Note
Go to the end to download the full example code.
ChemicalDrift - Transport and fate of organic compounds
from opendrift.readers import reader_netCDF_CF_generic
from opendrift.models.chemicaldrift import ChemicalDrift
from opendrift.readers.reader_constant import Reader as ConstantReader
from datetime import timedelta, datetime
import matplotlib.pyplot as plt
import numpy as np
o = ChemicalDrift(loglevel=0, seed=0)
# Norkyst
reader_norkyst = reader_netCDF_CF_generic.Reader('https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be')
mixed_layer = ConstantReader({'ocean_mixed_layer_thickness': 40})
o.add_reader([reader_norkyst,mixed_layer])
o.set_config('drift:vertical_mixing', True)
o.set_config('vertical_mixing:diffusivitymodel', 'windspeed_Large1994')
o.set_config('chemical:particle_diameter',30.e-6) # m
o.set_config('chemical:particle_diameter_uncertainty',5.e-6) # m
o.set_config('chemical:sediment:resuspension_critvel',0.15) # m/s
o.set_config('chemical:transformations:volatilization', True)
o.set_config('chemical:transformations:degradation', True)
o.set_config('chemical:transformations:degradation_mode', 'OverallRateConstants')
o.set_config('seed:LMM_fraction',.9)
o.set_config('seed:particle_fraction',.1)
o.set_config('general:coastline_action', 'previous')
o.init_chemical_compound("Phenanthrene")
# Modify half-life times with unrealistic values for this demo
o.set_config('chemical:transformations:t12_W_tot', 6.) # hours
o.set_config('chemical:transformations:t12_S_tot', 12.) # hours
o.list_configspec()
12:54:01 DEBUG opendrift.config:168: Adding 18 config items from __init__
12:54:01 DEBUG opendrift.config:178: Overwriting config item readers:max_number_of_fails
12:54:01 DEBUG opendrift.config:168: Adding 13 config items from __init__
12:54:01 INFO opendrift.models.basemodel:515: OpenDriftSimulation initialised (version 1.11.13 / v1.11.13-99-gd2132d3)
12:54:01 DEBUG opendrift.config:168: Adding 15 config items from oceandrift
12:54:01 DEBUG opendrift.config:178: Overwriting config item seed:z
12:54:01 DEBUG opendrift.config:168: Adding 71 config items from chemicaldrift
12:54:01 INFO opendrift.readers.reader_netCDF_CF_generic:102: Opening dataset: https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:02 DEBUG opendrift.readers.reader_netCDF_CF_generic:119: Finding coordinate variables.
12:54:02 DEBUG opendrift.readers.reader_netCDF_CF_generic:134: Parsing CF grid mapping dictionary: {'grid_mapping_name': 'polar_stereographic', 'straight_vertical_longitude_from_pole': 70.0, 'latitude_of_projection_origin': 90.0, 'standard_parallel': 60.0, 'false_easting': 3192800.0, 'false_northing': 1784000.0, 'semi_major_axis': 6378137.0, 'semi_minor_axis': 6356752.3142, 'proj4': '+proj=stere +lat_0=90 +lat_ts=60 +lon_0=70 +x_0=3192800 +y_0=1784000 +a=6378137 +b=6356752.3142 +units=m +no_defs +type=crs'}
12:54:02 INFO opendrift.readers.reader_netCDF_CF_generic:325: Detected dimensions: {'x': 'X', 'y': 'Y', 'z': 'depth', 'time': 'time'}
12:54:02 DEBUG opendrift.readers.reader_netCDF_CF_generic:361: Skipped variables without standard_name: ['angle', 'tke', 'ubar', 'vbar']
12:54:02 DEBUG opendrift.readers.basereader.variables:608: Setting buffer size 25 for reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be, assuming a maximum average speed of 5 m/s and time span of 1:00:00
12:54:02 DEBUG opendrift.readers.basereader:186: Variable mapping: ['sea_floor_depth_below_sea_level'] -> ['land_binary_mask'] is not activated
12:54:02 DEBUG opendrift.readers.basereader.variables:563: Adding variable mapping: ['x_wind', 'y_wind'] -> wind_speed
12:54:02 DEBUG opendrift.readers.basereader.variables:563: Adding variable mapping: ['x_sea_water_velocity', 'y_sea_water_velocity'] -> sea_water_speed
12:54:02 DEBUG opendrift.readers.basereader:186: Variable mapping: ['sea_floor_depth_below_sea_level'] -> ['land_binary_mask'] is not activated
12:54:02 DEBUG opendrift.models.basemodel.environment:328: Added reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:02 DEBUG opendrift.models.basemodel.environment:328: Added reader constant_reader
environment:constant:x_sea_water_velocity [None] float min: -15, max: 15 [m/s] Component of ocean c...
environment:fallback:x_sea_water_velocity [None] float min: -15, max: 15 [m/s] Component of ocean c...
environment:constant:y_sea_water_velocity [None] float min: -15, max: 15 [m/s] Component of ocean c...
environment:fallback:y_sea_water_velocity [None] float min: -15, max: 15 [m/s] Component of ocean c...
environment:constant:sea_surface_height [None] float min: None, max: None [None] Use constant value f...
environment:fallback:sea_surface_height [0] float min: None, max: None [None] Fallback value for s...
environment:constant:x_wind [None] float min: -50, max: 50 [m/s] Component of wind al...
environment:fallback:x_wind [0] float min: -50, max: 50 [m/s] Component of wind al...
environment:constant:y_wind [None] float min: -50, max: 50 [m/s] Component of wind al...
environment:fallback:y_wind [0] float min: -50, max: 50 [m/s] Component of wind al...
environment:constant:land_binary_mask [None] float min: 0, max: 1 [None] 1 is land, 0 is sea...
environment:fallback:land_binary_mask [None] float min: 0, max: 1 [None] 1 is land, 0 is sea...
environment:constant:sea_floor_depth_below_sea_level [None] float min: -20, max: 12000 [None] Depth of seafloor...
environment:fallback:sea_floor_depth_below_sea_level [10000] float min: -20, max: 12000 [None] Depth of seafloor...
environment:constant:ocean_vertical_diffusivity [None] float min: 0, max: 1 [None] Use constant value f...
environment:fallback:ocean_vertical_diffusivity [0.0001] float min: 0, max: 1 [None] Fallback value for o...
environment:constant:sea_water_temperature [None] float min: None, max: None [None] Use constant value f...
environment:fallback:sea_water_temperature [10] float min: None, max: None [None] Fallback value for s...
environment:constant:sea_water_salinity [None] float min: None, max: None [None] Use constant value f...
environment:fallback:sea_water_salinity [34] float min: None, max: None [None] Fallback value for s...
environment:constant:upward_sea_water_velocity [None] float min: None, max: None [None] Use constant value f...
environment:fallback:upward_sea_water_velocity [0] float min: None, max: None [None] Fallback value for u...
environment:constant:spm [None] float min: None, max: None [None] Use constant value f...
environment:fallback:spm [1] float min: None, max: None [None] Fallback value for s...
environment:constant:ocean_mixed_layer_thickness [None] float min: None, max: None [None] Use constant value f...
environment:fallback:ocean_mixed_layer_thickness [50] float min: None, max: None [None] Fallback value for o...
environment:constant:active_sediment_layer_thickness [None] float min: None, max: None [None] Use constant value f...
environment:fallback:active_sediment_layer_thickness [0.03] float min: None, max: None [None] Fallback value for a...
environment:constant:doc [None] float min: None, max: None [None] Use constant value f...
environment:fallback:doc [0.0] float min: None, max: None [None] Fallback value for d...
environment:constant:sea_water_ph_reported_on_total_scale [None] float min: None, max: None [None] Use constant value f...
environment:fallback:sea_water_ph_reported_on_total_scale [8.1] float min: None, max: None [None] Fallback value for s...
environment:constant:pH_sediment [None] float min: None, max: None [None] Use constant value f...
environment:fallback:pH_sediment [6.9] float min: None, max: None [None] Fallback value for p...
general:use_auto_landmask [True] bool A built-in GSHHG glo...
drift:current_uncertainty [0] float min: 0, max: 5 [m/s] Add gaussian perturb...
drift:current_uncertainty_uniform [0] float min: 0, max: 5 [m/s] Add gaussian perturb...
drift:max_speed [2.0] float min: 0, max: inf [seconds] Typical maximum spee...
readers:max_number_of_fails [1] int min: 0, max: 1000000.0 [number] Readers are discarde...
general:simulation_name [] str min length 0, max length 64 Name of simulation...
general:coastline_action [previous] enum ['none', 'stranding', 'previous'] None means that obje...
general:coastline_approximation_precision [0.001] float min: 0.0001, max: 0.005 [degrees] The precision of the...
general:time_step_minutes [60] float min: 0.01, max: 1440 [minutes] Calculation time ste...
general:time_step_output_minutes [None] float min: 1, max: 1440 [minutes] Output time step, i....
seed:ocean_only [True] bool If True, elements se...
seed:number [1] int min: 1, max: 100000000 [1] The number of elemen...
drift:max_age_seconds [None] float min: 0, max: inf [seconds] Elements will be dea...
drift:advection_scheme [euler] enum ['euler', 'runge-kutta', 'runge-kutta4'] Numerical advection ...
drift:horizontal_diffusivity [0] float min: 0, max: 100000 [m2/s] Add horizontal diffu...
drift:profiles_depth [50] float min: 0, max: None [meters] Environment profiles...
drift:wind_uncertainty [0] float min: 0, max: 5 [m/s] Add gaussian perturb...
drift:relative_wind [False] bool If True, wind drift ...
drift:deactivate_north_of [None] float min: -90, max: 90 [degrees] Elements are deactiv...
drift:deactivate_south_of [None] float min: -90, max: 90 [degrees] Elements are deactiv...
drift:deactivate_east_of [None] float min: -360, max: 360 [degrees] Elements are deactiv...
drift:deactivate_west_of [None] float min: -360, max: 360 [degrees] Elements are deactiv...
seed:origin_marker [0] float min: None, max: None [None] An integer kept cons...
seed:z [0] float min: -10000, max: 0 [m] Depth below sea leve...
seed:wind_drift_factor [0.02] float min: None, max: None [1] Elements at surface ...
seed:current_drift_factor [1] float min: None, max: None [1] Elements are moved w...
seed:terminal_velocity [0.0] float min: None, max: None [m/s] Terminal rise/sinkin...
seed:diameter [0.0] float min: None, max: None [m] Seeding value of dia...
seed:density [2650.0] float min: None, max: None [kg/m^3] Seeding value of den...
seed:specie [0] float min: None, max: None [] Seeding value of spe...
seed:mass [1000.0] float min: None, max: None [ug] Seeding value of mas...
seed:mass_degraded [0] float min: None, max: None [ug] Seeding value of mas...
seed:mass_degraded_water [0] float min: None, max: None [ug] Seeding value of mas...
seed:mass_degraded_sediment [0] float min: None, max: None [ug] Seeding value of mas...
seed:mass_volatilized [0] float min: None, max: None [ug] Seeding value of mas...
drift:vertical_advection [True] bool Advect elements with...
drift:vertical_mixing [True] bool Activate vertical mi...
vertical_mixing:timestep [60] float min: 0.1, max: 3600 [seconds] Time step used for i...
vertical_mixing:diffusivitymodel [windspeed_Large1994] enum ['environment', 'stepfunction', 'windspeed_Sundby1983', 'windspeed_Large1994', 'gls_tke', 'constant'] Algorithm/source use...
vertical_mixing:background_diffusivity [1.2e-05] float min: 0, max: 1 [m2s-1] Background diffusivi...
vertical_mixing:TSprofiles [False] bool Update T and S profi...
drift:wind_drift_depth [0.1] float min: 0, max: 10 [meters] The direct wind drif...
drift:stokes_drift [True] bool Advection elements w...
drift:stokes_drift_profile [Phillips] enum ['monochromatic', 'exponential', 'Phillips', 'windsea_swell'] Algorithm to calcula...
drift:use_tabularised_stokes_drift [False] bool If True, Stokes drif...
drift:tabularised_stokes_drift_fetch [25000] enum ['5000', '25000', '50000'] The fetch length whe...
general:seafloor_action [lift_to_seafloor] enum ['none', 'lift_to_seafloor', 'deactivate', 'previous'] "deactivate": elemen...
drift:truncate_ocean_model_below_m [None] float min: 0, max: 10000 [m] Ocean model data are...
seed:seafloor [False] bool Elements are seeded ...
chemical:transfer_setup [organics] enum ['Sandnesfj_Al', 'metals', '137Cs_rev', 'custom', 'organics'] ...
chemical:dynamic_partitioning [True] bool Toggle dynamic parti...
chemical:slowly_fraction [False] bool ...
chemical:irreversible_fraction [False] bool ...
chemical:dissolved_diameter [0] float min: 0, max: 0.0001 [m] ...
chemical:particle_diameter [3e-05] float min: 0, max: 0.0001 [m] ...
chemical:particle_concentration_half_depth [20] float min: 0, max: 100 [m] ...
chemical:doc_concentration_half_depth [1000] float min: 0, max: 1000 [m] ...
chemical:particle_diameter_uncertainty [5e-06] float min: 0, max: 0.0001 [m] ...
seed:LMM_fraction [0.9] float min: 0, max: 1 [] ...
seed:particle_fraction [0.1] float min: 0, max: 1 [] ...
chemical:species:LMM [True] bool Toggle LMM species...
chemical:species:LMMcation [False] bool ...
chemical:species:LMManion [False] bool ...
chemical:species:Colloid [False] bool ...
chemical:species:Humic_colloid [False] bool ...
chemical:species:Polymer [False] bool ...
chemical:species:Particle_reversible [True] bool ...
chemical:species:Particle_slowly_reversible [False] bool ...
chemical:species:Particle_irreversible [False] bool ...
chemical:species:Sediment_reversible [True] bool ...
chemical:species:Sediment_slowly_reversible [False] bool ...
chemical:species:Sediment_irreversible [False] bool ...
chemical:transformations:Kd [2.0] float min: 0, max: 1000000000.0 [m3/kg] ...
chemical:transformations:S0 [0.0] float min: 0, max: 100 [PSU] parameter controllin...
chemical:transformations:Dc [1.16e-05] float min: 0, max: 1000000.0 [] ...
chemical:transformations:slow_coeff [0] float min: 0, max: 1000000.0 [] ...
chemical:transformations:volatilization [True] bool Chemical is evaporat...
chemical:transformations:degradation [True] bool Chemical mass is deg...
chemical:transformations:degradation_mode [OverallRateConstants] enum ['OverallRateConstants'] ...
chemical:transformations:dissociation [nondiss] enum ['nondiss', 'acid', 'base', 'amphoter'] ...
chemical:transformations:LogKOW [4.505] float min: -3, max: 10 [Log L/Kg] ...
chemical:transformations:TrefKOW [25.0] float min: -3, max: 30 [C] ...
chemical:transformations:DeltaH_KOC_Sed [-24900.0] float min: -100000.0, max: 100000.0 [J/mol] ...
chemical:transformations:DeltaH_KOC_DOM [-25900.0] float min: -100000.0, max: 100000.0 [J/mol] ...
chemical:transformations:Setchenow [0.3026] float min: 0, max: 1 [L/mol] ...
chemical:transformations:pKa_acid [-1] float min: -1, max: 14 [] ...
chemical:transformations:pKa_base [-1] float min: -1, max: 14 [] ...
chemical:transformations:KOC_DOM [-1] float min: -1, max: 10000000000 [L/KgOC] ...
chemical:transformations:KOC_sed [-1] float min: -1, max: 10000000000 [L/KgOC] ...
chemical:transformations:fOC_SPM [0.05] float min: 0.01, max: 0.1 [gOC/g] ...
chemical:transformations:fOC_sed [0.05] float min: 0.01, max: 0.1 [gOC/g] ...
chemical:transformations:aggregation_rate [0] float min: 0, max: 1 [s-1] ...
chemical:transformations:t12_W_tot [6.0] float min: 1, max: None [hours] half life in water, ...
chemical:transformations:Tref_kWt [25.0] float min: -3, max: 30 [C] ...
chemical:transformations:DeltaH_kWt [50000.0] float min: -100000.0, max: 100000.0 [J/mol] ...
chemical:transformations:t12_S_tot [12.0] float min: 1, max: None [hours] half life in sedimen...
chemical:transformations:Tref_kSt [25.0] float min: -3, max: 30 [C] ...
chemical:transformations:DeltaH_kSt [50000.0] float min: -100000.0, max: 100000.0 [J/mol] ...
chemical:transformations:MolWt [178.226] float min: 50, max: 1000 [amu] molecular weight...
chemical:transformations:Henry [4.294e-05] float min: None, max: None [atm m3 mol-1] Henry constant...
chemical:transformations:Vpress [0.0222] float min: None, max: None [Pa] Vapour pressure...
chemical:transformations:Tref_Vpress [25.0] float min: None, max: None [C] Vapour pressure ref ...
chemical:transformations:DeltaH_Vpress [71733.0] float min: -100000.0, max: 115000.0 [J/mol] Enthalpy of volatili...
chemical:transformations:Solub [1.09] float min: None, max: None [g/m3] Solubility...
chemical:transformations:Tref_Solub [25.0] float min: None, max: None [C] Solubility ref temp...
chemical:transformations:DeltaH_Solub [34800.0] float min: -100000.0, max: 100000.0 [J/mol] Enthalpy of solubili...
chemical:sediment:mixing_depth [0.03] float min: 0, max: 100 [m] ...
chemical:sediment:density [2600] float min: 0, max: 10000 [kg/m3] ...
chemical:sediment:effective_fraction [0.9] float min: 0, max: 1 [] ...
chemical:sediment:corr_factor [0.1] float min: 0, max: 10 [] ...
chemical:sediment:porosity [0.6] float min: 0, max: 1 [] ...
chemical:sediment:layer_thickness [1] float min: 0, max: 100 [m] ...
chemical:sediment:desorption_depth [1] float min: 0, max: 100 [m] ...
chemical:sediment:desorption_depth_uncert [0.5] float min: 0, max: 100 [m] ...
chemical:sediment:resuspension_depth [1] float min: 0, max: 100 [m] ...
chemical:sediment:resuspension_depth_uncert [0.5] float min: 0, max: 100 [m] ...
chemical:sediment:resuspension_critvel [0.15] float min: 0, max: 1 [m/s] ...
chemical:sediment:burial_rate [3e-05] float min: 0, max: 10 [m/year] ...
chemical:sediment:buried_leaking_rate [0] float min: 0, max: 10 [s-1] ...
chemical:compound [Phenanthrene] enum ['Naphthalene', 'Phenanthrene', 'Fluoranthene', 'Benzo-a-anthracene', 'Benzo-a-pyrene', 'Dibenzo-ah-anthracene', 'C1-Naphthalene', 'Acenaphthene', 'Acenaphthylene', 'Fluorene', 'Dibenzothiophene', 'C2-Naphthalene', 'Anthracene', 'C3-Naphthalene', 'C1-Dibenzothiophene', 'Pyrene', 'C1-Phenanthrene', 'C2-Dibenzothiophene', 'C2-Phenanthrene', 'Benzo-b-fluoranthene', 'Chrysene', 'C3-Dibenzothiophene', 'C3-Phenanthrene', 'Benzo-k-fluoranthene', 'Benzo-ghi-perylene', 'Indeno-123cd-pyrene', 'Copper', 'Cadmium', 'Chromium', 'Lead', 'Vanadium', 'Zinc', 'Nickel', None] ...
Seeding 500 lagrangian elements each representign 2mg og target chemical
td=datetime.today()
time = td - timedelta(days=10)
latseed= 57.6; lonseed= 10.6 # Skagen
ntraj=500
iniz=np.random.rand(ntraj) * -10. # seeding the chemicals in the upper 10m
o.seed_elements(lonseed, latseed, z=iniz, radius=2000,number=ntraj,time=time, mass=2e3)
12:54:02 DEBUG opendrift.models.chemicaldrift:870: Partitioning coefficients (Tref,freshwater)
12:54:02 DEBUG opendrift.models.chemicaldrift:871: KOC_sed: 12953.922406542462 L/KgOC
12:54:02 DEBUG opendrift.models.chemicaldrift:872: KOC_SPM: 12953.922406542462 L/KgOC
12:54:02 DEBUG opendrift.models.chemicaldrift:873: KOC_DOM: 3004.29439651874 L/KgOC
12:54:02 DEBUG opendrift.models.chemicaldrift:885: Kd_sed: 647.6961203271231 L/Kg
12:54:02 DEBUG opendrift.models.chemicaldrift:886: Kd_SPM: 647.6961203271231 L/Kg
12:54:02 DEBUG opendrift.models.chemicaldrift:887: Kd_DOM: 1580.2588525688573 L/Kg
12:54:02 DEBUG opendrift.models.chemicaldrift:1104: nspecies: 5
12:54:02 DEBUG opendrift.models.chemicaldrift:1105: Transfer rates:
[[0.00000000e+00 1.75855513e-08 4.62500000e-07 2.88600000e-02
0.00000000e+00]
[4.06297347e-06 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00]
[9.91290450e-06 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00]
[9.91290450e-07 0.00000000e+00 0.00000000e+00 0.00000000e+00
3.16887646e-11]
[0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00]]
12:54:02 DEBUG opendrift.models.chemicaldrift:509: Initial partitioning:
12:54:02 DEBUG opendrift.models.chemicaldrift:511: 449 0 LMM
12:54:02 DEBUG opendrift.models.chemicaldrift:511: 0 1 Humic colloid
12:54:02 DEBUG opendrift.models.chemicaldrift:511: 51 2 Particle reversible
12:54:02 DEBUG opendrift.models.chemicaldrift:511: 0 3 Sediment reversible
12:54:02 DEBUG opendrift.models.chemicaldrift:511: 0 4 Sediment slowly reversible
12:54:02 INFO opendrift.models.basemodel.environment:218: Adding a dynamical landmask with max. priority based on assumed maximum speed of 2.0 m/s. Adding a customised landmask may be faster...
12:54:02 DEBUG opendrift.readers.basereader:186: Variable mapping: ['sea_floor_depth_below_sea_level'] -> ['land_binary_mask'] is not activated
12:54:07 DEBUG opendrift.models.basemodel.environment:328: Added reader global_landmask
12:54:07 INFO opendrift.models.basemodel.environment:245: Fallback values will be used for the following variables which have no readers:
12:54:07 INFO opendrift.models.basemodel.environment:248: ocean_vertical_diffusivity: 0.000100
12:54:07 INFO opendrift.models.basemodel.environment:248: spm: 1.000000
12:54:07 INFO opendrift.models.basemodel.environment:248: active_sediment_layer_thickness: 0.030000
12:54:07 INFO opendrift.models.basemodel.environment:248: doc: 0.000000
12:54:07 INFO opendrift.models.basemodel.environment:248: sea_water_ph_reported_on_total_scale: 8.100000
12:54:07 INFO opendrift.models.basemodel.environment:248: pH_sediment: 6.900000
12:54:07 DEBUG opendrift.models.basemodel:95: Changed mode from Mode.Config to Mode.Ready
Running model
o.run(steps=48*2, time_step=1800, time_step_output=1800)
12:54:07 DEBUG opendrift.models.basemodel:95: Changed mode from Mode.Ready to Mode.Run
12:54:07 DEBUG opendrift.models.basemodel:1778:
------------------------------------------------------
Software and hardware:
OpenDrift version 1.11.13
Platform: Linux, 5.15.0-1057-aws
68.56772994995117 GB memory
36 processors (x86_64)
NumPy version 1.26.4
SciPy version 1.14.1
Matplotlib version 3.9.1
NetCDF4 version 1.6.1
Xarray version 2024.10.0
ADIOS (adios_db) version 1.1.1
Copernicusmarine version 1.3.4
Python version 3.11.6 | packaged by conda-forge | (main, Oct 3 2023, 10:40:35) [GCC 12.3.0]
------------------------------------------------------
12:54:07 DEBUG opendrift.models.basemodel:1792: No output file is specified, neglecting export_buffer_length
12:54:07 DEBUG opendrift.models.basemodel:1910: Finalizing environment and preparing readers for simulation coverage ([4.69639997080836, 54.43270917325407, 16.516793922624256, 60.761642023034995]) and time (2024-11-01 12:54:02.760182 to 2024-11-03 12:54:02.760182)
12:54:07 DEBUG opendrift.models.basemodel.environment:180: Preparing https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be for extent [4.69639997080836, 54.43270917325407, 16.516793922624256, 60.761642023034995]
12:54:07 DEBUG opendrift.readers.basereader.structured:153: Clearing cache for reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be before starting new simulation
12:54:07 DEBUG opendrift.readers.basereader.variables:608: Setting buffer size 11 for reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be, assuming a maximum average speed of 2 m/s and time span of 1:00:00
12:54:07 DEBUG opendrift.readers.basereader.variables:549: Nothing more to prepare for https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:07 DEBUG opendrift.models.basemodel.environment:180: Preparing constant_reader for extent [4.69639997080836, 54.43270917325407, 16.516793922624256, 60.761642023034995]
12:54:07 DEBUG opendrift.readers.basereader.variables:549: Nothing more to prepare for constant_reader
12:54:07 DEBUG opendrift.models.basemodel.environment:180: Preparing global_landmask for extent [4.69639997080836, 54.43270917325407, 16.516793922624256, 60.761642023034995]
12:54:07 DEBUG opendrift.readers.basereader.variables:549: Nothing more to prepare for global_landmask
12:54:08 INFO opendrift.models.basemodel:936: Using existing reader for land_binary_mask
12:54:08 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:08 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:08 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:08 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:08 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:08 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:08 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:08 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:08 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:08 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:08 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:08 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:08 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:08 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:08 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:08 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:08 INFO opendrift.models.basemodel:947: All points are in ocean
12:54:08 INFO opendrift.models.chemicaldrift:352: Number of species: 5
12:54:08 INFO opendrift.models.chemicaldrift:354: 0 LMM
12:54:08 INFO opendrift.models.chemicaldrift:354: 1 Humic colloid
12:54:08 INFO opendrift.models.chemicaldrift:354: 2 Particle reversible
12:54:08 INFO opendrift.models.chemicaldrift:354: 3 Sediment reversible
12:54:08 INFO opendrift.models.chemicaldrift:354: 4 Sediment slowly reversible
12:54:08 INFO opendrift.models.chemicaldrift:357: transfer setup: organics
12:54:08 INFO opendrift.models.chemicaldrift:359: nspecies: 5
12:54:08 INFO opendrift.models.chemicaldrift:360: Transfer rates:
[[0.00000000e+00 1.75855513e-08 4.62500000e-07 2.88600000e-02
0.00000000e+00]
[4.06297347e-06 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00]
[9.91290450e-06 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00]
[9.91290450e-07 0.00000000e+00 0.00000000e+00 0.00000000e+00
3.16887646e-11]
[0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00]]
12:54:08 DEBUG opendrift.models.basemodel:891: to be seeded: 500, already seeded 0
12:54:08 DEBUG opendrift.models.basemodel:909: Released 500 new elements.
12:54:08 WARNING opendrift.models.basemodel:730: Seafloor check not being run because environment is missing. This will happen the first time the function is run but if it happens subsequently there is probably a problem.
12:54:08 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:08 INFO opendrift.models.basemodel:2038: 2024-11-01 12:54:02.760182 - step 1 of 96 - 500 active elements (0 deactivated)
12:54:08 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:08 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:08 DEBUG opendrift.models.basemodel:2057: 57.546223 <- latitude -> 57.64813
12:54:08 DEBUG opendrift.models.basemodel:2062: 10.507113 <- longitude -> 10.70608
12:54:08 DEBUG opendrift.models.basemodel:2067: -9.98847 <- z -> -0.046954762
12:54:08 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:08 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:08 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:08 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:08 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:08 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:08 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:08 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:08 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-01 12:00:00 (before)
2024-11-01 13:00:00 (after)
12:54:09 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
12:54:09 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:54:09 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:54:09 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:54:09 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:54:09 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'upward_sea_water_velocity', 'y_sea_water_velocity', 'sea_water_temperature', 'sea_water_salinity']
12:54:09 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 36x35x7) for time before (2024-11-01 12:00:00)
12:54:11 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
12:54:11 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:54:11 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:54:11 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:54:11 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:54:11 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'upward_sea_water_velocity', 'y_sea_water_velocity', 'sea_water_temperature', 'sea_water_salinity']
12:54:11 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 36x35x7) for time after (2024-11-01 13:00:00)
12:54:11 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-01 12:00:00) in space (linearNDFast)
12:54:11 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:11 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-01 13:00:00) in space (linearNDFast)
12:54:11 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:11 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-01 12:00:00, weight 0.10) and
after (2024-11-01 13:00:00, weight 0.90) in time
12:54:11 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:11 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.4929004930107 and -59.29392585710053 degrees.
12:54:11 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.4929004930107 and -59.29392585710053 degrees.
12:54:11 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:11 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:11 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:11 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:11 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:11 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:11 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:11 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:11 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:11 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:11 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:11 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:11 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:11 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:11 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:11 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:11 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:11 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:11 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:11 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:11 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:11 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:11 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:11 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:11 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:11 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:11 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:11 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:11 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:11 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.12365 (min) 0.150608 (max)
12:54:11 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0656712 (min) 0.121155 (max)
12:54:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.114427 (min) 0.159156 (max)
12:54:11 DEBUG opendrift.models.basemodel.environment:905: x_wind: 14.3416 (min) 15.1874 (max)
12:54:11 DEBUG opendrift.models.basemodel.environment:905: y_wind: -5.49693 (min) -4.63178 (max)
12:54:11 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:11 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.53077 (min) 23.882 (max)
12:54:11 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:11 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.7449 (min) 12.9606 (max)
12:54:11 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 31.8176 (min) 32.4191 (max)
12:54:11 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 6.57354e-06 (min) 0.000240899 (max)
12:54:11 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:11 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:11 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:11 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:11 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:11 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:11 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:11 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:11 DEBUG opendrift.models.basemodel:750: No elements hit seafloor.
12:54:11 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:11 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:11 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
/opt/conda/envs/opendrift/lib/python3.11/site-packages/numpy/core/fromnumeric.py:3504: RuntimeWarning: Mean of empty slice.
return _methods._mean(a, axis=axis, dtype=dtype,
/opt/conda/envs/opendrift/lib/python3.11/site-packages/numpy/core/_methods.py:129: RuntimeWarning: invalid value encountered in divide
ret = ret.dtype.type(ret / rcount)
12:54:11 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:11 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 1
12:54:11 DEBUG opendrift.models.chemicaldrift:1452: old species: [2]
12:54:11 DEBUG opendrift.models.chemicaldrift:1453: new species: [0]
12:54:11 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[0. 0. 0. 0. 0.]
[0. 0. 0. 0. 0.]
[1. 0. 0. 0. 0.]
[0. 0. 0. 0. 0.]
[0. 0. 0. 0. 0.]]
12:54:11 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:11 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:11 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:11 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:11 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.18527340756563998
12:54:11 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:11 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:11 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:11 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:11 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:54:11 DEBUG opendrift.models.oceandrift:618: 39 elements penetrated seafloor, lifting up
12:54:11 DEBUG opendrift.models.oceandrift:618: 54 elements penetrated seafloor, lifting up
12:54:11 DEBUG opendrift.models.oceandrift:618: 50 elements penetrated seafloor, lifting up
12:54:11 DEBUG opendrift.models.oceandrift:618: 54 elements penetrated seafloor, lifting up
12:54:11 DEBUG opendrift.models.oceandrift:618: 61 elements penetrated seafloor, lifting up
12:54:11 DEBUG opendrift.models.oceandrift:618: 61 elements penetrated seafloor, lifting up
12:54:11 DEBUG opendrift.models.oceandrift:618: 67 elements penetrated seafloor, lifting up
12:54:11 DEBUG opendrift.models.oceandrift:618: 59 elements penetrated seafloor, lifting up
12:54:11 DEBUG opendrift.models.oceandrift:618: 61 elements penetrated seafloor, lifting up
12:54:11 DEBUG opendrift.models.oceandrift:618: 60 elements penetrated seafloor, lifting up
12:54:11 DEBUG opendrift.models.oceandrift:618: 65 elements penetrated seafloor, lifting up
12:54:11 DEBUG opendrift.models.oceandrift:618: 61 elements penetrated seafloor, lifting up
12:54:11 DEBUG opendrift.models.oceandrift:618: 53 elements penetrated seafloor, lifting up
12:54:11 DEBUG opendrift.models.oceandrift:618: 64 elements penetrated seafloor, lifting up
12:54:11 DEBUG opendrift.models.oceandrift:618: 68 elements penetrated seafloor, lifting up
12:54:11 DEBUG opendrift.models.oceandrift:618: 63 elements penetrated seafloor, lifting up
12:54:11 DEBUG opendrift.models.oceandrift:618: 69 elements penetrated seafloor, lifting up
12:54:11 DEBUG opendrift.models.oceandrift:618: 74 elements penetrated seafloor, lifting up
12:54:11 DEBUG opendrift.models.oceandrift:618: 58 elements penetrated seafloor, lifting up
12:54:11 DEBUG opendrift.models.oceandrift:618: 68 elements penetrated seafloor, lifting up
12:54:11 DEBUG opendrift.models.oceandrift:618: 75 elements penetrated seafloor, lifting up
12:54:11 DEBUG opendrift.models.oceandrift:618: 78 elements penetrated seafloor, lifting up
12:54:11 DEBUG opendrift.models.oceandrift:618: 60 elements penetrated seafloor, lifting up
12:54:11 DEBUG opendrift.models.oceandrift:618: 73 elements penetrated seafloor, lifting up
12:54:11 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:11 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:11 DEBUG opendrift.models.oceandrift:618: 72 elements penetrated seafloor, lifting up
12:54:11 DEBUG opendrift.models.oceandrift:618: 71 elements penetrated seafloor, lifting up
12:54:11 DEBUG opendrift.models.oceandrift:618: 59 elements penetrated seafloor, lifting up
12:54:11 DEBUG opendrift.models.oceandrift:618: 57 elements penetrated seafloor, lifting up
12:54:11 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 0
12:54:11 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:11 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:11 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:11 INFO opendrift.models.chemicaldrift:1861: partitioning: [450, 0, 50, 0, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:11 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:11 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:11 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:11 DEBUG opendrift.models.basemodel:750: No elements hit seafloor.
12:54:11 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:11 INFO opendrift.models.basemodel:2038: 2024-11-01 13:24:02.760182 - step 2 of 96 - 500 active elements (0 deactivated)
12:54:11 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:11 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:11 DEBUG opendrift.models.basemodel:2057: 57.547929956434245 <- latitude -> 57.64786968233343
12:54:11 DEBUG opendrift.models.basemodel:2062: 10.50582543391829 <- longitude -> 10.708693910790979
12:54:11 DEBUG opendrift.models.basemodel:2067: -22.455628239942843 <- z -> -0.022925679715091185
12:54:11 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:11 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:11 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:11 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:11 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:11 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:11 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:11 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:11 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-01 13:00:00 (before)
2024-11-01 14:00:00 (after)
12:54:12 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
12:54:12 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:54:12 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:54:12 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:54:12 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:54:12 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'upward_sea_water_velocity', 'y_sea_water_velocity', 'sea_water_temperature', 'sea_water_salinity']
12:54:12 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 37x35x7) for time after (2024-11-01 14:00:00)
12:54:12 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-01 13:00:00) in space (linearNDFast)
12:54:12 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:12 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-01 14:00:00) in space (linearNDFast)
12:54:12 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:12 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-01 13:00:00, weight 0.60) and
after (2024-11-01 14:00:00, weight 0.40) in time
12:54:12 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:12 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.49418565848442 and -59.29131714489072 degrees.
12:54:12 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.49418565848442 and -59.29131714489072 degrees.
12:54:12 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:12 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:12 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:12 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:12 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:12 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:12 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:12 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:12 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:12 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:12 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:12 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:12 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:12 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:12 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:12 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:12 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:12 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:12 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:12 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:12 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:12 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:12 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.173886 (min) 0.132721 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0752946 (min) 0.144791 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.176738 (min) 0.212682 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: x_wind: 13.4599 (min) 14.3451 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: y_wind: -5.99001 (min) -5.05371 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.4461 (min) 24.0379 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.75 (min) 13.0651 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 31.8741 (min) 32.6609 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 3.41972e-06 (min) 0.000283812 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:12 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:12 DEBUG opendrift.models.basemodel:750: No elements hit seafloor.
12:54:12 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:12 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:12 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:12 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:12 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 30
12:54:12 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0]
12:54:12 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3]
12:54:12 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 30. 0.]
[ 0. 0. 0. 0. 0.]
[ 1. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:12 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:12 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:12 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:12 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:12 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.16999985686936311
12:54:12 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:12 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:12 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:12 DEBUG opendrift.models.oceandrift:618: 56 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 44 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 47 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 53 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 55 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 46 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 49 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 59 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 63 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 51 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 60 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 63 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 64 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 62 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 55 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 53 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 62 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 55 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 58 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 64 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 53 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 63 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 58 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:12 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:12 DEBUG opendrift.models.oceandrift:618: 49 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 68 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 66 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 61 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:12 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:12 DEBUG opendrift.models.oceandrift:618: 62 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 53 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 53 elements penetrated seafloor, lifting up
12:54:12 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 7
12:54:12 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:12 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 7 elements
12:54:12 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:12 INFO opendrift.models.chemicaldrift:1861: partitioning: [420, 0, 57, 23, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:12 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:12 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:12 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:12 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:12 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:12 INFO opendrift.models.basemodel:2038: 2024-11-01 13:54:02.760182 - step 3 of 96 - 500 active elements (0 deactivated)
12:54:12 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:12 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:12 DEBUG opendrift.models.basemodel:2057: 57.54951595623093 <- latitude -> 57.64793008518645
12:54:12 DEBUG opendrift.models.basemodel:2062: 10.505028270335549 <- longitude -> 10.708634933365154
12:54:12 DEBUG opendrift.models.basemodel:2067: -22.79195785522461 <- z -> 0.0
12:54:12 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:12 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:12 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:12 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:12 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-01 13:00:00 (before)
2024-11-01 14:00:00 (after)
12:54:12 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-01 13:00:00) in space (linearNDFast)
12:54:12 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:12 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-01 14:00:00) in space (linearNDFast)
12:54:12 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:12 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-01 13:00:00, weight 0.10) and
after (2024-11-01 14:00:00, weight 0.90) in time
12:54:12 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:12 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.4949828152958 and -59.29137612396542 degrees.
12:54:12 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.4949828152958 and -59.29137612396542 degrees.
12:54:12 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:12 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:12 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:12 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:12 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:12 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:12 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:12 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:12 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:12 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:12 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:12 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:12 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:12 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:12 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:12 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:12 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:12 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:12 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:12 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:12 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:12 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:12 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.168074 (min) 0.113882 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.110886 (min) 0.124073 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.235998 (min) 0.26408 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: x_wind: 12.5395 (min) 13.4379 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: y_wind: -6.20065 (min) -5.26212 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.40407 (min) 24.0921 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.7563 (min) 13.0721 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 31.8649 (min) 32.6881 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -5.94288e-06 (min) 0.000260328 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:12 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:12 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:12 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:12 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:12 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:12 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:12 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 29
12:54:12 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0]
12:54:12 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3]
12:54:12 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 59. 0.]
[ 0. 0. 0. 0. 0.]
[ 1. 0. 0. 0. 0.]
[ 0. 0. 7. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:12 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:12 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:12 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:12 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:12 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.15301006596946365
12:54:12 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:12 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:12 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:12 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 44 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 38 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 53 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 43 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 47 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 53 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 68 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 49 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 36 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 53 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 47 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 45 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 47 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 47 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 41 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 54 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 64 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 54 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
12:54:12 DEBUG opendrift.models.basemodel:755: Lifting 2 elements to seafloor.
12:54:12 DEBUG opendrift.models.oceandrift:618: 42 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 47 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 49 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 49 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 52 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 52 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 49 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 59 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 45 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 45 elements penetrated seafloor, lifting up
12:54:12 DEBUG opendrift.models.oceandrift:618: 49 elements penetrated seafloor, lifting up
12:54:12 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 4
12:54:12 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:12 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 4 elements
12:54:12 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:12 INFO opendrift.models.chemicaldrift:1861: partitioning: [391, 0, 61, 48, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:12 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:12 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:12 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:12 DEBUG opendrift.models.basemodel:750: No elements hit seafloor.
12:54:12 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:12 INFO opendrift.models.basemodel:2038: 2024-11-01 14:24:02.760182 - step 4 of 96 - 500 active elements (0 deactivated)
12:54:12 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:12 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:12 DEBUG opendrift.models.basemodel:2057: 57.55004846034986 <- latitude -> 57.647930085186445
12:54:12 DEBUG opendrift.models.basemodel:2062: 10.505028270335549 <- longitude -> 10.710270363373798
12:54:12 DEBUG opendrift.models.basemodel:2067: -22.79195785522461 <- z -> 0.0
12:54:12 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:12 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:12 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:12 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:12 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-01 14:00:00 (before)
2024-11-01 15:00:00 (after)
12:54:14 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
12:54:14 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:54:14 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:54:14 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:54:14 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:54:14 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'upward_sea_water_velocity', 'y_sea_water_velocity', 'sea_water_temperature', 'sea_water_salinity']
12:54:14 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 37x35x7) for time after (2024-11-01 15:00:00)
12:54:14 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-01 14:00:00) in space (linearNDFast)
12:54:14 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:14 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-01 15:00:00) in space (linearNDFast)
12:54:14 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:14 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-01 14:00:00, weight 0.60) and
after (2024-11-01 15:00:00, weight 0.40) in time
12:54:14 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:14 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.4949828152958 and -59.289740707420705 degrees.
12:54:14 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.4949828152958 and -59.289740707420705 degrees.
12:54:14 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:14 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:14 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:14 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:14 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:14 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:14 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:14 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:14 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:14 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:14 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:14 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:14 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:14 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:14 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:14 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:14 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:14 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:14 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:14 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:14 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:14 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:14 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:14 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:14 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:14 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:14 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:14 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:14 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:14 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.161571 (min) 0.104376 (max)
12:54:14 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0804268 (min) 0.123092 (max)
12:54:14 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.259126 (min) 0.286671 (max)
12:54:14 DEBUG opendrift.models.basemodel.environment:905: x_wind: 11.9061 (min) 12.7544 (max)
12:54:14 DEBUG opendrift.models.basemodel.environment:905: y_wind: -6.40628 (min) -5.5695 (max)
12:54:14 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:14 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.40407 (min) 24.2527 (max)
12:54:14 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:14 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.7437 (min) 13.0867 (max)
12:54:14 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 31.9162 (min) 32.7453 (max)
12:54:14 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -4.86154e-06 (min) 0.000232112 (max)
12:54:14 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:14 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:14 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:14 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:14 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:14 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:14 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:14 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:14 DEBUG opendrift.models.basemodel:750: No elements hit seafloor.
12:54:14 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:14 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:14 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:14 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:14 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 18
12:54:14 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0]
12:54:14 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3]
12:54:14 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 77. 0.]
[ 0. 0. 0. 0. 0.]
[ 1. 0. 0. 0. 0.]
[ 0. 0. 11. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:14 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:14 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:14 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:14 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:14 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.14395012076967684
12:54:14 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:14 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:14 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:14 DEBUG opendrift.models.oceandrift:618: 35 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 37 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 33 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 37 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 43 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 41 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 42 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 39 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 39 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:14 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:14 DEBUG opendrift.models.oceandrift:618: 52 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 48 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 58 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 43 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 50 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 46 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 38 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 54 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 51 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 38 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 40 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:14 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:14 DEBUG opendrift.models.oceandrift:618: 44 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 47 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 51 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 50 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 38 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 49 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 58 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 50 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 40 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 50 elements penetrated seafloor, lifting up
12:54:14 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 1
12:54:14 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:14 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 1 elements
12:54:14 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:14 INFO opendrift.models.chemicaldrift:1861: partitioning: [373, 0, 62, 65, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:14 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:14 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:14 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:14 DEBUG opendrift.models.basemodel:750: No elements hit seafloor.
12:54:14 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:14 INFO opendrift.models.basemodel:2038: 2024-11-01 14:54:02.760182 - step 5 of 96 - 500 active elements (0 deactivated)
12:54:14 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:14 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:14 DEBUG opendrift.models.basemodel:2057: 57.55174739367954 <- latitude -> 57.64821016372712
12:54:14 DEBUG opendrift.models.basemodel:2062: 10.505028270335549 <- longitude -> 10.709938555842978
12:54:14 DEBUG opendrift.models.basemodel:2067: -22.87772389022365 <- z -> 0.0
12:54:14 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:14 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:14 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:14 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:14 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:14 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:14 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:14 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:14 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-01 14:00:00 (before)
2024-11-01 15:00:00 (after)
12:54:14 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-01 14:00:00) in space (linearNDFast)
12:54:14 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:14 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-01 15:00:00) in space (linearNDFast)
12:54:14 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:14 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-01 14:00:00, weight 0.10) and
after (2024-11-01 15:00:00, weight 0.90) in time
12:54:14 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:14 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.4949828196721 and -59.29007250869632 degrees.
12:54:14 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.4949828196721 and -59.29007250869632 degrees.
12:54:14 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:14 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:14 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:14 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:14 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:14 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:14 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:14 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:14 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:14 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:14 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:14 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:14 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:14 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:14 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:14 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:14 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:14 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:14 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:14 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:14 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:14 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:14 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:14 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:14 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:14 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:14 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:14 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:14 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:14 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.130023 (min) 0.101501 (max)
12:54:14 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0744176 (min) 0.127927 (max)
12:54:14 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.270647 (min) 0.303203 (max)
12:54:14 DEBUG opendrift.models.basemodel.environment:905: x_wind: 11.1793 (min) 12.1991 (max)
12:54:14 DEBUG opendrift.models.basemodel.environment:905: y_wind: -6.61224 (min) -5.87574 (max)
12:54:14 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:14 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.40407 (min) 24.307 (max)
12:54:14 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:14 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.7292 (min) 13.092 (max)
12:54:14 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 31.9013 (min) 32.7647 (max)
12:54:14 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -5.961e-06 (min) 0.000217991 (max)
12:54:14 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:14 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:14 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:14 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:14 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:14 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:14 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:14 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:14 DEBUG opendrift.models.basemodel:750: No elements hit seafloor.
12:54:14 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:14 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:14 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:14 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:14 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 21
12:54:14 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0]
12:54:14 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3]
12:54:14 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 98. 0.]
[ 0. 0. 0. 0. 0.]
[ 1. 0. 0. 0. 0.]
[ 0. 0. 12. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:14 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:14 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:14 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:14 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:14 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.1385398920983909
12:54:14 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:14 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:14 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:14 DEBUG opendrift.models.oceandrift:618: 34 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 32 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:14 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:14 DEBUG opendrift.models.oceandrift:618: 39 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 37 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 34 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 33 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 43 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 50 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 33 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 48 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 36 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 49 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 39 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 45 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 42 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 45 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 35 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 39 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 50 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 50 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 34 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 49 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 48 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 43 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 37 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 41 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:14 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:14 DEBUG opendrift.models.oceandrift:618: 37 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 35 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:618: 43 elements penetrated seafloor, lifting up
12:54:14 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:14 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:14 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 3
12:54:14 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:14 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 3 elements
12:54:14 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:14 INFO opendrift.models.chemicaldrift:1861: partitioning: [352, 0, 65, 83, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:14 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:14 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:14 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:14 DEBUG opendrift.models.basemodel:750: No elements hit seafloor.
12:54:14 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:14 INFO opendrift.models.basemodel:2038: 2024-11-01 15:24:02.760182 - step 6 of 96 - 500 active elements (0 deactivated)
12:54:14 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:14 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:14 DEBUG opendrift.models.basemodel:2057: 57.55205180645491 <- latitude -> 57.64872024207769
12:54:14 DEBUG opendrift.models.basemodel:2062: 10.505028270335552 <- longitude -> 10.709756056462146
12:54:14 DEBUG opendrift.models.basemodel:2067: -23.094985961914062 <- z -> 0.0
12:54:14 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:14 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:14 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:14 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:14 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:14 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:14 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:14 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:14 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-01 15:00:00 (before)
2024-11-01 16:00:00 (after)
12:54:15 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
12:54:15 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:54:15 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:54:15 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:54:15 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:54:15 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'upward_sea_water_velocity', 'y_sea_water_velocity', 'sea_water_temperature', 'sea_water_salinity']
12:54:15 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 37x35x7) for time after (2024-11-01 16:00:00)
12:54:15 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-01 15:00:00) in space (linearNDFast)
12:54:15 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:15 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-01 16:00:00) in space (linearNDFast)
12:54:15 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:15 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-01 15:00:00, weight 0.60) and
after (2024-11-01 16:00:00, weight 0.40) in time
12:54:15 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:15 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.4949828196721 and -59.29025499778395 degrees.
12:54:15 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.4949828196721 and -59.29025499778395 degrees.
12:54:15 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:15 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:15 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:15 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:15 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:15 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:15 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:15 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:15 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:15 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:15 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:15 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:15 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:15 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:15 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:15 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:15 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:15 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:15 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:15 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:15 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:15 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:15 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:15 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:15 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:15 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:15 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:15 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:15 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:15 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.125305 (min) 0.116429 (max)
12:54:15 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0864417 (min) 0.144694 (max)
12:54:15 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.253494 (min) 0.290105 (max)
12:54:15 DEBUG opendrift.models.basemodel.environment:905: x_wind: 10.0934 (min) 11.2559 (max)
12:54:15 DEBUG opendrift.models.basemodel.environment:905: y_wind: -7.03425 (min) -6.17506 (max)
12:54:15 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:15 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.40407 (min) 24.3588 (max)
12:54:15 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:15 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.7156 (min) 13.0841 (max)
12:54:15 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 31.9123 (min) 32.7477 (max)
12:54:15 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -1.50954e-05 (min) 0.000208513 (max)
12:54:15 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:15 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:15 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:15 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:15 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:15 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:15 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:15 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:15 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:15 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:15 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:15 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:15 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:15 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 35
12:54:15 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 3 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0]
12:54:15 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 0 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3]
12:54:15 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 132. 0.]
[ 0. 0. 0. 0. 0.]
[ 1. 0. 0. 0. 0.]
[ 1. 0. 15. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:15 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:15 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:15 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:15 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:15 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.1262241729585807
12:54:15 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:15 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:15 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:15 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:618: 34 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:618: 40 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:618: 41 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:618: 43 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:618: 36 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:15 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:15 DEBUG opendrift.models.oceandrift:618: 38 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:15 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:15 DEBUG opendrift.models.oceandrift:618: 50 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:618: 38 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:618: 35 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:618: 38 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:618: 35 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:15 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:15 DEBUG opendrift.models.oceandrift:618: 35 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:618: 45 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:618: 43 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:618: 40 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:15 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:15 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:618: 34 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:618: 38 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:618: 34 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:15 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:15 DEBUG opendrift.models.oceandrift:618: 44 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:618: 39 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:618: 38 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:618: 43 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:15 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:15 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 2
12:54:15 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:15 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 2 elements
12:54:15 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:15 INFO opendrift.models.chemicaldrift:1861: partitioning: [319, 0, 67, 114, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:15 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:15 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:15 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:15 DEBUG opendrift.models.basemodel:750: No elements hit seafloor.
12:54:15 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:15 INFO opendrift.models.basemodel:2038: 2024-11-01 15:54:02.760182 - step 7 of 96 - 500 active elements (0 deactivated)
12:54:15 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:15 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:15 DEBUG opendrift.models.basemodel:2057: 57.55232241142234 <- latitude -> 57.64948808495636
12:54:15 DEBUG opendrift.models.basemodel:2062: 10.505028270335552 <- longitude -> 10.711119951948477
12:54:15 DEBUG opendrift.models.basemodel:2067: -23.223823537817225 <- z -> 0.0
12:54:15 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:15 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:15 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:15 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:15 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:15 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:15 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:15 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:15 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-01 15:00:00 (before)
2024-11-01 16:00:00 (after)
12:54:15 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-01 15:00:00) in space (linearNDFast)
12:54:15 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:15 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-01 16:00:00) in space (linearNDFast)
12:54:15 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:15 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-01 15:00:00, weight 0.10) and
after (2024-11-01 16:00:00, weight 0.90) in time
12:54:15 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:15 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.49498281571725 and -59.28889110048986 degrees.
12:54:15 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.49498281571725 and -59.28889110048986 degrees.
12:54:15 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:15 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:15 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:15 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:15 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:15 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:15 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:15 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:15 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:15 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:15 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:15 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:15 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:15 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:15 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:15 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:15 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:15 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:15 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:15 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:15 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:15 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:15 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:15 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:15 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:15 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:15 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:15 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:15 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:15 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.104853 (min) 0.105088 (max)
12:54:15 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0199963 (min) 0.155159 (max)
12:54:15 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.229491 (min) 0.26972 (max)
12:54:15 DEBUG opendrift.models.basemodel.environment:905: x_wind: 8.92205 (min) 10.1937 (max)
12:54:15 DEBUG opendrift.models.basemodel.environment:905: y_wind: -7.50784 (min) -6.43651 (max)
12:54:15 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:15 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.40407 (min) 24.4855 (max)
12:54:15 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:15 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.6691 (min) 13.0725 (max)
12:54:15 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 31.9253 (min) 32.7232 (max)
12:54:15 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -2.64977e-05 (min) 0.00023858 (max)
12:54:15 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:15 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:15 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:15 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:15 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:15 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:15 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:15 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:15 DEBUG opendrift.models.basemodel:750: No elements hit seafloor.
12:54:15 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:15 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:15 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:15 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:15 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 25
12:54:15 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 2 0 0]
12:54:15 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 0 3 3]
12:54:15 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 156. 0.]
[ 0. 0. 0. 0. 0.]
[ 2. 0. 0. 0. 0.]
[ 1. 0. 17. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:15 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:15 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:15 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:15 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:15 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.11373438107296943
12:54:15 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:15 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:15 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:15 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:618: 34 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:618: 38 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:618: 37 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:618: 37 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:15 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:15 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:15 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:15 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:618: 33 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:618: 32 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:15 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:15 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:618: 34 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:618: 37 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:618: 35 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:618: 35 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:618: 37 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:618: 38 elements penetrated seafloor, lifting up
12:54:15 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:54:15 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 9
12:54:15 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:15 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 9 elements
12:54:15 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:15 INFO opendrift.models.chemicaldrift:1861: partitioning: [296, 0, 75, 129, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:15 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:15 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:15 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:15 DEBUG opendrift.models.basemodel:750: No elements hit seafloor.
