcZd8u0aWaL19sJ6vOBz0VESBJNumUkLa5bnYyIyTb2b2bFO4jU2FsTwXNlF9NE5btlJE0AE73u\n",
"p1W4rwdm163PJrXe670eWJJynT2BUyRtjIiljTuLiMXdl2pmBRqFLhnY0nI/SOJlec6ZSsmN3kkA\n",
"Sed2u59W4b4CmCtpDrABOB1Y0FDIAbXHkr4BfG+qYDezoVYbKDFMt9bbSgSPSjxAutiqsdvY6jTt\n",
"c4+ITcAiYDlwK/AvEbFS0kJJCwdRoJkNxKi03GHLTUQOabrVmGvVcicilgHLGp67aJptP9Knusxs\n",
"sEYp3FcBJ5Bq/mHJtQwtX6FqNubypfy1cB+mW+tNp9Z1dGjTrcacw93MZgE7Ag9E8FDZxbSh9unC\n",
"4d6Ew93MRqlLBhzubXG4m9mohfvdpPupzvL9VKfncDezkQr3CF5gy8VMbr1Pw+FuZrUx7iMR7pm7\n",
"ZlpwuJvZSLXcM4d7Cw53szEmsQvwauBZUl/2qPBwyBYc7mbjrXaV5+25L3tUuOXegsPdbLyNYpcM\n",
"1M3rLrW+0n4cOdzNxtvheTlS4R7BU8A9wDakeeitgcPdbLwdlZc3l1pFd9w104TD3Wy8vTYvf1lq\n",
"Fd1xuDfhcDcbUxK7k27A8wzw65LL6YZHzDThcDcbX0fm5S0jNlKmxi33JhzuZuNrlPvboS7c87TF\n",
"Vsfhbja+Rrm/HeA+4DFgd2CvkmsZOg53s/E10i33CAJ3zUzL4W42hiRexpY+91FtuYPDfVotw13S\n",
"fEmrJK2WdPYUr58q6SZJN0i6TtLbiinVzPpof2Bn4L4IHii7mB443KfR9LJdSTOAC4CTgPXAtZKW\n",
"RsTKus1+GBHfzdu/Fvg2cFBB9ZpZf9T620eyS6aOh0NOo1XLfR6wJiLuioiNwBLg1PoNIuKputWd\n",
"gAf7W6KZFWDUT6bW1FruhzXdagy1CvdZwNq69XX5uZeQ9F5JK4FlwCf7V56ZFWSkT6bWuQPYBOwv\n",
"sUPZxQyTVrOpRTs7iYjvAN+RdCLwj2yZRvQlJC2uW52MiMl29m9mfVeJlnsEGyXWkLplDgZuLLmk\n",
"nkmaACZ63U+rcF9Pujy5Zjap9T6liPiZpJmSXhERD03x+uKuqjSzvpHYnhSEL7Klz3qUrSKF+6FU\n",
"INxzo3cSQNK53e6nVbfMCmCupDmStgVOB5bWbyDpQEnKj4/NxW0V7GY2NA4DZpBu0PFM2cX0gUfM\n",
"TKFpyz0iNklaBCwn/We4JCJWSlqYX78IeD/wIUkbgSeBDxRcs5n1pir97TUO9ym0vINJRCwjnSit\n",
"f+6iusdfBr7c/9LMrCCV6G+vU+ta8oiZOr5C1Wz8VK3lflteHiwxo9RKhojD3Wz8VKrlHsFjwL3A\n",
"9sB+JZczNBzuZmNE4pXAPqTzY3eXXE4/ud+9gcPdbLxsnnYgghdLraS/HO4NHO5m46Vq/e01DvcG\n",
"Dnez8VKp/vY6tREzR5RaxRBxuJuNl6q23G/Ky9fluerHnv8RzMZEHiZYa9lWKtwjuJ80NcqOwNyS\n",
"yxkKDnez8XEg8HJgXQSPlF1MAa7Py2NLrWJIONzNxkdVbtAxHYd7HYe72fio9bdX7WRqjcO9jsPd\n",
"bHyMTctdQqVWMgQc7mbjo+ot9w3AA8BuwJxySymfw91sDEjsBBxAuiXdbS02H0kRBFta78eUWcsw\n",
"cLibjYfjAQE3RvB82cUUyP3umcPdbDy8KS//X6lVFM/hnjnczcZDLdyvLLWK4tXC/fXjflJVEVH8\n",
"m0gBEBFj/Y9tVoZ8Of7DwK7A7Ijpb3I/6nKgP0I61lkRbCi5pJ70kp1uuZtV3+GksFtb5WCHrU6q\n",
"jnXXTFvhLmm+pFWSVks6e4rXPyjpJkm/lHSlpKOm2o+ZlWJcumRqHO60Ee6SZgAXAPNJLYAFkhpv\n",
"RHsH8JaIOAr4U+Cv+12omXXtzXlZ9ZOpNR4OSXst93nAmoi4KyI2AkuAU+s3iIirIuKxvPoLYN/+\n",
"lmlmPRiXkTI1brnTXrjPAtbWra/Lz03n94HLeinKzPpDYi/gIOBptsx5XnWrgaeA/ST2LLuYssxs\n",
"Y5u2h9NIeivwUbZ8DGx8fXHd6mRETLa7bzPrSq3V/osINpVayYBE8ILEjaQcOga4ouSSOiJpApjo\n",
"dT/thPt6YHbd+mzY+ox7Pol6MTA/IqacKzoiFndRo5l1b9y6ZGpuIIX7sYxYuOdG7ySApHO73U87\n",
"3TIrgLmS5kjaFjgdWFq/gaT9gH8Dfjci1nRbjJn13biNlKkZ+373li33iNgkaRGwHJgBXBIRKyUt\n",
