{ "cells": [ { "cell_type": "markdown", "id": "d741198e", "metadata": {}, "source": [ "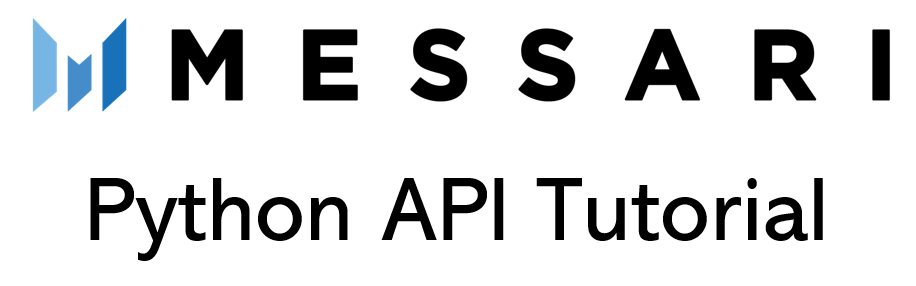" ] }, { "cell_type": "markdown", "id": "likely-radio", "metadata": {}, "source": [ "# Messari Python API Tutorial " ] }, { "cell_type": "markdown", "id": "demographic-pattern", "metadata": {}, "source": [ "This tutorial aims to be a quick guide to get you started using messari's python library." ] }, { "cell_type": "markdown", "id": "driving-disease", "metadata": {}, "source": [ "The first thing is to authenticate your account. You can create an API key by first creating a [messari account](https://messari.io/) and then navigating to https://messari.io/account/api." ] }, { "cell_type": "markdown", "id": "canadian-candy", "metadata": {}, "source": [ "After generating an API, import the library and add your key by running:" ] }, { "cell_type": "code", "execution_count": 1, "id": "daily-maldives", "metadata": {}, "outputs": [], "source": [ "from messari.messari import Messari\n", "messari = Messari('your_api_key')" ] }, { "cell_type": "markdown", "id": "equal-economics", "metadata": {}, "source": [ "## Library Structure" ] }, { "cell_type": "markdown", "id": "accessible-anaheim", "metadata": {}, "source": [ "The library is structured in three main sections represented by three separate modules:\n", "\n", "* Assets\n", "* Makets\n", "* Timeseries" ] }, { "cell_type": "markdown", "id": "eligible-surveillance", "metadata": {}, "source": [ "## Assets Module" ] }, { "cell_type": "markdown", "id": "vanilla-millennium", "metadata": {}, "source": [ "The assets module contains all the functions to pull asset specific data. The functions follow a get_* convention for simplicity of use. The available data functions are:\n", "\n", "* get_all_assets\n", " * Get the paginated list of all assets including metrics and profile.\n", "* get_asset\n", " * Get basic metadata for an asset.\n", "* get_asset_market_data\n", " * Get the latest market data for an asset.\n", "* get_asset_metrics\n", " * Get all the quantitative metrics for an asset.\n", "* get_asset_profile\n", " * Get all the qualitative information for an asset." ] }, { "cell_type": "markdown", "id": "shaped-lodge", "metadata": {}, "source": [ "#### Get all assets" ] }, { "cell_type": "markdown", "id": "german-hampton", "metadata": {}, "source": [ "If you are interested in getting bulk data for all assets that Messari supports use the get_all_assets function. The function returns lots of data so the default returned value is a python dictionary with asset slugs as keys and the asset data as the values.\n", "\n", "The parameters of the function are:\n", " * page: Page number starting at 1. Increment value to paginate through results.\n", " * limit: Limit of assets to return. Default is 20, max value is 500.\n", " * asset_fields: Single filter string or list of fields to filter data. Available fields include: \n", " * id\n", " * name\n", " * slug\n", " * metrics\n", " * profile\n", " \n", " * asset_metric: Single metric string to filter metric data. Available asset metrics include:\n", " * market_data\n", " * marketcap\n", " * supply\n", " * blockchain_stats_24_hours\n", " * market_data_liquidity\n", " * all_time_high\n", " * cycle_low\n", " * token_sale_stats\n", " * staking_stats\n", " * mining_stats\n", " * developer_activity\n", " * roi_data\n", " * roi_by_year\n", " * risk_metrics\n", " * misc_data\n", " * lend_rates\n", " * borrow_rates\n", " * loan_data\n", " * reddit\n", " * on_chain_data\n", " * exchange_flows\n", " * alert_messages\n", " \n", " * asset_profile_metric: Single profile metric string to filter profile data.\n", " * general\n", " * contributors\n", " * advisors\n", " * investors\n", " * ecosystem\n", " * economics\n", " * technology\n", " * governance\n", " * metadata\n", " \n", " * to_dataframe: Return data as pandas DataFrame or JSON. Default is set to JSON." ] }, { "cell_type": "code", "execution_count": 2, "id": "bibliographic-mileage", "metadata": {}, "outputs": [], "source": [ "response_data = messari.get_all_assets()" ] }, { "cell_type": "markdown", "id": "floppy-showcase", "metadata": {}, "source": [ "You can turned the results to a nice pandas DataFrame if the only information you are requesting is **metric data**. \n", "\n", "\n", "NOTE:\n", "**If you include any other asset fields or pass an asset profile metric the function will return an error**." ] }, { "cell_type": "code", "execution_count": 3, "id": "neutral-chambers", "metadata": {}, "outputs": [ { "data": { "text/html": [ "<div>\n", "<style scoped>\n", " .dataframe tbody tr th:only-of-type {\n", " vertical-align: middle;\n", " }\n", "\n", " .dataframe tbody tr th {\n", " vertical-align: top;\n", " }\n", "\n", " .dataframe thead th {\n", " text-align: right;\n", " }\n", "</style>\n", "<table border=\"1\" class=\"dataframe\">\n", " <thead>\n", " <tr style=\"text-align: right;\">\n", " <th></th>\n", " <th>metrics_market_data_price_usd</th>\n", " <th>metrics_market_data_price_btc</th>\n", " <th>metrics_market_data_price_eth</th>\n", " <th>metrics_market_data_volume_last_24_hours</th>\n", " <th>metrics_market_data_real_volume_last_24_hours</th>\n", " <th>metrics_market_data_volume_last_24_hours_overstatement_multiple</th>\n", " <th>metrics_market_data_percent_change_usd_last_1_hour</th>\n", " <th>metrics_market_data_percent_change_btc_last_1_hour</th>\n", " <th>metrics_market_data_percent_change_eth_last_1_hour</th>\n", " <th>metrics_market_data_percent_change_usd_last_24_hours</th>\n", " <th>...</th>\n", " <th>metrics_supply_distribution_supply_in_contracts_usd</th>\n", " <th>metrics_supply_distribution_supply_in_contracts_native_units</th>\n", " <th>metrics_supply_distribution_supply_shielded</th>\n", " <th>metrics_supply_distribution_supply_in_top_100_addresses</th>\n", " <th>metrics_supply_distribution_supply_in_top_10_percent_addresses</th>\n", " <th>metrics_supply_distribution_supply_in_top_1_percent_addresses</th>\n", " <th>metrics_supply_distribution_supply_in_utxo_in_loss</th>\n", " <th>metrics_supply_distribution_supply_in_utxo_in_profit</th>\n", " <th>metrics_alert_messages</th>\n", " <th>slug</th>\n", " </tr>\n", " </thead>\n", " <tbody>\n", " <tr>\n", " <th>bitcoin</th>\n", " <td>63549.138557</td>\n", " <td>1.000000</td>\n", " <td>14.000438</td>\n", " <td>6.696020e+09</td>\n", " <td>5.615102e+09</td>\n", " <td>1.192502</td>\n", " <td>-0.827104</td>\n", " <td>0.000000</td>\n", " <td>-0.262311</td>\n", " <td>-1.232825</td>\n", " <td>...</td>\n", " <td>NaN</td>\n", " <td>NaN</td>\n", " <td>None</td>\n", " <td>2.791041e+06</td>\n", " <td>1.869062e+07</td>\n", " <td>1.729667e+07</td>\n", " <td>272579.562794</td>\n", " <td>1.860070e+07</td>\n", " <td>None</td>\n", " <td>bitcoin</td>\n", " </tr>\n", " <tr>\n", " <th>ethereum</th>\n", " <td>4551.458697</td>\n", " <td>0.071621</td>\n", " <td>1.000000</td>\n", " <td>5.426996e+09</td>\n", " <td>4.641549e+09</td>\n", " <td>1.169221</td>\n", " <td>-0.566278</td>\n", " <td>0.263001</td>\n", " <td>0.000000</td>\n", " <td>-0.543660</td>\n", " <td>...</td>\n", " <td>1.367004e+11</td>\n", " <td>2.965612e+07</td>\n", " <td>None</td>\n", " <td>4.592305e+07</td>\n", " <td>1.169773e+08</td>\n", " <td>1.142389e+08</td>\n", " <td>NaN</td>\n", " <td>NaN</td>\n", " <td>None</td>\n", " <td>ethereum</td>\n", " </tr>\n", " <tr>\n", " <th>binance-coin</th>\n", " <td>631.461714</td>\n", " <td>0.009937</td>\n", " <td>0.138997</td>\n", " <td>1.027663e+09</td>\n", " <td>9.102840e+08</td>\n", " <td>1.128948</td>\n", " <td>-0.784175</td>\n", " <td>0.043287</td>\n", " <td>-0.219138</td>\n", " <td>-2.646269</td>\n", " <td>...</td>\n", " <td>NaN</td>\n", " <td>NaN</td>\n", " <td>None</td>\n", " <td>NaN</td>\n", " <td>NaN</td>\n", " <td>NaN</td>\n", " <td>NaN</td>\n", " <td>NaN</td>\n", " <td>None</td>\n", " <td>binance-coin</td>\n", " </tr>\n", " <tr>\n", " <th>tether</th>\n", " <td>1.002894</td>\n", " <td>0.000016</td>\n", " <td>0.000221</td>\n", " <td>3.636292e+10</td>\n", " <td>2.092726e+10</td>\n", " <td>1.737586</td>\n", " <td>-0.070167</td>\n", " <td>0.763250</td>\n", " <td>0.498937</td>\n", " <td>-0.773392</td>\n", " <td>...</td>\n", " <td>7.379604e+09</td>\n", " <td>7.377586e+09</td>\n", " <td>None</td>\n", " <td>1.730566e+10</td>\n", " <td>3.745281e+10</td>\n", " <td>3.609309e+10</td>\n", " <td>NaN</td>\n", " <td>NaN</td>\n", " <td>None</td>\n", " <td>tether</td>\n", " </tr>\n", " <tr>\n", " <th>solana</th>\n", " <td>234.662429</td>\n", " <td>0.003693</td>\n", " <td>0.051645</td>\n", " <td>1.589884e+09</td>\n", " <td>1.178056e+09</td>\n", " <td>1.349582</td>\n", " <td>-2.070842</td>\n", " <td>-1.254111</td>\n", " <td>-1.513132</td>\n", " <td>-0.639508</td>\n", " <td>...