12:54:15 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:15 INFO opendrift.models.basemodel:2038: 2024-11-01 16:24:02.760182 - step 8 of 96 - 500 active elements (0 deactivated)
12:54:15 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:15 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:15 DEBUG opendrift.models.basemodel:2057: 57.55199921050319 <- latitude -> 57.65004256957842
12:54:15 DEBUG opendrift.models.basemodel:2062: 10.505028270335552 <- longitude -> 10.710244659050545
12:54:15 DEBUG opendrift.models.basemodel:2067: -23.173423767089844 <- z -> 0.0
12:54:15 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:15 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:15 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:15 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:15 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:15 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:15 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:15 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:15 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-01 16:00:00 (before)
2024-11-01 17:00:00 (after)
12:54:17 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
12:54:17 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:54:17 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:54:17 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:54:17 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:54:17 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'upward_sea_water_velocity', 'y_sea_water_velocity', 'sea_water_temperature', 'sea_water_salinity']
12:54:17 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 37x35x7) for time after (2024-11-01 17:00:00)
12:54:17 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-01 16:00:00) in space (linearNDFast)
12:54:17 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:17 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-01 17:00:00) in space (linearNDFast)
12:54:17 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:17 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-01 16:00:00, weight 0.60) and
after (2024-11-01 17:00:00, weight 0.40) in time
12:54:17 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:17 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.49498281571725 and -59.28976640715407 degrees.
12:54:17 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.49498281571725 and -59.28976640715407 degrees.
12:54:17 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:17 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:17 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:17 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:17 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:17 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:17 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:17 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:17 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:17 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:17 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:17 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:17 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:17 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:17 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:17 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:17 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:17 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:17 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:17 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:17 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:17 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:17 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:17 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:17 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:17 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:17 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:17 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:17 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:17 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0851349 (min) 0.119632 (max)
12:54:17 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0274444 (min) 0.178876 (max)
12:54:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.179976 (min) 0.221986 (max)
12:54:17 DEBUG opendrift.models.basemodel.environment:905: x_wind: 7.78163 (min) 9.22624 (max)
12:54:17 DEBUG opendrift.models.basemodel.environment:905: y_wind: -8.45104 (min) -6.70366 (max)
12:54:17 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:17 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.40407 (min) 24.4615 (max)
12:54:17 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:17 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.6912 (min) 13.083 (max)
12:54:17 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 31.9847 (min) 32.752 (max)
12:54:17 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -3.85995e-05 (min) 0.000214248 (max)
12:54:17 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:17 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:17 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:17 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:17 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:17 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:17 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:17 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:17 DEBUG opendrift.models.basemodel:755: Lifting 2 elements to seafloor.
12:54:17 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:17 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:17 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:17 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:17 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 20
12:54:17 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 0 0 0 0 0 2 0 0 0 0 0 0 0 0 0 0 0]
12:54:17 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 3 3 3 3 3 0 3 3 3 3 3 3 3 3 3 3 3]
12:54:17 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 175. 0.]
[ 0. 0. 0. 0. 0.]
[ 3. 0. 0. 0. 0.]
[ 1. 0. 26. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:17 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:17 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:17 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:17 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:17 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.10321462693185099
12:54:17 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:17 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:17 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:17 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
12:54:17 DEBUG opendrift.models.basemodel:755: Lifting 2 elements to seafloor.
12:54:17 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:618: 38 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:618: 35 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:17 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:17 DEBUG opendrift.models.oceandrift:618: 36 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:17 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:17 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:618: 33 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:618: 33 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:618: 33 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:618: 32 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:618: 33 elements penetrated seafloor, lifting up
12:54:17 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 31
12:54:17 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:17 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 31 elements
12:54:17 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:17 INFO opendrift.models.chemicaldrift:1861: partitioning: [278, 0, 105, 117, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:17 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:17 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:17 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:17 DEBUG opendrift.models.basemodel:750: No elements hit seafloor.
12:54:17 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:17 INFO opendrift.models.basemodel:2038: 2024-11-01 16:54:02.760182 - step 9 of 96 - 500 active elements (0 deactivated)
12:54:17 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:17 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:17 DEBUG opendrift.models.basemodel:2057: 57.551931563229914 <- latitude -> 57.65168007238088
12:54:17 DEBUG opendrift.models.basemodel:2062: 10.505028270335552 <- longitude -> 10.7109340707622
12:54:17 DEBUG opendrift.models.basemodel:2067: -23.320623147696082 <- z -> 0.0
12:54:17 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:17 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:17 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:17 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:17 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:17 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:17 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:17 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:17 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-01 16:00:00 (before)
2024-11-01 17:00:00 (after)
12:54:17 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-01 16:00:00) in space (linearNDFast)
12:54:17 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:17 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-01 17:00:00) in space (linearNDFast)
12:54:17 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:17 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-01 16:00:00, weight 0.10) and
after (2024-11-01 17:00:00, weight 0.90) in time
12:54:17 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:17 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.49498281571725 and -59.28907699497381 degrees.
12:54:17 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.49498281571725 and -59.28907699497381 degrees.
12:54:17 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:17 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:17 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:17 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:17 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:17 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:17 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:17 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:17 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:17 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:17 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:17 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:17 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:17 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:17 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:17 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:17 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:17 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:17 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:17 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:17 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:17 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:17 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:17 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:17 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:17 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:17 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:17 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:17 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:17 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0781913 (min) 0.115832 (max)
12:54:17 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.00360124 (min) 0.204258 (max)
12:54:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.123261 (min) 0.166958 (max)
12:54:17 DEBUG opendrift.models.basemodel.environment:905: x_wind: 6.19139 (min) 8.67864 (max)
12:54:17 DEBUG opendrift.models.basemodel.environment:905: y_wind: -9.5183 (min) -6.97222 (max)
12:54:17 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:17 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.40407 (min) 24.5128 (max)
12:54:17 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:17 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.6708 (min) 13.0775 (max)
12:54:17 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 31.975 (min) 32.7366 (max)
12:54:17 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -5.08746e-05 (min) 0.000298963 (max)
12:54:17 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:17 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:17 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:17 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:17 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:17 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:17 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:17 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:17 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:17 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:17 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:17 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:17 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:17 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 21
12:54:17 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 0 0 0 0 2 0 0 0 0 0 0 0 0 0 0 0 0 0]
12:54:17 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 3 3 3 3 0 3 3 3 3 3 3 3 3 3 3 3 3 3]
12:54:17 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 195. 0.]
[ 0. 0. 0. 0. 0.]
[ 4. 0. 0. 0. 0.]
[ 1. 0. 57. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:17 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:17 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:17 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:17 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:17 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.0983616033334628
12:54:17 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:17 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:17 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:17 DEBUG opendrift.models.oceandrift:618: 34 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:618: 38 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:618: 32 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:17 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:17 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:618: 42 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:618: 35 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:17 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:17 DEBUG opendrift.models.oceandrift:618: 39 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:618: 32 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:17 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:17 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:618: 39 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:618: 38 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:618: 40 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:618: 36 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:618: 32 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:17 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:17 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:54:17 DEBUG opendrift.models.oceandrift:618: 33 elements penetrated seafloor, lifting up
12:54:17 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 50
12:54:17 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:17 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 50 elements
12:54:17 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:17 INFO opendrift.models.chemicaldrift:1861: partitioning: [259, 0, 154, 87, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:17 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:17 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:17 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:17 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:17 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:17 INFO opendrift.models.basemodel:2038: 2024-11-01 17:24:02.760182 - step 10 of 96 - 500 active elements (0 deactivated)
12:54:17 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:17 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:17 DEBUG opendrift.models.basemodel:2057: 57.55198976177515 <- latitude -> 57.65397750344318
12:54:17 DEBUG opendrift.models.basemodel:2062: 10.505028270335549 <- longitude -> 10.709850928462586
12:54:17 DEBUG opendrift.models.basemodel:2067: -23.173423767089844 <- z -> -0.02407885974394297
12:54:17 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:17 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:17 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:17 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:17 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:17 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:17 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:17 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:17 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-01 17:00:00 (before)
2024-11-01 18:00:00 (after)
12:54:18 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
12:54:18 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:54:18 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:54:18 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:54:18 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:54:18 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'upward_sea_water_velocity', 'y_sea_water_velocity', 'sea_water_temperature', 'sea_water_salinity']
12:54:18 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 39x35x7) for time after (2024-11-01 18:00:00)
12:54:18 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-01 17:00:00) in space (linearNDFast)
12:54:18 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:18 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-01 18:00:00) in space (linearNDFast)
12:54:18 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:18 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-01 17:00:00, weight 0.60) and
after (2024-11-01 18:00:00, weight 0.40) in time
12:54:18 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:18 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.49498281571725 and -59.29016014373088 degrees.
12:54:18 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.49498281571725 and -59.29016014373088 degrees.
12:54:18 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:18 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:18 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:18 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:18 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:18 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:18 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:18 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:18 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:18 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:18 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:18 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:18 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:18 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:18 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:18 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:18 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:18 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:18 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:18 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:18 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:18 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:18 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:18 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:18 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:18 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:18 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:18 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:18 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:18 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.083585 (min) 0.124834 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0194436 (min) 0.222218 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.0599932 (min) 0.104189 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: x_wind: 6.29392 (min) 8.21172 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: y_wind: -10.1534 (min) -7.73899 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.40407 (min) 24.4166 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.6672 (min) 13.0683 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0063 (min) 32.7142 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -5.41962e-05 (min) 0.000307902 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:18 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:18 DEBUG opendrift.models.basemodel:755: Lifting 2 elements to seafloor.
12:54:18 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:18 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:18 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:18 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:18 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 21
12:54:18 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 0 0 0 0 0 0 0 0 2 0 2 0 0 0 2 0 0 0]
12:54:18 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 3 3 3 3 3 3 3 3 0 3 0 3 3 3 0 3 3 3]
12:54:18 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 213. 0.]
[ 0. 0. 0. 0. 0.]
[ 7. 0. 0. 0. 0.]
[ 1. 0. 107. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:18 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:18 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:18 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:18 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:18 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.10621801551407149
12:54:18 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:18 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:18 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:18 DEBUG opendrift.models.oceandrift:618: 45 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:18 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:18 DEBUG opendrift.models.oceandrift:618: 34 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:618: 39 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:618: 35 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:618: 38 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:618: 36 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:618: 36 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:18 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:18 DEBUG opendrift.models.oceandrift:618: 35 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:618: 34 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:18 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:18 DEBUG opendrift.models.oceandrift:618: 32 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:618: 36 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:618: 43 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:18 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:18 DEBUG opendrift.models.oceandrift:618: 39 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:618: 32 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:618: 46 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:618: 34 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:18 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:18 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:618: 41 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:18 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:18 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:618: 38 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:618: 35 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:618: 43 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:618: 38 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:618: 39 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:18 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:18 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 47
12:54:18 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:18 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 45 elements
12:54:18 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:18 INFO opendrift.models.chemicaldrift:1861: partitioning: [244, 0, 196, 60, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:18 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:18 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:18 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:18 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:18 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:18 INFO opendrift.models.basemodel:2038: 2024-11-01 17:54:02.760182 - step 11 of 96 - 500 active elements (0 deactivated)
12:54:18 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:18 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:18 DEBUG opendrift.models.basemodel:2057: 57.55230400927336 <- latitude -> 57.65626497496362
12:54:18 DEBUG opendrift.models.basemodel:2062: 10.505028270335545 <- longitude -> 10.708856954954257
12:54:18 DEBUG opendrift.models.basemodel:2067: -23.173423767089844 <- z -> -0.041416398182518854
12:54:18 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:18 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:18 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:18 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:18 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:18 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:18 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:18 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:18 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-01 17:00:00 (before)
2024-11-01 18:00:00 (after)
12:54:18 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-01 17:00:00) in space (linearNDFast)
12:54:18 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:18 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-01 18:00:00) in space (linearNDFast)
12:54:18 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:18 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-01 17:00:00, weight 0.10) and
after (2024-11-01 18:00:00, weight 0.90) in time
12:54:18 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:18 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.4949828196721 and -59.29115410338839 degrees.
12:54:18 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.4949828196721 and -59.29115410338839 degrees.
12:54:18 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:18 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:18 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:18 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:18 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:18 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:18 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:18 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:18 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:18 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:18 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:18 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:18 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:18 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:18 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:18 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:18 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:18 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:18 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:18 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:18 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:18 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:18 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:18 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:18 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:18 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:18 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:18 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:18 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:18 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0802531 (min) 0.133739 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.039361 (min) 0.235182 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.0044764 (min) 0.0392227 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: x_wind: 6.72025 (min) 7.80394 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: y_wind: -10.6828 (min) -8.62912 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.40407 (min) 24.2999 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.6603 (min) 13.0581 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 31.9985 (min) 32.69 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -5.49691e-05 (min) 0.000257925 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:18 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:18 DEBUG opendrift.models.basemodel:755: Lifting 2 elements to seafloor.
12:54:18 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:18 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:18 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:18 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:18 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 17
12:54:18 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 0 0 0 0 0 0 0 0 0 0 0 3 0 0 0 0]
12:54:18 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 3 3 3 3 3 3 3 3 3 3 3 0 3 3 3 3]
12:54:18 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 228. 0.]
[ 0. 0. 0. 0. 0.]
[ 8. 0. 0. 0. 0.]
[ 2. 0. 152. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:18 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:18 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:18 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:18 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:18 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.11671587375673013
12:54:18 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:18 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:18 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:18 DEBUG opendrift.models.oceandrift:618: 46 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:18 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:18 DEBUG opendrift.models.oceandrift:618: 46 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:18 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:18 DEBUG opendrift.models.oceandrift:618: 43 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:18 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:18 DEBUG opendrift.models.oceandrift:618: 45 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:18 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:18 DEBUG opendrift.models.oceandrift:618: 35 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:18 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:18 DEBUG opendrift.models.oceandrift:618: 38 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:18 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:18 DEBUG opendrift.models.oceandrift:618: 45 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:18 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:18 DEBUG opendrift.models.oceandrift:618: 42 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:18 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:18 DEBUG opendrift.models.oceandrift:618: 35 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:18 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:18 DEBUG opendrift.models.oceandrift:618: 44 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
12:54:18 DEBUG opendrift.models.basemodel:755: Lifting 2 elements to seafloor.
12:54:18 DEBUG opendrift.models.oceandrift:618: 37 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:18 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:18 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:18 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:18 DEBUG opendrift.models.oceandrift:618: 32 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:18 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:18 DEBUG opendrift.models.oceandrift:618: 34 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:18 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:18 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:636: 3 elements reached seafloor, interacting with bottom
12:54:18 DEBUG opendrift.models.basemodel:755: Lifting 3 elements to seafloor.
12:54:18 DEBUG opendrift.models.oceandrift:618: 40 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:18 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:18 DEBUG opendrift.models.oceandrift:618: 38 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:18 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:18 DEBUG opendrift.models.oceandrift:618: 38 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:18 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:18 DEBUG opendrift.models.oceandrift:618: 39 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:18 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:18 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
12:54:18 DEBUG opendrift.models.basemodel:755: Lifting 2 elements to seafloor.
12:54:18 DEBUG opendrift.models.oceandrift:618: 34 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
12:54:18 DEBUG opendrift.models.basemodel:755: Lifting 2 elements to seafloor.
12:54:18 DEBUG opendrift.models.oceandrift:618: 45 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:18 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:18 DEBUG opendrift.models.oceandrift:618: 37 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:18 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:18 DEBUG opendrift.models.oceandrift:618: 40 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:18 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:18 DEBUG opendrift.models.oceandrift:618: 46 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:18 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:18 DEBUG opendrift.models.oceandrift:618: 39 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:18 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:18 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:18 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:18 DEBUG opendrift.models.oceandrift:618: 44 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
12:54:18 DEBUG opendrift.models.basemodel:755: Lifting 2 elements to seafloor.
12:54:18 DEBUG opendrift.models.oceandrift:618: 41 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:18 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:18 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:54:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:18 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:18 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 33
12:54:18 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:18 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 33 elements
12:54:18 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:18 INFO opendrift.models.chemicaldrift:1861: partitioning: [231, 0, 228, 41, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:18 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:18 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:18 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:18 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:18 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:18 INFO opendrift.models.basemodel:2038: 2024-11-01 18:24:02.760182 - step 12 of 96 - 500 active elements (0 deactivated)
12:54:18 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:18 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:18 DEBUG opendrift.models.basemodel:2057: 57.55294016751418 <- latitude -> 57.65921806811224
12:54:18 DEBUG opendrift.models.basemodel:2062: 10.505523291874429 <- longitude -> 10.708279866891802
12:54:18 DEBUG opendrift.models.basemodel:2067: -21.552392275061855 <- z -> 0.0
12:54:18 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:18 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:18 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:18 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:18 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:18 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:18 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:18 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:18 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-01 18:00:00 (before)
2024-11-01 19:00:00 (after)
12:54:20 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
12:54:20 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:54:20 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:54:20 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:54:20 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:54:20 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'upward_sea_water_velocity', 'y_sea_water_velocity', 'sea_water_temperature', 'sea_water_salinity']
12:54:20 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 40x35x7) for time after (2024-11-01 19:00:00)
12:54:20 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-01 18:00:00) in space (linearNDFast)
12:54:20 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:20 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-01 19:00:00) in space (linearNDFast)
12:54:20 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:20 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-01 18:00:00, weight 0.60) and
after (2024-11-01 19:00:00, weight 0.40) in time
12:54:20 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:20 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.49448779648193 and -59.291731192937945 degrees.
12:54:20 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.49448779648193 and -59.291731192937945 degrees.
12:54:20 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:20 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:20 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:20 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:20 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:20 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:20 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:20 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:20 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:20 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:20 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:20 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:20 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:20 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:20 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:20 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:20 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:20 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:20 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:20 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:20 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:20 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:20 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:20 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:20 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:20 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:20 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:20 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:20 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:20 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0786627 (min) 0.128225 (max)
12:54:20 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0150412 (min) 0.251418 (max)
12:54:20 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.0589935 (min) -0.0160615 (max)
12:54:20 DEBUG opendrift.models.basemodel.environment:905: x_wind: 6.73333 (min) 7.50633 (max)
12:54:20 DEBUG opendrift.models.basemodel.environment:905: y_wind: -10.7202 (min) -9.14396 (max)
12:54:20 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:20 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.40741 (min) 24.1913 (max)
12:54:20 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:20 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.6196 (min) 13.041 (max)
12:54:20 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 31.9888 (min) 32.6394 (max)
12:54:20 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -3.97172e-05 (min) 0.000302371 (max)
12:54:20 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:20 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:20 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:20 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:20 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:20 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:20 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:20 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 43 elements to seafloor.
12:54:20 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:20 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:20 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:20 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:20 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 16
12:54:20 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 2 2 0 0 0 0 0 0 0 0 0 2 2 0 0]
12:54:20 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 0 0 3 3 3 3 3 3 3 3 3 0 0 3 3]
12:54:20 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 240. 0.]
[ 0. 0. 0. 0. 0.]
[ 12. 0. 0. 0. 0.]
[ 2. 0. 185. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:20 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:20 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:20 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:20 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:20 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.11955758149956584
12:54:20 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:20 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:20 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:20 DEBUG opendrift.models.oceandrift:618: 42 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 54 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 54 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 42 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 54 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 54 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 40 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 55 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 55 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 50 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 56 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 56 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 38 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 56 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 56 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 46 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 56 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 56 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 32 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 56 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 56 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 38 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 56 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 56 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 38 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 56 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 56 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 43 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 56 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 56 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 36 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 56 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 56 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 37 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 56 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 56 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 38 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 56 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 56 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 44 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 56 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 56 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 51 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 57 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 57 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 35 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 57 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 57 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 38 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 57 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 57 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 33 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 57 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 57 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 36 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 57 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 57 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 33 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 57 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 57 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 50 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 57 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 57 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 38 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 57 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 57 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 37 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 58 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 58 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 38 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 58 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 58 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 33 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 59 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 59 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 59 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 59 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 44 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 60 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 60 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 35 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 60 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 60 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 37 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 60 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 60 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 41 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 60 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 60 elements to seafloor.
12:54:20 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 24
12:54:20 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:20 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 24 elements
12:54:20 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:20 INFO opendrift.models.chemicaldrift:1861: partitioning: [223, 0, 241, 36, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:20 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:20 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:20 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 37 elements to seafloor.
12:54:20 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:20 INFO opendrift.models.basemodel:2038: 2024-11-01 18:54:02.760182 - step 13 of 96 - 500 active elements (0 deactivated)
12:54:20 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:20 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:20 DEBUG opendrift.models.basemodel:2057: 57.55353677671186 <- latitude -> 57.66244687808314
12:54:20 DEBUG opendrift.models.basemodel:2062: 10.506028583031327 <- longitude -> 10.707548399992072
12:54:20 DEBUG opendrift.models.basemodel:2067: -20.835332435636346 <- z -> -0.03571873265289893
12:54:20 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:20 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:20 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:20 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:20 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:20 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:20 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:20 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:20 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-01 18:00:00 (before)
2024-11-01 19:00:00 (after)
12:54:20 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-01 18:00:00) in space (linearNDFast)
12:54:20 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:20 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-01 19:00:00) in space (linearNDFast)
12:54:20 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:20 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-01 18:00:00, weight 0.10) and
after (2024-11-01 19:00:00, weight 0.90) in time
12:54:20 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:20 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.49398249630868 and -59.29246266650582 degrees.
12:54:20 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.49398249630868 and -59.29246266650582 degrees.
12:54:20 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:20 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:20 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:20 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:20 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:20 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:20 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:20 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:20 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:20 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:20 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:20 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:20 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:20 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:20 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:20 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:20 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:20 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:20 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:20 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:20 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:20 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:20 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:20 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:20 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:20 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:20 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:20 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:20 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:20 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0574234 (min) 0.12769 (max)
12:54:20 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0398195 (min) 0.25265 (max)
12:54:20 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.111067 (min) -0.0692427 (max)
12:54:20 DEBUG opendrift.models.basemodel.environment:905: x_wind: 6.48411 (min) 7.35814 (max)
12:54:20 DEBUG opendrift.models.basemodel.environment:905: y_wind: -10.6209 (min) -9.57382 (max)
12:54:20 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:20 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.41003 (min) 24.0392 (max)
12:54:20 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:20 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.6294 (min) 13.0089 (max)
12:54:20 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 31.9994 (min) 32.5651 (max)
12:54:20 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -3.94813e-05 (min) 0.000275576 (max)
12:54:20 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:20 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:20 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:20 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:20 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:20 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:20 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:20 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 37 elements to seafloor.
12:54:20 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:20 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:20 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:20 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:20 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 14
12:54:20 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 0 0 0 0 0 0 0 0 2 0 0]
12:54:20 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 3 3 3 3 3 3 3 3 0 3 3]
12:54:20 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 253. 0.]
[ 0. 0. 0. 0. 0.]
[ 13. 0. 0. 7. 0.]
[ 2. 0. 209. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:20 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:20 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:20 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:20 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:20 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.12027491401047427
12:54:20 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:20 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:20 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:20 DEBUG opendrift.models.oceandrift:618: 47 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 49 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 49 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 46 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 49 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 49 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 54 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 50 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 50 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 52 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 53 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 53 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 36 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 53 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 53 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 46 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 53 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 53 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 47 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 53 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 53 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 52 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 54 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 54 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 43 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 55 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 55 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 44 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 56 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 56 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 44 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 57 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 57 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 38 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 57 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 57 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 37 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 57 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 57 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 38 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 57 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 57 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 42 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 59 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 59 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 59 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 59 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 59 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 59 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 40 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 59 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 59 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 39 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 59 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 59 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 40 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 60 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 60 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 45 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 60 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 60 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 46 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 60 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 60 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 35 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 60 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 60 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 60 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 60 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 35 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 60 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 60 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 46 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 60 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 60 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 46 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 61 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 61 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 32 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 63 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 63 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 36 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 63 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 63 elements to seafloor.
12:54:20 DEBUG opendrift.models.oceandrift:618: 50 elements penetrated seafloor, lifting up
12:54:20 DEBUG opendrift.models.oceandrift:636: 64 elements reached seafloor, interacting with bottom
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 64 elements to seafloor.
12:54:20 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 28
12:54:20 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:20 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 28 elements
12:54:20 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:20 INFO opendrift.models.chemicaldrift:1861: partitioning: [211, 0, 253, 36, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:20 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:20 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:20 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:20 DEBUG opendrift.models.basemodel:755: Lifting 38 elements to seafloor.
12:54:20 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:20 INFO opendrift.models.basemodel:2038: 2024-11-01 19:24:02.760182 - step 14 of 96 - 500 active elements (0 deactivated)
12:54:20 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:20 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:20 DEBUG opendrift.models.basemodel:2057: 57.554207304902256 <- latitude -> 57.66610425607013
12:54:20 DEBUG opendrift.models.basemodel:2062: 10.506520431155176 <- longitude -> 10.706972797417617
12:54:20 DEBUG opendrift.models.basemodel:2067: -22.504289528326215 <- z -> -0.05321538367861356
12:54:20 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:20 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:20 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:20 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:20 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:20 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:20 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:20 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:20 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-01 19:00:00 (before)
2024-11-01 20:00:00 (after)
12:54:21 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
12:54:21 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:54:21 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:54:21 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:54:21 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:54:21 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'upward_sea_water_velocity', 'y_sea_water_velocity', 'sea_water_temperature', 'sea_water_salinity']
12:54:21 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 40x35x7) for time after (2024-11-01 20:00:00)
12:54:21 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-01 19:00:00) in space (linearNDFast)
12:54:21 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:21 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-01 20:00:00) in space (linearNDFast)
12:54:21 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:21 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-01 19:00:00, weight 0.60) and
after (2024-11-01 20:00:00, weight 0.40) in time
12:54:21 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:21 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.49349065667316 and -59.29303826744648 degrees.
12:54:21 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.49349065667316 and -59.29303826744648 degrees.
12:54:21 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:21 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:21 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:21 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:21 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:21 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:21 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:21 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:21 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:21 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:21 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:21 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:21 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:21 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:21 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:21 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:21 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:21 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:21 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:21 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:21 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:21 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:21 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:21 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:21 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:21 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:21 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:21 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:21 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:21 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0626677 (min) 0.136405 (max)
12:54:21 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0367997 (min) 0.252281 (max)
12:54:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.151075 (min) -0.111059 (max)
12:54:21 DEBUG opendrift.models.basemodel.environment:905: x_wind: 5.64503 (min) 6.71321 (max)
12:54:21 DEBUG opendrift.models.basemodel.environment:905: y_wind: -10.7362 (min) -9.70071 (max)
12:54:21 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:21 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.41151 (min) 23.8806 (max)
12:54:21 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:21 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.6139 (min) 12.9816 (max)
12:54:21 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0328 (min) 32.541 (max)
12:54:21 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -3.45739e-05 (min) 0.00024674 (max)
12:54:21 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:21 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:21 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:21 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:21 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:21 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:21 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:21 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 41 elements to seafloor.
12:54:21 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:21 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:21 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:21 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:21 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 13
12:54:21 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 2 0 0 0 0 0 0 0 0 0 0 0]
12:54:21 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 0 3 3 3 3 3 3 3 3 3 3 3]
12:54:21 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 265. 0.]
[ 0. 0. 0. 0. 0.]
[ 14. 0. 0. 22. 0.]
[ 2. 0. 237. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:21 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:21 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:21 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:21 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:21 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.11392520230994971
12:54:21 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:21 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:21 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:21 DEBUG opendrift.models.oceandrift:618: 47 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 48 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 48 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 48 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 48 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 48 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 48 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 48 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 48 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 47 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 48 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 48 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 38 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 48 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 48 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 37 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 50 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 50 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 42 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 50 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 50 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 50 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 50 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 50 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 36 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 50 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 50 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 34 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 50 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 50 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 48 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 50 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 50 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 46 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 50 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 50 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 46 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 50 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 50 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 40 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 51 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 51 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 42 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 51 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 51 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 34 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 51 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 51 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 39 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 53 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 53 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 41 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 53 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 53 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 36 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 53 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 53 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 38 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 53 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 53 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 39 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 54 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 54 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 47 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 55 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 55 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 40 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 55 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 55 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 40 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 56 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 56 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 42 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 56 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 56 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 55 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 57 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 57 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 43 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 57 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 57 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 50 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 57 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 57 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 40 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 57 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 57 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 43 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 57 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 57 elements to seafloor.
12:54:21 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 19
12:54:21 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:21 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 19 elements
12:54:21 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:21 INFO opendrift.models.chemicaldrift:1861: partitioning: [200, 0, 262, 38, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:21 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:21 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:21 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 40 elements to seafloor.
12:54:21 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:21 INFO opendrift.models.basemodel:2038: 2024-11-01 19:54:02.760182 - step 15 of 96 - 500 active elements (0 deactivated)
12:54:21 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:21 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:21 DEBUG opendrift.models.basemodel:2057: 57.55480206827591 <- latitude -> 57.66964539618447
12:54:21 DEBUG opendrift.models.basemodel:2062: 10.506953311411934 <- longitude -> 10.70665780578116
12:54:21 DEBUG opendrift.models.basemodel:2067: -21.579226058684508 <- z -> -0.06167183837982826
12:54:21 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:21 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:21 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:21 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:21 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:21 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:21 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:21 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:21 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-01 19:00:00 (before)
2024-11-01 20:00:00 (after)
12:54:21 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-01 19:00:00) in space (linearNDFast)
12:54:21 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:21 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-01 20:00:00) in space (linearNDFast)
12:54:21 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:21 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-01 19:00:00, weight 0.10) and
after (2024-11-01 20:00:00, weight 0.90) in time
12:54:21 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:21 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.493057770294214 and -59.29335325519659 degrees.
12:54:21 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.493057770294214 and -59.29335325519659 degrees.
12:54:21 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:21 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:21 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:21 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:21 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:21 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:21 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:21 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:21 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:21 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:21 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:21 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:21 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:21 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:21 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:21 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:21 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:21 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:21 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:21 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:21 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:21 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:21 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:21 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:21 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:21 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:21 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:21 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:21 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:21 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0572297 (min) 0.148024 (max)
12:54:21 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0305508 (min) 0.254257 (max)
12:54:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.188084 (min) -0.149776 (max)
12:54:21 DEBUG opendrift.models.basemodel.environment:905: x_wind: 4.72685 (min) 5.86308 (max)
12:54:21 DEBUG opendrift.models.basemodel.environment:905: y_wind: -11.0095 (min) -9.72576 (max)
12:54:21 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:21 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.41045 (min) 23.7206 (max)
12:54:21 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:21 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.5803 (min) 12.9608 (max)
12:54:21 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0405 (min) 32.4959 (max)
12:54:21 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -3.40941e-05 (min) 0.000226913 (max)
12:54:21 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:21 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:21 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:21 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:21 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:21 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:21 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:21 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 40 elements to seafloor.
12:54:21 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:21 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:21 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:21 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:21 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 10
12:54:21 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 2 2 0 0 0 0 0 0 0]
12:54:21 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 0 0 3 3 3 3 3 3 3]
12:54:21 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 273. 0.]
[ 0. 0. 0. 0. 0.]
[ 16. 0. 0. 31. 0.]
[ 2. 0. 256. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:21 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:21 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:21 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:21 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:21 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.10891176177602202
12:54:21 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:21 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:21 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:21 DEBUG opendrift.models.oceandrift:618: 47 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 46 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 46 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 45 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 47 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 47 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 35 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 48 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 48 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 44 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 48 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 48 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 43 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 49 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 49 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 37 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 49 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 49 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 32 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 49 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 49 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 33 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 49 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 49 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 39 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 50 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 50 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 50 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 50 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 40 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 51 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 51 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 43 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 51 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 51 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 47 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 51 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 51 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 45 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 51 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 51 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 38 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 51 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 51 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 50 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 51 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 51 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 44 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 52 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 52 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 49 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 52 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 52 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 40 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 52 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 52 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 37 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 52 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 52 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 40 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 52 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 52 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 37 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 52 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 52 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 46 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 53 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 53 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 42 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 54 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 54 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 46 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 54 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 54 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 43 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 54 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 54 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 42 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 55 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 55 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 41 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 55 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 55 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 40 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 56 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 56 elements to seafloor.
12:54:21 DEBUG opendrift.models.oceandrift:618: 38 elements penetrated seafloor, lifting up
12:54:21 DEBUG opendrift.models.oceandrift:636: 56 elements reached seafloor, interacting with bottom
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 56 elements to seafloor.
12:54:21 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 15
12:54:21 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:21 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 15 elements
12:54:21 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:21 INFO opendrift.models.chemicaldrift:1861: partitioning: [194, 0, 265, 41, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:21 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:21 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:21 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:21 DEBUG opendrift.models.basemodel:755: Lifting 42 elements to seafloor.
12:54:21 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:21 INFO opendrift.models.basemodel:2038: 2024-11-01 20:24:02.760182 - step 16 of 96 - 500 active elements (0 deactivated)
12:54:21 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:21 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:21 DEBUG opendrift.models.basemodel:2057: 57.55558860602242 <- latitude -> 57.67346112768288
12:54:21 DEBUG opendrift.models.basemodel:2062: 10.50760480245287 <- longitude -> 10.70665967869377
12:54:21 DEBUG opendrift.models.basemodel:2067: -19.850355656317976 <- z -> 0.0
12:54:21 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:21 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:21 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:21 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:21 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:21 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:21 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:21 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:21 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-01 20:00:00 (before)
2024-11-01 21:00:00 (after)
12:54:23 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
12:54:23 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:54:23 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:54:23 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:54:23 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:54:23 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'upward_sea_water_velocity', 'y_sea_water_velocity', 'sea_water_temperature', 'sea_water_salinity']
12:54:23 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 41x34x7) for time after (2024-11-01 21:00:00)
12:54:23 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-01 20:00:00) in space (linearNDFast)
12:54:23 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:23 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-01 21:00:00) in space (linearNDFast)
12:54:23 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:23 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-01 20:00:00, weight 0.60) and
after (2024-11-01 21:00:00, weight 0.40) in time
12:54:23 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:23 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.4924062829339 and -59.29335138754496 degrees.
12:54:23 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.4924062829339 and -59.29335138754496 degrees.
12:54:23 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:23 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:23 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:23 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:23 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:23 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:23 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:23 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:23 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:23 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:23 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:23 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:23 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:23 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:23 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:23 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:23 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:23 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:23 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:23 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:23 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:23 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:23 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:23 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:23 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:23 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:23 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:23 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:23 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:23 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0644553 (min) 0.144357 (max)
12:54:23 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.00457507 (min) 0.257206 (max)
12:54:23 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.21793 (min) -0.179932 (max)
12:54:23 DEBUG opendrift.models.basemodel.environment:905: x_wind: 4.62147 (min) 5.45574 (max)
12:54:23 DEBUG opendrift.models.basemodel.environment:905: y_wind: -10.8425 (min) -9.49857 (max)
12:54:23 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:23 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.43581 (min) 23.5632 (max)
12:54:23 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:23 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.5733 (min) 12.9464 (max)
12:54:23 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0312 (min) 32.4805 (max)
12:54:23 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -4.72688e-05 (min) 0.000135797 (max)
12:54:23 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:23 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:23 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:23 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:23 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:23 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:23 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:23 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 43 elements to seafloor.
12:54:23 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:23 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:23 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:23 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:23 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 8
12:54:23 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 2 2 0 0 0 2 2]
12:54:23 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 0 0 3 3 3 0 0]
12:54:23 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 276. 0.]
[ 0. 0. 0. 0. 0.]
[ 21. 0. 0. 41. 0.]
[ 2. 0. 271. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:23 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:23 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:23 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:23 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:23 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.1038916981035083
12:54:23 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:23 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:23 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:23 DEBUG opendrift.models.oceandrift:618: 33 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 44 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 44 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 38 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 45 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 45 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 48 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 45 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 45 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 46 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 45 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 45 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 37 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 45 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 45 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 37 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 45 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 45 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 36 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 45 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 45 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 43 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 47 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 47 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 45 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 47 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 47 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 50 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 48 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 48 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 35 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 51 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 51 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 36 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 51 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 51 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 40 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 51 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 51 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 40 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 51 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 51 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 41 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 51 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 51 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 38 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 51 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 51 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 38 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 51 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 51 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 38 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 51 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 51 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 43 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 51 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 51 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 42 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 52 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 52 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 51 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 52 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 52 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 50 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 52 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 52 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 40 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 53 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 53 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 42 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 54 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 54 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 43 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 54 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 54 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 40 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 54 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 54 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 55 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 54 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 54 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 45 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 54 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 54 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 50 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 54 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 54 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 33 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 55 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 55 elements to seafloor.
12:54:23 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 14
12:54:23 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:23 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 14 elements
12:54:23 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:23 INFO opendrift.models.chemicaldrift:1861: partitioning: [196, 0, 263, 41, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:23 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:23 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:23 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 41 elements to seafloor.
12:54:23 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:23 INFO opendrift.models.basemodel:2038: 2024-11-01 20:54:02.760182 - step 17 of 96 - 500 active elements (0 deactivated)
12:54:23 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:23 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:23 DEBUG opendrift.models.basemodel:2057: 57.55566254634917 <- latitude -> 57.677156774195076
12:54:23 DEBUG opendrift.models.basemodel:2062: 10.507927244297795 <- longitude -> 10.708428876034864
12:54:23 DEBUG opendrift.models.basemodel:2067: -20.034610057829255 <- z -> -0.024652308890750985
12:54:23 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:23 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:23 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:23 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:23 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:23 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:23 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:23 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:23 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-01 20:00:00 (before)
2024-11-01 21:00:00 (after)
12:54:23 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-01 20:00:00) in space (linearNDFast)
12:54:23 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:23 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-01 21:00:00) in space (linearNDFast)
12:54:23 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:23 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-01 20:00:00, weight 0.10) and
after (2024-11-01 21:00:00, weight 0.90) in time
12:54:23 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:23 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.29158218131386 degrees.
12:54:23 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.29158218131386 degrees.
12:54:23 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:23 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:23 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:23 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:23 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:23 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:23 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:23 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:23 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:23 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:23 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:23 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:23 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:23 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:23 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:23 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:23 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:23 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:23 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:23 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:23 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:23 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:23 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:23 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:23 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:23 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:23 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:23 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:23 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:23 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0588131 (min) 0.139882 (max)
12:54:23 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0256131 (min) 0.259684 (max)
12:54:23 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.24594 (min) -0.207443 (max)
12:54:23 DEBUG opendrift.models.basemodel.environment:905: x_wind: 4.71258 (min) 5.18418 (max)
12:54:23 DEBUG opendrift.models.basemodel.environment:905: y_wind: -10.5041 (min) -9.15337 (max)
12:54:23 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:23 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 23.5535 (max)
12:54:23 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:23 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.5411 (min) 12.9326 (max)
12:54:23 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0365 (min) 32.4669 (max)
12:54:23 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -4.98173e-05 (min) 0.000158767 (max)
12:54:23 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:23 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:23 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:23 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:23 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:23 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:23 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:23 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 41 elements to seafloor.
12:54:23 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:23 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:23 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:23 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:23 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 10
12:54:23 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 0 2 0 0 0 0 0 0 0]
12:54:23 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 3 0 3 3 3 3 3 3 3]
12:54:23 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 284. 0.]
[ 0. 0. 0. 0. 0.]
[ 23. 0. 0. 52. 0.]
[ 2. 0. 285. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:23 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:23 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:23 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:23 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:23 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.09900951998569398
12:54:23 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:23 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:23 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:23 DEBUG opendrift.models.oceandrift:618: 42 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 49 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 49 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 33 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 49 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 49 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 50 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 50 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 50 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 49 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 50 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 50 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 33 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 51 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 51 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 43 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 51 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 51 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 36 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 52 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 52 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 53 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 52 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 52 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 36 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 53 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 53 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 40 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 53 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 53 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 36 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 53 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 53 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 46 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 53 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 53 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 42 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 55 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 55 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 47 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 55 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 55 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 50 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 56 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 56 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 41 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 57 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 57 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 47 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 58 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 58 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 49 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 58 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 58 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 41 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 58 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 58 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 45 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 59 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 59 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 41 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 59 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 59 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 40 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 60 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 60 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 35 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 60 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 60 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 42 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 61 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 61 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 32 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 62 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 62 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 37 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 62 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 62 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 38 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 62 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 62 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 62 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 62 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 45 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 62 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 62 elements to seafloor.
12:54:23 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
12:54:23 DEBUG opendrift.models.oceandrift:636: 62 elements reached seafloor, interacting with bottom
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 62 elements to seafloor.
12:54:23 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 20
12:54:23 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:23 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 20 elements
12:54:23 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:23 INFO opendrift.models.chemicaldrift:1861: partitioning: [190, 0, 268, 42, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:23 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:23 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:23 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:23 DEBUG opendrift.models.basemodel:755: Lifting 44 elements to seafloor.
12:54:23 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:23 INFO opendrift.models.basemodel:2038: 2024-11-01 21:24:02.760182 - step 18 of 96 - 500 active elements (0 deactivated)
12:54:23 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:23 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:23 DEBUG opendrift.models.basemodel:2057: 57.55623575146658 <- latitude -> 57.68083435102822
12:54:23 DEBUG opendrift.models.basemodel:2062: 10.507927244297795 <- longitude -> 10.709081988469578
12:54:23 DEBUG opendrift.models.basemodel:2067: -20.562786102294922 <- z -> -0.05892942346264615
12:54:23 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:23 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:23 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:23 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:23 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:23 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:23 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:23 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:23 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-01 21:00:00 (before)
2024-11-01 22:00:00 (after)
12:54:25 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
12:54:25 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:54:25 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:54:25 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:54:25 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:54:25 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'upward_sea_water_velocity', 'y_sea_water_velocity', 'sea_water_temperature', 'sea_water_salinity']
12:54:25 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 42x34x7) for time after (2024-11-01 22:00:00)
12:54:25 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-01 21:00:00) in space (linearNDFast)
12:54:25 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:25 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-01 22:00:00) in space (linearNDFast)
12:54:25 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:25 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-01 21:00:00, weight 0.60) and
after (2024-11-01 22:00:00, weight 0.40) in time
12:54:25 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:25 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.290929062072884 degrees.
12:54:25 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.290929062072884 degrees.
12:54:25 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:25 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:25 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:25 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:25 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:25 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:25 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:25 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:25 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:25 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:25 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:25 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:25 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:25 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:25 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:25 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:25 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:25 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:25 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:25 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:25 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:25 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:25 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:25 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:25 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:25 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:25 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:25 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:25 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:25 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.054276 (min) 0.126442 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0128727 (min) 0.259522 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.262379 (min) -0.225328 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:905: x_wind: 4.64299 (min) 4.98743 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:905: y_wind: -10.0108 (min) -8.7589 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 23.4217 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.5292 (min) 12.8989 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0569 (min) 32.4198 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -5.47327e-05 (min) 0.00017289 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:25 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 44 elements to seafloor.
12:54:25 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:25 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:25 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:25 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:25 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 10
12:54:25 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 2 0 0 0 2 0 0 0 0]
12:54:25 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 0 3 3 3 0 3 3 3 3]
12:54:25 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 291. 0.]
[ 0. 0. 0. 0. 0.]
[ 26. 0. 0. 65. 0.]
[ 2. 0. 305. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:25 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:25 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:25 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:25 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:25 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.08996748126720161
12:54:25 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:25 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:25 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:25 DEBUG opendrift.models.oceandrift:618: 41 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 49 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 49 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 34 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 49 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 49 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 34 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 50 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 50 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 44 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 50 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 50 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 39 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 50 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 50 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 49 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 50 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 50 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 42 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 50 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 50 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 44 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 51 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 51 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 51 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 51 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 33 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 51 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 51 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 36 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 51 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 51 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 39 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 52 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 52 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 37 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 52 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 52 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 52 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 52 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 52 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 52 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 32 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 53 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 53 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 53 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 53 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 35 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 53 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 53 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 53 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 53 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 53 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 53 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 38 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 55 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 55 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 32 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 55 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 55 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 44 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 56 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 56 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 46 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 58 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 58 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 33 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 58 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 58 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 41 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 58 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 58 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 44 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 58 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 58 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 38 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 58 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 58 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 49 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 58 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 58 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 46 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 58 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 58 elements to seafloor.
12:54:25 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 13
12:54:25 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:25 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 13 elements
12:54:25 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:25 INFO opendrift.models.chemicaldrift:1861: partitioning: [186, 0, 269, 45, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:25 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:25 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:25 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 46 elements to seafloor.
12:54:25 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:25 INFO opendrift.models.basemodel:2038: 2024-11-01 21:54:02.760182 - step 19 of 96 - 500 active elements (0 deactivated)
12:54:25 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:25 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:25 DEBUG opendrift.models.basemodel:2057: 57.556027696258475 <- latitude -> 57.684385543347375
12:54:25 DEBUG opendrift.models.basemodel:2062: 10.507927244297797 <- longitude -> 10.709836197706489
12:54:25 DEBUG opendrift.models.basemodel:2067: -20.791729048542106 <- z -> 0.0
12:54:25 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:25 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:25 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:25 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:25 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:25 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:25 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:25 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:25 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-01 21:00:00 (before)
2024-11-01 22:00:00 (after)
12:54:25 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-01 21:00:00) in space (linearNDFast)
12:54:25 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:25 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-01 22:00:00) in space (linearNDFast)
12:54:25 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:25 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-01 21:00:00, weight 0.10) and
after (2024-11-01 22:00:00, weight 0.90) in time
12:54:25 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:25 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.29017485289448 degrees.
12:54:25 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.29017485289448 degrees.
12:54:25 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:25 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:25 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:25 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:25 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:25 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:25 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:25 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:25 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:25 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:25 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:25 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:25 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:25 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:25 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:25 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:25 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:25 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:25 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:25 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:25 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:25 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:25 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:25 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:25 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:25 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:25 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:25 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:25 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:25 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0566504 (min) 0.162402 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0129792 (min) 0.264538 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.275377 (min) -0.240719 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:905: x_wind: 4.54749 (min) 4.9563 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:905: y_wind: -9.42283 (min) -8.35033 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 23.2785 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.5129 (min) 12.9027 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0632 (min) 32.4208 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -4.02055e-05 (min) 0.000139564 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:25 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 46 elements to seafloor.
12:54:25 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:25 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:25 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:25 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:25 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 15
12:54:25 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 0 0 0 0 0 0 0 2 0 0 0 2]
12:54:25 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 3 3 3 3 3 3 3 0 3 3 3 0]
12:54:25 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 304. 0.]
[ 0. 0. 0. 0. 0.]
[ 28. 0. 0. 74. 0.]
[ 2. 0. 318. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:25 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:25 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:25 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:25 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:25 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.08011040815228383
12:54:25 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:25 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:25 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:25 DEBUG opendrift.models.oceandrift:618: 35 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 58 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 58 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 59 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 59 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 60 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 60 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 34 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 60 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 60 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 61 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 61 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 34 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 61 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 61 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 40 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 61 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 61 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 43 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 62 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 62 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 33 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 62 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 62 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 62 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 62 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 43 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 62 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 62 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 35 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 62 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 62 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 62 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 62 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 36 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 64 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 64 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 64 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 64 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 37 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 64 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 64 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 37 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 64 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 64 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 36 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 64 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 64 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 37 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 65 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 65 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 65 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 65 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 38 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 66 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 66 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 67 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 67 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 33 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 68 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 68 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 33 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 69 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 69 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 69 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 69 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 32 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 69 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 69 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 36 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 69 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 69 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 33 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 70 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 70 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 71 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 71 elements to seafloor.
12:54:25 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:54:25 DEBUG opendrift.models.oceandrift:636: 71 elements reached seafloor, interacting with bottom
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 71 elements to seafloor.
12:54:25 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 27
12:54:25 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:25 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 27 elements
12:54:25 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:25 INFO opendrift.models.chemicaldrift:1861: partitioning: [175, 0, 281, 44, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:25 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:25 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:25 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:25 DEBUG opendrift.models.basemodel:755: Lifting 48 elements to seafloor.
12:54:25 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:25 INFO opendrift.models.basemodel:2038: 2024-11-01 22:24:02.760182 - step 20 of 96 - 500 active elements (0 deactivated)
12:54:25 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:25 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:25 DEBUG opendrift.models.basemodel:2057: 57.55623746526277 <- latitude -> 57.68787385868882
12:54:25 DEBUG opendrift.models.basemodel:2062: 10.507927244297797 <- longitude -> 10.711382257134218
12:54:25 DEBUG opendrift.models.basemodel:2067: -20.434601268618735 <- z -> -0.055096394011303396
12:54:25 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:25 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:25 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:25 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:25 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:25 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:25 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:25 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:25 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-01 22:00:00 (before)
2024-11-01 23:00:00 (after)
12:54:26 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
12:54:26 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:54:26 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:54:26 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:54:26 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:54:26 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'upward_sea_water_velocity', 'y_sea_water_velocity', 'sea_water_temperature', 'sea_water_salinity']
12:54:26 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 43x34x7) for time after (2024-11-01 23:00:00)
12:54:26 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-01 22:00:00) in space (linearNDFast)
12:54:26 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:26 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-01 23:00:00) in space (linearNDFast)
12:54:26 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:26 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-01 22:00:00, weight 0.60) and
after (2024-11-01 23:00:00, weight 0.40) in time
12:54:26 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:26 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.288628790917386 degrees.
12:54:26 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.288628790917386 degrees.
12:54:26 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:26 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:26 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:26 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:26 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:26 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:26 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:26 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:26 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:26 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:26 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:26 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:26 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:26 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:26 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:26 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:26 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:26 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:26 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:26 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:26 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:26 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:26 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0589177 (min) 0.158687 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0272287 (min) 0.263397 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.269133 (min) -0.237774 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: x_wind: 4.25587 (min) 4.68614 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: y_wind: -9.34069 (min) -8.32022 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 23.1524 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.495 (min) 12.898 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0614 (min) 32.4239 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -4.04443e-05 (min) 0.000105529 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:26 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 48 elements to seafloor.
12:54:26 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:26 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:26 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:26 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:26 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 12
12:54:26 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 2 0 2 2 2 0 0 2 0 2 2]
12:54:26 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 0 3 0 0 0 3 3 0 3 0 0]
12:54:26 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 309. 0.]
[ 0. 0. 0. 0. 0.]
[ 35. 0. 0. 87. 0.]
[ 2. 0. 345. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:26 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:26 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:26 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:26 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:26 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.0770496778766122
12:54:26 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:26 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:26 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:26 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 49 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 49 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 39 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 51 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 51 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 32 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 51 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 51 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 37 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 51 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 51 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 43 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 51 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 51 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 40 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 52 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 52 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 36 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 52 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 52 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 36 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 52 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 52 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 36 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 52 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 52 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 41 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 53 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 53 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 43 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 53 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 53 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 41 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 53 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 53 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 51 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 53 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 53 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 53 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 53 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 37 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 54 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 54 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 39 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 54 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 54 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 35 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 55 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 55 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 41 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 55 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 55 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 32 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 55 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 55 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 33 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 57 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 57 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 33 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 57 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 57 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 57 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 57 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 36 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 57 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 57 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 37 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 57 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 57 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 37 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 58 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 58 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 36 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 58 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 58 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 37 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 58 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 58 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 38 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 60 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 60 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 62 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 62 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 62 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 62 elements to seafloor.
12:54:26 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 18
12:54:26 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:26 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 18 elements
12:54:26 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:26 INFO opendrift.models.chemicaldrift:1861: partitioning: [177, 0, 279, 44, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:26 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:26 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:26 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 45 elements to seafloor.
12:54:26 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:26 INFO opendrift.models.basemodel:2038: 2024-11-01 22:54:02.760182 - step 21 of 96 - 500 active elements (0 deactivated)
12:54:26 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:26 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:26 DEBUG opendrift.models.basemodel:2057: 57.55667753658069 <- latitude -> 57.6901583822414
12:54:26 DEBUG opendrift.models.basemodel:2062: 10.507927244297797 <- longitude -> 10.713563241472622
12:54:26 DEBUG opendrift.models.basemodel:2067: -20.731156276619988 <- z -> -0.14645974601676237
12:54:26 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:26 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:26 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:26 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:26 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-01 22:00:00 (before)
2024-11-01 23:00:00 (after)
12:54:26 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-01 22:00:00) in space (linearNDFast)
12:54:26 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:26 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-01 23:00:00) in space (linearNDFast)
12:54:26 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:26 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-01 22:00:00, weight 0.10) and
after (2024-11-01 23:00:00, weight 0.90) in time
12:54:26 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:26 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.286447813668644 degrees.
12:54:26 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.286447813668644 degrees.
12:54:26 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:26 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:26 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:26 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:26 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:26 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:26 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:26 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:26 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:26 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:26 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:26 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:26 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:26 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:26 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:26 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:26 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:26 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:26 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:26 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:26 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:26 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:26 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0615586 (min) 0.156147 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0145613 (min) 0.259647 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.259104 (min) -0.229619 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: x_wind: 3.92995 (min) 4.34069 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: y_wind: -9.41305 (min) -8.38142 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 23.0898 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.4777 (min) 12.8687 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0734 (min) 32.4097 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -4.74275e-05 (min) 0.000119225 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:26 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 45 elements to seafloor.
12:54:26 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:26 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:26 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:26 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:26 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 11
12:54:26 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 0 0 0 0 2 0 0 2]
12:54:26 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 3 3 3 3 0 3 3 0]
12:54:26 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 318. 0.]
[ 0. 0. 0. 0. 0.]
[ 37. 0. 0. 100. 0.]
[ 2. 0. 363. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:26 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:26 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:26 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:26 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:26 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.07638705333773423
12:54:26 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:26 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:26 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:26 DEBUG opendrift.models.oceandrift:618: 33 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 53 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 53 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 54 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 54 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 34 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 55 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 55 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 39 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 55 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 55 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 41 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 56 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 56 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 57 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 57 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 32 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 58 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 58 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 37 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 60 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 60 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 37 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 62 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 62 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 34 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 62 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 62 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 36 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 63 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 63 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 44 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 63 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 63 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 35 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 63 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 63 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 36 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 64 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 64 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 33 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 64 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 64 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 40 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 64 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 64 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 66 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 66 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 40 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 68 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 68 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 68 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 68 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 68 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 68 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 32 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 69 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 69 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 35 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 69 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 69 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 69 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 69 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 35 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 69 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 69 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 69 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 69 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 36 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 69 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 69 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 70 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 70 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 70 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 70 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 70 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 70 elements to seafloor.
