Note
Go to the end to download the full example code.
Manual aggregate
from datetime import datetime, timedelta
import pandas as pd
import matplotlib.pyplot as plt
from opendrift.readers import reader_netCDF_CF_generic
from opendrift.readers import open_mfdataset_overlap
from opendrift.models.oceandrift import OceanDrift
#%
# Create manual aggregate from individual URLs, for NorKyst ocean model initialized at 00 hours every day
start_time = datetime.now().date()-timedelta(days=3)
end_time = datetime.now().date()-timedelta(days=1)
ds = open_mfdataset_overlap(
'https://thredds.met.no/thredds/dodsC/fou-hi/norkyst800m-1h/NorKyst-800m_ZDEPTHS_his.an.%Y%m%d%H.nc',
time_series=pd.date_range(start_time, end_time, freq='1D'))
#%
# Create reader from Xarray dataset
rm = reader_netCDF_CF_generic.Reader(ds, name='NorKyst manual aggregate')
print(rm)
om = OceanDrift()
om.add_reader(rm)
om.seed_elements(lon=4.5, lat=60.0, number=1000, radius=100, time=rm.start_time)
om.run(end_time=rm.end_time)
#%
# Second simulation using ready made aggregate from thredds
ot = OceanDrift()
ot.add_readers_from_list(['https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be'])
ot.seed_elements(lon=4.5, lat=60.0, number=1000, radius=100, time=rm.start_time)
ot.run(end_time=rm.end_time)
#%
# Simulation should be identical, but we see that manual aggregate is significantly slower than using thredds aggregate
om.animation(compare=ot,
legend=[f'NorKyst manual aggregate {om.timing["total time"]}',
f'NorKyst thredds aggregate {ot.timing["total time"]}'])
Opening individual URLs...
Concatenating...
===========================
Reader: NorKyst manual aggregate
Projection:
+proj=stere +lat_0=90 +lat_ts=60 +lon_0=70 +x_0=3192800 +y_0=1784000 +a=6378137 +b=6356752.3142 +units=m +no_defs +type=crs
Coverage: [degrees]
xmin: 0.000000 xmax: 2080800.000000 step: 800 numx: 2602
ymin: 0.000000 ymax: 720800.000000 step: 800 numy: 902
Corners (lon, lat):
( -1.58, 58.50) ( 23.71, 75.32)
( 9.19, 55.91) ( 38.06, 70.03)
Vertical levels [m]:
[ -0. -3. -10. -15. -25. -50. -75. -100. -150. -200.
-250. -300. -500. -1000. -2000. -3000.]
Available time range:
start: 2024-12-09 00:00:00 end: 2024-12-11 23:00:00 step: 1:00:00
72 times (0 missing)
Variables:
ocean_vertical_diffusivity
x_wind
y_wind
sea_floor_depth_below_sea_level
sea_water_salinity
sea_water_temperature
x_sea_water_velocity
eastward_sea_water_velocity
y_sea_water_velocity
northward_sea_water_velocity
upward_sea_water_velocity
sea_surface_height
latitude
longitude
wind_speed - derived from ['x_wind', 'y_wind']
sea_water_speed - derived from ['x_sea_water_velocity', 'y_sea_water_velocity']
===========================
20:11:15 DEBUG opendrift.config:168: Adding 18 config items from __init__
20:11:15 DEBUG opendrift.config:178: Overwriting config item readers:max_number_of_fails
20:11:15 DEBUG opendrift.config:168: Adding 5 config items from __init__
20:11:15 INFO opendrift.models.basemodel:515: OpenDriftSimulation initialised (version 1.12.0 / v1.12.0-26-g390e945)
20:11:15 DEBUG opendrift.config:168: Adding 15 config items from oceandrift
20:11:15 DEBUG opendrift.config:178: Overwriting config item seed:z
20:11:15 DEBUG opendrift.models.basemodel.environment:328: Added reader NorKyst manual aggregate
20:11:15 INFO opendrift.models.basemodel.environment:218: Adding a dynamical landmask with max. priority based on assumed maximum speed of 2.0 m/s. Adding a customised landmask may be faster...