</td>\n", " <td>NaN</td>\n", " <td>NaN</td>\n", " <td>None</td>\n", " <td>NaN</td>\n", " <td>NaN</td>\n", " <td>NaN</td>\n", " <td>NaN</td>\n", " <td>NaN</td>\n", " <td>None</td>\n", " <td>solana</td>\n", " </tr>\n", " </tbody>\n", "</table>\n", "<p>5 rows × 370 columns</p>\n", "</div>" ], "text/plain": [ " metrics_market_data_price_usd metrics_market_data_price_btc \\\n", "bitcoin 63549.138557 1.000000 \n", "ethereum 4551.458697 0.071621 \n", "binance-coin 631.461714 0.009937 \n", "tether 1.002894 0.000016 \n", "solana 234.662429 0.003693 \n", "\n", " metrics_market_data_price_eth \\\n", "bitcoin 14.000438 \n", "ethereum 1.000000 \n", "binance-coin 0.138997 \n", "tether 0.000221 \n", "solana 0.051645 \n", "\n", " metrics_market_data_volume_last_24_hours \\\n", "bitcoin 6.696020e+09 \n", "ethereum 5.426996e+09 \n", "binance-coin 1.027663e+09 \n", "tether 3.636292e+10 \n", "solana 1.589884e+09 \n", "\n", " metrics_market_data_real_volume_last_24_hours \\\n", "bitcoin 5.615102e+09 \n", "ethereum 4.641549e+09 \n", "binance-coin 9.102840e+08 \n", "tether 2.092726e+10 \n", "solana 1.178056e+09 \n", "\n", " metrics_market_data_volume_last_24_hours_overstatement_multiple \\\n", "bitcoin 1.192502 \n", "ethereum 1.169221 \n", "binance-coin 1.128948 \n", "tether 1.737586 \n", "solana 1.349582 \n", "\n", " metrics_market_data_percent_change_usd_last_1_hour \\\n", "bitcoin -0.827104 \n", "ethereum -0.566278 \n", "binance-coin -0.784175 \n", "tether -0.070167 \n", "solana -2.070842 \n", "\n", " metrics_market_data_percent_change_btc_last_1_hour \\\n", "bitcoin 0.000000 \n", "ethereum 0.263001 \n", "binance-coin 0.043287 \n", "tether 0.763250 \n", "solana -1.254111 \n", "\n", " metrics_market_data_percent_change_eth_last_1_hour \\\n", "bitcoin -0.262311 \n", "ethereum 0.000000 \n", "binance-coin -0.219138 \n", "tether 0.498937 \n", "solana -1.513132 \n", "\n", " metrics_market_data_percent_change_usd_last_24_hours ... \\\n", "bitcoin -1.232825 ... \n", "ethereum -0.543660 ... \n", "binance-coin -2.646269 ... \n", "tether -0.773392 ... \n", "solana -0.639508 ... \n", "\n", " metrics_supply_distribution_supply_in_contracts_usd \\\n", "bitcoin NaN \n", "ethereum 1.367004e+11 \n", "binance-coin NaN \n", "tether 7.379604e+09 \n", "solana NaN \n", "\n", " metrics_supply_distribution_supply_in_contracts_native_units \\\n", "bitcoin NaN \n", "ethereum 2.965612e+07 \n", "binance-coin NaN \n", "tether 7.377586e+09 \n", "solana NaN \n", "\n", " metrics_supply_distribution_supply_shielded \\\n", "bitcoin None \n", "ethereum None \n", "binance-coin None \n", "tether None \n", "solana None \n", "\n", " metrics_supply_distribution_supply_in_top_100_addresses \\\n", "bitcoin 2.791041e+06 \n", "ethereum 4.592305e+07 \n", "binance-coin NaN \n", "tether 1.730566e+10 \n", "solana NaN \n", "\n", " metrics_supply_distribution_supply_in_top_10_percent_addresses \\\n", "bitcoin 1.869062e+07 \n", "ethereum 1.169773e+08 \n", "binance-coin NaN \n", "tether 3.745281e+10 \n", "solana NaN \n", "\n", " metrics_supply_distribution_supply_in_top_1_percent_addresses \\\n", "bitcoin 1.729667e+07 \n", "ethereum 1.142389e+08 \n", "binance-coin NaN \n", "tether 3.609309e+10 \n", "solana NaN \n", "\n", " metrics_supply_distribution_supply_in_utxo_in_loss \\\n", "bitcoin 272579.562794 \n", "ethereum NaN \n", "binance-coin NaN \n", "tether NaN \n", "solana NaN \n", "\n", " metrics_supply_distribution_supply_in_utxo_in_profit \\\n", "bitcoin 1.860070e+07 \n", "ethereum NaN \n", "binance-coin NaN \n", "tether NaN \n", "solana NaN \n", "\n", " metrics_alert_messages slug \n", "bitcoin None bitcoin \n", "ethereum None ethereum \n", "binance-coin None binance-coin \n", "tether None tether \n", "solana None solana \n", "\n", "[5 rows x 370 columns]" ] }, "execution_count": 3, "metadata": {}, "output_type": "execute_result" } ], "source": [ "response_data_df = messari.get_all_assets(asset_fields=['metrics'], to_dataframe=True)\n", "response_data_df.head()" ] }, { "cell_type": "markdown", "id": "creative-oracle", "metadata": {}, "source": [ "Additionally, you can filter the metric data by passing an asset metric. For example you can get all the mining statistics for all the assets in the first page. " ] }, { "cell_type": "code", "execution_count": 4, "id": "advance-liability", "metadata": {}, "outputs": [ { "data": { "text/html": [ "<div>\n", "<style scoped>\n", " .dataframe tbody tr th:only-of-type {\n", " vertical-align: middle;\n", " }\n", "\n", " .dataframe tbody tr th {\n", " vertical-align: top;\n", " }\n", "\n", " .dataframe thead th {\n", " text-align: right;\n", " }\n", "</style>\n", "<table border=\"1\" class=\"dataframe\">\n", " <thead>\n", " <tr style=\"text-align: right;\">\n", " <th></th>\n", " <th>slug</th>\n", " <th>metrics_mining_stats_mining_algo</th>\n", " <th>metrics_mining_stats_network_hash_rate</th>\n", " <th>metrics_mining_stats_available_on_nicehash_percent</th>\n", " <th>metrics_mining_stats_1_hour_attack_cost</th>\n", " <th>metrics_mining_stats_24_hours_attack_cost</th>\n", " <th>metrics_mining_stats_attack_appeal</th>\n", " <th>metrics_mining_stats_hash_rate</th>\n", " <th>metrics_mining_stats_hash_rate_30d_average</th>\n", " <th>metrics_mining_stats_mining_revenue_per_hash_usd</th>\n", " <th>metrics_mining_stats_mining_revenue_per_hash_native_units</th>\n", " <th>metrics_mining_stats_mining_revenue_per_hash_per_second_usd</th>\n", " <th>metrics_mining_stats_mining_revenue_per_hash_per_second_native_units</th>\n", " <th>metrics_mining_stats_mining_revenue_from_fees_percent_last_24_hours</th>\n", " <th>metrics_mining_stats_mining_revenue_native</th>\n", " <th>metrics_mining_stats_mining_revenue_usd</th>\n", " <th>metrics_mining_stats_mining_revenue_total</th>\n", " <th>metrics_mining_stats_average_difficulty</th>\n", " </tr>\n", " </thead>\n", " <tbody>\n", " <tr>\n", " <th>bitcoin</th>\n", " <td>bitcoin</td>\n", " <td>SHA-256</td>\n", " <td>175785 PH/s</td>\n", " <td>0.204757</td>\n", " <td>None</td>\n", " <td>None</td>\n", " <td>None</td>\n", " <td>1.753464e+08</td>\n", " <td>1.578817e+08</td>\n", " <td>0.000004</td>\n", " <td>6.500000e-11</td>\n", " <td>0.367195</td>\n", " <td>0.000006</td>\n", " <td>0.890598</td>\n", " <td>990.067517</td>\n", " <td>None</td>\n", " <td>3.554869e+10</td>\n", " <td>2.246731e+13</td>\n", " </tr>\n", " <tr>\n", " <th>ethereum</th>\n", " <td>ethereum</td>\n", " <td>Ethash</td>\n", " <td>812 TH/s</td>\n", " <td>3.804177</td>\n", " <td>None</td>\n", " <td>None</td>\n", " <td>None</td>\n", " <td>8.070931e+02</td>\n", " <td>NaN</td>\n", " <td>0.959108</td>\n", " <td>2.080714e-04</td>\n", " <td>82866.973737</td>\n", " <td>17.977368</td>\n", " <td>78.515707</td>\n", " <td>14509.409289</td>\n", " <td>None</td>\n", " <td>2.431323e+10</td>\n", " <td>1.099016e+16</td>\n", " </tr>\n", " <tr>\n", " <th>binance-coin</th>\n", " <td>binance-coin</td>\n", " <td>None</td>\n", " <td>None</td>\n", " <td>NaN</td>\n", " <td>None</td>\n", " <td>None</td>\n", " <td>None</td>\n", " <td>NaN</td>\n", " <td>NaN</td>\n", " <td>NaN</td>\n", " <td>NaN</td>\n", " <td>NaN</td>\n", " <td>NaN</td>\n", " <td>NaN</td>\n", " <td>NaN</td>\n", " <td>None</td>\n", " <td>NaN</td>\n", " <td>NaN</td>\n", " </tr>\n", " <tr>\n", " <th>tether</th>\n", " <td>tether</td>\n", " <td>None</td>\n", " <td>None</td>\n", " <td>NaN</td>\n", " <td>None</td>\n", " <td>None</td>\n", " <td>None</td>\n", " <td>NaN</td>\n", " <td>NaN</td>\n", " <td>NaN</td>\n", " <td>NaN</td>\n", " <td>NaN</td>\n", " <td>NaN</td>\n", " <td>NaN</td>\n", " <td>NaN</td>\n", " <td>None</td>\n", " <td>NaN</td>\n", " <td>NaN</td>\n", " </tr>\n", " <tr>\n", " <th>solana</th>\n", " <td>solana</td>\n", " <td>None</td>\n", " <td>None</td>\n", " <td>NaN</td>\n", " <td>None</td>\n", " <td>None</td>\n", " <td>None</td>\n", " <td>NaN</td>\n", " <td>NaN</td>\n", " <td>NaN</td>\n", " <td>NaN</td>\n", " <td>NaN</td>\n", " <td>NaN</td>\n", " <td>NaN</td>\n", " <td>NaN</td>\n", " <td>None</td>\n", " <td>NaN</td>\n", " <td>NaN</td>\n", " </tr>\n", " </tbody>\n", "</table>\n", "</div>" ], "text/plain": [ " slug metrics_mining_stats_mining_algo \\\n", "bitcoin bitcoin SHA-256 \n", "ethereum ethereum Ethash \n", "binance-coin binance-coin None \n", "tether tether None \n", "solana solana None \n", "\n", " metrics_mining_stats_network_hash_rate \\\n", "bitcoin 175785 PH/s \n", "ethereum 812 TH/s \n", "binance-coin None \n", "tether None \n", "solana None \n", "\n", " metrics_mining_stats_available_on_nicehash_percent \\\n", "bitcoin 0.204757 \n", "ethereum 3.804177 \n", "binance-coin NaN \n", "tether NaN \n", "solana NaN \n", "\n", " metrics_mining_stats_1_hour_attack_cost \\\n", "bitcoin None \n", "ethereum None \n", "binance-coin None \n", "tether None \n", "solana None \n", "\n", " metrics_mining_stats_24_hours_attack_cost \\\n", "bitcoin None \n", "ethereum None \n", "binance-coin None \n", "tether None \n", "solana None \n", "\n", " metrics_mining_stats_attack_appeal \\\n", "bitcoin None \n", "ethereum None \n", "binance-coin None \n", "tether None \n", "solana None \n", "\n", " metrics_mining_stats_hash_rate \\\n", "bitcoin 1.753464e+08 \n", "ethereum 8.070931e+02 \n", "binance-coin NaN \n", "tether NaN \n", "solana NaN \n", "\n", " metrics_mining_stats_hash_rate_30d_average \\\n", "bitcoin 1.578817e+08 \n", "ethereum NaN \n", "binance-coin NaN \n", "tether NaN \n", "solana NaN \n", "\n", " metrics_mining_stats_mining_revenue_per_hash_usd \\\n", "bitcoin 0.000004 \n", "ethereum 0.959108 \n", "binance-coin NaN \n", "tether NaN \n", "solana NaN \n", "\n", " metrics_mining_stats_mining_revenue_per_hash_native_units \\\n", "bitcoin 6.500000e-11 \n", "ethereum 2.080714e-04 \n", "binance-coin NaN \n", "tether NaN \n", "solana NaN \n", "\n", " metrics_mining_stats_mining_revenue_per_hash_per_second_usd \\\n", "bitcoin 0.367195 \n", "ethereum 82866.973737 \n", "binance-coin NaN \n", "tether NaN \n", "solana NaN \n", "\n", " metrics_mining_stats_mining_revenue_per_hash_per_second_native_units \\\n", "bitcoin 0.000006 \n", "ethereum 17.977368 \n", "binance-coin NaN \n", "tether NaN \n", "solana NaN \n", "\n", " metrics_mining_stats_mining_revenue_from_fees_percent_last_24_hours \\\n", "bitcoin 0.890598 \n", "ethereum 78.515707 \n", "binance-coin NaN \n", "tether NaN \n", "solana NaN \n", "\n", " metrics_mining_stats_mining_revenue_native \\\n", "bitcoin 990.067517 \n", "ethereum 14509.409289 \n", "binance-coin NaN \n", "tether NaN \n", "solana NaN \n", "\n", " metrics_mining_stats_mining_revenue_usd \\\n", "bitcoin None \n", "ethereum None \n", "binance-coin None \n", "tether None \n", "solana None \n", "\n", " metrics_mining_stats_mining_revenue_total \\\n", "bitcoin 3.554869e+10 \n", "ethereum 2.431323e+10 \n", "binance-coin NaN \n", "tether NaN \n", "solana NaN \n", "\n", " metrics_mining_stats_average_difficulty \n", "bitcoin 2.246731e+13 \n", "ethereum 1.099016e+16 \n", "binance-coin NaN \n", "tether NaN \n", "solana NaN " ] }, "execution_count": 4, "metadata": {}, "output_type": "execute_result" } ], "source": [ "metric = 'mining_stats'\n", "response_data_df_market_data = messari.get_all_assets(asset_metric=metric, to_dataframe=True)\n", "response_data_df_market_data.head()" ] }, { "cell_type": "markdown", "id": "determined-longer", "metadata": {}, "source": [ "Lastly, if you are interested in getting metrics for all available assets, you can loop through all pages creating a pandas DataFrame per page then merging them all together!" ] }, { "cell_type": "code", "execution_count": 5, "id": "criminal-briefs", "metadata": {}, "outputs": [ { "name": "stdout", "output_type": "stream", "text": [ "Number of assets in DataFrame 2000\n" ] }, { "data": { "text/html": [ "<div>\n", "<style scoped>\n", " .dataframe tbody tr th:only-of-type {\n", " vertical-align: middle;\n", " }\n", "\n", " .dataframe tbody tr th {\n", " vertical-align: top;\n", " }\n", "\n", " .dataframe thead th {\n", " text-align: right;\n", " }\n", "</style>\n", "<table border=\"1\" class=\"dataframe\">\n", " <thead>\n", " <tr style=\"text-align: right;\">\n", " <th></th>\n", " <th>slug</th>\n", " <th>metrics_marketcap_rank</th>\n", " <th>metrics_marketcap_marketcap_dominance_percent</th>\n", " <th>metrics_marketcap_current_marketcap_usd</th>\n", " <th>metrics_marketcap_y_2050_marketcap_usd</th>\n", " <th>metrics_marketcap_y_plus10_marketcap_usd</th>\n", " <th>metrics_marketcap_liquid_marketcap_usd</th>\n", " <th>metrics_marketcap_volume_turnover_last_24_hours_percent</th>\n", " <th>metrics_marketcap_realized_marketcap_usd</th>\n", " <th>metrics_marketcap_outstanding_marketcap_usd</th>\n", " </tr>\n", " </thead>\n", " <tbody>\n", " <tr>\n", " <th>bitcoin</th>\n", " <td>bitcoin</td>\n", " <td>1</td>\n", " <td>43.232673</td>\n", " <td>1.208326e+12</td>\n", " <td>1.343606e+12</td>\n", " <td>1.322402e+12</td>\n", " <td>1.208853e+12</td>\n", " <td>0.475198</td>\n", " <td>4.571419e+11</td>\n", " <td>1.227371e+12</td>\n", " </tr>\n", " <tr>\n", " <th>ethereum</th>\n", " <td>ethereum</td>\n", " <td>2</td>\n", " <td>19.194453</td>\n", " <td>5.364731e+11</td>\n", " <td>6.177026e+11</td>\n", " <td>5.600073e+11</td>\n", " <td>5.305415e+11</td>\n", " <td>0.896623</td>\n", " <td>2.662798e+11</td>\n", " <td>5.414160e+11</td>\n", " </tr>\n", " <tr>\n", " <th>binance-coin</th>\n", " <td>binance-coin</td>\n", " <td>3</td>\n", " <td>3.788568</td>\n", " <td>1.058881e+11</td>\n", " <td>6.348166e+10</td>\n", " <td>6.877961e+10</td>\n", " <td>6.877961e+10</td>\n", " <td>1.355334</td>\n", " <td>NaN</td>\n", " <td>NaN</td>\n", " </tr>\n", " <tr>\n", " <th>tether</th>\n", " <td>tether</td>\n", " <td>4</td>\n", " <td>2.651714</td>\n", " <td>7.411376e+10</td>\n", " <td>NaN</td>\n", " <td>NaN</td>\n", " <td>NaN</td>\n", " <td>NaN</td>\n", " <td>3.784762e+10</td>\n", " <td>3.784412e+10</td>\n", " </tr>\n", " <tr>\n", " <th>solana</th>\n", " <td>solana</td>\n", " <td>5</td>\n", " <td>2.572619</td>\n", " <td>7.190312e+10</td>\n", " <td>2.241007e+11</td>\n", " <td>1.759675e+11</td>\n", " <td>1.188495e+11</td>\n", " <td>1.020476</td>\n", " <td>NaN</td>\n", " <td>NaN</td>\n", " </tr>\n", " </tbody>\n", "</table>\n", "</div>" ], "text/plain": [ " slug metrics_marketcap_rank \\\n", "bitcoin bitcoin 1 \n", "ethereum ethereum 2 \n", "binance-coin binance-coin 3 \n", "tether tether 4 \n", "solana solana 5 \n", "\n", " metrics_marketcap_marketcap_dominance_percent \\\n", "bitcoin 43.232673 \n", "ethereum 19.194453 \n", "binance-coin 3.788568 \n", "tether 2.651714 \n", "solana 2.572619 \n", "\n", " metrics_marketcap_current_marketcap_usd \\\n", "bitcoin 1.208326e+12 \n", "ethereum 5.364731e+11 \n", "binance-coin 1.058881e+11 \n", "tether 7.411376e+10 \n", "solana 7.190312e+10 \n", "\n", " metrics_marketcap_y_2050_marketcap_usd \\\n", "bitcoin 1.343606e+12 \n", "ethereum 6.177026e+11 \n", "binance-coin 6.348166e+10 \n", "tether NaN \n", "solana 2.241007e+11 \n", "\n", " metrics_marketcap_y_plus10_marketcap_usd \\\n", "bitcoin 1.322402e+12 \n", "ethereum 5.600073e+11 \n", "binance-coin 6.877961e+10 \n", "tether NaN \n", "solana 1.759675e+11 \n", "\n", " metrics_marketcap_liquid_marketcap_usd \\\n", "bitcoin 1.208853e+12 \n", "ethereum 5.305415e+11 \n", "binance-coin 6.877961e+10 \n", "tether NaN \n", "solana 1.188495e+11 \n", "\n", " metrics_marketcap_volume_turnover_last_24_hours_percent \\\n", "bitcoin 0.475198 \n", "ethereum 0.896623 \n", "binance-coin 1.355334 \n", "tether NaN \n", "solana 1.020476 \n", "\n", " metrics_marketcap_realized_marketcap_usd \\\n", "bitcoin 4.571419e+11 \n", "ethereum 2.662798e+11 \n", "binance-coin NaN \n", "tether 3.784762e+10 \n", "solana NaN \n", "\n", " metrics_marketcap_outstanding_marketcap_usd \n", "bitcoin 1.227371e+12 \n", "ethereum 5.414160e+11 \n", "binance-coin NaN \n", "tether 3.784412e+10 \n", "solana NaN " ] }, "execution_count": 5, "metadata": {}, "output_type": "execute_result" } ], "source": [ "import pandas as pd\n", "dfs = [] # list to hold metric DataFrames\n", "for i in range(1, 5, 1): \n", " df = messari.get_all_assets(page=1, limit=500, asset_metric='marketcap', to_dataframe=True)\n", " dfs.append(df)\n", "merged_df = pd.concat(dfs)\n", "print(f'Number of assets in DataFrame {len(merged_df)}')\n", "merged_df.head()" ] }, { "cell_type": "markdown", "id": "permanent-ebony", "metadata": {}, "source": [ "#### Get asset\n", "\n", "The *get_asset* function is very useful when you are trying to get the metadata of an asset. The default value back from this functions is a pandas DataFrame\n", "\n", "The paremeters of the function are:\n", "\n", "* asset_slugs: Single asset slug string or list of asset slugs (i.e. bitcoin).\n", "* asset_fields: Single filter string or list of fields to filter data. Available fields include:\n", " * id\n", " * symbol\n", " * name\n", " * slug\n", "* to_dataframe: Return data as DataFrame or JSON. Default is set to DataFrame." ] }, { "cell_type": "code", "execution_count": 6, "id": "occupied-leader", "metadata": {}, "outputs": [ { "data": { "text/html": [ "<div>\n", "<style scoped>\n", " .dataframe tbody tr th:only-of-type {\n", " vertical-align: middle;\n", " }\n", "\n", " .dataframe tbody tr th {\n", " vertical-align: top;\n", " }\n", "\n", " .dataframe thead th {\n", " text-align: right;\n", " }\n", "</style>\n", "<table border=\"1\" class=\"dataframe\">\n", " <thead>\n", " <tr style=\"text-align: right;\">\n", " <th></th>\n", " <th>id</th>\n", " <th>symbol</th>\n", " <th>name</th>\n", " <th>slug</th>\n", " <th>contract_addresses</th>\n", " <th>_internal_temp_agora_id</th>\n", " </tr>\n", " </thead>\n", " <tbody>\n", " <tr>\n", " <th>bitcoin</th>\n", " <td>1e31218a-e44e-4285-820c-8282ee222035</td>\n", " <td>BTC</td>\n", " <td>Bitcoin</td>\n", " <td>bitcoin</td>\n", " <td>[]</td>\n", " <td>9793eae6-f374-46b4-8764-c2d224429791</td>\n", " </tr>\n", " <tr>\n", " <th>ethereum</th>\n", " <td>21c795f5-1bfd-40c3-858e-e9d7e820c6d0</td>\n", " <td>ETH</td>\n", " <td>Ethereum</td>\n", " <td>ethereum</td>\n", " <td>[{'platform': 'avalanche', 'contract_address':...</td>\n", " <td>263d8b01-5a96-41f0-85d5-09687bbbf7ca</td>\n", " </tr>\n", " <tr>\n", " <th>tether</th>\n", " <td>51f8ea5e-f426-4f40-939a-db7e05495374</td>\n", " <td>USDT</td>\n", " <td>Tether</td>\n", " <td>tether</td>\n", " <td>[{'platform': 'arbitrum-one', 'contract_addres...</td>\n", " <td>1eaf063a-b8dd-4cfb-95dd-a06338565720</td>\n", " </tr>\n", " </tbody>\n", "</table>\n", "</div>" ], "text/plain": [ " id symbol name slug \\\n", "bitcoin 1e31218a-e44e-4285-820c-8282ee222035 BTC Bitcoin bitcoin \n", "ethereum 21c795f5-1bfd-40c3-858e-e9d7e820c6d0 ETH Ethereum ethereum \n", "tether 51f8ea5e-f426-4f40-939a-db7e05495374 USDT Tether tether \n", "\n", " contract_addresses \\\n", "bitcoin [] \n", "ethereum [{'platform': 'avalanche', 'contract_address':... \n", "tether [{'platform': 'arbitrum-one', 'contract_addres... \n", "\n", " _internal_temp_agora_id \n", "bitcoin 9793eae6-f374-46b4-8764-c2d224429791 \n", "ethereum 263d8b01-5a96-41f0-85d5-09687bbbf7ca \n", "tether 1eaf063a-b8dd-4cfb-95dd-a06338565720 " ] }, "execution_count": 6, "metadata": {}, "output_type": "execute_result" } ], "source": [ "assets = ['bitcoin', 'ethereum', 'tether']\n", "asset_metadata = messari.get_asset(asset_slugs=assets)\n", "asset_metadata.head()" ] }, { "cell_type": "markdown", "id": "editorial-process", "metadata": {}, "source": [ "If you are only interested in some fields, you filter the data by passing the asset fields of interest. " ] }, { "cell_type": "code", "execution_count": 7, "id": "offshore-longitude", "metadata": {}, "outputs": [ { "data": { "text/html": [ "<div>\n", "<style scoped>\n", " .dataframe tbody tr th:only-of-type {\n", " vertical-align: middle;\n", " }\n", "\n", " .dataframe tbody tr th {\n", " vertical-align: top;\n", " }\n", "\n", " .dataframe thead th {\n", " text-align: right;\n", " }\n", "</style>\n", "<table border=\"1\" class=\"dataframe\">\n", " <thead>\n", " <tr style=\"text-align: right;\">\n", " <th></th>\n", " <th>id</th>\n", " <th>name</th>\n", " <th>slug</th>\n", " </tr>\n", " </thead>\n", " <tbody>\n", " <tr>\n", " <th>bitcoin</th>\n", " <td>1e31218a-e44e-4285-820c-8282ee222035</td>\n", " <td>Bitcoin</td>\n", " <td>bitcoin</td>\n", " </tr>\n", " <tr>\n", " <th>ethereum</th>\n", " <td>21c795f5-1bfd-40c3-858e-e9d7e820c6d0</td>\n", " <td>Ethereum</td>\n", " <td>ethereum</td>\n", " </tr>\n", " <tr>\n", " <th>tether</th>\n", " <td>51f8ea5e-f426-4f40-939a-db7e05495374</td>\n", " <td>Tether</td>\n", " <td>tether</td>\n", " </tr>\n", " </tbody>\n", "</table>\n", "</div>" ], "text/plain": [ " id name slug\n", "bitcoin 1e31218a-e44e-4285-820c-8282ee222035 Bitcoin bitcoin\n", "ethereum 21c795f5-1bfd-40c3-858e-e9d7e820c6d0 Ethereum ethereum\n", "tether 51f8ea5e-f426-4f40-939a-db7e05495374 Tether tether" ] }, "execution_count": 7, "metadata": {}, "output_type": "execute_result" } ], "source": [ "fields = ['id', 'name']\n", "asset_metadata_filtered = messari.get_asset(asset_slugs=assets, asset_fields=fields)\n", "asset_metadata_filtered.head()" ] }, { "cell_type": "markdown", "id": "solved-toddler", "metadata": {}, "source": [ "#### Get asset profile\n", "\n", "The *get_asset_profile* function can be used to get profile information about an asset. The default value back from this functions is a python dictionary due to the high number of text fileds. \n", "\n", "The paremeters of the function are:\n", "\n", "* asset_slugs: Single asset slug string or list of asset slugs (i.e. bitcoin).