12:54:26 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:54:26 DEBUG opendrift.models.oceandrift:636: 71 elements reached seafloor, interacting with bottom
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 71 elements to seafloor.
12:54:26 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 24
12:54:26 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:26 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 24 elements
12:54:26 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:26 INFO opendrift.models.chemicaldrift:1861: partitioning: [170, 0, 283, 47, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:26 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:26 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:26 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:26 DEBUG opendrift.models.basemodel:755: Lifting 49 elements to seafloor.
12:54:26 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:26 INFO opendrift.models.basemodel:2038: 2024-11-01 23:24:02.760182 - step 22 of 96 - 500 active elements (0 deactivated)
12:54:26 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:26 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:26 DEBUG opendrift.models.basemodel:2057: 57.557053896137354 <- latitude -> 57.69237860834174
12:54:26 DEBUG opendrift.models.basemodel:2062: 10.507927244297795 <- longitude -> 10.716149364926846
12:54:26 DEBUG opendrift.models.basemodel:2067: -20.628101348876953 <- z -> -0.003784594030365497
12:54:26 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:26 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:26 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:26 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:26 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-01 23:00:00 (before)
2024-11-02 00:00:00 (after)
12:54:28 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
12:54:28 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:54:28 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:54:28 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:54:28 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:54:28 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'upward_sea_water_velocity', 'y_sea_water_velocity', 'sea_water_temperature', 'sea_water_salinity']
12:54:28 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 45x33x7) for time after (2024-11-02 00:00:00)
12:54:28 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-01 23:00:00) in space (linearNDFast)
12:54:28 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:28 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-02 00:00:00) in space (linearNDFast)
12:54:28 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:28 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-01 23:00:00, weight 0.60) and
after (2024-11-02 00:00:00, weight 0.40) in time
12:54:28 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:28 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.28386169000053 degrees.
12:54:28 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.28386169000053 degrees.
12:54:28 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:28 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:28 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:28 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:28 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:28 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:28 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:28 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:28 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:28 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:28 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:28 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:28 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:28 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:28 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:28 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:28 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:28 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:28 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:28 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:28 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:28 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:28 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:28 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:28 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:28 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:28 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:28 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:28 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:28 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0628839 (min) 0.143291 (max)
12:54:28 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0035772 (min) 0.241951 (max)
12:54:28 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.227599 (min) -0.199226 (max)
12:54:28 DEBUG opendrift.models.basemodel.environment:905: x_wind: 3.76246 (min) 4.07898 (max)
12:54:28 DEBUG opendrift.models.basemodel.environment:905: y_wind: -9.01039 (min) -7.92807 (max)
12:54:28 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:28 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 23.0053 (max)
12:54:28 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:28 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.4603 (min) 12.885 (max)
12:54:28 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0828 (min) 32.3911 (max)
12:54:28 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -4.7803e-05 (min) 0.000116949 (max)
12:54:28 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:28 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:28 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:28 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:28 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:28 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:28 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:28 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 49 elements to seafloor.
12:54:28 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:28 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:28 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:28 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:28 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 13
12:54:28 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 0 2 2 2 2 0 0 0 0 0 0 0]
12:54:28 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 3 0 0 0 0 3 3 3 3 3 3 3]
12:54:28 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 326. 0.]
[ 0. 0. 0. 0. 0.]
[ 42. 0. 0. 118. 0.]
[ 2. 0. 387. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:28 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:28 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:28 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:28 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:28 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.07024973397054424
12:54:28 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:28 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:28 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:28 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 55 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 55 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 55 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 55 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 34 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 55 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 55 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 34 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 56 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 56 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 38 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 56 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 56 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 42 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 57 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 57 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 32 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 57 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 57 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 32 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 57 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 57 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 37 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 60 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 60 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 60 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 60 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 38 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 60 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 60 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 60 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 60 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 38 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 61 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 61 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 36 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 61 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 61 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 33 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 61 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 61 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 61 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 61 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 61 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 61 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 61 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 61 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 61 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 61 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 61 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 61 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 61 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 61 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 62 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 62 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 62 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 62 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 33 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 63 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 63 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 63 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 63 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 63 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 63 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 64 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 64 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 64 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 64 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 64 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 64 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 33 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 65 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 65 elements to seafloor.
12:54:28 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 17
12:54:28 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:28 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 17 elements
12:54:28 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:28 INFO opendrift.models.chemicaldrift:1861: partitioning: [167, 0, 285, 48, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:28 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:28 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:28 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 52 elements to seafloor.
12:54:28 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:28 INFO opendrift.models.basemodel:2038: 2024-11-01 23:54:02.760182 - step 23 of 96 - 500 active elements (0 deactivated)
12:54:28 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:28 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:28 DEBUG opendrift.models.basemodel:2057: 57.5571117037965 <- latitude -> 57.69475567743255
12:54:28 DEBUG opendrift.models.basemodel:2062: 10.507927244297791 <- longitude -> 10.719298034324067
12:54:28 DEBUG opendrift.models.basemodel:2067: -21.214200279945004 <- z -> 0.0
12:54:28 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:28 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:28 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:28 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:28 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:28 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:28 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:28 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:28 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-01 23:00:00 (before)
2024-11-02 00:00:00 (after)
12:54:28 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-01 23:00:00) in space (linearNDFast)
12:54:28 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:28 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-02 00:00:00) in space (linearNDFast)
12:54:28 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:28 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-01 23:00:00, weight 0.10) and
after (2024-11-02 00:00:00, weight 0.90) in time
12:54:28 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:28 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.280713015460876 degrees.
12:54:28 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.280713015460876 degrees.
12:54:28 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:28 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:28 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:28 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:28 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:28 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:28 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:28 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:28 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:28 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:28 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:28 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:28 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:28 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:28 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:28 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:28 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:28 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:28 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:28 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:28 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:28 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:28 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:28 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:28 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:28 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:28 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:28 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:28 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:28 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0638833 (min) 0.134941 (max)
12:54:28 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0123738 (min) 0.221179 (max)
12:54:28 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.190333 (min) -0.162632 (max)
12:54:28 DEBUG opendrift.models.basemodel.environment:905: x_wind: 3.63311 (min) 3.94296 (max)
12:54:28 DEBUG opendrift.models.basemodel.environment:905: y_wind: -8.47549 (min) -7.34732 (max)
12:54:28 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:28 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 22.934 (max)
12:54:28 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:28 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.443 (min) 12.8525 (max)
12:54:28 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0804 (min) 32.3923 (max)
12:54:28 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -4.6435e-05 (min) 7.37536e-05 (max)
12:54:28 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:28 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:28 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:28 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:28 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:28 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:28 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:28 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 52 elements to seafloor.
12:54:28 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:28 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:28 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:28 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:28 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 9
12:54:28 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 0 0 2 0 0 2]
12:54:28 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 3 3 0 3 3 0]
12:54:28 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 333. 0.]
[ 0. 0. 0. 0. 0.]
[ 44. 0. 0. 128. 0.]
[ 2. 0. 404. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:28 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:28 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:28 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:28 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:28 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.0630379268728737
12:54:28 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:28 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:28 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:28 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 55 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 55 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 37 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 56 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 56 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 56 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 56 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 56 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 56 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 32 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 56 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 56 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 57 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 57 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 57 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 57 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 57 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 57 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 34 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 57 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 57 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 32 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 58 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 58 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 34 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 58 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 58 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 58 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 58 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 58 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 58 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 35 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 58 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 58 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 58 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 58 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 59 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 59 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 59 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 59 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 59 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 59 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 35 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 59 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 59 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 59 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 59 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 59 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 59 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 59 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 59 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 33 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 59 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 59 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 32 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 59 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 59 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 36 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 61 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 61 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 34 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 61 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 61 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 38 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 61 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 61 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 37 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 62 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 62 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 63 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 63 elements to seafloor.
12:54:28 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:54:28 DEBUG opendrift.models.oceandrift:636: 63 elements reached seafloor, interacting with bottom
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 63 elements to seafloor.
12:54:28 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 11
12:54:28 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:28 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 11 elements
12:54:28 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:28 INFO opendrift.models.chemicaldrift:1861: partitioning: [162, 0, 286, 52, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:28 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:28 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:28 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:28 DEBUG opendrift.models.basemodel:755: Lifting 52 elements to seafloor.
12:54:28 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:28 INFO opendrift.models.basemodel:2038: 2024-11-02 00:24:02.760182 - step 24 of 96 - 500 active elements (0 deactivated)
12:54:28 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:28 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:28 DEBUG opendrift.models.basemodel:2057: 57.556911706264586 <- latitude -> 57.696540917622116
12:54:28 DEBUG opendrift.models.basemodel:2062: 10.507927244297788 <- longitude -> 10.72315512277209
12:54:28 DEBUG opendrift.models.basemodel:2067: -20.871809047199196 <- z -> -0.0063617955476087396
12:54:28 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:28 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:28 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:28 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:28 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:28 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:28 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:28 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:28 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-02 00:00:00 (before)
2024-11-02 01:00:00 (after)
12:54:29 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
12:54:29 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:54:29 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:54:29 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:54:29 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:54:29 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'upward_sea_water_velocity', 'y_sea_water_velocity', 'sea_water_temperature', 'sea_water_salinity']
12:54:29 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 46x33x7) for time after (2024-11-02 01:00:00)
12:54:29 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-02 00:00:00) in space (linearNDFast)
12:54:29 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:29 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-02 01:00:00) in space (linearNDFast)
12:54:29 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:29 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-02 00:00:00, weight 0.60) and
after (2024-11-02 01:00:00, weight 0.40) in time
12:54:29 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:29 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.27685593004771 degrees.
12:54:29 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.27685593004771 degrees.
12:54:29 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:29 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:29 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:29 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:29 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:29 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:29 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:29 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:29 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:29 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:29 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:29 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:29 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:29 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:29 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:29 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:29 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:29 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:29 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:29 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:29 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:29 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:29 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:29 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:29 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:29 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:29 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:29 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:29 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:29 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0747746 (min) 0.134313 (max)
12:54:29 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0282774 (min) 0.17336 (max)
12:54:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.163983 (min) -0.113867 (max)
12:54:29 DEBUG opendrift.models.basemodel.environment:905: x_wind: 3.30028 (min) 3.66265 (max)
12:54:29 DEBUG opendrift.models.basemodel.environment:905: y_wind: -8.40814 (min) -7.29881 (max)
12:54:29 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:29 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 22.9305 (max)
12:54:29 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:29 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.4254 (min) 12.8171 (max)
12:54:29 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.072 (min) 32.3958 (max)
12:54:29 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -4.01724e-05 (min) 9.97436e-05 (max)
12:54:29 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:29 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:29 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:29 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:29 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:29 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:29 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:29 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 52 elements to seafloor.
12:54:29 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:29 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:29 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:29 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:29 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 5
12:54:29 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 2 2 0 0]
12:54:29 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 0 0 3 3]
12:54:29 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 335. 0.]
[ 0. 0. 0. 0. 0.]
[ 47. 0. 0. 136. 0.]
[ 2. 0. 415. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:29 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:29 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:29 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:29 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:29 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.06065995886662093
12:54:29 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:29 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:29 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:29 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 54 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 54 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 54 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 54 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 54 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 54 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 54 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 54 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 32 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 55 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 55 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 34 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 55 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 55 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 55 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 55 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 55 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 55 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 56 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 56 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 56 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 56 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 58 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 58 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 58 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 58 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 58 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 58 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 58 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 58 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 60 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 60 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 60 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 60 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 37 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 61 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 61 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 61 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 61 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 61 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 61 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 62 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 62 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 62 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 62 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 62 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 62 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 34 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 62 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 62 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 62 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 62 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 35 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 63 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 63 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 63 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 63 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 63 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 63 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 36 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 63 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 63 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 63 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 63 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 63 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 63 elements to seafloor.
12:54:29 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 3
12:54:29 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:29 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 3 elements
12:54:29 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:29 INFO opendrift.models.chemicaldrift:1861: partitioning: [163, 0, 277, 60, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:29 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:29 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:29 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 60 elements to seafloor.
12:54:29 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:29 INFO opendrift.models.basemodel:2038: 2024-11-02 00:54:02.760182 - step 25 of 96 - 500 active elements (0 deactivated)
12:54:29 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:29 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:29 DEBUG opendrift.models.basemodel:2057: 57.55645466659944 <- latitude -> 57.69722347793617
12:54:29 DEBUG opendrift.models.basemodel:2062: 10.507927244297788 <- longitude -> 10.726323470546117
12:54:29 DEBUG opendrift.models.basemodel:2067: -20.771619293833222 <- z -> 0.0
12:54:29 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:29 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:29 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:29 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:29 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:29 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:29 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:29 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:29 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-02 00:00:00 (before)
2024-11-02 01:00:00 (after)
12:54:29 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-02 00:00:00) in space (linearNDFast)
12:54:29 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:29 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-02 01:00:00) in space (linearNDFast)
12:54:29 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:29 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-02 00:00:00, weight 0.10) and
after (2024-11-02 01:00:00, weight 0.90) in time
12:54:29 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:29 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.273687582701726 degrees.
12:54:29 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.273687582701726 degrees.
12:54:29 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:29 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:29 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:29 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:29 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:29 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:29 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:29 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:29 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:29 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:29 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:29 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:29 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:29 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:29 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:29 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:29 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:29 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:29 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:29 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:29 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:29 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:29 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:29 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:29 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:29 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:29 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:29 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:29 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:29 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0884694 (min) 0.117332 (max)
12:54:29 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0563767 (min) 0.130255 (max)
12:54:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.140821 (min) -0.057829 (max)
12:54:29 DEBUG opendrift.models.basemodel.environment:905: x_wind: 2.90434 (min) 3.39116 (max)
12:54:29 DEBUG opendrift.models.basemodel.environment:905: y_wind: -8.45076 (min) -7.3821 (max)
12:54:29 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:29 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 23.0093 (max)
12:54:29 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:29 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.4077 (min) 12.809 (max)
12:54:29 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0644 (min) 32.3969 (max)
12:54:29 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -5.94608e-05 (min) 0.000139998 (max)
12:54:29 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:29 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:29 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:29 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:29 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:29 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:29 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:29 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 60 elements to seafloor.
12:54:29 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:29 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:29 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:29 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:29 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 8
12:54:29 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 0 2 0 0 0 0 0]
12:54:29 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 3 0 3 3 3 3 3]
12:54:29 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 341. 0.]
[ 0. 0. 0. 0. 0.]
[ 49. 0. 0. 145. 0.]
[ 2. 0. 418. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:29 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:29 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:29 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:29 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:29 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.0594833491176225
12:54:29 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:29 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:29 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:29 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 66 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 66 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 32 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 66 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 66 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 66 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 66 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 66 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 66 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 66 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 66 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 34 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 66 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 66 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 33 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 66 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 66 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 32 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 66 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 66 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 66 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 66 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 67 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 67 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 67 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 67 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 68 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 68 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 69 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 69 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 69 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 69 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 70 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 70 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 70 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 70 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 70 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 70 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 70 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 70 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 70 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 70 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 70 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 70 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 72 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 72 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 72 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 72 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 72 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 72 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 73 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 73 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 74 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 74 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 37 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 75 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 75 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 76 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 76 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 76 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 76 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 77 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 77 elements to seafloor.
12:54:29 DEBUG opendrift.models.oceandrift:618: 33 elements penetrated seafloor, lifting up
12:54:29 DEBUG opendrift.models.oceandrift:636: 77 elements reached seafloor, interacting with bottom
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 77 elements to seafloor.
12:54:29 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 0
12:54:29 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:29 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:29 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:29 INFO opendrift.models.chemicaldrift:1861: partitioning: [159, 0, 264, 77, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:29 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:29 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:29 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:29 DEBUG opendrift.models.basemodel:755: Lifting 77 elements to seafloor.
12:54:29 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:29 INFO opendrift.models.basemodel:2038: 2024-11-02 01:24:02.760182 - step 26 of 96 - 500 active elements (0 deactivated)
12:54:29 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:29 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:29 DEBUG opendrift.models.basemodel:2057: 57.55613149836466 <- latitude -> 57.696897572963266
12:54:29 DEBUG opendrift.models.basemodel:2062: 10.507927244297793 <- longitude -> 10.729334746669291
12:54:29 DEBUG opendrift.models.basemodel:2067: -20.428923511790632 <- z -> -0.03617489761685033
12:54:29 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:29 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:29 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:29 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:29 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:29 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:29 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:29 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:29 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-02 01:00:00 (before)
2024-11-02 02:00:00 (after)
12:54:31 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
12:54:31 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:54:31 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:54:31 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:54:31 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:54:31 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'upward_sea_water_velocity', 'y_sea_water_velocity', 'sea_water_temperature', 'sea_water_salinity']
12:54:31 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 46x33x7) for time after (2024-11-02 02:00:00)
12:54:31 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-02 01:00:00) in space (linearNDFast)
12:54:31 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:31 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-02 02:00:00) in space (linearNDFast)
12:54:31 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:31 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-02 01:00:00, weight 0.60) and
after (2024-11-02 02:00:00, weight 0.40) in time
12:54:31 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:31 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.27067630226131 degrees.
12:54:31 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.27067630226131 degrees.
12:54:31 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:31 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:31 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:31 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:31 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:31 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:31 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:31 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:31 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:31 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:31 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:31 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:31 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:31 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:31 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:31 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:31 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:31 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:31 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:31 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:31 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:31 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:31 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:31 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:31 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:31 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:31 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:31 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:31 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:31 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0697512 (min) 0.0998458 (max)
12:54:31 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.07694 (min) 0.0997487 (max)
12:54:31 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.0579733 (min) -0.00225112 (max)
12:54:31 DEBUG opendrift.models.basemodel.environment:905: x_wind: 2.63073 (min) 3.14551 (max)
12:54:31 DEBUG opendrift.models.basemodel.environment:905: y_wind: -8.33842 (min) -7.19455 (max)
12:54:31 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:31 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 23.1742 (max)
12:54:31 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:31 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.3903 (min) 12.7932 (max)
12:54:31 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0595 (min) 32.3991 (max)
12:54:31 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -4.13681e-05 (min) 0.000167488 (max)
12:54:31 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:31 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:31 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:31 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:31 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:31 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:31 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:31 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:31 DEBUG opendrift.models.basemodel:755: Lifting 77 elements to seafloor.
12:54:31 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:31 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:31 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:31 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:31 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 12
12:54:31 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 0 0 2 0 2 0 0 0 2]
12:54:31 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 3 3 0 3 0 3 3 3 0]
12:54:31 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 350. 0.]
[ 0. 0. 0. 0. 0.]
[ 52. 0. 0. 156. 0.]
[ 2. 0. 418. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:31 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:31 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:31 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:31 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:31 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.057051929982269246
12:54:31 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:31 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:31 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:31 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:636: 87 elements reached seafloor, interacting with bottom
12:54:31 DEBUG opendrift.models.basemodel:755: Lifting 87 elements to seafloor.
12:54:31 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:636: 87 elements reached seafloor, interacting with bottom
12:54:31 DEBUG opendrift.models.basemodel:755: Lifting 87 elements to seafloor.
12:54:31 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:636: 88 elements reached seafloor, interacting with bottom
12:54:31 DEBUG opendrift.models.basemodel:755: Lifting 88 elements to seafloor.
12:54:31 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:636: 88 elements reached seafloor, interacting with bottom
12:54:31 DEBUG opendrift.models.basemodel:755: Lifting 88 elements to seafloor.
12:54:31 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:636: 88 elements reached seafloor, interacting with bottom
12:54:31 DEBUG opendrift.models.basemodel:755: Lifting 88 elements to seafloor.
12:54:31 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:636: 89 elements reached seafloor, interacting with bottom
12:54:31 DEBUG opendrift.models.basemodel:755: Lifting 89 elements to seafloor.
12:54:31 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:636: 89 elements reached seafloor, interacting with bottom
12:54:31 DEBUG opendrift.models.basemodel:755: Lifting 89 elements to seafloor.
12:54:31 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:636: 89 elements reached seafloor, interacting with bottom
12:54:31 DEBUG opendrift.models.basemodel:755: Lifting 89 elements to seafloor.
12:54:31 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:636: 89 elements reached seafloor, interacting with bottom
12:54:31 DEBUG opendrift.models.basemodel:755: Lifting 89 elements to seafloor.
12:54:31 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:636: 90 elements reached seafloor, interacting with bottom
12:54:31 DEBUG opendrift.models.basemodel:755: Lifting 90 elements to seafloor.
12:54:31 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:636: 90 elements reached seafloor, interacting with bottom
12:54:31 DEBUG opendrift.models.basemodel:755: Lifting 90 elements to seafloor.
12:54:31 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:636: 91 elements reached seafloor, interacting with bottom
12:54:31 DEBUG opendrift.models.basemodel:755: Lifting 91 elements to seafloor.
12:54:31 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:636: 91 elements reached seafloor, interacting with bottom
12:54:31 DEBUG opendrift.models.basemodel:755: Lifting 91 elements to seafloor.
12:54:31 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:636: 91 elements reached seafloor, interacting with bottom
12:54:31 DEBUG opendrift.models.basemodel:755: Lifting 91 elements to seafloor.
12:54:31 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:636: 91 elements reached seafloor, interacting with bottom
12:54:31 DEBUG opendrift.models.basemodel:755: Lifting 91 elements to seafloor.
12:54:31 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:636: 92 elements reached seafloor, interacting with bottom
12:54:31 DEBUG opendrift.models.basemodel:755: Lifting 92 elements to seafloor.
12:54:31 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:636: 92 elements reached seafloor, interacting with bottom
12:54:31 DEBUG opendrift.models.basemodel:755: Lifting 92 elements to seafloor.
12:54:31 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:636: 93 elements reached seafloor, interacting with bottom
12:54:31 DEBUG opendrift.models.basemodel:755: Lifting 93 elements to seafloor.
12:54:31 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:636: 93 elements reached seafloor, interacting with bottom
12:54:31 DEBUG opendrift.models.basemodel:755: Lifting 93 elements to seafloor.
12:54:31 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:636: 93 elements reached seafloor, interacting with bottom
12:54:31 DEBUG opendrift.models.basemodel:755: Lifting 93 elements to seafloor.
12:54:31 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:636: 93 elements reached seafloor, interacting with bottom
12:54:31 DEBUG opendrift.models.basemodel:755: Lifting 93 elements to seafloor.
12:54:31 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:636: 93 elements reached seafloor, interacting with bottom
12:54:31 DEBUG opendrift.models.basemodel:755: Lifting 93 elements to seafloor.
12:54:31 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:636: 94 elements reached seafloor, interacting with bottom
12:54:31 DEBUG opendrift.models.basemodel:755: Lifting 94 elements to seafloor.
12:54:31 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:636: 94 elements reached seafloor, interacting with bottom
12:54:31 DEBUG opendrift.models.basemodel:755: Lifting 94 elements to seafloor.
12:54:31 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:636: 95 elements reached seafloor, interacting with bottom
12:54:31 DEBUG opendrift.models.basemodel:755: Lifting 95 elements to seafloor.
12:54:31 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:636: 95 elements reached seafloor, interacting with bottom
12:54:31 DEBUG opendrift.models.basemodel:755: Lifting 95 elements to seafloor.
12:54:31 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:636: 95 elements reached seafloor, interacting with bottom
12:54:31 DEBUG opendrift.models.basemodel:755: Lifting 95 elements to seafloor.
12:54:31 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:636: 96 elements reached seafloor, interacting with bottom
12:54:31 DEBUG opendrift.models.basemodel:755: Lifting 96 elements to seafloor.
12:54:31 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:636: 96 elements reached seafloor, interacting with bottom
12:54:31 DEBUG opendrift.models.basemodel:755: Lifting 96 elements to seafloor.
12:54:31 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:636: 96 elements reached seafloor, interacting with bottom
12:54:31 DEBUG opendrift.models.basemodel:755: Lifting 96 elements to seafloor.
12:54:31 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 0
12:54:31 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:31 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:31 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:31 INFO opendrift.models.chemicaldrift:1861: partitioning: [153, 0, 251, 96, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:31 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:31 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:31 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:31 DEBUG opendrift.models.basemodel:755: Lifting 96 elements to seafloor.
12:54:31 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:31 INFO opendrift.models.basemodel:2038: 2024-11-02 01:54:02.760182 - step 27 of 96 - 500 active elements (0 deactivated)
12:54:31 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:31 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:31 DEBUG opendrift.models.basemodel:2057: 57.55521267063158 <- latitude -> 57.695958487514005
12:54:31 DEBUG opendrift.models.basemodel:2062: 10.507927244297788 <- longitude -> 10.731878812516857
12:54:31 DEBUG opendrift.models.basemodel:2067: -22.45853837590581 <- z -> 0.0
12:54:31 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:31 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:31 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:31 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:31 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:31 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:31 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:31 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:31 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-02 01:00:00 (before)
2024-11-02 02:00:00 (after)
12:54:31 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-02 01:00:00) in space (linearNDFast)
12:54:31 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:31 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-02 02:00:00) in space (linearNDFast)
12:54:31 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:31 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-02 01:00:00, weight 0.10) and
after (2024-11-02 02:00:00, weight 0.90) in time
12:54:31 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:31 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.268132223706104 degrees.
12:54:31 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.268132223706104 degrees.
12:54:31 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:31 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:31 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:31 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:31 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:31 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:31 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:31 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:31 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:31 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:31 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:31 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:31 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:31 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:31 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:31 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:31 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:31 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:31 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:31 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:31 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:31 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:31 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:31 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:31 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:31 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:31 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:31 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:31 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:31 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0611782 (min) 0.0881958 (max)
12:54:31 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.135962 (min) 0.0613539 (max)
12:54:31 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.036436 (min) 0.0546415 (max)
12:54:31 DEBUG opendrift.models.basemodel.environment:905: x_wind: 2.38742 (min) 2.93132 (max)
12:54:31 DEBUG opendrift.models.basemodel.environment:905: y_wind: -8.18685 (min) -6.93994 (max)
12:54:31 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:31 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 23.3056 (max)
12:54:31 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:31 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.373 (min) 12.768 (max)
12:54:31 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0553 (min) 32.4017 (max)
12:54:31 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -2.61392e-05 (min) 0.000163974 (max)
12:54:31 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:31 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:31 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:31 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:31 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:31 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:31 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:31 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:31 DEBUG opendrift.models.basemodel:750: No elements hit seafloor.
12:54:31 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:31 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:31 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:31 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:31 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 16
12:54:31 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 0 0 0 2 0 0 2 0 2 0 2 0 0]
12:54:31 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 3 3 3 0 3 3 0 3 0 3 0 3 3]
12:54:31 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 362. 0.]
[ 0. 0. 0. 0. 0.]
[ 56. 0. 0. 166. 0.]
[ 2. 0. 418. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:31 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:31 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:31 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:31 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:31 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.054342501427355416
12:54:31 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:31 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:31 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:31 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:31 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:31 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:31 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:31 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:31 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:31 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:31 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:31 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:618: 32 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:618: 32 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:54:31 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:31 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:31 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:54:31 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 0
12:54:31 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:31 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:31 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:31 INFO opendrift.models.chemicaldrift:1861: partitioning: [145, 0, 247, 108, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:31 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:31 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:31 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:31 DEBUG opendrift.models.basemodel:750: No elements hit seafloor.
12:54:31 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:31 INFO opendrift.models.basemodel:2038: 2024-11-02 02:24:02.760182 - step 28 of 96 - 500 active elements (0 deactivated)
12:54:31 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:31 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:31 DEBUG opendrift.models.basemodel:2057: 57.55316255960143 <- latitude -> 57.6937610614929
12:54:31 DEBUG opendrift.models.basemodel:2062: 10.507927244297784 <- longitude -> 10.73432633078667
12:54:31 DEBUG opendrift.models.basemodel:2067: -23.305593490600586 <- z -> 0.0
12:54:31 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:31 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:31 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:31 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:31 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:31 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:31 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:31 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:31 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-02 02:00:00 (before)
2024-11-02 03:00:00 (after)
12:54:32 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
12:54:32 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:54:32 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:54:32 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:54:32 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:54:32 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'upward_sea_water_velocity', 'y_sea_water_velocity', 'sea_water_temperature', 'sea_water_salinity']
12:54:32 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 46x34x7) for time after (2024-11-02 03:00:00)
12:54:32 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-02 02:00:00) in space (linearNDFast)
12:54:32 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:32 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-02 03:00:00) in space (linearNDFast)
12:54:32 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:32 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-02 02:00:00, weight 0.60) and
after (2024-11-02 03:00:00, weight 0.40) in time
12:54:32 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:32 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.265684711726685 degrees.
12:54:32 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.265684711726685 degrees.
12:54:32 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:32 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:32 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:32 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:32 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:32 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:32 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:32 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:32 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:32 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:32 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:32 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:32 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:32 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:32 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:32 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:32 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:32 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:32 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:32 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:32 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:32 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:32 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:32 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:32 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:32 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:32 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:32 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:32 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:32 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0581469 (min) 0.0818758 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.10842 (min) 0.0667281 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.0747757 (min) 0.0887503 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:905: x_wind: 2.11663 (min) 2.7434 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:905: y_wind: -8.05935 (min) -6.82701 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 23.3056 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.3516 (min) 12.7521 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.054 (min) 32.4 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -1.71068e-05 (min) 0.000162784 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:32 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:32 DEBUG opendrift.models.basemodel:750: No elements hit seafloor.
12:54:32 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:32 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:32 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:32 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:32 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 6
12:54:32 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 2 2 0]
12:54:32 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 0 0 3]
12:54:32 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 366. 0.]
[ 0. 0. 0. 0. 0.]
[ 58. 0. 0. 166. 0.]
[ 2. 0. 418. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:32 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:32 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:32 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:32 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:32 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.052211623092402855
12:54:32 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:32 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:32 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:32 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:32 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:32 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:32 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:32 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:32 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:32 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
12:54:32 DEBUG opendrift.models.basemodel:755: Lifting 2 elements to seafloor.
12:54:32 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:32 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:32 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:32 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:32 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:32 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:32 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:32 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:32 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:32 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:32 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:636: 3 elements reached seafloor, interacting with bottom
12:54:32 DEBUG opendrift.models.basemodel:755: Lifting 3 elements to seafloor.
12:54:32 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:54:32 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 0
12:54:32 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:32 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:32 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:32 INFO opendrift.models.chemicaldrift:1861: partitioning: [143, 0, 245, 112, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:32 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:32 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:32 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:32 DEBUG opendrift.models.basemodel:750: No elements hit seafloor.
12:54:32 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:32 INFO opendrift.models.basemodel:2038: 2024-11-02 02:54:02.760182 - step 29 of 96 - 500 active elements (0 deactivated)
12:54:32 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:32 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:32 DEBUG opendrift.models.basemodel:2057: 57.55141022659644 <- latitude -> 57.69227692496304
12:54:32 DEBUG opendrift.models.basemodel:2062: 10.507927244297788 <- longitude -> 10.736794314133244
12:54:32 DEBUG opendrift.models.basemodel:2067: -23.305593490600586 <- z -> -0.09479595608545405
12:54:32 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:32 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:32 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:32 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:32 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:32 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:32 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:32 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:32 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-02 02:00:00 (before)
2024-11-02 03:00:00 (after)
12:54:32 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-02 02:00:00) in space (linearNDFast)
12:54:32 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:32 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-02 03:00:00) in space (linearNDFast)
12:54:32 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:32 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-02 02:00:00, weight 0.10) and
after (2024-11-02 03:00:00, weight 0.90) in time
12:54:32 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:32 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.26321672946642 degrees.
12:54:32 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.26321672946642 degrees.
12:54:32 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:32 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:32 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:32 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:32 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:32 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:32 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:32 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:32 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:32 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:32 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:32 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:32 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:32 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:32 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:32 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:32 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:32 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:32 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:32 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:32 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:32 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:32 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:32 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:32 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:32 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:32 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:32 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:32 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:32 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.05213 (min) 0.0791932 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.132475 (min) 0.0695367 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.0933529 (min) 0.124851 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.83904 (min) 2.60891 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:905: y_wind: -7.97086 (min) -6.74915 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 23.3439 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.3293 (min) 12.7537 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0533 (min) 32.4002 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -1.80345e-05 (min) 0.000171223 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:32 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:32 DEBUG opendrift.models.basemodel:750: No elements hit seafloor.
12:54:32 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:32 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:32 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:32 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:32 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 9
12:54:32 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 2 0 0 0 0 2 0 2]
12:54:32 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 0 3 3 3 3 0 3 0]
12:54:32 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 371. 0.]
[ 0. 0. 0. 0. 0.]
[ 62. 0. 0. 166. 0.]
[ 2. 0. 418. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:32 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:32 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:32 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:32 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:32 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.05077028197513493
12:54:32 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:32 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:32 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:32 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:32 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:32 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:636: 3 elements reached seafloor, interacting with bottom
12:54:32 DEBUG opendrift.models.basemodel:755: Lifting 3 elements to seafloor.
12:54:32 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:32 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:32 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:32 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:32 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:32 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:32 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:32 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:32 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:54:32 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:32 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:32 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 0
12:54:32 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:32 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:32 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:32 INFO opendrift.models.chemicaldrift:1861: partitioning: [142, 0, 241, 117, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:32 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:32 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:32 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:32 DEBUG opendrift.models.basemodel:750: No elements hit seafloor.
12:54:32 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:32 INFO opendrift.models.basemodel:2038: 2024-11-02 03:24:02.760182 - step 30 of 96 - 500 active elements (0 deactivated)
12:54:32 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:32 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:32 DEBUG opendrift.models.basemodel:2057: 57.54926911215627 <- latitude -> 57.69149884712332
12:54:32 DEBUG opendrift.models.basemodel:2062: 10.507927244297784 <- longitude -> 10.739181318845493
12:54:32 DEBUG opendrift.models.basemodel:2067: -23.343875885009766 <- z -> -0.016791146279787145
12:54:32 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:32 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:32 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:32 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:32 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:32 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:32 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:32 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:32 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-02 03:00:00 (before)
2024-11-02 04:00:00 (after)
12:54:34 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
12:54:34 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:54:34 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:54:34 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:54:34 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:54:34 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'upward_sea_water_velocity', 'y_sea_water_velocity', 'sea_water_temperature', 'sea_water_salinity']
12:54:34 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 47x34x7) for time after (2024-11-02 04:00:00)
12:54:34 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-02 03:00:00) in space (linearNDFast)
12:54:34 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:34 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-02 04:00:00) in space (linearNDFast)
12:54:34 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:34 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-02 03:00:00, weight 0.60) and
after (2024-11-02 04:00:00, weight 0.40) in time
12:54:34 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:34 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.26082972703545 degrees.
12:54:34 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.26082972703545 degrees.
12:54:34 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:34 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:34 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:34 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:34 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:34 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:34 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:34 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:34 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:34 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:34 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:34 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:34 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:34 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:34 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:34 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:34 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:34 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:34 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:34 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:34 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:34 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:34 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:34 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:34 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:34 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:34 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:34 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:34 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:34 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0358606 (min) 0.0643145 (max)
12:54:34 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.118243 (min) 0.0753503 (max)
12:54:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.0873241 (min) 0.123817 (max)
12:54:34 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.46418 (min) 2.24726 (max)
12:54:34 DEBUG opendrift.models.basemodel.environment:905: y_wind: -7.60687 (min) -6.35719 (max)
12:54:34 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:34 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 23.3523 (max)
12:54:34 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:34 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.3086 (min) 12.7169 (max)
12:54:34 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0528 (min) 32.4018 (max)
12:54:34 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -1.87741e-05 (min) 0.000147069 (max)
12:54:34 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:34 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:34 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:34 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:34 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:34 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:34 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:34 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:34 DEBUG opendrift.models.basemodel:750: No elements hit seafloor.
12:54:34 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:34 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:34 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:34 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:34 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 9
12:54:34 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 2 2 2 0 0 2]
12:54:34 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 0 0 0 3 3 0]
12:54:34 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 376. 0.]
[ 0. 0. 0. 0. 0.]
[ 66. 0. 0. 166. 0.]
[ 2. 0. 418. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:34 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:34 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:34 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:34 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:34 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.04545433417588522
12:54:34 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:34 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:34 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:34 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:34 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:34 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:34 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:34 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:34 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:34 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:34 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:34 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 0
12:54:34 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:34 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:34 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:34 INFO opendrift.models.chemicaldrift:1861: partitioning: [141, 0, 237, 122, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:34 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:34 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:34 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:34 DEBUG opendrift.models.basemodel:750: No elements hit seafloor.
12:54:34 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:34 INFO opendrift.models.basemodel:2038: 2024-11-02 03:54:02.760182 - step 31 of 96 - 500 active elements (0 deactivated)
12:54:34 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:34 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:34 DEBUG opendrift.models.basemodel:2057: 57.54735802132532 <- latitude -> 57.69117243125497
12:54:34 DEBUG opendrift.models.basemodel:2062: 10.507927244297786 <- longitude -> 10.740989335892005
12:54:34 DEBUG opendrift.models.basemodel:2067: -23.343875885009766 <- z -> -0.12683121928626861
12:54:34 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:34 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:34 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:34 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:34 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:34 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:34 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:34 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:34 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-02 03:00:00 (before)
2024-11-02 04:00:00 (after)
12:54:34 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-02 03:00:00) in space (linearNDFast)
12:54:34 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:34 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-02 04:00:00) in space (linearNDFast)
12:54:34 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:34 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-02 03:00:00, weight 0.10) and
after (2024-11-02 04:00:00, weight 0.90) in time
12:54:34 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:34 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.25902170573533 degrees.
12:54:34 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.25902170573533 degrees.
12:54:34 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:34 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:34 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:34 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:34 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:34 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:34 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:34 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:34 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:34 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:34 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:34 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:34 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:34 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:34 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:34 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:34 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:34 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:34 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:34 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:34 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:34 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:34 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:34 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:34 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:34 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:34 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:34 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:34 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:34 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0204524 (min) 0.0557239 (max)
12:54:34 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.087249 (min) 0.095071 (max)
12:54:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.0753525 (min) 0.11316 (max)
12:54:34 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.06524 (min) 1.84431 (max)
12:54:34 DEBUG opendrift.models.basemodel.environment:905: y_wind: -7.21131 (min) -5.88744 (max)
12:54:34 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:34 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 23.4282 (max)
12:54:34 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:34 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.2883 (min) 12.6977 (max)
12:54:34 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0523 (min) 32.4009 (max)
12:54:34 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -2.02994e-05 (min) 0.000108862 (max)
12:54:34 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:34 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:34 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:34 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:34 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:34 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:34 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:34 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:34 DEBUG opendrift.models.basemodel:750: No elements hit seafloor.
12:54:34 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:34 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:34 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:34 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:34 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 3
12:54:34 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 0 0]
12:54:34 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 3 3]
12:54:34 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 378. 0.]
[ 0. 0. 0. 0. 0.]
[ 67. 0. 0. 166. 0.]
[ 2. 0. 418. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:34 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:34 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:34 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:34 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:34 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.04003932879537386
12:54:34 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:34 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:34 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:34 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
12:54:34 DEBUG opendrift.models.basemodel:755: Lifting 2 elements to seafloor.
12:54:34 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:34 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:34 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
12:54:34 DEBUG opendrift.models.basemodel:755: Lifting 2 elements to seafloor.
12:54:34 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:34 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:34 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
12:54:34 DEBUG opendrift.models.basemodel:755: Lifting 2 elements to seafloor.
12:54:34 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
12:54:34 DEBUG opendrift.models.basemodel:755: Lifting 2 elements to seafloor.
12:54:34 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:34 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:34 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:34 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:34 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:34 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:34 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
12:54:34 DEBUG opendrift.models.basemodel:755: Lifting 2 elements to seafloor.
12:54:34 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:34 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:34 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:34 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:34 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 0
12:54:34 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:34 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:34 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:34 INFO opendrift.models.chemicaldrift:1861: partitioning: [140, 0, 236, 124, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:34 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:34 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:34 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:34 DEBUG opendrift.models.basemodel:750: No elements hit seafloor.
12:54:34 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:34 INFO opendrift.models.basemodel:2038: 2024-11-02 04:24:02.760182 - step 32 of 96 - 500 active elements (0 deactivated)
12:54:34 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:34 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:34 DEBUG opendrift.models.basemodel:2057: 57.545947867446465 <- latitude -> 57.69178178418153
12:54:34 DEBUG opendrift.models.basemodel:2062: 10.507927244297786 <- longitude -> 10.742547131503079
12:54:34 DEBUG opendrift.models.basemodel:2067: -23.343875885009766 <- z -> -0.22549948823350752
12:54:34 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:34 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:34 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:34 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:34 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:34 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:34 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:34 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:34 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-02 04:00:00 (before)
2024-11-02 05:00:00 (after)
12:54:35 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
12:54:35 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:54:35 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:54:35 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:54:35 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:54:35 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'upward_sea_water_velocity', 'y_sea_water_velocity', 'sea_water_temperature', 'sea_water_salinity']
12:54:35 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 47x34x7) for time after (2024-11-02 05:00:00)
12:54:35 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-02 04:00:00) in space (linearNDFast)
12:54:35 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:35 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-02 05:00:00) in space (linearNDFast)
12:54:35 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:35 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-02 04:00:00, weight 0.60) and
after (2024-11-02 05:00:00, weight 0.40) in time
12:54:35 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:35 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.25746391390637 degrees.
12:54:35 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.25746391390637 degrees.
12:54:35 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:35 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:35 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:35 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:35 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:35 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:35 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:35 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:35 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:35 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:35 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:35 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:35 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:35 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:35 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:35 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:35 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:35 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:35 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:35 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:35 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:35 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:35 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:35 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:35 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:35 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:35 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:35 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:35 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:35 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0153298 (min) 0.0622211 (max)
12:54:35 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0566553 (min) 0.126865 (max)
12:54:35 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.0434745 (min) 0.0787651 (max)
12:54:35 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.16545 (min) 2.05457 (max)
12:54:35 DEBUG opendrift.models.basemodel.environment:905: y_wind: -6.82264 (min) -5.36165 (max)
12:54:35 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:35 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 23.4936 (max)
12:54:35 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:35 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.2703 (min) 12.6902 (max)
12:54:35 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0512 (min) 32.4001 (max)
12:54:35 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -3.0452e-05 (min) 8.99488e-05 (max)
12:54:35 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:35 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:35 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:35 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:35 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:35 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:35 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:35 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:35 DEBUG opendrift.models.basemodel:750: No elements hit seafloor.
12:54:35 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:35 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:35 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:35 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:35 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 8
12:54:35 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 2 0 0 0 0 0 2]
12:54:35 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 0 3 3 3 3 3 0]
12:54:35 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 384. 0.]
[ 0. 0. 0. 0. 0.]
[ 69. 0. 0. 166. 0.]
[ 2. 0. 418. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:35 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:35 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:35 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:35 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:35 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.036648949095825084
12:54:35 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:35 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:35 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:35 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:35 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:35 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:35 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:35 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:35 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:35 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:35 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:35 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:35 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:35 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
12:54:35 DEBUG opendrift.models.basemodel:755: Lifting 2 elements to seafloor.
12:54:35 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:35 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:35 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:35 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:35 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:35 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:35 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:35 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:35 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 0
12:54:35 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:35 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:35 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:35 INFO opendrift.models.chemicaldrift:1861: partitioning: [136, 0, 234, 130, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:35 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:35 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:35 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:35 DEBUG opendrift.models.basemodel:750: No elements hit seafloor.
12:54:35 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:35 INFO opendrift.models.basemodel:2038: 2024-11-02 04:54:02.760182 - step 33 of 96 - 500 active elements (0 deactivated)
12:54:35 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:35 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:35 DEBUG opendrift.models.basemodel:2057: 57.5450321820403 <- latitude -> 57.692591076088796
12:54:35 DEBUG opendrift.models.basemodel:2062: 10.507927244297786 <- longitude -> 10.743930933456019
12:54:35 DEBUG opendrift.models.basemodel:2067: -23.343875885009766 <- z -> -0.051379484224083685
12:54:35 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:35 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:35 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:35 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:35 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:35 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:35 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:35 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:35 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-02 04:00:00 (before)
2024-11-02 05:00:00 (after)
12:54:35 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-02 04:00:00) in space (linearNDFast)
12:54:35 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:35 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-02 05:00:00) in space (linearNDFast)
12:54:35 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:35 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-02 04:00:00, weight 0.10) and
after (2024-11-02 05:00:00, weight 0.90) in time
12:54:35 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:35 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.25608011320063 degrees.
12:54:35 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.25608011320063 degrees.
12:54:35 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:35 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:35 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:35 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:35 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:35 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:35 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:35 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:35 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:35 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:35 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:35 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:35 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:35 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:35 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:35 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:35 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:35 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:35 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:35 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:35 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:35 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:35 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:35 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:35 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:35 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:35 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:35 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:35 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:35 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0104792 (min) 0.0744281 (max)
12:54:35 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0364853 (min) 0.156104 (max)
12:54:35 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.00497208 (min) 0.0385173 (max)
12:54:35 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.38927 (min) 2.42574 (max)
12:54:35 DEBUG opendrift.models.basemodel.environment:905: y_wind: -6.44203 (min) -4.82197 (max)
12:54:35 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:35 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 23.5371 (max)
12:54:35 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:35 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.253 (min) 12.6808 (max)
12:54:35 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0498 (min) 32.4019 (max)
12:54:35 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -4.16895e-05 (min) 7.01545e-05 (max)
12:54:35 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:35 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:35 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:35 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:35 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:35 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:35 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:35 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:35 DEBUG opendrift.models.basemodel:755: Lifting 2 elements to seafloor.
12:54:35 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:35 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:35 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:35 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:35 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 11
12:54:35 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 2 2 0 0 0 0 0 0 2]
12:54:35 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 0 0 3 3 3 3 3 3 0]
12:54:35 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 392. 0.]
[ 0. 0. 0. 0. 0.]
[ 72. 0. 0. 166. 0.]
[ 2. 0. 418. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:35 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:35 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:35 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:35 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:35 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.034083390221358686
12:54:35 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:35 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:35 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:35 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:636: 4 elements reached seafloor, interacting with bottom
12:54:35 DEBUG opendrift.models.basemodel:755: Lifting 4 elements to seafloor.
12:54:35 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:35 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:35 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:35 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:35 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:35 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:35 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:35 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:54:35 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 17
12:54:35 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:35 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 16 elements
12:54:35 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:35 INFO opendrift.models.chemicaldrift:1861: partitioning: [131, 0, 247, 122, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:35 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:35 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:35 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:35 DEBUG opendrift.models.basemodel:750: No elements hit seafloor.
12:54:35 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:35 INFO opendrift.models.basemodel:2038: 2024-11-02 05:24:02.760182 - step 34 of 96 - 500 active elements (0 deactivated)
12:54:35 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:35 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:35 DEBUG opendrift.models.basemodel:2057: 57.54444249167825 <- latitude -> 57.69479966684548
12:54:35 DEBUG opendrift.models.basemodel:2062: 10.507927244297786 <- longitude -> 10.745282885264173
12:54:35 DEBUG opendrift.models.basemodel:2067: -23.343875885009766 <- z -> -0.10644638061975953
12:54:35 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:35 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:35 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:35 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:35 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:35 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:35 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:35 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:35 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-02 05:00:00 (before)
2024-11-02 06:00:00 (after)
12:54:37 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
12:54:37 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:54:37 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:54:37 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:54:37 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:54:37 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'upward_sea_water_velocity', 'y_sea_water_velocity', 'sea_water_temperature', 'sea_water_salinity']
12:54:37 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 47x34x7) for time after (2024-11-02 06:00:00)
12:54:37 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-02 05:00:00) in space (linearNDFast)
12:54:37 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:37 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-02 06:00:00) in space (linearNDFast)
12:54:37 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:37 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-02 05:00:00, weight 0.60) and
after (2024-11-02 06:00:00, weight 0.40) in time
12:54:37 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:37 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.254728160578885 degrees.
12:54:37 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.254728160578885 degrees.
12:54:37 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:37 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:37 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:37 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:37 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:37 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:37 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:37 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:37 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:37 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:37 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:37 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:37 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:37 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:37 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:37 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:37 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:37 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:37 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:37 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:37 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:37 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:37 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:37 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:37 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:37 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:37 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:37 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:37 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:37 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0111082 (min) 0.0838884 (max)
12:54:37 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.039481 (min) 0.17885 (max)
12:54:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.0359195 (min) -0.00770549 (max)
12:54:37 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.56035 (min) 2.34323 (max)
12:54:37 DEBUG opendrift.models.basemodel.environment:905: y_wind: -6.02711 (min) -4.32714 (max)
12:54:37 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:37 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 23.5735 (max)
12:54:37 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:37 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.2375 (min) 12.6784 (max)
12:54:37 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.047 (min) 32.4042 (max)
12:54:37 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -4.35991e-05 (min) 6.76376e-05 (max)
12:54:37 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:37 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:37 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:37 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:37 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:37 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:37 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:37 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 122 elements to seafloor.
12:54:37 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:37 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:37 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:37 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:37 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 5
12:54:37 DEBUG opendrift.models.chemicaldrift:1452: old species: [3 0 0 2 2]
12:54:37 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 3 3 0 0]
12:54:37 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 394. 0.]
[ 0. 0. 0. 0. 0.]
[ 74. 0. 0. 166. 0.]
[ 3. 0. 434. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:37 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:37 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:37 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:37 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:37 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.030067995948474043
12:54:37 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:37 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:37 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:37 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 123 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 123 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 124 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 124 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 124 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 124 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 125 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 125 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 125 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 125 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 126 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 126 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 127 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 127 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 127 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 127 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 128 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 128 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 130 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 130 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 130 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 130 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 130 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 130 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 131 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 131 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 131 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 131 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 131 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 131 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 131 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 131 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 131 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 131 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 131 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 131 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 131 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 131 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 131 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 131 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 131 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 131 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 132 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 132 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 132 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 132 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 133 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 133 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 133 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 133 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 133 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 133 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 134 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 134 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 135 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 135 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 135 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 135 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 136 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 136 elements to seafloor.
12:54:37 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 41
12:54:37 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:37 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 41 elements
12:54:37 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:37 INFO opendrift.models.chemicaldrift:1861: partitioning: [132, 0, 273, 95, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:37 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:37 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:37 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 99 elements to seafloor.
12:54:37 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:37 INFO opendrift.models.basemodel:2038: 2024-11-02 05:54:02.760182 - step 35 of 96 - 500 active elements (0 deactivated)
12:54:37 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:37 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:37 DEBUG opendrift.models.basemodel:2057: 57.54380438276804 <- latitude -> 57.69754290828132
12:54:37 DEBUG opendrift.models.basemodel:2062: 10.50792724429779 <- longitude -> 10.746922746167112
12:54:37 DEBUG opendrift.models.basemodel:2067: -23.343873977661133 <- z -> -0.08955944445797126
12:54:37 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:37 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:37 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:37 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:37 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:37 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:37 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:37 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:37 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-02 05:00:00 (before)
2024-11-02 06:00:00 (after)
12:54:37 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-02 05:00:00) in space (linearNDFast)
12:54:37 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:37 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-02 06:00:00) in space (linearNDFast)
12:54:37 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:37 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-02 05:00:00, weight 0.10) and
after (2024-11-02 06:00:00, weight 0.90) in time
12:54:37 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:37 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.25308829809036 degrees.
12:54:37 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.25308829809036 degrees.
12:54:37 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:37 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:37 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:37 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:37 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:37 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:37 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:37 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:37 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:37 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:37 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:37 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:37 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:37 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:37 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:37 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:37 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:37 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:37 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:37 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:37 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:37 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:37 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:37 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:37 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:37 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:37 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:37 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:37 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:37 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.013094 (min) 0.0950682 (max)
12:54:37 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.000311567 (min) 0.200836 (max)
12:54:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.0776646 (min) -0.0505911 (max)
12:54:37 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.71837 (min) 2.17267 (max)
12:54:37 DEBUG opendrift.models.basemodel.environment:905: y_wind: -5.60806 (min) -3.84343 (max)
12:54:37 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:37 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 23.6282 (max)
12:54:37 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:37 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.2225 (min) 12.6653 (max)
12:54:37 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0438 (min) 32.4116 (max)
12:54:37 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -4.31989e-05 (min) 6.5086e-05 (max)
12:54:37 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:37 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:37 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:37 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:37 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:37 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:37 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:37 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 99 elements to seafloor.
12:54:37 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:37 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:37 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:37 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:37 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 9
12:54:37 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 2 0 0 2 0 0]
12:54:37 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 0 3 3 0 3 3]
12:54:37 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 401. 0.]
[ 0. 0. 0. 0. 0.]
[ 76. 0. 0. 179. 0.]
[ 3. 0. 475. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:37 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:37 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:37 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:37 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:37 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.025934781477048714
12:54:37 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:37 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:37 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:37 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 102 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 102 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 102 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 102 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 102 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 102 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 102 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 102 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 103 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 103 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 103 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 103 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 103 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 103 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 103 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 103 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 103 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 103 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 103 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 103 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 103 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 103 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 103 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 103 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 104 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 104 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 104 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 104 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 104 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 104 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 104 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 104 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 104 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 104 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 104 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 104 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 104 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 104 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 105 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 105 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 106 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 106 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 106 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 106 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 106 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 106 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 106 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 106 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 106 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 106 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 107 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 107 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 107 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 107 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 109 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 109 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 111 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 111 elements to seafloor.
12:54:37 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:37 DEBUG opendrift.models.oceandrift:636: 111 elements reached seafloor, interacting with bottom
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 111 elements to seafloor.
12:54:37 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 31
12:54:37 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:37 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 31 elements
12:54:37 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:37 INFO opendrift.models.chemicaldrift:1861: partitioning: [127, 0, 293, 80, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:37 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:37 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:37 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:37 DEBUG opendrift.models.basemodel:755: Lifting 82 elements to seafloor.
12:54:37 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:37 INFO opendrift.models.basemodel:2038: 2024-11-02 06:24:02.760182 - step 36 of 96 - 500 active elements (0 deactivated)
12:54:37 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:37 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:37 DEBUG opendrift.models.basemodel:2057: 57.543809418419805 <- latitude -> 57.70077887938966
12:54:37 DEBUG opendrift.models.basemodel:2062: 10.507927244297786 <- longitude -> 10.74875655871666
12:54:37 DEBUG opendrift.models.basemodel:2067: -21.799676808925888 <- z -> -0.19828932828500298
12:54:37 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:37 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:37 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:37 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:37 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:37 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:37 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:37 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:37 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-02 06:00:00 (before)
2024-11-02 07:00:00 (after)
12:54:39 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
12:54:39 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:54:39 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:54:39 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:54:39 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:54:39 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'upward_sea_water_velocity', 'y_sea_water_velocity', 'sea_water_temperature', 'sea_water_salinity']
12:54:39 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 48x34x7) for time after (2024-11-02 07:00:00)
12:54:39 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-02 06:00:00) in space (linearNDFast)
12:54:39 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:39 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-02 07:00:00) in space (linearNDFast)
12:54:39 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:39 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-02 06:00:00, weight 0.60) and
after (2024-11-02 07:00:00, weight 0.40) in time
12:54:39 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:39 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.25125448156575 degrees.
