{ "cells": [ { "cell_type": "markdown", "metadata": {}, "source": [ "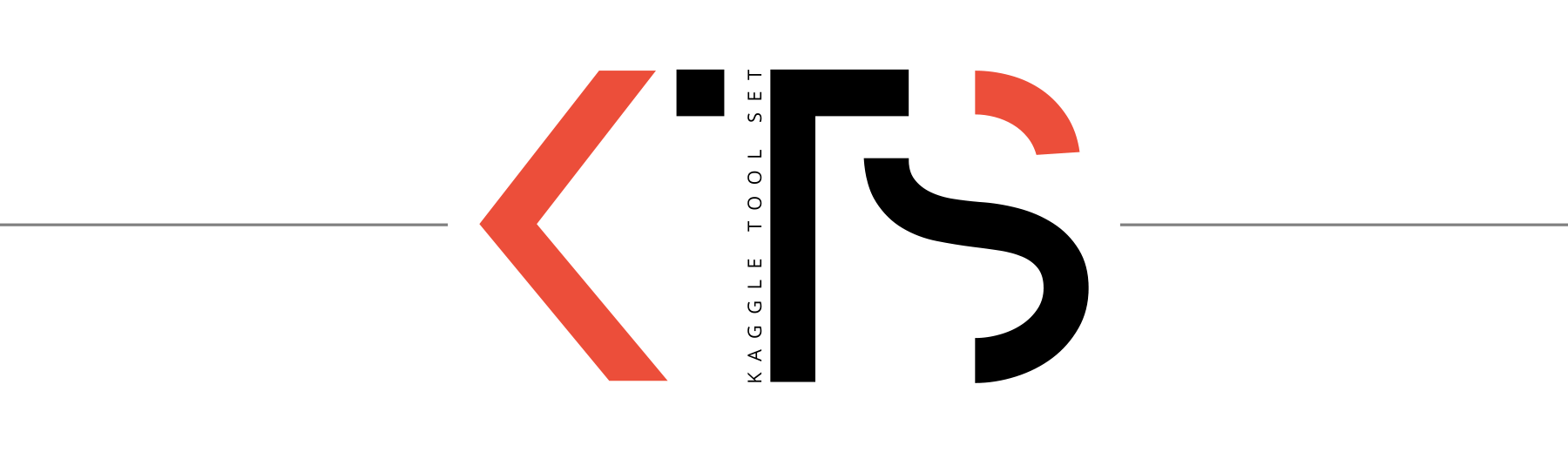\n", "# Stacking Guide" ] }, { "cell_type": "code", "execution_count": 1, "metadata": {}, "outputs": [ { "data": { "text/html": [ "
stack(experiment_id, noise_level, random_state)\n", "
>>> stl.stack('ABCDEF')\n", ">>> stl.stack('ABCDEF', noise_level=0.3, random_state=42)\n", ">>> stl.concat([stl.stack('ABCDEF'), stl.stack('GHIJKL')])\n", "
\n", " | KPBVAI | \n", "
---|---|
PassengerId | \n", "\n", " |
1 | \n", "0.109578 | \n", "
2 | \n", "0.985013 | \n", "
3 | \n", "0.635773 | \n", "
4 | \n", "0.906962 | \n", "
5 | \n", "0.132054 | \n", "
6 | \n", "0.124416 | \n", "
7 | \n", "0.322831 | \n", "
8 | \n", "0.599516 | \n", "
9 | \n", "0.520683 | \n", "
10 | \n", "0.922890 | \n", "
\n", " | KPBVAI | \n", "
---|---|
PassengerId | \n", "\n", " |
892 | \n", "0.150248 | \n", "
893 | \n", "0.589290 | \n", "
894 | \n", "0.107736 | \n", "
895 | \n", "0.229384 | \n", "
896 | \n", "0.756447 | \n", "
897 | \n", "0.191558 | \n", "
898 | \n", "0.758887 | \n", "
899 | \n", "0.271479 | \n", "
900 | \n", "0.785848 | \n", "
901 | \n", "0.282044 | \n", "
\n", " | KPBVAI | \n", "
---|---|
PassengerId | \n", "\n", " |
1 | \n", "0.112647 | \n", "
2 | \n", "0.977061 | \n", "
3 | \n", "0.683239 | \n", "
4 | \n", "0.921687 | \n", "
\n", " | KPBVAI | \n", "
---|---|
PassengerId | \n", "\n", " |
1 | \n", "0.077990 | \n", "
2 | \n", "1.005335 | \n", "
3 | \n", "0.609979 | \n", "
4 | \n", "0.933971 | \n", "