\n", "* asset_profile_metric: Single profile metric string to filter profile data. Available metrics include:\n", " * general\n", " * contributors\n", " * advisors\n", " * investors\n", " * ecosystem\n", " * economics\n", " * technology\n", " * governance\n", " * metadata" ] }, { "cell_type": "code", "execution_count": 8, "id": "subsequent-brown", "metadata": {}, "outputs": [ { "data": { "text/plain": [ "'Bitcoin is the first <a href=\"https://messari.io/resource/distributed-systems\">distributed consensus-based</a>, censorship-resistant, permissionless, peer-to-peer payment settlement network with a provably scarce, programmable, native currency. Bitcoin (BTC), the native asset of the Bitcoin blockchain, is the world\\'s first digital currency without a central bank or administrator. The Bitcoin network is an emergent decentralized monetary institution that exists through the interplay between <a href=\"https://messari.io/resource/node\">full nodes</a>, <a href=\"https://messari.io/resource/mining\">miners</a>, and developers. It is set by a social contract that is created and opted into by the users of the network and hardened through <a href=\"https://messari.io/c/resource/game-theory\">game theory</a> and <a href=\"https://messari.io/resource/cryptography\">cryptography</a>. Bitcoin is the first, oldest, and largest cryptocurrency in the world.'" ] }, "execution_count": 8, "metadata": {}, "output_type": "execute_result" } ], "source": [ "asset_profile_data = messari.get_asset_profile(asset_slugs=assets)\n", "asset_profile_data['bitcoin']['profile_general_overview_project_details']" ] }, { "cell_type": "markdown", "id": "resident-study", "metadata": {}, "source": [ "You can filter the results by passing an asset profile metric. For example, if you are interested in looking at the investor list of Uniswap, you can get it by running: " ] }, { "cell_type": "code", "execution_count": 9, "id": "polar-quality", "metadata": {}, "outputs": [ { "data": { "text/plain": [ "{'Uniswap': {'id': '1d51479d-68f6-4886-8644-2a55ea9007bf',\n", " 'slug': 'uniswap',\n", " 'profile_investors_individuals': [],\n", " 'profile_investors_organizations': [{'slug': 'coinbase-ventures',\n", " 'name': 'Coinbase Ventures',\n", " 'logo': 'https://messari.s3.amazonaws.com/images/agora-images/HVEeWmV4_400x400.png',\n", " 'description': 'Coinbase Ventures invests in companies building the open financial system. It provides financing to early-stage companies that have the teams and ideas that can move the cryptoasset industry forward.'},\n", " {'slug': 'defiance-capital',\n", " 'name': 'Defiance Capital',\n", " 'logo': 'https://messari.s3.amazonaws.com/images/agora-images/0%3Fe%3D1609372800%26v%3Dbeta%26t%3D2wLf66PgCxyDRFc1oqSaql8bXLUiujOQY_YzpuTdjzU',\n", " 'description': 'Leading DeFi focused Cryptoasset Fund combining fundamental research and activist investment strategy.'},\n", " {'slug': 'paradigm',\n", " 'name': 'Paradigm',\n", " 'logo': 'https://messari.s3.amazonaws.com/images/agora-images/gv546pvglklhdkr64jpe',\n", " 'description': 'A digital asset investment firm'},\n", " {'slug': 'three-arrows-capital',\n", " 'name': 'Three Arrows Capital',\n", " 'logo': 'https://messari.s3.amazonaws.com/images/agora-images/EZPJ3XBUwAACZ0E.jpg',\n", " 'description': 'Three Arrows Capital Pte. Ltd. is a hedge fund manager established in 2012 and focused on providing superior risk-adjusted returns, founded by Su Zhu and Kyle Davies.'},\n", " {'slug': 'parafi-capital',\n", " 'name': 'ParaFi Capital',\n", " 'logo': 'https://messari.s3.amazonaws.com/images/agora-images/HjNuqyoH_400x400.jpg',\n", " 'description': None},\n", " {'slug': 'delphi-digital',\n", " 'name': 'Delphi Digital',\n", " 'logo': 'https://messari.s3.amazonaws.com/images/agora-images/iNTyjk96_400x400.jpg',\n", " 'description': 'Delphi Digital is an independent research and consulting boutique specializing in the digital asset market.'}]}}" ] }, "execution_count": 9, "metadata": {}, "output_type": "execute_result" } ], "source": [ "asset = 'Uniswap'\n", "profile_metric = 'investors'\n", "governance_data = messari.get_asset_profile(asset_slugs=asset, asset_profile_metric=profile_metric)\n", "governance_data" ] }, { "cell_type": "markdown", "id": "written-dancing", "metadata": {}, "source": [ "#### Get asset metric\n", "\n", "The *get_asset_metric* function allows you to pull quantitative data for an asset. The default value back from this function is a pandas DataFrame. \n", "\n", "The paremeters of the function are:\n", "\n", "* asset_slugs: Single asset slug string or list of asset slugs (i.e. bitcoin).\n", "* asset_metric: Single metric string to filter metric data. Available metrics include:\n", " * market_data\n", " * marketcap\n", " * supply\n", " * blockchain_stats_24_hours\n", " * market_data_liquidity\n", " * all_time_high\n", " * cycle_low\n", " * token_sale_stats\n", " * staking_stats\n", " * mining_stats\n", " * developer_activity\n", " * roi_data\n", " * roi_by_year\n", " * risk_metrics\n", " * misc_data\n", " * lend_rates\n", " * borrow_rates\n", " * loan_data\n", " * reddit\n", " * on_chain_data\n", " * exchange_flows\n", " * alert_messages\n", " \n", "* to_dataframe: Return data as DataFrame or JSON. Default is set to DataFrame." ] }, { "cell_type": "markdown", "id": "bridal-executive", "metadata": {}, "source": [ "The function will return all avaiable metrics for the assets supplied." ] }, { "cell_type": "code", "execution_count": 11, "id": "minute-panama", "metadata": {}, "outputs": [ { "data": { "text/html": [ "<div>\n", "<style scoped>\n", " .dataframe tbody tr th:only-of-type {\n", " vertical-align: middle;\n", " }\n", "\n", " .dataframe tbody tr th {\n", " vertical-align: top;\n", " }\n", "\n", " .dataframe thead th {\n", " text-align: right;\n", " }\n", "</style>\n", "<table border=\"1\" class=\"dataframe\">\n", " <thead>\n", " <tr style=\"text-align: right;\">\n", " <th></th>\n", " <th>id</th>\n", " <th>symbol</th>\n", " <th>name</th>\n", " <th>slug</th>\n", " <th>contract_addresses</th>\n", " <th>_internal_temp_agora_id</th>\n", " <th>market_data_price_usd</th>\n", " <th>market_data_price_btc</th>\n", " <th>market_data_price_eth</th>\n", " <th>market_data_volume_last_24_hours</th>\n", " <th>...</th>\n", " <th>supply_distribution_supply_in_addresses_balance_greater_1_native_units</th>\n", " <th>supply_distribution_supply_in_contracts_usd</th>\n", " <th>supply_distribution_supply_in_contracts_native_units</th>\n", " <th>supply_distribution_supply_shielded</th>\n", " <th>supply_distribution_supply_in_top_100_addresses</th>\n", " <th>supply_distribution_supply_in_top_10_percent_addresses</th>\n", " <th>supply_distribution_supply_in_top_1_percent_addresses</th>\n", " <th>supply_distribution_supply_in_utxo_in_loss</th>\n", " <th>supply_distribution_supply_in_utxo_in_profit</th>\n", " <th>alert_messages</th>\n", " </tr>\n", " </thead>\n", " <tbody>\n", " <tr>\n", " <th>bitcoin</th>\n", " <td>1e31218a-e44e-4285-820c-8282ee222035</td>\n", " <td>BTC</td>\n", " <td>Bitcoin</td>\n", " <td>bitcoin</td>\n", " <td>[]</td>\n", " <td>9793eae6-f374-46b4-8764-c2d224429791</td>\n", " <td>63877.744122</td>\n", " <td>1.000000</td>\n", " <td>14.003232</td>\n", " <td>6.696020e+09</td>\n", " <td>...</td>\n", " <td>1.787605e+07</td>\n", " <td>NaN</td>\n", " <td>NaN</td>\n", " <td>None</td>\n", " <td>2.791041e+06</td>\n", " <td>1.869062e+07</td>\n", " <td>1.729667e+07</td>\n", " <td>272579.562794</td>\n", " <td>1.860070e+07</td>\n", " <td>None</td>\n", " </tr>\n", " <tr>\n", " <th>ethereum</th>\n", " <td>21c795f5-1bfd-40c3-858e-e9d7e820c6d0</td>\n", " <td>ETH</td>\n", " <td>Ethereum</td>\n", " <td>ethereum</td>\n", " <td>[{'platform': 'avalanche', 'contract_address':...</td>\n", " <td>263d8b01-5a96-41f0-85d5-09687bbbf7ca</td>\n", " <td>4572.992315</td>\n", " <td>0.071590</td>\n", " <td>1.000000</td>\n", " <td>5.426996e+09</td>\n", " <td>...</td>\n", " <td>1.153521e+08</td>\n", " <td>1.367004e+11</td>\n", " <td>2.965612e+07</td>\n", " <td>None</td>\n", " <td>4.592305e+07</td>\n", " <td>1.169773e+08</td>\n", " <td>1.142389e+08</td>\n", " <td>NaN</td>\n", " <td>NaN</td>\n", " <td>None</td>\n", " </tr>\n", " <tr>\n", " <th>tether</th>\n", " <td>51f8ea5e-f426-4f40-939a-db7e05495374</td>\n", " <td>USDT</td>\n", " <td>Tether</td>\n", " <td>tether</td>\n", " <td>[{'platform': 'arbitrum-one', 'contract_addres...</td>\n", " <td>1eaf063a-b8dd-4cfb-95dd-a06338565720</td>\n", " <td>1.003057</td>\n", " <td>0.000016</td>\n", " <td>0.000220</td>\n", " <td>3.636292e+10</td>\n", " <td>...