12:54:39 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.25125448156575 degrees.
12:54:39 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:39 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:39 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:39 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:39 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:39 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:39 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:39 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:39 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:39 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:39 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:39 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:39 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:39 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:39 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:39 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:39 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:39 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:39 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:39 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:39 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:39 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:39 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:39 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:39 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:39 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:39 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:39 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:39 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:39 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0137187 (min) 0.108299 (max)
12:54:39 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0165457 (min) 0.213439 (max)
12:54:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.119182 (min) -0.0943171 (max)
12:54:39 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.56372 (min) 2.03168 (max)
12:54:39 DEBUG opendrift.models.basemodel.environment:905: y_wind: -5.15962 (min) -3.55335 (max)
12:54:39 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:39 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 23.7499 (max)
12:54:39 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:39 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.2115 (min) 12.6414 (max)
12:54:39 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0401 (min) 32.4179 (max)
12:54:39 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -4.65182e-05 (min) 5.771e-05 (max)
12:54:39 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:39 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:39 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:39 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:39 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:39 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:39 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:39 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 82 elements to seafloor.
12:54:39 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:39 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:39 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:39 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:39 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 12
12:54:39 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 2 0 0 0 0 2 0 0 0 0]
12:54:39 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 0 3 3 3 3 0 3 3 3 3]
12:54:39 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 411. 0.]
[ 0. 0. 0. 0. 0.]
[ 78. 0. 0. 188. 0.]
[ 3. 0. 506. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:39 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:39 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:39 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:39 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:39 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.02184352119932653
12:54:39 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:39 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:39 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:39 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 92 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 92 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 93 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 93 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 93 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 93 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 93 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 93 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 94 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 94 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 94 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 94 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 94 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 94 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 94 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 94 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 95 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 95 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 96 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 96 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 97 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 97 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 99 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 99 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 100 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 100 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 100 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 100 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 100 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 100 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 100 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 100 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 102 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 102 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 103 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 103 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 103 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 103 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 103 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 103 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 104 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 104 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 104 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 104 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 104 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 104 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 105 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 105 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 105 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 105 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 106 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 106 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 107 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 107 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 108 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 108 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 109 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 109 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 109 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 109 elements to seafloor.
12:54:39 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 41
12:54:39 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:39 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 41 elements
12:54:39 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:39 INFO opendrift.models.chemicaldrift:1861: partitioning: [119, 0, 313, 68, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:39 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:39 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:39 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 72 elements to seafloor.
12:54:39 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:39 INFO opendrift.models.basemodel:2038: 2024-11-02 06:54:02.760182 - step 37 of 96 - 500 active elements (0 deactivated)
12:54:39 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:39 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:39 DEBUG opendrift.models.basemodel:2057: 57.544076836545145 <- latitude -> 57.704228465478266
12:54:39 DEBUG opendrift.models.basemodel:2062: 10.50792724429779 <- longitude -> 10.750852086699235
12:54:39 DEBUG opendrift.models.basemodel:2067: -22.191991436722375 <- z -> -0.11878339174923402
12:54:39 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:39 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:39 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:39 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:39 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:39 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:39 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:39 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:39 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-02 06:00:00 (before)
2024-11-02 07:00:00 (after)
12:54:39 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-02 06:00:00) in space (linearNDFast)
12:54:39 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:39 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-02 07:00:00) in space (linearNDFast)
12:54:39 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:39 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-02 06:00:00, weight 0.10) and
after (2024-11-02 07:00:00, weight 0.90) in time
12:54:39 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:39 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.2491589454995 degrees.
12:54:39 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.2491589454995 degrees.
12:54:39 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:39 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:39 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:39 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:39 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:39 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:39 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:39 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:39 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:39 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:39 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:39 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:39 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:39 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:39 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:39 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:39 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:39 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:39 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:39 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:39 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:39 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:39 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:39 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:39 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:39 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:39 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:39 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:39 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:39 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0151142 (min) 0.120485 (max)
12:54:39 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0229946 (min) 0.224228 (max)
12:54:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.161657 (min) -0.138301 (max)
12:54:39 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.33934 (min) 1.88951 (max)
12:54:39 DEBUG opendrift.models.basemodel.environment:905: y_wind: -4.70593 (min) -2.80402 (max)
12:54:39 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:39 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 23.9009 (max)
12:54:39 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:39 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.2015 (min) 12.6426 (max)
12:54:39 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0363 (min) 32.4085 (max)
12:54:39 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -5.23554e-05 (min) 2.48666e-05 (max)
12:54:39 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:39 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:39 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:39 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:39 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:39 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:39 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:39 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 72 elements to seafloor.
12:54:39 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:39 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:39 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:39 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:39 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 9
12:54:39 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 2 0 0 0 2 2 0]
12:54:39 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 0 3 3 3 0 0 3]
12:54:39 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 417. 0.]
[ 0. 0. 0. 0. 0.]
[ 81. 0. 0. 207. 0.]
[ 3. 0. 547. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:39 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:39 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:39 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:39 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:39 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.018040247414535988
12:54:39 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:39 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:39 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:39 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 75 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 75 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 76 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 76 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 77 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 77 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 79 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 79 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 79 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 79 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 80 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 80 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 80 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 80 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 80 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 80 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 81 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 81 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 81 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 81 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 82 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 82 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 84 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 84 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 84 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 84 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 84 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 84 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 85 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 85 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 85 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 85 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 85 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 85 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 85 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 85 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 86 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 86 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 88 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 88 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 89 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 89 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 89 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 89 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 89 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 89 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 89 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 89 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 89 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 89 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 89 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 89 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 89 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 89 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 89 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 89 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 89 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 89 elements to seafloor.
12:54:39 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:39 DEBUG opendrift.models.oceandrift:636: 90 elements reached seafloor, interacting with bottom
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 90 elements to seafloor.
12:54:39 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 28
12:54:39 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:39 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 28 elements
12:54:39 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:39 INFO opendrift.models.chemicaldrift:1861: partitioning: [116, 0, 322, 62, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:39 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:39 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:39 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:39 DEBUG opendrift.models.basemodel:755: Lifting 63 elements to seafloor.
12:54:39 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:39 INFO opendrift.models.basemodel:2038: 2024-11-02 07:24:02.760182 - step 38 of 96 - 500 active elements (0 deactivated)
12:54:39 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:39 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:39 DEBUG opendrift.models.basemodel:2057: 57.544448483812246 <- latitude -> 57.70785241604968
12:54:39 DEBUG opendrift.models.basemodel:2062: 10.50792724429779 <- longitude -> 10.753174446417304
12:54:39 DEBUG opendrift.models.basemodel:2067: -23.469180394485143 <- z -> -0.21857308178288876
12:54:39 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:39 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:39 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:39 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:39 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:39 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:39 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:39 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:39 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-02 07:00:00 (before)
2024-11-02 08:00:00 (after)
12:54:40 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
12:54:40 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:54:40 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:54:40 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:54:40 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:54:40 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'upward_sea_water_velocity', 'y_sea_water_velocity', 'sea_water_temperature', 'sea_water_salinity']
12:54:40 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 49x34x7) for time after (2024-11-02 08:00:00)
12:54:40 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-02 07:00:00) in space (linearNDFast)
12:54:40 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:40 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-02 08:00:00) in space (linearNDFast)
12:54:40 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:40 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-02 07:00:00, weight 0.60) and
after (2024-11-02 08:00:00, weight 0.40) in time
12:54:40 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:40 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.246836599058405 degrees.
12:54:40 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.246836599058405 degrees.
12:54:40 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:40 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:40 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:40 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:40 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:40 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:40 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:40 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:40 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:40 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:40 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:40 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:40 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:40 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:40 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:40 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:40 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:40 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:40 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:40 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:40 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:40 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:40 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:40 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:40 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:40 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:40 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:40 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:40 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:40 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0154086 (min) 0.129855 (max)
12:54:40 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0336945 (min) 0.22152 (max)
12:54:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.194829 (min) -0.172094 (max)
12:54:40 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.52129 (min) 2.46091 (max)
12:54:40 DEBUG opendrift.models.basemodel.environment:905: y_wind: -4.24245 (min) -1.26979 (max)
12:54:40 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:40 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 24.0816 (max)
12:54:40 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:40 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.1934 (min) 12.6623 (max)
12:54:40 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0329 (min) 32.4139 (max)
12:54:40 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -5.26551e-05 (min) 2.66621e-05 (max)
12:54:40 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:40 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:40 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:40 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:40 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:40 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:40 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:40 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 63 elements to seafloor.
12:54:40 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:40 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:40 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:40 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:40 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 9
12:54:40 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 3 0 0 2 2 2 0 0]
12:54:40 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 0 3 3 0 0 0 3 3]
12:54:40 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 422. 0.]
[ 0. 0. 0. 0. 0.]
[ 84. 0. 0. 223. 0.]
[ 4. 0. 575. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:40 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:40 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:40 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:40 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:40 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.0155953154524567
12:54:40 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:40 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:40 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:40 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 67 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 67 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 68 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 68 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 69 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 69 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 70 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 70 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 70 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 70 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 72 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 72 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 72 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 72 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 72 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 72 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 72 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 72 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 72 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 72 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 72 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 72 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 73 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 73 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 74 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 74 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 75 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 75 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 77 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 77 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 77 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 77 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 79 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 79 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 79 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 79 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 79 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 79 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 79 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 79 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 80 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 80 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 80 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 80 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 80 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 80 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 80 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 80 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 81 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 81 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 81 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 81 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 81 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 81 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 82 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 82 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 82 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 82 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 83 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 83 elements to seafloor.
12:54:40 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 20
12:54:40 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:40 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 20 elements
12:54:40 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:40 INFO opendrift.models.chemicaldrift:1861: partitioning: [115, 0, 322, 63, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:40 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:40 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:40 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 65 elements to seafloor.
12:54:40 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:40 INFO opendrift.models.basemodel:2038: 2024-11-02 07:54:02.760182 - step 39 of 96 - 500 active elements (0 deactivated)
12:54:40 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:40 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:40 DEBUG opendrift.models.basemodel:2057: 57.544993067304524 <- latitude -> 57.71141610560957
12:54:40 DEBUG opendrift.models.basemodel:2062: 10.507927244297788 <- longitude -> 10.755708386644068
12:54:40 DEBUG opendrift.models.basemodel:2067: -23.257047079893436 <- z -> -0.16888460870525113
12:54:40 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:40 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:40 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:40 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:40 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:40 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:40 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:40 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:40 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-02 07:00:00 (before)
2024-11-02 08:00:00 (after)
12:54:40 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-02 07:00:00) in space (linearNDFast)
12:54:40 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:40 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-02 08:00:00) in space (linearNDFast)
12:54:40 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:40 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-02 07:00:00, weight 0.10) and
after (2024-11-02 08:00:00, weight 0.90) in time
12:54:40 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:40 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.244302655180604 degrees.
12:54:40 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.244302655180604 degrees.
12:54:40 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:40 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:40 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:40 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:40 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:40 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:40 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:40 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:40 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:40 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:40 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:40 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:40 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:40 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:40 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:40 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:40 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:40 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:40 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:40 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:40 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:40 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:40 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:40 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:40 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:40 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:40 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:40 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:40 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:40 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.015279 (min) 0.140984 (max)
12:54:40 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0476259 (min) 0.22108 (max)
12:54:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.225916 (min) -0.203377 (max)
12:54:40 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.80386 (min) 3.23802 (max)
12:54:40 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.77473 (min) 0.406824 (max)
12:54:40 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:40 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 24.2949 (max)
12:54:40 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:40 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.1857 (min) 12.6393 (max)
12:54:40 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0295 (min) 32.4151 (max)
12:54:40 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -5.61005e-05 (min) 2.94793e-05 (max)
12:54:40 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:40 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:40 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:40 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:40 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:40 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:40 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:40 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 65 elements to seafloor.
12:54:40 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:40 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:40 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:40 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:40 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 8
12:54:40 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 2 0 2 2 0 2 0]
12:54:40 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 0 3 0 0 3 0 3]
12:54:40 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 426. 0.]
[ 0. 0. 0. 0. 0.]
[ 88. 0. 0. 240. 0.]
[ 4. 0. 595. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:40 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:40 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:40 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:40 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:40 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.0138531864027513
12:54:40 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:40 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:40 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:40 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 69 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 69 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 69 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 69 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 71 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 71 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 73 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 73 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 73 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 73 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 73 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 73 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 75 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 75 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 76 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 76 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 78 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 78 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 78 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 78 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 79 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 79 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 79 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 79 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 79 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 79 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 80 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 80 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 80 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 80 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 81 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 81 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 82 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 82 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 82 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 82 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 84 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 84 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 84 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 84 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 84 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 84 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 84 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 84 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 84 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 84 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 84 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 84 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 84 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 84 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 84 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 84 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 84 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 84 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 84 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 84 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 84 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 84 elements to seafloor.
12:54:40 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:40 DEBUG opendrift.models.oceandrift:636: 84 elements reached seafloor, interacting with bottom
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 84 elements to seafloor.
12:54:40 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 20
12:54:40 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:40 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 20 elements
12:54:40 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:40 INFO opendrift.models.chemicaldrift:1861: partitioning: [115, 0, 321, 64, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:40 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:40 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:40 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:40 DEBUG opendrift.models.basemodel:755: Lifting 66 elements to seafloor.
12:54:40 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:40 INFO opendrift.models.basemodel:2038: 2024-11-02 08:24:02.760182 - step 40 of 96 - 500 active elements (0 deactivated)
12:54:40 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:40 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:40 DEBUG opendrift.models.basemodel:2057: 57.54576281541569 <- latitude -> 57.71498915197099
12:54:40 DEBUG opendrift.models.basemodel:2062: 10.507927244297786 <- longitude -> 10.758461219680814
12:54:40 DEBUG opendrift.models.basemodel:2067: -22.040998458862305 <- z -> -0.17158867782879228
12:54:40 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:40 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:40 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:40 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:40 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:40 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:40 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:40 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:40 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-02 08:00:00 (before)
2024-11-02 09:00:00 (after)
12:54:42 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
12:54:42 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:54:42 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:54:42 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:54:42 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:54:42 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'upward_sea_water_velocity', 'y_sea_water_velocity', 'sea_water_temperature', 'sea_water_salinity']
12:54:42 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 50x34x7) for time after (2024-11-02 09:00:00)
12:54:42 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-02 08:00:00) in space (linearNDFast)
12:54:42 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:42 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-02 09:00:00) in space (linearNDFast)
12:54:42 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:42 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-02 08:00:00, weight 0.60) and
after (2024-11-02 09:00:00, weight 0.40) in time
12:54:42 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:42 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.24154981285297 degrees.
12:54:42 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.24154981285297 degrees.
12:54:42 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:42 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:42 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:42 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:42 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:42 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:42 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:42 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:42 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:42 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:42 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:42 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:42 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:42 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:42 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:42 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:42 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:42 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:42 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:42 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:42 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:42 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:42 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0176863 (min) 0.149033 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0561312 (min) 0.216381 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.251658 (min) -0.228762 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: x_wind: 2.26147 (min) 3.43606 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.38952 (min) 0.530409 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 24.544 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.1802 (min) 12.6206 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0263 (min) 32.4184 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -5.60552e-05 (min) 3.74604e-05 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:42 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 66 elements to seafloor.
12:54:42 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:42 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:42 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:42 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:42 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 8
12:54:42 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 0 2 2 2 0 2 0]
12:54:42 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 3 0 0 0 3 0 3]
12:54:42 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 429. 0.]
[ 0. 0. 0. 0. 0.]
[ 93. 0. 0. 257. 0.]
[ 4. 0. 615. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:42 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:42 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:42 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:42 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:42 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.013056855488438983
12:54:42 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:42 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:42 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:42 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 69 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 69 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 69 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 69 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 70 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 70 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 72 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 72 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 72 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 72 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 72 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 72 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 72 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 72 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 72 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 72 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 73 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 73 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 73 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 73 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 73 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 73 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 74 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 74 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 74 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 74 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 75 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 75 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 75 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 75 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 76 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 76 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 76 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 76 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 76 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 76 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 76 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 76 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 76 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 76 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 77 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 77 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 77 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 77 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 77 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 77 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 77 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 77 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 77 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 77 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 77 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 77 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 77 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 77 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 77 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 77 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 79 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 79 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 79 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 79 elements to seafloor.
12:54:42 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 14
12:54:42 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:42 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 14 elements
12:54:42 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:42 INFO opendrift.models.chemicaldrift:1861: partitioning: [117, 0, 318, 65, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:42 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:42 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:42 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 68 elements to seafloor.
12:54:42 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:42 INFO opendrift.models.basemodel:2038: 2024-11-02 08:54:02.760182 - step 41 of 96 - 500 active elements (0 deactivated)
12:54:42 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:42 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:42 DEBUG opendrift.models.basemodel:2057: 57.546670029911205 <- latitude -> 57.71848625238399
12:54:42 DEBUG opendrift.models.basemodel:2062: 10.507927244297788 <- longitude -> 10.761582338546727
12:54:42 DEBUG opendrift.models.basemodel:2067: -23.865843067865118 <- z -> -0.1412563465202641
12:54:42 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:42 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:42 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:42 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:42 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-02 08:00:00 (before)
2024-11-02 09:00:00 (after)
12:54:42 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-02 08:00:00) in space (linearNDFast)
12:54:42 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:42 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-02 09:00:00) in space (linearNDFast)
12:54:42 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:42 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-02 08:00:00, weight 0.10) and
after (2024-11-02 09:00:00, weight 0.90) in time
12:54:42 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:42 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.23842870043155 degrees.
12:54:42 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.23842870043155 degrees.
12:54:42 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:42 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:42 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:42 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:42 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:42 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:42 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:42 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:42 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:42 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:42 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:42 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:42 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:42 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:42 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:42 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:42 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:42 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:42 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:42 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:42 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:42 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:42 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0207217 (min) 0.154334 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0629976 (min) 0.209742 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.276448 (min) -0.252783 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: x_wind: 2.7427 (min) 3.72864 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.03567 (min) 0.394839 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 24.8377 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.1752 (min) 12.6161 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0231 (min) 32.4326 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -6.3523e-05 (min) 5.56355e-05 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:42 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 68 elements to seafloor.
12:54:42 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:42 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:42 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:42 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:42 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 12
12:54:42 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 2 0 2 2 2 2 0 2 2]
12:54:42 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 0 3 0 0 0 0 3 0 0]
12:54:42 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 434. 0.]
[ 0. 0. 0. 0. 0.]
[100. 0. 0. 269. 0.]
[ 4. 0. 629. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:42 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:42 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:42 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:42 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:42 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.012834419003037643
12:54:42 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:42 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:42 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:42 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 71 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 71 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 71 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 71 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 72 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 72 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 73 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 73 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 73 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 73 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 73 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 73 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 73 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 73 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 74 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 74 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 77 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 77 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 79 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 79 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 79 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 79 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 79 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 79 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 79 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 79 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 81 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 81 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 81 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 81 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 81 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 81 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 81 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 81 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 82 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 82 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 82 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 82 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 83 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 83 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 83 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 83 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 84 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 84 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 84 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 84 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 85 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 85 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 85 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 85 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 85 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 85 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 85 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 85 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 85 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 85 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 85 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 85 elements to seafloor.
12:54:42 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:42 DEBUG opendrift.models.oceandrift:636: 86 elements reached seafloor, interacting with bottom
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 86 elements to seafloor.
12:54:42 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 20
12:54:42 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:42 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 20 elements
12:54:42 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:42 INFO opendrift.models.chemicaldrift:1861: partitioning: [119, 0, 315, 66, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:42 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:42 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:42 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:42 DEBUG opendrift.models.basemodel:755: Lifting 70 elements to seafloor.
12:54:42 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:42 INFO opendrift.models.basemodel:2038: 2024-11-02 09:24:02.760182 - step 42 of 96 - 500 active elements (0 deactivated)
12:54:42 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:42 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:42 DEBUG opendrift.models.basemodel:2057: 57.54768822044489 <- latitude -> 57.72187605044533
12:54:42 DEBUG opendrift.models.basemodel:2062: 10.50792724429779 <- longitude -> 10.76463125545098
12:54:42 DEBUG opendrift.models.basemodel:2067: -23.44603233823851 <- z -> -0.18216424267564368
12:54:42 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:42 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:42 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:42 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:42 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-02 09:00:00 (before)
2024-11-02 10:00:00 (after)
12:54:44 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
12:54:44 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:54:44 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:54:44 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:54:44 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:54:44 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'upward_sea_water_velocity', 'y_sea_water_velocity', 'sea_water_temperature', 'sea_water_salinity']
12:54:44 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 51x34x7) for time after (2024-11-02 10:00:00)
12:54:44 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-02 09:00:00) in space (linearNDFast)
12:54:44 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:44 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-02 10:00:00) in space (linearNDFast)
12:54:44 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:44 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-02 09:00:00, weight 0.60) and
after (2024-11-02 10:00:00, weight 0.40) in time
12:54:44 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:44 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.235379794126864 degrees.
12:54:44 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.235379794126864 degrees.
12:54:44 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:44 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:44 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:44 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:44 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:44 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:44 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:44 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:44 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:44 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:44 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:44 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:44 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:44 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:44 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:44 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:44 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:44 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:44 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:44 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:44 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:44 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:44 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:44 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:44 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:44 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:44 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:44 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:44 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:44 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0229281 (min) 0.163281 (max)
12:54:44 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.063246 (min) 0.208341 (max)
12:54:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.289582 (min) -0.265969 (max)
12:54:44 DEBUG opendrift.models.basemodel.environment:905: x_wind: 3.06976 (min) 3.7517 (max)
12:54:44 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.65271 (min) 0.297635 (max)
12:54:44 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:44 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 25.1802 (max)
12:54:44 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:44 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.1707 (min) 12.6229 (max)
12:54:44 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0205 (min) 32.4585 (max)
12:54:44 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -5.84973e-05 (min) 6.76729e-05 (max)
12:54:44 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:44 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:44 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:44 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:44 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:44 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:44 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:44 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 70 elements to seafloor.
12:54:44 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:44 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:44 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:44 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:44 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 8
12:54:44 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 2 0 0 0 2 0 2]
12:54:44 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 0 3 3 3 0 3 0]
12:54:44 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 439. 0.]
[ 0. 0. 0. 0. 0.]
[103. 0. 0. 285. 0.]
[ 4. 0. 649. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:44 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:44 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:44 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:44 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:44 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.012522286827496985
12:54:44 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:44 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:44 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:44 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 72 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 72 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 72 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 72 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 72 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 72 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 73 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 73 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 73 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 73 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 73 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 73 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 74 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 74 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 74 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 74 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 74 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 74 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 74 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 74 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 74 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 74 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 75 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 75 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 75 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 75 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 76 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 76 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 76 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 76 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 76 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 76 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 76 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 76 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 76 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 76 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 76 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 76 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 76 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 76 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 76 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 76 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 76 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 76 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 76 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 76 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 78 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 78 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 78 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 78 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 79 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 79 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 79 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 79 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 79 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 79 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 79 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 79 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 79 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 79 elements to seafloor.
12:54:44 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 13
12:54:44 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:44 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 13 elements
12:54:44 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:44 INFO opendrift.models.chemicaldrift:1861: partitioning: [117, 0, 317, 66, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:44 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:44 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:44 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 68 elements to seafloor.
12:54:44 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:44 INFO opendrift.models.basemodel:2038: 2024-11-02 09:54:02.760182 - step 43 of 96 - 500 active elements (0 deactivated)
12:54:44 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:44 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:44 DEBUG opendrift.models.basemodel:2057: 57.548710424927876 <- latitude -> 57.72518481398373
12:54:44 DEBUG opendrift.models.basemodel:2062: 10.507927244297791 <- longitude -> 10.76792761177955
12:54:44 DEBUG opendrift.models.basemodel:2067: -23.58350944519043 <- z -> -0.0464839941093359
12:54:44 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:44 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:44 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:44 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:44 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:44 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:44 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:44 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:44 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-02 09:00:00 (before)
2024-11-02 10:00:00 (after)
12:54:44 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-02 09:00:00) in space (linearNDFast)
12:54:44 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:44 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-02 10:00:00) in space (linearNDFast)
12:54:44 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:44 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-02 09:00:00, weight 0.10) and
after (2024-11-02 10:00:00, weight 0.90) in time
12:54:44 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:44 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.23208341862784 degrees.
12:54:44 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.23208341862784 degrees.
12:54:44 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:44 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:44 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:44 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:44 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:44 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:44 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:44 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:44 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:44 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:44 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:44 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:44 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:44 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:44 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:44 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:44 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:44 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:44 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:44 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:44 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:44 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:44 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:44 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:44 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:44 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:44 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:44 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:44 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:44 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0249293 (min) 0.171543 (max)
12:54:44 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0648843 (min) 0.205401 (max)
12:54:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.300056 (min) -0.276465 (max)
12:54:44 DEBUG opendrift.models.basemodel.environment:905: x_wind: 3.05845 (min) 3.69611 (max)
12:54:44 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.24448 (min) 0.0218166 (max)
12:54:44 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:44 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 25.5926 (max)
12:54:44 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:44 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.1663 (min) 12.6167 (max)
12:54:44 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0181 (min) 32.5451 (max)
12:54:44 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -7.50428e-05 (min) 7.71025e-05 (max)
12:54:44 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:44 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:44 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:44 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:44 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:44 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:44 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:44 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 68 elements to seafloor.
12:54:44 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:44 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:44 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:44 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:44 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 11
12:54:44 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 2 2 2 0 2 0 0 2 2 2]
12:54:44 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 0 0 0 3 0 3 3 0 0 0]
12:54:44 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 442. 0.]
[ 0. 0. 0. 0. 0.]
[111. 0. 0. 293. 0.]
[ 4. 0. 662. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:44 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:44 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:44 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:44 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:44 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.012617583588727315
12:54:44 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:44 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:44 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:44 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 70 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 70 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 71 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 71 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 71 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 71 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 71 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 71 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 71 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 71 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 71 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 71 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 71 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 71 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 71 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 71 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 72 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 72 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 73 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 73 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 73 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 73 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 73 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 73 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 74 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 74 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 74 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 74 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 74 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 74 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 75 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 75 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 75 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 75 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 75 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 75 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 75 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 75 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 75 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 75 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 77 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 77 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 79 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 79 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 79 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 79 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 80 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 80 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 80 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 80 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 80 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 80 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 80 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 80 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 80 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 80 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 80 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 80 elements to seafloor.
12:54:44 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:44 DEBUG opendrift.models.oceandrift:636: 80 elements reached seafloor, interacting with bottom
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 80 elements to seafloor.
12:54:44 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 13
12:54:44 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:44 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 13 elements
12:54:44 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:44 INFO opendrift.models.chemicaldrift:1861: partitioning: [122, 0, 311, 67, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:44 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:44 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:44 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:44 DEBUG opendrift.models.basemodel:755: Lifting 70 elements to seafloor.
12:54:44 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:44 INFO opendrift.models.basemodel:2038: 2024-11-02 10:24:02.760182 - step 44 of 96 - 500 active elements (0 deactivated)
12:54:44 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:44 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:44 DEBUG opendrift.models.basemodel:2057: 57.54975910738507 <- latitude -> 57.72835605877766
12:54:44 DEBUG opendrift.models.basemodel:2062: 10.507927244297791 <- longitude -> 10.771376401254297
12:54:44 DEBUG opendrift.models.basemodel:2067: -23.21321468936303 <- z -> -0.09103562257482656
12:54:44 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:44 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:44 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:44 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:44 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:44 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:44 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:44 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:44 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-02 10:00:00 (before)
2024-11-02 11:00:00 (after)
12:54:45 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
12:54:45 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:54:45 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:54:45 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:54:45 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:54:45 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'upward_sea_water_velocity', 'y_sea_water_velocity', 'sea_water_temperature', 'sea_water_salinity']
12:54:45 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 52x34x7) for time after (2024-11-02 11:00:00)
12:54:45 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-02 10:00:00) in space (linearNDFast)
12:54:45 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:45 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-02 11:00:00) in space (linearNDFast)
12:54:45 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:46 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-02 10:00:00, weight 0.60) and
after (2024-11-02 11:00:00, weight 0.40) in time
12:54:46 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:46 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.22863464214128 degrees.
12:54:46 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.22863464214128 degrees.
12:54:46 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:46 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:46 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:46 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:46 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:46 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:46 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:46 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:46 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:46 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:46 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:46 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:46 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:46 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:46 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:46 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:46 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:46 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:46 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:46 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:46 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:46 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:46 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:46 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:46 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:46 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:46 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:46 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:46 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:46 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0295436 (min) 0.178555 (max)
12:54:46 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0688616 (min) 0.197343 (max)
12:54:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.295013 (min) -0.271915 (max)
12:54:46 DEBUG opendrift.models.basemodel.environment:905: x_wind: 2.9978 (min) 3.6107 (max)
12:54:46 DEBUG opendrift.models.basemodel.environment:905: y_wind: -1.85281 (min) 0.156519 (max)
12:54:46 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:46 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 25.979 (max)
12:54:46 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:46 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.1633 (min) 12.6207 (max)
12:54:46 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0159 (min) 32.6405 (max)
12:54:46 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -7.50689e-05 (min) 8.76781e-05 (max)
12:54:46 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:46 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:46 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:46 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:46 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:46 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:46 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:46 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 70 elements to seafloor.
12:54:46 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:46 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:46 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:46 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:46 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 8
12:54:46 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 0 2 2 0 0 0 2]
12:54:46 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 3 0 0 3 3 3 0]
12:54:46 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 446. 0.]
[ 0. 0. 0. 0. 0.]
[115. 0. 0. 304. 0.]
[ 4. 0. 675. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:46 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:46 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:46 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:46 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:46 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.011388265871967609
12:54:46 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:46 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:46 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:46 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 73 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 73 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 73 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 73 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 73 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 73 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 73 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 73 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 73 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 73 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 74 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 74 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 74 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 74 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 74 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 74 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 74 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 74 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 74 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 74 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 74 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 74 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 74 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 74 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 74 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 74 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 75 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 75 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 75 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 75 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 75 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 75 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 75 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 75 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 75 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 75 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 75 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 75 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 77 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 77 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 77 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 77 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 77 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 77 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 78 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 78 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 78 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 78 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 78 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 78 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 78 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 78 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 78 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 78 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 78 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 78 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 78 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 78 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 79 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 79 elements to seafloor.
12:54:46 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 11
12:54:46 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:46 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 11 elements
12:54:46 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:46 INFO opendrift.models.chemicaldrift:1861: partitioning: [122, 0, 310, 68, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:46 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:46 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:46 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 68 elements to seafloor.
12:54:46 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:46 INFO opendrift.models.basemodel:2038: 2024-11-02 10:54:02.760182 - step 45 of 96 - 500 active elements (0 deactivated)
12:54:46 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:46 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:46 DEBUG opendrift.models.basemodel:2057: 57.55087207234947 <- latitude -> 57.73109309931837
12:54:46 DEBUG opendrift.models.basemodel:2062: 10.507927244297791 <- longitude -> 10.775025400207618
12:54:46 DEBUG opendrift.models.basemodel:2067: -25.424163775395648 <- z -> -0.05499588744967587
12:54:46 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:46 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:46 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:46 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:46 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:46 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:46 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:46 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:46 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-02 10:00:00 (before)
2024-11-02 11:00:00 (after)
12:54:46 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-02 10:00:00) in space (linearNDFast)
12:54:46 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:46 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-02 11:00:00) in space (linearNDFast)
12:54:46 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:46 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-02 10:00:00, weight 0.10) and
after (2024-11-02 11:00:00, weight 0.90) in time
12:54:46 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:46 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.22498563070566 degrees.
12:54:46 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.22498563070566 degrees.
12:54:46 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:46 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:46 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:46 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:46 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:46 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:46 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:46 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:46 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:46 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:46 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:46 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:46 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:46 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:46 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:46 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:46 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:46 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:46 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:46 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:46 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:46 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:46 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:46 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:46 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:46 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:46 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:46 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:46 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:46 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0348049 (min) 0.185276 (max)
12:54:46 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0660913 (min) 0.188112 (max)
12:54:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.29008 (min) -0.263276 (max)
12:54:46 DEBUG opendrift.models.basemodel.environment:905: x_wind: 2.87568 (min) 3.43616 (max)
12:54:46 DEBUG opendrift.models.basemodel.environment:905: y_wind: -1.47209 (min) 0.359962 (max)
12:54:46 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:46 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 26.4483 (max)
12:54:46 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:46 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.1606 (min) 12.6272 (max)
12:54:46 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0136 (min) 32.8367 (max)
12:54:46 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000108794 (min) 9.46503e-05 (max)
12:54:46 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:46 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:46 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:46 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:46 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:46 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:46 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:46 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 68 elements to seafloor.
12:54:46 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:46 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:46 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:46 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:46 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 6
12:54:46 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 0 0 0 2 2]
12:54:46 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 3 3 3 0 0]
12:54:46 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 449. 0.]
[ 0. 0. 0. 0. 0.]
[118. 0. 0. 312. 0.]
[ 4. 0. 686. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:46 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:46 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:46 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:46 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:46 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.010085251616607628
12:54:46 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:46 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:46 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:46 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 73 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 73 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 74 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 74 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 74 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 74 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 74 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 74 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 75 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 75 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 75 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 75 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 75 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 75 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 75 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 75 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 75 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 75 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 75 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 75 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 75 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 75 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 76 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 76 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 76 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 76 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 78 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 78 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 79 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 79 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 80 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 80 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 80 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 80 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 80 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 80 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 80 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 80 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 80 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 80 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 81 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 81 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 82 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 82 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 83 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 83 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 84 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 84 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 85 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 85 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 86 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 86 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 86 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 86 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 86 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 86 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 86 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 86 elements to seafloor.
12:54:46 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:46 DEBUG opendrift.models.oceandrift:636: 87 elements reached seafloor, interacting with bottom
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 87 elements to seafloor.
12:54:46 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 17
12:54:46 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:46 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 17 elements
12:54:46 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:46 INFO opendrift.models.chemicaldrift:1861: partitioning: [122, 0, 308, 70, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:46 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:46 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:46 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:46 DEBUG opendrift.models.basemodel:755: Lifting 70 elements to seafloor.
12:54:46 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:46 INFO opendrift.models.basemodel:2038: 2024-11-02 11:24:02.760182 - step 46 of 96 - 500 active elements (0 deactivated)
12:54:46 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:46 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:46 DEBUG opendrift.models.basemodel:2057: 57.55194026136295 <- latitude -> 57.73339651075414
12:54:46 DEBUG opendrift.models.basemodel:2062: 10.507927244297791 <- longitude -> 10.77934998846324
12:54:46 DEBUG opendrift.models.basemodel:2067: -24.164421968816136 <- z -> 0.0
12:54:46 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:46 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:46 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:46 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:46 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:46 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:46 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:46 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:46 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-02 11:00:00 (before)
2024-11-02 12:00:00 (after)
12:54:47 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
12:54:47 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:54:47 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:54:47 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:54:47 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:54:47 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'upward_sea_water_velocity', 'y_sea_water_velocity', 'sea_water_temperature', 'sea_water_salinity']
12:54:47 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 53x34x7) for time after (2024-11-02 12:00:00)
12:54:47 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-02 11:00:00) in space (linearNDFast)
12:54:47 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:47 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-02 12:00:00) in space (linearNDFast)
12:54:47 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:47 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-02 11:00:00, weight 0.60) and
after (2024-11-02 12:00:00, weight 0.40) in time
12:54:47 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:47 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.220661043780524 degrees.
12:54:47 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.220661043780524 degrees.
12:54:47 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:47 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:47 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:47 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:47 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:47 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:47 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:47 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:47 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:47 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:47 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:47 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:47 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:47 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:47 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:47 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:47 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:47 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:47 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:47 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:47 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:47 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:47 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:47 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:47 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:47 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:47 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:47 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:47 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:47 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0379423 (min) 0.187292 (max)
12:54:47 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.061228 (min) 0.172414 (max)
12:54:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.263989 (min) -0.233255 (max)
12:54:47 DEBUG opendrift.models.basemodel.environment:905: x_wind: 3.00283 (min) 3.51407 (max)
12:54:47 DEBUG opendrift.models.basemodel.environment:905: y_wind: -0.972696 (min) 0.598941 (max)
12:54:47 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:47 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 27.3562 (max)
12:54:47 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:47 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.1601 (min) 12.6295 (max)
12:54:47 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0122 (min) 32.6853 (max)
12:54:47 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -8.70405e-05 (min) 0.000103618 (max)
12:54:47 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:47 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:47 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:47 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:47 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:47 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:47 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:47 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 70 elements to seafloor.
12:54:47 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:47 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:47 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:47 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:47 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 13
12:54:47 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 2 0 2 0 3 3 2 0 2 0 3 0]
12:54:47 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 0 3 0 3 0 0 0 3 0 3 0 3]
12:54:47 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 455. 0.]
[ 0. 0. 0. 0. 0.]
[122. 0. 0. 328. 0.]
[ 7. 0. 703. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:47 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:47 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:47 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:47 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:47 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.009603563033238244
12:54:47 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:47 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:47 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:47 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 74 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 74 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 75 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 75 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 75 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 75 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 75 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 75 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 75 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 75 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 75 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 75 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 75 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 75 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 75 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 75 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 75 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 75 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 75 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 75 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 75 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 75 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 75 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 75 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 75 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 75 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 77 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 77 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 77 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 77 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 77 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 77 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 77 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 77 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 77 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 77 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 77 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 77 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 79 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 79 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 80 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 80 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 80 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 80 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 82 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 82 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 82 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 82 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 82 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 82 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 83 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 83 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 83 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 83 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 83 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 83 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 83 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 83 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 83 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 83 elements to seafloor.
12:54:47 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 6
12:54:47 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:47 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 6 elements
12:54:47 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:47 INFO opendrift.models.chemicaldrift:1861: partitioning: [123, 0, 300, 77, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:47 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:47 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:47 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 77 elements to seafloor.
12:54:47 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:47 INFO opendrift.models.basemodel:2038: 2024-11-02 11:54:02.760182 - step 47 of 96 - 500 active elements (0 deactivated)
12:54:47 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:47 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:47 DEBUG opendrift.models.basemodel:2057: 57.55194026136295 <- latitude -> 57.7349702850928
12:54:47 DEBUG opendrift.models.basemodel:2062: 10.507927244297791 <- longitude -> 10.784448290910369
12:54:47 DEBUG opendrift.models.basemodel:2067: -24.031581150136397 <- z -> -0.19547187525089915
12:54:47 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:47 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:47 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:47 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:47 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:47 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:47 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:47 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:47 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-02 11:00:00 (before)
2024-11-02 12:00:00 (after)
12:54:47 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-02 11:00:00) in space (linearNDFast)
12:54:47 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:47 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-02 12:00:00) in space (linearNDFast)
12:54:47 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:47 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-02 11:00:00, weight 0.10) and
after (2024-11-02 12:00:00, weight 0.90) in time
12:54:47 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:47 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.21556273034001 degrees.
12:54:47 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.21556273034001 degrees.
12:54:47 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:47 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:47 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:47 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:47 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:47 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:47 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:47 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:47 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:47 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:47 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:47 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:47 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:47 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:47 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:47 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:47 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:47 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:47 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:47 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:47 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:47 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:47 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:47 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:47 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:47 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:47 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:47 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:47 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:47 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0405538 (min) 0.192295 (max)
12:54:47 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0305358 (min) 0.152522 (max)
12:54:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.232327 (min) -0.196287 (max)
12:54:47 DEBUG opendrift.models.basemodel.environment:905: x_wind: 3.1917 (min) 3.62783 (max)
12:54:47 DEBUG opendrift.models.basemodel.environment:905: y_wind: -0.468211 (min) 0.838058 (max)
12:54:47 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:47 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 27.8983 (max)
12:54:47 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:47 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.1601 (min) 12.6269 (max)
12:54:47 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.011 (min) 32.8008 (max)
12:54:47 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000124385 (min) 0.000112974 (max)
12:54:47 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:47 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:47 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:47 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:47 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:47 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:47 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:47 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 77 elements to seafloor.
12:54:47 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:47 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:47 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:47 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:47 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 3
12:54:47 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 2 2]
12:54:47 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 0 0]
12:54:47 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 455. 0.]
[ 0. 0. 0. 0. 0.]
[125. 0. 0. 338. 0.]
[ 7. 0. 709. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:47 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:47 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:47 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:47 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:47 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.009646752004510937
12:54:47 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:47 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:47 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:47 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 77 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 77 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 78 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 78 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 78 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 78 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 78 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 78 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 78 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 78 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 78 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 78 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 79 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 79 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 79 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 79 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 79 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 79 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 81 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 81 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 82 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 82 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 82 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 82 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 82 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 82 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 82 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 82 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 82 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 82 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 82 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 82 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 82 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 82 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 82 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 82 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 84 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 84 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 85 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 85 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 87 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 87 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 89 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 89 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 89 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 89 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 90 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 90 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 91 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 91 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 91 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 91 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 91 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 91 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 91 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 91 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 92 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 92 elements to seafloor.
12:54:47 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
12:54:47 DEBUG opendrift.models.oceandrift:636: 92 elements reached seafloor, interacting with bottom
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 92 elements to seafloor.
12:54:47 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 3
12:54:47 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:47 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 3 elements
12:54:47 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:47 INFO opendrift.models.chemicaldrift:1861: partitioning: [126, 0, 285, 89, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:47 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:47 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:47 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:47 DEBUG opendrift.models.basemodel:755: Lifting 91 elements to seafloor.
12:54:47 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:47 INFO opendrift.models.basemodel:2038: 2024-11-02 12:24:02.760182 - step 48 of 96 - 500 active elements (0 deactivated)
12:54:47 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:47 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:47 DEBUG opendrift.models.basemodel:2057: 57.55194026136295 <- latitude -> 57.73581371966198
12:54:47 DEBUG opendrift.models.basemodel:2062: 10.507927244297791 <- longitude -> 10.789037097603444
12:54:47 DEBUG opendrift.models.basemodel:2067: -27.898298263549805 <- z -> -0.09465982191588995
12:54:47 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:47 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:47 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:47 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:47 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:47 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:47 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:47 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:47 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-02 12:00:00 (before)
2024-11-02 13:00:00 (after)
12:54:49 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
12:54:49 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:54:49 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:54:49 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:54:49 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:54:49 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'upward_sea_water_velocity', 'y_sea_water_velocity', 'sea_water_temperature', 'sea_water_salinity']
12:54:49 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 53x35x7) for time after (2024-11-02 13:00:00)
12:54:49 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-02 12:00:00) in space (linearNDFast)
12:54:49 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:49 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-02 13:00:00) in space (linearNDFast)
12:54:49 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:49 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-02 12:00:00, weight 0.60) and
after (2024-11-02 13:00:00, weight 0.40) in time
12:54:49 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:49 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.210973930458906 degrees.
12:54:49 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.210973930458906 degrees.
12:54:49 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:49 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:49 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:49 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:49 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:49 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:49 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:49 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:49 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:49 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:49 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:49 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:49 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:49 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:49 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:49 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:49 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:49 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:49 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:49 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:49 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:49 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:49 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:49 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:49 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:49 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:49 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:49 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0515665 (min) 0.187641 (max)
12:54:49 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0265386 (min) 0.145204 (max)
12:54:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.183562 (min) -0.142713 (max)
12:54:49 DEBUG opendrift.models.basemodel.environment:905: x_wind: 3.64788 (min) 4.09761 (max)
12:54:49 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0.105807 (min) 1.07559 (max)
12:54:49 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:49 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 28.1605 (max)
12:54:49 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:49 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.161 (min) 12.62 (max)
12:54:49 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0106 (min) 32.8865 (max)
12:54:49 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000141445 (min) 0.000120599 (max)
12:54:49 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:49 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:49 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:49 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:49 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:49 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:49 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:49 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 89 elements to seafloor.
12:54:49 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:49 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:49 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:49 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:49 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 5
12:54:49 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 0 2 0 2]
12:54:49 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 3 0 3 0]
12:54:49 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 457. 0.]
[ 0. 0. 0. 0. 0.]
[128. 0. 0. 353. 0.]
[ 7. 0. 712. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:49 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:49 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:49 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:49 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:49 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.012163877412838149
12:54:49 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:49 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:49 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:49 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 91 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 91 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 91 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 91 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 91 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 91 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 91 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 91 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 91 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 91 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 91 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 91 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 91 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 91 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 92 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 92 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 92 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 92 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 92 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 92 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 92 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 92 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 92 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 92 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 92 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 92 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 93 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 93 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 93 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 93 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 94 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 94 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 94 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 94 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 94 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 94 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 94 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 94 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 94 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 94 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 96 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 96 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 96 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 96 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 96 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 96 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 96 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 96 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 97 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 97 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 98 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 98 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 98 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 98 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 99 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 99 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 99 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 99 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 99 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 99 elements to seafloor.
12:54:49 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 2
12:54:49 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:49 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 2 elements
12:54:49 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:49 INFO opendrift.models.chemicaldrift:1861: partitioning: [127, 0, 276, 97, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:49 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:49 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:49 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 97 elements to seafloor.
12:54:49 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:49 INFO opendrift.models.basemodel:2038: 2024-11-02 12:54:02.760182 - step 49 of 96 - 500 active elements (0 deactivated)
12:54:49 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:49 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:49 DEBUG opendrift.models.basemodel:2057: 57.55194026136295 <- latitude -> 57.7358403990689
12:54:49 DEBUG opendrift.models.basemodel:2062: 10.507927244297791 <- longitude -> 10.794052393309514
12:54:49 DEBUG opendrift.models.basemodel:2067: -27.898296356201172 <- z -> -0.014334493211464183
12:54:49 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:49 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:49 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:49 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:49 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:49 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:49 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:49 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:49 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-02 12:00:00 (before)
2024-11-02 13:00:00 (after)
12:54:49 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-02 12:00:00) in space (linearNDFast)
12:54:49 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:49 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-02 13:00:00) in space (linearNDFast)
12:54:49 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:49 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-02 12:00:00, weight 0.10) and
after (2024-11-02 13:00:00, weight 0.90) in time
12:54:49 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:49 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.20595863477506 degrees.
12:54:49 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.20595863477506 degrees.
12:54:49 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:49 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:49 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:49 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:49 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:49 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:49 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:49 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:49 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:49 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:49 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:49 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:49 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:49 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:49 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:49 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:49 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:49 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:49 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:49 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:49 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:49 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:49 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:49 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:49 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:49 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:49 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:49 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0425667 (min) 0.186144 (max)
12:54:49 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.105832 (min) 0.130959 (max)
12:54:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.130562 (min) -0.0855383 (max)
12:54:49 DEBUG opendrift.models.basemodel.environment:905: x_wind: 4.06931 (min) 4.68674 (max)
12:54:49 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0.690437 (min) 1.34288 (max)
12:54:49 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:49 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 28.6412 (max)
12:54:49 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:49 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.1577 (min) 12.6093 (max)
12:54:49 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0104 (min) 33.022 (max)
12:54:49 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000117701 (min) 0.000131296 (max)
12:54:49 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:49 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:49 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:49 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:49 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:49 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:49 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:49 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 97 elements to seafloor.
12:54:49 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:49 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:49 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:49 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:49 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 8
12:54:49 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 2 2 2 0 2 2 2]
12:54:49 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 0 0 0 3 0 0 0]
12:54:49 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 458. 0.]
[ 0. 0. 0. 0. 0.]
[135. 0. 0. 361. 0.]
[ 7. 0. 714. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:49 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:49 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:49 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:49 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:49 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.01627693738404866
12:54:49 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:49 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:49 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:49 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 98 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 98 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 98 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 98 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 98 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 98 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 98 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 98 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 98 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 98 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 98 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 98 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 98 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 98 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 98 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 98 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 100 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 100 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 100 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 100 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 101 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 101 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 101 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 101 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 102 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 102 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 102 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 102 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 102 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 102 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 102 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 102 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 102 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 102 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 103 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 103 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 104 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 104 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 104 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 104 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 104 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 104 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 104 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 104 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 105 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 105 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 105 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 105 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 105 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 105 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 105 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 105 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 105 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 105 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 105 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 105 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 105 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 105 elements to seafloor.
12:54:49 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
12:54:49 DEBUG opendrift.models.oceandrift:636: 105 elements reached seafloor, interacting with bottom
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 105 elements to seafloor.
12:54:49 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 0
12:54:49 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:49 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:49 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:49 INFO opendrift.models.chemicaldrift:1861: partitioning: [133, 0, 262, 105, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:49 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:49 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:49 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:49 DEBUG opendrift.models.basemodel:755: Lifting 106 elements to seafloor.
12:54:49 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:49 INFO opendrift.models.basemodel:2038: 2024-11-02 13:24:02.760182 - step 50 of 96 - 500 active elements (0 deactivated)
12:54:49 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:49 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:49 DEBUG opendrift.models.basemodel:2057: 57.55194026136295 <- latitude -> 57.734972112214464
12:54:49 DEBUG opendrift.models.basemodel:2062: 10.507927244297791 <- longitude -> 10.798540388009537
12:54:49 DEBUG opendrift.models.basemodel:2067: -28.385516496731128 <- z -> -0.017679485094048597
12:54:49 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:49 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:49 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:49 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:49 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:49 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:49 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:49 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:49 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-02 13:00:00 (before)
2024-11-02 14:00:00 (after)
12:54:50 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
12:54:50 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:54:50 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:54:50 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:54:50 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:54:50 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'upward_sea_water_velocity', 'y_sea_water_velocity', 'sea_water_temperature', 'sea_water_salinity']
12:54:50 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 53x35x7) for time after (2024-11-02 14:00:00)
12:54:50 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-02 13:00:00) in space (linearNDFast)
12:54:50 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:50 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-02 14:00:00) in space (linearNDFast)
12:54:50 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:50 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-02 13:00:00, weight 0.60) and
after (2024-11-02 14:00:00, weight 0.40) in time
12:54:50 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:50 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.20147063377901 degrees.
12:54:50 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.20147063377901 degrees.
12:54:51 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:51 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:51 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:51 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:51 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:51 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:51 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:51 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:51 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:51 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:51 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:51 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:51 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:51 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:51 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:51 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:51 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:51 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:51 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:51 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:51 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:51 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:51 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:51 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:51 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:51 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:51 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:51 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:51 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:51 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0650264 (min) 0.179292 (max)
12:54:51 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.153444 (min) 0.109047 (max)
12:54:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.0707486 (min) -0.0349618 (max)
12:54:51 DEBUG opendrift.models.basemodel.environment:905: x_wind: 4.45956 (min) 5.10637 (max)
12:54:51 DEBUG opendrift.models.basemodel.environment:905: y_wind: 1.0031 (min) 1.50816 (max)
12:54:51 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:51 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 29.0208 (max)
12:54:51 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:51 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.1527 (min) 12.5954 (max)
12:54:51 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0118 (min) 32.9163 (max)
12:54:51 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -9.36092e-05 (min) 0.000266067 (max)
12:54:51 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:51 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:51 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:51 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:51 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:51 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:51 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:51 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 105 elements to seafloor.
12:54:51 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:51 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:51 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:51 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:51 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 2
12:54:51 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 2]
12:54:51 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 0]
12:54:51 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 459. 0.]
[ 0. 0. 0. 0. 0.]
[136. 0. 0. 368. 0.]
[ 7. 0. 714. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:51 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:51 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:51 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:51 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:51 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.01966736121431829
12:54:51 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:51 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:51 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:51 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 106 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 106 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 106 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 106 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 106 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 106 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 107 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 107 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 107 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 107 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 107 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 107 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 107 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 107 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 107 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 107 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 107 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 107 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 108 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 108 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 108 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 108 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 108 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 108 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 109 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 109 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 109 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 109 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 109 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 109 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 109 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 109 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 109 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 109 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 109 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 109 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 110 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 110 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 110 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 110 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 110 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 110 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 111 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 111 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 112 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 112 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 113 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 113 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 114 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 114 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 114 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 114 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 114 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 114 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 114 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 114 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 115 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 115 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 116 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 116 elements to seafloor.
12:54:51 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 1
12:54:51 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:51 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 1 elements
12:54:51 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:51 INFO opendrift.models.chemicaldrift:1861: partitioning: [133, 0, 252, 115, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:51 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:51 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:51 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 115 elements to seafloor.
12:54:51 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:51 INFO opendrift.models.basemodel:2038: 2024-11-02 13:54:02.760182 - step 51 of 96 - 500 active elements (0 deactivated)
12:54:51 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:51 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:51 DEBUG opendrift.models.basemodel:2057: 57.55194026136295 <- latitude -> 57.73469488537275
12:54:51 DEBUG opendrift.models.basemodel:2062: 10.507927244297791 <- longitude -> 10.801843879384888
12:54:51 DEBUG opendrift.models.basemodel:2067: -27.898296356201172 <- z -> 0.0
12:54:51 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:51 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:51 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:51 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:51 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:51 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:51 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:51 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:51 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-02 13:00:00 (before)
2024-11-02 14:00:00 (after)
12:54:51 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-02 13:00:00) in space (linearNDFast)
12:54:51 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:51 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-02 14:00:00) in space (linearNDFast)
12:54:51 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:51 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-02 13:00:00, weight 0.10) and
after (2024-11-02 14:00:00, weight 0.90) in time
12:54:51 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:51 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.19816715032627 degrees.
12:54:51 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.19816715032627 degrees.
12:54:51 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:51 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:51 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:51 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:51 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:51 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:51 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:51 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:51 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:51 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:51 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:51 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:51 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:51 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:51 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:51 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:51 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:51 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:51 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:51 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:51 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:51 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:51 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:51 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:51 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:51 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:51 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:51 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:51 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:51 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0731646 (min) 0.180261 (max)
12:54:51 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.174959 (min) 0.114161 (max)
12:54:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.00924865 (min) 0.0153375 (max)
12:54:51 DEBUG opendrift.models.basemodel.environment:905: x_wind: 4.78164 (min) 5.52736 (max)
12:54:51 DEBUG opendrift.models.basemodel.environment:905: y_wind: 1.20087 (min) 1.64609 (max)
12:54:51 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:51 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 29.3864 (max)
12:54:51 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:51 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.1541 (min) 12.6095 (max)
12:54:51 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0112 (min) 33.1737 (max)
12:54:51 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -4.3147e-05 (min) 0.000445215 (max)
12:54:51 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:51 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:51 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:51 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:51 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:51 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:51 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:51 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 43 elements to seafloor.
