{ "cells": [ { "cell_type": "markdown", "metadata": {}, "source": [ "# An Example Notebook\n", "\n", "This notebook is meant for testing conversion to other formats.\n", "\n", "It contains [Markdown cells](#Markdown), [code cells](#Code-Cells) with [different kinds of outputs](#Special-Display-Formats) and [raw cells](#Raw-Cells) with different formats." ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "## Markdown\n", "\n", "We can use *emphasis*, **boldface**, `preformatted text`.\n", "\n", "> It looks like strike-out text is not supported: ~~strikethrough~~.\n", "\n", "* Red\n", "* Green\n", "* Blue\n", "\n", "***\n", "\n", "1. One\n", "1. Two\n", "1. Three" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "### Equations\n", "\n", "Equations can be formatted really nicely, either inline, like $\\text{e}^{i\\pi} = -1$, or on a separate line, like\n", "\n", "$$\n", "\\int_{-\\infty}^\\infty f(x) \\delta(x - x_0) dx = f(x_0)\n", "$$" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "### Code\n", "\n", "We can also write code with nice syntax highlighting:\n", "\n", "```python\n", "print(\"Hello, world!\")\n", "```" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "### Tables\n", "\n", "A | B | A and B\n", "------|-------|--------\n", "False | False | False\n", "True | False | False\n", "False | True | False\n", "True | True | True" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "### Images\n", "\n", "PNG file (local): \n", "\n", "SVG file (local): \n", "\n", "PNG file (remote): 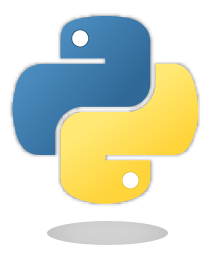\n", "\n", "SVG file (remote): " ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "### Links to Other Notebooks\n", "\n", "Relative links to local notebooks can be used: [a link to a notebook in a subdirectory](subdir/another.ipynb), [a link to an orphan notebook](orphan.ipynb) (latter won't work in LaTeX output, because orphan pages are not included there).\n", "\n", "This is how a link is created in Markdown:\n", "\n", "```\n", "[a link to a notebook in a subdirectory](subdir/another.ipynb)\n", "```\n", "\n", "Markdown also supports *reference-style* links: [a reference-style link][mylink], [another version of the same link][mylink].\n", "\n", "[mylink]: subdir/another.ipynb\n", "\n", "These can be created with this syntax:\n", "\n", "```\n", "[a reference-style link][mylink]\n", "\n", "[mylink]: subdir/another.ipynb\n", "```\n", "\n", "Links to sub-sections are also possible, e.g. [this subsection](subdir/another.ipynb#A-Sub-Section).\n", "\n", "This link was created with:\n", "\n", "```\n", "[this subsection](subdir/another.ipynb#A-Sub-Section)\n", "```\n", "\n", "You just have to remember to replace spaces with hyphens!\n", "\n", "BTW, links to sections of the current notebook work, too, e.g. [beginning of this section](#Links-to-Other-Notebooks).\n", "\n", "This can be done, as expected, like this:\n", "\n", "```\n", "[beginning of this section](#Links-to-Other-Notebooks)\n", "```" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "## Code Cells\n", "\n", "An empty code cell:" ] }, { "cell_type": "code", "execution_count": null, "metadata": { "collapsed": true }, "outputs": [], "source": [] }, { "cell_type": "markdown", "metadata": {}, "source": [ "A cell with no output:" ] }, { "cell_type": "code", "execution_count": null, "metadata": { "collapsed": true }, "outputs": [], "source": [ "None" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "A simple output:" ] }, { "cell_type": "code", "execution_count": null, "metadata": { "collapsed": false }, "outputs": [], "source": [ "6 * 7" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "The standard output stream:" ] }, { "cell_type": "code", "execution_count": null, "metadata": { "collapsed": false }, "outputs": [], "source": [ "print('Hello, world!')" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "Normal output + standard output" ] }, { "cell_type": "code", "execution_count": null, "metadata": { "collapsed": false }, "outputs": [], "source": [ "print('Hello, world!')\n", "6 * 7" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "The standard error stream is highlighted and displayed just below the code cell.\n", "The standard output stream comes afterwards (with no special highlighting).\n", "Finally, the \"normal\" output is displayed." ] }, { "cell_type": "code", "execution_count": null, "metadata": { "collapsed": false }, "outputs": [], "source": [ "import logging\n", "logging.warning('I am a warning and I will appear on the standard error stream')\n", "print('I will appear on the standard output stream')\n", "'I am the \"normal\" output'" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "## Special Display Formats\n", "\n", "See [IPython example notebook](https://nbviewer.jupyter.org/github/ipython/ipython/blob/master/examples/IPython Kernel/Rich Output.ipynb).