</td>\n", " <td>3.783364e+10</td>\n", " <td>7.379604e+09</td>\n", " <td>7.377586e+09</td>\n", " <td>None</td>\n", " <td>1.730566e+10</td>\n", " <td>3.745281e+10</td>\n", " <td>3.609309e+10</td>\n", " <td>NaN</td>\n", " <td>NaN</td>\n", " <td>None</td>\n", " </tr>\n", " </tbody>\n", "</table>\n", "<p>3 rows × 375 columns</p>\n", "</div>" ], "text/plain": [ " id symbol name slug \\\n", "bitcoin 1e31218a-e44e-4285-820c-8282ee222035 BTC Bitcoin bitcoin \n", "ethereum 21c795f5-1bfd-40c3-858e-e9d7e820c6d0 ETH Ethereum ethereum \n", "tether 51f8ea5e-f426-4f40-939a-db7e05495374 USDT Tether tether \n", "\n", " contract_addresses \\\n", "bitcoin [] \n", "ethereum [{'platform': 'avalanche', 'contract_address':... \n", "tether [{'platform': 'arbitrum-one', 'contract_addres... \n", "\n", " _internal_temp_agora_id market_data_price_usd \\\n", "bitcoin 9793eae6-f374-46b4-8764-c2d224429791 63877.744122 \n", "ethereum 263d8b01-5a96-41f0-85d5-09687bbbf7ca 4572.992315 \n", "tether 1eaf063a-b8dd-4cfb-95dd-a06338565720 1.003057 \n", "\n", " market_data_price_btc market_data_price_eth \\\n", "bitcoin 1.000000 14.003232 \n", "ethereum 0.071590 1.000000 \n", "tether 0.000016 0.000220 \n", "\n", " market_data_volume_last_24_hours ... \\\n", "bitcoin 6.696020e+09 ... \n", "ethereum 5.426996e+09 ... \n", "tether 3.636292e+10 ... \n", "\n", " supply_distribution_supply_in_addresses_balance_greater_1_native_units \\\n", "bitcoin 1.787605e+07 \n", "ethereum 1.153521e+08 \n", "tether 3.783364e+10 \n", "\n", " supply_distribution_supply_in_contracts_usd \\\n", "bitcoin NaN \n", "ethereum 1.367004e+11 \n", "tether 7.379604e+09 \n", "\n", " supply_distribution_supply_in_contracts_native_units \\\n", "bitcoin NaN \n", "ethereum 2.965612e+07 \n", "tether 7.377586e+09 \n", "\n", " supply_distribution_supply_shielded \\\n", "bitcoin None \n", "ethereum None \n", "tether None \n", "\n", " supply_distribution_supply_in_top_100_addresses \\\n", "bitcoin 2.791041e+06 \n", "ethereum 4.592305e+07 \n", "tether 1.730566e+10 \n", "\n", " supply_distribution_supply_in_top_10_percent_addresses \\\n", "bitcoin 1.869062e+07 \n", "ethereum 1.169773e+08 \n", "tether 3.745281e+10 \n", "\n", " supply_distribution_supply_in_top_1_percent_addresses \\\n", "bitcoin 1.729667e+07 \n", "ethereum 1.142389e+08 \n", "tether 3.609309e+10 \n", "\n", " supply_distribution_supply_in_utxo_in_loss \\\n", "bitcoin 272579.562794 \n", "ethereum NaN \n", "tether NaN \n", "\n", " supply_distribution_supply_in_utxo_in_profit alert_messages \n", "bitcoin 1.860070e+07 None \n", "ethereum NaN None \n", "tether NaN None \n", "\n", "[3 rows x 375 columns]" ] }, "execution_count": 11, "metadata": {}, "output_type": "execute_result" } ], "source": [ "asset_metric_df = messari.get_asset_metrics(asset_slugs=assets)\n", "asset_metric_df.head()" ] }, { "cell_type": "markdown", "id": "intelligent-boston", "metadata": {}, "source": [ "Also, you can provide an asset metric to filter the metrics data." ] }, { "cell_type": "code", "execution_count": 12, "id": "functioning-franklin", "metadata": {}, "outputs": [ { "data": { "text/html": [ "<div>\n", "<style scoped>\n", " .dataframe tbody tr th:only-of-type {\n", " vertical-align: middle;\n", " }\n", "\n", " .dataframe tbody tr th {\n", " vertical-align: top;\n", " }\n", "\n", " .dataframe thead th {\n", " text-align: right;\n", " }\n", "</style>\n", "<table border=\"1\" class=\"dataframe\">\n", " <thead>\n", " <tr style=\"text-align: right;\">\n", " <th></th>\n", " <th>id</th>\n", " <th>symbol</th>\n", " <th>marketcap_rank</th>\n", " <th>marketcap_marketcap_dominance_percent</th>\n", " <th>marketcap_current_marketcap_usd</th>\n", " <th>marketcap_y_2050_marketcap_usd</th>\n", " <th>marketcap_y_plus10_marketcap_usd</th>\n", " <th>marketcap_liquid_marketcap_usd</th>\n", " <th>marketcap_volume_turnover_last_24_hours_percent</th>\n", " <th>marketcap_realized_marketcap_usd</th>\n", " <th>marketcap_outstanding_marketcap_usd</th>\n", " </tr>\n", " </thead>\n", " <tbody>\n", " <tr>\n", " <th>bitcoin</th>\n", " <td>1e31218a-e44e-4285-820c-8282ee222035</td>\n", " <td>BTC</td>\n", " <td>1</td>\n", " <td>43.218651</td>\n", " <td>1.206339e+12</td>\n", " <td>1.341395e+12</td>\n", " <td>1.320226e+12</td>\n", " <td>1.206865e+12</td>\n", " <td>0.475864</td>\n", " <td>4.571419e+11</td>\n", " <td>1.227371e+12</td>\n", " </tr>\n", " <tr>\n", " <th>ethereum</th>\n", " <td>21c795f5-1bfd-40c3-858e-e9d7e820c6d0</td>\n", " <td>ETH</td>\n", " <td>2</td>\n", " <td>19.189260</td>\n", " <td>5.356194e+11</td>\n", " <td>6.167196e+11</td>\n", " <td>5.591161e+11</td>\n", " <td>5.296972e+11</td>\n", " <td>0.897591</td>\n", " <td>2.662798e+11</td>\n", " <td>5.414160e+11</td>\n", " </tr>\n", " <tr>\n", " <th>tether</th>\n", " <td>51f8ea5e-f426-4f40-939a-db7e05495374</td>\n", " <td>USDT</td>\n", " <td>4</td>\n", " <td>2.655952</td>\n", " <td>7.413415e+10</td>\n", " <td>NaN</td>\n", " <td>NaN</td>\n", " <td>NaN</td>\n", " <td>NaN</td>\n", " <td>3.784762e+10</td>\n", " <td>3.784412e+10</td>\n", " </tr>\n", " </tbody>\n", "</table>\n", "</div>" ], "text/plain": [ " id symbol marketcap_rank \\\n", "bitcoin 1e31218a-e44e-4285-820c-8282ee222035 BTC 1 \n", "ethereum 21c795f5-1bfd-40c3-858e-e9d7e820c6d0 ETH 2 \n", "tether 51f8ea5e-f426-4f40-939a-db7e05495374 USDT 4 \n", "\n", " marketcap_marketcap_dominance_percent \\\n", "bitcoin 43.218651 \n", "ethereum 19.189260 \n", "tether 2.655952 \n", "\n", " marketcap_current_marketcap_usd marketcap_y_2050_marketcap_usd \\\n", "bitcoin 1.206339e+12 1.341395e+12 \n", "ethereum 5.356194e+11 6.167196e+11 \n", "tether 7.413415e+10 NaN \n", "\n", " marketcap_y_plus10_marketcap_usd marketcap_liquid_marketcap_usd \\\n", "bitcoin 1.320226e+12 1.206865e+12 \n", "ethereum 5.591161e+11 5.296972e+11 \n", "tether NaN NaN \n", "\n", " marketcap_volume_turnover_last_24_hours_percent \\\n", "bitcoin 0.475864 \n", "ethereum 0.897591 \n", "tether NaN \n", "\n", " marketcap_realized_marketcap_usd \\\n", "bitcoin 4.571419e+11 \n", "ethereum 2.662798e+11 \n", "tether 3.784762e+10 \n", "\n", " marketcap_outstanding_marketcap_usd \n", "bitcoin 1.227371e+12 \n", "ethereum 5.414160e+11 \n", "tether 3.784412e+10 " ] }, "execution_count": 12, "metadata": {}, "output_type": "execute_result" } ], "source": [ "metric = 'marketcap'\n", "asset_metric_df_marketcap = messari.get_asset_metrics(asset_slugs=assets, asset_metric=metric)\n", "asset_metric_df_marketcap.head()" ] }, { "cell_type": "markdown", "id": "random-scott", "metadata": {}, "source": [ "#### Get asset market data\n", "\n", "The *get_asset_market_data* function pull the latest market data for an asset. The default value back from this function is a pandas DataFrame. \n", "\n", "The paremeters of the function are:\n", "\n", "* asset_slugs: Single asset slug string or list of asset slugs (i.e. bitcoin). \n", "* to_dataframe: Return data as DataFrame or JSON. Default is set to DataFrame." ] }, { "cell_type": "code", "execution_count": 13, "id": "absolute-column", "metadata": {}, "outputs": [ { "data": { "text/html": [ "<div>\n", "<style scoped>\n", " .dataframe tbody tr th:only-of-type {\n", " vertical-align: middle;\n", " }\n", "\n", " .dataframe tbody tr th {\n", " vertical-align: top;\n", " }\n", "\n", " .dataframe thead th {\n", " text-align: right;\n", " }\n", "</style>\n", "<table border=\"1\" class=\"dataframe\">\n", " <thead>\n", " <tr style=\"text-align: right;\">\n", " <th></th>\n", " <th>id</th>\n", " <th>symbol</th>\n", " <th>market_data_price_usd</th>\n", " <th>market_data_price_btc</th>\n", " <th>market_data_price_eth</th>\n", " <th>market_data_volume_last_24_hours</th>\n", " <th>market_data_real_volume_last_24_hours</th>\n", " <th>market_data_volume_last_24_hours_overstatement_multiple</th>\n", " <th>market_data_percent_change_usd_last_1_hour</th>\n", " <th>market_data_percent_change_btc_last_1_hour</th>\n", " <th>...</th>\n", " <th>market_data_ohlcv_last_1_hour_high</th>\n", " <th>market_data_ohlcv_last_1_hour_low</th>\n", " <th>market_data_ohlcv_last_1_hour_close</th>\n", " <th>market_data_ohlcv_last_1_hour_volume</th>\n", " <th>market_data_ohlcv_last_24_hour_open</th>\n", " <th>market_data_ohlcv_last_24_hour_high</th>\n", " <th>market_data_ohlcv_last_24_hour_low</th>\n", " <th>market_data_ohlcv_last_24_hour_close</th>\n", " <th>market_data_ohlcv_last_24_hour_volume</th>\n", " <th>market_data_last_trade_at</th>\n", " </tr>\n", " </thead>\n", " <tbody>\n", " <tr>\n", " <th>bitcoin</th>\n", " <td>1e31218a-e44e-4285-820c-8282ee222035</td>\n", " <td>BTC</td>\n", " <td>63895.998103</td>\n", " <td>1.000000</td>\n", " <td>14.003232</td>\n", " <td>6.696020e+09</td>\n", " <td>5.743741e+09</td>\n", " <td>1.165794</td>\n", " <td>-0.109754</td>\n", " <td>0.000000</td>\n", " <td>...</td>\n", " <td>64143.421918</td>\n", " <td>63387.991540</td>\n", " <td>63897.011656</td>\n", " <td>3.345042e+08</td>\n", " <td>64197.144700</td>\n", " <td>66395.435549</td>\n", " <td>63387.152997</td>\n", " <td>63895.998103</td>\n", " <td>6.741573e+09</td>\n", " <td>2021-11-15T21:06:13.498Z</td>\n", " </tr>\n", " <tr>\n", " <th>ethereum</th>\n", " <td>21c795f5-1bfd-40c3-858e-e9d7e820c6d0</td>\n", " <td>ETH</td>\n", " <td>4574.191597</td>\n", " <td>0.071588</td>\n", " <td>1.000000</td>\n", " <td>5.426996e+09</td>\n", " <td>4.757243e+09</td>\n", " <td>1.140786</td>\n", " <td>0.057229</td>\n", " <td>0.166956</td>\n", " <td>...</td>\n", " <td>4579.045515</td>\n", " <td>4542.824040</td>\n", " <td>4574.409617</td>\n", " <td>2.546684e+08</td>\n", " <td>4550.062092</td>\n", " <td>4771.906982</td>\n", " <td>4539.207138</td>\n", " <td>4574.191597</td>\n", " <td>5.409993e+09</td>\n", " <td>2021-11-15T21:06:13.976Z</td>\n", " </tr>\n", " <tr>\n", " <th>tether</th>\n", " <td>51f8ea5e-f426-4f40-939a-db7e05495374</td>\n", " <td>USDT</td>\n", " <td>1.003025</td>\n", " <td>0.000016</td>\n", " <td>0.000220</td>\n", " <td>3.636292e+10</td>\n", " <td>2.145719e+10</td>\n", " <td>1.694673</td>\n", " <td>-0.040783</td>\n", " <td>0.066726</td>\n", " <td>...