12:54:51 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:51 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:51 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:51 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:51 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 9
12:54:51 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 0 0 0 0 2 2 2 0]
12:54:51 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 3 3 3 3 0 0 0 3]
12:54:51 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 464. 0.]
[ 0. 0. 0. 0. 0.]
[140. 0. 0. 378. 0.]
[ 7. 0. 715. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:51 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:51 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:51 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:51 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:51 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.023160226569524798
12:54:51 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:51 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:51 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:51 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 43 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 43 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 43 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 43 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 43 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 43 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 45 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 45 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 44 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 44 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 43 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 43 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 43 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 43 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 43 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 43 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 43 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 43 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 43 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 43 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 43 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 43 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 43 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 43 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 43 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 43 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 43 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 43 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 43 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 43 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 43 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 43 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 43 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 43 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 43 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 43 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 43 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 43 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 43 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 43 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 43 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 43 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 43 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 43 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 43 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 43 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 43 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 43 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 44 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 44 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 43 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 43 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 43 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 43 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 43 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 43 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 43 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 43 elements to seafloor.
12:54:51 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
12:54:51 DEBUG opendrift.models.oceandrift:636: 43 elements reached seafloor, interacting with bottom
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 43 elements to seafloor.
12:54:51 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 1
12:54:51 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:51 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 1 elements
12:54:51 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:51 INFO opendrift.models.chemicaldrift:1861: partitioning: [132, 0, 249, 119, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:51 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:51 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:51 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:51 DEBUG opendrift.models.basemodel:755: Lifting 43 elements to seafloor.
12:54:51 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:51 INFO opendrift.models.basemodel:2038: 2024-11-02 14:24:02.760182 - step 52 of 96 - 500 active elements (0 deactivated)
12:54:51 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:51 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:51 DEBUG opendrift.models.basemodel:2057: 57.55194026136295 <- latitude -> 57.73469488537275
12:54:51 DEBUG opendrift.models.basemodel:2062: 10.507927244297788 <- longitude -> 10.804697015907568
12:54:51 DEBUG opendrift.models.basemodel:2067: -27.898296356201172 <- z -> -0.20776819603589625
12:54:51 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:51 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:51 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:51 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:51 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:51 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:51 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:51 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:51 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-02 14:00:00 (before)
2024-11-02 15:00:00 (after)
12:54:52 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
12:54:52 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:54:52 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:54:52 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:54:52 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:54:52 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'upward_sea_water_velocity', 'y_sea_water_velocity', 'sea_water_temperature', 'sea_water_salinity']
12:54:52 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 54x36x7) for time after (2024-11-02 15:00:00)
12:54:52 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-02 14:00:00) in space (linearNDFast)
12:54:52 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:52 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-02 15:00:00) in space (linearNDFast)
12:54:52 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:52 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-02 14:00:00, weight 0.60) and
after (2024-11-02 15:00:00, weight 0.40) in time
12:54:52 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:52 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.195314020558634 degrees.
12:54:52 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.195314020558634 degrees.
12:54:52 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:52 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:52 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:52 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:52 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:52 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:52 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:52 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:52 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:52 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:52 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:52 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:52 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:52 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:52 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:52 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:52 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:52 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:52 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:52 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:52 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:52 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:52 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:52 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:52 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:52 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:52 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:52 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:52 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:52 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0853255 (min) 0.165809 (max)
12:54:52 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.161188 (min) 0.130799 (max)
12:54:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.0314115 (min) 0.0485887 (max)
12:54:52 DEBUG opendrift.models.basemodel.environment:905: x_wind: 5.14719 (min) 5.89069 (max)
12:54:52 DEBUG opendrift.models.basemodel.environment:905: y_wind: 1.70441 (min) 2.17037 (max)
12:54:52 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:52 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 29.7604 (max)
12:54:52 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:52 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.1471 (min) 12.6087 (max)
12:54:52 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0116 (min) 33.1769 (max)
12:54:52 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -5.85403e-05 (min) 0.000588876 (max)
12:54:52 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:52 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:52 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:52 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:52 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:52 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:52 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:52 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:52 DEBUG opendrift.models.basemodel:750: No elements hit seafloor.
12:54:52 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:52 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:52 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:52 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:52 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 2
12:54:52 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0]
12:54:52 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3]
12:54:52 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 466. 0.]
[ 0. 0. 0. 0. 0.]
[140. 0. 0. 378. 0.]
[ 7. 0. 716. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:52 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:52 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:52 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:52 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:52 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.027526094670843992
12:54:52 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:52 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:52 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:52 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:52 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:52 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
12:54:52 DEBUG opendrift.models.basemodel:755: Lifting 2 elements to seafloor.
12:54:52 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:52 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:52 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:52 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:52 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:52 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:52 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:52 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:52 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
12:54:52 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 1
12:54:52 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:52 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 1 elements
12:54:52 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:52 INFO opendrift.models.chemicaldrift:1861: partitioning: [130, 0, 250, 120, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:52 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:52 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:52 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:52 DEBUG opendrift.models.basemodel:750: No elements hit seafloor.
12:54:52 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:52 INFO opendrift.models.basemodel:2038: 2024-11-02 14:54:02.760182 - step 53 of 96 - 500 active elements (0 deactivated)
12:54:52 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:52 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:52 DEBUG opendrift.models.basemodel:2057: 57.55194026136295 <- latitude -> 57.73469488537275
12:54:52 DEBUG opendrift.models.basemodel:2062: 10.507927244297788 <- longitude -> 10.808174086537477
12:54:52 DEBUG opendrift.models.basemodel:2067: -27.898296356201172 <- z -> 0.0
12:54:52 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:52 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:52 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:52 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:52 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:52 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:52 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:52 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:52 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-02 14:00:00 (before)
2024-11-02 15:00:00 (after)
12:54:52 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-02 14:00:00) in space (linearNDFast)
12:54:52 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:52 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-02 15:00:00) in space (linearNDFast)
12:54:52 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:52 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-02 14:00:00, weight 0.10) and
after (2024-11-02 15:00:00, weight 0.90) in time
12:54:52 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:52 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.19183694429101 degrees.
12:54:52 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.19183694429101 degrees.
12:54:52 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:52 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:52 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:52 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:52 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:52 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:52 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:52 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:52 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:52 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:52 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:52 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:52 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:52 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:52 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:52 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:52 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:52 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:52 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:52 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:52 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:52 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:52 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:52 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:52 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:52 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:52 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:52 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:52 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:52 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.095421 (min) 0.152348 (max)
12:54:52 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.139684 (min) 0.127458 (max)
12:54:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.0666935 (min) 0.077 (max)
12:54:52 DEBUG opendrift.models.basemodel.environment:905: x_wind: 5.53757 (min) 6.27311 (max)
12:54:52 DEBUG opendrift.models.basemodel.environment:905: y_wind: 2.17473 (min) 2.91253 (max)
12:54:52 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:52 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 29.6783 (max)
12:54:52 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:52 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.1356 (min) 12.6029 (max)
12:54:52 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0123 (min) 33.1803 (max)
12:54:52 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -5.75482e-05 (min) 0.000723751 (max)
12:54:52 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:52 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:52 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:52 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:52 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:52 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:52 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:52 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:52 DEBUG opendrift.models.basemodel:750: No elements hit seafloor.
12:54:52 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:52 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:52 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:52 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:52 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 11
12:54:52 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 0 0 2 2 2 0 0 0 2 2]
12:54:52 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 3 3 0 0 0 3 3 3 0 0]
12:54:52 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 471. 0.]
[ 0. 0. 0. 0. 0.]
[146. 0. 0. 378. 0.]
[ 7. 0. 717. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:52 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:52 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:52 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:52 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:52 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.0331040977514505
12:54:52 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:52 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:52 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:52 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:52 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:52 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:52 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:52 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:52 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:52 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:52 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:52 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
12:54:52 DEBUG opendrift.models.basemodel:755: Lifting 2 elements to seafloor.
12:54:52 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:52 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:52 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:52 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:52 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:52 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:52 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
12:54:52 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
12:54:52 DEBUG opendrift.models.basemodel:755: Lifting 2 elements to seafloor.
12:54:52 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 0
12:54:52 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:52 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:52 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:52 INFO opendrift.models.chemicaldrift:1861: partitioning: [131, 0, 244, 125, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:52 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:52 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:52 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:52 DEBUG opendrift.models.basemodel:750: No elements hit seafloor.
12:54:52 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:52 INFO opendrift.models.basemodel:2038: 2024-11-02 15:24:02.760182 - step 54 of 96 - 500 active elements (0 deactivated)
12:54:52 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:52 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:52 DEBUG opendrift.models.basemodel:2057: 57.55194026136295 <- latitude -> 57.73469488537275
12:54:52 DEBUG opendrift.models.basemodel:2062: 10.507927244297784 <- longitude -> 10.811536004492092
12:54:52 DEBUG opendrift.models.basemodel:2067: -27.898296356201172 <- z -> -0.09734920460722629
12:54:52 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:52 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:52 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:52 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:52 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:52 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:52 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:52 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:52 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-02 15:00:00 (before)
2024-11-02 16:00:00 (after)
12:54:53 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
12:54:53 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:54:53 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:54:53 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:54:53 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:54:53 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'upward_sea_water_velocity', 'y_sea_water_velocity', 'sea_water_temperature', 'sea_water_salinity']
12:54:53 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 54x36x7) for time after (2024-11-02 16:00:00)
12:54:53 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-02 15:00:00) in space (linearNDFast)
12:54:53 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:53 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-02 16:00:00) in space (linearNDFast)
12:54:53 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:53 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-02 15:00:00, weight 0.60) and
after (2024-11-02 16:00:00, weight 0.40) in time
12:54:53 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:53 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.18847503073722 degrees.
12:54:53 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.18847503073722 degrees.
12:54:53 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:53 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:53 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:53 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:53 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:53 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:53 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:53 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:53 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:53 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:53 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:53 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:53 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:53 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:53 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:53 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:53 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:53 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:53 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:53 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:53 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:53 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:53 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:53 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:53 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:53 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:53 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:53 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:53 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:53 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0859256 (min) 0.146104 (max)
12:54:53 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.147005 (min) 0.145943 (max)
12:54:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.0647627 (min) 0.0803373 (max)
12:54:53 DEBUG opendrift.models.basemodel.environment:905: x_wind: 5.13829 (min) 6.50433 (max)
12:54:53 DEBUG opendrift.models.basemodel.environment:905: y_wind: 2.7831 (min) 3.55956 (max)
12:54:53 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:53 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 29.8165 (max)
12:54:53 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:53 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.1319 (min) 12.5957 (max)
12:54:53 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0136 (min) 33.2595 (max)
12:54:53 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -7.56154e-05 (min) 0.000712131 (max)
12:54:53 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:53 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:53 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:53 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:53 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:53 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:53 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:53 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:53 DEBUG opendrift.models.basemodel:750: No elements hit seafloor.
12:54:53 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:53 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:53 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:53 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:53 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 9
12:54:53 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 2 2 2 0 0 2 0]
12:54:53 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 0 0 0 3 3 0 3]
12:54:53 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 476. 0.]
[ 0. 0. 0. 0. 0.]
[150. 0. 0. 378. 0.]
[ 7. 0. 717. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:53 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:53 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:53 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:53 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:53 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.03684003864758817
12:54:53 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:53 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:53 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:53 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
12:54:53 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:53 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:53 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:53 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:53 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:53 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:53 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:53 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:54:53 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:53 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:53 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:53 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:53 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:53 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
12:54:53 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
12:54:53 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
12:54:53 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:53 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:53 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:53 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:53 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:53 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:53 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:53 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:53 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:53 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:53 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:53 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:53 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:53 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:53 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:53 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:53 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:53 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:53 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:53 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:53 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:53 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:53 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:53 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 1
12:54:53 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:53 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 1 elements
12:54:53 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:53 INFO opendrift.models.chemicaldrift:1861: partitioning: [130, 0, 241, 129, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:53 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:53 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:53 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:53 DEBUG opendrift.models.basemodel:750: No elements hit seafloor.
12:54:53 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:53 INFO opendrift.models.basemodel:2038: 2024-11-02 15:54:02.760182 - step 55 of 96 - 500 active elements (0 deactivated)
12:54:53 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:53 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:53 DEBUG opendrift.models.basemodel:2057: 57.55194026136295 <- latitude -> 57.73231897103816
12:54:53 DEBUG opendrift.models.basemodel:2062: 10.507927244297784 <- longitude -> 10.814150299791384
12:54:53 DEBUG opendrift.models.basemodel:2067: -26.836649344955717 <- z -> -0.02546945458800709
12:54:53 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:53 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:53 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:53 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:53 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:53 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:53 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:53 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:53 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-02 15:00:00 (before)
2024-11-02 16:00:00 (after)
12:54:53 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-02 15:00:00) in space (linearNDFast)
12:54:53 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:53 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-02 16:00:00) in space (linearNDFast)
12:54:53 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:53 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-02 15:00:00, weight 0.10) and
after (2024-11-02 16:00:00, weight 0.90) in time
12:54:53 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:53 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.18586073427156 degrees.
12:54:54 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.18586073427156 degrees.
12:54:54 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:54 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:54 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:54 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:54 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:54 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:54 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:54 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:54 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:54 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:54 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:54 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:54 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:54 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:54 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:54 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:54 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:54 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:54 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:54 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:54 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:54 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:54 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:54 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:54 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:54 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:54 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:54 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:54 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:54 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.111484 (min) 0.121821 (max)
12:54:54 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.128252 (min) 0.166855 (max)
12:54:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.0571343 (min) 0.0816683 (max)
12:54:54 DEBUG opendrift.models.basemodel.environment:905: x_wind: 4.4599 (min) 6.7765 (max)
12:54:54 DEBUG opendrift.models.basemodel.environment:905: y_wind: 3.36306 (min) 4.17943 (max)
12:54:54 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:54 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 29.7363 (max)
12:54:54 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:54 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.1252 (min) 12.5933 (max)
12:54:54 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0152 (min) 33.2908 (max)
12:54:54 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.00011068 (min) 0.000535182 (max)
12:54:54 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:54 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:54 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:54 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:54 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:54 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:54 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:54 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:54 DEBUG opendrift.models.basemodel:750: No elements hit seafloor.
12:54:54 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:54 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:54 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:54 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:54 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 5
12:54:54 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 0 2]
12:54:54 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 3 0]
12:54:54 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 480. 0.]
[ 0. 0. 0. 0. 0.]
[151. 0. 0. 378. 0.]
[ 7. 0. 718. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:54 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:54 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:54 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:54 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:54 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.04190394001297115
12:54:54 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:54 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:54 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:54 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:54:54 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:54 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:54 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:54 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:54 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:54 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:54 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:54 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:54:54 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:54:54 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:54:54 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:54 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:54 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:54 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:54 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:54 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:54 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:54 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:54 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:54 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:54 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:54 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:54 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:54 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:54 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:54 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:54 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:54 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:54 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
12:54:54 DEBUG opendrift.models.basemodel:755: Lifting 2 elements to seafloor.
12:54:54 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:54:54 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:54 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:54 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:54 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:54 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:54:54 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:54 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:54:54 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:54:54 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:54:54 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:54:54 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:54:54 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:54 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 1
12:54:54 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:54 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 1 elements
12:54:54 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:54 INFO opendrift.models.chemicaldrift:1861: partitioning: [127, 0, 241, 132, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:54 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:54 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:54 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:54 DEBUG opendrift.models.basemodel:750: No elements hit seafloor.
12:54:54 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:54 INFO opendrift.models.basemodel:2038: 2024-11-02 16:24:02.760182 - step 56 of 96 - 500 active elements (0 deactivated)
12:54:54 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:54 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:54 DEBUG opendrift.models.basemodel:2057: 57.55194026136295 <- latitude -> 57.730246148198944
12:54:54 DEBUG opendrift.models.basemodel:2062: 10.507927244297784 <- longitude -> 10.817007657133123
12:54:54 DEBUG opendrift.models.basemodel:2067: -27.901130919051205 <- z -> -0.05697187422289446
12:54:54 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:54 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:54 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:54 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:54 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:54 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:54 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:54 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:54 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-02 16:00:00 (before)
2024-11-02 17:00:00 (after)
12:54:55 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
12:54:55 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:54:55 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:54:55 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:54:55 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:54:55 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'upward_sea_water_velocity', 'y_sea_water_velocity', 'sea_water_temperature', 'sea_water_salinity']
12:54:55 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 54x37x7) for time after (2024-11-02 17:00:00)
12:54:55 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-02 16:00:00) in space (linearNDFast)
12:54:55 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:55 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-02 17:00:00) in space (linearNDFast)
12:54:55 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:55 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-02 16:00:00, weight 0.60) and
after (2024-11-02 17:00:00, weight 0.40) in time
12:54:55 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:55 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.1830033624003 degrees.
12:54:55 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.1830033624003 degrees.
12:54:55 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:55 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:55 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:55 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:55 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:55 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:55 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:55 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:55 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:55 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:55 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:55 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:55 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:55 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:55 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:55 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:55 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:55 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:55 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:55 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:55 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:55 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:55 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:55 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:55 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:55 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:55 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:55 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:55 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:55 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.125216 (min) 0.125121 (max)
12:54:55 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0750203 (min) 0.207823 (max)
12:54:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.0303161 (min) 0.0557833 (max)
12:54:55 DEBUG opendrift.models.basemodel.environment:905: x_wind: 4.24637 (min) 6.81122 (max)
12:54:55 DEBUG opendrift.models.basemodel.environment:905: y_wind: 3.60832 (min) 4.06494 (max)
12:54:55 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:55 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 29.476 (max)
12:54:55 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:55 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.1131 (min) 12.5929 (max)
12:54:55 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0165 (min) 33.269 (max)
12:54:55 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000122905 (min) 0.000453442 (max)
12:54:55 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:55 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:55 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:55 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:55 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:55 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:55 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:55 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:55 DEBUG opendrift.models.basemodel:750: No elements hit seafloor.
12:54:55 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:55 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:55 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:55 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:55 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 8
12:54:55 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 2 0 0 0 0 0 0]
12:54:55 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 0 3 3 3 3 3 3]
12:54:55 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 487. 0.]
[ 0. 0. 0. 0. 0.]
[152. 0. 0. 378. 0.]
[ 7. 0. 719. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:55 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:55 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:55 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:55 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:55 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.04374140201109799
12:54:55 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:55 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:55 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:55 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:55 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:55 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:55 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:55 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:55 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:55 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:55 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:55 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:55 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:55 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:55 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:55 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:55 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:55 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:55 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:55 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:55 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:55 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:54:55 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 1
12:54:55 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:55 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 1 elements
12:54:55 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:55 INFO opendrift.models.chemicaldrift:1861: partitioning: [121, 0, 241, 138, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:55 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:55 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:55 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:55 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:55 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:55 INFO opendrift.models.basemodel:2038: 2024-11-02 16:54:02.760182 - step 57 of 96 - 500 active elements (0 deactivated)
12:54:55 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:55 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:55 DEBUG opendrift.models.basemodel:2057: 57.55194026136295 <- latitude -> 57.73096580601897
12:54:55 DEBUG opendrift.models.basemodel:2062: 10.507927244297784 <- longitude -> 10.820781709513506
12:54:55 DEBUG opendrift.models.basemodel:2067: -27.987463726432846 <- z -> -0.09159979697441498
12:54:55 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:55 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:55 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:55 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:55 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:55 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:55 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:55 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:55 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-02 16:00:00 (before)
2024-11-02 17:00:00 (after)
12:54:55 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-02 16:00:00) in space (linearNDFast)
12:54:55 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:55 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-02 17:00:00) in space (linearNDFast)
12:54:55 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:55 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-02 16:00:00, weight 0.10) and
after (2024-11-02 17:00:00, weight 0.90) in time
12:54:55 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:55 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.17922932561817 degrees.
12:54:55 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.17922932561817 degrees.
12:54:55 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:55 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:55 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:55 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:55 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:55 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:55 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:55 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:55 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:55 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:55 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:55 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:55 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:55 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:55 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:55 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:55 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:55 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:55 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:55 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:55 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:55 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:55 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:55 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:55 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:55 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:55 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:55 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:55 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:55 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.135257 (min) 0.13708 (max)
12:54:55 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.00557713 (min) 0.186882 (max)
12:54:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.00191062 (min) 0.0232682 (max)
12:54:55 DEBUG opendrift.models.basemodel.environment:905: x_wind: 4.14794 (min) 6.81027 (max)
12:54:55 DEBUG opendrift.models.basemodel.environment:905: y_wind: 3.10852 (min) 4.0818 (max)
12:54:55 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:55 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 29.7149 (max)
12:54:55 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:55 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.0997 (min) 12.5929 (max)
12:54:55 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0158 (min) 33.0047 (max)
12:54:55 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000172209 (min) 0.000450248 (max)
12:54:55 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:55 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:55 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:55 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:55 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:55 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:55 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:55 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:55 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:55 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:55 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:55 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:55 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:55 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 9
12:54:55 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 2 2 2 2 0 0 0 0]
12:54:55 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 0 0 0 0 3 3 3 3]
12:54:55 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 492. 0.]
[ 0. 0. 0. 0. 0.]
[156. 0. 0. 378. 0.]
[ 7. 0. 720. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:55 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:55 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:55 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:55 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:55 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.044724616819475836
12:54:55 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:55 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:55 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:55 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:55 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:55 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:55 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:55 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:55 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:55 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:55 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:55 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:55 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:55 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
12:54:55 DEBUG opendrift.models.basemodel:755: Lifting 2 elements to seafloor.
12:54:55 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:55 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:55 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:55 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:55 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:55 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:55 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:55 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:55 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
12:54:55 DEBUG opendrift.models.basemodel:755: Lifting 2 elements to seafloor.
12:54:55 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
12:54:55 DEBUG opendrift.models.basemodel:755: Lifting 2 elements to seafloor.
12:54:55 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
12:54:55 DEBUG opendrift.models.basemodel:755: Lifting 2 elements to seafloor.
12:54:55 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
12:54:55 DEBUG opendrift.models.basemodel:755: Lifting 2 elements to seafloor.
12:54:55 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
12:54:55 DEBUG opendrift.models.basemodel:755: Lifting 2 elements to seafloor.
12:54:55 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
12:54:55 DEBUG opendrift.models.basemodel:755: Lifting 2 elements to seafloor.
12:54:55 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:55 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:55 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:55 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:55 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:55 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:55 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:55 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:55 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:55 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:55 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:636: 3 elements reached seafloor, interacting with bottom
12:54:55 DEBUG opendrift.models.basemodel:755: Lifting 3 elements to seafloor.
12:54:55 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:55 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:55 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
12:54:55 DEBUG opendrift.models.basemodel:755: Lifting 2 elements to seafloor.
12:54:55 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:55 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:55 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
12:54:55 DEBUG opendrift.models.basemodel:755: Lifting 2 elements to seafloor.
12:54:55 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
12:54:55 DEBUG opendrift.models.basemodel:755: Lifting 2 elements to seafloor.
12:54:55 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:55 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:55 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:55 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:55 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:55 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:54:55 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:55 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 1
12:54:55 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:55 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 1 elements
12:54:55 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:55 INFO opendrift.models.chemicaldrift:1861: partitioning: [120, 0, 238, 142, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:55 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:55 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:55 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:55 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:54:55 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:55 INFO opendrift.models.basemodel:2038: 2024-11-02 17:24:02.760182 - step 58 of 96 - 500 active elements (0 deactivated)
12:54:55 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:55 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:55 DEBUG opendrift.models.basemodel:2057: 57.55194026136295 <- latitude -> 57.73260719063008
12:54:55 DEBUG opendrift.models.basemodel:2062: 10.507927244297784 <- longitude -> 10.822465937050191
12:54:55 DEBUG opendrift.models.basemodel:2067: -27.634235949635165 <- z -> -0.1627957124870885
12:54:55 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:55 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:55 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:55 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:55 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:55 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:55 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:55 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:55 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-02 17:00:00 (before)
2024-11-02 18:00:00 (after)
12:54:57 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
12:54:57 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:54:57 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:54:57 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:54:57 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:54:57 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'upward_sea_water_velocity', 'y_sea_water_velocity', 'sea_water_temperature', 'sea_water_salinity']
12:54:57 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 54x37x7) for time after (2024-11-02 18:00:00)
12:54:57 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-02 17:00:00) in space (linearNDFast)
12:54:57 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:57 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-02 18:00:00) in space (linearNDFast)
12:54:57 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:57 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-02 17:00:00, weight 0.60) and
after (2024-11-02 18:00:00, weight 0.40) in time
12:54:57 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:57 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.177545088208355 degrees.
12:54:57 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.177545088208355 degrees.
12:54:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:57 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:57 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:57 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:57 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:57 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:57 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:57 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.132761 (min) 0.121808 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0042723 (min) 0.230681 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.0433602 (min) -0.0196673 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: x_wind: 4.33909 (min) 7.78963 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: y_wind: 2.15743 (min) 3.88815 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 29.7462 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.085 (min) 12.6011 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0137 (min) 33.2664 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000161207 (min) 0.0004101 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:57 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 142 elements to seafloor.
12:54:57 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:57 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:57 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:57 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:57 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 7
12:54:57 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 3 2 0 2 2]
12:54:57 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 0 0 3 0 0]
12:54:57 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 495. 0.]
[ 0. 0. 0. 0. 0.]
[159. 0. 0. 378. 0.]
[ 8. 0. 721. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:57 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:57 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:57 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:57 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:57 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.04943817321812917
12:54:57 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:57 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:57 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:57 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 144 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 144 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 145 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 145 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 145 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 145 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 146 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 146 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 146 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 146 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 146 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 146 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 146 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 146 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 146 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 146 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 146 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 146 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 146 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 146 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 146 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 146 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 146 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 146 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 146 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 146 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 147 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 147 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 147 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 147 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 147 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 147 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 147 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 147 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 148 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 148 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 148 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 148 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 149 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 149 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 149 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 149 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 149 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 149 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 149 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 149 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 149 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 149 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 149 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 149 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 149 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 149 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 149 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 149 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 149 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 149 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 150 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 150 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 150 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 150 elements to seafloor.
12:54:57 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 3
12:54:57 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:57 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 3 elements
12:54:57 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:57 INFO opendrift.models.chemicaldrift:1861: partitioning: [121, 0, 232, 147, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:57 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:57 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:57 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 147 elements to seafloor.
12:54:57 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:57 INFO opendrift.models.basemodel:2038: 2024-11-02 17:54:02.760182 - step 59 of 96 - 500 active elements (0 deactivated)
12:54:57 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:57 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:57 DEBUG opendrift.models.basemodel:2057: 57.55194026136295 <- latitude -> 57.73523121004887
12:54:57 DEBUG opendrift.models.basemodel:2062: 10.507927244297788 <- longitude -> 10.825775326298254
12:54:57 DEBUG opendrift.models.basemodel:2067: -28.463413294671838 <- z -> 0.0
12:54:57 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:57 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-02 17:00:00 (before)
2024-11-02 18:00:00 (after)
12:54:57 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-02 17:00:00) in space (linearNDFast)
12:54:57 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:57 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-02 18:00:00) in space (linearNDFast)
12:54:57 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:57 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-02 17:00:00, weight 0.10) and
after (2024-11-02 18:00:00, weight 0.90) in time
12:54:57 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:57 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.17423570486884 degrees.
12:54:57 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.17423570486884 degrees.
12:54:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:57 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:57 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:57 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:57 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:57 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:57 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:57 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.146199 (min) 0.156586 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0613359 (min) 0.237479 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.09015 (min) -0.0645413 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: x_wind: 4.60195 (min) 9.56794 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: y_wind: 1.17873 (min) 3.59035 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 29.9872 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.07 (min) 12.6222 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0115 (min) 33.256 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000196819 (min) 0.000360802 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:57 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 147 elements to seafloor.
12:54:57 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:57 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:57 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:57 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:57 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 6
12:54:57 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 0 2 0]
12:54:57 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 3 0 3]
12:54:57 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 500. 0.]
[ 0. 0. 0. 0. 0.]
[160. 0. 0. 384. 0.]
[ 8. 0. 724. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:57 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:57 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:57 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:57 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:57 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.06775953977592646
12:54:57 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:57 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:57 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:57 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 152 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 152 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 152 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 152 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 153 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 153 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 153 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 153 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 153 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 153 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 153 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 153 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 153 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 153 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 154 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 154 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 154 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 154 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 154 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 154 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 154 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 154 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 155 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 155 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 156 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 156 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 156 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 156 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 156 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 156 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 156 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 156 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 156 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 156 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 156 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 156 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 156 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 156 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 156 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 156 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 156 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 156 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 156 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 156 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 156 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 156 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 156 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 156 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 156 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 156 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 156 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 156 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 156 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 156 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 156 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 156 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 156 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 156 elements to seafloor.
12:54:57 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:54:57 DEBUG opendrift.models.oceandrift:636: 156 elements reached seafloor, interacting with bottom
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 156 elements to seafloor.
12:54:57 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 28
12:54:57 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:57 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 28 elements
12:54:57 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:57 INFO opendrift.models.chemicaldrift:1861: partitioning: [117, 0, 255, 128, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:57 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:57 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:57 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:57 DEBUG opendrift.models.basemodel:755: Lifting 129 elements to seafloor.
12:54:57 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:57 INFO opendrift.models.basemodel:2038: 2024-11-02 18:24:02.760182 - step 60 of 96 - 500 active elements (0 deactivated)
12:54:57 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:57 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:57 DEBUG opendrift.models.basemodel:2057: 57.55194026136295 <- latitude -> 57.7385015501923
12:54:57 DEBUG opendrift.models.basemodel:2062: 10.507927244297788 <- longitude -> 10.826962108651518
12:54:57 DEBUG opendrift.models.basemodel:2067: -27.60655557621735 <- z -> -0.0018194577435436815
12:54:57 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:57 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-02 18:00:00 (before)
2024-11-02 19:00:00 (after)
12:54:59 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
12:54:59 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:54:59 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:54:59 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:54:59 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:54:59 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'upward_sea_water_velocity', 'y_sea_water_velocity', 'sea_water_temperature', 'sea_water_salinity']
12:54:59 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 54x37x7) for time after (2024-11-02 19:00:00)
12:54:59 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-02 18:00:00) in space (linearNDFast)
12:54:59 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:59 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-02 19:00:00) in space (linearNDFast)
12:54:59 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:59 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-02 18:00:00, weight 0.60) and
after (2024-11-02 19:00:00, weight 0.40) in time
12:54:59 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:59 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.17304891930376 degrees.
12:54:59 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.17304891930376 degrees.
12:54:59 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:59 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:59 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:59 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:59 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:59 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:59 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:59 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:59 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:59 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:59 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:59 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:59 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:59 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:59 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:59 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:59 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:59 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:59 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:59 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:59 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:59 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:59 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:59 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:59 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:59 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:59 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:59 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.130898 (min) 0.20681 (max)
12:54:59 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0179978 (min) 0.260432 (max)
12:54:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.135586 (min) -0.109557 (max)
12:54:59 DEBUG opendrift.models.basemodel.environment:905: x_wind: 4.45505 (min) 10.1593 (max)
12:54:59 DEBUG opendrift.models.basemodel.environment:905: y_wind: 1.05022 (min) 3.74446 (max)
12:54:59 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:59 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 30.4592 (max)
12:54:59 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:59 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.0548 (min) 12.6229 (max)
12:54:59 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0081 (min) 33.1516 (max)
12:54:59 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000178091 (min) 0.000295221 (max)
12:54:59 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:59 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:59 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:59 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:59 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:59 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:59 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:59 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 128 elements to seafloor.
12:54:59 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:59 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:59 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:59 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:59 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 9
12:54:59 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 2 0 2 0 0 2 0 2]
12:54:59 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 0 3 0 3 3 0 3 0]
12:54:59 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 505. 0.]
[ 0. 0. 0. 0. 0.]
[164. 0. 0. 388. 0.]
[ 8. 0. 752. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:59 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:59 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:59 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:59 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:59 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.07584664618242301
12:54:59 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:59 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:59 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:59 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 133 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 133 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 134 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 134 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 135 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 135 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 135 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 135 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 135 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 135 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 135 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 135 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 135 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 135 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 138 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 138 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 138 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 138 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 139 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 139 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 139 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 139 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 139 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 139 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 139 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 139 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 139 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 139 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 139 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 139 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 139 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 139 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 139 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 139 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 140 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 140 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 141 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 141 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 141 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 141 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 141 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 141 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 141 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 141 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 141 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 141 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 142 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 142 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 142 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 142 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 144 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 144 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 145 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 145 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 145 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 145 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 145 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 145 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 145 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 145 elements to seafloor.
12:54:59 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 47
12:54:59 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:59 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 47 elements
12:54:59 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:59 INFO opendrift.models.chemicaldrift:1861: partitioning: [116, 0, 286, 98, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:59 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:59 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:59 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 99 elements to seafloor.
12:54:59 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:59 INFO opendrift.models.basemodel:2038: 2024-11-02 18:54:02.760182 - step 61 of 96 - 500 active elements (0 deactivated)
12:54:59 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:59 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:59 DEBUG opendrift.models.basemodel:2057: 57.55194026136295 <- latitude -> 57.74099451427405
12:54:59 DEBUG opendrift.models.basemodel:2062: 10.507927244297784 <- longitude -> 10.828558111741007
12:54:59 DEBUG opendrift.models.basemodel:2067: -26.595206768749364 <- z -> -0.058665183402733384
12:54:59 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:59 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:59 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:59 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:59 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:59 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:59 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:59 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:59 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-02 18:00:00 (before)
2024-11-02 19:00:00 (after)
12:54:59 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-02 18:00:00) in space (linearNDFast)
12:54:59 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:59 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-02 19:00:00) in space (linearNDFast)
12:54:59 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:59 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-02 18:00:00, weight 0.10) and
after (2024-11-02 19:00:00, weight 0.90) in time
12:54:59 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:54:59 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.17145291700773 degrees.
12:54:59 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.17145291700773 degrees.
12:54:59 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:59 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:59 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:59 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:59 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:59 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:59 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:59 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:54:59 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:59 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:59 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:59 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:59 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:59 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:59 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:59 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:59 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:59 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:54:59 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:59 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:59 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:59 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:59 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:59 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:54:59 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:54:59 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:54:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:54:59 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:59 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0656837 (min) 0.237026 (max)
12:54:59 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0596398 (min) 0.250711 (max)
12:54:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.184441 (min) -0.154458 (max)
12:54:59 DEBUG opendrift.models.basemodel.environment:905: x_wind: 4.20669 (min) 10.5305 (max)
12:54:59 DEBUG opendrift.models.basemodel.environment:905: y_wind: 1.08416 (min) 4.01046 (max)
12:54:59 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:59 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 30.932 (max)
12:54:59 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:54:59 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.0397 (min) 12.6174 (max)
12:54:59 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0044 (min) 33.3845 (max)
12:54:59 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000180068 (min) 0.000276053 (max)
12:54:59 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:54:59 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:54:59 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:54:59 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:54:59 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:54:59 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:54:59 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:59 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 99 elements to seafloor.
12:54:59 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:59 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:54:59 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:54:59 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:54:59 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 8
12:54:59 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 2 0 0 3 2 0]
12:54:59 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 0 3 3 0 0 3]
12:54:59 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 510. 0.]
[ 0. 0. 0. 0. 0.]
[166. 0. 0. 400. 0.]
[ 9. 0. 799. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:54:59 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:54:59 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:59 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:54:59 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:54:59 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.08140580367999528
12:54:59 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:59 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:54:59 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:54:59 DEBUG opendrift.models.oceandrift:618: 38 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 103 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 103 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 103 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 103 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 103 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 103 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 103 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 103 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 103 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 103 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 104 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 104 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 104 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 104 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 105 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 105 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 105 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 105 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 105 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 105 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 105 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 105 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 105 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 105 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 105 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 105 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 109 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 109 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 109 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 109 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 109 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 109 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 109 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 109 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 109 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 109 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 110 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 110 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 110 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 110 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 110 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 110 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 110 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 110 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 110 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 110 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 113 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 113 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 113 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 113 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 114 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 114 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 114 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 114 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 114 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 114 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 115 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 115 elements to seafloor.
12:54:59 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:54:59 DEBUG opendrift.models.oceandrift:636: 115 elements reached seafloor, interacting with bottom
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 115 elements to seafloor.
12:54:59 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 30
12:54:59 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:54:59 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 30 elements
12:54:59 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:54:59 INFO opendrift.models.chemicaldrift:1861: partitioning: [114, 0, 301, 85, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:54:59 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:54:59 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:54:59 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:54:59 DEBUG opendrift.models.basemodel:755: Lifting 87 elements to seafloor.
12:54:59 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:59 INFO opendrift.models.basemodel:2038: 2024-11-02 19:24:02.760182 - step 62 of 96 - 500 active elements (0 deactivated)
12:54:59 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:59 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:59 DEBUG opendrift.models.basemodel:2057: 57.55194026136295 <- latitude -> 57.74447981055248
12:54:59 DEBUG opendrift.models.basemodel:2062: 10.507927244297782 <- longitude -> 10.829522259891151
12:54:59 DEBUG opendrift.models.basemodel:2067: -27.242672465785752 <- z -> -0.08956634391756285
12:54:59 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:59 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:59 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:54:59 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:59 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:54:59 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:59 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:54:59 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:54:59 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-02 19:00:00 (before)
2024-11-02 20:00:00 (after)
12:55:02 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
12:55:02 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:55:02 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:55:02 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:55:02 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:55:02 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'upward_sea_water_velocity', 'y_sea_water_velocity', 'sea_water_temperature', 'sea_water_salinity']
12:55:02 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 55x37x7) for time after (2024-11-02 20:00:00)
12:55:02 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-02 19:00:00) in space (linearNDFast)
12:55:02 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:02 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-02 20:00:00) in space (linearNDFast)
12:55:02 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:02 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-02 19:00:00, weight 0.60) and
after (2024-11-02 20:00:00, weight 0.40) in time
12:55:02 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:55:02 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.17048876067474 degrees.
12:55:02 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.17048876067474 degrees.
12:55:02 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:02 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:02 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:02 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:02 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:02 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:02 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:02 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:55:02 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:02 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:02 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:02 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:02 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:02 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:02 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:02 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:02 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:02 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:55:02 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:02 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:02 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:02 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:02 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:02 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:02 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:55:02 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:55:02 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:55:02 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:55:02 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:02 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.133717 (min) 0.198679 (max)
12:55:02 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0262423 (min) 0.223447 (max)
12:55:02 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.22644 (min) -0.19282 (max)
12:55:02 DEBUG opendrift.models.basemodel.environment:905: x_wind: 4.14499 (min) 10.4525 (max)
12:55:02 DEBUG opendrift.models.basemodel.environment:905: y_wind: 1.28891 (min) 4.16291 (max)
12:55:02 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:02 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 31.5536 (max)
12:55:02 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:55:02 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.0234 (min) 12.6186 (max)
12:55:02 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0006 (min) 33.2644 (max)
12:55:02 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000176485 (min) 0.00028207 (max)
12:55:02 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:55:02 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:55:02 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:55:02 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:55:02 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:55:02 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:55:02 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:02 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 86 elements to seafloor.
12:55:02 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:02 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:55:02 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:55:02 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:55:02 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 4
12:55:02 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 0 3 0]
12:55:02 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 3 0 3]
12:55:02 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 512. 0.]
[ 0. 0. 0. 0. 0.]
[167. 0. 0. 413. 0.]
[ 10. 0. 829. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:55:02 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:55:02 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:02 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:55:02 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:55:02 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.08048697382811715
12:55:02 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:02 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:55:02 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:55:02 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 86 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 86 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 87 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 87 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 87 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 87 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 87 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 87 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 37 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 88 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 88 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 32 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 89 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 89 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 33 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 90 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 90 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 91 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 91 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 91 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 91 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 92 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 92 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 92 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 92 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 93 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 93 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 93 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 93 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 93 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 93 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 93 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 93 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 93 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 93 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 93 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 93 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 93 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 93 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 93 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 93 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 93 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 93 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 93 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 93 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 36 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 93 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 93 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 94 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 94 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 95 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 95 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 95 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 95 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 95 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 95 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 95 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 95 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 95 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 95 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 95 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 95 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 95 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 95 elements to seafloor.
12:55:02 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 10
12:55:02 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:55:02 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 10 elements
12:55:02 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:02 INFO opendrift.models.chemicaldrift:1861: partitioning: [114, 0, 301, 85, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:55:02 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:55:02 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:55:02 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 85 elements to seafloor.
12:55:02 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:02 INFO opendrift.models.basemodel:2038: 2024-11-02 19:54:02.760182 - step 63 of 96 - 500 active elements (0 deactivated)
12:55:02 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:02 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:02 DEBUG opendrift.models.basemodel:2057: 57.55194026136295 <- latitude -> 57.747438460450134
12:55:02 DEBUG opendrift.models.basemodel:2062: 10.507927244297782 <- longitude -> 10.830979870042016
12:55:02 DEBUG opendrift.models.basemodel:2067: -26.7667293548584 <- z -> 0.0
12:55:02 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:02 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:02 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:55:02 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:02 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:55:02 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:02 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:02 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:55:02 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-02 19:00:00 (before)
2024-11-02 20:00:00 (after)
12:55:02 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-02 19:00:00) in space (linearNDFast)
12:55:02 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:02 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-02 20:00:00) in space (linearNDFast)
12:55:02 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:02 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-02 19:00:00, weight 0.10) and
after (2024-11-02 20:00:00, weight 0.90) in time
12:55:02 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:55:02 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.16903115495657 degrees.
12:55:02 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.16903115495657 degrees.
12:55:02 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:02 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:02 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:02 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:02 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:02 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:02 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:02 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:55:02 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:02 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:02 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:02 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:02 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:02 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:02 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:02 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:02 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:02 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:55:02 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:02 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:02 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:02 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:02 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:02 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:02 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:55:02 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:55:02 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:55:02 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:55:02 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:02 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.09922 (min) 0.215525 (max)
12:55:02 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.039837 (min) 0.188774 (max)
12:55:02 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.266454 (min) -0.22939 (max)
12:55:02 DEBUG opendrift.models.basemodel.environment:905: x_wind: 4.05677 (min) 10.3432 (max)
12:55:02 DEBUG opendrift.models.basemodel.environment:905: y_wind: 1.51869 (min) 4.30776 (max)
12:55:02 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:02 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 32.3475 (max)
12:55:02 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:55:02 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 12.0068 (min) 12.6189 (max)
12:55:02 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 31.9968 (min) 33.0937 (max)
12:55:02 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.00017785 (min) 0.000307441 (max)
12:55:02 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:55:02 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:55:02 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:55:02 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:55:02 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:55:02 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:55:02 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:02 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 85 elements to seafloor.
12:55:02 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:02 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:55:02 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:55:02 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:55:02 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 9
12:55:02 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 2 2 2 0 0 0 0 2]
12:55:02 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 0 0 0 3 3 3 3 0]
12:55:02 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 516. 0.]
[ 0. 0. 0. 0. 0.]
[172. 0. 0. 422. 0.]
[ 10. 0. 839. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:55:02 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:55:02 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:02 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:55:02 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:55:02 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.07906769454081942
12:55:02 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:02 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:55:02 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:55:02 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 89 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 89 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 89 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 89 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 89 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 89 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 90 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 90 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 91 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 91 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 32 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 91 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 91 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 33 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 91 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 91 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 91 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 91 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 92 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 92 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 92 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 92 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 92 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 92 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 92 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 92 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 93 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 93 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 93 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 93 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 93 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 93 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 93 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 93 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 93 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 93 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 93 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 93 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 94 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 94 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 94 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 94 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 95 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 95 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 96 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 96 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 96 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 96 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 96 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 96 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 96 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 96 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 97 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 97 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 97 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 97 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 97 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 97 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 98 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 98 elements to seafloor.
12:55:02 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:02 DEBUG opendrift.models.oceandrift:636: 98 elements reached seafloor, interacting with bottom
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 98 elements to seafloor.
12:55:02 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 10
12:55:02 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:55:02 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 10 elements
12:55:02 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:02 INFO opendrift.models.chemicaldrift:1861: partitioning: [115, 0, 297, 88, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:55:02 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:55:02 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:55:02 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:55:02 DEBUG opendrift.models.basemodel:755: Lifting 88 elements to seafloor.
12:55:02 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:02 INFO opendrift.models.basemodel:2038: 2024-11-02 20:24:02.760182 - step 64 of 96 - 500 active elements (0 deactivated)
12:55:02 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:02 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:02 DEBUG opendrift.models.basemodel:2057: 57.55194026136295 <- latitude -> 57.74987936215713
12:55:02 DEBUG opendrift.models.basemodel:2062: 10.507927244297782 <- longitude -> 10.834538183264849
12:55:02 DEBUG opendrift.models.basemodel:2067: -30.800214946828493 <- z -> -0.05809662065405441
12:55:02 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:02 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:02 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:55:02 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:02 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:55:02 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:02 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:02 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:55:02 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-02 20:00:00 (before)
2024-11-02 21:00:00 (after)
12:55:04 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
12:55:04 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:55:04 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:55:04 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:55:04 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:55:04 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'upward_sea_water_velocity', 'y_sea_water_velocity', 'sea_water_temperature', 'sea_water_salinity']
12:55:04 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 55x37x7) for time after (2024-11-02 21:00:00)
12:55:04 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-02 20:00:00) in space (linearNDFast)
12:55:04 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:04 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-02 21:00:00) in space (linearNDFast)
12:55:04 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:04 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-02 20:00:00, weight 0.60) and
after (2024-11-02 21:00:00, weight 0.40) in time
12:55:04 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:55:04 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.1654728465205 degrees.
12:55:04 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.1654728465205 degrees.
12:55:04 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:04 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:04 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:04 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:04 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:55:04 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:04 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:04 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:04 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:04 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:04 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:04 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:55:04 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:04 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:04 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:04 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:04 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:04 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:55:04 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:55:04 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:55:04 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:55:04 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:04 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.210666 (min) 0.211962 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0394622 (min) 0.207107 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.297646 (min) -0.260281 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: x_wind: 4.12759 (min) 10.424 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: y_wind: 1.56873 (min) 4.51446 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 33.0415 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 11.9887 (min) 12.6218 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 31.993 (min) 33.4763 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000245774 (min) 0.00027004 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:04 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 88 elements to seafloor.
12:55:04 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:04 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:55:04 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:55:04 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:55:04 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 5
12:55:04 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 2 0 2 2]
12:55:04 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 0 3 0 0]
12:55:04 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 518. 0.]
[ 0. 0. 0. 0. 0.]
[175. 0. 0. 431. 0.]
[ 10. 0. 849. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:55:04 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:55:04 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:04 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:55:04 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:55:04 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.08058862276530745
12:55:04 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:04 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:55:04 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:55:04 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 90 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 90 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 90 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 90 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 90 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 90 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 90 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 90 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 90 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 90 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 90 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 90 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 90 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 90 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 90 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 90 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 90 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 90 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 90 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 90 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 90 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 90 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 90 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 90 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 90 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 90 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 91 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 91 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 91 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 91 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 91 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 91 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 92 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 92 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 92 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 92 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 92 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 92 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 93 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 93 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 93 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 93 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 93 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 93 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 93 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 93 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 93 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 93 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 93 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 93 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 93 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 93 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 94 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 94 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 94 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 94 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 94 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 94 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 94 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 94 elements to seafloor.
12:55:04 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 5
12:55:04 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:55:04 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 5 elements
12:55:04 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:04 INFO opendrift.models.chemicaldrift:1861: partitioning: [116, 0, 295, 89, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:55:04 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:55:04 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:55:04 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 90 elements to seafloor.
12:55:04 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:04 INFO opendrift.models.basemodel:2038: 2024-11-02 20:54:02.760182 - step 65 of 96 - 500 active elements (0 deactivated)
12:55:04 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:04 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:04 DEBUG opendrift.models.basemodel:2057: 57.55194026136295 <- latitude -> 57.7507777937513
12:55:04 DEBUG opendrift.models.basemodel:2062: 10.507927244297784 <- longitude -> 10.834781596238217
12:55:04 DEBUG opendrift.models.basemodel:2067: -31.978910528808633 <- z -> 0.0
12:55:04 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:55:04 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:55:04 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:04 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:55:04 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-02 20:00:00 (before)
2024-11-02 21:00:00 (after)
12:55:04 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-02 20:00:00) in space (linearNDFast)
12:55:04 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:04 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-02 21:00:00) in space (linearNDFast)
12:55:04 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:04 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-02 20:00:00, weight 0.10) and
after (2024-11-02 21:00:00, weight 0.90) in time
12:55:04 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:55:04 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.165229420264524 degrees.
12:55:04 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.165229420264524 degrees.
12:55:04 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:04 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:04 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:04 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:04 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:55:04 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:04 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:04 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:04 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:04 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:04 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:04 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:55:04 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:04 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:04 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:04 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:04 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:04 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:55:04 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:55:04 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:55:04 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:55:04 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:04 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.148659 (min) 0.227659 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0406482 (min) 0.226344 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.326653 (min) -0.290107 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: x_wind: 4.20627 (min) 10.5513 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: y_wind: 1.5756 (min) 4.7287 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 33.4602 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 11.9703 (min) 12.6254 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 31.9893 (min) 33.2127 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000347968 (min) 0.000268485 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:04 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 90 elements to seafloor.
12:55:04 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:04 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:55:04 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:55:04 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:55:04 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 6
12:55:04 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 2 2 2 0 0]
12:55:04 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 0 0 0 3 3]
12:55:04 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 520. 0.]
[ 0. 0. 0. 0. 0.]
[179. 0. 0. 435. 0.]
[ 10. 0. 854. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:55:04 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:55:04 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:04 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:55:04 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:55:04 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.08287957911186335
12:55:04 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:04 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:55:04 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:55:04 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 92 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 92 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 92 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 92 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 92 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 92 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 94 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 94 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 95 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 95 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 95 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 95 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 95 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 95 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 95 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 95 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 95 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 95 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 95 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 95 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 95 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 95 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 95 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 95 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 95 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 95 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 96 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 96 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 96 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 96 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 96 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 96 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 96 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 96 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 96 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 96 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 97 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 97 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 97 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 97 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 97 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 97 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 97 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 97 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 97 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 97 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 97 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 97 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 97 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 97 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 98 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 98 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 98 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 98 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 98 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 98 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 98 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 98 elements to seafloor.
12:55:04 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:55:04 DEBUG opendrift.models.oceandrift:636: 99 elements reached seafloor, interacting with bottom
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 99 elements to seafloor.
12:55:04 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 7
12:55:04 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:55:04 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 7 elements
12:55:04 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:04 INFO opendrift.models.chemicaldrift:1861: partitioning: [118, 0, 290, 92, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:55:04 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:55:04 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:55:04 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:55:04 DEBUG opendrift.models.basemodel:755: Lifting 92 elements to seafloor.
12:55:04 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:04 INFO opendrift.models.basemodel:2038: 2024-11-02 21:24:02.760182 - step 66 of 96 - 500 active elements (0 deactivated)
12:55:04 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:04 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:04 DEBUG opendrift.models.basemodel:2057: 57.55194026136295 <- latitude -> 57.75236976361738
12:55:04 DEBUG opendrift.models.basemodel:2062: 10.507927244297784 <- longitude -> 10.840727905373226
12:55:04 DEBUG opendrift.models.basemodel:2067: -32.5482063293457 <- z -> 0.0
12:55:04 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:55:04 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:55:04 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:04 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:55:04 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-02 21:00:00 (before)
2024-11-02 22:00:00 (after)
12:55:08 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
12:55:08 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:55:08 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:55:08 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:55:08 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:55:08 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'upward_sea_water_velocity', 'y_sea_water_velocity', 'sea_water_temperature', 'sea_water_salinity']
12:55:08 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 56x37x7) for time after (2024-11-02 22:00:00)
12:55:08 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-02 21:00:00) in space (linearNDFast)
12:55:08 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:08 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-02 22:00:00) in space (linearNDFast)
12:55:08 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:08 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-02 21:00:00, weight 0.60) and
after (2024-11-02 22:00:00, weight 0.40) in time
12:55:08 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:55:08 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.15928311059946 degrees.
12:55:08 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.15928311059946 degrees.
12:55:08 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:08 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:08 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:08 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:08 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:08 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:08 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:08 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:55:08 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:08 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:08 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:08 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:08 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:08 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:08 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:08 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:08 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:08 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:55:08 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:08 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:08 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:08 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:08 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:08 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:08 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:55:08 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:55:08 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:55:08 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:55:08 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:08 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.184768 (min) 0.230733 (max)
12:55:08 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0159169 (min) 0.193146 (max)
12:55:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.345635 (min) -0.309497 (max)
12:55:08 DEBUG opendrift.models.basemodel.environment:905: x_wind: 4.2209 (min) 10.6778 (max)
12:55:08 DEBUG opendrift.models.basemodel.environment:905: y_wind: 1.67862 (min) 4.67334 (max)
12:55:08 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:08 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 33.8677 (max)
12:55:08 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:55:08 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 11.9533 (min) 12.6354 (max)
12:55:08 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 31.9856 (min) 33.731 (max)
12:55:08 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000161148 (min) 0.00034647 (max)
12:55:08 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:55:08 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:55:08 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:55:08 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:55:08 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:55:08 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:55:08 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:08 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 92 elements to seafloor.
12:55:08 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:08 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:55:08 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:55:08 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:55:08 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 4
12:55:08 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 2 2 2]
12:55:08 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 0 0 0]
12:55:08 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 520. 0.]
[ 0. 0. 0. 0. 0.]
[183. 0. 0. 443. 0.]
[ 10. 0. 861. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:55:08 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:55:08 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:08 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:55:08 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:55:08 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.08488591702210331
12:55:08 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:08 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:55:08 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:55:08 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 92 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 92 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 92 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 92 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 92 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 92 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 93 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 93 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 93 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 93 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 93 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 93 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 93 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 93 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 93 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 93 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 93 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 93 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 94 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 94 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 94 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 94 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 94 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 94 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 94 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 94 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 94 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 94 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 94 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 94 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 94 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 94 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 94 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 94 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 94 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 94 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 94 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 94 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 96 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 96 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 97 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 97 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 97 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 97 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 98 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 98 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 98 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 98 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 99 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 99 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 100 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 100 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 100 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 100 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 100 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 100 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 102 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 102 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 103 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 103 elements to seafloor.
