Macroの基
関数(
class(
Generator
[ Generator式 ]
Format
Macroの基
#!
#coding: utf-8
# python Marco
import uno
from com.sun.star.awt import Rectangle
#
def omsgbox(message):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), 'messbox', 1, 'Title', message)
return msgbox.execute()
def oHello():
try:
otext='Hello World in Python!!'
omsgbox(otext)
except:
pass
#
# [ Note ]
# Apache OpenOffice 4.0では createMessageBox の引数が変更になる模様( hanyaさんHome Pageより )
# LibreOffice4.0 では変更されていない。
# 多分
from com.sun.star.awt import Rectangle
def omsgbox(message):
・
・
msgbox = toolkit.createMessageBox(window, Rectangle(), 'messbox' , 1, 'Title', message)
↓
↓
from com.sun.star.awt.MessageBoxType import MESSAGEBOX
def omsgbox(message):
・
・
msgbox = toolkit.createMessageBox(window, MESSAGEBOX , 1, 'Title', message)
#
# つまり、Rectangle(), 'messbox' の2つの引数 → MESSAGEBOX の1つの引数になると思われる。
#
# Message Box : MESSAGEBOX
# Information Box : INFOBOX
# Error Box : ERRORBOX
# Warining Box : WARNINGBOX
# Question Box : QUERYBOX
#
# 因みに Apache OpenOffice4.0( Windows版 ) の Python versionは 2.7.5
#!
#coding: utf-8
# python Marco
import uno
#
def helloWorld():
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
win = frame.getContainerWindow()
toolkit = win.getToolkit()
rect = uno.createUnoStruct("com.sun.star.awt.Rectangle")
title = "title"
msg = "Hello World in Python!!"
msgbox = toolkit.createMessageBox(win, rect, "messbox", 1, title, msg)
msgbox.execute()
#
g_exportedScripts = helloWorld,
# [ LibreOffice ]
#
#!
#coding: utf-8
# python Marco
import re
import uno
import sys
import traceback
from com.sun.star.awt import Rectangle
def omsgbox(oMessage='',oBtnType=1,oTitle='Title',oMsgType='messbox' ):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), oMsgType , oBtnType, oTitle, oMessage)
return msgbox.execute()
def oTest():
try:
oDisp = u'処理を続けますか?'
oAns = omsgbox(oDisp,2,'Normal Box','messbox' )
oDisp = 'Return → ' + str(oAns)
except Exception as er:
oDisp = ''
oDisp = str(traceback.format_exc()) + '\n' + str(er)
finally:
omsgbox(oDisp,1)
#
#
# [ Apache OpenOffice ]
#!
#coding: utf-8
# python Marco
import re
import uno
import sys
import traceback
from com.sun.star.awt.MessageBoxType import MESSAGEBOX
def omsgbox(oMessage='',oBtnType=1,oTitle='Title'):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, MESSAGEBOX , oBtnType, oTitle, oMessage)
return msgbox.execute()
def oTest():
try:
oDisp = u'処理を続けますか?'
oAns = omsgbox(oDisp,2,'Normal Box')
oDisp = u'Return → ' + str(oAns)
except Exception as er:
oDisp = ''
oDisp = str(traceback.format_exc()) + '\n' + str(er)
finally:
omsgbox(oDisp,1)
#
# [ Button Type ]
# Ok : 1
# Ok / Cancel : 2
# Yes / No : 3
# Yes / No / Cancel : 4
# Retry / Cancel : 5
# Cancel / Retry / Ignore : 6
#
# [ Retrun ]
# Ok : 1
# Cancel(中止) : 0
# Yes : 2
# No : 3
# Retry : 4
# Ignore : 5
#!
#coding: utf-8
# python Marco
import re
import uno
import sys
import traceback
from com.sun.star.awt import Rectangle
def omsgbox(oMessage='',oBtnType=1,oTitle='Title',oMsgType='messbox'):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), oMsgType, oBtnType, oTitle, oMessage)
return msgbox.execute()
def oTest():
try:
oDisp = u'処理を続けますか?'
oAns = omsgbox(oDisp,1,'Information Box','infobox')
oDisp = 'Return → ' + str(oAns)
except Exception as er:
oDisp = ''
oDisp = str(traceback.format_exc()) + '\n' + str(er)
finally:
omsgbox(oDisp,1)
# Information Boxの場合はButton Type = 1(「Ok」)のみ
#!
#coding: utf-8
# python Marco
import re
import uno
import sys
import traceback
from com.sun.star.awt import Rectangle
def omsgbox(oMessage='',oBtnType=1,oTitle='Title',oMsgType='messbox'):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), oMsgType, oBtnType, oTitle, oMessage)
return msgbox.execute()
def oTest():
try:
oDisp = u'処理を続けますか?'
oAns = omsgbox(oDisp,3,'Error Box','errorbox')
oDisp = 'Return → ' + str(oAns)
except Exception as er:
oDisp = ''
oDisp = str(traceback.format_exc()) + '\n' + str(er)
finally:
omsgbox(oDisp,1)
#!
#coding: utf-8
# python Marco
import re
import uno
import sys
import traceback
from com.sun.star.awt import Rectangle
def omsgbox(oMessage='',oBtnType=1,oTitle='Title',oMsgType='messbox'):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), oMsgType, oBtnType, oTitle, oMessage)
return msgbox.execute()
def oTest():
try:
oDisp = u'処理を続けますか?'
oAns = omsgbox(oDisp,4,'Warning Box','warningbox')
oDisp = 'Return → ' + str(oAns)
except Exception as er:
oDisp = ''
oDisp = str(traceback.format_exc()) + '\n' + str(er)
finally:
omsgbox(oDisp,1)
#
# [ Note ]
# Return値に注意
# 2 : Yes
# 3 : No
# 0 : Cancel
#!
#coding: utf-8
# python Marco
import re
import uno
import sys
import traceback
from com.sun.star.awt import Rectangle
def omsgbox(oMessage='',oBtnType=1,oTitle='Title',oMsgType='messbox'):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), oMsgType, oBtnType, oTitle, oMessage)
return msgbox.execute()
def oTest():
try:
oDisp = u'処理を続けますか?'
oAns = omsgbox(oDisp,6,'Question Box','querybox')
oDisp = 'Return → ' + str(oAns)
except Exception as er:
oDisp = ''
oDisp = str(traceback.format_exc()) + '\n' + str(er)
finally:
omsgbox(oDisp,1)
#!