</td>\n", " <td>1.007714</td>\n", " <td>0.998665</td>\n", " <td>1.003238</td>\n", " <td>1.195032e+09</td>\n", " <td>1.010482</td>\n", " <td>1.016971</td>\n", " <td>0.993462</td>\n", " <td>1.003025</td>\n", " <td>2.631996e+10</td>\n", " <td>2021-11-15T21:06:13.889Z</td>\n", " </tr>\n", " </tbody>\n", "</table>\n", "<p>3 rows × 25 columns</p>\n", "</div>" ], "text/plain": [ " id symbol market_data_price_usd \\\n", "bitcoin 1e31218a-e44e-4285-820c-8282ee222035 BTC 63895.998103 \n", "ethereum 21c795f5-1bfd-40c3-858e-e9d7e820c6d0 ETH 4574.191597 \n", "tether 51f8ea5e-f426-4f40-939a-db7e05495374 USDT 1.003025 \n", "\n", " market_data_price_btc market_data_price_eth \\\n", "bitcoin 1.000000 14.003232 \n", "ethereum 0.071588 1.000000 \n", "tether 0.000016 0.000220 \n", "\n", " market_data_volume_last_24_hours \\\n", "bitcoin 6.696020e+09 \n", "ethereum 5.426996e+09 \n", "tether 3.636292e+10 \n", "\n", " market_data_real_volume_last_24_hours \\\n", "bitcoin 5.743741e+09 \n", "ethereum 4.757243e+09 \n", "tether 2.145719e+10 \n", "\n", " market_data_volume_last_24_hours_overstatement_multiple \\\n", "bitcoin 1.165794 \n", "ethereum 1.140786 \n", "tether 1.694673 \n", "\n", " market_data_percent_change_usd_last_1_hour \\\n", "bitcoin -0.109754 \n", "ethereum 0.057229 \n", "tether -0.040783 \n", "\n", " market_data_percent_change_btc_last_1_hour ... \\\n", "bitcoin 0.000000 ... \n", "ethereum 0.166956 ... \n", "tether 0.066726 ... \n", "\n", " market_data_ohlcv_last_1_hour_high \\\n", "bitcoin 64143.421918 \n", "ethereum 4579.045515 \n", "tether 1.007714 \n", "\n", " market_data_ohlcv_last_1_hour_low \\\n", "bitcoin 63387.991540 \n", "ethereum 4542.824040 \n", "tether 0.998665 \n", "\n", " market_data_ohlcv_last_1_hour_close \\\n", "bitcoin 63897.011656 \n", "ethereum 4574.409617 \n", "tether 1.003238 \n", "\n", " market_data_ohlcv_last_1_hour_volume \\\n", "bitcoin 3.345042e+08 \n", "ethereum 2.546684e+08 \n", "tether 1.195032e+09 \n", "\n", " market_data_ohlcv_last_24_hour_open \\\n", "bitcoin 64197.144700 \n", "ethereum 4550.062092 \n", "tether 1.010482 \n", "\n", " market_data_ohlcv_last_24_hour_high \\\n", "bitcoin 66395.435549 \n", "ethereum 4771.906982 \n", "tether 1.016971 \n", "\n", " market_data_ohlcv_last_24_hour_low \\\n", "bitcoin 63387.152997 \n", "ethereum 4539.207138 \n", "tether 0.993462 \n", "\n", " market_data_ohlcv_last_24_hour_close \\\n", "bitcoin 63895.998103 \n", "ethereum 4574.191597 \n", "tether 1.003025 \n", "\n", " market_data_ohlcv_last_24_hour_volume market_data_last_trade_at \n", "bitcoin 6.741573e+09 2021-11-15T21:06:13.498Z \n", "ethereum 5.409993e+09 2021-11-15T21:06:13.976Z \n", "tether 2.631996e+10 2021-11-15T21:06:13.889Z \n", "\n", "[3 rows x 25 columns]" ] }, "execution_count": 13, "metadata": {}, "output_type": "execute_result" } ], "source": [ "market_data = messari.get_asset_market_data(asset_slugs=assets)\n", "market_data.head()" ] }, { "cell_type": "markdown", "id": "favorite-montreal", "metadata": {}, "source": [ "## Markets Module" ] }, { "cell_type": "markdown", "id": "sonic-courtesy", "metadata": {}, "source": [ "### Get all markets" ] }, { "cell_type": "markdown", "id": "pressed-disabled", "metadata": {}, "source": [ "The *get_all_markets* function pulls all information of all the exchanges and pairs supported by Messari's API. The default return value of this function is a pandas DataFrame. \n", "\n", "The paremeters of the function are:\n", "\n", "* page: Page number starting at 1. Increment value to paginate through results.\n", "* limit: Limit of assets to return. Default is 20, max value is 500.\n", "* to_dataframe: Return data as DataFrame or list of dictionaries. Default is set to DataFrame." ] }, { "cell_type": "code", "execution_count": 14, "id": "united-recruitment", "metadata": {}, "outputs": [ { "data": { "text/html": [ "<div>\n", "<style scoped>\n", " .dataframe tbody tr th:only-of-type {\n", " vertical-align: middle;\n", " }\n", "\n", " .dataframe tbody tr th {\n", " vertical-align: top;\n", " }\n", "\n", " .dataframe thead th {\n", " text-align: right;\n", " }\n", "</style>\n", "<table border=\"1\" class=\"dataframe\">\n", " <thead>\n", " <tr style=\"text-align: right;\">\n", " <th></th>\n", " <th>id</th>\n", " <th>exchange_id</th>\n", " <th>base_asset_id</th>\n", " <th>quote_asset_id</th>\n", " <th>class</th>\n", " <th>trade_start</th>\n", " <th>trade_end</th>\n", " <th>version</th>\n", " <th>excluded_from_price</th>\n", " <th>exchange_name</th>\n", " <th>base_asset_symbol</th>\n", " <th>quote_asset_symbol</th>\n", " <th>pair</th>\n", " <th>price_usd</th>\n", " <th>vwap_weight</th>\n", " <th>volume_last_24_hours</th>\n", " <th>has_real_volume</th>\n", " <th>deviation_from_vwap_percent</th>\n", " <th>last_trade_at</th>\n", " </tr>\n", " <tr>\n", " <th>exchange_slug</th>\n", " <th></th>\n", " <th></th>\n", " <th></th>\n", " <th></th>\n", " <th></th>\n", " <th></th>\n", " <th></th>\n", " <th></th>\n", " <th></th>\n", " <th></th>\n", " <th></th>\n", " <th></th>\n", " <th></th>\n", " <th></th>\n", " <th></th>\n", " <th></th>\n", " <th></th>\n", " <th></th>\n", " <th></th>\n", " </tr>\n", " </thead>\n", " <tbody>\n", " <tr>\n", " <th>uniswap</th>\n", " <td>0006addf-d3d2-491a-8a66-36236445c527</td>\n", " <td>6efbfc8a-18c9-4c6f-aa78-feea100521cf</td>\n", " <td>3f548a16-866f-4e2c-b3e9-5df6d32d9083</td>\n", " <td>51f8ea5e-f426-4f40-939a-db7e05495374</td>\n", " <td>spot</td>\n", " <td>2020-08-30T16:40:06Z</td>\n", " <td>None</td>\n", " <td>1</td>\n", " <td>False</td>\n", " <td>Uniswap (v2)</td>\n", " <td>UOS</td>\n", " <td>USDT</td>\n", " <td>UOS-USDT</td>\n", " <td>NaN</td>\n", " <td>0.0</td>\n", " <td>NaN</td>\n", " <td>False</td>\n", " <td>NaN</td>\n", " <td>None</td>\n", " </tr>\n", " <tr>\n", " <th>yobit</th>\n", " <td>000bd377-28be-488c-80cc-cd18e90ba3ff</td>\n", " <td>2087a29e-6a27-4d51-ba3b-d9f112f93c38</td>\n", " <td>c10e0960-9175-4c44-9390-86a8de17c2a1</td>\n", " <td>1e31218a-e44e-4285-820c-8282ee222035</td>\n", " <td>spot</td>\n", " <td>2019-04-12T10:49:20Z</td>\n", " <td>2019-04-25T18:24:16Z</td>\n", " <td>1</td>\n", " <td>True</td>\n", " <td>YoBit</td>\n", " <td>LUC</td>\n", " <td>BTC</td>\n", " <td>LUC-BTC</td>\n", " <td>NaN</td>\n", " <td>0.0</td>\n", " <td>NaN</td>\n", " <td>False</td>\n", " <td>NaN</td>\n", " <td>None</td>\n", " </tr>\n", " <tr>\n", " <th>binance</th>\n", " <td>000de500-0515-4d00-8e73-be8b327cc298</td>\n", " <td>d8b0ea44-1963-451e-ac37-aead4ba3b4c7</td>\n", " <td>6d667f96-24f2-420b-9a22-73f5cd594bcf</td>\n", " <td>1e31218a-e44e-4285-820c-8282ee222035</td>\n", " <td>spot</td>\n", " <td>2019-09-24T18:58:30Z</td>\n", " <td>None</td>\n", " <td>1</td>\n", " <td>False</td>\n", " <td>Binance</td>\n", " <td>XTZ</td>\n", " <td>BTC</td>\n", " <td>XTZ-BTC</td>\n", " <td>5.720711</td>\n", " <td>1.0</td>\n", " <td>3.398368e+06</td>\n", " <td>True</td>\n", " <td>0.002639</td>\n", " <td>2021-11-15T21:06:00Z</td>\n", " </tr>\n", " <tr>\n", " <th>binance</th>\n", " <td>000ef1f2-89e2-4096-b02f-feaa11b7467d</td>\n", " <td>d8b0ea44-1963-451e-ac37-aead4ba3b4c7</td>\n", " <td>577bebe9-f569-44a1-aed8-0484325fddf2</td>\n", " <td>7dc551ba-cfed-4437-a027-386044415e3e</td>\n", " <td>spot</td>\n", " <td>2020-09-15T20:51:42Z</td>\n", " <td>2021-09-30T08:59:59Z</td>\n", " <td>1</td>\n", " <td>False</td>\n", " <td>Binance</td>\n", " <td>SWRV</td>\n", " <td>BNB</td>\n", " <td>SWRV-BNB</td>\n", " <td>NaN</td>\n", " <td>0.0</td>\n", " <td>NaN</td>\n", " <td>False</td>\n", " <td>NaN</td>\n", " <td>None</td>\n", " </tr>\n", " <tr>\n", " <th>binance</th>\n", " <td>000f7f7c-5994-4c18-92f2-1ff37f167665</td>\n", " <td>d8b0ea44-1963-451e-ac37-aead4ba3b4c7</td>\n", " <td>362f0140-ecdd-4205-b8a0-36f0fd5d8167</td>\n", " <td>74cc014e-d925-496a-928b-b43c0393c455</td>\n", " <td>spot</td>\n", " <td>2021-03-19T08:00:00Z</td>\n", " <td>None</td>\n", " <td>1</td>\n", " <td>False</td>\n", " <td>Binance</td>\n", " <td>ADA</td>\n", " <td>RUB</td>\n", " <td>ADA-RUB</td>\n", " <td>NaN</td>\n", " <td>0.0</td>\n", " <td>NaN</td>\n", " <td>False</td>\n", " <td>NaN</td>\n", " <td>None</td>\n", " </tr>\n", " </tbody>\n", "</table>\n", "</div>" ], "text/plain": [ " id \\\n", "exchange_slug \n", "uniswap 0006addf-d3d2-491a-8a66-36236445c527 \n", "yobit 000bd377-28be-488c-80cc-cd18e90ba3ff \n", "binance 000de500-0515-4d00-8e73-be8b327cc298 \n", "binance 000ef1f2-89e2-4096-b02f-feaa11b7467d \n", "binance 000f7f7c-5994-4c18-92f2-1ff37f167665 \n", "\n", " exchange_id \\\n", "exchange_slug \n", "uniswap 6efbfc8a-18c9-4c6f-aa78-feea100521cf \n", "yobit 2087a29e-6a27-4d51-ba3b-d9f112f93c38 \n", "binance d8b0ea44-1963-451e-ac37-aead4ba3b4c7 \n", "binance d8b0ea44-1963-451e-ac37-aead4ba3b4c7 \n", "binance d8b0ea44-1963-451e-ac37-aead4ba3b4c7 \n", "\n", " base_asset_id \\\n", "exchange_slug \n", "uniswap 3f548a16-866f-4e2c-b3e9-5df6d32d9083 \n", "yobit c10e0960-9175-4c44-9390-86a8de17c2a1 \n", "binance 6d667f96-24f2-420b-9a22-73f5cd594bcf \n", "binance 577bebe9-f569-44a1-aed8-0484325fddf2 \n", "binance 362f0140-ecdd-4205-b8a0-36f0fd5d8167 \n", "\n", " quote_asset_id class \\\n", "exchange_slug \n", "uniswap 51f8ea5e-f426-4f40-939a-db7e05495374 spot \n", "yobit 1e31218a-e44e-4285-820c-8282ee222035 spot \n", "binance 1e31218a-e44e-4285-820c-8282ee222035 spot \n", "binance 7dc551ba-cfed-4437-a027-386044415e3e spot \n", "binance 74cc014e-d925-496a-928b-b43c0393c455 spot \n", "\n", " trade_start trade_end version \\\n", "exchange_slug \n", "uniswap 2020-08-30T16:40:06Z None 1 \n", "yobit 2019-04-12T10:49:20Z 2019-04-25T18:24:16Z 1 \n", "binance 2019-09-24T18:58:30Z None 1 \n", "binance 2020-09-15T20:51:42Z 2021-09-30T08:59:59Z 1 \n", "binance 2021-03-19T08:00:00Z None 1 \n", "\n", " excluded_from_price exchange_name base_asset_symbol \\\n", "exchange_slug \n", "uniswap False Uniswap (v2) UOS \n", "yobit True YoBit LUC \n", "binance False Binance XTZ \n", "binance False Binance SWRV \n", "binance False Binance ADA \n", "\n", " quote_asset_symbol pair price_usd vwap_weight \\\n", "exchange_slug \n", "uniswap USDT UOS-USDT NaN 0.0 \n", "yobit BTC LUC-BTC NaN 0.0 \n", "binance BTC XTZ-BTC 5.720711 1.0 \n", "binance BNB SWRV-BNB NaN 0.0 \n", "binance RUB ADA-RUB NaN 0.0 \n", "\n", " volume_last_24_hours has_real_volume \\\n", "exchange_slug \n", "uniswap NaN False \n", "yobit NaN False \n", "binance 3.398368e+06 True \n", "binance NaN False \n", "binance NaN False \n", "\n", " deviation_from_vwap_percent last_trade_at \n", "exchange_slug \n", "uniswap NaN None \n", "yobit NaN None \n", "binance 0.002639 2021-11-15T21:06:00Z \n", "binance NaN None \n", "binance NaN None " ] }, "execution_count": 14, "metadata": {}, "output_type": "execute_result" } ], "source": [ "markets_df = messari.get_all_markets()\n", "markets_df.head()" ] }, { "cell_type": "markdown", "id": "fixed-lindsay", "metadata": {}, "source": [ "### Timeseries Module" ] }, { "cell_type": "markdown", "id": "stretch-lodging", "metadata": {}, "source": [ "### Get metric timeseries" ] }, { "cell_type": "markdown", "id": "pleasant-indonesian", "metadata": {}, "source": [ "The *get_metric_timeseries* function allows you to pull time series data for a number of metrics. The default return value of this function is a pandas DataFrame. \n", "\n", "The paremeters of the function are:\n", "\n", "* asset_slugs: Single asset slug string or list of asset slugs (i.e. bitcoin).