{ "cells": [ { "cell_type": "markdown", "metadata": {}, "source": [ "# Python 入門\n", "\n", "\n", "\n", "## 事前須知\n", "\n", "* 今天重點放在幫沒寫過程式的朋友們打基礎\n", "* 碰到問題請馬上發問\n", "* 歡迎隨時打斷 (如果有哪邊聽不懂,那一定是我跳太快,請馬上反應)\n", "\n", "\n", "\n", "\n", "## 等等,Python 到底是啥啊?\n", "\n", "\n", "\n", "\n", "Python 是一個由 Guido van Rossum 在 1991 年 2 月釋出的程式語言,\n", "發展至今大約過了 20 餘年,\n", "廣泛使用於科學計算、網頁後端開發、系統工具等地方。\n", "\n", "Python 這名字是源自於當時 Guido van Rossum 在觀賞的 BBC 喜劇 「Monty Python's Flying Circus」。\n", "\n", "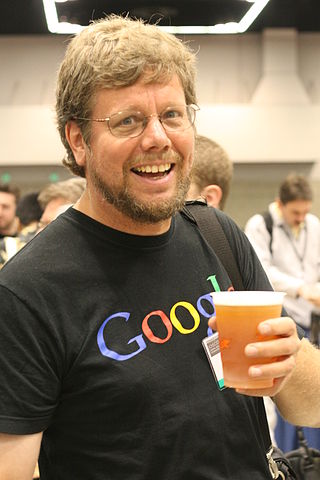\n", "\n", "----\n", "\n", "> [給稍微有經驗的程式設計師]\n", "> \n", "> Python 主要的特性\n", ">\n", "> * 強型別\n", "> * 物件導向\n", "> * 動態型別\n", "> * 直譯式\n", "\n" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "----\n", "\n", "大家可以先連上這個網站,\n", "可以直接在網頁上面寫 Python 練習 : **https://try.jupyter.org/**\n", "\n", "----" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "## 基本運算" ] }, { "cell_type": "code", "execution_count": 1, "metadata": { "collapsed": false }, "outputs": [ { "data": { "text/plain": [ "3" ] }, "execution_count": 1, "metadata": {}, "output_type": "execute_result" } ], "source": [ "1+2 # 加法" ] }, { "cell_type": "code", "execution_count": 2, "metadata": { "collapsed": false }, "outputs": [ { "data": { "text/plain": [ "-1" ] }, "execution_count": 2, "metadata": {}, "output_type": "execute_result" } ], "source": [ "3-4 # 減法" ] }, { "cell_type": "code", "execution_count": 3, "metadata": { "collapsed": false }, "outputs": [ { "data": { "text/plain": [ "30" ] }, "execution_count": 3, "metadata": {}, "output_type": "execute_result" } ], "source": [ "5*6 # 乘法" ] }, { "cell_type": "code", "execution_count": 4, "metadata": { "collapsed": false }, "outputs": [ { "data": { "text/plain": [ "0.875" ] }, "execution_count": 4, "metadata": {}, "output_type": "execute_result" } ], "source": [ "7/8 # 除法" ] }, { "cell_type": "code", "execution_count": 5, "metadata": { "collapsed": false }, "outputs": [ { "data": { "text/plain": [ "0" ] }, "execution_count": 5, "metadata": {}, "output_type": "execute_result" } ], "source": [ "7//8 # 除法,無條件捨去到個位數" ] }, { "cell_type": "code", "execution_count": 6, "metadata": { "collapsed": false }, "outputs": [ { "data": { "text/plain": [ "1" ] }, "execution_count": 6, "metadata": {}, "output_type": "execute_result" } ], "source": [ "10%9 # 取餘數" ] }, { "cell_type": "code", "execution_count": 7, "metadata": { "collapsed": false }, "outputs": [ { "data": { "text/plain": [ "1024" ] }, "execution_count": 7, "metadata": {}, "output_type": "execute_result" } ], "source": [ "2 ** 10 # 指數" ] }, { "cell_type": "code", "execution_count": 8, "metadata": { "collapsed": false }, "outputs": [ { "data": { "text/plain": [ "1.4142135623730951" ] }, "execution_count": 8, "metadata": {}, "output_type": "execute_result" } ], "source": [ "2 ** (1/2) # 開根號" ] }, { "cell_type": "code", "execution_count": 9, "metadata": { "collapsed": false }, "outputs": [ { "data": { "text/plain": [ "(1+2j)" ] }, "execution_count": 9, "metadata": {}, "output_type": "execute_result" } ], "source": [ "1+2j # 複數 (這裡虛部用 j 表示)" ] }, { "cell_type": "code", "execution_count": 10, "metadata": { "collapsed": false }, "outputs": [ { "data": { "text/plain": [ "(-5+10j)" ] }, "execution_count": 10, "metadata": {}, "output_type": "execute_result" } ], "source": [ "(1+2j) * (3+4j) # 複數乘法" ] }, { "cell_type": "code", "execution_count": 11, "metadata": { "collapsed": false }, "outputs": [ { "data": { "text/plain": [ "(0.36691394948660344+1.9660554808224875j)" ] }, "execution_count": 11, "metadata": {}, "output_type": "execute_result" } ], "source": [ "2 ** (1+2j) # 複數指數" ] }, { "cell_type": "code", "execution_count": 12, "metadata": { "collapsed": false }, "outputs": [ { "data": { "text/plain": [ "1" ] }, "execution_count": 12, "metadata": {}, "output_type": "execute_result" } ], "source": [ "abs(-1) # absolute, 絕對值" ] }, { "cell_type": "code", "execution_count": 13, "metadata": { "collapsed": false }, "outputs": [ { "data": { "text/plain": [ "2.23606797749979" ] }, "execution_count": 13, "metadata": {}, "output_type": "execute_result" } ], "source": [ "abs(1+2j) # absolute, 絕對值" ] }, { "cell_type": "code", "execution_count": 14, "metadata": { "collapsed": false }, "outputs": [ { "data": { "text/plain": [ "3.142" ] }, "execution_count": 