"zK9fBPwJsDtwoSSAjRExr7iyzawVie2AN+TVq8uspQRjH+6+QtWsoiTeSOqOuTVi871Tx4LENsCT\n",
"wLbArhE8XnJJXfEVqmY2lXHtkiGCjWyZt/51ZdZSFoe7WXWN28VLjca6a8bhblZBeQKtcR0pU+Nw\n",
"N7PKOQDYG3iQdFHPOHK4m1nlbG6155kSx9HNwAvAYRI7lF3MoDnczapp3LtkiOBZ4BZSzh1dcjkD\n",
"53A3q6baydSxGynT4Jq8fEupVZTA4W5WMRK7AkcCG4HrSi6nbJfn5TtLraIEDnez6jkeEHB9BM+U\n",
"XUzJfgi8CJwgsVPZxQySw92setwlk0XwCKlrZhv6MNPiKHG4m1VPrQviZ6VWMTyW5+VYdc043M0q\n",
"RGI2qVvmGUZsqtsCOdzNbOSdlpfLIniq1EqGx7XAo8BciQPKLmZQHO5m1fL+vPxWqVUMkXwHqh/m\n",
"1bFpvTvczSpCYh/gBOB54PsllzNsxq5rxuFuVh3vIw2BvHxU5y8vUC3c35bneq88h7tZdbhLZhoR\n",
"rAVWAjsDbyy5nIFwuJtVgMSepHHcm2i4x7FtNlZdM22Fu6T5klZJWi3p7CleP1TSVZKelfTp/pdp\n",
"Zi2cSrrH8b/nC3dsaw73epJmABcA84HDgQWSDmvY7CHgE8B5fa/QzNpR65K5tNQqhttPgeeAYyVe\n",
"WXYxRWun5T4PWBMRd0XERmAJqZWwWUQ8EBErSBMVmdkASewGnESaQ+U7JZcztCJ4mhTwAt5RcjmF\n",
"ayfcZwFr69bX5efMbDi8mzR3yk8ieKDsYobc2HTNtBPu43oXF7NR4S6Z9tXC/eR8n9nKmtnGNuuB\n",
"2XXrs0mt945JWly3OhkRk93sx8wSiZ1J58MAvl1mLSPiFlKmzQKOAm4qt5ytSZqgDzNYthPuK4C5\n",
"kuYAG4DTgQXT1dVsRxGxuIPazKy1dwHbAVdGsKHsYoZdBCFxOfAR0h/FoQv33OidBJB0brf7adkt\n",
"ExGbgEWkjzO3Av8SESslLZS0MBewj6S1wB8CX5B0j6SxmhjfrCTukuncWPS7K6L4LnVJARARle7j\n",
"MhskiZcDDwA7AvtHcE/JJY0EiT2Ae0knoedG8OuSS5pWL9npK1TNRtfvkYL9Ggd7+yJ4GPjfpG7k\n",
"T5VcTmHccjcbQbnVvgZ4NfA7Ee6W6YTEa4FfAk8Ds3PgDx233M3Gz0JSsN+IR8l0LIKbgcuBHUj/\n",
"lpXjlrvZiJHYEbgD2At4TwTfK7mkkSRxMunk6r3AnAieL7mkrbjlbjZeFpGC/Rrg/5Zcyyi7ArgZ\n",
"eBXTD+8eWQ53sxEisQvw2bz6xxG+grxb+d/uf+bVT1ftilWHu9loOQvYA/g5qeVpvfkm8BvgtVRs\n",
"MjGHu9mIkNgdqN0v4QtutfcugueAr+bVSt2LwuFuNjo+DexKuiHHT8oupkK+ThoSeXIeIlkJDnez\n",
"EZBvLnFWXv3jMmupmjzG/Rt59Y/KrKWfHO5mo+HzwE7AZRFcVXYxFXQ+aXrzD0q8quxi+sHhbjbk\n",
"JD5Eukw+gD8puZxKimAN6S5W2wBflUY/G0f+AMyqLF9oc0le/VQE15VZT8WdAzxOmmlz5Lu+fIWq\n",
"2ZCSOIZ0z8+dgPMi+K8ll1R5EqeQLgx7GUMwZ4+vUDWrGIk5wGWkYF8CnF1qQWMigmVsuUjsH/If\n",
"2JHklrvZkMnzjV8JHAr8GDglj8e2AchXqv4taUrltcBxEdxXTi1uuZtVgsSuwFJSsP8KOM3BPlj5\n",
"4rCPA1eR7hn9bYntyq2qcw53syEgIYkzgduAN5NuQn9KBI+WW9l4yn9QTyO13N8IfCPPxjkyHO5m\n",
"JZM4mnTi9B+AvYGrgZMiWFdqYWMugt8Ap5KuXl0ArJL4wKhMMNYy3CXNl7RK0mpJU57UkfSV/PpN\n",
"kkb2BITZIEnsLvEV4HrgBNL9UD8CvDmC20otzgCI4AbgraSf0b6kicZ+IvG6UgtrQ9NwlzQDuACY\n",
"DxwOLJB0WMM27wIOioi5wMeACwuqdahJmii7hiJV+fgGeWwScyXOklhOmo3wE/mlrwAHR/B3EbzY\n",
"3/es7s8Oij++CK4B5pHy7UHgROA6iQsljhjWlnyrlvs8YE1E3BURG0lDsk5t2OY9wN8DRMQvgN0k\n",
"7d33SoffRNkFFGyi7AIKNNHvHUpsK3GQxDskPibxFYnVwO2kS91PJl0N+UPgmAjOKrB/faKg/Q6L\n",
"iaLfIIIXIrgYmMuWqQo+TjrpfZ/Ev0oskjhyWK5undni9VmkEwo164Dj29hmXyhn6NAgSMwE3vfS\n",
"Z//D4RL/sZSCBmJkj2+6VpW2LN96pLT5TjwiNXpqX7X1bab42g7YGdilYTmL9Dsw1S/5w8APSGPY\n",
