Note
Go to the end to download the full example code.
Decoding sensor space data with generalization across time and conditions#
This example runs the analysis described in [1]. It illustrates how one can fit a linear classifier to identify a discriminatory topography at a given time instant and subsequently assess whether this linear model can accurately predict all of the time samples of a second set of conditions.
# Authors: Jean-Rémi King <jeanremi.king@gmail.com>
# Alexandre Gramfort <alexandre.gramfort@inria.fr>
# Denis Engemann <denis.engemann@gmail.com>
#
# License: BSD-3-Clause
# Copyright the MNE-Python contributors.
import matplotlib.pyplot as plt
from sklearn.linear_model import LogisticRegression
from sklearn.pipeline import make_pipeline
from sklearn.preprocessing import StandardScaler
import mne
from mne.datasets import sample
from mne.decoding import GeneralizingEstimator
print(__doc__)
# Preprocess data
data_path = sample.data_path()
# Load and filter data, set up epochs
meg_path = data_path / "MEG" / "sample"
raw_fname = meg_path / "sample_audvis_filt-0-40_raw.fif"
events_fname = meg_path / "sample_audvis_filt-0-40_raw-eve.fif"
raw = mne.io.read_raw_fif(raw_fname, preload=True)
picks = mne.pick_types(raw.info, meg=True, exclude="bads") # Pick MEG channels
raw.filter(1.0, 30.0, fir_design="firwin") # Band pass filtering signals
events = mne.read_events(events_fname)
event_id = {
"Auditory/Left": 1,
"Auditory/Right": 2,
"Visual/Left": 3,
"Visual/Right": 4,
}
tmin = -0.050
tmax = 0.400
# decimate to make the example faster to run, but then use verbose='error' in
# the Epochs constructor to suppress warning about decimation causing aliasing
decim = 2
epochs = mne.Epochs(
raw,
events,
event_id=event_id,
tmin=tmin,
tmax=tmax,
proj=True,
picks=picks,
baseline=None,
preload=True,
reject=dict(mag=5e-12),
decim=decim,
verbose="error",
)
Opening raw data file /home/circleci/mne_data/MNE-sample-data/MEG/sample/sample_audvis_filt-0-40_raw.fif...
Read a total of 4 projection items:
PCA-v1 (1 x 102) idle
PCA-v2 (1 x 102) idle
PCA-v3 (1 x 102) idle
Average EEG reference (1 x 60) idle
Range : 6450 ... 48149 = 42.956 ... 320.665 secs
Ready.
Reading 0 ... 41699 = 0.000 ... 277.709 secs...
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 1 - 30 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 1.00
- Lower transition bandwidth: 1.00 Hz (-6 dB cutoff frequency: 0.50 Hz)
- Upper passband edge: 30.00 Hz
- Upper transition bandwidth: 7.50 Hz (-6 dB cutoff frequency: 33.75 Hz)
- Filter length: 497 samples (3.310 s)
[Parallel(n_jobs=1)]: Done 17 tasks | elapsed: 0.0s
[Parallel(n_jobs=1)]: Done 71 tasks | elapsed: 0.1s
[Parallel(n_jobs=1)]: Done 161 tasks | elapsed: 0.3s
[Parallel(n_jobs=1)]: Done 287 tasks | elapsed: 0.5s
We will train the classifier on all left visual vs auditory trials and test on all right visual vs auditory trials.