14, "metadata": {}, "output_type": "execute_result" } ], "source": [ "round(3.1415926, 3) # 四捨五入到小數點後第 3 位" ] }, { "cell_type": "code", "execution_count": 15, "metadata": { "collapsed": false }, "outputs": [ { "data": { "text/plain": [ "3.1416" ] }, "execution_count": 15, "metadata": {}, "output_type": "execute_result" } ], "source": [ "round(3.1415926, 4) # 四捨五入到小數點後第 4 位" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "# 熟能生巧\n", "\n", "練習以下狀況\n", "\n", "* 42 的 100 次方\n", "* 虛數 i 的平方\n", "* 42 的四次方根\n", "\n", "補充 : 運算有先後順序,可以用括弧來確保自己想要的順序" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "# 賦值 (Assign)\n", "\n", "用 `=` 來給名字" ] }, { "cell_type": "code", "execution_count": 16, "metadata": { "collapsed": true }, "outputs": [], "source": [ "a = 1\n", "b = 1.5\n", "c = \"asd\"" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "# 型別 (Type)\n", "\n", "在程式語言中,型別概念上來說就像是物品的種類,例如:「這群是筆,那群是橡皮擦」。\n", "\n", "\n", "## Python 的內建型別 (Built-in Types)\n", "\n", "* int (整數)\n", "* float (浮點數)\n", "* complex (複數)\n", "* str (字串)\n", "* tuple\n", "* list\n", "* dict\n", "* set\n", "* ..." ] }, { "cell_type": "code", "execution_count": 17, "metadata": { "collapsed": false, "scrolled": true }, "outputs": [ { "data": { "text/plain": [ "int" ] }, "execution_count": 17, "metadata": {}, "output_type": "execute_result" } ], "source": [ "type(42) # 用 type 可以得知型別" ] }, { "cell_type": "code", "execution_count": 18, "metadata": { "collapsed": false }, "outputs": [ { "data": { "text/plain": [ "float" ] }, "execution_count": 18, "metadata": {}, "output_type": "execute_result" } ], "source": [ "type(3.14159265358979323846)" ] }, { "cell_type": "code", "execution_count": 19, "metadata": { "collapsed": false }, "outputs": [ { "data": { "text/plain": [ "'測試'" ] }, "execution_count": 19, "metadata": {}, "output_type": "execute_result" } ], "source": [ "\"測試\" # str, 字串" ] }, { "cell_type": "code", "execution_count": 20, "metadata": { "collapsed": false }, "outputs": [ { "data": { "text/plain": [ "'\\n我\\n是\\n多\\n行\\n字\\n串\\n'" ] }, "execution_count": 20, "metadata": {}, "output_type": "execute_result" } ], "source": [ "\"\"\"\n", "我\n", "是\n", "多\n", "行\n", "字\n", "串\n", "\"\"\" # 用 3 個 \" 夾起來" ] }, { "cell_type": "code", "execution_count": 21, "metadata": { "collapsed": false }, "outputs": [ { "data": { "text/plain": [ "'\\n我\\n是\\n多\\n行\\n字\\n串\\n'" ] }, "execution_count": 21, "metadata": {}, "output_type": "execute_result" } ], "source": [ "'''\n", "我\n", "是\n", "多\n", "行\n", "字\n", "串\n", "''' # 也可以用 3 個 ' 夾起來" ] }, { "cell_type": "code", "execution_count": 22, "metadata": { "collapsed": false }, "outputs": [ { "data": { "text/plain": [ "(42, 'O w O')" ] }, "execution_count": 22, "metadata": {}, "output_type": "execute_result" } ], "source": [ "(42, 'O w O') # tuple, 可以放入各種東西的大箱子,放完後不能更動" ] }, { "cell_type": "code", "execution_count": 23, "metadata": { "collapsed": false }, "outputs": [ { "data": { "text/plain": [ "42" ] }, "execution_count": 23, "metadata": {}, "output_type": "execute_result" } ], "source": [ "foo = (42, 'O w O')\n", "foo[0] # subscript operator 可以取出其中的值\n", " # 注意,index 從 0 開始算" ] }, { "cell_type": "code", "execution_count": 24, "metadata": { "collapsed": false }, "outputs": [ { "data": { "text/plain": [ "[42, 'O w O']" ] }, "execution_count": 24, "metadata": {}, "output_type": "execute_result" } ], "source": [ "[42, 'O w O'] # list 和 tuple 相似,但放完後可以更動" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "> 結構 (給比較有經驗的人)\n", "\n", "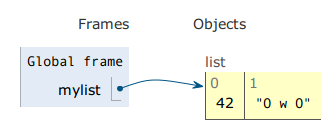" ] }, { "cell_type": "code", "execution_count": 25, "metadata": { "collapsed": false }, "outputs": [ { "data": { "text/plain": [ "{'a': 1, 'b': 42}" ] }, "execution_count": 25, "metadata": {}, "output_type": "execute_result" } ], "source": [ "{'a': 1, 'b': 42} # dict,冒號左邊的是 key、右邊的是 value,用 key 可以查到 value (想像查字典的狀況)" ] }, { "cell_type": "code", "execution_count": 26, "metadata": { "collapsed": false }, "outputs": [ { "data": { "text/plain": [ "42" ] }, "execution_count": 26, "metadata": {}, "output_type": "execute_result" } ], "source": [ "d = {'a': 1, 'b': 42}\n", "d['b']" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "> 結構\n", "\n", "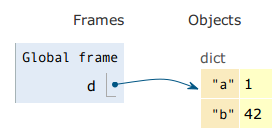" ] }, { "cell_type": "code", "execution_count": 27, "metadata": { "collapsed": false }, "outputs": [ { "data": { "text/plain": [ "{1, 2, 3}" ] }, "execution_count": 27, "metadata": {}, "output_type": "execute_result" } ], "source": [ "{1, 2, 3, 3, 3} # set,數學上的集合,裡面的東西不會重複,也沒有順序" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "> 結構\n", "\n", "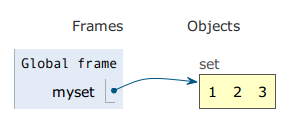" ] }, { "cell_type": "code", "execution_count": 28, "metadata": { "collapsed": false }, "outputs": [ { "data": { "text/plain": [ "{1, 2, 3, 4}" ] }, "execution_count": 28, "metadata": {}, "output_type": "execute_result" } ], "source": [ "A = {1, 2, 3}\n", "B = {1, 2, 4}\n", "A | B # 取聯集" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "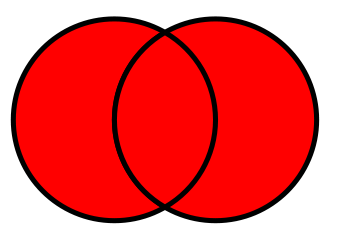" ] }, { "cell_type": "code", "execution_count": 29, "metadata": { "collapsed": false }, "outputs": [ { "data": { "text/plain": [ "{1, 2}" ] }, "execution_count": 29, "metadata": {}, "output_type": "execute_result" } ], "source": [ "A & B # 取交集" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "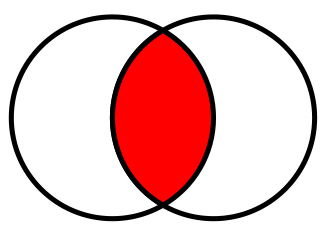" ] }, { "cell_type": "code", "execution_count": 30, "metadata": { "collapsed": false }, "outputs": [ { "data": { "text/plain": [ "{3, 4}" ] }, "execution_count": 30, "metadata": {}, "output_type": "execute_result" } ], "source": [ "A ^ B # 取對稱差" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "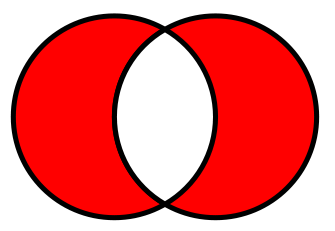" ] }, { "cell_type": "code", "execution_count": 31, "metadata": { "collapsed": false }, "outputs": [ { "data": { "text/plain": [ "{3}" ] }, "execution_count": 31, "metadata": {}, "output_type": "execute_result" } ], "source": [ "A - B # 取差集" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "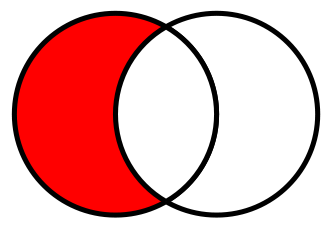" ] }, { "cell_type": "code", "execution_count": 32, "metadata": { "collapsed": false, "scrolled": true }, "outputs": [ { "data": { "text/plain": [ "['__abs__',\n", " '__add__',\n", " '__and__',\n", " '__bool__',\n", " '__ceil__',\n", " '__class__',\n", " '__delattr__',\n", " '__dir__',\n", " '__divmod__',\n", " '__doc__',\n", " '__eq__',\n", " '__float__',\n", " '__floor__',\n", " '__floordiv__',\n", " '__format__',\n", " '__ge__',\n", " '__getattribute__',\n", " '__getnewargs__',\n", " '__gt__',\n", " '__hash__',\n", " '__index__',\n", " '__init__',\n", " '__int__',\n", " '__invert__',\n", " '__le__',\n", " '__lshift__',\n", " '__lt__',\n", " '__mod__',\n", " '__mul__',\n", " '__ne__',\n", " '__neg__',\n", " '__new__',\n", " '__or__',\n", " '__pos__',\n", " '__pow__',\n", " '__radd__',\n", " '__rand__',\n", " '__rdivmod__',\n", " '__reduce__',\n", " '__reduce_ex__',\n", " '__repr__',\n", " '__rfloordiv__',\n", " '__rlshift__',\n", " '__rmod__',\n", " '__rmul__',\n", " '__ror__',\n", " '__round__',\n", " '__rpow__',\n", " '__rrshift__',\n", " '__rshift__',\n", " '__rsub__',\n", " '__rtruediv__',\n", " '__rxor__',\n", " '__setattr__',\n", " '__sizeof__',\n", " '__str__',\n", " '__sub__',\n", " '__subclasshook__',\n", " '__truediv__',\n", " '__trunc__',\n", " '__xor__',\n", " 'bit_length',\n", " 'conjugate',\n", " 'denominator',\n", " 'from_bytes',\n", " 'imag',\n", " 'numerator',\n", " 'real',\n", " 'to_bytes']" ] }, "execution_count": 32, "metadata": {}, "output_type": "execute_result" } ], "source": [ "dir(int) # 檢查可以使用的 method" ] }, { "cell_type": "code", "execution_count": 33, "metadata": { "collapsed": false, "scrolled": true }, "outputs": [ { "name": "stdout", "output_type": "stream", "text": [ "Help on class int in module builtins:\n", "\n", "class int(object)\n", " | int(x=0) -> integer\n", " | int(x, base=10) -> integer\n", " | \n", " | Convert a number or string to an integer, or return 0 if no arguments\n", " | are given. If x is a number, return x.__int__(). For floating point\n", " | numbers, this truncates towards zero.\n", " | \n", " | If x is not a number or if base is given, then x must be a string,\n", " | bytes, or bytearray instance representing an integer literal in the\n", " | given base. The literal can be preceded by '+' or '-' and be surrounded\n", " | by whitespace. The base defaults to 10. Valid bases are 0 and 2-36.\n", " | Base 0 means to interpret the base from the string as an integer literal.\n", " | >>> int('0b100', base=0)\n", " | 4\n", " | \n", " | Methods defined here:\n", " | \n", " | __abs__(self, /)\n", " | abs(self)\n", " | \n", " | __add__(self, value, /)\n", " | Return self+value.