"l0fwYC8HZ4OV/wD/ocTFwOeAt5NvUp6/AJ6U2ED6ZFb/9TjwHPBs3ddzwAvAi/mr9nhTBNf2Umur\n",
"cG93EHzjL9CU31cbs1ldGsXw60CVj0/vH9Ab7QGckb/QgD7QSzp3MO9UjiE7vp2Ag/NX13r9v9Hq\n",
"48N60jjPmtmw1Rn8xm32zc+ZmVlJWrXcVwBzJc0BNgCns/WNZJeSbti7RNJvAY9GxEu6ZHxlqpnZ\n",
"YDUN94jYJGkRsByYAVwSESslLcyvXxQRl0l6l6Q1wFOkoVxmZlaigcwtY2Zmg1XYkB1Je0i6QtLt\n",
"ki6XtNsU28yW9GNJt0j6laRPFlVPP1T9gq5Wxyfpg/m4finpSklHlVFnt9r5+eXtjpO0SdJpg6yv\n",
"V23+/5yQdEP+fZsccIk9aeP/556SfiDpxnx8v1dCmV2R9LeS7pN0c5NtOsuWiCjkC/gy8Nn8+Gzg\n",
"i1Nssw/wuvx4J9K8GocVVVOPxzMDWAPMIQ2Du7GxVuBdwGX58fHA1WXX3efjeyOwa348v2rHV7fd\n",
"j0hzer+/7Lr7/PPbDbgF2Dev71l23X0+vsXAX9aODXgImFl27W0e34nAMcDN07zecbYUOdh+88VN\n",
"efnexg0i4jcRcWN+/CSwkjRmdBhV/YKulscXEVdFxGN59RekkVGjop2fH6QrRr9FmgpglLRzfGcA\n",
"l0bEOoCIGKUx9u0c372kaw3Iy4ciYtMAa+xaRPwMeKTJJh1nS5HhvndsGTVzH2lCpGnlETnHkEJj\n",
"GE11sdasNrYZlQBs5/jq/T7pQpxR0fL4JM0iBUZtCo1ROiHVzs9vLrBH7gpdIenMgVXXu3aO72Lg\n",
"CEkbgJuAswZU2yB0nC2thkI2JekKUtdKo/9WvxIR0ewCJkk7kVpLZ+UW/DDq6wVdQ6jtOiW9Ffgo\n",
"aWraUdHO8Z0PfC7/fxXTX906jNo5vm2AY0lXVe4AXCXp6ohYXWhl/dHO8X0euDEiJiQdCFwh6eiI\n",
"eKLg2galo2zpKdwj4h3TVpFODuwTEb+R9Crg/mm22wa4FPiniPhOL/UUrOoXdLVzfOSTqBcD8yOi\n",
"2cfIYdPO8b2edL0GpD7bUyRtjIilgymxJ+0c31rgwYh4BnhG0k+Bo4FRCPd2ju9NwJ8DRMSvJd0J\n",
"HEK6XmfUdZwtRXbLLAU+nB9/GNgquHPr6BLg1og4v8Ba+mHzBV2StiVd0NX4S78U+BDAdBd0DbGW\n",
"xydpP+CLyZN7AAABAUlEQVTfgN+NiDUl1NiLlscXEQdExGsi4jWkT5J/MCLBDu39//wucIKkGZJ2\n",
"IJ2Yu3XAdXarneNbBZwEkPujDwHuGGiVxek8Wwo8+7sHaca724HLgd3y868Gvp8fn0CaJOdG4Ib8\n",
"Nb/sM9dNjukU0oieNcA5+bmFwMK6bS7Ir98EHFt2zf08PuBvSCMQaj+ra8quud8/v7ptvwGcVnbN\n",
"/T4+4DOkETM3A58su+Z+Hh/p09b38u/ezcAZZdfcwbF9kzQLwPOkT1gf7TVbfBGTmVkFDcW8w2Zm\n",
"1l8OdzOzCnK4m5lVkMPdzKyCHO5mZhXkcDczqyCHu5lZBTnczcwq6P8D4igGjQtUaXMAAAAASUVO\n",
"RK5CYII=\n"
],
"text/plain": [
""
]
},
"metadata": {},
"output_type": "display_data"
}
],
"source": [
"a = 1.0 # advection speed\n",
"\n",
"m = 50 # number of cells\n",
"dx = 1./m # Size of 1 grid cell\n",
"x = np.arange(-dx/2, 1.+dx/2, dx) # Cell centers, including ghost cells\n",
"\n",
"t = 0. # Initial time\n",
"T = 0.5 # Final time\n",
"dt = 0.8 * dx / a # Time step\n",
"\n",
"Q = np.exp(-200*(x-0.2)**2) # Initial data\n",
"Qnew = np.empty(Q.shape)\n",
"\n",
"while t < T:\n",
" \n",
" # Extrapolation at boundaries:\n",
" Qnew[0] = Q[1]\n",
" Qnew[-1] = Q[-2]\n",
" \n",
" for i in range(1,len(x)):\n",
" Qnew[i] = Q[i] - a*dt/dx * (Q[i]-Q[i-1])\n",
" \n",
" Q = Qnew.copy()\n",
" t = t + dt\n",
" \n",
"plt.plot(x,Q,linewidth = 2)\n",