clf = make_pipeline(
StandardScaler(),
LogisticRegression(solver="liblinear"), # liblinear is faster than lbfgs
)
time_gen = GeneralizingEstimator(clf, scoring="roc_auc", n_jobs=None, verbose=True)
# Fit classifiers on the epochs where the stimulus was presented to the left.
# Note that the experimental condition y indicates auditory or visual
time_gen.fit(X=epochs["Left"].get_data(copy=False), y=epochs["Left"].events[:, 2] > 2)
0%| | Fitting GeneralizingEstimator : 0/35 [00:00<?, ?it/s]
6%|▌ | Fitting GeneralizingEstimator : 2/35 [00:00<00:00, 55.69it/s]
11%|█▏ | Fitting GeneralizingEstimator : 4/35 [00:00<00:00, 57.52it/s]
17%|█▋ | Fitting GeneralizingEstimator : 6/35 [00:00<00:00, 58.16it/s]
26%|██▌ | Fitting GeneralizingEstimator : 9/35 [00:00<00:00, 66.29it/s]
34%|███▍ | Fitting GeneralizingEstimator : 12/35 [00:00<00:00, 71.27it/s]
43%|████▎ | Fitting GeneralizingEstimator : 15/35 [00:00<00:00, 74.58it/s]
51%|█████▏ | Fitting GeneralizingEstimator : 18/35 [00:00<00:00, 76.97it/s]
60%|██████ | Fitting GeneralizingEstimator : 21/35 [00:00<00:00, 78.56it/s]
66%|██████▌ | Fitting GeneralizingEstimator : 23/35 [00:00<00:00, 75.44it/s]
74%|███████▍ | Fitting GeneralizingEstimator : 26/35 [00:00<00:00, 77.12it/s]
80%|████████ | Fitting GeneralizingEstimator : 28/35 [00:00<00:00, 75.09it/s]
89%|████████▊ | Fitting GeneralizingEstimator : 31/35 [00:00<00:00, 76.60it/s]
94%|█████████▍| Fitting GeneralizingEstimator : 33/35 [00:00<00:00, 74.85it/s]
100%|██████████| Fitting GeneralizingEstimator : 35/35 [00:00<00:00, 76.48it/s]
Score on the epochs where the stimulus was presented to the right.
scores = time_gen.score(
X=epochs["Right"].get_data(copy=False), y=epochs["Right"].events[:, 2] > 2
)
0%| | Scoring GeneralizingEstimator : 0/1225 [00:00<?, ?it/s]
1%| | Scoring GeneralizingEstimator : 13/1225 [00:00<00:03, 353.97it/s]
2%|▏ | Scoring GeneralizingEstimator : 25/1225 [00:00<00:03, 354.82it/s]
3%|▎ | Scoring GeneralizingEstimator : 37/1225 [00:00<00:03, 355.35it/s]
4%|▍ | Scoring GeneralizingEstimator : 49/1225 [00:00<00:03, 355.40it/s]
5%|▍ | Scoring GeneralizingEstimator : 61/1225 [00:00<00:03, 355.36it/s]
6%|▌ | Scoring GeneralizingEstimator : 73/1225 [00:00<00:03, 355.47it/s]
7%|▋ | Scoring GeneralizingEstimator : 85/1225 [00:00<00:03, 355.55it/s]
8%|▊ | Scoring GeneralizingEstimator : 98/1225 [00:00<00:03, 359.95it/s]
9%|▉ | Scoring GeneralizingEstimator : 110/1225 [00:00<00:03, 359.41it/s]
10%|█ | Scoring GeneralizingEstimator : 123/1225 [00:00<00:03, 361.10it/s]
11%|█ | Scoring GeneralizingEstimator : 135/1225 [00:00<00:03, 360.28it/s]