\n", "* asset_metric: Single metric string to filter timeseries data. Available metrics include:\n", "\n", " * blk.cnt\n", " - The sum count of blocks created each day.\n", " * txn.fee.avg\n", " - The USD value of the mean fee per transaction.\n", " * txn.cnt\n", " - The sum count of transactions that interval. Transactions represent a bundle of intended actions to alter \n", " the ledger initiated by a user (human or machine). Transactions are counted whether they execute or not\n", " and whether they result in the transfer of native units.\n", " * txn.tsfr.val.adj\n", " - The sum USD value of all native units transferred removing noise and certain artifacts.\n", " * sply.circ\n", " - The circulating supply acknowledges that tokens may be held by projects/foundations which have no intent\n", " to sell down their positions, but which have not locked up supply in a formal contract.\n", " Thus, circulating supply does not include known project treasury holdings (which can be significant).\n", " Note that an investor must carefully consider both liquid and circulating supplies when evaluating\n", " an asset, and the two can vary significantly. A risk of depending entirely on circulating supply is that\n", " the number can change dramatically based on discretionary sales from project treasuries.\n", " * mcap.circ\n", " - The circulating marketcap is the price of the asset multiplied by the circulating supply. If no price\n", " is found for an asset because no trades occurred, the last price of the last trade is used. After 30 days\n", " with no trades, a marketcap of 0 is reported until trading resumes.\n", " * reddit.subscribers\n", " - The number of subscribers on the asset's primary subreddit\n", " * iss.rate\n", " - The percentage of new native units (continuous) issued over that interval, extrapolated to one year\n", " (i.e., multiplied by 365), and divided by the current supply at the end of that interval.\n", " Also referred to as the annual inflation rate.\n", " * mcap.realized\n", " - The sum USD value based on the USD closing price on the day that a native unit last moved\n", " (i.e., last transacted) for all native units.\n", " * bitwise.volume\n", " - It is well known that many exchanges conduct wash trading practices in order to inflate trading volume.\n", " They are incentivized to report inflated volumes in order to attract traders. \"Bitwise Volume\" refers to\n", " the total volume over 10 exchanges identified by Bitwise to be free of wash trading activities.\n", " They tend to be regulated exchanges. However, that does not necessarily mean that the volume reported by\n", " other exchanges is 100% wash trades. As such, the Bitwise Volume underestimates the total volume.\n", " * txn.tsfr.val.avg\n", " - The sum USD value of native units transferred divided by the count of transfers\n", " (i.e., the mean \"size\" in USD of a transfer).\n", " * act.addr.cnt\n", " - The sum count of unique addresses that were active in the network (either as a recipient or originator of\n", " a ledger change) that interval. All parties in a ledger change action (recipients and originators)\n", " are counted. Individual addresses are not double-counted.\n", " * fees.ntv\n", " - The sum of all fees paid to miners in native units. Fees do not include new issuance.\n", " * exch.flow.in.usd.incl\n", " - The sum USD value sent to exchanges that interval, including exchange to exchange activity.\n", " * blk.size.byte\n", " - The sum of the size (in bytes) of all blocks created each day.\n", " * txn.tsfr.val.med\n", " - The median transfer value in US dollars.\n", " * exch.flow.in.ntv.incl\n", " - The amount of the asset sent to exchanges that interval, including exchange to exchange activity.\n", " * exch.flow.out.usd\n", " - The sum USD value withdrawn from exchanges that interval, excluding exchange to exchange activity.'\n", " * txn.vol\n", " - The sum USD value of all native units transferred (i.e., the aggregate size in USD of all transfers).\n", " * fees\n", " - The sum USD value of all fees paid to miners that interval. Fees do not include new issuance.\n", " * exch.flow.out.ntv.incl\n", " - The amount of the asset withdrawn from exchanges that interval, including exchange to exchange activity.\n", " * exch.flow.out.usd.incl\n", " - The sum USD value withdrawn from exchanges that interval, including exchange to exchange activity.\n", " * txn.fee.med\n", " - The USD value of the median fee per transaction.\n", " * min.rev.ntv\n", " - The sum of all miner revenue, which constitutes fees plus newly issued native units.\n", " * exch.sply.usd\n", " - The sum USD value of all native units held in hot or cold exchange wallets.\n", " * diff.avg\n", " - The mean difficulty of finding a hash that meets the protocol-designated requirement\n", " (i.e., the difficulty of finding a new block) that interval. The requirement is unique to each applicable\n", " cryptocurrency protocol.\n", " * daily.shp\n", " - The Sharpe ratio (performance of the asset compared to a \"risk-free\" asset) over a window of time).\n", " * txn.tsfr.cnt\n", " - The sum count of transfers that interval. Transfers represent movements of native units from one ledger\n", " entity to another distinct ledger entity. Only transfers that are the result of a transaction and that\n", " have a positive (non-zero) value are counted.\n", " * exch.flow.in.ntv\n", " - The amount of the asset sent to exchanges that interval, excluding exchange to exchange activity.\n", " * new.iss.usd\n", " - The sum USD value of new native units issued that interval. Only those native units that are issued by\n", " a protocol-mandated continuous emission schedule are included (i.e., units manually released from escrow\n", " or otherwise disbursed are not included).\n", " * mcap.dom\n", " - The marketcap dominance is the asset's percentage share of total crypto circulating marketcap.\n", " * daily.vol\n", " - The annualized standard-deviation of daily returns over a window of time.\n", " * reddit.active.users\n", " - The number of active users on the asset's primary subreddit\n", " * exch.sply\n", " - The sum of all native units held in hot or cold exchange wallets\n", " * nvt.adj\n", " - The ratio of the network value (or market capitalization, current supply) divided by the adjusted\n", " transfer value. Also referred to as NVT.\n", " * exch.flow.out.ntv\n", " - The amount of the asset withdrawn from exchanges that interval, excluding exchange to exchange activity.\n", " * min.rev.usd\n", " - The sum USD value of all miner revenue, which constitutes fees plus newly issued native units, represented\n", " as the US dollar amount earned if all native units were sold at the closing price on the same day.\n", " * bitwise.price\n", " - Volume weighted average price over Bitwise 10 exchanges.\n", " * new.iss.ntv\n", " - The sum of new native units issued that interval. Only those native units that are issued by a\n", " protocol-mandated continuous emission schedule are included (i.e., units manually released from escrow or\n", " otherwise disbursed are not included).\n", " * blk.size.bytes.avg\n", " - The mean size (in bytes) of all blocks created.\n", " * hashrate\n", " - The mean rate at which miners are solving hashes that interval.\n", " * exch.flow.in.usd\n", " - The sum USD value sent to exchanges that interval, excluding exchange to exchange activity.\n", " * price\n", " - Volume weighted average price computed using Messari Methodology\n", " * real.vol\n", " - It is well known that many exchanges conduct wash trading practices in order to inflate trading volume.\n", " They are incentivized to report inflated volumes in order to attract traders. \"Real Volume\" refers to the\n", " total volume on the exchanges that we believe with high level of confidence are free of wash trading\n", " activities. However, that does not necessarily mean that the volume reported by other exchanges is 100%\n", " wash trades. As such, the Messari \"Real Volume\" applies a penalty to these exchanges to discount the\n", " volume believed to come from wash trading activity. For more information, see our methodology page.\n", " \n", "* start: Starting date string for timeseries data. A default start date will provided if not specified.\n", "* end: Ending date string for timeseries data. A default end date will provided if not specified.