---
uid: Uno.Workshop.Counter.XAML.MVUX
---
# Counter App using XAML and MVUX
[Download the complete XAML + MVUX sample](https://github.com/unoplatform/Uno.Samples/tree/master/reference/Counter/XAML-MVUX)
[!INCLUDE [Intro](includes/include-intro.md)]
In this tutorial you will learn how to:
- Create a new Project with Uno Platform using Visual Studio Template Wizard or the **dotnet new** command
- Add elements to the XAML file to define the layout of the application
- Add code to the C# file to implement the application logic using the [Model-View-Update-eXtended (MVUX)](xref:Uno.Extensions.Mvux.Overview) pattern
- Use data binding to connect the UI to the application logic
To complete this tutorial you don't need any prior knowledge of the Uno Platform, XAML, or C#.
[!INCLUDE [VS](includes/include-create.md)]
## [Visual Studio](#tab/vs)
> [!NOTE]
> If you don't have the **Uno Platform Extension for Visual Studio** installed, follow [these instructions](xref:Uno.GetStarted.vs2022).
- Launch **Visual Studio** and click on **Create new project** on the Start Window. Alternatively, if you're already in Visual Studio, click **New, Project** from the **File** menu.
- Type `Uno Platform` in the search box
- Click **Uno Platform App**, then **Next**
- Name the project `Counter` and click **Create**
At this point you'll enter the **Uno Platform Template Wizard**, giving you options to customize the generated application. For this tutorial, we're only going to configure the markup language and the presentation framework.
- Select **Blank** in **Presets** selection
- Select the **Presentation** tab and choose **MVUX**
- Select the **Markup** tab and choose **XAML**
Before completing the wizard, take a look through each of the sections and see what other options are available. You can always come back and create a new project with different options later. For more information on all the template options, see [Using the Uno Platform Template](xref:Uno.GettingStarted.UsingWizard).
- Click **Create** to complete the wizard
The template will create a solution with a single cross-platform project, named `Counter`, ready to run.
## [Command Line](#tab/cli)
> [!NOTE]
> If you don't have the Uno Platform dotnet new templates installed, follow [dotnet new templates for Uno Platform](xref:Uno.GetStarted.dotnet-new).
From the command line, run the following command:
```dotnetcli
dotnet new unoapp -preset blank -presentation mvux -markup xaml -o Counter
```
This will create a new folder called **Counter** containing the new application.
If you want to discover all the options available in the **unoapp** template, run the following command:
```dotnetcli
dotnet new unoapp -h
```
Also, for more information on all the template options, see [Using the Uno Platform Template](xref:Uno.GettingStarted.UsingWizard).
---
[!INCLUDE [Counter Solution](includes/include-solution.md)]
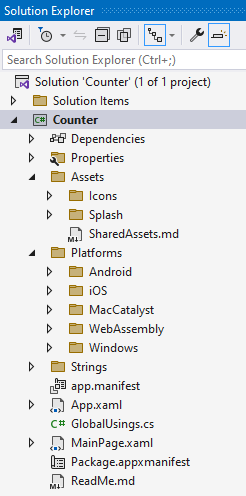
[!INCLUDE [Main Window](includes/include-mainwindow.md)]
[!INCLUDE [Main Page - XAML](includes/include-mainpage-xaml.md)]
[!INCLUDE [Main Page - Layout](includes/include-mainpage-layout.md)]
[!INCLUDE [Main Page - Image](includes/include-image-xaml.md)]
[!INCLUDE [Main Page - Change Layout](includes/include-mainpage-change-layout.md)]
[!INCLUDE [Main Page - Other Elements](includes/include-elements-xaml.md)]
[!INCLUDE [Main Model](includes/include-mvux.md)]
## Data Binding
Now that we have the **`MainModel`** class, we can update the **`MainPage`** to use data binding to connect the UI to the application logic.
- Add a **`DataContext`** element to the **`Page`** element in the **MainPage.xaml** file.
```xml
```
- Update the **`TextBlock`** by removing the **`Text`** attribute, replacing it with two **`Run`** elements, and binding the **`Text`** property of the second **`Run`** element to the **`Countable.Count`** property of the **MainViewModel**.
```xml
```
- Update the **`TextBox`** by binding the **`Text`** property to the **`Countable.Step`** property of the **MainViewModel**. The **`Mode`** of the binding is set to **`TwoWay`** so that the **`Countable.Step`** property is updated when the user changes the value in the **`TextBox`**.
```xml
```
- Update the **`Button`** to add a **`Command`** attribute that is bound to the **`IncrementCounter`** task of the **`MainViewModel`**.
```xml
```
The final code for **MainPage.xaml** should look like this:
```xml
```
[!INCLUDE [Wrap Up](includes/include-wrap.md)]
If you want to see the completed application, you can download the source code from [GitHub](https://github.com/unoplatform/Uno.Samples/tree/master/reference/Counter/XAML-MVUX).