12:55:08 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 4
12:55:08 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:55:08 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 4 elements
12:55:08 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:08 INFO opendrift.models.chemicaldrift:1861: partitioning: [122, 0, 279, 99, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:55:08 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:55:08 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:55:08 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 100 elements to seafloor.
12:55:08 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:08 INFO opendrift.models.basemodel:2038: 2024-11-02 21:54:02.760182 - step 67 of 96 - 500 active elements (0 deactivated)
12:55:08 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:08 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:08 DEBUG opendrift.models.basemodel:2057: 57.55194026136296 <- latitude -> 57.75321666162107
12:55:08 DEBUG opendrift.models.basemodel:2062: 10.507927244297784 <- longitude -> 10.847335856189884
12:55:08 DEBUG opendrift.models.basemodel:2067: -32.56110382080078 <- z -> 0.0
12:55:08 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:08 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:08 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:55:08 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:08 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:55:08 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:08 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:08 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:55:08 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-02 21:00:00 (before)
2024-11-02 22:00:00 (after)
12:55:08 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-02 21:00:00) in space (linearNDFast)
12:55:08 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:08 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-02 22:00:00) in space (linearNDFast)
12:55:08 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:08 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-02 21:00:00, weight 0.10) and
after (2024-11-02 22:00:00, weight 0.90) in time
12:55:08 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:55:08 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.15267516022156 degrees.
12:55:08 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.15267516022156 degrees.
12:55:08 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:08 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:08 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:08 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:08 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:08 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:08 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:08 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:55:08 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:08 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:08 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:08 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:08 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:08 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:08 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:08 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:08 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:08 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:55:08 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:08 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:08 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:08 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:08 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:08 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:08 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:55:08 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:55:08 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:55:08 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:55:08 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:08 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.150581 (min) 0.231387 (max)
12:55:08 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.032225 (min) 0.240986 (max)
12:55:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.362135 (min) -0.326247 (max)
12:55:08 DEBUG opendrift.models.basemodel.environment:905: x_wind: 4.14425 (min) 10.804 (max)
12:55:08 DEBUG opendrift.models.basemodel.environment:905: y_wind: 1.77836 (min) 4.64309 (max)
12:55:08 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:08 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 34.3362 (max)
12:55:08 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:55:08 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 11.9365 (min) 12.6333 (max)
12:55:08 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 31.9819 (min) 33.238 (max)
12:55:08 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000272718 (min) 0.000177382 (max)
12:55:08 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:55:08 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:55:08 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:55:08 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:55:08 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:55:08 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:55:08 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:08 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 99 elements to seafloor.
12:55:08 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:08 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:55:08 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:55:08 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:55:08 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 6
12:55:08 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 2 0 0 2 2]
12:55:08 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 0 3 3 0 0]
12:55:08 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 522. 0.]
[ 0. 0. 0. 0. 0.]
[187. 0. 0. 454. 0.]
[ 10. 0. 865. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:55:08 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:55:08 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:08 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:55:08 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:55:08 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.086896314958662
12:55:08 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:08 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:55:08 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:55:08 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 101 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 101 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 102 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 102 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 102 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 102 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 102 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 102 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 102 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 102 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 102 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 102 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 102 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 102 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 102 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 102 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 103 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 103 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 103 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 103 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 104 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 104 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 104 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 104 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 104 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 104 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 104 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 104 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 105 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 105 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 105 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 105 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 105 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 105 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 105 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 105 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 105 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 105 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 107 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 107 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 107 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 107 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 107 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 107 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 107 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 107 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 107 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 107 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 107 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 107 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 108 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 108 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 109 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 109 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 109 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 109 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 109 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 109 elements to seafloor.
12:55:08 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:55:08 DEBUG opendrift.models.oceandrift:636: 109 elements reached seafloor, interacting with bottom
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 109 elements to seafloor.
12:55:08 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 6
12:55:08 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:55:08 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 6 elements
12:55:08 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:08 INFO opendrift.models.chemicaldrift:1861: partitioning: [124, 0, 273, 103, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:55:08 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:55:08 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:55:08 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:55:08 DEBUG opendrift.models.basemodel:755: Lifting 104 elements to seafloor.
12:55:08 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:08 INFO opendrift.models.basemodel:2038: 2024-11-02 22:24:02.760182 - step 68 of 96 - 500 active elements (0 deactivated)
12:55:08 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:08 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:08 DEBUG opendrift.models.basemodel:2057: 57.551940261362965 <- latitude -> 57.75456653179687
12:55:08 DEBUG opendrift.models.basemodel:2062: 10.507927244297784 <- longitude -> 10.851579321783976
12:55:08 DEBUG opendrift.models.basemodel:2067: -32.94283676147461 <- z -> -0.04501769086317231
12:55:08 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:08 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:08 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:55:08 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:08 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:55:08 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:08 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:08 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:55:08 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-02 22:00:00 (before)
2024-11-02 23:00:00 (after)
12:55:09 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
12:55:09 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:55:09 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:55:09 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:55:09 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:55:09 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'upward_sea_water_velocity', 'y_sea_water_velocity', 'sea_water_temperature', 'sea_water_salinity']
12:55:09 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 56x38x7) for time after (2024-11-02 23:00:00)
12:55:09 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-02 22:00:00) in space (linearNDFast)
12:55:09 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:09 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-02 23:00:00) in space (linearNDFast)
12:55:09 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:09 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-02 22:00:00, weight 0.60) and
after (2024-11-02 23:00:00, weight 0.40) in time
12:55:09 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:55:09 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.148431696797935 degrees.
12:55:09 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.148431696797935 degrees.
12:55:09 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:09 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:09 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:09 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:09 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:09 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:09 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:09 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:55:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:09 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:09 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:09 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:09 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:09 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:09 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:09 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:09 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:09 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:55:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:09 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:09 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:09 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:09 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:09 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:09 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:55:09 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:55:09 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:55:09 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:55:09 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:09 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.110513 (min) 0.259217 (max)
12:55:09 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.00767637 (min) 0.241478 (max)
12:55:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.364208 (min) -0.326515 (max)
12:55:09 DEBUG opendrift.models.basemodel.environment:905: x_wind: 4.69675 (min) 10.5547 (max)
12:55:09 DEBUG opendrift.models.basemodel.environment:905: y_wind: 2.31534 (min) 4.58081 (max)
12:55:09 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:09 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 34.8105 (max)
12:55:09 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:55:09 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 11.9184 (min) 12.6317 (max)
12:55:09 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 31.9786 (min) 33.5019 (max)
12:55:09 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000247606 (min) 0.000158684 (max)
12:55:09 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:55:09 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:55:09 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:55:09 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:55:09 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:55:09 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:55:09 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:09 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:55:09 DEBUG opendrift.models.basemodel:755: Lifting 103 elements to seafloor.
12:55:09 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:09 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:55:09 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:55:09 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:55:09 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 4
12:55:09 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 2 2 2]
12:55:09 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 0 0 0]
12:55:09 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 522. 0.]
[ 0. 0. 0. 0. 0.]
[191. 0. 0. 462. 0.]
[ 10. 0. 871. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:55:09 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:55:09 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:09 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:55:09 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:55:10 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.08472206848171007
12:55:10 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:10 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:55:10 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:55:10 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 103 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 103 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 103 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 103 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 103 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 103 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 103 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 103 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 104 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 104 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 104 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 104 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 104 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 104 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 104 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 104 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 104 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 104 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 104 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 104 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 104 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 104 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 104 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 104 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 104 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 104 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 104 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 104 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 104 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 104 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 104 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 104 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 105 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 105 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 105 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 105 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 105 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 105 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 105 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 105 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 105 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 105 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 105 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 105 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 105 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 105 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 105 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 105 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 106 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 106 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 106 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 106 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 106 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 106 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 106 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 106 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 107 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 107 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 107 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 107 elements to seafloor.
12:55:10 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 5
12:55:10 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:55:10 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 5 elements
12:55:10 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:10 INFO opendrift.models.chemicaldrift:1861: partitioning: [128, 0, 270, 102, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:55:10 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:55:10 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:55:10 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 103 elements to seafloor.
12:55:10 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:10 INFO opendrift.models.basemodel:2038: 2024-11-02 22:54:02.760182 - step 69 of 96 - 500 active elements (0 deactivated)
12:55:10 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:10 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:10 DEBUG opendrift.models.basemodel:2057: 57.55194026136297 <- latitude -> 57.75535643796114
12:55:10 DEBUG opendrift.models.basemodel:2062: 10.507927244297784 <- longitude -> 10.85392094296063
12:55:10 DEBUG opendrift.models.basemodel:2067: -32.94283676147461 <- z -> 0.0
12:55:10 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:10 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:10 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:55:10 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:10 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:55:10 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:10 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:10 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:55:10 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-02 22:00:00 (before)
2024-11-02 23:00:00 (after)
12:55:10 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-02 22:00:00) in space (linearNDFast)
12:55:10 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:10 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-02 23:00:00) in space (linearNDFast)
12:55:10 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:10 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-02 22:00:00, weight 0.10) and
after (2024-11-02 23:00:00, weight 0.90) in time
12:55:10 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:55:10 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.14609006805468 degrees.
12:55:10 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.14609006805468 degrees.
12:55:10 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:10 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:10 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:10 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:10 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:10 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:10 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:10 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:55:10 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:10 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:10 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:10 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:10 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:10 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:10 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:10 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:10 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:10 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:55:10 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:10 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:10 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:10 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:10 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:10 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:10 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:55:10 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:55:10 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:55:10 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:55:10 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:10 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.137995 (min) 0.234925 (max)
12:55:10 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0201537 (min) 0.237025 (max)
12:55:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.362708 (min) -0.322726 (max)
12:55:10 DEBUG opendrift.models.basemodel.environment:905: x_wind: 5.01748 (min) 10.2235 (max)
12:55:10 DEBUG opendrift.models.basemodel.environment:905: y_wind: 2.87465 (min) 4.51816 (max)
12:55:10 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:10 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 35.224 (max)
12:55:10 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:55:10 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 11.9001 (min) 12.6311 (max)
12:55:10 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 31.9754 (min) 33.437 (max)
12:55:10 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000357236 (min) 0.000164893 (max)
12:55:10 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:55:10 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:55:10 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:55:10 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:55:10 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:55:10 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:55:10 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:10 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 102 elements to seafloor.
12:55:10 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:10 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:55:10 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:55:10 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:55:10 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 5
12:55:10 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 0 2 3 2]
12:55:10 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 3 0 0 0]
12:55:10 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 523. 0.]
[ 0. 0. 0. 0. 0.]
[194. 0. 0. 466. 0.]
[ 11. 0. 876. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:55:10 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:55:10 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:10 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:55:10 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:55:10 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.08174891473896226
12:55:10 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:10 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:55:10 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:55:10 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 102 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 102 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 102 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 102 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 102 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 102 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 102 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 102 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 102 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 102 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 102 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 102 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 102 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 102 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 102 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 102 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 102 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 102 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 103 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 103 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 103 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 103 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 103 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 103 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 103 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 103 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 103 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 103 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 103 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 103 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 103 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 103 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 104 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 104 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 104 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 104 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 105 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 105 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 105 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 105 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 105 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 105 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 106 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 106 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 106 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 106 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 106 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 106 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 106 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 106 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 106 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 106 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 106 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 106 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 106 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 106 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 106 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 106 elements to seafloor.
12:55:10 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:10 DEBUG opendrift.models.oceandrift:636: 106 elements reached seafloor, interacting with bottom
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 106 elements to seafloor.
12:55:10 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 2
12:55:10 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:55:10 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 2 elements
12:55:10 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:10 INFO opendrift.models.chemicaldrift:1861: partitioning: [131, 0, 265, 104, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:55:10 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:55:10 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:55:10 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:55:10 DEBUG opendrift.models.basemodel:755: Lifting 104 elements to seafloor.
12:55:10 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:10 INFO opendrift.models.basemodel:2038: 2024-11-02 23:24:02.760182 - step 70 of 96 - 500 active elements (0 deactivated)
12:55:10 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:10 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:10 DEBUG opendrift.models.basemodel:2057: 57.55194026136298 <- latitude -> 57.75729939543267
12:55:10 DEBUG opendrift.models.basemodel:2062: 10.507927244297784 <- longitude -> 10.86062669877825
12:55:10 DEBUG opendrift.models.basemodel:2067: -35.22397994995117 <- z -> -0.1299883315758231
12:55:10 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:10 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:10 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:55:10 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:10 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:55:10 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:10 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:10 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:55:10 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-02 23:00:00 (before)
2024-11-03 00:00:00 (after)
12:55:11 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
12:55:11 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:55:11 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:55:11 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:55:11 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:55:11 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'upward_sea_water_velocity', 'y_sea_water_velocity', 'sea_water_temperature', 'sea_water_salinity']
12:55:11 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 56x38x7) for time after (2024-11-03 00:00:00)
12:55:11 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-02 23:00:00) in space (linearNDFast)
12:55:11 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:11 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-03 00:00:00) in space (linearNDFast)
12:55:11 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:11 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-02 23:00:00, weight 0.60) and
after (2024-11-03 00:00:00, weight 0.40) in time
12:55:11 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:55:11 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.13938431225394 degrees.
12:55:11 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.13938431225394 degrees.
12:55:11 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:11 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:11 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:11 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:11 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:11 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:11 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:11 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:55:11 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:11 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:11 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:11 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:11 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:11 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:11 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:11 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:11 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:11 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:55:11 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:11 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:11 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:11 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:11 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:11 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:11 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:55:11 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:55:11 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:55:11 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:55:11 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:11 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0748364 (min) 0.271187 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0459149 (min) 0.223752 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.34157 (min) -0.295619 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: x_wind: 6.57243 (min) 10.8519 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: y_wind: 2.70525 (min) 3.68957 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 35.3607 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 11.8882 (min) 12.6322 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 31.9727 (min) 33.4019 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000319036 (min) 0.000172966 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:11 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:55:11 DEBUG opendrift.models.basemodel:755: Lifting 104 elements to seafloor.
12:55:11 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:11 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:55:11 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:55:11 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:55:11 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 2
12:55:11 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 2]
12:55:11 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 0]
12:55:11 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 523. 0.]
[ 0. 0. 0. 0. 0.]
[196. 0. 0. 470. 0.]
[ 11. 0. 878. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:55:11 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:55:11 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:11 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:55:11 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:55:11 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.09060989746638531
12:55:11 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:11 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:55:11 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:55:11 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:55:11 DEBUG opendrift.models.oceandrift:636: 104 elements reached seafloor, interacting with bottom
12:55:11 DEBUG opendrift.models.basemodel:755: Lifting 104 elements to seafloor.
12:55:11 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:11 DEBUG opendrift.models.oceandrift:636: 104 elements reached seafloor, interacting with bottom
12:55:11 DEBUG opendrift.models.basemodel:755: Lifting 104 elements to seafloor.
12:55:11 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:11 DEBUG opendrift.models.oceandrift:636: 104 elements reached seafloor, interacting with bottom
12:55:11 DEBUG opendrift.models.basemodel:755: Lifting 104 elements to seafloor.
12:55:11 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:11 DEBUG opendrift.models.oceandrift:636: 104 elements reached seafloor, interacting with bottom
12:55:11 DEBUG opendrift.models.basemodel:755: Lifting 104 elements to seafloor.
12:55:11 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:55:11 DEBUG opendrift.models.oceandrift:636: 105 elements reached seafloor, interacting with bottom
12:55:11 DEBUG opendrift.models.basemodel:755: Lifting 105 elements to seafloor.
12:55:11 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:11 DEBUG opendrift.models.oceandrift:636: 105 elements reached seafloor, interacting with bottom
12:55:11 DEBUG opendrift.models.basemodel:755: Lifting 105 elements to seafloor.
12:55:11 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:11 DEBUG opendrift.models.oceandrift:636: 106 elements reached seafloor, interacting with bottom
12:55:11 DEBUG opendrift.models.basemodel:755: Lifting 106 elements to seafloor.
12:55:11 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:55:11 DEBUG opendrift.models.oceandrift:636: 106 elements reached seafloor, interacting with bottom
12:55:11 DEBUG opendrift.models.basemodel:755: Lifting 106 elements to seafloor.
12:55:11 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:11 DEBUG opendrift.models.oceandrift:636: 106 elements reached seafloor, interacting with bottom
12:55:11 DEBUG opendrift.models.basemodel:755: Lifting 106 elements to seafloor.
12:55:11 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:11 DEBUG opendrift.models.oceandrift:636: 106 elements reached seafloor, interacting with bottom
12:55:11 DEBUG opendrift.models.basemodel:755: Lifting 106 elements to seafloor.
12:55:11 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:11 DEBUG opendrift.models.oceandrift:636: 106 elements reached seafloor, interacting with bottom
12:55:11 DEBUG opendrift.models.basemodel:755: Lifting 106 elements to seafloor.
12:55:11 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:11 DEBUG opendrift.models.oceandrift:636: 106 elements reached seafloor, interacting with bottom
12:55:11 DEBUG opendrift.models.basemodel:755: Lifting 106 elements to seafloor.
12:55:11 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:11 DEBUG opendrift.models.oceandrift:636: 106 elements reached seafloor, interacting with bottom
12:55:11 DEBUG opendrift.models.basemodel:755: Lifting 106 elements to seafloor.
12:55:11 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:11 DEBUG opendrift.models.oceandrift:636: 107 elements reached seafloor, interacting with bottom
12:55:11 DEBUG opendrift.models.basemodel:755: Lifting 107 elements to seafloor.
12:55:11 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:11 DEBUG opendrift.models.oceandrift:636: 107 elements reached seafloor, interacting with bottom
12:55:11 DEBUG opendrift.models.basemodel:755: Lifting 107 elements to seafloor.
12:55:11 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:55:11 DEBUG opendrift.models.oceandrift:636: 107 elements reached seafloor, interacting with bottom
12:55:11 DEBUG opendrift.models.basemodel:755: Lifting 107 elements to seafloor.
12:55:11 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:11 DEBUG opendrift.models.oceandrift:636: 107 elements reached seafloor, interacting with bottom
12:55:11 DEBUG opendrift.models.basemodel:755: Lifting 107 elements to seafloor.
12:55:11 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:11 DEBUG opendrift.models.oceandrift:636: 108 elements reached seafloor, interacting with bottom
12:55:11 DEBUG opendrift.models.basemodel:755: Lifting 108 elements to seafloor.
12:55:11 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:11 DEBUG opendrift.models.oceandrift:636: 108 elements reached seafloor, interacting with bottom
12:55:11 DEBUG opendrift.models.basemodel:755: Lifting 108 elements to seafloor.
12:55:11 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:55:11 DEBUG opendrift.models.oceandrift:636: 108 elements reached seafloor, interacting with bottom
12:55:11 DEBUG opendrift.models.basemodel:755: Lifting 108 elements to seafloor.
12:55:11 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:55:11 DEBUG opendrift.models.oceandrift:636: 108 elements reached seafloor, interacting with bottom
12:55:11 DEBUG opendrift.models.basemodel:755: Lifting 108 elements to seafloor.
12:55:11 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:11 DEBUG opendrift.models.oceandrift:636: 109 elements reached seafloor, interacting with bottom
12:55:11 DEBUG opendrift.models.basemodel:755: Lifting 109 elements to seafloor.
12:55:11 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:11 DEBUG opendrift.models.oceandrift:636: 109 elements reached seafloor, interacting with bottom
12:55:11 DEBUG opendrift.models.basemodel:755: Lifting 109 elements to seafloor.
12:55:11 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:55:11 DEBUG opendrift.models.oceandrift:636: 110 elements reached seafloor, interacting with bottom
12:55:11 DEBUG opendrift.models.basemodel:755: Lifting 110 elements to seafloor.
12:55:11 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:11 DEBUG opendrift.models.oceandrift:636: 110 elements reached seafloor, interacting with bottom
12:55:11 DEBUG opendrift.models.basemodel:755: Lifting 110 elements to seafloor.
12:55:11 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:55:11 DEBUG opendrift.models.oceandrift:636: 110 elements reached seafloor, interacting with bottom
12:55:11 DEBUG opendrift.models.basemodel:755: Lifting 110 elements to seafloor.
12:55:11 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:55:11 DEBUG opendrift.models.oceandrift:636: 110 elements reached seafloor, interacting with bottom
12:55:11 DEBUG opendrift.models.basemodel:755: Lifting 110 elements to seafloor.
12:55:11 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:55:11 DEBUG opendrift.models.oceandrift:636: 110 elements reached seafloor, interacting with bottom
12:55:11 DEBUG opendrift.models.basemodel:755: Lifting 110 elements to seafloor.
12:55:11 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:11 DEBUG opendrift.models.oceandrift:636: 110 elements reached seafloor, interacting with bottom
12:55:11 DEBUG opendrift.models.basemodel:755: Lifting 110 elements to seafloor.
12:55:11 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:11 DEBUG opendrift.models.oceandrift:636: 110 elements reached seafloor, interacting with bottom
12:55:11 DEBUG opendrift.models.basemodel:755: Lifting 110 elements to seafloor.
12:55:11 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 2
12:55:11 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:55:11 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 2 elements
12:55:11 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:11 INFO opendrift.models.chemicaldrift:1861: partitioning: [133, 0, 259, 108, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:55:11 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:55:11 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:55:11 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:55:11 DEBUG opendrift.models.basemodel:755: Lifting 109 elements to seafloor.
12:55:11 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:11 INFO opendrift.models.basemodel:2038: 2024-11-02 23:54:02.760182 - step 71 of 96 - 500 active elements (0 deactivated)
12:55:11 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:11 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:11 DEBUG opendrift.models.basemodel:2057: 57.551940261362994 <- latitude -> 57.7566529636948
12:55:11 DEBUG opendrift.models.basemodel:2062: 10.507927244297784 <- longitude -> 10.866888846753497
12:55:11 DEBUG opendrift.models.basemodel:2067: -35.22397994995117 <- z -> -0.07317162160432947
12:55:11 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:11 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:11 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:55:11 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:11 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:55:11 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:11 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:11 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:55:11 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-02 23:00:00 (before)
2024-11-03 00:00:00 (after)
12:55:11 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-02 23:00:00) in space (linearNDFast)
12:55:11 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:11 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-03 00:00:00) in space (linearNDFast)
12:55:11 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:11 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-02 23:00:00, weight 0.10) and
after (2024-11-03 00:00:00, weight 0.90) in time
12:55:11 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:55:11 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.13312215394704 degrees.
12:55:11 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.13312215394704 degrees.
12:55:11 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:11 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:11 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:11 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:11 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:11 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:11 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:11 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:55:11 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:11 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:11 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:11 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:11 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:11 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:11 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:11 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:11 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:11 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:55:11 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:11 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:11 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:11 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:11 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:11 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:11 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:55:11 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:55:11 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:55:11 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:55:11 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:11 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0798565 (min) 0.277679 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0739601 (min) 0.220394 (max)
12:55:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.31557 (min) -0.260509 (max)
12:55:12 DEBUG opendrift.models.basemodel.environment:905: x_wind: 8.43299 (min) 11.6406 (max)
12:55:12 DEBUG opendrift.models.basemodel.environment:905: y_wind: 2.33308 (min) 2.83297 (max)
12:55:12 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:12 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 35.2626 (max)
12:55:12 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:55:12 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 11.878 (min) 12.6472 (max)
12:55:12 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 31.9701 (min) 33.3919 (max)
12:55:12 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000268223 (min) 0.000217867 (max)
12:55:12 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:55:12 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:55:12 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:55:12 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:55:12 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:55:12 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:55:12 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:12 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:55:12 DEBUG opendrift.models.basemodel:755: Lifting 108 elements to seafloor.
12:55:12 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:12 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:55:12 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:55:12 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:55:12 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 6
12:55:12 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 0 0 2 2 2]
12:55:12 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 3 3 0 0 0]
12:55:12 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 525. 0.]
[ 0. 0. 0. 0. 0.]
[200. 0. 0. 476. 0.]
[ 11. 0. 880. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:55:12 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:55:12 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:12 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:55:12 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:55:12 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.10214630338045957
12:55:12 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:12 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:55:12 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:55:12 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:55:12 DEBUG opendrift.models.oceandrift:636: 110 elements reached seafloor, interacting with bottom
12:55:12 DEBUG opendrift.models.basemodel:755: Lifting 110 elements to seafloor.
12:55:12 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:12 DEBUG opendrift.models.oceandrift:636: 111 elements reached seafloor, interacting with bottom
12:55:12 DEBUG opendrift.models.basemodel:755: Lifting 111 elements to seafloor.
12:55:12 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:12 DEBUG opendrift.models.oceandrift:636: 111 elements reached seafloor, interacting with bottom
12:55:12 DEBUG opendrift.models.basemodel:755: Lifting 111 elements to seafloor.
12:55:12 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:12 DEBUG opendrift.models.oceandrift:636: 111 elements reached seafloor, interacting with bottom
12:55:12 DEBUG opendrift.models.basemodel:755: Lifting 111 elements to seafloor.
12:55:12 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:12 DEBUG opendrift.models.oceandrift:636: 112 elements reached seafloor, interacting with bottom
12:55:12 DEBUG opendrift.models.basemodel:755: Lifting 112 elements to seafloor.
12:55:12 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:12 DEBUG opendrift.models.oceandrift:636: 112 elements reached seafloor, interacting with bottom
12:55:12 DEBUG opendrift.models.basemodel:755: Lifting 112 elements to seafloor.
12:55:12 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:12 DEBUG opendrift.models.oceandrift:636: 112 elements reached seafloor, interacting with bottom
12:55:12 DEBUG opendrift.models.basemodel:755: Lifting 112 elements to seafloor.
12:55:12 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:55:12 DEBUG opendrift.models.oceandrift:636: 112 elements reached seafloor, interacting with bottom
12:55:12 DEBUG opendrift.models.basemodel:755: Lifting 112 elements to seafloor.
12:55:12 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:12 DEBUG opendrift.models.oceandrift:636: 113 elements reached seafloor, interacting with bottom
12:55:12 DEBUG opendrift.models.basemodel:755: Lifting 113 elements to seafloor.
12:55:12 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:12 DEBUG opendrift.models.oceandrift:636: 113 elements reached seafloor, interacting with bottom
12:55:12 DEBUG opendrift.models.basemodel:755: Lifting 113 elements to seafloor.
12:55:12 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:12 DEBUG opendrift.models.oceandrift:636: 113 elements reached seafloor, interacting with bottom
12:55:12 DEBUG opendrift.models.basemodel:755: Lifting 113 elements to seafloor.
12:55:12 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:12 DEBUG opendrift.models.oceandrift:636: 113 elements reached seafloor, interacting with bottom
12:55:12 DEBUG opendrift.models.basemodel:755: Lifting 113 elements to seafloor.
12:55:12 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:55:12 DEBUG opendrift.models.oceandrift:636: 113 elements reached seafloor, interacting with bottom
12:55:12 DEBUG opendrift.models.basemodel:755: Lifting 113 elements to seafloor.
12:55:12 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:12 DEBUG opendrift.models.oceandrift:636: 114 elements reached seafloor, interacting with bottom
12:55:12 DEBUG opendrift.models.basemodel:755: Lifting 114 elements to seafloor.
12:55:12 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:12 DEBUG opendrift.models.oceandrift:636: 114 elements reached seafloor, interacting with bottom
12:55:12 DEBUG opendrift.models.basemodel:755: Lifting 114 elements to seafloor.
12:55:12 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:12 DEBUG opendrift.models.oceandrift:636: 115 elements reached seafloor, interacting with bottom
12:55:12 DEBUG opendrift.models.basemodel:755: Lifting 115 elements to seafloor.
12:55:12 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:12 DEBUG opendrift.models.oceandrift:636: 115 elements reached seafloor, interacting with bottom
12:55:12 DEBUG opendrift.models.basemodel:755: Lifting 115 elements to seafloor.
12:55:12 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:55:12 DEBUG opendrift.models.oceandrift:636: 115 elements reached seafloor, interacting with bottom
12:55:12 DEBUG opendrift.models.basemodel:755: Lifting 115 elements to seafloor.
12:55:12 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:12 DEBUG opendrift.models.oceandrift:636: 115 elements reached seafloor, interacting with bottom
12:55:12 DEBUG opendrift.models.basemodel:755: Lifting 115 elements to seafloor.
12:55:12 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:12 DEBUG opendrift.models.oceandrift:636: 115 elements reached seafloor, interacting with bottom
12:55:12 DEBUG opendrift.models.basemodel:755: Lifting 115 elements to seafloor.
12:55:12 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:55:12 DEBUG opendrift.models.oceandrift:636: 115 elements reached seafloor, interacting with bottom
12:55:12 DEBUG opendrift.models.basemodel:755: Lifting 115 elements to seafloor.
12:55:12 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:12 DEBUG opendrift.models.oceandrift:636: 115 elements reached seafloor, interacting with bottom
12:55:12 DEBUG opendrift.models.basemodel:755: Lifting 115 elements to seafloor.
12:55:12 DEBUG opendrift.models.oceandrift:618: 34 elements penetrated seafloor, lifting up
12:55:12 DEBUG opendrift.models.oceandrift:636: 115 elements reached seafloor, interacting with bottom
12:55:12 DEBUG opendrift.models.basemodel:755: Lifting 115 elements to seafloor.
12:55:12 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:55:12 DEBUG opendrift.models.oceandrift:636: 116 elements reached seafloor, interacting with bottom
12:55:12 DEBUG opendrift.models.basemodel:755: Lifting 116 elements to seafloor.
12:55:12 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:12 DEBUG opendrift.models.oceandrift:636: 116 elements reached seafloor, interacting with bottom
12:55:12 DEBUG opendrift.models.basemodel:755: Lifting 116 elements to seafloor.
12:55:12 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:12 DEBUG opendrift.models.oceandrift:636: 117 elements reached seafloor, interacting with bottom
12:55:12 DEBUG opendrift.models.basemodel:755: Lifting 117 elements to seafloor.
12:55:12 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:55:12 DEBUG opendrift.models.oceandrift:636: 117 elements reached seafloor, interacting with bottom
12:55:12 DEBUG opendrift.models.basemodel:755: Lifting 117 elements to seafloor.
12:55:12 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:55:12 DEBUG opendrift.models.oceandrift:636: 117 elements reached seafloor, interacting with bottom
12:55:12 DEBUG opendrift.models.basemodel:755: Lifting 117 elements to seafloor.
12:55:12 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:12 DEBUG opendrift.models.oceandrift:636: 117 elements reached seafloor, interacting with bottom
12:55:12 DEBUG opendrift.models.basemodel:755: Lifting 117 elements to seafloor.
12:55:12 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:12 DEBUG opendrift.models.oceandrift:636: 117 elements reached seafloor, interacting with bottom
12:55:12 DEBUG opendrift.models.basemodel:755: Lifting 117 elements to seafloor.
12:55:12 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 2
12:55:12 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:55:12 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 2 elements
12:55:12 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:12 INFO opendrift.models.chemicaldrift:1861: partitioning: [135, 0, 250, 115, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:55:12 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:55:12 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:55:12 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:55:12 DEBUG opendrift.models.basemodel:755: Lifting 116 elements to seafloor.
12:55:12 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:12 INFO opendrift.models.basemodel:2038: 2024-11-03 00:24:02.760182 - step 72 of 96 - 500 active elements (0 deactivated)
12:55:12 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:12 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:12 DEBUG opendrift.models.basemodel:2057: 57.551940261362994 <- latitude -> 57.75545759176469
12:55:12 DEBUG opendrift.models.basemodel:2062: 10.507927244297784 <- longitude -> 10.874004142661656
12:55:12 DEBUG opendrift.models.basemodel:2067: -35.22397994995117 <- z -> -0.06572108065030535
12:55:12 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:12 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:12 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:55:12 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:12 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:55:12 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:12 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:12 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:55:12 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-03 00:00:00 (before)
2024-11-03 01:00:00 (after)
12:55:13 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
12:55:13 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:55:13 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:55:13 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:55:13 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:55:13 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'upward_sea_water_velocity', 'y_sea_water_velocity', 'sea_water_temperature', 'sea_water_salinity']
12:55:13 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 57x39x7) for time after (2024-11-03 01:00:00)
12:55:13 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-03 00:00:00) in space (linearNDFast)
12:55:13 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:13 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-03 01:00:00) in space (linearNDFast)
12:55:13 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:13 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-03 00:00:00, weight 0.60) and
after (2024-11-03 01:00:00, weight 0.40) in time
12:55:13 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:55:13 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.126006868460905 degrees.
12:55:13 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.126006868460905 degrees.
12:55:13 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:13 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:13 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:13 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:13 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:13 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:13 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:13 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:55:13 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:13 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:13 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:13 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:13 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:13 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:13 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:13 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:13 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:13 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:55:13 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:13 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:13 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:13 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:13 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:13 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:13 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:55:13 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:55:13 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:55:13 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:55:13 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:13 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0872668 (min) 0.304515 (max)
12:55:13 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.128965 (min) 0.229114 (max)
12:55:13 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.266726 (min) -0.20571 (max)
12:55:13 DEBUG opendrift.models.basemodel.environment:905: x_wind: 8.67494 (min) 11.9601 (max)
12:55:13 DEBUG opendrift.models.basemodel.environment:905: y_wind: 2.48419 (min) 2.84609 (max)
12:55:13 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:13 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 35.224 (max)
12:55:13 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:55:13 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 11.8706 (min) 12.6472 (max)
12:55:13 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 31.9693 (min) 33.4978 (max)
12:55:13 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.00023736 (min) 0.000242904 (max)
12:55:13 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:55:13 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:55:13 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:55:13 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:55:13 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:55:13 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:55:13 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:13 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 115 elements to seafloor.
12:55:13 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:13 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:55:13 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:55:13 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:55:13 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 7
12:55:13 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 2 2 2 0 2 0]
12:55:13 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 0 0 0 3 0 3]
12:55:13 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 527. 0.]
[ 0. 0. 0. 0. 0.]
[205. 0. 0. 483. 0.]
[ 11. 0. 882. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:55:13 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:55:13 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:13 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:55:13 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:55:13 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.10820950431935791
12:55:13 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:13 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:55:13 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:55:13 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 117 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 117 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 117 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 117 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 120 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 120 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 120 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 120 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 120 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 120 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 120 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 120 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 120 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 120 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 120 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 120 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 120 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 120 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 120 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 120 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 120 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 120 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 121 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 121 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 121 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 121 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 122 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 122 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 122 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 122 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 122 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 122 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 122 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 122 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 122 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 122 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 123 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 123 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 123 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 123 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 123 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 123 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 123 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 123 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 123 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 123 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 123 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 123 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 123 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 123 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 123 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 123 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 123 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 123 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 123 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 123 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 123 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 123 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 123 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 123 elements to seafloor.
12:55:13 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 1
12:55:13 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:55:13 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 1 elements
12:55:13 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:13 INFO opendrift.models.chemicaldrift:1861: partitioning: [138, 0, 240, 122, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:55:13 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:55:13 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:55:13 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 123 elements to seafloor.
12:55:13 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:13 INFO opendrift.models.basemodel:2038: 2024-11-03 00:54:02.760182 - step 73 of 96 - 500 active elements (0 deactivated)
12:55:13 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:13 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:13 DEBUG opendrift.models.basemodel:2057: 57.551940261362994 <- latitude -> 57.753893230430826
12:55:13 DEBUG opendrift.models.basemodel:2062: 10.507927244297784 <- longitude -> 10.87781395227404
12:55:13 DEBUG opendrift.models.basemodel:2067: -35.22397994995117 <- z -> -0.062289897704120745
12:55:13 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:13 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:13 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:55:13 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:13 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:55:13 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:13 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:13 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:55:13 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-03 00:00:00 (before)
2024-11-03 01:00:00 (after)
12:55:13 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-03 00:00:00) in space (linearNDFast)
12:55:13 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:13 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-03 01:00:00) in space (linearNDFast)
12:55:13 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:13 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-03 00:00:00, weight 0.10) and
after (2024-11-03 01:00:00, weight 0.90) in time
12:55:13 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:55:13 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.12219706421402 degrees.
12:55:13 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.12219706421402 degrees.
12:55:13 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:13 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:13 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:13 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:13 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:13 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:13 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:13 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:55:13 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:13 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:13 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:13 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:13 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:13 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:13 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:13 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:13 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:13 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:55:13 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:13 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:13 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:13 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:13 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:13 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:13 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:55:13 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:55:13 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:55:13 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:55:13 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:13 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0947906 (min) 0.31873 (max)
12:55:13 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.203732 (min) 0.196885 (max)
12:55:13 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.212226 (min) -0.14671 (max)
12:55:13 DEBUG opendrift.models.basemodel.environment:905: x_wind: 8.51611 (min) 12.1555 (max)
12:55:13 DEBUG opendrift.models.basemodel.environment:905: y_wind: 2.58374 (min) 3.08571 (max)
12:55:13 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:13 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 35.358 (max)
12:55:13 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:55:13 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 11.8638 (min) 12.6595 (max)
12:55:13 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 31.9689 (min) 33.4696 (max)
12:55:13 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000232381 (min) 0.000383121 (max)
12:55:13 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:55:13 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:55:13 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:55:13 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:55:13 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:55:13 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:55:13 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:13 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 122 elements to seafloor.
12:55:13 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:13 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:55:13 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:55:13 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:55:13 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 8
12:55:13 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 2 0 3 0 0 2 2]
12:55:13 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 0 3 0 3 3 0 0]
12:55:13 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 530. 0.]
[ 0. 0. 0. 0. 0.]
[209. 0. 0. 489. 0.]
[ 12. 0. 883. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:55:13 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:55:13 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:13 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:55:13 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:55:13 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.11278489978761372
12:55:13 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:13 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:55:13 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:55:13 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 124 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 124 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 124 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 124 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 124 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 124 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 124 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 124 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 124 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 124 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 124 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 124 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 126 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 126 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 127 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 127 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 128 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 128 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 128 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 128 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 128 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 128 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 128 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 128 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 130 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 130 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 130 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 130 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 130 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 130 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 130 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 130 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 130 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 130 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 131 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 131 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 132 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 132 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 132 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 132 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 132 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 132 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 132 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 132 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 132 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 132 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 132 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 132 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 133 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 133 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 133 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 133 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 133 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 133 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 133 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 133 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 133 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 133 elements to seafloor.
12:55:13 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:13 DEBUG opendrift.models.oceandrift:636: 133 elements reached seafloor, interacting with bottom
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 133 elements to seafloor.
12:55:13 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 12
12:55:13 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:55:13 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 12 elements
12:55:13 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:13 INFO opendrift.models.chemicaldrift:1861: partitioning: [140, 0, 239, 121, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:55:13 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:55:13 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:55:13 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:55:13 DEBUG opendrift.models.basemodel:755: Lifting 123 elements to seafloor.
12:55:13 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:13 INFO opendrift.models.basemodel:2038: 2024-11-03 01:24:02.760182 - step 74 of 96 - 500 active elements (0 deactivated)
12:55:13 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:13 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:13 DEBUG opendrift.models.basemodel:2057: 57.551940261362994 <- latitude -> 57.75164872742578
12:55:13 DEBUG opendrift.models.basemodel:2062: 10.507927244297786 <- longitude -> 10.881145544566667
12:55:13 DEBUG opendrift.models.basemodel:2067: -35.22397994995117 <- z -> 0.0
12:55:13 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:13 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:13 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:55:13 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:13 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:55:13 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:13 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:13 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:55:13 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-03 01:00:00 (before)
2024-11-03 02:00:00 (after)
12:55:15 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
12:55:15 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:55:15 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:55:15 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:55:15 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:55:15 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'upward_sea_water_velocity', 'y_sea_water_velocity', 'sea_water_temperature', 'sea_water_salinity']
12:55:15 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 57x39x7) for time after (2024-11-03 02:00:00)
12:55:15 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-03 01:00:00) in space (linearNDFast)
12:55:15 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:15 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-03 02:00:00) in space (linearNDFast)
12:55:15 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:15 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-03 01:00:00, weight 0.60) and
after (2024-11-03 02:00:00, weight 0.40) in time
12:55:15 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:55:15 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.11886546596012 degrees.
12:55:15 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.11886546596012 degrees.
12:55:15 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:15 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:15 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:15 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:15 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:15 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:15 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:15 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:55:15 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:15 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:15 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:15 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:15 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:15 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:15 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:15 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:15 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:15 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:55:15 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:15 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:15 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:15 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:15 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:15 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:15 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:55:15 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:55:15 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:55:15 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:55:15 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:15 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.109709 (min) 0.323762 (max)
12:55:15 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.233729 (min) 0.158031 (max)
12:55:15 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.149581 (min) -0.0893203 (max)
12:55:15 DEBUG opendrift.models.basemodel.environment:905: x_wind: 7.85228 (min) 12.6078 (max)
12:55:15 DEBUG opendrift.models.basemodel.environment:905: y_wind: 2.78593 (min) 3.43987 (max)
12:55:15 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:15 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 35.383 (max)
12:55:15 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:55:15 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 11.8662 (min) 12.6659 (max)
12:55:15 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 31.9731 (min) 33.5448 (max)
12:55:15 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000164018 (min) 0.000459636 (max)
12:55:15 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:55:15 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:55:15 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:55:15 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:55:15 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:55:15 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:55:15 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:15 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 121 elements to seafloor.
12:55:15 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:15 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:55:15 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:55:15 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:55:15 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 10
12:55:15 DEBUG opendrift.models.chemicaldrift:1452: old species: [3 2 0 0 2 0 0 0 0 0]
12:55:15 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 0 3 3 0 3 3 3 3 3]
12:55:15 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 537. 0.]
[ 0. 0. 0. 0. 0.]
[211. 0. 0. 498. 0.]
[ 13. 0. 895. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:55:15 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:55:15 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:15 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:55:15 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:55:15 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.12235631651166104
12:55:15 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:15 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:55:15 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:55:15 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 127 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 127 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 127 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 127 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 127 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 127 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 127 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 127 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 127 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 127 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 127 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 127 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 127 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 127 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 127 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 127 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 127 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 127 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 127 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 127 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 127 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 127 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 127 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 127 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 127 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 127 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 127 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 127 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 127 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 127 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 128 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 128 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 128 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 128 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 128 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 128 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 129 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 129 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 129 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 129 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 129 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 129 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 129 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 129 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 129 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 129 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 129 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 129 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 129 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 129 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 129 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 129 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 129 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 129 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 129 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 129 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 129 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 129 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 129 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 129 elements to seafloor.
12:55:15 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 8
12:55:15 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:55:15 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 8 elements
12:55:15 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:15 INFO opendrift.models.chemicaldrift:1861: partitioning: [136, 0, 243, 121, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:55:15 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:55:15 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:55:15 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 121 elements to seafloor.
12:55:15 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:15 INFO opendrift.models.basemodel:2038: 2024-11-03 01:54:02.760182 - step 75 of 96 - 500 active elements (0 deactivated)
12:55:15 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:15 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:15 DEBUG opendrift.models.basemodel:2057: 57.551940261362994 <- latitude -> 57.749573061198866
12:55:15 DEBUG opendrift.models.basemodel:2062: 10.507927244297786 <- longitude -> 10.890380137964025
12:55:15 DEBUG opendrift.models.basemodel:2067: -35.38302993774414 <- z -> 0.0
12:55:15 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:15 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:15 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:55:15 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:15 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:55:15 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:15 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:15 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:55:15 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-03 01:00:00 (before)
2024-11-03 02:00:00 (after)
12:55:15 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-03 01:00:00) in space (linearNDFast)
12:55:15 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:15 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-03 02:00:00) in space (linearNDFast)
12:55:15 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:15 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-03 01:00:00, weight 0.10) and
after (2024-11-03 02:00:00, weight 0.90) in time
12:55:15 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:55:15 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.109630871568555 degrees.
12:55:15 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.109630871568555 degrees.
12:55:15 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:15 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:15 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:15 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:15 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:15 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:15 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:15 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:55:15 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:15 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:15 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:15 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:15 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:15 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:15 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:15 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:15 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:15 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:55:15 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:15 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:15 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:15 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:15 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:15 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:15 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:55:15 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:55:15 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:55:15 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:55:15 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:15 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.138754 (min) 0.316606 (max)
12:55:15 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.256524 (min) 0.136 (max)
12:55:15 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.0849192 (min) -0.032322 (max)
12:55:15 DEBUG opendrift.models.basemodel.environment:905: x_wind: 7.06342 (min) 13.1511 (max)
12:55:15 DEBUG opendrift.models.basemodel.environment:905: y_wind: 2.95997 (min) 4.15795 (max)
12:55:15 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:15 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 35.383 (max)
12:55:15 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:55:15 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 11.8709 (min) 12.6708 (max)
12:55:15 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 31.9786 (min) 33.5056 (max)
12:55:15 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.00014362 (min) 0.000487119 (max)
12:55:15 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:55:15 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:55:15 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:55:15 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:55:15 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:55:15 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:55:15 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:15 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 121 elements to seafloor.
12:55:15 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:15 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:55:15 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:55:15 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:55:15 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 10
12:55:15 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 0 2 2 2 0 0 2 0 2]
12:55:15 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 3 0 0 0 3 3 0 3 0]
12:55:15 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 541. 0.]
[ 0. 0. 0. 0. 0.]
[217. 0. 0. 500. 0.]
[ 13. 0. 903. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:55:15 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:55:15 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:15 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:55:15 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:55:15 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.13420607172655716
12:55:15 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:15 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:55:15 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:55:15 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 126 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 126 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 126 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 126 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 126 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 126 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 126 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 126 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 126 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 126 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 126 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 126 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 127 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 127 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 127 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 127 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 127 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 127 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 127 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 127 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 127 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 127 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 127 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 127 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 127 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 127 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 127 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 127 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 127 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 127 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 127 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 127 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 127 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 127 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 127 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 127 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 127 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 127 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 127 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 127 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 127 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 127 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 127 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 127 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 127 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 127 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 127 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 127 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 127 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 127 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 127 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 127 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 127 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 127 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 127 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 127 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 128 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 128 elements to seafloor.
12:55:15 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:15 DEBUG opendrift.models.oceandrift:636: 128 elements reached seafloor, interacting with bottom
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 128 elements to seafloor.
12:55:15 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 13
12:55:15 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:55:15 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 13 elements
12:55:15 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:15 INFO opendrift.models.chemicaldrift:1861: partitioning: [138, 0, 247, 115, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:55:15 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:55:15 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:55:15 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:55:15 DEBUG opendrift.models.basemodel:755: Lifting 115 elements to seafloor.
12:55:15 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:15 INFO opendrift.models.basemodel:2038: 2024-11-03 02:24:02.760182 - step 76 of 96 - 500 active elements (0 deactivated)
12:55:15 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:15 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:15 DEBUG opendrift.models.basemodel:2057: 57.551940261362994 <- latitude -> 57.74655934535845
12:55:15 DEBUG opendrift.models.basemodel:2062: 10.507927244297786 <- longitude -> 10.89791071702224
12:55:15 DEBUG opendrift.models.basemodel:2067: -35.38302993774414 <- z -> 0.0
12:55:15 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:15 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:15 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:55:15 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:15 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:55:15 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:15 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:15 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:55:15 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-03 02:00:00 (before)
2024-11-03 03:00:00 (after)
12:55:21 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
12:55:21 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:55:21 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:55:21 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:55:21 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:55:21 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'upward_sea_water_velocity', 'y_sea_water_velocity', 'sea_water_temperature', 'sea_water_salinity']
12:55:21 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 57x41x7) for time after (2024-11-03 03:00:00)
12:55:21 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-03 02:00:00) in space (linearNDFast)
12:55:21 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:21 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-03 03:00:00) in space (linearNDFast)
12:55:21 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:21 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-03 02:00:00, weight 0.60) and
after (2024-11-03 03:00:00, weight 0.40) in time
12:55:21 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:55:21 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.10210029194618 degrees.
12:55:21 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.10210029194618 degrees.
12:55:21 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:21 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:21 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:21 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:21 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:21 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:21 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:21 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:55:21 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:21 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:21 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:21 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:21 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:21 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:21 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:21 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:21 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:21 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:55:21 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:21 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:21 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:21 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:21 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:21 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:21 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:55:21 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:55:21 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:55:21 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:55:21 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:21 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.133518 (min) 0.271367 (max)
12:55:21 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.26437 (min) 0.129919 (max)
12:55:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.0309368 (min) 0.013297 (max)
12:55:21 DEBUG opendrift.models.basemodel.environment:905: x_wind: 8.48252 (min) 13.4113 (max)
12:55:21 DEBUG opendrift.models.basemodel.environment:905: y_wind: 3.13733 (min) 3.99337 (max)
12:55:21 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:21 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 35.383 (max)
12:55:21 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:55:21 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 11.8874 (min) 12.6617 (max)
12:55:21 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 31.9902 (min) 33.5854 (max)
12:55:21 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000159743 (min) 0.000511535 (max)
12:55:21 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:55:21 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:55:21 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:55:21 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:55:21 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:55:21 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:55:21 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:21 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:55:21 DEBUG opendrift.models.basemodel:755: Lifting 55 elements to seafloor.
12:55:21 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:21 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:55:21 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:55:21 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:55:21 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 6
12:55:21 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 2 2 0 2]
12:55:21 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 0 0 3 0]
12:55:21 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 544. 0.]
[ 0. 0. 0. 0. 0.]
[220. 0. 0. 503. 0.]
[ 13. 0. 916. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:55:21 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:55:21 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:21 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:55:21 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:55:21 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.13907057637328318
12:55:21 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:21 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:55:21 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:55:21 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:636: 55 elements reached seafloor, interacting with bottom
12:55:21 DEBUG opendrift.models.basemodel:755: Lifting 55 elements to seafloor.
12:55:21 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:636: 56 elements reached seafloor, interacting with bottom
12:55:21 DEBUG opendrift.models.basemodel:755: Lifting 56 elements to seafloor.
12:55:21 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:636: 55 elements reached seafloor, interacting with bottom
12:55:21 DEBUG opendrift.models.basemodel:755: Lifting 55 elements to seafloor.
12:55:21 DEBUG opendrift.models.oceandrift:618: 32 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:636: 56 elements reached seafloor, interacting with bottom
12:55:21 DEBUG opendrift.models.basemodel:755: Lifting 56 elements to seafloor.
12:55:21 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:636: 55 elements reached seafloor, interacting with bottom
12:55:21 DEBUG opendrift.models.basemodel:755: Lifting 55 elements to seafloor.
12:55:21 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:636: 55 elements reached seafloor, interacting with bottom
12:55:21 DEBUG opendrift.models.basemodel:755: Lifting 55 elements to seafloor.
12:55:21 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:636: 55 elements reached seafloor, interacting with bottom
12:55:21 DEBUG opendrift.models.basemodel:755: Lifting 55 elements to seafloor.
12:55:21 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:636: 55 elements reached seafloor, interacting with bottom
12:55:21 DEBUG opendrift.models.basemodel:755: Lifting 55 elements to seafloor.
12:55:21 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:636: 55 elements reached seafloor, interacting with bottom
12:55:21 DEBUG opendrift.models.basemodel:755: Lifting 55 elements to seafloor.
12:55:21 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:636: 55 elements reached seafloor, interacting with bottom
12:55:21 DEBUG opendrift.models.basemodel:755: Lifting 55 elements to seafloor.
12:55:21 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:636: 55 elements reached seafloor, interacting with bottom
12:55:21 DEBUG opendrift.models.basemodel:755: Lifting 55 elements to seafloor.
12:55:21 DEBUG opendrift.models.oceandrift:618: 33 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:636: 56 elements reached seafloor, interacting with bottom
12:55:21 DEBUG opendrift.models.basemodel:755: Lifting 56 elements to seafloor.
12:55:21 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:636: 56 elements reached seafloor, interacting with bottom
12:55:21 DEBUG opendrift.models.basemodel:755: Lifting 56 elements to seafloor.
12:55:21 DEBUG opendrift.models.oceandrift:618: 33 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:636: 55 elements reached seafloor, interacting with bottom
12:55:21 DEBUG opendrift.models.basemodel:755: Lifting 55 elements to seafloor.
12:55:21 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:636: 55 elements reached seafloor, interacting with bottom
12:55:21 DEBUG opendrift.models.basemodel:755: Lifting 55 elements to seafloor.
12:55:21 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:636: 55 elements reached seafloor, interacting with bottom
12:55:21 DEBUG opendrift.models.basemodel:755: Lifting 55 elements to seafloor.
12:55:21 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:636: 55 elements reached seafloor, interacting with bottom
12:55:21 DEBUG opendrift.models.basemodel:755: Lifting 55 elements to seafloor.
12:55:21 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:636: 55 elements reached seafloor, interacting with bottom
12:55:21 DEBUG opendrift.models.basemodel:755: Lifting 55 elements to seafloor.
12:55:21 DEBUG opendrift.models.oceandrift:618: 33 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:636: 55 elements reached seafloor, interacting with bottom
12:55:21 DEBUG opendrift.models.basemodel:755: Lifting 55 elements to seafloor.
12:55:21 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:636: 55 elements reached seafloor, interacting with bottom
12:55:21 DEBUG opendrift.models.basemodel:755: Lifting 55 elements to seafloor.
12:55:21 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:636: 55 elements reached seafloor, interacting with bottom
12:55:21 DEBUG opendrift.models.basemodel:755: Lifting 55 elements to seafloor.
12:55:21 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:636: 55 elements reached seafloor, interacting with bottom
12:55:21 DEBUG opendrift.models.basemodel:755: Lifting 55 elements to seafloor.
12:55:21 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:636: 55 elements reached seafloor, interacting with bottom
12:55:21 DEBUG opendrift.models.basemodel:755: Lifting 55 elements to seafloor.
12:55:21 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:636: 55 elements reached seafloor, interacting with bottom
12:55:21 DEBUG opendrift.models.basemodel:755: Lifting 55 elements to seafloor.
12:55:21 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:636: 56 elements reached seafloor, interacting with bottom
12:55:21 DEBUG opendrift.models.basemodel:755: Lifting 56 elements to seafloor.
12:55:21 DEBUG opendrift.models.oceandrift:618: 33 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:636: 55 elements reached seafloor, interacting with bottom
12:55:21 DEBUG opendrift.models.basemodel:755: Lifting 55 elements to seafloor.