\n", " | \n", " | __and__(self, value, /)\n", " | Return self&value.\n", " | \n", " | __bool__(self, /)\n", " | self != 0\n", " | \n", " | __ceil__(...)\n", " | Ceiling of an Integral returns itself.\n", " | \n", " | __divmod__(self, value, /)\n", " | Return divmod(self, value).\n", " | \n", " | __eq__(self, value, /)\n", " | Return self==value.\n", " | \n", " | __float__(self, /)\n", " | float(self)\n", " | \n", " | __floor__(...)\n", " | Flooring an Integral returns itself.\n", " | \n", " | __floordiv__(self, value, /)\n", " | Return self//value.\n", " | \n", " | __format__(...)\n", " | \n", " | __ge__(self, value, /)\n", " | Return self>=value.\n", " | \n", " | __getattribute__(self, name, /)\n", " | Return getattr(self, name).\n", " | \n", " | __getnewargs__(...)\n", " | \n", " | __gt__(self, value, /)\n", " | Return self>value.\n", " | \n", " | __hash__(self, /)\n", " | Return hash(self).\n", " | \n", " | __index__(self, /)\n", " | Return self converted to an integer, if self is suitable for use as an index into a list.\n", " | \n", " | __int__(self, /)\n", " | int(self)\n", " | \n", " | __invert__(self, /)\n", " | ~self\n", " | \n", " | __le__(self, value, /)\n", " | Return self<=value.\n", " | \n", " | __lshift__(self, value, /)\n", " | Return self<<value.\n", " | \n", " | __lt__(self, value, /)\n", " | Return self<value.\n", " | \n", " | __mod__(self, value, /)\n", " | Return self%value.\n", " | \n", " | __mul__(self, value, /)\n", " | Return self*value.\n", " | \n", " | __ne__(self, value, /)\n", " | Return self!=value.\n", " | \n", " | __neg__(self, /)\n", " | -self\n", " | \n", " | __new__(*args, **kwargs) from builtins.type\n", " | Create and return a new object. See help(type) for accurate signature.\n", " | \n", " | __or__(self, value, /)\n", " | Return self|value.\n", " | \n", " | __pos__(self, /)\n", " | +self\n", " | \n", " | __pow__(self, value, mod=None, /)\n", " | Return pow(self, value, mod).\n", " | \n", " | __radd__(self, value, /)\n", " | Return value+self.\n", " | \n", " | __rand__(self, value, /)\n", " | Return value&self.\n", " | \n", " | __rdivmod__(self, value, /)\n", " | Return divmod(value, self).\n", " | \n", " | __repr__(self, /)\n", " | Return repr(self).\n", " | \n", " | __rfloordiv__(self, value, /)\n", " | Return value//self.\n", " | \n", " | __rlshift__(self, value, /)\n", " | Return value<<self.\n", " | \n", " | __rmod__(self, value, /)\n", " | Return value%self.\n", " | \n", " | __rmul__(self, value, /)\n", " | Return value*self.\n", " | \n", " | __ror__(self, value, /)\n", " | Return value|self.\n", " | \n", " | __round__(...)\n", " | Rounding an Integral returns itself.\n", " | Rounding with an ndigits argument also returns an integer.\n", " | \n", " | __rpow__(self, value, mod=None, /)\n", " | Return pow(value, self, mod).\n", " | \n", " | __rrshift__(self, value, /)\n", " | Return value>>self.\n", " | \n", " | __rshift__(self, value, /)\n", " | Return self>>value.\n", " | \n", " | __rsub__(self, value, /)\n", " | Return value-self.\n", " | \n", " | __rtruediv__(self, value, /)\n", " | Return value/self.\n", " | \n", " | __rxor__(self, value, /)\n", " | Return value^self.\n", " | \n", " | __sizeof__(...)\n", " | Returns size in memory, in bytes\n", " | \n", " | __str__(self, /)\n", " | Return str(self).\n", " | \n", " | __sub__(self, value, /)\n", " | Return self-value.\n", " | \n", " | __truediv__(self, value, /)\n", " | Return self/value.\n", " | \n", " | __trunc__(...)\n", " | Truncating an Integral returns itself.\n", " | \n", " | __xor__(self, value, /)\n", " | Return self^value.\n", " | \n", " | bit_length(...)\n", " | int.bit_length() -> int\n", " | \n", " | Number of bits necessary to represent self in binary.\n", " | >>> bin(37)\n", " | '0b100101'\n", " | >>> (37).bit_length()\n", " | 6\n", " | \n", " | conjugate(...)\n", " | Returns self, the complex conjugate of any int.\n", " | \n", " | from_bytes(...) from builtins.type\n", " | int.from_bytes(bytes, byteorder, *, signed=False) -> int\n", " | \n", " | Return the integer represented by the given array of bytes.\n", " | \n", " | The bytes argument must either support the buffer protocol or be an\n", " | iterable object producing bytes. Bytes and bytearray are examples of\n", " | built-in objects that support the buffer protocol.