\n", "* interval: Interval of timeseries data. Default value is set to 1d. For any given interval, at most 2016 points will be returned. For example, with interval=5m, the maximum range of the request is 2016 * 5 minutes = 7 days. With interval=1h, the maximum range is 2016 * 1 hour = 84 days. Exceeding the maximum range will result in an error, which can be solved by reducing the date range specified in the request. Interval options include:\n", " * 1m\n", " * 5m\n", " * 15m\n", " * 30m\n", " * 1hr\n", " * 1d\n", " * 1w\n", " \n", "* to_dataframe: Return data as DataFrame or JSON. Default is set to DataFrame." ] }, { "cell_type": "code", "execution_count": 15, "id": "duplicate-numbers", "metadata": {}, "outputs": [ { "data": { "text/html": [ "<div>\n", "<style scoped>\n", " .dataframe tbody tr th:only-of-type {\n", " vertical-align: middle;\n", " }\n", "\n", " .dataframe tbody tr th {\n", " vertical-align: top;\n", " }\n", "\n", " .dataframe thead tr th {\n", " text-align: left;\n", " }\n", "\n", " .dataframe thead tr:last-of-type th {\n", " text-align: right;\n", " }\n", "</style>\n", "<table border=\"1\" class=\"dataframe\">\n", " <thead>\n", " <tr>\n", " <th></th>\n", " <th colspan=\"5\" halign=\"left\">bitcoin</th>\n", " <th colspan=\"5\" halign=\"left\">ethereum</th>\n", " <th colspan=\"5\" halign=\"left\">tether</th>\n", " </tr>\n", " <tr>\n", " <th></th>\n", " <th>open</th>\n", " <th>high</th>\n", " <th>low</th>\n", " <th>close</th>\n", " <th>volume</th>\n", " <th>open</th>\n", " <th>high</th>\n", " <th>low</th>\n", " <th>close</th>\n", " <th>volume</th>\n", " <th>open</th>\n", " <th>high</th>\n", " <th>low</th>\n", " <th>close</th>\n", " <th>volume</th>\n", " </tr>\n", " <tr>\n", " <th>timestamp</th>\n", " <th></th>\n", " <th></th>\n", " <th></th>\n", " <th></th>\n", " <th></th>\n", " <th></th>\n", " <th></th>\n", " <th></th>\n", " <th></th>\n", " <th></th>\n", " <th></th>\n", " <th></th>\n", " <th></th>\n", " <th></th>\n", " <th></th>\n", " </tr>\n", " </thead>\n", " <tbody>\n", " <tr>\n", " <th>2020-06-01</th>\n", " <td>9446.359337</td>\n", " <td>10411.368446</td>\n", " <td>9415.969996</td>\n", " <td>10207.837560</td>\n", " <td>2.546875e+09</td>\n", " <td>231.418675</td>\n", " <td>251.880973</td>\n", " <td>230.555621</td>\n", " <td>248.420898</td>\n", " <td>8.967023e+08</td>\n", " <td>0.999376</td>\n", " <td>1.031920</td>\n", " <td>0.992700</td>\n", " <td>1.001592</td>\n", " <td>4.317591e+09</td>\n", " </tr>\n", " <tr>\n", " <th>2020-06-02</th>\n", " <td>10207.170730</td>\n", " <td>10235.407915</td>\n", " <td>9247.901259</td>\n", " <td>9519.293396</td>\n", " <td>4.445485e+09</td>\n", " <td>247.088209</td>\n", " <td>253.667458</td>\n", " <td>224.949475</td>\n", " <td>237.718348</td>\n", " <td>1.048456e+09</td>\n", " <td>1.002874</td>\n", " <td>1.008979</td>\n", " <td>0.982826</td>\n", " <td>1.000406</td>\n", " <td>5.734804e+09</td>\n", " </tr>\n", " <tr>\n", " <th>2020-06-03</th>\n", " <td>9528.264497</td>\n", " <td>9694.116996</td>\n", " <td>9376.263171</td>\n", " <td>9665.937361</td>\n", " <td>1.789072e+09</td>\n", " <td>237.857362</td>\n", " <td>245.205872</td>\n", " <td>233.249205</td>\n", " <td>244.559197</td>\n", " <td>4.947757e+08</td>\n", " <td>0.998064</td>\n", " <td>1.011970</td>\n", " <td>0.992956</td>\n", " <td>1.000404</td>\n", " <td>2.841139e+09</td>\n", " </tr>\n", " <tr>\n", " <th>2020-06-04</th>\n", " <td>9656.485925</td>\n", " <td>9883.971306</td>\n", " <td>9449.824258</td>\n", " <td>9788.178936</td>\n", " <td>2.183610e+09</td>\n", " <td>244.445697</td>\n", " <td>246.610619</td>\n", " <td>235.973443</td>\n", " <td>243.221280</td>\n", " <td>6.650226e+08</td>\n", " <td>1.001681</td>\n", " <td>1.011502</td>\n", " <td>0.983112</td>\n", " <td>1.000884</td>\n", " <td>3.353472e+09</td>\n", " </tr>\n", " <tr>\n", " <th>2020-06-05</th>\n", " <td>9790.242751</td>\n", " <td>9856.404984</td>\n", " <td>9586.252232</td>\n", " <td>9617.090421</td>\n", " <td>1.965504e+09</td>\n", " <td>243.344371</td>\n", " <td>247.945284</td>\n", " <td>239.014255</td>\n", " <td>239.974526</td>\n", " <td>5.535803e+08</td>\n", " <td>1.001512</td>\n", " <td>1.005469</td>\n", " <td>0.988998</td>\n", " <td>1.000122</td>\n", " <td>3.036441e+09</td>\n", " </tr>\n", " <tr>\n", " <th>...</th>\n", " <td>...</td>\n", " <td>...</td>\n", " <td>...</td>\n", " <td>...</td>\n", " <td>...</td>\n", " <td>...</td>\n", " <td>...</td>\n", " <td>...</td>\n", " <td>...</td>\n", " <td>...</td>\n", " <td>...</td>\n", " <td>...</td>\n", " <td>...</td>\n", " <td>...</td>\n", " <td>...</td>\n", " </tr>\n", " <tr>\n", " <th>2020-12-28</th>\n", " <td>26248.564564</td>\n", " <td>27469.396137</td>\n", " <td>26069.019129</td>\n", " <td>27032.289152</td>\n", " <td>5.653047e+09</td>\n", " <td>684.007261</td>\n", " <td>747.146848</td>\n", " <td>680.802699</td>\n", " <td>729.225118</td>\n", " <td>4.131698e+09</td>\n", " <td>0.998800</td>\n", " <td>1.009537</td>\n", " <td>0.988917</td>\n", " <td>0.998043</td>\n", " <td>1.323048e+10</td>\n", " </tr>\n", " <tr>\n", " <th>2020-12-29</th>\n", " <td>27036.832984</td>\n", " <td>27387.310760</td>\n", " <td>25832.269524</td>\n", " <td>27360.005185</td>\n", " <td>5.558736e+09</td>\n", " <td>729.372510</td>\n", " <td>739.503440</td>\n", " <td>688.494556</td>\n", " <td>731.413028</td>\n", " <td>2.731104e+09</td>\n", " <td>0.998220</td>\n", " <td>1.009277</td>\n", " <td>0.982313</td>\n", " <td>0.998654</td>\n", " <td>1.281210e+10</td>\n", " </tr>\n", " <tr>\n", " <th>2020-12-30</th>\n", " <td>27363.633892</td>\n", " <td>28999.010850</td>\n", " <td>27337.600922</td>\n", " <td>28886.315853</td>\n", " <td>7.442424e+09</td>\n", " <td>731.617969</td>\n", " <td>758.444774</td>\n", " <td>716.975766</td>\n", " <td>752.458953</td>\n", " <td>2.537980e+09</td>\n", " <td>1.000533</td>\n", " <td>1.008489</td>\n", " <td>0.986462</td>\n", " <td>1.000573</td>\n", " <td>1.245562e+10</td>\n", " </tr>\n", " <tr>\n", " <th>2020-12-31</th>\n", " <td>28893.616815</td>\n", " <td>29304.876699</td>\n", " <td>27852.684695</td>\n", " <td>28961.164191</td>\n", " <td>7.007439e+09</td>\n", " <td>752.636151</td>\n", " <td>756.208116</td>\n", " <td>722.118872</td>\n", " <td>737.255391</td>\n", " <td>2.125796e+09</td>\n", " <td>1.000973</td>\n", " <td>1.013499</td>\n", " <td>0.980359</td>\n", " <td>1.002135</td>\n", " <td>1.049599e+10</td>\n", " </tr>\n", " <tr>\n", " <th>2021-01-01</th>\n", " <td>28963.116282</td>\n", " <td>29674.366390</td>\n", " <td>28692.320100</td>\n", " <td>29402.176951</td>\n", " <td>5.206879e+09</td>\n", " <td>737.183725</td>\n", " <td>750.432877</td>\n", " <td>716.538809</td>\n", " <td>730.756766</td>\n", " <td>1.702361e+09</td>\n", " <td>1.001124</td>\n", " <td>1.013243</td>\n", " <td>0.991402</td>\n", " <td>1.002321</td>\n", " <td>1.136276e+10</td>\n", " </tr>\n", " </tbody>\n", "</table>\n", "<p>215 rows × 15 columns</p>\n", "</div>" ], "text/plain": [ " bitcoin \\\n", " open high low close \n", "timestamp \n", "2020-06-01 9446.359337 10411.368446 9415.969996 10207.837560 \n", "2020-06-02 10207.170730 10235.407915 9247.901259 9519.293396 \n", "2020-06-03 9528.264497 9694.116996 9376.263171 9665.937361 \n", "2020-06-04 9656.485925 9883.971306 9449.824258 9788.178936 \n", "2020-06-05 9790.242751 9856.404984 9586.252232 9617.090421 \n", "... ... ... ... ... \n", "2020-12-28 26248.564564 27469.396137 26069.019129 27032.289152 \n", "2020-12-29 27036.832984 27387.310760 25832.269524 27360.005185 \n", "2020-12-30 27363.633892 28999.010850 27337.600922 28886.315853 \n", "2020-12-31 28893.616815 29304.876699 27852.684695 28961.164191 \n", "2021-01-01 28963.116282 29674.366390 28692.320100 29402.176951 \n", "\n", " ethereum \\\n", " volume open high low close \n", "timestamp \n", "2020-06-01 2.546875e+09 231.418675 251.880973 230.555621 248.420898 \n", "2020-06-02 4.445485e+09 247.088209 253.667458 224.949475 237.718348 \n", "2020-06-03 1.789072e+09 237.857362 245.205872 233.249205 244.559197 \n", "2020-06-04 2.183610e+09 244.445697 246.610619 235.973443 243.221280 \n", "2020-06-05 1.965504e+09 243.344371 247.945284 239.014255 239.974526 \n", "... ... ... ... ... ... \n", "2020-12-28 5.653047e+09 684.007261 747.146848 680.802699 729.225118 \n", "2020-12-29 5.558736e+09 729.372510 739.503440 688.494556 731.413028 \n", "2020-12-30 7.442424e+09 731.617969 758.444774 716.975766 752.458953 \n", "2020-12-31 7.007439e+09 752.636151 756.208116 722.118872 737.255391 \n", "2021-01-01 5.206879e+09 737.183725 750.432877 716.538809 730.756766 \n", "\n", " tether \n", " volume open high low close volume \n", "timestamp \n", "2020-06-01 8.967023e+08 0.999376 1.031920 0.992700 1.001592 4.317591e+09 \n", "2020-06-02 1.048456e+09 1.002874 1.008979 0.982826 1.000406 5.734804e+09 \n", "2020-06-03 4.947757e+08 0.998064 1.011970 0.992956 1.000404 2.841139e+09 \n", "2020-06-04 6.650226e+08 1.001681 1.011502 0.983112 1.000884 3.353472e+09 \n", "2020-06-05 5.535803e+08 1.001512 1.005469 0.988998 1.000122 3.036441e+09 \n", "... ... ... ... ... ... ... \n", "2020-12-28 4.131698e+09 0.998800 1.009537 0.988917 0.998043 1.323048e+10 \n", "2020-12-29 2.731104e+09 0.998220 1.009277 0.982313 0.998654 1.281210e+10 \n", "2020-12-30 2.537980e+09 1.000533 1.008489 0.986462 1.000573 1.245562e+10 \n", "2020-12-31 2.125796e+09 1.000973 1.013499 0.980359 1.002135 1.049599e+10 \n", "2021-01-01 1.702361e+09 1.001124 1.013243 0.991402 1.002321 1.136276e+10 \n", "\n", "[215 rows x 15 columns]" ] }, "execution_count": 15, "metadata": {}, "output_type": "execute_result" } ], "source": [ "metric = 'price'\n", "start = '2020-06-01'\n", "end = '2021-01-01'\n", "timeseries_df = messari.get_metric_timeseries(asset_slugs=assets, asset_metric=metric, start=start, end=end)\n", "timeseries_df" ] } ], "metadata": { "kernelspec": { "display_name": "Python 3 (ipykernel)", "language": "python", "name": "python3" }, "language_info": { "codemirror_mode": { "name": "ipython", "version": 3 }, "file_extension": ".py", "mimetype": "text/x-python", "name": "python", "nbconvert_exporter": "python", "pygments_lexer": "ipython3", "version": "3.9.6" } }, "nbformat": 4, "nbformat_minor": 5 }