#coding: utf-8
# python Marco
import uno
import sys
import traceback
from com.sun.star.awt import Rectangle
from com.sun.star.awt import WindowDescriptor
from com.sun.star.awt.WindowClass import MODALTOP
from com.sun.star.awt.VclWindowPeerAttribute import OK,OK_CANCEL,\
YES_NO,YES_NO_CANCEL,RETRY_CANCEL
def omsgbox(dMsg,dBtnType = OK,dTitle = 'Title',dMsgType = 'messbox'):
"""shows messagebox"""
dDoc = XSCRIPTCONTEXT.getDocument() # <= Attension
dWin = dDoc.CurrentController.Frame.ContainerWindow
# Describe window properties
dDescriptor = WindowDescriptor()
dDescriptor.Type = MODALTOP
dDescriptor.WindowServiceName = dMsgType
dDescriptor.ParentIndex = -1
dDescriptor.Parent = dWin
dDescriptor.Bounds = Rectangle()
dDescriptor.WindowAttributes = dBtnType
#
tk = dWin.getToolkit()
dMsgbox = tk.createWindow(dDescriptor)
dMsgbox.setMessageText(dMsg)
dMsgbox.setCaptionText(dTitle)
return dMsgbox.execute()
#
def oTest():
try:
oDisp = 'Success'
omsgbox(oDisp,RETRY_CANCEL,'Messagebox')
except Exception as er:
oDisp = ''
oDisp = str(traceback.format_exc()) + '\n' + str(er)
omsgbox(oDisp)
#coding: utf-8
# python Marco
import uno
from com.sun.star.awt import Rectangle
def omsgbox(message):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), 'messbox', 1, 'Title', message)
return msgbox.execute()
def oTest():
a=b=1
try:
if a==1 and b==1:
otext='a=1 and b=1'
else: # <= Not to be forgotten :
otext='a!=1 or b!=1'
#
omsgbox(otext)
except:
pass
# python Marco
import uno
from com.sun.star.awt import Rectangle
def omsgbox(message):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), 'messbox', 1, 'Title', message)
return msgbox.execute()
def oTest():
try:
domain = 'jp'
if domain == "jp":
oC = 'Japan'
elif domain == "kr":
oC = 'Koria'
elif domain == "cn": #elif と else の違い
oC = 'China'
else:
oC = 'other'
#
omsgbox(oC)
except:
pass
#!
#coding: utf-8
# python Marco
import uno,os
from com.sun.star.awt import Rectangle
def omsgbox(message):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), 'messbox', 1, 'Title', message)
return msgbox.execute()
def oTest():
try:
oDir = 'c:/temp/MacroPython/temp'
oDirExist = os.path.isdir(oDir)
oDisp=''
a=1
if oDirExist != 1: # trueの時は「1」、falseの時は「0」を使う。!= 'true'ではelse以降が使えない
oDisp = 'The Directory is created!!'
else:
oDisp = 'The Directory existed!!'
omsgbox(oDisp)
except:
pass
#!
#coding: utf-8
# python Marco
import uno
from com.sun.star.awt import Rectangle
def omsgbox(message):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), 'messbox', 1, 'Title', message)
return msgbox.execute()
def oTest():
try:
num = 1
while num < 5:
oDisp = 'num = ' + str(num)
omsgbox(oDisp)
num += 1
except:
pass
#!
#coding: utf-8
# python Marco
import uno
from com.sun.star.awt import Rectangle
def omsgbox(message):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), 'messbox', 1, 'Title', message)
return msgbox.execute()
def oTest():
try:
num = 0
while num<=10:
num += 1;
else:
oDisp = num
omsgbox(oDisp)
except:
pass
#!
#coding: utf-8
# python Marco
import uno
from com.sun.star.awt import Rectangle
def omsgbox(message):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), 'messbox', 1, 'Title', message)
return msgbox.execute()
def oTest():
try:
for num in [4, 3, 12]:
oDisp = 'num = ' + str(num)
omsgbox(oDisp)
except:
pass
#!
#coding: utf-8
# python Marco
import uno
from com.sun.star.awt import Rectangle
def omsgbox(message):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), 'messbox', 1, 'Title', message)
return msgbox.execute()
def oTest():
try:
for str in "Hello":
oDisp = str
omsgbox(oDisp)
except:
pass
#!
#coding: utf-8
# python Marco
import uno
import sys
import traceback
from com.sun.star.awt import Rectangle
def omsgbox(message):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), 'messbox', 1, 'Title', message)
return msgbox.execute()
def oTest():
try:
oVal = 0
#for文
for i in range(10): # Rangeは不可( Basicの for i=0 to 9 と同じ )
oVal = oVal + i;
#omsgbox(i)
else:
oDisp = u'[ for文 ]\n → ' + str(oVal);
except Exception as er:
oDisp = ''
oDisp = str(traceback.format_exc()) + '\n' + str(er)
finally:
omsgbox(oDisp)
#!
#coding: utf-8
# python Marco
import uno
from com.sun.star.awt import Rectangle
def omsgbox(message):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), 'messbox', 1, 'Title', message)
return msgbox.execute()
def oTest():
try:
oSum = 0
for oNum in range(1, 10):
oSum += oNum;
else:
oDisp = oSum
omsgbox(oDisp)
except:
pass
#!
#coding: utf-8
# python Marco
import uno
import sys
import traceback
from com.sun.star.awt import Rectangle
def omsgbox(message):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), 'messbox', 1, 'Title', message)
return msgbox.execute()
def oTest():
try:
oVal = 0
#for文
for i in range(10): # Rangeは不可( Basicの for i=0 to 9 と同じ )
oVal = oVal + i;
if i == 5:
break;#omsgbox(i)
else:
pass;
oDisp = u'[ for文 ]\n → ' + str(oVal)
except Exception as er:
oDisp = ''
oDisp = str(traceback.format_exc()) + '\n' + str(er)
finally:
omsgbox(oDisp)
#!
#coding: utf-8
# python Marco
import uno
import sys
import traceback
from com.sun.star.awt import Rectangle
def omsgbox(message):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), 'messbox', 1, 'Title', message)
return msgbox.execute()
def oTest():
try:
oVal = 0
#for文
for i in range(100): # Rangeは不可( Basicの for i=0 to 9 と同じ )
if i % 10: # 10で割り切れないものはfalse( 0 )以外を足していく
continue;
else:
oVal = oVal + i;
else:
pass;
oDisp = u'[ for文 ]\n → ' + str(oVal)
except Exception as er:
oDisp = ''
oDisp = str(traceback.format_exc()) + '\n' + str(er)
finally:
omsgbox(oDisp)
#!