\n", " | \n", " | The byteorder argument determines the byte order used to represent the\n", " | integer. If byteorder is 'big', the most significant byte is at the\n", " | beginning of the byte array. If byteorder is 'little', the most\n", " | significant byte is at the end of the byte array. To request the native\n", " | byte order of the host system, use `sys.byteorder' as the byte order value.\n", " | \n", " | The signed keyword-only argument indicates whether two's complement is\n", " | used to represent the integer.\n", " | \n", " | to_bytes(...)\n", " | int.to_bytes(length, byteorder, *, signed=False) -> bytes\n", " | \n", " | Return an array of bytes representing an integer.\n", " | \n", " | The integer is represented using length bytes. An OverflowError is\n", " | raised if the integer is not representable with the given number of\n", " | bytes.\n", " | \n", " | The byteorder argument determines the byte order used to represent the\n", " | integer. If byteorder is 'big', the most significant byte is at the\n", " | beginning of the byte array. If byteorder is 'little', the most\n", " | significant byte is at the end of the byte array. To request the native\n", " | byte order of the host system, use `sys.byteorder' as the byte order value.\n", " | \n", " | The signed keyword-only argument determines whether two's complement is\n", " | used to represent the integer. If signed is False and a negative integer\n", " | is given, an OverflowError is raised.\n", " | \n", " | ----------------------------------------------------------------------\n", " | Data descriptors defined here:\n", " | \n", " | denominator\n", " | the denominator of a rational number in lowest terms\n", " | \n", " | imag\n", " | the imaginary part of a complex number\n", " | \n", " | numerator\n", " | the numerator of a rational number in lowest terms\n", " | \n", " | real\n", " | the real part of a complex number\n", "\n" ] } ], "source": [ "help(int) # 看文件上的解說" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "# 真偽值\n", "\n", "* True/False\n", "* `>` `>=` `<` `<=` `==` `!=` `in`\n", "* and/or/not\n", "* is\n", "* 各種 empty 狀況\n", " - 0, 1\n", " - \"\", \"abc\"\n", " - (,), (1, 2)\n", " - [], [1, 2]\n", " - set(), {1, 2}" ] }, { "cell_type": "code", "execution_count": 34, "metadata": { "collapsed": false }, "outputs": [ { "data": { "text/plain": [ "True" ] }, "execution_count": 34, "metadata": {}, "output_type": "execute_result" } ], "source": [ "True" ] }, { "cell_type": "code", "execution_count": 35, "metadata": { "collapsed": false }, "outputs": [ { "data": { "text/plain": [ "False" ] }, "execution_count": 35, "metadata": {}, "output_type": "execute_result" } ], "source": [ "False" ] }, { "cell_type": "code", "execution_count": 36, "metadata": { "collapsed": false }, "outputs": [ { "data": { "text/plain": [ "True" ] }, "execution_count": 36, "metadata": {}, "output_type": "execute_result" } ], "source": [ "3 > 2" ] }, { "cell_type": "code", "execution_count": 37, "metadata": { "collapsed": false }, "outputs": [ { "data": { "text/plain": [ "False" ] }, "execution_count": 37, "metadata": {}, "output_type": "execute_result" } ], "source": [ "5 <= 1" ] }, { "cell_type": "code", "execution_count": 38, "metadata": { "collapsed": false }, "outputs": [ { "data": { "text/plain": [ "True" ] }, "execution_count": 38, "metadata": {}, "output_type": "execute_result" } ], "source": [ "1 != 2" ] }, { "cell_type": "code", "execution_count": 39, "metadata": { "collapsed": false }, "outputs": [ { "data": { "text/plain": [ "True" ] }, "execution_count": 39, "metadata": {}, "output_type": "execute_result" } ], "source": [ "5 in [1, 2, 3, 4, 5]" ] }, { "cell_type": "code", "execution_count": 40, "metadata": { "collapsed": false }, "outputs": [ { "data": { "text/plain": [ "False" ] }, "execution_count": 40, "metadata": {}, "output_type": "execute_result" } ], "source": [ "bool(0) # 用 bool 來看各個資料是 True 還是 False" ] }, { "cell_type": "code", "execution_count": 41, "metadata": { "collapsed": false }, "outputs": [ { "data": { "text/plain": [ "True" ] }, "execution_count": 41, "metadata": {}, "output_type": "execute_result" } ], "source": [ "bool(42)" ] }, { "cell_type": "code", "execution_count": 