12%|█▏ | Scoring GeneralizingEstimator : 147/1225 [00:00<00:02, 359.78it/s]
13%|█▎ | Scoring GeneralizingEstimator : 159/1225 [00:00<00:02, 359.33it/s]
14%|█▍ | Scoring GeneralizingEstimator : 172/1225 [00:00<00:02, 359.86it/s]
15%|█▌ | Scoring GeneralizingEstimator : 184/1225 [00:00<00:02, 359.51it/s]
16%|█▌ | Scoring GeneralizingEstimator : 196/1225 [00:00<00:02, 359.18it/s]
17%|█▋ | Scoring GeneralizingEstimator : 209/1225 [00:00<00:02, 361.48it/s]
18%|█▊ | Scoring GeneralizingEstimator : 221/1225 [00:00<00:02, 360.92it/s]
19%|█▉ | Scoring GeneralizingEstimator : 233/1225 [00:00<00:02, 360.45it/s]
20%|██ | Scoring GeneralizingEstimator : 245/1225 [00:00<00:02, 360.07it/s]
21%|██ | Scoring GeneralizingEstimator : 258/1225 [00:00<00:02, 359.69it/s]
22%|██▏ | Scoring GeneralizingEstimator : 270/1225 [00:00<00:02, 359.32it/s]
23%|██▎ | Scoring GeneralizingEstimator : 282/1225 [00:00<00:02, 359.04it/s]
24%|██▍ | Scoring GeneralizingEstimator : 295/1225 [00:00<00:02, 360.91it/s]
25%|██▌ | Scoring GeneralizingEstimator : 307/1225 [00:00<00:02, 360.62it/s]
26%|██▌ | Scoring GeneralizingEstimator : 319/1225 [00:00<00:02, 360.25it/s]
27%|██▋ | Scoring GeneralizingEstimator : 331/1225 [00:00<00:02, 359.83it/s]
28%|██▊ | Scoring GeneralizingEstimator : 343/1225 [00:00<00:02, 359.57it/s]
29%|██▉ | Scoring GeneralizingEstimator : 356/1225 [00:00<00:02, 361.25it/s]
30%|███ | Scoring GeneralizingEstimator : 368/1225 [00:01<00:02, 360.13it/s]
31%|███ | Scoring GeneralizingEstimator : 380/1225 [00:01<00:02, 359.84it/s]
32%|███▏ | Scoring GeneralizingEstimator : 392/1225 [00:01<00:02, 359.60it/s]
33%|███▎ | Scoring GeneralizingEstimator : 404/1225 [00:01<00:02, 359.32it/s]
34%|███▍ | Scoring GeneralizingEstimator : 417/1225 [00:01<00:02, 359.62it/s]
35%|███▌ | Scoring GeneralizingEstimator : 429/1225 [00:01<00:02, 359.39it/s]
36%|███▌ | Scoring GeneralizingEstimator : 442/1225 [00:01<00:02, 360.87it/s]
37%|███▋ | Scoring GeneralizingEstimator : 454/1225 [00:01<00:02, 360.50it/s]
38%|███▊ | Scoring GeneralizingEstimator : 467/1225 [00:01<00:02, 361.78it/s]
39%|███▉ | Scoring GeneralizingEstimator : 479/1225 [00:01<00:02, 361.45it/s]
40%|████ | Scoring GeneralizingEstimator : 491/1225 [00:01<00:02, 361.17it/s]
41%|████ | Scoring GeneralizingEstimator : 504/1225 [00:01<00:01, 360.99it/s]
42%|████▏ | Scoring GeneralizingEstimator : 516/1225 [00:01<00:01, 360.74it/s]
43%|████▎ | Scoring GeneralizingEstimator : 528/1225 [00:01<00:01, 360.48it/s]
44%|████▍ | Scoring GeneralizingEstimator : 540/1225 [00:01<00:01, 360.20it/s]
45%|████▌ | Scoring GeneralizingEstimator : 552/1225 [00:01<00:01, 359.93it/s]