#coding: utf-8
# python Marco
import uno, keyword
from com.sun.star.awt import Rectangle
def omsgbox(message):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), 'messbox', 1, 'Title', message)
return msgbox.execute()
def oTest():
try:
oKeyWord = keyword.kwlist
omsgbox(str(oKeyWord))
except:
pass
#!
#coding: utf-8
# python Marco
import uno
import sys
import traceback
from com.sun.star.awt import Rectangle
def omsgbox(message):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), 'messbox', 1, 'Title', message)
return msgbox.execute()
def oTest():
try:
oVal = 100
#インクリメント
oVal += 1
oData1 = oVal
#デクリメント
oVal -= 2
oData2 = oVal
#
oDisp = u'[ インクリメント / デクリメント ]\n'\
+ u'デクリメント\n → ' + str(oData1) + '\n'\
+ u'デクリメント\n → ' + str(oData2)
except Exception as er:
oDisp = ''
oDisp = str(traceback.format_exc()) + '\n' + str(er)
finally:
omsgbox(oDisp)
#
# [ 注意 ]
# oVal++ ではError
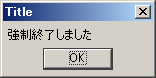
#!
#coding: utf-8
# python Marco
import uno
import sys
import traceback
from com.sun.star.awt import Rectangle
def omsgbox(message):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), 'messbox', 1, 'Title', message)
return msgbox.execute()
def oTest():
try:
oVal = 0
#for文
for i in range(10): # Rangeは不可( Basicの for i=0 to 9 と同じ )
oVal = oVal + i;
if i == 5:
oDisp = u'[ Exit文 ]\n'\
+ u'iが5になったので終了します。';
omsgbox(oDisp);
sys.exit()
except Exception as er:
oDisp = ''
oDisp = str(traceback.format_exc()) + '\n' + str(er)
except SystemExit:
oDisp = u'強制終了しました'
finally:
omsgbox(oDisp)
#!
#coding: utf-8
# python Marco
import uno
import sys
import traceback
from com.sun.star.awt import Rectangle
def omsgbox(message):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), 'messbox', 1, 'Title', message)
return msgbox.execute()
def oTest():
try:
oVal = 0
#for文
for i in range(100): # Rangeは不可( Basicの for i=0 to 9 と同じ )
if i % 10: # 10で割り切れないものはfalse( 0 )以外を足していく
continue;
else:
oVal = oVal + i;
else:
pass;
oDisp = u'[ for文 ]\n → ' + str(oVal)
except Exception as er:
oDisp = ''
oDisp = str(traceback.format_exc()) + '\n' + str(er)
finally:
oDisp = str('[ Finally ]') + '\n' + oDisp
omsgbox(oDisp)
関数
#!
#coding: utf-8
# python Marco
import uno
import sys
import traceback
from com.sun.star.awt import Rectangle
def omsgbox(message):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), 'messbox', 1, 'Title', message)
return msgbox.execute()
#
def getName():
name = "Fit"
return name
#
def getMaker():
maker = "Honda"
return maker
def oTest():
try:
#関数からname / makerを取得
oData1 = getName()
oData2 = getMaker()
oDisp = u'[ 関数 ]\n 車種 \t → ' + str(oData1) + u'\n メーカー \t → ' + str(oData2)
except Exception as er:
oDisp = ''
oDisp = str(traceback.format_exc()) + '\n' + str(er)
finally:
omsgbox(oDisp)
#!
#coding: utf-8
# python Marco
import uno
import sys
import traceback
from com.sun.star.awt import Rectangle
def omsgbox(message):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), 'messbox', 1, 'Title', message)
return msgbox.execute()
def oTest():
try:
oDisp = u'[ 定義されている関数/変数 ]' + '\n'
oDisp = oDisp + str(dir()) + '\n'
oDisp = oDisp + u'[ uno ] \n'
for i in dir(uno):
oDisp = oDisp + str(i) + '\n';
else:
pass
except Exception as er:
oDisp = ''
oDisp = str(traceback.format_exc()) + '\n' + str(er)
finally:
omsgbox(oDisp)
#!
#coding: utf-8
# python Marco
import uno
import sys
import traceback
from com.sun.star.awt import Rectangle
def omsgbox(message):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), 'messbox', 1, 'Title', message)
return msgbox.execute()
def otest():
try:
oLambFunc = lambda x,y,z: x+y+z
oLamb = oLambFunc(1,2,3)
#
def oFunc(x,y,z):
return x+y+z
oDefFunc = oFunc(1,2,3)
#
oDisp = u' 無名関数利用 \n\t → ' + str(oLamb) + '\n\n def関数利用 \n\t → ' + str(oDefFunc)
except Exception as er:
oDisp = ''
oDisp = str(traceback.format_exc()) + '\n' + str(er)
finally:
omsgbox(oDisp)
#
# lambaで作った関数は「無名関数」と呼ばれており,関数Objectが持つ関数名(__name__)が定義されない。
#!
#coding: utf-8
# python Marco
import uno
import sys
import traceback
from com.sun.star.awt import Rectangle
def omsgbox(message):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), 'messbox', 1, 'Title', message)
return msgbox.execute()
def otest():
try:
oFilter = filter(lambda x:x % 2 !=0 and x % 3 !=0,range(5,15))
#
oDisp = unicode('[ Filter関数 ]\n\n 2又は3で割切れない値抽出\n','utf-8')
oDisp = oDisp + 'filter(lambda x % 2 !=0 and x % 3 !=0,range(5,15) = ' + str(oFilter)
except:
oDisp = traceback.format_exc(sys.exc_info()[2])
finally:
omsgbox(oDisp)
#!
#coding: utf-8
# python Marco
import uno
import sys
import traceback
from com.sun.star.awt import Rectangle
def omsgbox(message):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), 'messbox', 1, 'Title', message)
return msgbox.execute()
def otest():
try:
oFilter = filter(lambda x:x % 2 !=0 and x % 3 !=0,range(5,15))
#
oDisp = u'[ Filter関数(3.3系) ]\n\n 5-14の間で 2 又は 3 で割切れない値抽出\n'
oDisp = oDisp + 'filter(lambda x % 2 !=0 and x % 3 !=0,range(5,15) = ' + str(tuple(oFilter))
except Exception as er:
oDisp = ''
oDisp = str(traceback.format_exc()) + '\n' + str(er)
finally:
omsgbox(oDisp)
#
# [ Note ]
# filter()のReturnはiterable
#!