42, "metadata": { "collapsed": false }, "outputs": [ { "data": { "text/plain": [ "(False, True)" ] }, "execution_count": 42, "metadata": {}, "output_type": "execute_result" } ], "source": [ "bool(\"\"), bool(\"abc\")" ] }, { "cell_type": "code", "execution_count": 43, "metadata": { "collapsed": false }, "outputs": [ { "data": { "text/plain": [ "(False, True)" ] }, "execution_count": 43, "metadata": {}, "output_type": "execute_result" } ], "source": [ "bool(tuple()), bool((1, 2))" ] }, { "cell_type": "code", "execution_count": 44, "metadata": { "collapsed": false }, "outputs": [ { "data": { "text/plain": [ "(False, True)" ] }, "execution_count": 44, "metadata": {}, "output_type": "execute_result" } ], "source": [ "bool([]), bool([1, 2])" ] }, { "cell_type": "code", "execution_count": 45, "metadata": { "collapsed": false }, "outputs": [ { "data": { "text/plain": [ "(False, True)" ] }, "execution_count": 45, "metadata": {}, "output_type": "execute_result" } ], "source": [ "bool(set()), bool({1, 2})" ] }, { "cell_type": "code", "execution_count": 46, "metadata": { "collapsed": false }, "outputs": [ { "data": { "text/plain": [ "(False, True)" ] }, "execution_count": 46, "metadata": {}, "output_type": "execute_result" } ], "source": [ "bool(dict()), bool({'a': 1, 'b': 42})" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "# 流程控制\n", "\n", "* if ... elif ... else\n", "* for ... in ...\n", "* break\n", "* continue\n", "* while ... else ..." ] }, { "cell_type": "code", "execution_count": 47, "metadata": { "collapsed": false }, "outputs": [ { "name": "stdout", "output_type": "stream", "text": [ "Yes\n" ] } ], "source": [ "if 3 > 2:\n", " print('Yes')\n", "else:\n", " print('Nooooooo, why can you come here?') # 如果 3 > 2 的話印出 'Yes',否則印出 'Nooooooo, why can you come here?'\n", " # 注意縮排是有影響的" ] }, { "cell_type": "code", "execution_count": 48, "metadata": { "collapsed": false }, "outputs": [ { "name": "stdout", "output_type": "stream", "text": [ "Yes\n" ] } ], "source": [ "if 2 > 3:\n", " print('No')\n", "elif 5 > 4:\n", " print('Yes')\n", "else:\n", " print('@_@?') # 如果 2 > 3 的話印出 'No',要不然 5 > 4 的話就印出 'Yes',否則印出 '@_@?'" ] }, { "cell_type": "code", "execution_count": 49, "metadata": { "collapsed": false }, "outputs": [ { "name": "stdout", "output_type": "stream", "text": [ "1\n", "2\n", "3\n", "4\n" ] } ], "source": [ "for i in [1, 2, 3, 4]: # i 會依序變成 1, 2, 3, 4 來執行下面 block 的程式,在這邊則是會被印出來\n", " print(i)" ] }, { "cell_type": "code", "execution_count": 50, "metadata": { "collapsed": false }, "outputs": [ { "name": "stdout", "output_type": "stream", "text": [ "1\n", "2\n" ] } ], "source": [ "for i in [1, 2, 3, 4]:\n", " if i == 3:\n", " break # 使用 break 來提早離開,當 i 變成 3 時就離開這個 for loop\n", " print(i)" ] }, { "cell_type": "code", "execution_count": 51, "metadata": { "collapsed": false, "scrolled": true }, "outputs": [ { "name": "stdout", "output_type": "stream", "text": [ "1\n", "2\n", "3\n", "4\n", "End\n" ] } ], "source": [ "for i in [1, 2, 3, 4]:\n", " print(i)\n", "else:\n", " print('End') # 如果前面的 for 成功跑完的話會執行這段" ] }, { "cell_type": "code", "execution_count": 52, "metadata": { "collapsed": false }, "outputs": [ { "name": "stdout", "output_type": "stream", "text": [ "1\n", "2\n" ] } ], "source": [ "for i in [1, 2, 3, 4]:\n", " if i == 3:\n", " break\n", " print(i)\n", "else:\n", " print('End') # 如果前面的 for 成功跑完的話會執行這段" ] }, { "cell_type": "code", "execution_count": 53, "metadata": { "collapsed": false }, "outputs": [ { "name": "stdout", "output_type": "stream", "text": [ "1\n", "2\n", "4\n", "End\n" ] } ], "source": [ "for i in [1, 2, 3, 4]:\n", " if i == 3:\n", " continue # continue 可以跳過這次後面的 code,進入下一輪\n", " print(i)\n", "else:\n", " print('End') # 如果前面的 for 成功跑完的話會執行這段" ] }, { "cell_type": "code", "execution_count": 54, "metadata": { "collapsed": false }, "outputs": [ { "name": "stdout", "output_type": "stream", "text": [ "4\n", "3\n", "2\n", "1\n" ] } ], "source": [ "i = 4\n", "while i > 0: # 當 while 後面接的條件一直符合時就會繼續執行\n", " print(i)\n", " i = i - 1" ] }, { "cell_type": "code", "execution_count": 55, "metadata": { "collapsed": false }, "outputs": [ { "name": "stdout", "output_type": "stream", "text": [ "4\n", "3\n", "2\n", "1\n", "End\n" ] } ], "source": [ "i = 4\n", "while i > 0: # 當 while 後面接的條件一直符合時就會繼續執行\n", " print(i)\n", " i = i - 