46%|████▌ | Scoring GeneralizingEstimator : 564/1225 [00:01<00:01, 359.66it/s]
47%|████▋ | Scoring GeneralizingEstimator : 577/1225 [00:01<00:01, 360.90it/s]
48%|████▊ | Scoring GeneralizingEstimator : 589/1225 [00:01<00:01, 360.66it/s]
49%|████▉ | Scoring GeneralizingEstimator : 601/1225 [00:01<00:01, 359.35it/s]
50%|█████ | Scoring GeneralizingEstimator : 613/1225 [00:01<00:01, 359.11it/s]
51%|█████ | Scoring GeneralizingEstimator : 626/1225 [00:01<00:01, 359.54it/s]
52%|█████▏ | Scoring GeneralizingEstimator : 638/1225 [00:01<00:01, 359.35it/s]
53%|█████▎ | Scoring GeneralizingEstimator : 650/1225 [00:01<00:01, 359.15it/s]
54%|█████▍ | Scoring GeneralizingEstimator : 662/1225 [00:01<00:01, 358.97it/s]
55%|█████▌ | Scoring GeneralizingEstimator : 675/1225 [00:01<00:01, 360.40it/s]
56%|█████▌ | Scoring GeneralizingEstimator : 688/1225 [00:01<00:01, 361.04it/s]
57%|█████▋ | Scoring GeneralizingEstimator : 700/1225 [00:01<00:01, 360.78it/s]
58%|█████▊ | Scoring GeneralizingEstimator : 712/1225 [00:01<00:01, 360.53it/s]
59%|█████▉ | Scoring GeneralizingEstimator : 724/1225 [00:02<00:01, 360.22it/s]
60%|██████ | Scoring GeneralizingEstimator : 737/1225 [00:02<00:01, 360.31it/s]
61%|██████ | Scoring GeneralizingEstimator : 749/1225 [00:02<00:01, 360.06it/s]
62%|██████▏ | Scoring GeneralizingEstimator : 761/1225 [00:02<00:01, 359.84it/s]
63%|██████▎ | Scoring GeneralizingEstimator : 773/1225 [00:02<00:01, 359.64it/s]
64%|██████▍ | Scoring GeneralizingEstimator : 785/1225 [00:02<00:01, 359.43it/s]
65%|██████▌ | Scoring GeneralizingEstimator : 798/1225 [00:02<00:01, 360.80it/s]
66%|██████▌ | Scoring GeneralizingEstimator : 810/1225 [00:02<00:01, 360.50it/s]
67%|██████▋ | Scoring GeneralizingEstimator : 823/1225 [00:02<00:01, 360.91it/s]
68%|██████▊ | Scoring GeneralizingEstimator : 835/1225 [00:02<00:01, 360.68it/s]
69%|██████▉ | Scoring GeneralizingEstimator : 847/1225 [00:02<00:01, 360.45it/s]
70%|███████ | Scoring GeneralizingEstimator : 859/1225 [00:02<00:01, 360.23it/s]
71%|███████ | Scoring GeneralizingEstimator : 871/1225 [00:02<00:00, 359.97it/s]
72%|███████▏ | Scoring GeneralizingEstimator : 883/1225 [00:02<00:00, 359.77it/s]
73%|███████▎ | Scoring GeneralizingEstimator : 896/1225 [00:02<00:00, 360.15it/s]
74%|███████▍ | Scoring GeneralizingEstimator : 908/1225 [00:02<00:00, 359.85it/s]
75%|███████▌ | Scoring GeneralizingEstimator : 921/1225 [00:02<00:00, 361.15it/s]
76%|███████▌ | Scoring GeneralizingEstimator : 933/1225 [00:02<00:00, 360.91it/s]
77%|███████▋ | Scoring GeneralizingEstimator : 945/1225 [00:02<00:00, 360.54it/s]