#coding: utf-8
# python Marco
import uno
import sys
import traceback
from com.sun.star.awt import Rectangle
def omsgbox(message):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), 'messbox', 1, 'Title', message)
return msgbox.execute()
def otest():
try:
oMap = map(lambda x:pow(x,3),range(5,11))
#
oDisp = unicode('[ map関数 ]\n\n 元の値に3乗したList作成\n','utf-8')
oDisp = oDisp + 'map(lambda x:pow(x,3),range(5,11)) = ' + str(oMap)
except:
oDisp = traceback.format_exc(sys.exc_info()[2])
finally:
omsgbox(oDisp)
#!
#coding: utf-8
# python Marco
import uno
import sys
import traceback
from com.sun.star.awt import Rectangle
def omsgbox(message):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), 'messbox', 1, 'Title', message)
return msgbox.execute()
def otest():
try:
oMap = map(lambda x:pow(x,3),range(5,11)) # 5,6,7,8,9,10
#
oDisp = u'[ map関数(3.3系) ]\n\n 元の値に3乗したList作成\n'
oDisp = oDisp + 'map(lambda x:pow(x,3),range(5,11)) = ' + str(tuple(oMap))
except Exception as er:
oDisp = ''
oDisp = str(traceback.format_exc()) + '\n' + str(er)
finally:
omsgbox(oDisp)
#
# [ Note ]
# map()のReturnはiterable
#!
#coding: utf-8
# python Marco
import uno
import sys
import traceback
from com.sun.star.awt import Rectangle
def omsgbox(message):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), 'messbox', 1, 'Title', message)
return msgbox.execute()
def otest():
try:
oInit = 3 # 初期値
oReduce = reduce(lambda x,y:x*y,range(1,6),oInit)
# 上式を別Codeで記すと以下の様になる。
oCal = oInit
for v in range(1,6):
oCal = oCal * v
#
oDisp = unicode('[ reduce関数 ]\n\n List要素に順に関数を適用する\n','utf-8')
oDisp = oDisp + 'reduce(lambda x,y:x*y,range(1,6)) = ' + str(oReduce) + '\n\n'
oDisp = oDisp + unicode('別Codeでの計算結果 => ','utf-8') + str(oCal)
except:
oDisp = traceback.format_exc(sys.exc_info()[2])
finally:
omsgbox(oDisp)
#!
#coding: utf-8
# python Marco
import uno
from functools import reduce
import sys
import traceback
from com.sun.star.awt import Rectangle
def omsgbox(message):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), 'messbox', 1, 'Title', message)
return msgbox.execute()
def otest():
try:
oInit = 3 # 初期値
oReduce = reduce(lambda x,y:x*y,range(1,6),oInit)
# 上式を別Codeで記すと以下の様になる。
oCal = oInit
for v in range(1,6):
oCal = oCal * v
#
oDisp = u'[ reduce関数(3.3系) ]\n\n List要素に順に関数を適用する\n'
oDisp = oDisp + 'reduce(lambda x,y:x*y,range(1,6)) = ' + str(oReduce) + '\n\n'
oDisp = oDisp + u'別Codeでの計算結果 → ' + str(oCal)
except Exception as er:
oDisp = ''
oDisp = str(traceback.format_exc()) + '\n' + str(er)
finally:
omsgbox(oDisp)
#!
#coding: utf-8
# python Marco
import uno
import sys
import traceback
from com.sun.star.awt import Rectangle
def omsgbox(message):
"""shows message定義"""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), 'messbox', 1, 'Title', message)
return msgbox.execute()
def otest():
try:
oDocStr = omsgbox.__doc__
oDisp = unicode('[ documentation文字列 ]\n','utf-8')\
+ unicode(oDocStr,'utf-8')
except:
oDisp = traceback.format_exc(sys.exc_info()[2])
finally:
omsgbox(oDisp)
#
# def文やclass文のすぐ下にある文字列はdocumentation string(ドキュメンテーション文字列)と呼ばれる。
# 関数やclassのの説明を記す
#!
#coding: utf-8
# python Marco
import uno
import sys
import traceback
from com.sun.star.awt import Rectangle
def omsgbox(message):
"""shows message定義"""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), 'messbox', 1, 'Title', message)
return msgbox.execute()
def otest():
try:
oDocStr = omsgbox.__doc__
oDisp = u'[ documentation文字列(3.3系) ]\n' + str(oDocStr)
except Exception as er:
oDisp = ''
oDisp = str(traceback.format_exc()) + '\n' + str(er)
finally:
omsgbox(oDisp)
#!
#coding: utf-8
# python Marco
import inspect
import uno
import sys
import traceback
from com.sun.star.awt import Rectangle
def omsgbox(message):
"""shows message定義"""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), 'messbox', 1, 'Title', message)
return msgbox.execute()
def otest():
try:
oDocStr = inspect.getdoc(omsgbox)
oDisp = u'[ documentation文字列 ]\n'\
+ unicode(oDocStr,'utf-8')
except:
oDisp = traceback.format_exc(sys.exc_info()[2])
finally:
omsgbox(oDisp)
#!
#coding: utf-8
# python Marco
import inspect
import uno
import sys
import traceback
from com.sun.star.awt import Rectangle
def omsgbox(message):
"""shows message定義"""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), 'messbox', 1, 'Title', message)
return msgbox.execute()
def otest():
try:
oDocStr = inspect.getdoc(omsgbox)
oDisp = u'[ documentation文字列2(3.3系) ]\n' + str(oDocStr)
except Exception as er:
oDisp = ''
oDisp = str(traceback.format_exc()) + '\n' + str(er)
finally:
omsgbox(oDisp)
#!
#coding: utf-8
# python Marco
import inspect
import uno
import sys
import traceback
from com.sun.star.awt import Rectangle
def omsgbox(message):
"""shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), 'messbox', 1, 'Title', message)
return msgbox.execute()
# Class Toyota
class Toyota: # Classは不可
compact = 'Vitz'
maker = 'Toyota'
# Compact車とメーカーを取得する関数
def __init__(self, arg1,arg2):
self.shashu = arg1
self.maker = arg2
#
def oTest():
try:
oIsClass1 = inspect.isfunction(Toyota)
oIsClass2 = inspect.isfunction(omsgbox)
oDisp = u'[ functionのCheck ]\n'\
+ 'Is Toypta function? : ' + str(oIsClass1) + '\n'\
+ 'Is omsgbox function? : ' + str(oIsClass2)
except Exception as er:
oDisp = ''
oDisp = str(traceback.format_exc()) + '\n' + str(er)
finally:
omsgbox(oDisp)
Class
#!