\n", "\n", "TODO: tables? e.g. Pandas DataFrame?" ] }, { "cell_type": "code", "execution_count": null, "metadata": { "collapsed": false }, "outputs": [], "source": [ "from IPython.display import display, Image, SVG, Math, YouTubeVideo" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "### Local Image Files" ] }, { "cell_type": "code", "execution_count": null, "metadata": { "collapsed": false }, "outputs": [], "source": [ "i = Image(filename='images/notebook_icon.png')\n", "i" ] }, { "cell_type": "code", "execution_count": null, "metadata": { "collapsed": false }, "outputs": [], "source": [ "display(i)" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "For some reason this doesn't work with `Image(...)`:" ] }, { "cell_type": "code", "execution_count": null, "metadata": { "collapsed": false }, "outputs": [], "source": [ "SVG(filename='images/python_logo.svg')" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "### Image URLs" ] }, { "cell_type": "code", "execution_count": null, "metadata": { "collapsed": false }, "outputs": [], "source": [ "Image(url='https://www.python.org/static/img/python-logo-large.png')" ] }, { "cell_type": "code", "execution_count": null, "metadata": { "collapsed": false }, "outputs": [], "source": [ "Image(url='https://www.python.org/static/img/python-logo-large.png', embed=True)" ] }, { "cell_type": "code", "execution_count": null, "metadata": { "collapsed": false }, "outputs": [], "source": [ "Image(url='http://jupyter.org/assets/nav_logo.svg')" ] }, { "cell_type": "code", "execution_count": null, "metadata": { "collapsed": false }, "outputs": [], "source": [ "Image(url='https://www.python.org/static/favicon.ico')" ] }, { "cell_type": "code", "execution_count": null, "metadata": { "collapsed": false }, "outputs": [], "source": [ "Image(url='http://python.org/images/python-logo.gif')" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "### Math" ] }, { "cell_type": "code", "execution_count": null, "metadata": { "collapsed": false }, "outputs": [], "source": [ "eq = Math(r\"\\int_{-\\infty}^\\infty f(x) \\delta(x - x_0) dx = f(x_0)\")\n", "eq" ] }, { "cell_type": "code", "execution_count": null, "metadata": { "collapsed": false }, "outputs": [], "source": [ "display(eq)" ] }, { "cell_type": "code", "execution_count": null, "metadata": { "collapsed": false }, "outputs": [], "source": [ "%%latex\n", "\\begin{equation}\n", "\\int_{-\\infty}^\\infty f(x) \\delta(x - x_0) dx = f(x_0)\n", "\\end{equation}" ] }, { "cell_type": "code", "execution_count": null, "metadata": { "collapsed": false }, "outputs": [], "source": [ "YouTubeVideo('iV2ViNJFZC8')" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "## Raw Cells\n", "\n", "Cells with the cell type \"Raw NBConvert\" can have different formats.\n", "This information is stored in the notebook metadata.\n", "To select the format from within Jupyter, switch the cell toolbar to \"Raw Cell Format\"." ] }, { "cell_type": "raw", "metadata": {}, "source": [ "By default (if no cell format is selected), the cell content is included (without any conversion) in both the HTML and LaTeX output.\n", "This is typically not useful at all." ] }, { "cell_type": "raw", "metadata": { "raw_mimetype": "text/restructuredtext" }, "source": [ "Raw cells in \"reST\" format are interpreted as reStructuredText_ and parsed by Sphinx_.\n", "The result is visible in both HTML and LaTeX output.\n", "This way, links from Jupyter notebooks to reST pages are possible, e.g. :doc:`rst`.\n", "\n", ".. _reStructuredText: http://sphinx-doc.org/rest.html\n", ".. _Sphinx: http://sphinx-doc.org/" ] }, { "cell_type": "raw", "metadata": { "raw_mimetype": "text/markdown" }, "source": [ "Raw cells in \"Markdown\" format are interpreted as [Markdown](https://daringfireball.net/projects/markdown/) and the result is included in both HTML and LaTeX output.\n", "Since the Jupyter Notebook also supports \"normal\" Markdown cells, this might not be useful *at all*." ] }, { "cell_type": "raw", "metadata": { "raw_mimetype": "text/html" }, "source": [ "
Raw cells in “HTML” format are only included in HTML output (without any conversion).\n", "This might not be very useful, since raw HTML code is also allowed within “normal” Markdown cells.
\n", "Raw cells in “LaTeX” format are only included in LaTeX output.
" ] }, { "cell_type": "raw", "metadata": { "raw_mimetype": "text/latex" }, "source": [ "Raw cells in ``LaTeX'' format are only included in \\LaTeX\\ output (without any conversion).\n", "\n", "Raw cells in ``HTML'' format are only included in HTML output.\n", "This might not be \\emph{very useful}, since raw HTML code is also allowed within ``normal'' Markdown cells." ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "Raw cells in \"Python\" format are not shown at all (nor acted upon in any way)." ] }, { "cell_type": "raw", "metadata": { "raw_mimetype": "text/x-python" }, "source": [ "print(\"I'm a raw Python cell!\")" ] } ], "metadata": { "celltoolbar": "Raw Cell Format", "kernelspec": { "display_name": "Python 3", "language": "python", "name": "python3" }, "language_info": { "codemirror_mode": { "name": "ipython", "version": 3 }, "file_extension": ".py", "mimetype": "text/x-python", "name": "python", "nbconvert_exporter": "python", "pygments_lexer": "ipython3", "version": "3.4.4" } }, "nbformat": 4, "nbformat_minor": 0 }