"plt.title('t = '+str(t));"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Notice how we set up a grid that contains an extra cell at each end, outside of the problem domain $[0,1]$. These are called **ghost cells** and are often useful in handling the solution at the grid boundaries."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
""
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
" The technique we have used to set the ghost cell values above, by copying the last value inside the grid to the ghost cells, is known as **zero-order extrapolation**. It is useful for allowing waves to pass out of the domain (so-called *non-reflecting* boundaries). Note that we don't actually need the ghost cell at the right end for the upwind method, but for other methods we will."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"The upwind method is simple, but it is not very accurate. Notice how the computed solution becomes wider and shorter over time. This behavior is referred to as *dissipation*."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"### Exercise"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Now do the following with the code above:\n",
"\n",
"1. Set $m=1000$ or more and notice that it takes some time to compute the solution. Rewrite the inner loop (over $i$) as a single line with no loop, using numpy slicing. For large values of $m$, the code with slicing is much faster.\n",
"1. Notice that the last step of the simulation goes past time $T$. Modify the code so that the last step is adjusted to exactly reach $T$.\n",
"2. Change the code so that animation of the solution versus time is plotted. You will want to accumulate frames of the solution in a list and then use the same kind of code we used above to animate the exact solution.\n",
"3. Add some code to plot the exact solution.\n",
"\n",
"*Extra credit*: change the left boundary condition so that there is a sinusoidal wave coming in from the left:\n",
"$$u(0,t) = \\sin(20 \\pi t).$$\n",
"What do you notice about the sinusoid as it moves into the domain?"
]
},
{
"cell_type": "code",
"execution_count": null,
"metadata": {
"collapsed": false
},
"outputs": [],
"source": []
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"After making it through the exercise above, you should feel pretty comfortable with the basics of scientific programming in Python."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"## The CFL condition"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Take a look at the line of code that sets the time step:\n",