#coding: utf-8
# python Marco
import uno
import sys
import traceback
from com.sun.star.awt import Rectangle
def omsgbox(message):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), 'messbox', 1, 'Title', message)
return msgbox.execute()
# Class Toyota
class Toyota: # Classは不可
shashu = 'Vitz'
maker = 'Toyota'
# 車種を取得する関数
def __init__(self, arg1,arg2):
self.shashu = arg1
self.maker = arg2
# Class Honda
class Honda: # Classは不可
shashu = 'Fit'
maker = 'Honda'
# 車種を取得する関数
def __init__(self, arg1,arg2):
self.shashu = arg1
self.maker = arg2
# Class Nissan
class Nissan: # Classは不可
shashu = 'March'
maker = 'Nissan'
# 車種を取得する関数
def __init__(self, arg1,arg2):
self.shashu = arg1
self.maker = arg2
#
def oTest():
try:
# classからname / makerを取得
oData1t = Toyota.shashu
oData2t = Toyota.maker
oData1h = Honda.shashu
oData2h = Honda.maker
oData1n = Nissan.shashu
oData2n = Nissan.maker
#
oDisp = u'[ Class ]\n'\
+ u'\t 車種 → ' + str(oData1t) + '\n\t' + u'メーカー → ' + str(oData2t) + '\n\n'\
+ u'\t 車種 → ' + str(oData1h) + '\n\t' + u'メーカー → ' + str(oData2h) + '\n\n'\
+ u'\t 車種 → ' + str(oData1n) + '\n\t' + u'メーカー → ' + str(oData2n)
except Exception as er:
oDisp = ''
oDisp = str(traceback.format_exc()) + '\n' + str(er)
finally:
omsgbox(oDisp)
#
# [ 注意 ]
# 1) class名の先頭文字は大文字。但し、これは単なる慣例であり、要求ではない。
# 2) Python には class 文でインスタンス変数を定義する特別な構文はありません。
# 3) 関数のローカル変数と同様に、インスタンス変数への代入が行われると、Python はその変数をインスタンス内に生成します。
# 4) __init__()という関数(method)は初期化method。
# 5) Pythonのclassには、Underscore( _ )2つで始まるmethodが何種類か利用でき、それらは特殊methodと呼ばれています。__init__()も特殊methodの一種です。
# 6) 第1引数にはselfを定義しておきます。この引数は、classのinstance object自体が暗黙に渡されます。
#
#!
#coding: utf-8
# python Marco
import uno
import sys
import traceback
from com.sun.star.awt import Rectangle
def omsgbox(message):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), 'messbox', 1, 'Title', message)
return msgbox.execute()
# Class Toyota
class Toyota: # Classは不可
compact = 'Vitz'
maker = 'Toyota'
# Compact車とメーカーを取得する関数
def __init__(self, arg1,arg2):
self.shashu = arg1
self.maker = arg2
# Sub Class
class SubToyota(Toyota): # class名の先頭文字は大文字
rv = 'RAV-4'
def __init__(self,arg1):
self.rv = arg1
#
def oTest():
try:
# classからname / makerを取得
oData1t = SubToyota.maker
oData2t = SubToyota.compact
oData3t = SubToyota.rv
#
oDisp = u'[ Classの継承 ]\n\t'\
+ u'メーカー ⇒ ' + str(oData1t) + '\n\t' + u'Compact車種 ⇒ ' + str(oData2t) + '\n\t' + u'RV車種 ⇒ ' + str(oData3t)
except Exception as er:
oDisp = ''
oDisp = str(traceback.format_exc()) + '\n' + str(er)
finally:
omsgbox(oDisp)
#!
#coding: utf-8
# python Marco
import uno
import sys
import traceback
from com.sun.star.awt import Rectangle
def omsgbox(message):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), 'messbox', 1, 'Title', message)
return msgbox.execute()
def oTest():
try:
class V(): # class名の先頭文字は大文字
pass
def oDiff(a,b):
return a-b
#
setattr(V,'a',10)
setattr(V,'b',5)
oData1 = oDiff(5,2) # 5-2
oData2 = V.a
oData3 = V.b
#
oDisp = u'[ setattr ]\n\t' + 'oData1 = ' + str(oData1) + '\n\t' + 'oData2 = ' + str(oData2) + '\n\t' + 'oData3 = ' + str(oData3)
except Exception as er:
oDisp = ''
oDisp = str(traceback.format_exc()) + '\n' + str(er)
finally:
omsgbox(oDisp)
#!
#coding: utf-8
# python Marco
import uno
import sys
import traceback
from com.sun.star.awt import Rectangle
def omsgbox(message):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), 'messbox', 1, 'Title', message)
return msgbox.execute()
def oTest():
try:
class V(): # class名の先頭文字は大文字
pass
def oDiff(a,b):
return a-b
#
setattr(V,'a',oDiff(10,2))
setattr(V,'b','OK')
oData1 = oDiff(5,2) # 5-2
oData2 = getattr(V,'a') # = V.a
oData3 = getattr(V,'b') # = V.b
#
oDisp = u'[ setattr / getattr ]\n\t' + 'oData1 = ' + str(oData1) + '\n\t' + 'oData2 = ' + str(oData2) + '\n\t'\
+ 'oData3 = ' + str(oData3)
except Exception as er:
oDisp = ''
oDisp = str(traceback.format_exc()) + '\n' + str(er)
finally:
omsgbox(oDisp)
#!
#coding: utf-8
# python Marco
import uno
import sys
import traceback
from com.sun.star.awt import Rectangle
def omsgbox(message):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), 'messbox', 1, 'Title', message)
return msgbox.execute()
def oTest():
try:
class V(): # class名の先頭文字は大文字
pass
def oDiff(a,b):
return a-b
#
setattr(V,'a',oDiff(10,2))
setattr(V,'b','OK')
oData1 = hasattr(V,'a')
oData2 = hasattr(V,'b')
oData3 = hasattr(V,'c')
#
oDisp = u'[ hasattr ]\n\t' + 'hasattr(V,\'a\') = ' + str(oData1) + '\n\t' + 'hasattr(V,\'b\') = ' + str(oData2) + '\n\t'\
+ 'hasattr(V,\'c\') = ' + str(oData3)
except Exception as er:
oDisp = ''
oDisp = str(traceback.format_exc()) + '\n' + str(er)
finally:
omsgbox(oDisp)
#!