78%|███████▊ | Scoring GeneralizingEstimator : 957/1225 [00:02<00:00, 360.29it/s]
79%|███████▉ | Scoring GeneralizingEstimator : 970/1225 [00:02<00:00, 360.20it/s]
80%|████████ | Scoring GeneralizingEstimator : 982/1225 [00:02<00:00, 360.00it/s]
81%|████████ | Scoring GeneralizingEstimator : 994/1225 [00:02<00:00, 359.76it/s]
82%|████████▏ | Scoring GeneralizingEstimator : 1006/1225 [00:02<00:00, 359.59it/s]
83%|████████▎ | Scoring GeneralizingEstimator : 1019/1225 [00:02<00:00, 360.77it/s]
84%|████████▍ | Scoring GeneralizingEstimator : 1031/1225 [00:02<00:00, 360.45it/s]
85%|████████▌ | Scoring GeneralizingEstimator : 1043/1225 [00:02<00:00, 360.20it/s]
86%|████████▌ | Scoring GeneralizingEstimator : 1056/1225 [00:02<00:00, 361.39it/s]
87%|████████▋ | Scoring GeneralizingEstimator : 1068/1225 [00:02<00:00, 360.29it/s]
88%|████████▊ | Scoring GeneralizingEstimator : 1080/1225 [00:02<00:00, 360.07it/s]
89%|████████▉ | Scoring GeneralizingEstimator : 1092/1225 [00:03<00:00, 359.82it/s]
90%|█████████ | Scoring GeneralizingEstimator : 1104/1225 [00:03<00:00, 359.64it/s]
91%|█████████ | Scoring GeneralizingEstimator : 1116/1225 [00:03<00:00, 359.45it/s]
92%|█████████▏| Scoring GeneralizingEstimator : 1129/1225 [00:03<00:00, 360.07it/s]
93%|█████████▎| Scoring GeneralizingEstimator : 1141/1225 [00:03<00:00, 359.85it/s]
94%|█████████▍| Scoring GeneralizingEstimator : 1153/1225 [00:03<00:00, 359.64it/s]
95%|█████████▌| Scoring GeneralizingEstimator : 1165/1225 [00:03<00:00, 359.49it/s]
96%|█████████▌| Scoring GeneralizingEstimator : 1177/1225 [00:03<00:00, 359.32it/s]
97%|█████████▋| Scoring GeneralizingEstimator : 1190/1225 [00:03<00:00, 359.30it/s]
98%|█████████▊| Scoring GeneralizingEstimator : 1202/1225 [00:03<00:00, 359.12it/s]
99%|█████████▉| Scoring GeneralizingEstimator : 1214/1225 [00:03<00:00, 358.90it/s]
100%|██████████| Scoring GeneralizingEstimator : 1225/1225 [00:03<00:00, 360.38it/s]
100%|██████████| Scoring GeneralizingEstimator : 1225/1225 [00:03<00:00, 360.06it/s]
Plot
fig, ax = plt.subplots(layout="constrained")
im = ax.matshow(
scores,
vmin=0,
vmax=1.0,
cmap="RdBu_r",
origin="lower",
extent=epochs.times[[0, -1, 0, -1]],
)
ax.axhline(0.0, color="k")
ax.axvline(0.0, color="k")
ax.xaxis.set_ticks_position("bottom")
ax.set_xlabel(
'Condition: "Right"\nTesting Time (s)',
)
ax.set_ylabel('Condition: "Left"\nTraining Time (s)')
ax.set_title("Generalization across time and condition", fontweight="bold")
fig.colorbar(im, ax=ax, label="Performance (ROC AUC)")
plt.show()
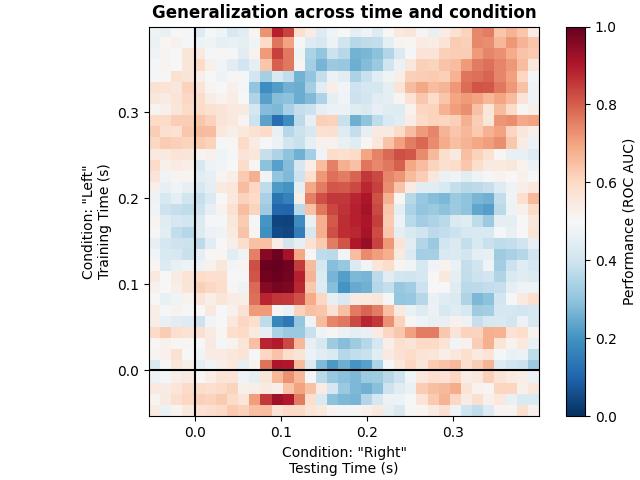
References#
Total running time of the script: (0 minutes 5.765 seconds)