"```python\n",
" dt = 0.8 * dx / a \n",
"```\n",
"You might be wondering where that formula came from. Rearranging that equation, we have\n",
"$$a \\frac{\\Delta t}{\\Delta x} = 0.8.$$\n",
"The quantity $\\nu = a \\frac{\\Delta t}{\\Delta x}$ is the distance the exact solution moves during each time step, in units of grid cells. It is referred to as the *CFL number* or just the *Courant number* after the authors Courant, Friedrichs and Lewy who [established its importance](http://www.stat.uchicago.edu/~lekheng/courses/302/classics/courant-friedrichs-lewy.pdf). Try the following values of $\\nu$ in the code above, and compare the results with those you obtained already using $\\nu=0.8$.\n",
"1. $\\nu = 1.0$\n",
"2. $\\nu = 1.5$\n",
"3. $\\nu = 0.1$\n",
"\n",
"Finally, try setting $a$ to a negative value. What happens?"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"The results you have observed can be explained as follows. Over a time step of size $\\Dt$, the solution moves by an amount $a \\Dt$. So $q(x_i,t_n)$ should be given exactly by $q(x_i-a\\Dt,t_{n-1})$. This is referred to as the *domain of dependence* of the solution.\n",
"\n",
"The upwind method uses the values $Q_i^{n-1}$ and $Q_{i-1}^{n-1}$ to compute $Q_i^n$. The points $(x_{i-1},t_n)$ and $(x_i,t_n)$ (as well as the locations of solution values they depend, and the ones those depend on, and so forth) are the *numerical domain of dependence*.\n",
"\n",
"For this to work, the true domain of dependence $x_i-a\\Dt$ must lie within the numerical domain of dependence, as $\\Dt,\\Dx \\to 0$. This is known as the [CFL condition](http://en.wikipedia.org/wiki/Courant%E2%80%93Friedrichs%E2%80%93Lewy_condition).\n",
"\n",
"For the upwind method, that means that we must have\n",
"$$x_i - \\Dx \\le x_i - a \\Dt \\le x_i$$\n",
"or in other words\n",
"$$0 \\le a \\frac{\\Dt}{\\Dx} \\le 1.$$\n",
"\n",
"If the CFL condition is violated, the information that is used by the numerical method doesn't include the true information that influences the exact solution, so the numerical solution cannot be convergent."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"## The Lax-Friedrichs method"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"The upwind method gets its name from the fact that it uses the value $U_{i-1}$ and not $U_{i+1}$. Assuming $a>0$, the correct solution value $U_i^{n+1}$ should come from a point to the left of $x_i$ at time $t_n$ (i.e., the wind blows to the right, so $x_{i-1}$ is *upwind* of $x_i$).\n",