#coding: utf-8
# python Marco
import uno
import sys
import traceback
from com.sun.star.awt import Rectangle
def omsgbox(message):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), 'messbox', 1, 'Title', message)
return msgbox.execute()
def oTest():
try:
class V(): # class名の先頭文字は大文字
pass
def oDiff(a,b):
return a-b
#
setattr(V,'a',oDiff(10,2))
setattr(V,'b','OK')
oData1 = str(hasattr(V,'a'))
oData2 = str(hasattr(V,'b'))
#
delattr(V,'a') # = del oV.a
oDel1 = str(hasattr(V,'a'))
del V.b
oDel2 = str(hasattr(V,'b'))
#
oDisp = u'[ delattr / del ]\n\t' + u'削除前hasattr(V,\'a\') = ' + oData1 + '\n\t' + u'削除前hasattr(V,\'b\') = ' + oData2 + '\n\t'\
+ u'削除後hasattr(V,\'a\') = ' + oDel1 + '\n\t' + u'削除後hasattr(V,\'b\') = ' + oDel2
except Exception as er:
oDisp = ''
oDisp = str(traceback.format_exc()) + '\n' + str(er)
finally:
omsgbox(oDisp)
#!
#coding: utf-8
# python Marco
import uno
import sys
import traceback
from com.sun.star.awt import Rectangle
def omsgbox(oMessage='',oBtnType=1,oTitle='Title',oMsgType='messbox'):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), oMsgType, oBtnType, oTitle, oMessage)
return msgbox.execute()
class Toyota:
"""A simple example class"""
shashu = 'Vitz'
maker = 'Toyota'
# 車種を取得する関数
def __init__(self, arg1,arg2):
self.shashu = arg1
self.maker = arg2
def otest():
try:
oDisp = str(Toyota.__doc__) + '\n\t' + str(Toyota.shashu) + '\n\t'+ str(Toyota.maker)
except Exception as er:
oDisp = ''
oDisp = str(traceback.format_exc()) + '\n' + str(er)
finally:
omsgbox(oDisp,1)
#
# def文やclass文のすぐ下にある文字列はdocumentation string(ドキュメンテーション文字列)と呼ばれる。
# 関数やclassのの説明を記す
#!
#coding: utf-8
# python Marco
import inspect
import uno
import sys
import traceback
from com.sun.star.awt import Rectangle
def omsgbox(oMessage='',oBtnType=1,oTitle='Title',oMsgType='messbox'):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), oMsgType, oBtnType, oTitle, oMessage)
return msgbox.execute()
class Toyota:
"""TOYOTAの車種"""
shashu = 'Vitz'
maker = 'Toyota'
# 車種を取得する関数
def __init__(self, arg1,arg2):
self.shashu = arg1
self.maker = arg2
def otest():
try:
oDocStr = inspect.getdoc(Toyota)
oDisp = u'[ classのdocumentation文字列 ]\n' + str(oDocStr) #2.7系 : unicode(oDocStr,'utf-8')
except Exception as er:
oDisp = ''
oDisp = str(traceback.format_exc()) + '\n' + str(er)
finally:
omsgbox(oDisp,1)
#!
#coding: utf-8
# python Marco
import uno
import sys
import traceback
from com.sun.star.awt import Rectangle
def omsgbox(oMessage='',oBtnType=1,oTitle='Title',oMsgType='messbox'):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), oMsgType, oBtnType, oTitle, oMessage)
return msgbox.execute()
# Class Toyota
class Toyota: # Classは不可 / class名の先頭文字は大文字
compact = 'Vitz'
maker = 'Toyota'
# Compact車とメーカーを取得する関数
def __init__(self, arg1,arg2):
self.shashu = arg1
self.maker = arg2
# Class Honda
class Honda: # Classは不可 / class名の先頭文字は大文字
shashu = 'Fit'
maker = 'Honda'
# 車種を取得する関数
def __init__(self, arg1,arg2):
self.shashu = arg1
self.maker = arg2
# Sub Class
class SubToyota(Toyota): # class名の先頭文字は大文字
rv = 'RAV-4'
def __init__(self,arg1):
self.rv = arg1
#
def oTest():
try:
oIsSubCls1 = issubclass(SubToyota,Toyota)
oIsSubCls2 = issubclass(SubToyota,Honda)
oDisp = u'[ 任意のclassのSub ClassかCheck ]\n\t' + u' 「SubToyota」は calss「Toyota」のSubclassか? ⇒ '\
+ str(oIsSubCls1) + '\n\n\t' + u' 「SubToyota」は calss「Honda」のSubclassか? ⇒ ' + str(oIsSubCls2)
except Exception as er:
oDisp = ''
oDisp = str(traceback.format_exc()) + '\n' + str(er)
finally:
omsgbox(oDisp,1)
#!
#coding: utf-8
# python Marco
import inspect
import uno
import sys
import traceback
from com.sun.star.awt import Rectangle
def omsgbox(oMessage='',oBtnType=1,oTitle='Title',oMsgType='messbox'):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), oMsgType, oBtnType, oTitle, oMessage)
return msgbox.execute()
# Class Toyota
class Toyota: # Classは不可
compact = 'Vitz'
maker = 'Toyota'
# Compact車とメーカーを取得する関数
def __init__(self, arg1,arg2):
self.shashu = arg1
self.maker = arg2
# Sub Class
class SubToyota(Toyota): # class名の先頭文字は大文字
rv = 'RAV-4'
def __init__(self,arg1):
self.rv = arg1
#
def oTest():
try:
oIsClass1 = inspect.isclass(Toyota)
oIsClass2 = inspect.isclass(SubToyota)
oIsClass3 = inspect.isclass(omsgbox)
oDisp = u'[ classのCheck ]\n\t' + 'Is Toypta class? : ' + str(oIsClass1) + '\n\t'\
+ 'Is SubToypta class? : ' + str(oIsClass2) + '\n\t' + 'Is omsgbox class? : ' + str(oIsClass3)
except Exception as er:
oDisp = ''
oDisp = str(traceback.format_exc()) + '\n' + str(er)
finally:
omsgbox(oDisp,1)
#!