12:55:21 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:636: 56 elements reached seafloor, interacting with bottom
12:55:21 DEBUG opendrift.models.basemodel:755: Lifting 56 elements to seafloor.
12:55:21 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:636: 55 elements reached seafloor, interacting with bottom
12:55:21 DEBUG opendrift.models.basemodel:755: Lifting 55 elements to seafloor.
12:55:21 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:636: 55 elements reached seafloor, interacting with bottom
12:55:21 DEBUG opendrift.models.basemodel:755: Lifting 55 elements to seafloor.
12:55:21 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:636: 56 elements reached seafloor, interacting with bottom
12:55:21 DEBUG opendrift.models.basemodel:755: Lifting 56 elements to seafloor.
12:55:21 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 8
12:55:21 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:55:21 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 7 elements
12:55:21 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:21 INFO opendrift.models.chemicaldrift:1861: partitioning: [138, 0, 251, 111, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:55:21 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:55:21 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:55:21 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:55:21 DEBUG opendrift.models.basemodel:755: Lifting 55 elements to seafloor.
12:55:21 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:21 INFO opendrift.models.basemodel:2038: 2024-11-03 02:54:02.760182 - step 77 of 96 - 500 active elements (0 deactivated)
12:55:21 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:21 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:21 DEBUG opendrift.models.basemodel:2057: 57.551940261362994 <- latitude -> 57.74349818099065
12:55:21 DEBUG opendrift.models.basemodel:2062: 10.507927244297786 <- longitude -> 10.905389518820304
12:55:21 DEBUG opendrift.models.basemodel:2067: -35.38302993774414 <- z -> 0.0
12:55:21 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:21 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:21 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:55:21 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:21 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:55:21 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:21 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:21 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:55:21 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-03 02:00:00 (before)
2024-11-03 03:00:00 (after)
12:55:21 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-03 02:00:00) in space (linearNDFast)
12:55:21 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:21 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-03 03:00:00) in space (linearNDFast)
12:55:21 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:21 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-03 02:00:00, weight 0.10) and
after (2024-11-03 03:00:00, weight 0.90) in time
12:55:21 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:55:21 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.094621496337595 degrees.
12:55:21 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.094621496337595 degrees.
12:55:21 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:21 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:21 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:21 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:21 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:21 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:21 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:21 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:55:21 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:21 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:21 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:21 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:21 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:21 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:21 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:21 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:21 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:21 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:55:21 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:21 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:21 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:21 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:21 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:21 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:21 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:55:21 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:55:21 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:55:21 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:55:21 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:21 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.107537 (min) 0.30618 (max)
12:55:21 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.291227 (min) 0.109451 (max)
12:55:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.0204012 (min) 0.0588616 (max)
12:55:21 DEBUG opendrift.models.basemodel.environment:905: x_wind: 10.4483 (min) 13.6462 (max)
12:55:21 DEBUG opendrift.models.basemodel.environment:905: y_wind: 3.28825 (min) 3.71748 (max)
12:55:21 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:21 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 35.383 (max)
12:55:21 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:55:21 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 11.9068 (min) 12.6491 (max)
12:55:21 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0034 (min) 33.609 (max)
12:55:21 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000136498 (min) 0.000546007 (max)
12:55:21 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:55:21 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:55:21 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:55:21 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:55:21 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:55:21 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:55:21 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:21 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:55:21 DEBUG opendrift.models.basemodel:750: No elements hit seafloor.
12:55:21 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:21 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:55:21 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:55:21 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:55:21 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 5
12:55:21 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 2 2 2]
12:55:21 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 0 0 0]
12:55:21 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 546. 0.]
[ 0. 0. 0. 0. 0.]
[223. 0. 0. 503. 0.]
[ 13. 0. 923. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:55:21 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:55:21 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:21 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:55:21 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:55:21 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.1432171977577045
12:55:21 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:21 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:55:21 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:55:21 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:55:21 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:55:21 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:55:21 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:55:21 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:55:21 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:55:21 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:55:21 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:55:21 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:618: 33 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:55:21 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:55:22 DEBUG opendrift.models.oceandrift:618: 36 elements penetrated seafloor, lifting up
12:55:22 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:55:22 DEBUG opendrift.models.oceandrift:618: 34 elements penetrated seafloor, lifting up
12:55:22 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 17
12:55:22 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:55:22 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 16 elements
12:55:22 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:22 INFO opendrift.models.chemicaldrift:1861: partitioning: [139, 0, 264, 97, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:55:22 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:55:22 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:55:22 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:55:22 DEBUG opendrift.models.basemodel:750: No elements hit seafloor.
12:55:22 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:22 INFO opendrift.models.basemodel:2038: 2024-11-03 03:24:02.760182 - step 78 of 96 - 500 active elements (0 deactivated)
12:55:22 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:22 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:22 DEBUG opendrift.models.basemodel:2057: 57.551940261362994 <- latitude -> 57.740259268228876
12:55:22 DEBUG opendrift.models.basemodel:2062: 10.507927244297786 <- longitude -> 10.908714729132955
12:55:22 DEBUG opendrift.models.basemodel:2067: -33.887704631100405 <- z -> 0.0
12:55:22 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:22 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:22 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:55:22 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:22 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:55:22 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:22 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:22 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:55:22 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-03 03:00:00 (before)
2024-11-03 04:00:00 (after)
12:55:23 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
12:55:23 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:55:23 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:55:23 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:55:23 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:55:23 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'upward_sea_water_velocity', 'y_sea_water_velocity', 'sea_water_temperature', 'sea_water_salinity']
12:55:23 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 57x41x7) for time after (2024-11-03 04:00:00)
12:55:23 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-03 03:00:00) in space (linearNDFast)
12:55:23 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:23 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-03 04:00:00) in space (linearNDFast)
12:55:23 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:23 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-03 03:00:00, weight 0.60) and
after (2024-11-03 04:00:00, weight 0.40) in time
12:55:23 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:55:23 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.09129628340517 degrees.
12:55:23 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.09129628340517 degrees.
12:55:23 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:23 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:23 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:23 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:23 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:23 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:23 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:23 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:55:23 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:23 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:23 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:23 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:23 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:23 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:23 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:23 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:23 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:23 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:55:23 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:23 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:23 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:23 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:23 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:23 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:23 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:55:23 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:55:23 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:55:23 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:55:23 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:23 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.121741 (min) 0.261695 (max)
12:55:23 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.287647 (min) 0.075013 (max)
12:55:23 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.0486363 (min) 0.076393 (max)
12:55:23 DEBUG opendrift.models.basemodel.environment:905: x_wind: 11.257 (min) 13.5033 (max)
12:55:23 DEBUG opendrift.models.basemodel.environment:905: y_wind: 2.7973 (min) 3.40071 (max)
12:55:23 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:23 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 34.6041 (max)
12:55:23 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:55:23 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 11.9199 (min) 12.6324 (max)
12:55:23 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0164 (min) 33.5386 (max)
12:55:23 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -4.61158e-05 (min) 0.000525171 (max)
12:55:23 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:55:23 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:55:23 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:55:23 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:55:23 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:55:23 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:55:23 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:23 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:55:23 DEBUG opendrift.models.basemodel:750: No elements hit seafloor.
12:55:23 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:23 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:55:23 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:55:23 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:55:23 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 6
12:55:23 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 0 2 0 0 0]
12:55:23 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 3 0 3 3 3]
12:55:23 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 550. 0.]
[ 0. 0. 0. 0. 0.]
[225. 0. 0. 503. 0.]
[ 13. 0. 939. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:55:23 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:55:23 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:23 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:55:23 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:55:23 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.1395818007655069
12:55:23 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:23 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:55:23 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:55:23 DEBUG opendrift.models.oceandrift:618: 34 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:618: 32 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:55:23 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:55:23 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:55:23 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:55:23 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:55:23 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:55:23 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:55:23 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:55:23 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:55:23 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:55:23 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:55:23 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:55:23 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:55:23 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:55:23 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:55:23 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:55:23 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:55:23 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:55:23 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:618: 32 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:55:23 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:55:23 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 4
12:55:23 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:55:23 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 4 elements
12:55:23 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:23 INFO opendrift.models.chemicaldrift:1861: partitioning: [137, 0, 266, 97, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:55:23 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:55:23 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:55:23 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:55:23 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:55:23 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:23 INFO opendrift.models.basemodel:2038: 2024-11-03 03:54:02.760182 - step 79 of 96 - 500 active elements (0 deactivated)
12:55:23 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:23 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:23 DEBUG opendrift.models.basemodel:2057: 57.551940261362994 <- latitude -> 57.737056736128956
12:55:23 DEBUG opendrift.models.basemodel:2062: 10.507927244297786 <- longitude -> 10.912109616089696
12:55:23 DEBUG opendrift.models.basemodel:2067: -30.314208949727174 <- z -> 0.0
12:55:23 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:23 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:23 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:55:23 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:23 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:55:23 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:23 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:23 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:55:23 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-03 03:00:00 (before)
2024-11-03 04:00:00 (after)
12:55:23 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-03 03:00:00) in space (linearNDFast)
12:55:23 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:23 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-03 04:00:00) in space (linearNDFast)
12:55:23 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:23 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-03 03:00:00, weight 0.10) and
after (2024-11-03 04:00:00, weight 0.90) in time
12:55:23 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:55:23 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.0879013898558 degrees.
12:55:23 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.0879013898558 degrees.
12:55:23 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:23 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:23 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:23 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:23 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:23 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:23 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:23 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:55:23 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:23 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:23 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:23 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:23 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:23 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:23 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:23 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:23 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:23 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:55:23 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:23 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:23 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:23 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:23 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:23 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:23 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:55:23 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:55:23 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:55:23 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:55:23 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:23 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0815316 (min) 0.241198 (max)
12:55:23 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.291961 (min) 0.0642425 (max)
12:55:23 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.0709433 (min) 0.08754 (max)
12:55:23 DEBUG opendrift.models.basemodel.environment:905: x_wind: 11.7791 (min) 13.3838 (max)
12:55:23 DEBUG opendrift.models.basemodel.environment:905: y_wind: 2.09059 (min) 3.00333 (max)
12:55:23 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:23 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 33.8333 (max)
12:55:23 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:55:23 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 11.9315 (min) 12.6148 (max)
12:55:23 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0295 (min) 33.47 (max)
12:55:23 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -5.84029e-05 (min) 0.000466748 (max)
12:55:23 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:55:23 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:55:23 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:55:23 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:55:23 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:55:23 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:55:23 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:23 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:55:23 DEBUG opendrift.models.basemodel:750: No elements hit seafloor.
12:55:23 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:23 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:55:23 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:55:23 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:55:23 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 2
12:55:23 DEBUG opendrift.models.chemicaldrift:1452: old species: [3 0]
12:55:23 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 3]
12:55:23 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 551. 0.]
[ 0. 0. 0. 0. 0.]
[225. 0. 0. 503. 0.]
[ 14. 0. 943. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:55:23 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:55:23 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:23 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:55:23 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:55:23 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.13573385609983735
12:55:23 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:23 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:55:23 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:55:23 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:55:23 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:55:23 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:55:23 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:55:23 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:55:23 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:55:23 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
12:55:23 DEBUG opendrift.models.basemodel:755: Lifting 2 elements to seafloor.
12:55:23 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:55:23 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:55:23 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
12:55:23 DEBUG opendrift.models.basemodel:755: Lifting 2 elements to seafloor.
12:55:23 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:55:23 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:55:23 DEBUG opendrift.models.oceandrift:618: 33 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
12:55:23 DEBUG opendrift.models.basemodel:755: Lifting 2 elements to seafloor.
12:55:23 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:55:23 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:55:23 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:23 DEBUG opendrift.models.oceandrift:636: 3 elements reached seafloor, interacting with bottom
12:55:23 DEBUG opendrift.models.basemodel:755: Lifting 3 elements to seafloor.
12:55:23 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 6
12:55:23 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:55:23 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 2 elements
12:55:23 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:23 INFO opendrift.models.chemicaldrift:1861: partitioning: [137, 0, 268, 95, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:55:23 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:55:23 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:55:23 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:55:23 DEBUG opendrift.models.basemodel:750: No elements hit seafloor.
12:55:23 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:23 INFO opendrift.models.basemodel:2038: 2024-11-03 04:24:02.760182 - step 80 of 96 - 500 active elements (0 deactivated)
12:55:23 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:23 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:23 DEBUG opendrift.models.basemodel:2057: 57.551940261362994 <- latitude -> 57.73410010995989
12:55:23 DEBUG opendrift.models.basemodel:2062: 10.507927244297786 <- longitude -> 10.916491632912209
12:55:23 DEBUG opendrift.models.basemodel:2067: -29.03647440270521 <- z -> 0.0
12:55:23 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:23 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:23 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:55:23 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:23 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:55:23 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:23 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:23 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:55:23 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-03 04:00:00 (before)
2024-11-03 05:00:00 (after)
12:55:25 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
12:55:25 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:55:25 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:55:25 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:55:25 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:55:25 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'upward_sea_water_velocity', 'y_sea_water_velocity', 'sea_water_temperature', 'sea_water_salinity']
12:55:25 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 57x41x7) for time after (2024-11-03 05:00:00)
12:55:25 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-03 04:00:00) in space (linearNDFast)
12:55:25 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:25 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-03 05:00:00) in space (linearNDFast)
12:55:25 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:25 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-03 04:00:00, weight 0.60) and
after (2024-11-03 05:00:00, weight 0.40) in time
12:55:25 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:55:25 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.08351937568545 degrees.
12:55:25 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.08351937568545 degrees.
12:55:25 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:25 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:25 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:25 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:25 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:25 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:25 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:25 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:55:25 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:25 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:25 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:25 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:25 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:25 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:25 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:25 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:25 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:25 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:55:25 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:25 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:25 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:25 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:25 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:25 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:25 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:55:25 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:55:25 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:55:25 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:55:25 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:25 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0793043 (min) 0.218757 (max)
12:55:25 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.280825 (min) 0.102658 (max)
12:55:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.0579555 (min) 0.0749855 (max)
12:55:25 DEBUG opendrift.models.basemodel.environment:905: x_wind: 11.4773 (min) 13.1717 (max)
12:55:25 DEBUG opendrift.models.basemodel.environment:905: y_wind: 1.49239 (min) 2.18489 (max)
12:55:25 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:25 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 33.2512 (max)
12:55:25 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:55:25 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 11.9507 (min) 12.6052 (max)
12:55:25 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.046 (min) 33.4879 (max)
12:55:25 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -7.11723e-05 (min) 0.000390683 (max)
12:55:25 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:55:25 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:55:25 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:55:25 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:55:25 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:55:25 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:55:25 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:25 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:55:25 DEBUG opendrift.models.basemodel:750: No elements hit seafloor.
12:55:25 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:25 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:55:25 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:55:25 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:55:25 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 9
12:55:25 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 2 2 0 2 2 0 2]
12:55:25 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 0 0 3 0 0 3 0]
12:55:25 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 555. 0.]
[ 0. 0. 0. 0. 0.]
[230. 0. 0. 503. 0.]
[ 14. 0. 945. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:55:25 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:55:25 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:25 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:55:25 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:55:25 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.12881187133308603
12:55:25 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:25 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:55:25 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:55:25 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:55:25 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:55:25 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:55:25 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:55:25 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:55:25 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:55:25 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:55:25 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:55:25 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:55:25 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:55:25 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
12:55:25 DEBUG opendrift.models.basemodel:755: Lifting 2 elements to seafloor.
12:55:25 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:618: 34 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:55:25 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:55:25 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:55:25 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:55:25 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
12:55:25 DEBUG opendrift.models.basemodel:755: Lifting 2 elements to seafloor.
12:55:25 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:55:25 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:55:25 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:25 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 1
12:55:25 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:55:25 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 1 elements
12:55:25 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:25 INFO opendrift.models.chemicaldrift:1861: partitioning: [138, 0, 264, 98, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:55:25 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:55:25 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:55:25 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:55:25 DEBUG opendrift.models.basemodel:750: No elements hit seafloor.
12:55:25 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:25 INFO opendrift.models.basemodel:2038: 2024-11-03 04:54:02.760182 - step 81 of 96 - 500 active elements (0 deactivated)
12:55:25 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:25 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:25 DEBUG opendrift.models.basemodel:2057: 57.551940261362994 <- latitude -> 57.731400870448844
12:55:25 DEBUG opendrift.models.basemodel:2062: 10.507927244297786 <- longitude -> 10.920626034778534
12:55:25 DEBUG opendrift.models.basemodel:2067: -30.719180881737906 <- z -> 0.0
12:55:25 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:25 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:25 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:55:25 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:25 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:55:25 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:25 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:25 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:55:25 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-03 04:00:00 (before)
2024-11-03 05:00:00 (after)
12:55:25 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-03 04:00:00) in space (linearNDFast)
12:55:25 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:25 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-03 05:00:00) in space (linearNDFast)
12:55:25 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:25 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-03 04:00:00, weight 0.10) and
after (2024-11-03 05:00:00, weight 0.90) in time
12:55:25 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:55:25 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.07938497411385 degrees.
12:55:25 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.07938497411385 degrees.
12:55:25 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:25 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:25 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:25 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:25 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:25 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:25 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:25 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:55:25 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:25 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:25 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:25 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:25 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:25 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:25 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:25 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:25 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:25 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:55:25 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:25 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:25 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:25 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:25 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:25 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:25 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:55:25 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:55:25 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:55:25 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:55:25 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:25 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0794634 (min) 0.210814 (max)
12:55:25 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.265142 (min) 0.153097 (max)
12:55:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.0391046 (min) 0.0573536 (max)
12:55:25 DEBUG opendrift.models.basemodel.environment:905: x_wind: 10.9716 (min) 13.1367 (max)
12:55:25 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0.9073 (min) 1.31526 (max)
12:55:25 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:25 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 32.6878 (max)
12:55:25 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:55:25 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 11.9511 (min) 12.5977 (max)
12:55:25 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0588 (min) 33.5401 (max)
12:55:25 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000151149 (min) 0.00026384 (max)
12:55:25 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:55:25 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:55:25 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:55:25 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:55:25 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:55:25 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:55:25 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:25 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:55:25 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:55:25 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:25 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:55:25 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:55:25 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:55:25 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 10
12:55:25 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 2 0 2 0 0 2 0 2 0]
12:55:25 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 0 3 0 3 3 0 3 0 3]
12:55:25 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 560. 0.]
[ 0. 0. 0. 0. 0.]
[235. 0. 0. 503. 0.]
[ 14. 0. 946. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:55:25 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:55:25 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:25 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:55:25 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:55:25 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.1255695131322028
12:55:25 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:25 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:55:25 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:55:25 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:55:25 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:55:25 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:55:25 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:55:25 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:55:25 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:55:25 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:55:25 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:55:25 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:55:25 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:55:25 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:55:25 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:55:25 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:25 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:55:25 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:55:25 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:55:25 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 2
12:55:25 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:55:25 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:55:25 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:25 INFO opendrift.models.chemicaldrift:1861: partitioning: [138, 0, 259, 103, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:55:25 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:55:25 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:55:25 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:55:25 DEBUG opendrift.models.basemodel:750: No elements hit seafloor.
12:55:25 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:25 INFO opendrift.models.basemodel:2038: 2024-11-03 05:24:02.760182 - step 82 of 96 - 500 active elements (0 deactivated)
12:55:25 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:25 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:25 DEBUG opendrift.models.basemodel:2057: 57.551940261362994 <- latitude -> 57.729443034150144
12:55:25 DEBUG opendrift.models.basemodel:2062: 10.507927244297786 <- longitude -> 10.926986270400926
12:55:25 DEBUG opendrift.models.basemodel:2067: -31.019152417445305 <- z -> 0.0
12:55:25 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:25 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:25 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:55:25 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:25 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:55:25 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:25 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:25 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:55:25 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-03 05:00:00 (before)
2024-11-03 06:00:00 (after)
12:55:28 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
12:55:28 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:55:28 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:55:28 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:55:28 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:55:28 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'upward_sea_water_velocity', 'y_sea_water_velocity', 'sea_water_temperature', 'sea_water_salinity']
12:55:28 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 56x41x7) for time after (2024-11-03 06:00:00)
12:55:28 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-03 05:00:00) in space (linearNDFast)
12:55:28 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:28 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-03 06:00:00) in space (linearNDFast)
12:55:28 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:28 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-03 05:00:00, weight 0.60) and
after (2024-11-03 06:00:00, weight 0.40) in time
12:55:28 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:55:28 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.073024741018706 degrees.
12:55:28 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.073024741018706 degrees.
12:55:28 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:28 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:28 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:28 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:28 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:28 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:28 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:28 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:55:28 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:28 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:28 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:28 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:28 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:28 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:28 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:28 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:28 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:28 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:55:28 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:28 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:28 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:28 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:28 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:28 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:28 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:55:28 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:55:28 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:55:28 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:55:28 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:28 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.077497 (min) 0.195451 (max)
12:55:28 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.23832 (min) 0.161617 (max)
12:55:28 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.00197819 (min) 0.0205703 (max)
12:55:28 DEBUG opendrift.models.basemodel.environment:905: x_wind: 11.0865 (min) 13.1965 (max)
12:55:28 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0.789179 (min) 1.04498 (max)
12:55:28 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:28 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 32.2981 (max)
12:55:28 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:55:28 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 11.9486 (min) 12.6 (max)
12:55:28 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0621 (min) 33.6119 (max)
12:55:28 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000187938 (min) 0.000220126 (max)
12:55:28 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:55:28 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:55:28 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:55:28 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:55:28 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:55:28 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:55:28 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:28 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:55:28 DEBUG opendrift.models.basemodel:750: No elements hit seafloor.
12:55:28 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:28 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:55:28 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:55:28 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:55:28 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 10
12:55:28 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 2 2 2 2 0 0 0 2 2]
12:55:28 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 0 0 0 0 3 3 3 0 0]
12:55:28 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 564. 0.]
[ 0. 0. 0. 0. 0.]
[241. 0. 0. 503. 0.]
[ 14. 0. 946. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:55:28 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:55:28 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:28 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:55:28 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:55:28 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.1265206749154087
12:55:28 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:28 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:55:28 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:55:28 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:618: 37 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
12:55:28 DEBUG opendrift.models.basemodel:755: Lifting 2 elements to seafloor.
12:55:28 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
12:55:28 DEBUG opendrift.models.basemodel:755: Lifting 2 elements to seafloor.
12:55:28 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:55:28 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:55:28 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:55:28 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:55:28 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:55:28 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:55:28 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:55:28 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:55:28 DEBUG opendrift.models.oceandrift:618: 33 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
12:55:28 DEBUG opendrift.models.basemodel:755: Lifting 1 elements to seafloor.
12:55:28 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:28 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 1
12:55:28 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:55:28 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 1 elements
12:55:28 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:28 INFO opendrift.models.chemicaldrift:1861: partitioning: [140, 0, 254, 106, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:55:28 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:55:28 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:55:28 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:55:28 DEBUG opendrift.models.basemodel:750: No elements hit seafloor.
12:55:28 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:28 INFO opendrift.models.basemodel:2038: 2024-11-03 05:54:02.760182 - step 83 of 96 - 500 active elements (0 deactivated)
12:55:28 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:28 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:28 DEBUG opendrift.models.basemodel:2057: 57.551940261362994 <- latitude -> 57.72811091125663
12:55:28 DEBUG opendrift.models.basemodel:2062: 10.50792724429779 <- longitude -> 10.930283085187547
12:55:28 DEBUG opendrift.models.basemodel:2067: -31.301779383346737 <- z -> -0.11440301039516182
12:55:28 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:28 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:28 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:55:28 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:28 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:55:28 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:28 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:28 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:55:28 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-03 05:00:00 (before)
2024-11-03 06:00:00 (after)
12:55:28 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-03 05:00:00) in space (linearNDFast)
12:55:28 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:28 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-03 06:00:00) in space (linearNDFast)
12:55:28 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:28 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-03 05:00:00, weight 0.10) and
after (2024-11-03 06:00:00, weight 0.90) in time
12:55:28 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:55:28 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.0697279249464 degrees.
12:55:28 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.0697279249464 degrees.
12:55:28 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:28 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:28 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:28 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:28 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:28 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:28 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:28 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:55:28 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:28 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:28 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:28 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:28 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:28 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:28 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:28 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:28 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:28 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:55:28 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:28 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:28 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:28 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:28 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:28 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:28 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:55:28 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:55:28 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:55:28 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:55:28 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:28 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0750042 (min) 0.233032 (max)
12:55:28 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.211315 (min) 0.200241 (max)
12:55:28 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.0430158 (min) -0.0189971 (max)
12:55:28 DEBUG opendrift.models.basemodel.environment:905: x_wind: 11.168 (min) 13.2591 (max)
12:55:28 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0.676349 (min) 1.11219 (max)
12:55:28 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:28 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 32.1756 (max)
12:55:28 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:55:28 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 11.946 (min) 12.6048 (max)
12:55:28 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0655 (min) 33.5868 (max)
12:55:28 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000218552 (min) 0.00020476 (max)
12:55:28 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:55:28 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:55:28 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:55:28 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:55:28 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:55:28 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:55:28 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:28 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:55:28 DEBUG opendrift.models.basemodel:755: Lifting 106 elements to seafloor.
12:55:28 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:28 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:55:28 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:55:28 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:55:28 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 5
12:55:28 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 0 2]
12:55:28 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 3 0]
12:55:28 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 568. 0.]
[ 0. 0. 0. 0. 0.]
[242. 0. 0. 503. 0.]
[ 14. 0. 947. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:55:28 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:55:28 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:28 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:55:28 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:55:28 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.1278783759600531
12:55:28 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:28 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:55:28 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:55:28 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:636: 111 elements reached seafloor, interacting with bottom
12:55:28 DEBUG opendrift.models.basemodel:755: Lifting 111 elements to seafloor.
12:55:28 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:636: 111 elements reached seafloor, interacting with bottom
12:55:28 DEBUG opendrift.models.basemodel:755: Lifting 111 elements to seafloor.
12:55:28 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:636: 111 elements reached seafloor, interacting with bottom
12:55:28 DEBUG opendrift.models.basemodel:755: Lifting 111 elements to seafloor.
12:55:28 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:636: 112 elements reached seafloor, interacting with bottom
12:55:28 DEBUG opendrift.models.basemodel:755: Lifting 112 elements to seafloor.
12:55:28 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:636: 112 elements reached seafloor, interacting with bottom
12:55:28 DEBUG opendrift.models.basemodel:755: Lifting 112 elements to seafloor.
12:55:28 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:636: 112 elements reached seafloor, interacting with bottom
12:55:28 DEBUG opendrift.models.basemodel:755: Lifting 112 elements to seafloor.
12:55:28 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:636: 113 elements reached seafloor, interacting with bottom
12:55:28 DEBUG opendrift.models.basemodel:755: Lifting 113 elements to seafloor.
12:55:28 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:636: 113 elements reached seafloor, interacting with bottom
12:55:28 DEBUG opendrift.models.basemodel:755: Lifting 113 elements to seafloor.
12:55:28 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:636: 114 elements reached seafloor, interacting with bottom
12:55:28 DEBUG opendrift.models.basemodel:755: Lifting 114 elements to seafloor.
12:55:28 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:636: 114 elements reached seafloor, interacting with bottom
12:55:28 DEBUG opendrift.models.basemodel:755: Lifting 114 elements to seafloor.
12:55:28 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:636: 115 elements reached seafloor, interacting with bottom
12:55:28 DEBUG opendrift.models.basemodel:755: Lifting 115 elements to seafloor.
12:55:28 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:636: 115 elements reached seafloor, interacting with bottom
12:55:28 DEBUG opendrift.models.basemodel:755: Lifting 115 elements to seafloor.
12:55:28 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:636: 116 elements reached seafloor, interacting with bottom
12:55:28 DEBUG opendrift.models.basemodel:755: Lifting 116 elements to seafloor.
12:55:28 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:636: 116 elements reached seafloor, interacting with bottom
12:55:28 DEBUG opendrift.models.basemodel:755: Lifting 116 elements to seafloor.
12:55:28 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:636: 116 elements reached seafloor, interacting with bottom
12:55:28 DEBUG opendrift.models.basemodel:755: Lifting 116 elements to seafloor.
12:55:28 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:636: 116 elements reached seafloor, interacting with bottom
12:55:28 DEBUG opendrift.models.basemodel:755: Lifting 116 elements to seafloor.
12:55:28 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:636: 117 elements reached seafloor, interacting with bottom
12:55:28 DEBUG opendrift.models.basemodel:755: Lifting 117 elements to seafloor.
12:55:28 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:636: 117 elements reached seafloor, interacting with bottom
12:55:28 DEBUG opendrift.models.basemodel:755: Lifting 117 elements to seafloor.
12:55:28 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:636: 118 elements reached seafloor, interacting with bottom
12:55:28 DEBUG opendrift.models.basemodel:755: Lifting 118 elements to seafloor.
12:55:28 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:636: 119 elements reached seafloor, interacting with bottom
12:55:28 DEBUG opendrift.models.basemodel:755: Lifting 119 elements to seafloor.
12:55:28 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:636: 119 elements reached seafloor, interacting with bottom
12:55:28 DEBUG opendrift.models.basemodel:755: Lifting 119 elements to seafloor.
12:55:28 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:636: 119 elements reached seafloor, interacting with bottom
12:55:28 DEBUG opendrift.models.basemodel:755: Lifting 119 elements to seafloor.
12:55:28 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:636: 120 elements reached seafloor, interacting with bottom
12:55:28 DEBUG opendrift.models.basemodel:755: Lifting 120 elements to seafloor.
12:55:28 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:636: 120 elements reached seafloor, interacting with bottom
12:55:28 DEBUG opendrift.models.basemodel:755: Lifting 120 elements to seafloor.
12:55:28 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:636: 120 elements reached seafloor, interacting with bottom
12:55:28 DEBUG opendrift.models.basemodel:755: Lifting 120 elements to seafloor.
12:55:28 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:636: 120 elements reached seafloor, interacting with bottom
12:55:28 DEBUG opendrift.models.basemodel:755: Lifting 120 elements to seafloor.
12:55:28 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:636: 120 elements reached seafloor, interacting with bottom
12:55:28 DEBUG opendrift.models.basemodel:755: Lifting 120 elements to seafloor.
12:55:28 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:636: 121 elements reached seafloor, interacting with bottom
12:55:28 DEBUG opendrift.models.basemodel:755: Lifting 121 elements to seafloor.
12:55:28 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:636: 122 elements reached seafloor, interacting with bottom
12:55:28 DEBUG opendrift.models.basemodel:755: Lifting 122 elements to seafloor.
12:55:28 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:28 DEBUG opendrift.models.oceandrift:636: 122 elements reached seafloor, interacting with bottom
12:55:28 DEBUG opendrift.models.basemodel:755: Lifting 122 elements to seafloor.
12:55:28 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 3
12:55:28 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:55:28 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 3 elements
12:55:28 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:28 INFO opendrift.models.chemicaldrift:1861: partitioning: [137, 0, 244, 119, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:55:28 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:55:28 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:55:28 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:55:28 DEBUG opendrift.models.basemodel:755: Lifting 119 elements to seafloor.
12:55:28 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:28 INFO opendrift.models.basemodel:2038: 2024-11-03 06:24:02.760182 - step 84 of 96 - 500 active elements (0 deactivated)
12:55:28 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:28 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:28 DEBUG opendrift.models.basemodel:2057: 57.551940261363 <- latitude -> 57.72719873868593
12:55:28 DEBUG opendrift.models.basemodel:2062: 10.507927244297786 <- longitude -> 10.932497522176444
12:55:28 DEBUG opendrift.models.basemodel:2067: -30.610788345336914 <- z -> 0.0
12:55:28 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:28 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:28 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:55:28 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:28 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:55:28 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:28 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:28 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:55:28 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-03 06:00:00 (before)
2024-11-03 07:00:00 (after)
12:55:30 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
12:55:30 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:55:30 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:55:30 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:55:30 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:55:30 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'upward_sea_water_velocity', 'y_sea_water_velocity', 'sea_water_temperature', 'sea_water_salinity']
12:55:30 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 57x41x7) for time after (2024-11-03 07:00:00)
12:55:30 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-03 06:00:00) in space (linearNDFast)
12:55:30 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:30 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-03 07:00:00) in space (linearNDFast)
12:55:30 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:30 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-03 06:00:00, weight 0.60) and
after (2024-11-03 07:00:00, weight 0.40) in time
12:55:30 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:55:30 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.067513499257295 degrees.
12:55:30 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.067513499257295 degrees.
12:55:30 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:30 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:30 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:30 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:30 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:30 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:30 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:30 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:55:30 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:30 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:30 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:30 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:30 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:30 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:30 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:30 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:30 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:30 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:55:30 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:30 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:30 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:30 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:30 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:30 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:30 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:55:30 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:55:30 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:55:30 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:55:30 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:30 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0786255 (min) 0.235987 (max)
12:55:30 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.181364 (min) 0.200985 (max)
12:55:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.0890056 (min) -0.0587582 (max)
12:55:30 DEBUG opendrift.models.basemodel.environment:905: x_wind: 11.0457 (min) 13.456 (max)
12:55:30 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0.645193 (min) 1.36483 (max)
12:55:30 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:30 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 32.4917 (max)
12:55:30 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:55:30 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 11.9446 (min) 12.6099 (max)
12:55:30 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0699 (min) 33.5848 (max)
12:55:30 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000193091 (min) 0.000182606 (max)
12:55:30 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:55:30 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:55:30 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:55:30 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:55:30 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:55:30 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:55:30 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:30 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:55:30 DEBUG opendrift.models.basemodel:755: Lifting 119 elements to seafloor.
12:55:30 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:30 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:55:30 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:55:30 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:55:30 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 8
12:55:30 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 2 2 0 0 0]
12:55:30 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 0 0 3 3 3]
12:55:30 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 574. 0.]
[ 0. 0. 0. 0. 0.]
[244. 0. 0. 515. 0.]
[ 14. 0. 950. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:55:30 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:55:30 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:30 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:55:30 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:55:30 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.13184735883642817
12:55:30 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:30 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:55:30 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:55:30 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:30 DEBUG opendrift.models.oceandrift:636: 125 elements reached seafloor, interacting with bottom
12:55:30 DEBUG opendrift.models.basemodel:755: Lifting 125 elements to seafloor.
12:55:30 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:30 DEBUG opendrift.models.oceandrift:636: 125 elements reached seafloor, interacting with bottom
12:55:30 DEBUG opendrift.models.basemodel:755: Lifting 125 elements to seafloor.
12:55:30 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:55:30 DEBUG opendrift.models.oceandrift:636: 125 elements reached seafloor, interacting with bottom
12:55:30 DEBUG opendrift.models.basemodel:755: Lifting 125 elements to seafloor.
12:55:30 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:30 DEBUG opendrift.models.oceandrift:636: 125 elements reached seafloor, interacting with bottom
12:55:30 DEBUG opendrift.models.basemodel:755: Lifting 125 elements to seafloor.
12:55:30 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:30 DEBUG opendrift.models.oceandrift:636: 125 elements reached seafloor, interacting with bottom
12:55:30 DEBUG opendrift.models.basemodel:755: Lifting 125 elements to seafloor.
12:55:30 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:30 DEBUG opendrift.models.oceandrift:636: 126 elements reached seafloor, interacting with bottom
12:55:30 DEBUG opendrift.models.basemodel:755: Lifting 126 elements to seafloor.
12:55:30 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:30 DEBUG opendrift.models.oceandrift:636: 126 elements reached seafloor, interacting with bottom
12:55:30 DEBUG opendrift.models.basemodel:755: Lifting 126 elements to seafloor.
12:55:30 DEBUG opendrift.models.oceandrift:618: 36 elements penetrated seafloor, lifting up
12:55:30 DEBUG opendrift.models.oceandrift:636: 126 elements reached seafloor, interacting with bottom
12:55:30 DEBUG opendrift.models.basemodel:755: Lifting 126 elements to seafloor.
12:55:30 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:55:30 DEBUG opendrift.models.oceandrift:636: 126 elements reached seafloor, interacting with bottom
12:55:30 DEBUG opendrift.models.basemodel:755: Lifting 126 elements to seafloor.
12:55:30 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:30 DEBUG opendrift.models.oceandrift:636: 126 elements reached seafloor, interacting with bottom
12:55:30 DEBUG opendrift.models.basemodel:755: Lifting 126 elements to seafloor.
12:55:30 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
12:55:30 DEBUG opendrift.models.oceandrift:636: 126 elements reached seafloor, interacting with bottom
12:55:30 DEBUG opendrift.models.basemodel:755: Lifting 126 elements to seafloor.
12:55:30 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:55:30 DEBUG opendrift.models.oceandrift:636: 126 elements reached seafloor, interacting with bottom
12:55:30 DEBUG opendrift.models.basemodel:755: Lifting 126 elements to seafloor.
12:55:30 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:30 DEBUG opendrift.models.oceandrift:636: 126 elements reached seafloor, interacting with bottom
12:55:30 DEBUG opendrift.models.basemodel:755: Lifting 126 elements to seafloor.
12:55:30 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:30 DEBUG opendrift.models.oceandrift:636: 127 elements reached seafloor, interacting with bottom
12:55:30 DEBUG opendrift.models.basemodel:755: Lifting 127 elements to seafloor.
12:55:30 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:55:30 DEBUG opendrift.models.oceandrift:636: 127 elements reached seafloor, interacting with bottom
12:55:30 DEBUG opendrift.models.basemodel:755: Lifting 127 elements to seafloor.
12:55:30 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:55:30 DEBUG opendrift.models.oceandrift:636: 127 elements reached seafloor, interacting with bottom
12:55:30 DEBUG opendrift.models.basemodel:755: Lifting 127 elements to seafloor.
12:55:30 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:30 DEBUG opendrift.models.oceandrift:636: 127 elements reached seafloor, interacting with bottom
12:55:30 DEBUG opendrift.models.basemodel:755: Lifting 127 elements to seafloor.
12:55:30 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:30 DEBUG opendrift.models.oceandrift:636: 127 elements reached seafloor, interacting with bottom
12:55:30 DEBUG opendrift.models.basemodel:755: Lifting 127 elements to seafloor.
12:55:30 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:55:30 DEBUG opendrift.models.oceandrift:636: 127 elements reached seafloor, interacting with bottom
12:55:30 DEBUG opendrift.models.basemodel:755: Lifting 127 elements to seafloor.
12:55:30 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:30 DEBUG opendrift.models.oceandrift:636: 127 elements reached seafloor, interacting with bottom
12:55:30 DEBUG opendrift.models.basemodel:755: Lifting 127 elements to seafloor.
12:55:30 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:55:30 DEBUG opendrift.models.oceandrift:636: 127 elements reached seafloor, interacting with bottom
12:55:30 DEBUG opendrift.models.basemodel:755: Lifting 127 elements to seafloor.
12:55:30 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:30 DEBUG opendrift.models.oceandrift:636: 128 elements reached seafloor, interacting with bottom
12:55:30 DEBUG opendrift.models.basemodel:755: Lifting 128 elements to seafloor.
12:55:30 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:30 DEBUG opendrift.models.oceandrift:636: 128 elements reached seafloor, interacting with bottom
12:55:30 DEBUG opendrift.models.basemodel:755: Lifting 128 elements to seafloor.
12:55:30 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:30 DEBUG opendrift.models.oceandrift:636: 128 elements reached seafloor, interacting with bottom
12:55:30 DEBUG opendrift.models.basemodel:755: Lifting 128 elements to seafloor.
12:55:30 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:30 DEBUG opendrift.models.oceandrift:636: 129 elements reached seafloor, interacting with bottom
12:55:30 DEBUG opendrift.models.basemodel:755: Lifting 129 elements to seafloor.
12:55:30 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:55:30 DEBUG opendrift.models.oceandrift:636: 129 elements reached seafloor, interacting with bottom
12:55:30 DEBUG opendrift.models.basemodel:755: Lifting 129 elements to seafloor.
12:55:30 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:30 DEBUG opendrift.models.oceandrift:636: 129 elements reached seafloor, interacting with bottom
12:55:30 DEBUG opendrift.models.basemodel:755: Lifting 129 elements to seafloor.
12:55:30 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:55:30 DEBUG opendrift.models.oceandrift:636: 129 elements reached seafloor, interacting with bottom
12:55:30 DEBUG opendrift.models.basemodel:755: Lifting 129 elements to seafloor.
12:55:30 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:55:30 DEBUG opendrift.models.oceandrift:636: 129 elements reached seafloor, interacting with bottom
12:55:30 DEBUG opendrift.models.basemodel:755: Lifting 129 elements to seafloor.
12:55:30 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:55:30 DEBUG opendrift.models.oceandrift:636: 129 elements reached seafloor, interacting with bottom
12:55:30 DEBUG opendrift.models.basemodel:755: Lifting 129 elements to seafloor.
12:55:30 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 4
12:55:30 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:55:30 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 4 elements
12:55:30 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:30 INFO opendrift.models.chemicaldrift:1861: partitioning: [133, 0, 242, 125, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:55:30 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:55:30 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:55:30 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:55:30 DEBUG opendrift.models.basemodel:755: Lifting 126 elements to seafloor.
12:55:30 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:30 INFO opendrift.models.basemodel:2038: 2024-11-03 06:54:02.760182 - step 85 of 96 - 500 active elements (0 deactivated)
12:55:30 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:30 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:30 DEBUG opendrift.models.basemodel:2057: 57.551940261363 <- latitude -> 57.72665211113996
12:55:30 DEBUG opendrift.models.basemodel:2062: 10.507927244297782 <- longitude -> 10.937716931378839
12:55:30 DEBUG opendrift.models.basemodel:2067: -30.763144181288407 <- z -> 0.0
12:55:30 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:30 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:30 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:55:30 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:30 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:55:30 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:30 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:30 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:55:30 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-03 06:00:00 (before)
2024-11-03 07:00:00 (after)
12:55:30 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-03 06:00:00) in space (linearNDFast)
12:55:30 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:30 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-03 07:00:00) in space (linearNDFast)
12:55:30 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:30 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-03 06:00:00, weight 0.10) and
after (2024-11-03 07:00:00, weight 0.90) in time
12:55:30 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:55:30 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.062294088191074 degrees.
12:55:30 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.062294088191074 degrees.
12:55:30 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:30 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:30 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:30 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:30 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:30 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:30 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:30 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:55:30 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:30 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:30 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:30 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:30 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:30 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:30 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:30 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:30 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:30 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:55:30 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:30 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:30 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:30 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:30 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:30 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:30 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:55:30 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:55:30 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:55:30 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:55:30 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:30 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0802252 (min) 0.24583 (max)
12:55:30 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.153983 (min) 0.199064 (max)
12:55:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.134743 (min) -0.0998938 (max)
12:55:30 DEBUG opendrift.models.basemodel.environment:905: x_wind: 10.8755 (min) 13.6895 (max)
12:55:30 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0.525238 (min) 1.90615 (max)
12:55:30 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:30 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 32.4734 (max)
12:55:30 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:55:30 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 11.9396 (min) 12.615 (max)
12:55:30 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0744 (min) 33.5865 (max)
12:55:30 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000192438 (min) 0.000164342 (max)
12:55:30 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:55:30 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:55:30 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:55:30 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:55:30 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:55:30 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:55:30 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:30 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:55:30 DEBUG opendrift.models.basemodel:755: Lifting 125 elements to seafloor.
12:55:30 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:30 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:55:30 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:55:30 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:55:30 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 7
12:55:30 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 2 0 2 0 2 0]
12:55:30 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 0 3 0 3 0 3]
12:55:30 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 577. 0.]
[ 0. 0. 0. 0. 0.]
[248. 0. 0. 519. 0.]
[ 14. 0. 954. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:55:30 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:55:30 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:30 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:55:30 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:55:30 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.13693337851910406
12:55:30 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:30 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:55:30 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:55:30 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:30 DEBUG opendrift.models.oceandrift:636: 128 elements reached seafloor, interacting with bottom
12:55:31 DEBUG opendrift.models.basemodel:755: Lifting 128 elements to seafloor.
12:55:31 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:31 DEBUG opendrift.models.oceandrift:636: 128 elements reached seafloor, interacting with bottom
12:55:31 DEBUG opendrift.models.basemodel:755: Lifting 128 elements to seafloor.
12:55:31 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:31 DEBUG opendrift.models.oceandrift:636: 128 elements reached seafloor, interacting with bottom
12:55:31 DEBUG opendrift.models.basemodel:755: Lifting 128 elements to seafloor.
12:55:31 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:31 DEBUG opendrift.models.oceandrift:636: 128 elements reached seafloor, interacting with bottom
12:55:31 DEBUG opendrift.models.basemodel:755: Lifting 128 elements to seafloor.
12:55:31 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:31 DEBUG opendrift.models.oceandrift:636: 129 elements reached seafloor, interacting with bottom
12:55:31 DEBUG opendrift.models.basemodel:755: Lifting 129 elements to seafloor.
12:55:31 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:31 DEBUG opendrift.models.oceandrift:636: 129 elements reached seafloor, interacting with bottom
12:55:31 DEBUG opendrift.models.basemodel:755: Lifting 129 elements to seafloor.
12:55:31 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:55:31 DEBUG opendrift.models.oceandrift:636: 129 elements reached seafloor, interacting with bottom
12:55:31 DEBUG opendrift.models.basemodel:755: Lifting 129 elements to seafloor.
12:55:31 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:31 DEBUG opendrift.models.oceandrift:636: 129 elements reached seafloor, interacting with bottom
12:55:31 DEBUG opendrift.models.basemodel:755: Lifting 129 elements to seafloor.
12:55:31 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:55:31 DEBUG opendrift.models.oceandrift:636: 129 elements reached seafloor, interacting with bottom
12:55:31 DEBUG opendrift.models.basemodel:755: Lifting 129 elements to seafloor.
12:55:31 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:31 DEBUG opendrift.models.oceandrift:636: 129 elements reached seafloor, interacting with bottom
12:55:31 DEBUG opendrift.models.basemodel:755: Lifting 129 elements to seafloor.
12:55:31 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:31 DEBUG opendrift.models.oceandrift:636: 129 elements reached seafloor, interacting with bottom
12:55:31 DEBUG opendrift.models.basemodel:755: Lifting 129 elements to seafloor.
12:55:31 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:31 DEBUG opendrift.models.oceandrift:636: 129 elements reached seafloor, interacting with bottom
12:55:31 DEBUG opendrift.models.basemodel:755: Lifting 129 elements to seafloor.
12:55:31 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
12:55:31 DEBUG opendrift.models.oceandrift:636: 129 elements reached seafloor, interacting with bottom
12:55:31 DEBUG opendrift.models.basemodel:755: Lifting 129 elements to seafloor.
12:55:31 DEBUG opendrift.models.oceandrift:618: 36 elements penetrated seafloor, lifting up
12:55:31 DEBUG opendrift.models.oceandrift:636: 129 elements reached seafloor, interacting with bottom
12:55:31 DEBUG opendrift.models.basemodel:755: Lifting 129 elements to seafloor.
12:55:31 DEBUG opendrift.models.oceandrift:618: 36 elements penetrated seafloor, lifting up
12:55:31 DEBUG opendrift.models.oceandrift:636: 129 elements reached seafloor, interacting with bottom
12:55:31 DEBUG opendrift.models.basemodel:755: Lifting 129 elements to seafloor.
12:55:31 DEBUG opendrift.models.oceandrift:618: 34 elements penetrated seafloor, lifting up
12:55:31 DEBUG opendrift.models.oceandrift:636: 129 elements reached seafloor, interacting with bottom
12:55:31 DEBUG opendrift.models.basemodel:755: Lifting 129 elements to seafloor.
12:55:31 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:55:31 DEBUG opendrift.models.oceandrift:636: 129 elements reached seafloor, interacting with bottom
12:55:31 DEBUG opendrift.models.basemodel:755: Lifting 129 elements to seafloor.
12:55:31 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:55:31 DEBUG opendrift.models.oceandrift:636: 129 elements reached seafloor, interacting with bottom
12:55:31 DEBUG opendrift.models.basemodel:755: Lifting 129 elements to seafloor.
12:55:31 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:31 DEBUG opendrift.models.oceandrift:636: 129 elements reached seafloor, interacting with bottom
12:55:31 DEBUG opendrift.models.basemodel:755: Lifting 129 elements to seafloor.
12:55:31 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:55:31 DEBUG opendrift.models.oceandrift:636: 129 elements reached seafloor, interacting with bottom
12:55:31 DEBUG opendrift.models.basemodel:755: Lifting 129 elements to seafloor.
12:55:31 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:55:31 DEBUG opendrift.models.oceandrift:636: 129 elements reached seafloor, interacting with bottom
12:55:31 DEBUG opendrift.models.basemodel:755: Lifting 129 elements to seafloor.
12:55:31 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:55:31 DEBUG opendrift.models.oceandrift:636: 129 elements reached seafloor, interacting with bottom
12:55:31 DEBUG opendrift.models.basemodel:755: Lifting 129 elements to seafloor.
12:55:31 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:31 DEBUG opendrift.models.oceandrift:636: 129 elements reached seafloor, interacting with bottom
12:55:31 DEBUG opendrift.models.basemodel:755: Lifting 129 elements to seafloor.
12:55:31 DEBUG opendrift.models.oceandrift:618: 34 elements penetrated seafloor, lifting up
12:55:31 DEBUG opendrift.models.oceandrift:636: 129 elements reached seafloor, interacting with bottom
12:55:31 DEBUG opendrift.models.basemodel:755: Lifting 129 elements to seafloor.
12:55:31 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:55:31 DEBUG opendrift.models.oceandrift:636: 129 elements reached seafloor, interacting with bottom
12:55:31 DEBUG opendrift.models.basemodel:755: Lifting 129 elements to seafloor.
12:55:31 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:31 DEBUG opendrift.models.oceandrift:636: 129 elements reached seafloor, interacting with bottom
12:55:31 DEBUG opendrift.models.basemodel:755: Lifting 129 elements to seafloor.
12:55:31 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:55:31 DEBUG opendrift.models.oceandrift:636: 129 elements reached seafloor, interacting with bottom
12:55:31 DEBUG opendrift.models.basemodel:755: Lifting 129 elements to seafloor.
12:55:31 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:55:31 DEBUG opendrift.models.oceandrift:636: 130 elements reached seafloor, interacting with bottom
12:55:31 DEBUG opendrift.models.basemodel:755: Lifting 130 elements to seafloor.
12:55:31 DEBUG opendrift.models.oceandrift:618: 33 elements penetrated seafloor, lifting up
12:55:31 DEBUG opendrift.models.oceandrift:636: 130 elements reached seafloor, interacting with bottom
12:55:31 DEBUG opendrift.models.basemodel:755: Lifting 130 elements to seafloor.
12:55:31 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:31 DEBUG opendrift.models.oceandrift:636: 130 elements reached seafloor, interacting with bottom
12:55:31 DEBUG opendrift.models.basemodel:755: Lifting 130 elements to seafloor.
12:55:31 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 12
12:55:31 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:55:31 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 12 elements
12:55:31 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:31 INFO opendrift.models.chemicaldrift:1861: partitioning: [134, 0, 248, 118, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:55:31 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:55:31 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:55:31 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:55:31 DEBUG opendrift.models.basemodel:755: Lifting 119 elements to seafloor.
12:55:31 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:31 INFO opendrift.models.basemodel:2038: 2024-11-03 07:24:02.760182 - step 86 of 96 - 500 active elements (0 deactivated)
12:55:31 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:31 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:31 DEBUG opendrift.models.basemodel:2057: 57.551940261363 <- latitude -> 57.72657633403384
12:55:31 DEBUG opendrift.models.basemodel:2062: 10.507927244297784 <- longitude -> 10.939749492221956
12:55:31 DEBUG opendrift.models.basemodel:2067: -31.01678466796875 <- z -> 0.0
12:55:31 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:31 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:31 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:55:31 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:31 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:55:31 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:31 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:31 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:55:31 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-03 07:00:00 (before)
2024-11-03 08:00:00 (after)
12:55:32 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
12:55:32 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:55:32 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:55:32 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:55:32 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:55:32 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'upward_sea_water_velocity', 'y_sea_water_velocity', 'sea_water_temperature', 'sea_water_salinity']
12:55:32 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 57x41x7) for time after (2024-11-03 08:00:00)
12:55:32 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-03 07:00:00) in space (linearNDFast)
12:55:32 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:32 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-03 08:00:00) in space (linearNDFast)
12:55:32 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:32 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-03 07:00:00, weight 0.60) and
after (2024-11-03 08:00:00, weight 0.40) in time
12:55:32 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:55:32 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.060261524224295 degrees.
12:55:32 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.060261524224295 degrees.
12:55:32 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:32 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:32 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:32 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:32 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:32 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:32 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:32 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:55:32 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:32 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:32 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:32 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:32 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:32 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:32 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:32 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:32 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:32 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:55:32 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:32 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:32 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:32 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:32 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:32 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:32 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:55:32 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:55:32 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:55:32 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:55:32 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:32 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.068379 (min) 0.240273 (max)
12:55:32 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.142218 (min) 0.184595 (max)
12:55:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.177067 (min) -0.132094 (max)
12:55:32 DEBUG opendrift.models.basemodel.environment:905: x_wind: 10.9381 (min) 13.9128 (max)
12:55:32 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0.800576 (min) 2.13531 (max)
12:55:32 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:32 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 32.7887 (max)
12:55:32 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:55:32 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 11.9381 (min) 12.6161 (max)
12:55:32 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0768 (min) 33.5954 (max)
12:55:32 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000203365 (min) 0.000162256 (max)
12:55:32 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:55:32 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:55:32 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:55:32 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:55:32 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:55:32 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:55:32 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:32 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 118 elements to seafloor.
12:55:32 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:32 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:55:32 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:55:32 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:55:32 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 10
12:55:32 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 2 2 0 2 0 0 0 0 0]
12:55:32 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 0 0 3 0 3 3 3 3 3]
12:55:32 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 584. 0.]
[ 0. 0. 0. 0. 0.]
[251. 0. 0. 521. 0.]
[ 14. 0. 966. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:55:32 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:55:32 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:32 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:55:32 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:55:32 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.14223266608861632
12:55:32 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:32 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:55:32 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:55:32 DEBUG opendrift.models.oceandrift:618: 35 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 126 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 126 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 32 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 126 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 126 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 126 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 126 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 127 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 127 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 128 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 128 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 130 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 130 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 131 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 131 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 131 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 131 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 131 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 131 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 131 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 131 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 131 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 131 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 131 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 131 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 132 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 132 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 132 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 132 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 132 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 132 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 132 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 132 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 132 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 132 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 132 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 132 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 132 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 132 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 132 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 132 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 132 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 132 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 132 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 132 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 132 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 132 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 132 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 132 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 132 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 132 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 132 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 132 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 132 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 132 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 132 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 132 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 133 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 133 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 133 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 133 elements to seafloor.