1\n", "else:\n", " print('End')" ] }, { "cell_type": "code", "execution_count": 56, "metadata": { "collapsed": false }, "outputs": [ { "name": "stdout", "output_type": "stream", "text": [ "4\n" ] } ], "source": [ "i = 4\n", "while i > 0: # 當 while 後面接的條件一直符合時就會繼續執行\n", " if i == 3:\n", " break\n", " print(i)\n", " i = i - 1\n", "else:\n", " print('End')" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "# 更多字串操作\n", "\n", "* string formatting\n", "* 字串串接\n", "* slice : `start:stop[:step]`" ] }, { "cell_type": "code", "execution_count": 57, "metadata": { "collapsed": false }, "outputs": [ { "data": { "text/plain": [ "'1 | 2'" ] }, "execution_count": 57, "metadata": {}, "output_type": "execute_result" } ], "source": [ "'{} | {}'.format(1, 2) # 用 {} 表示要留著填空,後面用 .format 傳入要填進去的值" ] }, { "cell_type": "code", "execution_count": 58, "metadata": { "collapsed": false }, "outputs": [ { "data": { "text/plain": [ "'~ [1, 2, 3, 4] ~'" ] }, "execution_count": 58, "metadata": {}, "output_type": "execute_result" } ], "source": [ "'~ {} ~'.format([1, 2, 3, 4])" ] }, { "cell_type": "code", "execution_count": 59, "metadata": { "collapsed": false }, "outputs": [ { "data": { "text/plain": [ "'上上下下左右左右BA'" ] }, "execution_count": 59, "metadata": {}, "output_type": "execute_result" } ], "source": [ "'上上下下' + '左右左右' + 'BA' # 用 + 可以把字串接起來" ] }, { "cell_type": "code", "execution_count": 60, "metadata": { "collapsed": true }, "outputs": [], "source": [ "s = 'abcdefghijklmnopqrstuvwxyz'" ] }, { "cell_type": "code", "execution_count": 61, "metadata": { "collapsed": false }, "outputs": [ { "data": { "text/plain": [ "'a'" ] }, "execution_count": 61, "metadata": {}, "output_type": "execute_result" } ], "source": [ "s[0]" ] }, { "cell_type": "code", "execution_count": 62, "metadata": { "collapsed": false }, "outputs": [ { "data": { "text/plain": [ "'bcd'" ] }, "execution_count": 62, "metadata": {}, "output_type": "execute_result" } ], "source": [ "s[1:4] # index 1 到 index 3 (index 4 結束)" ] }, { "cell_type": "code", "execution_count": 63, "metadata": { "collapsed": false }, "outputs": [ { "data": { "text/plain": [ "'z'" ] }, "execution_count": 63, "metadata": {}, "output_type": "execute_result" } ], "source": [ "s[-1] # 負的 index 會倒回去" ] }, { "cell_type": "code", "execution_count": 64, "metadata": { "collapsed": false }, "outputs": [ { "data": { "text/plain": [ "'bdfh'" ] }, "execution_count": 64, "metadata": {}, "output_type": "execute_result" } ], "source": [ "s[1:8:2] # index 1 到 index 7,每次跳兩步" ] }, { "cell_type": "code", "execution_count": 65, "metadata": { "collapsed": false }, "outputs": [ { "data": { "text/plain": [ "'zxvt'" ] }, "execution_count": 65, "metadata": {}, "output_type": "execute_result" } ], "source": [ "s[-1:-9:-2]" ] }, { "cell_type": "code", "execution_count": 66, "metadata": { "collapsed": false }, "outputs": [ { "data": { "text/plain": [ "'zyxwvutsrqponmlkjihgfedcba'" ] }, "execution_count": 66, "metadata": {}, "output_type": "execute_result" } ], "source": [ "s[::-1] # 不指定的話會自動帶入值" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "# Function\n", "\n", "一些常用的 code 會寫成 function,之後要使用時直接呼叫就可以了" ] }, { "cell_type": "code", "execution_count": 67, "metadata": { "collapsed": false, "scrolled": true }, "outputs": [ { "name": "stdout", "output_type": "stream", "text": [ "Hello ~\n" ] } ], "source": [ "def hello(): # 用 def 定義一個 function 叫作 hello,負責輸出 'Hello ~'\n", " print('Hello ~')\n", "\n", "hello() # 呼叫" ] }, { "cell_type": "code", "execution_count": 68, "metadata": { "collapsed": false }, "outputs": [ { "name": "stdout", "output_type": "stream", "text": [ "42\n" ] } ], "source": [ "def f_42():\n", " return 42 # 用 return 來回傳資料\n", "\n", "x = f_42()\n", "print(x)" ] }, { "cell_type": "code", "execution_count": 69, "metadata": { "collapsed": false }, "outputs": [ { "name": "stdout", "output_type": "stream", "text": [ "13 15\n" ] } ], "source": [ "def f(a, b=3): # a 和 b 是參數,b 預設是 3\n", " return a+b\n", "\n", "x = f(10)\n", "y = f(10, 5)\n", "print(x, y)" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "# 總複習\n", "\n", "* 認識 Python\n", "* 基本運算\n", " - 加減乘除\n", " - 取餘數\n", " - 指數\n", " - 複數\n", " - 除法,無條件捨去到個位數 (//)\n", " - 絕對值 (abs)\n", " - 四捨五入 (round)\n", "* 型別\n", " - 基本型別\n", " + int\n", " + float\n", " + str\n", " * `\"`\n", " * `'`\n", " * `\"\"\"`\n", " * `'''`\n", " * `\\` escape\n", " + tuple\n", " + list\n", " + dict\n", " + set\n", " * `|`\n", " * `&`\n", " * `^`\n", "* 輔助工具\n", " - dir\n", " - help\n", " - type\n", "* 賦值 (Assign)\n", "* 真偽值\n", " - True/False\n", " - `>` `>=` `<` `<=` `==` `!