#coding: utf-8
# python Marco
import uno
import sys
import traceback
from com.sun.star.awt import Rectangle
def omsgbox(oMessage='',oBtnType=1,oTitle='Title',oMsgType='messbox'):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), oMsgType, oBtnType, oTitle, oMessage)
return msgbox.execute()
# Class Toyota
class Toyota: # Classは不可 / class名の先頭文字は大文字
compact = 'Vitz'
maker = 'Toyota'
# Compact車とメーカーを取得する関数
def __init__(self, arg1,arg2):
self.compact = arg1
self.maker = arg2
# Class Honda
class Honda: # Classは不可 / class名の先頭文字は大文字
hybrid = 'Fit'
# 車種を取得する関数
def __init__(self, arg1):
self.hybrid = arg1
# Sub Class
class SubClass(Toyota,Honda): # 複数の基底classの定義 / class名の先頭文字は大文字
rv = 'RAV-4'
def __init__(self,arg1):
self.rv = arg1
#
def oTest():
try:
oCompact = SubClass.compact
oHybrid = SubClass.hybrid
oRV = SubClass.rv
oDisp = u'[ 多重継承 ]\n\t' + str(oCompact) + '\n\t' + str(oHybrid) + '\n\t' + str(oRV)
except Exception as er:
oDisp = ''
oDisp = str(traceback.format_exc()) + '\n' + str(er)
finally:
omsgbox(oDisp,1)
#!
#coding: utf-8
# python Marco
import uno
import sys
import traceback
from com.sun.star.awt import Rectangle
def omsgbox(oMessage='',oBtnType=1,oTitle='Title',oMsgType='messbox'):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), oMsgType, oBtnType, oTitle, oMessage)
return msgbox.execute()
# propety関数を使わない
class Cal_1(object): # class名の先頭文字は大文字
def __init__(self):
self.__x = None
def getx(self):
return self.__x
def setx(self, value):
self.__x = value
def delx(self):
del self.__x
# propety関数利用
class Cal_2(object): # class名の先頭文字は大文字
def __init__(self):
self.__x = None
def getx(self):
return self.__x
def setx(self, value):
self.__x = value
def delx(self):
del self.__x
x = property(getx,setx)
# propety関数と@decorate構文利用
def oProp(func):
return property(**func())
class Cal_3(object): # class名の先頭文字は大文字
def __init__(self):
self.__x = None
#
@oProp
def x():
def fget(self):
return self.__x
def fset(self, value):
self.__x = value
return locals()
#
def otest():
try:
# property関数不使用
oNo_Prop1 = Cal_1()
oNo_Prop1.setx(10)
oNoPropVal_1 = oNo_Prop1.getx()
oDisp = u'1) property関数不使用\n\t' + str(oNoPropVal_1) + '\n\n'
#
# property関数利用
oNo_Prop2 = Cal_2()
oNo_Prop2.x = 20
oNoPropVal_2 = oNo_Prop2.x
oDisp = oDisp + u'2) property関数利用\n\t' + str(oNoPropVal_2) + '\n\n'
#
# propety関数と@decorate構文利用
oNo_Prop3 = Cal_3()
oNo_Prop3.x = 30
oNoPropVal_3 = oNo_Prop3.x
oDisp = oDisp + u'3) propety関数と@decorate構文利用\n\t' + str(oNoPropVal_3)
except Exception as er:
oDisp = ''
oDisp = str(traceback.format_exc()) + '\n' + str(er)
finally:
omsgbox(oDisp,1)
Generator
#!
#coding: utf-8
# python Marco
import sys
import uno
import sys
import traceback
from com.sun.star.awt import Rectangle
def omsgbox(oMessage='',oBtnType=1,oTitle='Title',oMsgType='messbox'):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), oMsgType, oBtnType, oTitle, oMessage)
return msgbox.execute()
# Generator
def iterDef():
for i in range(5,8):
yield i*i
# 関数1
def oRtnDef1():
for j1 in range(5,8):
return j1*j1
# 関数2
def oRtnDef2():
oRtnList = []
for j2 in range(5,8):
oRtnList.append(j2*j2)
return oRtnList
#
def oTest():
try:
# Genrator利用(iterable取得)
oIter = iterDef()
oDisp = u'[ Generator ]\n Return\n ⇒\t' + str(oIter) + '\n\t = ' + str(tuple(oIter))
for k in oIter:
oDisp = oDisp + '\n\t\t' + str(k)
#
oDisp = oDisp + '\n\n' + u' ******************** \n'
#
# 関数利用1
oFunc1 = oRtnDef1()
oDisp = oDisp + u'[ 関数の利用1 ]\n Return : ' + str(oFunc1) + '\n\n'
# 以下のCodeはError
#for k in oFunc1:
# oDisp = oDisp + str(k) + '\n'
# 関数利用2(Listを取得)
oFunc2 = oRtnDef2()
oDisp = oDisp + u'[ 関数の利用2 ]\n Return : ' + str(oFunc2)
for k in oFunc2:
oDisp = oDisp + '\n\t\t' + str(k)
except Exception as er:
oDisp = ''
oDisp = str(traceback.format_exc()) + '\n' + str(er)
finally:
omsgbox(oDisp,1,'Generator')
[ Generator式 ]
#!
#coding: utf-8
# python Marco
import uno
import sys
import traceback
from com.sun.star.awt import Rectangle
def omsgbox(oMessage='',oBtnType=1,oTitle='Title',oMsgType='messbox'):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), oMsgType, oBtnType, oTitle, oMessage)
return msgbox.execute()
def oTest():
try:
oSumSqr = sum(i*i for i in range(10))
oDisp= u'[ 平方和 ]\n\t' + u'0^2 + 1^2 + 2^2 + ・・・ + 9^2\n\t = ' + str(oSumSqr)
except Exception as er:
oDisp = ''
oDisp = str(traceback.format_exc()) + '\n' + str(er)
finally:
omsgbox(oDisp,1,u'平方和')
#!
#coding: utf-8
# python Marco
import uno
import sys
import traceback
from com.sun.star.awt import Rectangle
def omsgbox(oMessage='',oBtnType=1,oTitle='Title',oMsgType='messbox'):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), oMsgType, oBtnType, oTitle, oMessage)
return msgbox.execute()
def oTest():
try:
oList1 = [10,20,30]
oList2 = [7,5,3]
oInnerProduct = sum(x*y for x,y in zip(oList1,oList2))
oDisp= u'[ 内積 ]\n\t 10*7 + 20*5 + 30*3\n\t = ' + str(oInnerProduct)
except Exception as er:
oDisp = ''
oDisp = str(traceback.format_exc()) + '\n' + str(er)
finally:
omsgbox(oDisp,1,u'内積')
Format
#!
#coding: utf-8
# python Marco
import uno
import sys
import traceback
from com.sun.star.awt import Rectangle
def omsgbox(oMessage='',oBtnType=1,oTitle='Title',oMsgType='messbox'):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), oMsgType, oBtnType, oTitle, oMessage)
return msgbox.execute()
def otest():
try:
oList1 = [1,2,3,4]
oList2 = ['apple','orange','melon','banana']
oZip = zip(oList1,oList2)
oDisp = ''
for v, w in oZip:
oDisp = oDisp + 'Item No.{0} is {1}'.format(v,w) + '\n'
except Exception as er:
oDisp = ''
oDisp = str(traceback.format_exc()) + '\n' + str(er)
finally:
omsgbox(oDisp,1)
#!