12:55:32 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 7
12:55:32 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:55:32 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 7 elements
12:55:32 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:32 INFO opendrift.models.chemicaldrift:1861: partitioning: [130, 0, 244, 126, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:55:32 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:55:32 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:55:32 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 126 elements to seafloor.
12:55:32 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:32 INFO opendrift.models.basemodel:2038: 2024-11-03 07:54:02.760182 - step 87 of 96 - 500 active elements (0 deactivated)
12:55:32 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:32 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:32 DEBUG opendrift.models.basemodel:2057: 57.551940261363 <- latitude -> 57.72652806057434
12:55:32 DEBUG opendrift.models.basemodel:2062: 10.507927244297782 <- longitude -> 10.942388146548195
12:55:32 DEBUG opendrift.models.basemodel:2067: -31.314517974853516 <- z -> 0.0
12:55:32 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:32 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:32 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:55:32 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:32 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:55:32 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:32 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:32 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:55:32 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-03 07:00:00 (before)
2024-11-03 08:00:00 (after)
12:55:32 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-03 07:00:00) in space (linearNDFast)
12:55:32 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:32 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-03 08:00:00) in space (linearNDFast)
12:55:32 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:32 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-03 07:00:00, weight 0.10) and
after (2024-11-03 08:00:00, weight 0.90) in time
12:55:32 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:55:32 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.05762287113345 degrees.
12:55:32 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.05762287113345 degrees.
12:55:32 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:32 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:32 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:32 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:32 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:32 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:32 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:32 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:55:32 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:32 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:32 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:32 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:32 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:32 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:32 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:32 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:32 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:32 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:55:32 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:32 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:32 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:32 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:32 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:32 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:32 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:55:32 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:55:32 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:55:32 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:55:32 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:32 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0699763 (min) 0.252946 (max)
12:55:32 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.138915 (min) 0.158669 (max)
12:55:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.220096 (min) -0.162629 (max)
12:55:32 DEBUG opendrift.models.basemodel.environment:905: x_wind: 11.0584 (min) 14.1655 (max)
12:55:32 DEBUG opendrift.models.basemodel.environment:905: y_wind: 1.12971 (min) 2.30488 (max)
12:55:32 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:32 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 33.0719 (max)
12:55:32 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:55:32 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 11.9375 (min) 12.6161 (max)
12:55:32 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0792 (min) 33.5553 (max)
12:55:32 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000175906 (min) 0.000157532 (max)
12:55:32 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:55:32 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:55:32 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:55:32 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:55:32 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:55:32 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:55:32 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:32 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 126 elements to seafloor.
12:55:32 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:32 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:55:32 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:55:32 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:55:32 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 6
12:55:32 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 2 0 0 2]
12:55:32 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 0 3 3 0]
12:55:32 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 588. 0.]
[ 0. 0. 0. 0. 0.]
[253. 0. 0. 529. 0.]
[ 14. 0. 973. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:55:32 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:55:32 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:32 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:55:32 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:55:32 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.1483797153971406
12:55:32 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:32 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:55:32 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:55:32 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 130 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 130 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 130 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 130 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 130 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 130 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 130 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 130 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 130 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 130 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 130 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 130 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 130 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 130 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 130 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 130 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 130 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 130 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 130 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 130 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 130 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 130 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 130 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 130 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 130 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 130 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 130 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 130 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 130 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 130 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 130 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 130 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 131 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 131 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 131 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 131 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 131 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 131 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 132 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 132 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 133 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 133 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 134 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 134 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 134 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 134 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 134 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 134 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 134 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 134 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 135 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 135 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 135 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 135 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 135 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 135 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 138 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 138 elements to seafloor.
12:55:32 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
12:55:32 DEBUG opendrift.models.oceandrift:636: 138 elements reached seafloor, interacting with bottom
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 138 elements to seafloor.
12:55:32 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 6
12:55:32 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:55:32 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 6 elements
12:55:32 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:32 INFO opendrift.models.chemicaldrift:1861: partitioning: [128, 0, 240, 132, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:55:32 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:55:32 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:55:32 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:55:32 DEBUG opendrift.models.basemodel:755: Lifting 132 elements to seafloor.
12:55:32 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:32 INFO opendrift.models.basemodel:2038: 2024-11-03 08:24:02.760182 - step 88 of 96 - 500 active elements (0 deactivated)
12:55:32 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:32 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:32 DEBUG opendrift.models.basemodel:2057: 57.551940261363 <- latitude -> 57.72619077458436
12:55:32 DEBUG opendrift.models.basemodel:2062: 10.507927244297782 <- longitude -> 10.945226231522454
12:55:32 DEBUG opendrift.models.basemodel:2067: -31.314517974853516 <- z -> 0.0
12:55:32 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:32 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:32 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:55:32 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:32 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:55:32 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:32 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:32 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:55:32 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-03 08:00:00 (before)
2024-11-03 09:00:00 (after)
12:55:34 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
12:55:34 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:55:34 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:55:34 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:55:34 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:55:34 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'upward_sea_water_velocity', 'y_sea_water_velocity', 'sea_water_temperature', 'sea_water_salinity']
12:55:34 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 57x41x7) for time after (2024-11-03 09:00:00)
12:55:34 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-03 08:00:00) in space (linearNDFast)
12:55:34 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:34 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
/root/project/opendrift/readers/interpolation/interpolators.py:17: RuntimeWarning: overflow encountered in cast
data[mask] = np.finfo(np.float64).min
12:55:34 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
12:55:34 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
12:55:34 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
12:55:34 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
12:55:34 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
12:55:34 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
12:55:34 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-03 09:00:00) in space (linearNDFast)
12:55:34 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:34 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
12:55:34 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
12:55:34 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
12:55:34 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
12:55:34 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
12:55:34 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
12:55:34 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
12:55:34 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-03 08:00:00, weight 0.60) and
after (2024-11-03 09:00:00, weight 0.40) in time
12:55:34 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:55:34 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.05478478127861 degrees.
12:55:34 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.05478478127861 degrees.
12:55:34 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:34 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:34 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:34 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:34 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:34 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:34 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:34 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:55:34 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:34 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:34 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:34 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:34 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:34 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:34 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:34 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:34 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:34 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:55:34 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:34 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:34 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:34 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:34 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:34 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:34 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:55:34 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:55:34 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:55:34 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:55:34 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:34 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0798418 (min) 0.267901 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.139626 (min) 0.154191 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.249591 (min) -0.188803 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:905: x_wind: 11.2819 (min) 14.3319 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:905: y_wind: 1.13954 (min) 2.20242 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 33.0123 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 11.9374 (min) 12.6141 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0832 (min) 33.5318 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000141169 (min) 0.000168621 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:34 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 132 elements to seafloor.
12:55:34 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:34 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:55:34 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:55:34 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:55:34 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 9
12:55:34 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 0 0 0 2 0 2 2 2]
12:55:34 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 3 3 3 0 3 0 0 0]
12:55:34 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 592. 0.]
[ 0. 0. 0. 0. 0.]
[258. 0. 0. 537. 0.]
[ 14. 0. 979. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:55:34 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:55:34 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:34 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:55:34 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:55:34 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.15168754240502774
12:55:34 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:34 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:55:34 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:55:34 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 138 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 138 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 32 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 138 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 138 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 138 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 138 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 138 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 138 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 139 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 139 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 139 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 139 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 140 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 140 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 142 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 142 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 142 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 142 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 142 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 142 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 142 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 142 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 142 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 142 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 144 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 144 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 144 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 144 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 144 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 144 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 144 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 144 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 144 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 144 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 144 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 144 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 33 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 144 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 144 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 144 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 144 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 144 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 144 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 32 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 145 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 145 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 145 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 145 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 145 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 145 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 145 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 145 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 145 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 145 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 145 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 145 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 145 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 145 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 145 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 145 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 146 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 146 elements to seafloor.
12:55:34 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 3
12:55:34 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:55:34 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 3 elements
12:55:34 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:34 INFO opendrift.models.chemicaldrift:1861: partitioning: [129, 0, 228, 143, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:55:34 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:55:34 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:55:34 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 143 elements to seafloor.
12:55:34 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:34 INFO opendrift.models.basemodel:2038: 2024-11-03 08:54:02.760182 - step 89 of 96 - 500 active elements (0 deactivated)
12:55:34 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:34 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:34 DEBUG opendrift.models.basemodel:2057: 57.551940261363 <- latitude -> 57.72573373658247
12:55:34 DEBUG opendrift.models.basemodel:2062: 10.507927244297782 <- longitude -> 10.952155138191362
12:55:34 DEBUG opendrift.models.basemodel:2067: -31.314517974853516 <- z -> 0.0
12:55:34 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:34 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:34 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:55:34 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:34 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:55:34 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:34 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:34 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:55:34 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-03 08:00:00 (before)
2024-11-03 09:00:00 (after)
12:55:34 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-03 08:00:00) in space (linearNDFast)
12:55:34 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:34 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-03 09:00:00) in space (linearNDFast)
12:55:34 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:34 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-03 08:00:00, weight 0.10) and
after (2024-11-03 09:00:00, weight 0.90) in time
12:55:34 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:55:34 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.04785587778002 degrees.
12:55:34 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.04785587778002 degrees.
12:55:34 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:34 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:34 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:34 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:34 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:34 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:34 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:34 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:55:34 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:34 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:34 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:34 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:34 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:34 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:34 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:34 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:34 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:34 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:55:34 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:34 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:34 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:34 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:34 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:34 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:34 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:55:34 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:55:34 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:55:34 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:55:34 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:34 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0854327 (min) 0.239819 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.133814 (min) 0.148828 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.275612 (min) -0.214108 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:905: x_wind: 11.531 (min) 14.467 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:905: y_wind: 1.07032 (min) 2.02609 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 33.1831 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 11.9374 (min) 12.6116 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0875 (min) 33.563 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000181145 (min) 0.000166231 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:34 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 143 elements to seafloor.
12:55:34 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:34 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:55:34 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:55:34 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:55:34 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 9
12:55:34 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 0 2 0 0 0 2 2 2]
12:55:34 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 3 0 3 3 3 0 0 0]
12:55:34 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 596. 0.]
[ 0. 0. 0. 0. 0.]
[263. 0. 0. 547. 0.]
[ 14. 0. 982. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:55:34 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:55:34 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:34 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:55:34 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:55:34 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.15396835927715982
12:55:34 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:34 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:55:34 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:55:34 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 147 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 147 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 147 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 147 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 147 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 147 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 147 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 147 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 147 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 147 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 147 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 147 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 147 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 147 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 147 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 147 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 147 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 147 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 147 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 147 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 148 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 148 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 148 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 148 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 148 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 148 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 148 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 148 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 148 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 148 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 149 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 149 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 149 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 149 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 149 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 149 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 150 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 150 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 150 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 150 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 151 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 151 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 151 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 151 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 151 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 151 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 153 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 153 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 153 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 153 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 153 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 153 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 154 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 154 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 154 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 154 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 155 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 155 elements to seafloor.
12:55:34 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:55:34 DEBUG opendrift.models.oceandrift:636: 155 elements reached seafloor, interacting with bottom
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 155 elements to seafloor.
12:55:34 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 4
12:55:34 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:55:34 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 4 elements
12:55:34 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:34 INFO opendrift.models.chemicaldrift:1861: partitioning: [130, 0, 219, 151, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:55:34 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:55:34 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:55:34 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:55:34 DEBUG opendrift.models.basemodel:755: Lifting 151 elements to seafloor.
12:55:34 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:34 INFO opendrift.models.basemodel:2038: 2024-11-03 09:24:02.760182 - step 90 of 96 - 500 active elements (0 deactivated)
12:55:34 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:34 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:34 DEBUG opendrift.models.basemodel:2057: 57.551940261363 <- latitude -> 57.725106560786216
12:55:34 DEBUG opendrift.models.basemodel:2062: 10.50792724429778 <- longitude -> 10.954733007104418
12:55:34 DEBUG opendrift.models.basemodel:2067: -31.314517974853516 <- z -> 0.0
12:55:34 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:34 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:34 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:55:34 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:34 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:55:34 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:34 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:34 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:55:34 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-03 09:00:00 (before)
2024-11-03 10:00:00 (after)
12:55:35 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
12:55:35 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:55:35 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:55:35 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:55:35 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:55:35 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'upward_sea_water_velocity', 'y_sea_water_velocity', 'sea_water_temperature', 'sea_water_salinity']
12:55:35 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 57x41x7) for time after (2024-11-03 10:00:00)
12:55:35 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-03 09:00:00) in space (linearNDFast)
12:55:35 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:35 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-03 10:00:00) in space (linearNDFast)
12:55:35 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:35 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-03 09:00:00, weight 0.60) and
after (2024-11-03 10:00:00, weight 0.40) in time
12:55:35 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:55:36 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.04527801308114 degrees.
12:55:36 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.04527801308114 degrees.
12:55:36 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:36 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:36 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:36 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:36 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:36 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:36 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:36 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:55:36 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:36 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:36 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:36 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:36 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:36 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:36 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:36 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:36 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:36 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:55:36 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:36 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:36 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:36 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:36 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:36 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:36 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:55:36 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:55:36 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:55:36 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:55:36 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:36 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0886618 (min) 0.265251 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.136998 (min) 0.142903 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.291321 (min) -0.231477 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:905: x_wind: 11.833 (min) 14.5232 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0.793958 (min) 1.57169 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 33.2345 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 11.9374 (min) 12.605 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0932 (min) 33.5533 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000178719 (min) 0.0001687 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:36 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 151 elements to seafloor.
12:55:36 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:36 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:55:36 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:55:36 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:55:36 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 5
12:55:36 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 2 2 2 0]
12:55:36 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 0 0 0 3]
12:55:36 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 598. 0.]
[ 0. 0. 0. 0. 0.]
[266. 0. 0. 555. 0.]
[ 14. 0. 986. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:55:36 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:55:36 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:36 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:55:36 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:55:36 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.15403351740388893
12:55:36 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:36 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:55:36 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:55:36 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 154 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 154 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 154 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 154 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 155 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 155 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 155 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 155 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 155 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 155 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 155 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 155 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 155 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 155 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 155 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 155 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 155 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 155 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 155 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 155 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 155 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 155 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 155 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 155 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 156 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 156 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 156 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 156 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 156 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 156 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 156 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 156 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 156 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 156 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 156 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 156 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 156 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 156 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 156 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 156 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 157 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 157 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 157 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 157 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 157 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 157 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 157 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 157 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 157 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 157 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 158 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 158 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 158 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 158 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 158 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 158 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 158 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 158 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 158 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 158 elements to seafloor.
12:55:36 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 0
12:55:36 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:55:36 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:55:36 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:36 INFO opendrift.models.chemicaldrift:1861: partitioning: [131, 0, 211, 158, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:55:36 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:55:36 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:55:36 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 158 elements to seafloor.
12:55:36 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:36 INFO opendrift.models.basemodel:2038: 2024-11-03 09:54:02.760182 - step 91 of 96 - 500 active elements (0 deactivated)
12:55:36 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:36 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:36 DEBUG opendrift.models.basemodel:2057: 57.551940261363 <- latitude -> 57.724969401966916
12:55:36 DEBUG opendrift.models.basemodel:2062: 10.50792724429778 <- longitude -> 10.962731452986517
12:55:36 DEBUG opendrift.models.basemodel:2067: -31.314517974853516 <- z -> 0.0
12:55:36 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:36 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:36 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:55:36 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:36 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:55:36 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:36 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:36 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:55:36 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-03 09:00:00 (before)
2024-11-03 10:00:00 (after)
12:55:36 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-03 09:00:00) in space (linearNDFast)
12:55:36 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:36 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-03 10:00:00) in space (linearNDFast)
12:55:36 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:36 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-03 09:00:00, weight 0.10) and
after (2024-11-03 10:00:00, weight 0.90) in time
12:55:36 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:55:36 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.03727956350933 degrees.
12:55:36 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.03727956350933 degrees.
12:55:36 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:36 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:36 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:36 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:36 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:36 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:36 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:36 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:55:36 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:36 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:36 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:36 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:36 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:36 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:36 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:36 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:36 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:36 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:55:36 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:36 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:36 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:36 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:36 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:36 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:36 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:55:36 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:55:36 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:55:36 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:55:36 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:36 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0888919 (min) 0.282152 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.14388 (min) 0.136447 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.304476 (min) -0.246801 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:905: x_wind: 12.1482 (min) 14.5615 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0.46631 (min) 1.05807 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 33.3509 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 11.9374 (min) 12.5975 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.0991 (min) 33.5296 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000170026 (min) 0.000177854 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:36 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 158 elements to seafloor.
12:55:36 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:36 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:55:36 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:55:36 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:55:36 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 9
12:55:36 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 0 0 0 0 0 2 2 3]
12:55:36 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 3 3 3 3 3 0 0 0]
12:55:36 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 603. 0.]
[ 0. 0. 0. 0. 0.]
[269. 0. 0. 560. 0.]
[ 15. 0. 986. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:55:36 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:55:36 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:36 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:55:36 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:55:36 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.15389621084424718
12:55:36 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:36 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:55:36 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:55:36 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 163 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 163 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 163 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 163 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 163 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 163 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 163 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 163 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 163 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 163 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 163 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 163 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 163 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 163 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 163 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 163 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 163 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 163 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 163 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 163 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 163 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 163 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 163 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 163 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 163 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 163 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 163 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 163 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 163 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 163 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 163 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 163 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 163 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 163 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 163 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 163 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 163 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 163 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 163 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 163 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 164 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 164 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 164 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 164 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 164 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 164 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 164 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 164 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 164 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 164 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 165 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 165 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 165 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 165 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 165 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 165 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 165 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 165 elements to seafloor.
12:55:36 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:36 DEBUG opendrift.models.oceandrift:636: 165 elements reached seafloor, interacting with bottom
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 165 elements to seafloor.
12:55:36 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 0
12:55:36 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:55:36 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:55:36 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:36 INFO opendrift.models.chemicaldrift:1861: partitioning: [130, 0, 205, 165, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:55:36 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:55:36 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:55:36 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:55:36 DEBUG opendrift.models.basemodel:755: Lifting 165 elements to seafloor.
12:55:36 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:36 INFO opendrift.models.basemodel:2038: 2024-11-03 10:24:02.760182 - step 92 of 96 - 500 active elements (0 deactivated)
12:55:36 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:36 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:36 DEBUG opendrift.models.basemodel:2057: 57.551940261363 <- latitude -> 57.72511550382602
12:55:36 DEBUG opendrift.models.basemodel:2062: 10.50792724429778 <- longitude -> 10.96795212032329
12:55:36 DEBUG opendrift.models.basemodel:2067: -31.314517974853516 <- z -> 0.0
12:55:36 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:36 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:36 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:55:36 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:36 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:55:36 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:36 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:36 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:55:36 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-03 10:00:00 (before)
2024-11-03 11:00:00 (after)
12:55:37 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
12:55:37 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:55:37 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:55:37 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:55:37 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:55:37 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'upward_sea_water_velocity', 'y_sea_water_velocity', 'sea_water_temperature', 'sea_water_salinity']
12:55:37 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 57x41x7) for time after (2024-11-03 11:00:00)
12:55:37 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-03 10:00:00) in space (linearNDFast)
12:55:37 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:37 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-03 11:00:00) in space (linearNDFast)
12:55:37 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:37 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-03 10:00:00, weight 0.60) and
after (2024-11-03 11:00:00, weight 0.40) in time
12:55:37 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:55:37 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.03205888682951 degrees.
12:55:37 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.03205888682951 degrees.
12:55:37 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:37 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:37 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:37 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:37 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:37 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:37 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:37 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:55:37 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:37 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:37 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:37 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:37 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:37 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:37 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:37 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:37 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:37 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:55:37 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:37 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:37 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:37 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:37 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:37 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:37 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:55:37 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:55:37 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:55:37 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:55:37 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:37 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0906679 (min) 0.272348 (max)
12:55:37 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.126461 (min) 0.133954 (max)
12:55:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.308955 (min) -0.246911 (max)
12:55:37 DEBUG opendrift.models.basemodel.environment:905: x_wind: 12.3964 (min) 14.9216 (max)
12:55:37 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0.221417 (min) 0.852213 (max)
12:55:37 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:37 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 33.5741 (max)
12:55:37 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:55:37 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 11.9379 (min) 12.5898 (max)
12:55:37 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.1067 (min) 33.5156 (max)
12:55:37 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000159539 (min) 0.00018042 (max)
12:55:37 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:55:37 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:55:37 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:55:37 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:55:37 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:55:37 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:55:37 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:37 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 165 elements to seafloor.
12:55:37 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:37 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:55:37 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:55:37 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:55:37 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 2
12:55:37 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0]
12:55:37 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3]
12:55:37 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 605. 0.]
[ 0. 0. 0. 0. 0.]
[269. 0. 0. 563. 0.]
[ 15. 0. 986. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:55:37 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:55:37 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:37 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:55:37 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:55:37 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.1612294374420514
12:55:37 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:37 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:55:37 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:55:37 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 167 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 167 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 167 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 167 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 167 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 167 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 169 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 169 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 169 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 169 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 169 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 169 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 169 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 169 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 170 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 170 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 170 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 170 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 170 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 170 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 170 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 170 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 170 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 170 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 170 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 170 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 170 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 170 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 32 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 170 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 170 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 170 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 170 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 170 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 170 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 171 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 171 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 171 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 171 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 171 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 171 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 171 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 171 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 171 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 171 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 172 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 172 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 172 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 172 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 172 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 172 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 172 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 172 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 173 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 173 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 173 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 173 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 173 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 173 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 174 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 174 elements to seafloor.
12:55:37 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 1
12:55:37 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:55:37 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 1 elements
12:55:37 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:37 INFO opendrift.models.chemicaldrift:1861: partitioning: [128, 0, 199, 173, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:55:37 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:55:37 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:55:37 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 173 elements to seafloor.
12:55:37 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:37 INFO opendrift.models.basemodel:2038: 2024-11-03 10:54:02.760182 - step 93 of 96 - 500 active elements (0 deactivated)
12:55:37 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:37 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:37 DEBUG opendrift.models.basemodel:2057: 57.551940261363 <- latitude -> 57.72485390498136
12:55:37 DEBUG opendrift.models.basemodel:2062: 10.50792724429778 <- longitude -> 10.970412014589373
12:55:37 DEBUG opendrift.models.basemodel:2067: -31.70957465568901 <- z -> -0.019354522564795085
12:55:37 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:37 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:37 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:55:37 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:37 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:55:37 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:37 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:37 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:55:37 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-03 10:00:00 (before)
2024-11-03 11:00:00 (after)
12:55:37 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-03 10:00:00) in space (linearNDFast)
12:55:37 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:37 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-03 11:00:00) in space (linearNDFast)
12:55:37 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:37 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-03 10:00:00, weight 0.10) and
after (2024-11-03 11:00:00, weight 0.90) in time
12:55:37 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:55:37 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.02959899860305 degrees.
12:55:37 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.02959899860305 degrees.
12:55:37 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:37 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:37 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:37 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:37 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:37 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:37 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:37 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:55:37 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:37 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:37 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:37 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:37 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:37 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:37 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:37 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:37 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:37 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:55:37 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:37 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:37 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:37 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:37 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:37 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:37 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:55:37 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:55:37 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:55:37 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:55:37 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:37 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0939497 (min) 0.291283 (max)
12:55:37 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.134348 (min) 0.12861 (max)
12:55:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.311285 (min) -0.24421 (max)
12:55:37 DEBUG opendrift.models.basemodel.environment:905: x_wind: 12.6281 (min) 15.3735 (max)
12:55:37 DEBUG opendrift.models.basemodel.environment:905: y_wind: -0.00298511 (min) 0.715098 (max)
12:55:37 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:37 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 33.6723 (max)
12:55:37 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:55:37 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 11.9386 (min) 12.5822 (max)
12:55:37 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.1148 (min) 33.5203 (max)
12:55:37 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000138342 (min) 0.000182459 (max)
12:55:37 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:55:37 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:55:37 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:55:37 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:55:37 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:55:37 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:55:37 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:37 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 173 elements to seafloor.
12:55:37 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:37 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:55:37 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:55:37 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:55:37 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 3
12:55:37 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 0 2]
12:55:37 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 3 0]
12:55:37 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 606. 0.]
[ 0. 0. 0. 0. 0.]
[271. 0. 0. 570. 0.]
[ 15. 0. 987. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:55:37 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:55:37 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:37 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:55:37 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:55:37 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.1709465985195681
12:55:37 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:37 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:55:37 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:55:37 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 174 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 174 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 174 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 174 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 174 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 174 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 174 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 174 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 174 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 174 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 174 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 174 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 174 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 174 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 174 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 174 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 174 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 174 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 174 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 174 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 176 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 176 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 176 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 176 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 176 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 176 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 176 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 176 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 176 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 176 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 178 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 178 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 178 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 178 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 178 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 178 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 179 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 179 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 180 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 180 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 180 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 180 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 180 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 180 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 180 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 180 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 181 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 181 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 181 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 181 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 181 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 181 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 181 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 181 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 181 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 181 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 181 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 181 elements to seafloor.
12:55:37 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:37 DEBUG opendrift.models.oceandrift:636: 181 elements reached seafloor, interacting with bottom
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 181 elements to seafloor.
12:55:37 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 2
12:55:37 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:55:37 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 2 elements
12:55:37 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:37 INFO opendrift.models.chemicaldrift:1861: partitioning: [129, 0, 192, 179, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:55:37 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:55:37 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:55:37 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:55:37 DEBUG opendrift.models.basemodel:755: Lifting 179 elements to seafloor.
12:55:37 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:37 INFO opendrift.models.basemodel:2038: 2024-11-03 11:24:02.760182 - step 94 of 96 - 500 active elements (0 deactivated)
12:55:37 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:37 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:37 DEBUG opendrift.models.basemodel:2057: 57.551940261363 <- latitude -> 57.7243575620698
12:55:37 DEBUG opendrift.models.basemodel:2062: 10.507927244297782 <- longitude -> 10.972881483218789
12:55:37 DEBUG opendrift.models.basemodel:2067: -31.78515057568834 <- z -> 0.0
12:55:37 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:37 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:37 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:55:37 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:37 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:55:37 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:37 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:37 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:55:37 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-03 11:00:00 (before)
2024-11-03 12:00:00 (after)
12:55:39 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
12:55:39 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:55:39 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:55:39 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:55:39 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:55:39 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'upward_sea_water_velocity', 'y_sea_water_velocity', 'sea_water_temperature', 'sea_water_salinity']
12:55:39 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 58x41x7) for time after (2024-11-03 12:00:00)
12:55:39 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-03 11:00:00) in space (linearNDFast)
12:55:39 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:39 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-03 12:00:00) in space (linearNDFast)
12:55:39 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:39 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-03 11:00:00, weight 0.60) and
after (2024-11-03 12:00:00, weight 0.40) in time
12:55:39 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:55:39 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.02712953289162 degrees.
12:55:39 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.02712953289162 degrees.
12:55:39 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:39 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:39 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:39 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:39 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:39 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:39 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:39 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:55:39 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:39 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:39 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:39 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:39 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:39 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:39 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:39 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:39 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:39 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:55:39 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:39 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:39 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:39 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:39 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:39 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:39 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:55:39 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:55:39 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:55:39 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:55:39 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:39 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0981978 (min) 0.314967 (max)
12:55:39 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.147516 (min) 0.131944 (max)
12:55:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.290112 (min) -0.220981 (max)
12:55:39 DEBUG opendrift.models.basemodel.environment:905: x_wind: 12.6433 (min) 15.6267 (max)
12:55:39 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0.0985751 (min) 0.899369 (max)
12:55:39 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:39 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 33.8086 (max)
12:55:39 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:55:39 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 11.9427 (min) 12.5745 (max)
12:55:39 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.1246 (min) 33.5111 (max)
12:55:39 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000176581 (min) 0.000184626 (max)
12:55:39 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:55:39 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:55:39 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:55:39 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:55:39 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:55:39 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:55:39 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:39 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 179 elements to seafloor.
12:55:39 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:39 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:55:39 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:55:39 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:55:39 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 4
12:55:39 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 2 0]
12:55:39 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 0 2]
12:55:39 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 1. 608. 0.]
[ 0. 0. 0. 0. 0.]
[272. 0. 0. 577. 0.]
[ 15. 0. 989. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:55:39 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 1 elements
12:55:39 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:39 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:55:39 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:55:39 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.17670840428730997
12:55:39 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:39 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:55:39 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:55:39 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 181 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 181 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 181 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 181 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 182 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 182 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 182 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 182 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 182 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 182 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 182 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 182 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 182 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 182 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 182 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 182 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 182 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 182 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 182 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 182 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 182 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 182 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 182 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 182 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 183 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 183 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 183 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 183 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 183 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 183 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 183 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 183 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 183 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 183 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 183 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 183 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 184 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 184 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 184 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 184 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 184 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 184 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 184 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 184 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 184 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 184 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 184 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 184 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 184 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 184 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 184 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 184 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 184 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 184 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 185 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 185 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 185 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 185 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 185 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 185 elements to seafloor.
12:55:39 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 1
12:55:39 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:55:39 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 1 elements
12:55:39 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:39 INFO opendrift.models.chemicaldrift:1861: partitioning: [127, 0, 189, 184, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:55:39 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:55:39 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:55:39 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 184 elements to seafloor.
12:55:39 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:39 INFO opendrift.models.basemodel:2038: 2024-11-03 11:54:02.760182 - step 95 of 96 - 500 active elements (0 deactivated)
12:55:39 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:39 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:39 DEBUG opendrift.models.basemodel:2057: 57.551940261363 <- latitude -> 57.723198825600186
12:55:39 DEBUG opendrift.models.basemodel:2062: 10.507927244297782 <- longitude -> 10.978272690278903
12:55:39 DEBUG opendrift.models.basemodel:2067: -32.26204013828517 <- z -> 0.0
12:55:39 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:39 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:39 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:55:39 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:39 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:55:39 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:39 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:39 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:55:39 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-03 11:00:00 (before)
2024-11-03 12:00:00 (after)
12:55:39 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-03 11:00:00) in space (linearNDFast)
12:55:39 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:39 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-03 12:00:00) in space (linearNDFast)
12:55:39 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:39 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-03 11:00:00, weight 0.10) and
after (2024-11-03 12:00:00, weight 0.90) in time
12:55:39 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:55:39 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.021738306258314 degrees.
12:55:39 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.021738306258314 degrees.
12:55:39 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:39 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:39 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:39 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:39 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:39 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:39 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:39 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:55:39 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:39 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:39 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:39 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:39 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:39 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:39 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:39 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:39 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:39 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:55:39 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:39 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:39 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:39 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:39 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:39 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:39 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:55:39 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:55:39 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:55:39 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:55:39 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:39 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.106177 (min) 0.322141 (max)
12:55:39 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.160609 (min) 0.114573 (max)
12:55:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.26312 (min) -0.193257 (max)
12:55:39 DEBUG opendrift.models.basemodel.environment:905: x_wind: 12.605 (min) 15.8272 (max)
12:55:39 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0.280846 (min) 1.18548 (max)
12:55:39 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:39 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 33.9509 (max)
12:55:39 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:55:39 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 11.9477 (min) 12.5669 (max)
12:55:39 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.1347 (min) 33.4767 (max)
12:55:39 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000133455 (min) 0.000198547 (max)
12:55:39 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:55:39 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:55:39 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:55:39 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:55:39 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:55:39 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:55:39 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:39 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 184 elements to seafloor.
12:55:39 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:39 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:55:39 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:55:39 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:55:39 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 4
12:55:39 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 2 0 2]
12:55:39 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 0 3 0]
12:55:39 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 1. 610. 0.]
[ 0. 0. 0. 0. 0.]
[274. 0. 0. 581. 0.]
[ 15. 0. 990. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:55:39 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:55:39 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:39 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:55:39 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:55:39 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.1814765980488404
12:55:39 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:39 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:55:39 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:55:39 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 186 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 186 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 187 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 187 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 187 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 187 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 187 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 187 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 187 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 187 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 188 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 188 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 188 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 188 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 188 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 188 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 188 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 188 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 188 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 188 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 188 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 188 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 189 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 189 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 190 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 190 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 190 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 190 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 190 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 190 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 190 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 190 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 190 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 190 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 190 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 190 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 190 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 190 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 190 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 190 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 191 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 191 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 191 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 191 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 191 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 191 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 192 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 192 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 192 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 192 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 192 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 192 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 193 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 193 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 193 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 193 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 193 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 193 elements to seafloor.
12:55:39 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:55:39 DEBUG opendrift.models.oceandrift:636: 193 elements reached seafloor, interacting with bottom
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 193 elements to seafloor.
12:55:39 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 3
12:55:39 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:55:39 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 3 elements
12:55:39 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:39 INFO opendrift.models.chemicaldrift:1861: partitioning: [127, 0, 183, 190, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:55:39 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:55:39 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:55:39 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 500
12:55:39 DEBUG opendrift.models.basemodel:755: Lifting 190 elements to seafloor.
12:55:39 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:39 INFO opendrift.models.basemodel:2038: 2024-11-03 12:24:02.760182 - step 96 of 96 - 500 active elements (0 deactivated)
12:55:39 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:39 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:39 DEBUG opendrift.models.basemodel:2057: 57.551940261363 <- latitude -> 57.721713727146344
12:55:39 DEBUG opendrift.models.basemodel:2062: 10.507927244297782 <- longitude -> 10.98531359850546
12:55:39 DEBUG opendrift.models.basemodel:2067: -31.314517974853516 <- z -> -0.02878665458341434
12:55:39 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:39 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:39 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
12:55:39 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:39 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
12:55:39 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:39 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:39 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
12:55:39 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-03 12:00:00 (before)
2024-11-03 13:00:00 (after)
12:55:40 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
12:55:40 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:55:40 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:55:40 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:55:40 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:55:40 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'upward_sea_water_velocity', 'y_sea_water_velocity', 'sea_water_temperature', 'sea_water_salinity']
12:55:40 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 58x41x7) for time after (2024-11-03 13:00:00)
12:55:40 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-03 12:00:00) in space (linearNDFast)
12:55:40 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:40 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-03 13:00:00) in space (linearNDFast)
12:55:40 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:40 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-03 12:00:00, weight 0.60) and
after (2024-11-03 13:00:00, weight 0.40) in time
12:55:40 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
12:55:40 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.01469741362941 degrees.
12:55:40 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.492083840123236 and -59.01469741362941 degrees.
12:55:40 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:40 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:40 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:40 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:40 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:40 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:40 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:40 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
12:55:40 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:40 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:40 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:40 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:40 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:40 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:40 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:40 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:40 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:40 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:55:40 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:40 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:40 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:40 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:40 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:40 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:40 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
12:55:40 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
12:55:40 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
12:55:40 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
12:55:40 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:40 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.131574 (min) 0.310277 (max)
12:55:40 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.187899 (min) 0.122615 (max)
12:55:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.217161 (min) -0.1494 (max)
12:55:40 DEBUG opendrift.models.basemodel.environment:905: x_wind: 12.6266 (min) 15.4266 (max)
12:55:40 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0.39485 (min) 1.07265 (max)
12:55:40 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:40 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.42652 (min) 34.1118 (max)
12:55:40 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
12:55:40 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 11.9487 (min) 12.5555 (max)
12:55:40 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 32.146 (min) 33.4809 (max)
12:55:40 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000160499 (min) 0.000212834 (max)
12:55:40 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
12:55:40 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
12:55:40 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
12:55:40 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
12:55:40 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
12:55:40 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
12:55:40 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:40 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:55:40 DEBUG opendrift.models.basemodel:755: Lifting 190 elements to seafloor.
12:55:40 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:40 DEBUG opendrift.models.basemodel:2109: Calling ChemicalDrift.update()
12:55:40 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
12:55:40 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
12:55:40 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 8
12:55:40 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 2 0 0 2 2 2]
12:55:40 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 0 3 3 0 0 0]
12:55:40 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 1. 614. 0.]
[ 0. 0. 0. 0. 0.]
[278. 0. 0. 588. 0.]
[ 15. 0. 993. 0. 0.]
[ 0. 0. 0. 0. 0.]]
12:55:40 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
12:55:40 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:40 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
12:55:40 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
12:55:40 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.17243609860989148
12:55:40 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:40 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
12:55:40 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
12:55:40 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:40 DEBUG opendrift.models.oceandrift:636: 194 elements reached seafloor, interacting with bottom
12:55:40 DEBUG opendrift.models.basemodel:755: Lifting 194 elements to seafloor.
12:55:40 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:40 DEBUG opendrift.models.oceandrift:636: 194 elements reached seafloor, interacting with bottom
12:55:40 DEBUG opendrift.models.basemodel:755: Lifting 194 elements to seafloor.
12:55:40 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
12:55:40 DEBUG opendrift.models.oceandrift:636: 194 elements reached seafloor, interacting with bottom
12:55:40 DEBUG opendrift.models.basemodel:755: Lifting 194 elements to seafloor.
12:55:40 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:55:40 DEBUG opendrift.models.oceandrift:636: 194 elements reached seafloor, interacting with bottom
12:55:40 DEBUG opendrift.models.basemodel:755: Lifting 194 elements to seafloor.
12:55:40 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:40 DEBUG opendrift.models.oceandrift:636: 194 elements reached seafloor, interacting with bottom
12:55:40 DEBUG opendrift.models.basemodel:755: Lifting 194 elements to seafloor.
12:55:40 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
12:55:40 DEBUG opendrift.models.oceandrift:636: 194 elements reached seafloor, interacting with bottom
12:55:40 DEBUG opendrift.models.basemodel:755: Lifting 194 elements to seafloor.
12:55:40 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:40 DEBUG opendrift.models.oceandrift:636: 194 elements reached seafloor, interacting with bottom
12:55:40 DEBUG opendrift.models.basemodel:755: Lifting 194 elements to seafloor.
12:55:40 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:40 DEBUG opendrift.models.oceandrift:636: 194 elements reached seafloor, interacting with bottom
12:55:40 DEBUG opendrift.models.basemodel:755: Lifting 194 elements to seafloor.
12:55:40 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:40 DEBUG opendrift.models.oceandrift:636: 194 elements reached seafloor, interacting with bottom
12:55:40 DEBUG opendrift.models.basemodel:755: Lifting 194 elements to seafloor.
12:55:40 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
12:55:40 DEBUG opendrift.models.oceandrift:636: 194 elements reached seafloor, interacting with bottom
12:55:40 DEBUG opendrift.models.basemodel:755: Lifting 194 elements to seafloor.
12:55:40 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:40 DEBUG opendrift.models.oceandrift:636: 194 elements reached seafloor, interacting with bottom
12:55:40 DEBUG opendrift.models.basemodel:755: Lifting 194 elements to seafloor.
12:55:40 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
12:55:40 DEBUG opendrift.models.oceandrift:636: 194 elements reached seafloor, interacting with bottom
12:55:40 DEBUG opendrift.models.basemodel:755: Lifting 194 elements to seafloor.
12:55:40 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
12:55:40 DEBUG opendrift.models.oceandrift:636: 194 elements reached seafloor, interacting with bottom
12:55:40 DEBUG opendrift.models.basemodel:755: Lifting 194 elements to seafloor.
12:55:40 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
12:55:40 DEBUG opendrift.models.oceandrift:636: 194 elements reached seafloor, interacting with bottom
12:55:40 DEBUG opendrift.models.basemodel:755: Lifting 194 elements to seafloor.
12:55:40 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:55:40 DEBUG opendrift.models.oceandrift:636: 194 elements reached seafloor, interacting with bottom
12:55:40 DEBUG opendrift.models.basemodel:755: Lifting 194 elements to seafloor.
12:55:40 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:40 DEBUG opendrift.models.oceandrift:636: 194 elements reached seafloor, interacting with bottom
12:55:40 DEBUG opendrift.models.basemodel:755: Lifting 194 elements to seafloor.
12:55:40 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:40 DEBUG opendrift.models.oceandrift:636: 195 elements reached seafloor, interacting with bottom
12:55:40 DEBUG opendrift.models.basemodel:755: Lifting 195 elements to seafloor.
12:55:40 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:40 DEBUG opendrift.models.oceandrift:636: 195 elements reached seafloor, interacting with bottom
12:55:40 DEBUG opendrift.models.basemodel:755: Lifting 195 elements to seafloor.
12:55:40 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:40 DEBUG opendrift.models.oceandrift:636: 195 elements reached seafloor, interacting with bottom
12:55:40 DEBUG opendrift.models.basemodel:755: Lifting 195 elements to seafloor.
12:55:40 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:40 DEBUG opendrift.models.oceandrift:636: 195 elements reached seafloor, interacting with bottom
12:55:40 DEBUG opendrift.models.basemodel:755: Lifting 195 elements to seafloor.
12:55:40 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:40 DEBUG opendrift.models.oceandrift:636: 195 elements reached seafloor, interacting with bottom
12:55:40 DEBUG opendrift.models.basemodel:755: Lifting 195 elements to seafloor.
12:55:40 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:40 DEBUG opendrift.models.oceandrift:636: 195 elements reached seafloor, interacting with bottom
12:55:40 DEBUG opendrift.models.basemodel:755: Lifting 195 elements to seafloor.
12:55:40 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:40 DEBUG opendrift.models.oceandrift:636: 195 elements reached seafloor, interacting with bottom
12:55:40 DEBUG opendrift.models.basemodel:755: Lifting 195 elements to seafloor.
12:55:40 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
12:55:40 DEBUG opendrift.models.oceandrift:636: 195 elements reached seafloor, interacting with bottom
12:55:40 DEBUG opendrift.models.basemodel:755: Lifting 195 elements to seafloor.
12:55:40 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
12:55:40 DEBUG opendrift.models.oceandrift:636: 196 elements reached seafloor, interacting with bottom
12:55:40 DEBUG opendrift.models.basemodel:755: Lifting 196 elements to seafloor.
12:55:40 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
12:55:40 DEBUG opendrift.models.oceandrift:636: 196 elements reached seafloor, interacting with bottom
12:55:40 DEBUG opendrift.models.basemodel:755: Lifting 196 elements to seafloor.
12:55:40 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
12:55:40 DEBUG opendrift.models.oceandrift:636: 196 elements reached seafloor, interacting with bottom
12:55:40 DEBUG opendrift.models.basemodel:755: Lifting 196 elements to seafloor.
12:55:40 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
12:55:40 DEBUG opendrift.models.oceandrift:636: 197 elements reached seafloor, interacting with bottom
12:55:40 DEBUG opendrift.models.basemodel:755: Lifting 197 elements to seafloor.
12:55:40 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
12:55:40 DEBUG opendrift.models.oceandrift:636: 197 elements reached seafloor, interacting with bottom
12:55:40 DEBUG opendrift.models.basemodel:755: Lifting 197 elements to seafloor.
12:55:40 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
12:55:40 DEBUG opendrift.models.oceandrift:636: 197 elements reached seafloor, interacting with bottom
12:55:40 DEBUG opendrift.models.basemodel:755: Lifting 197 elements to seafloor.
12:55:40 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 4
12:55:40 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
12:55:40 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 4 elements
12:55:40 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
12:55:40 INFO opendrift.models.chemicaldrift:1861: partitioning: [127, 0, 180, 193, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
12:55:40 DEBUG opendrift.models.basemodel:1661: Horizontal diffusivity is 0, no random walk.
12:55:40 DEBUG opendrift.models.basemodel:2124: 500 active elements (0 deactivated)
12:55:40 DEBUG opendrift.models.basemodel:2153: Cleaning up
12:55:40 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:40 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:40 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:40 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:40 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:40 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
12:55:40 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
12:55:40 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:40 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:40 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:40 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:40 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:40 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:40 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:40 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:40 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:40 DEBUG opendrift.models.basemodel:711: No elements hit coastline.
12:55:40 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:40 DEBUG opendrift.models.basemodel:95: Changed mode from Mode.Run to Mode.Result
Print and plot results
print('Final speciation:')
for isp,sp in enumerate(o.name_species):
print ('{:32}: {:>6}'.format(sp,sum(o.elements.specie==isp)))
print('Number of transformations:')
for isp in range(o.nspecies):
print('{}'.format(['{:>9}'.format(np.int32(item)) for item in o.ntransformations[isp,:]]) )
mass = o.get_property('mass')
mass_d = o.get_property('mass_degraded')
mass_v = o.get_property('mass_volatilized')
m_pre = sum(mass[0][-1])
m_deg = sum(mass_d[0][-1])
m_vol = sum(mass_v[0][-1])
m_tot = m_pre + m_deg + m_vol
print('Mass budget for target chemical:')
print('mass preserved : {:.3f}'.format(m_pre * 1e-6),' g {:.3f}'.format(m_pre/m_tot*100),'%')
print('mass degraded : {:.3f}'.format(m_deg * 1e-6),' g {:.3f}'.format(m_deg/m_tot*100),'%')
print('mass volatilized : {:.3f}'.format(m_vol * 1e-6),' g {:.3f}'.format(m_vol/m_tot*100),'%')
legend=['dissolved', '', 'SPM', 'sediment', '']
o.animation_profile(color='specie',
markersize='mass',
markersize_scaling=30,
alpha=.5,
vmin=0,vmax=o.nspecies-1,
legend = legend,
legend_loc = 3,
fps = 10
)
Final speciation:
LMM : 127
Humic colloid : 0
Particle reversible : 180
Sediment reversible : 193
Sediment slowly reversible : 0
Number of transformations:
[' 0', ' 0', ' 1', ' 614', ' 0']
[' 0', ' 0', ' 0', ' 0', ' 0']
[' 278', ' 0', ' 0', ' 591', ' 0']
[' 15', ' 0', ' 997', ' 0', ' 0']
[' 0', ' 0', ' 0', ' 0', ' 0']
Mass budget for target chemical:
mass preserved : 0.442 g 44.208 %
mass degraded : 0.553 g 55.274 %
mass volatilized : 0.005 g 0.518 %
12:55:40 DEBUG opendrift.models.basemodel:3044: Saving animation..
12:55:41 INFO opendrift.models.basemodel:4613: Saving animation to /root/project/docs/source/gallery/animations/example_chemicaldrift_0.gif...
12:55:54 DEBUG opendrift.models.basemodel:4651: MPLBACKEND = agg
12:55:54 DEBUG opendrift.models.basemodel:4652: DISPLAY = None
12:55:54 DEBUG opendrift.models.basemodel:4653: Time to save animation: 0:00:13.290432
12:55:54 INFO opendrift.models.basemodel:3251: Time to make animation: 0:00:13.527874
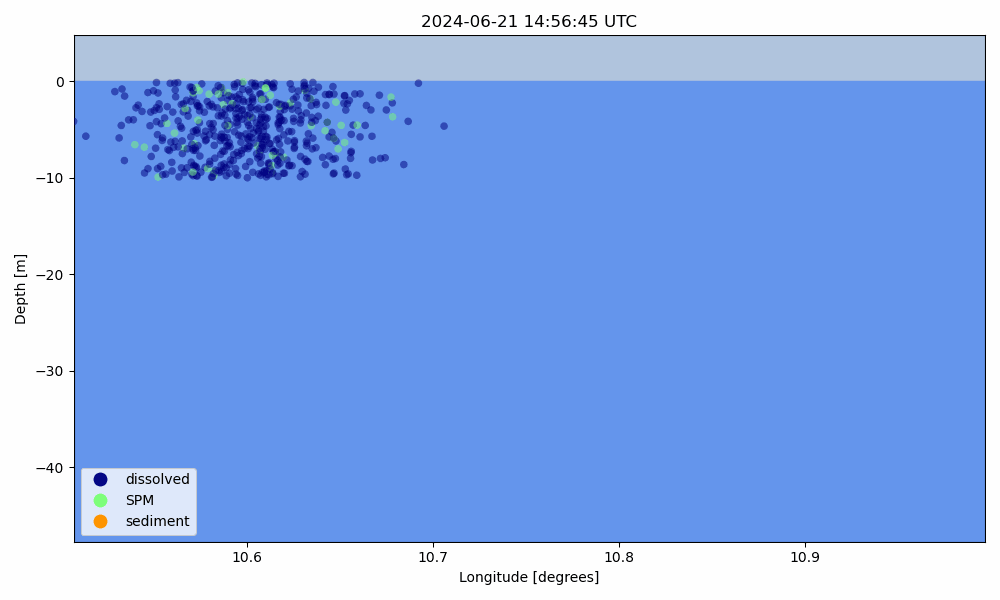
o.animation(color='specie',
markersize='mass',
markersize_scaling=100,
alpha=.5,
vmin=0,vmax=o.nspecies-1,
colorbar=False,
fast = True,
legend = legend,
legend_loc = 3,
fps=10
)
12:55:54 DEBUG opendrift.models.basemodel:2365: Setting up map: corners=None, fast=True, lscale=None
12:55:54 WARNING opendrift.models.basemodel:2411: Plotting fast. This will make your plots less accurate.
12:55:56 DEBUG opendrift.models.basemodel:3044: Saving animation..
12:55:56 INFO opendrift.models.basemodel:4613: Saving animation to /root/project/docs/source/gallery/animations/example_chemicaldrift_1.gif...
12:57:05 DEBUG opendrift.models.basemodel:4651: MPLBACKEND = agg
12:57:05 DEBUG opendrift.models.basemodel:4652: DISPLAY = None
12:57:05 DEBUG opendrift.models.basemodel:4653: Time to save animation: 0:01:08.741871
12:57:05 INFO opendrift.models.basemodel:3037: Time to make animation: 0:01:10.787355
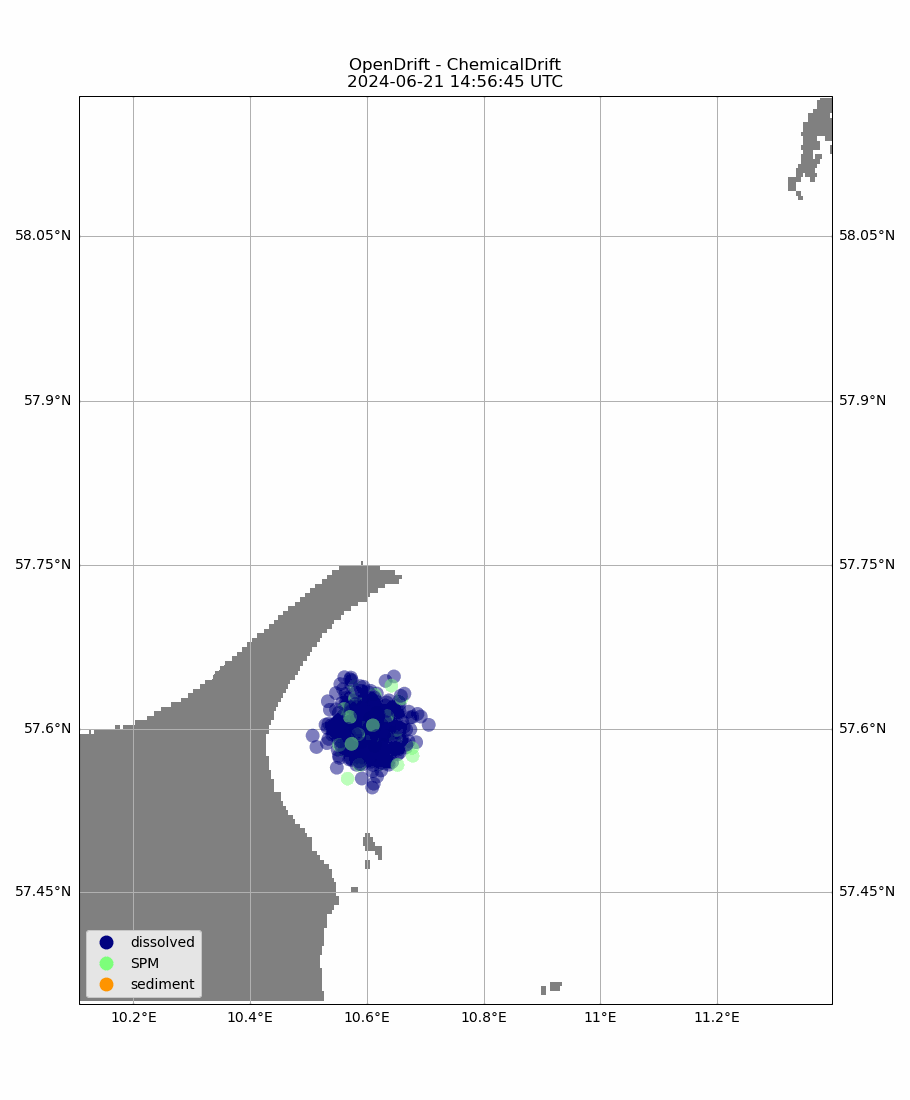
o.plot_mass(legend = legend,
time_unit = 'hours',
title = 'Chemical mass budget')
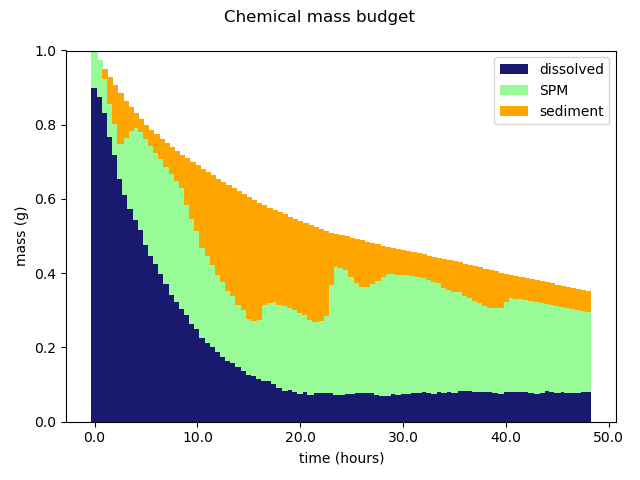
dissolved : 0.0779141875g (17.624420646478193%)
SPM : 0.21429625g (48.474448289188054%)
sediment : 0.14987040625g (33.901131064333754%)
/root/project/opendrift/models/chemicaldrift.py:3006: UserWarning: set_ticklabels() should only be used with a fixed number of ticks, i.e. after set_ticks() or using a FixedLocator.
ax.axes.get_xaxis().set_ticklabels(np.round(ax.axes.get_xticks() * time_conversion_factor))
Total running time of the script: (3 minutes 10.019 seconds)