"\n",
"This bias is fine for the advection equation, where we know everything moves in the same direction. But for more complicated conservation laws, things may move in either direction. It will be useful to have a method that uses information from both directions. The simplest such method is known as the **Lax-Friedrichs** method. For the conservation law $q_t + f(q)_x$, this method is\n",
"\n",
"$$Q_i^{n+1} = \\frac{1}{2}(Q_{i-1}^n + Q_{i+1}^n) - \\frac{\\Dt}{2\\Dx}\\left(f(Q_{i+1}^n) - f(Q_{i-1}^n)\\right).$$\n",
"\n",
"Notice that the flux difference term clearly approximates $f(q)_x$. Meanwhile, the value of $q$ itself is approximated by taking the average of two neighboring values. This average makes this method dissipative too (but it ensures that the solution is stable)."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"### Exercise"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"1. What does the CFL condition imply for the time step when using the Lax-Friedrichs method?\n",
"\n",
"2. In the cell below, implement the Lax-Friedrichs method for advection."
]
},
{
"cell_type": "code",
"execution_count": null,
"metadata": {
"collapsed": false
},
"outputs": [],
"source": []
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"*Extra credit*: Compute the norm of the difference between the approximate and exact solution. How does it change if you decrease $\\Dx$?"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"## Accuracy"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"The methods we have used so far (i.e., the *upwind method* and the *Lax-Friedrichs method*) are both dissipative. Furthermore, both of these methods are only *first order accurate*, meaning that if we reduce the values of $\\Dt$ and $\\Dx$ by a factor of two, the overall error decreases only by a factor of two. In [Lesson 3](Lesson_03_High-resolution_methods.ipynb), we will learn about more accurate methods. But first, in [Lesson 2](Lesson_02_Traffic.ipynb) we'll look at a model for traffic flow."
]
}
],
"metadata": {
"kernelspec": {
"display_name": "Python 2",
"language": "python",
"name": "python2"
},
"language_info": {
"codemirror_mode": {
"name": "ipython",
"version": 2
},
"file_extension": ".py",
"mimetype": "text/x-python",
"name": "python",
"nbconvert_exporter": "python",
"pygments_lexer": "ipython2",
"version": "2.7.9"
}
},
"nbformat": 4,
"nbformat_minor": 0
}