=` `in`\n", " - and/or/not\n", " - is\n", " - 各種 empty 狀況\n", " + 0, 1\n", " + \"\", \"abc\"\n", " + (,), (1, 2)\n", " + [], [1, 2]\n", " + set(), {1, 2}\n", "* 流程控制 (注意冒號和縮排)\n", " - if ... elif ... else\n", " - for ... in ...\n", " - break\n", " - continue\n", " - while ... else ...\n", "* 更多字串操作\n", " - format string\n", " - 字串串接\n", " - slice : `start:stop[:step]`\n", "* function\n", " - def\n", " - return\n", " - arguments\n", " + defaults\n", " - function call\n", "* Slice\n", " - slice(start, stop[, step])\n", " - a[0], a[-1], a[1:99:3], a[-1:-11:-2], a[::-1] (不打的話就自動帶入頭尾), range(-1, -11, -2)\n" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "# 其他還有很多還沒包含的議題 (未全部列出)\n", "\n", "\n", "## Python 內建 features\n", "\n", "* method\n", " - list.pop, list.remove, list.append\n", " - ...\n", "* 轉型別\n", " - str(123)\n", "* 打包\n", " - `*`\n", " - `**`\n", "* module\n", " - import ...\n", " - from ... import ...\n", "* class\n", " - method\n", "* list comprehension\n", "* lambda function\n", "* 內建函數 (builtins)\n", " - len\n", " - sum\n", " - count\n", "* 三元運算子\n", "* 生成器 (generator / yield)\n", "* 例外事件處理 (try-except)\n", "* 函數裝飾 (decorator)\n", "* metaprogramming\n", "* 更多字串操作\n", " - string encoding/decoding\n", "* Python 3 和 Python 2 的差異\n", "\n", "## Python - 科學運算\n", "\n", "### SciPy\n", "\n", "* NumPy - 矩陣相關操作\n", "* Matplotlib - plotting\n", "* SymPy - symbolic mathematics\n", "* Pandas - Python Data Analysis Library\n", "* Scikits\n", "\n", "\n", "## 網頁開發\n", "\n", "* Django\n", "* Flask" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "# 其他資源\n", "\n", "* [PyFormat Using % and .format() for great good!](https://pyformat.info/)\n", "* [CheckiO](https://checkio.org/)\n", "* [Python Documentation](https://docs.python.org/3/)\n", "* [The Hitchhiker's Guide to Python!](http://docs.python-guide.org/en/latest/)\n", "* [Dive Into Python 3](http://getpython3.com/diveintopython3/)\n", "* [Why Are There So Many Pythons? A Python Implementation Comparison](http://www.toptal.com/python/why-are-there-so-many-pythons)" ] }, { "cell_type": "markdown", "metadata": { "collapsed": true }, "source": [ "# CheckiO\n", "\n", "[CheckiO](https://checkio.org/) 是一個專門為 Python 做的線上解題系統,上面有許多題目可以練習,解出來後還可以看別人的解法來學習、討論。\n", "\n", "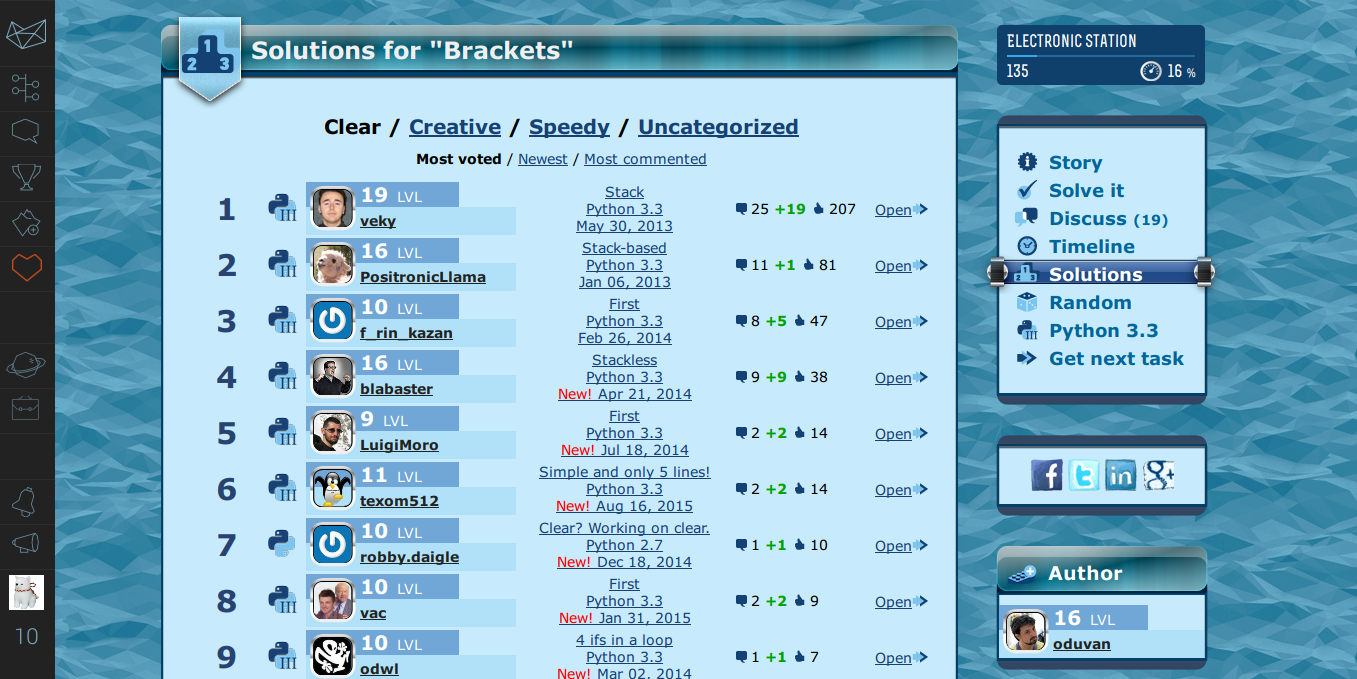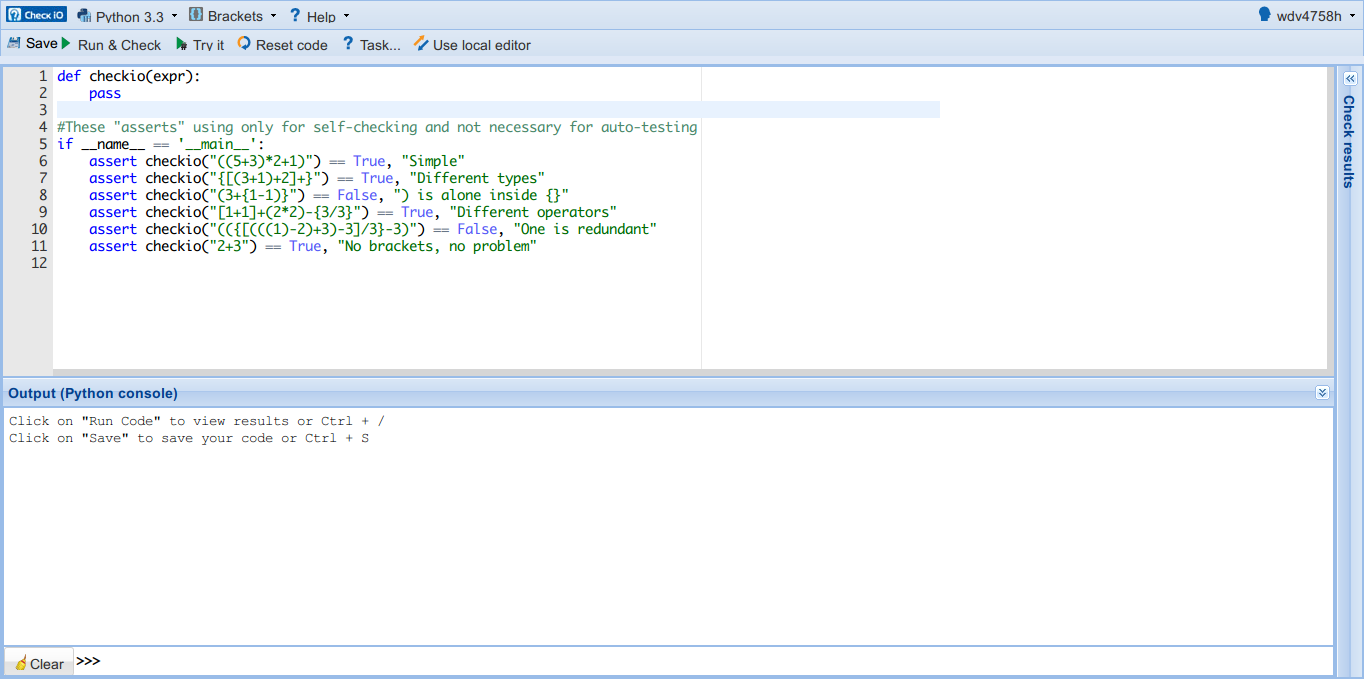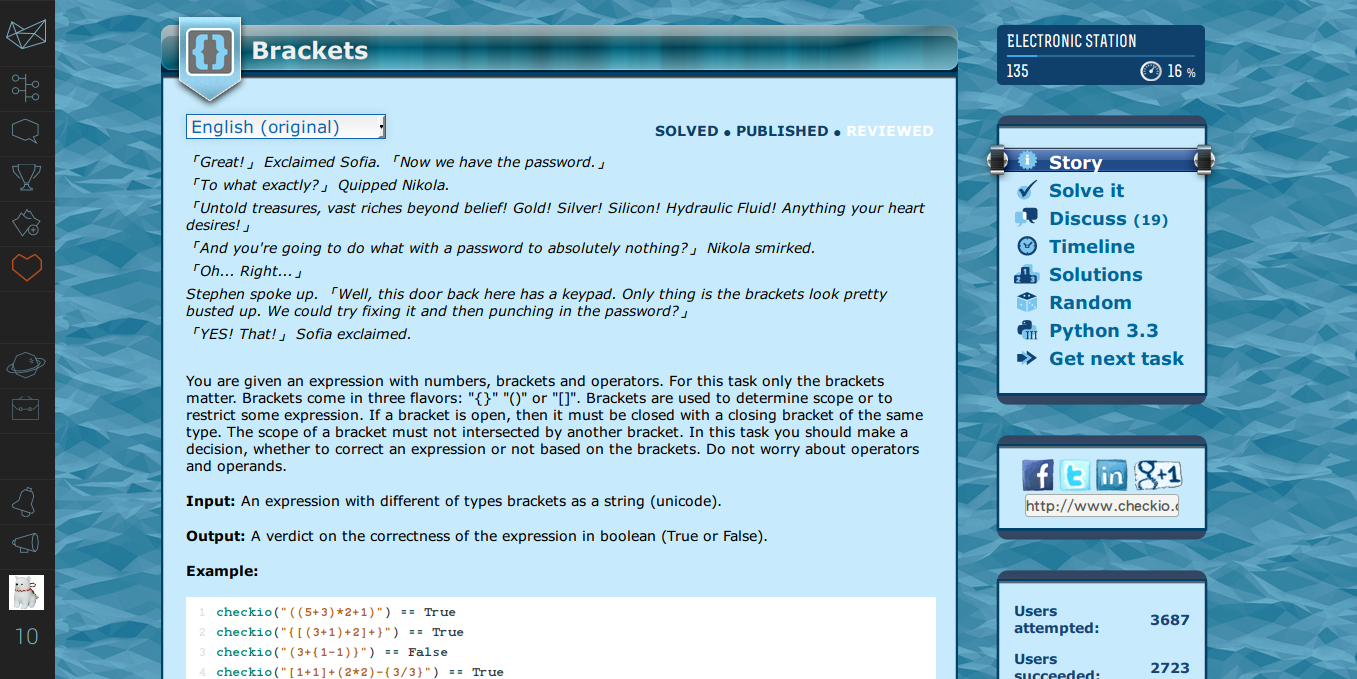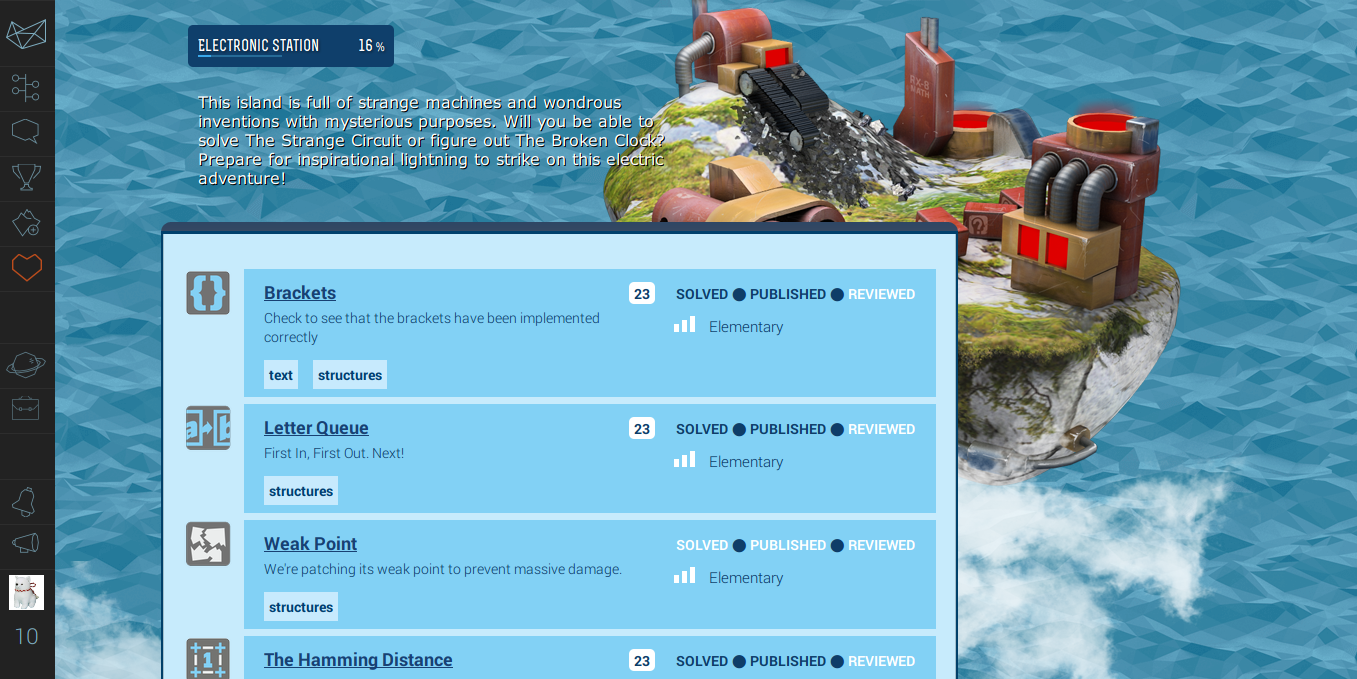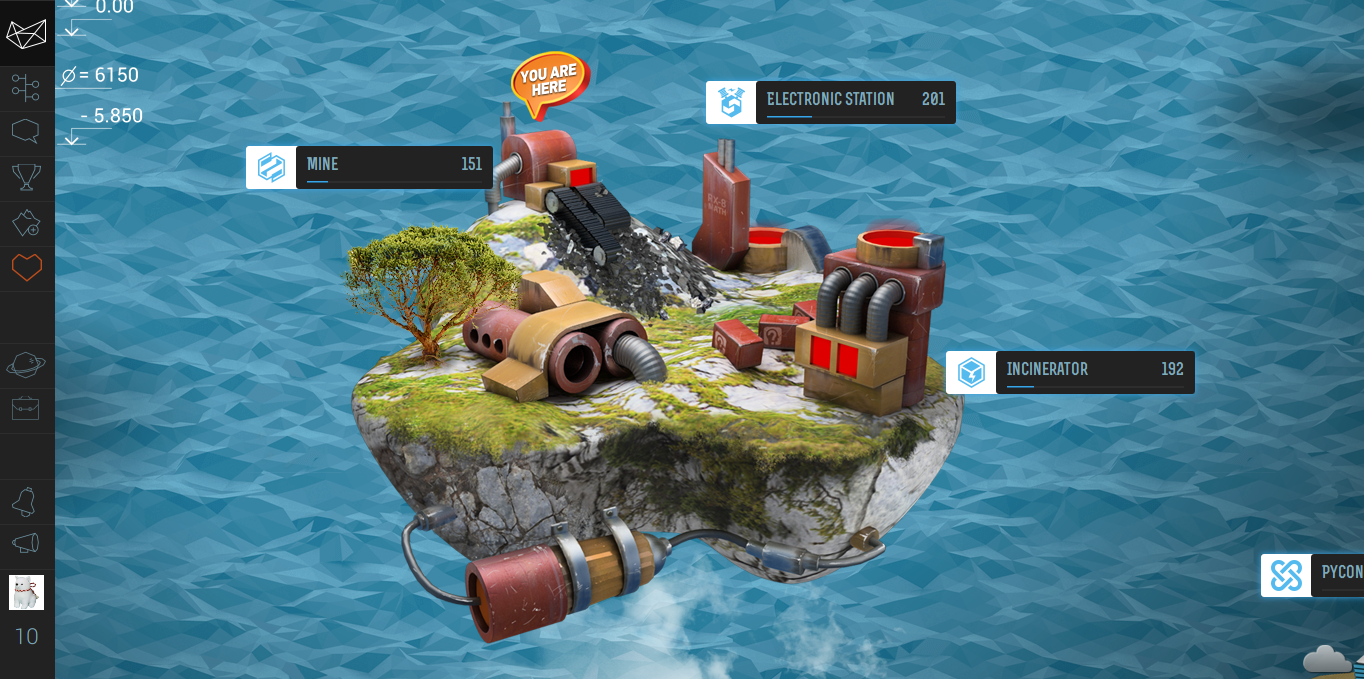" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "# 安裝\n", "\n", "## Linux\n", "\n", "通常系統內建就會有 Python,另外可以用內建的套件管理裝 Python 3\n", "\n", "\n", "## Mac\n", "\n", "系統上有 Python 2.7,可以用 MacPorts 或 Homebrew 安裝 Python 3\n", "\n", "\n", "## Windows\n", "\n", "可以從官網下載安裝檔\n", "\n", "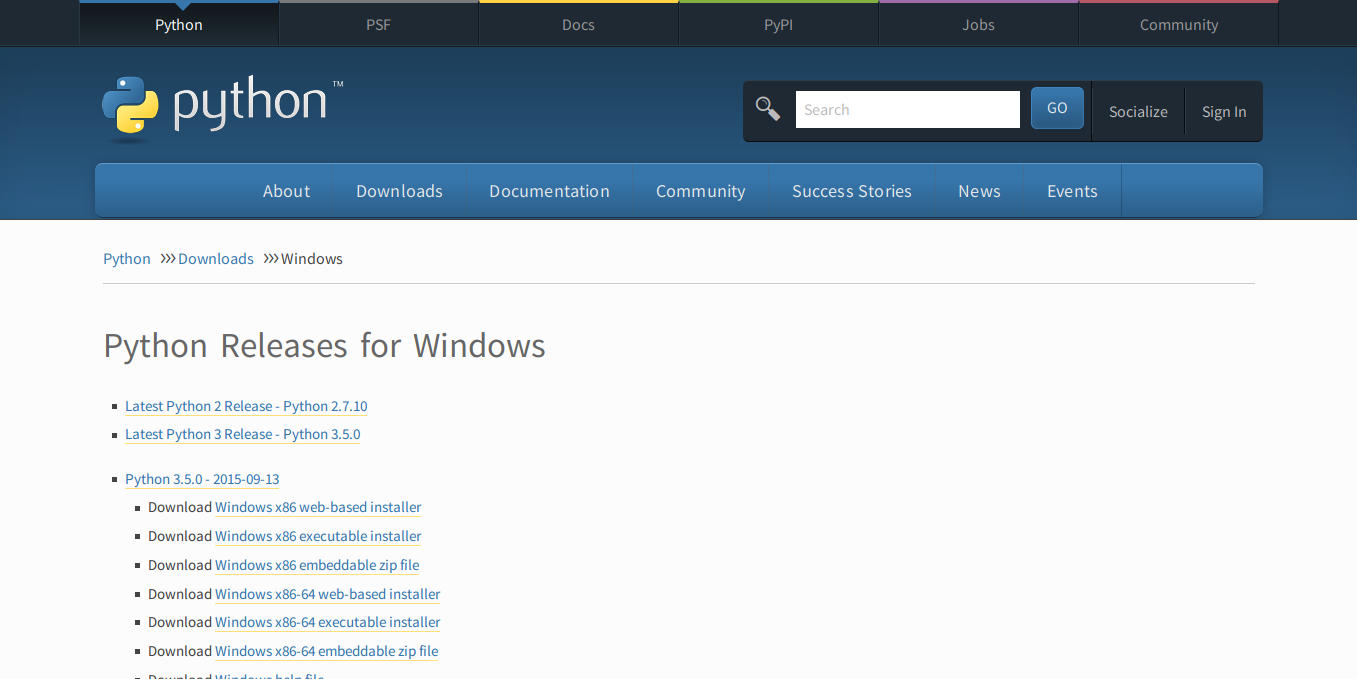" ] }, { "cell_type": "markdown", "metadata": {}, "source": [ "# 額外資訊\n", "\n", "## Python 實作\n", "\n", "[名詞解釋]\n", "\n", "* \"Python\" 指的是程式語言的規範\n", "* \"CPython\" 是官方 \"Python\" 直譯器實作的名稱\n", "\n", "### CPython\n", "\n", "Python 官方實作,核心部份使用 C 語言撰寫,部份 Standard Library 使用 Python 撰寫,對於 Python 的支援最完整。\n", "\n", "\n", "### PyPy\n", "\n", "利用 JIT (just-in-time compiler) 技術加速 Python 程式的專案,目標是要相容於 CPython 的同時大幅提升效能。\n", "\n", "> [更多訊息]\n", ">\n", "> PyPy 是用 RPython framework 撰寫的 Python interpreter,RPython 是一個 Python 的子集,RPython 限制了一些比較動態的 feature,\n", "> 藉此可以做 type inference,之後再做分析、優化,並生出 Tracing JIT、Garbage Collector。\n", "\n", "\n", "### Jython\n", "\n", "Java 版的 Python 實作,可以把 Python code 轉成 Java bytecode 在 JVM (Java Virtual Machine) 執行,除此之外可以 import Java 的 class\n", "\n", "\n", "### IronPython\n", "\n", "利用 .NET framework 實作的 Python,可以使用 .NET framework 的 libraries" ] } ], "metadata": { "kernelspec": { "display_name": "Python 3", "language": "python", "name": "python3" }, "language_info": { "codemirror_mode": { "name": "ipython", "version": 3 }, "file_extension": ".py", "mimetype": "text/x-python", "name": "python", "nbconvert_exporter": "python", "pygments_lexer": "ipython3", "version": "3.5.1" } }, "nbformat": 4, "nbformat_minor": 0 }