#coding: utf-8
# python Marco
import uno
import sys
import traceback
from com.sun.star.awt import Rectangle
def omsgbox(oMessage='',oBtnType=1,oTitle='Title',oMsgType='messbox'):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), oMsgType, oBtnType, oTitle, oMessage)
return msgbox.execute()
def otest():
try:
oDisp = 'This {food} is {fruit}.'.format(food='watermelon',fruit='vegetables')
except Exception as er:
oDisp = ''
oDisp = str(traceback.format_exc()) + '\n' + str(er)
finally:
omsgbox(oDisp,1)
#!
#coding: utf-8
# python Marco
import uno
import sys
import traceback
from com.sun.star.awt import Rectangle
import math
def omsgbox(oMessage='',oBtnType=1,oTitle='Title',oMsgType='messbox'):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), oMsgType, oBtnType, oTitle, oMessage)
return msgbox.execute()
def otest():
try:
oDisp = u'[ format Option(:) ]\n' + 'The value of PI is approximately {0:.3f}'.format(math.pi)
for x in range(1,11):
oDisp = oDisp + '\n\t\t' + '{0:2d} {1:3d} {2:4d}'.format(x, x*x, x*x*x)
except Exception as er:
oDisp = ''
oDisp = str(traceback.format_exc()) + '\n' + str(er)
finally:
omsgbox(oDisp,1)
#
# ':'の後ろに整数をつけると、そのFieldの最低文字数幅を指定出来る。
#!
#coding: utf-8
# python Marco
import uno
import sys
import traceback
from com.sun.star.awt import Rectangle
def omsgbox(oMessage='',oBtnType=1,oTitle='Title',oMsgType='messbox'):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), oMsgType, oBtnType, oTitle, oMessage)
return msgbox.execute()
def otest():
try:
oTable = {'Apache OpenOffice': 3.3, 'LibreOffice': 3.4, 'Lotus Symphony': 3.0}
oTDisp1 = ''
for app, ver in oTable.items():
oTDisp1 = oTDisp1 + '{0:10} ⇒ {1:0.1f}'.format(app,ver) + '\n'
oTDisp2 = 'LibreOffice: %(LibreOffice)f; Apache OpenOffice: %(Apache OpenOffice)f; Lotus Symphony: %(Lotus Symphony)f' % oTable
oTDisp3 = ('Lotus Symphony:{0[Lotus Symphony]:f};LibreOffice:{0[LibreOffice]:f};Apache OpenOffice:{0[Apache OpenOffice]:f}'.format(oTable))
oDisp = u'[ Dictionary型DataのFormat ]\n\n'
oDisp = oDisp + oTDisp1+ '\n' + oTDisp2 + '\n\n' + oTDisp3
except Exception as er:
oDisp = ''
oDisp = str(traceback.format_exc()) + '\n' + str(er)
finally:
omsgbox(oDisp,1)
#!
#coding: utf-8
# python Marco
import uno
import sys
import traceback
from com.sun.star.awt import Rectangle
def omsgbox(oMessage='',oBtnType=1,oTitle='Title',oMsgType='messbox'):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), oMsgType, oBtnType, oTitle, oMessage)
return msgbox.execute()
def otest():
try:
oDisp = u'[ 固定カラムで表示(rjust) ]\n' + '123456781234567812345678'
for x in range(11,21):
oDisp = oDisp + '\n' + repr(x).rjust(8) + repr(x*x).rjust(8) + repr(x*x*x).rjust(8)
except Exception as er:
oDisp = ''
oDisp = str(traceback.format_exc()) + '\n' + str(er)
finally:
omsgbox(oDisp,1)
#!
#coding: utf-8
# python Marco
import uno
import sys
import traceback
from com.sun.star.awt import Rectangle
def omsgbox(oMessage='',oBtnType=1,oTitle='Title',oMsgType='messbox'):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), oMsgType, oBtnType, oTitle, oMessage)
return msgbox.execute()
def otest():
try:
oDisp = u'[ 固定カラムで表示(center) ]\n' + '123456781234567812345678'
for x in range(11,21):
oDisp = oDisp + '\n' + repr(x).center(8) + repr(x*x).center(8) + repr(x*x*x).center(8)
except Exception as er:
oDisp = ''
oDisp = str(traceback.format_exc()) + '\n' + str(er)
finally:
omsgbox(oDisp,1)
#!
#coding: utf-8
# python Marco
import uno
import sys
import traceback
from com.sun.star.awt import Rectangle
def omsgbox(oMessage='',oBtnType=1,oTitle='Title',oMsgType='messbox'):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), oMsgType, oBtnType, oTitle, oMessage)
return msgbox.execute()
def otest():
try:
oDisp = u'[ 固定カラムで表示(ljust) ]\n' + '123456781234567812345678'
for x in range(11,21):
oDisp = oDisp + '\n' + repr(x).ljust(8) + repr(x*x).ljust(8) + repr(x*x*x).ljust(8)
except Exception as er:
oDisp = ''
oDisp = str(traceback.format_exc()) + '\n' + str(er)
finally:
omsgbox(oDisp,1)
#!
#coding: utf-8
# python Marco
import uno
import sys
import traceback
from com.sun.star.awt import Rectangle
def omsgbox(oMessage='',oBtnType=1,oTitle='Title',oMsgType='messbox'):
# """shows message."""
desktop = XSCRIPTCONTEXT.getDesktop()
frame = desktop.getCurrentFrame()
window = frame.getContainerWindow()
toolkit = window.getToolkit()
msgbox = toolkit.createMessageBox(window, Rectangle(), oMsgType, oBtnType, oTitle, oMessage)
return msgbox.execute()
def otest():
try:
oStrNum = '12'
oReprNum1 = u'-3.14'
oReprNum2 = u'3.12459265359'
oDisp = u'[ 数値文字列の左側を「0」で詰める ]\n\t' + oStrNum + u' ⇒ ' + oStrNum.zfill(10) + '\n\t'\
+ oReprNum1 + u' ⇒ ' + oReprNum1.zfill(10) + '\n\t' + oReprNum2 + u' ⇒ ' + oReprNum2.zfill(10)
except Exception as er:
oDisp = ''
oDisp = str(traceback.format_exc()) + '\n' + str(er)
finally:
omsgbox(oDisp,1)