20:11:15 DEBUG opendrift.readers.basereader:186: Variable mapping: ['sea_floor_depth_below_sea_level'] -> ['land_binary_mask'] is not activated
20:11:18 DEBUG opendrift.models.basemodel.environment:328: Added reader global_landmask
20:11:18 INFO opendrift.models.basemodel.environment:245: Fallback values will be used for the following variables which have no readers:
20:11:18 INFO opendrift.models.basemodel.environment:248: sea_surface_wave_significant_height: 0.000000
20:11:18 INFO opendrift.models.basemodel.environment:248: sea_surface_wave_stokes_drift_x_velocity: 0.000000
20:11:18 INFO opendrift.models.basemodel.environment:248: sea_surface_wave_stokes_drift_y_velocity: 0.000000
20:11:18 INFO opendrift.models.basemodel.environment:248: sea_surface_wave_period_at_variance_spectral_density_maximum: 0.000000
20:11:18 INFO opendrift.models.basemodel.environment:248: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0.000000
20:11:18 INFO opendrift.models.basemodel.environment:248: sea_surface_swell_wave_to_direction: 0.000000
20:11:18 INFO opendrift.models.basemodel.environment:248: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0.000000
20:11:18 INFO opendrift.models.basemodel.environment:248: sea_surface_swell_wave_significant_height: 0.000000
20:11:18 INFO opendrift.models.basemodel.environment:248: sea_surface_wind_wave_to_direction: 0.000000
20:11:18 INFO opendrift.models.basemodel.environment:248: sea_surface_wind_wave_mean_period: 0.000000
20:11:18 INFO opendrift.models.basemodel.environment:248: sea_surface_wind_wave_significant_height: 0.000000
20:11:18 INFO opendrift.models.basemodel.environment:248: surface_downward_x_stress: 0.000000
20:11:18 INFO opendrift.models.basemodel.environment:248: surface_downward_y_stress: 0.000000
20:11:18 INFO opendrift.models.basemodel.environment:248: turbulent_kinetic_energy: 0.000000
20:11:18 INFO opendrift.models.basemodel.environment:248: turbulent_generic_length_scale: 0.000000
20:11:18 INFO opendrift.models.basemodel.environment:248: ocean_mixed_layer_thickness: 50.000000
20:11:18 DEBUG opendrift.models.basemodel:95: Changed mode from Mode.Config to Mode.Ready
20:11:18 DEBUG opendrift.models.basemodel:95: Changed mode from Mode.Ready to Mode.Run
20:11:19 DEBUG opendrift.models.basemodel:1777:
------------------------------------------------------
Software and hardware:
OpenDrift version 1.12.0
Platform: Linux, 5.15.0-1057-aws
68.56775283813477 GB memory
36 processors (x86_64)
NumPy version 1.26.4
SciPy version 1.14.1
Matplotlib version 3.9.1
NetCDF4 version 1.6.1
Xarray version 2024.11.0
ADIOS (adios_db) version 1.2.5
Copernicusmarine version 1.3.5
Python version 3.11.6 | packaged by conda-forge | (main, Oct 3 2023, 10:40:35) [GCC 12.3.0]
------------------------------------------------------
20:11:19 DEBUG opendrift.models.basemodel:1791: No output file is specified, neglecting export_buffer_length
20:11:19 DEBUG opendrift.models.basemodel:1909: Finalizing environment and preparing readers for simulation coverage ([-4.71627286447143, 55.39190523302233, 13.715758357177728, 64.60825116956556]) and time (2024-12-09 00:00:00 to 2024-12-11 23:00:00)
20:11:19 DEBUG opendrift.models.basemodel.environment:180: Preparing NorKyst manual aggregate for extent [-4.71627286447143, 55.39190523302233, 13.715758357177728, 64.60825116956556]
20:11:19 DEBUG opendrift.readers.basereader.structured:153: Clearing cache for reader NorKyst manual aggregate before starting new simulation
20:11:19 DEBUG opendrift.readers.basereader.variables:608: Setting buffer size 11 for reader NorKyst manual aggregate, assuming a maximum average speed of 2 m/s and time span of 1:00:00
20:11:19 DEBUG opendrift.readers.basereader.variables:549: Nothing more to prepare for NorKyst manual aggregate
20:11:19 DEBUG opendrift.models.basemodel.environment:180: Preparing global_landmask for extent [-4.71627286447143, 55.39190523302233, 13.715758357177728, 64.60825116956556]
20:11:19 DEBUG opendrift.readers.basereader.variables:549: Nothing more to prepare for global_landmask
20:11:19 INFO opendrift.models.basemodel:935: Using existing reader for land_binary_mask
20:11:19 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:11:19 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:11:19 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:11:19 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:11:19 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:11:19 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:11:19 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:11:19 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:11:19 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:11:19 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:11:19 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:11:19 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:11:19 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:11:19 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:11:19 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:11:19 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:11:19 INFO opendrift.models.basemodel:946: All points are in ocean
20:11:19 DEBUG opendrift.models.basemodel:890: to be seeded: 1000, already seeded 0
20:11:19 DEBUG opendrift.models.basemodel:908: Released 1000 new elements.
20:11:19 WARNING opendrift.models.basemodel:729: Seafloor check not being run because environment is missing. This will happen the first time the function is run but if it happens subsequently there is probably a problem.
20:11:19 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:11:19 INFO opendrift.models.basemodel:2035: 2024-12-09 00:00:00 - step 1 of 71 - 1000 active elements (0 deactivated)
20:11:19 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:11:19 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:11:19 DEBUG opendrift.models.basemodel:2054: 59.99731 <- latitude -> 60.002846
20:11:19 DEBUG opendrift.models.basemodel:2059: 4.4945407 <- longitude -> 4.504945
20:11:19 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:11:19 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:11:19 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:11:19 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:11:19 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:11:19 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:11:19 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:11:19 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:11:19 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:11:19 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-09 00:00:00 (before)
2024-12-09 01:00:00 (after)
20:11:31 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:11:31 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:11:31 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:11:31 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:11:31 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:11:31 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:11:31 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 22x23x7) for time before (2024-12-09 00:00:00)
20:11:31 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-09 00:00:00) in space (linearNDFast)
20:11:31 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:11:31 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:11:31 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.50547356643384 and -65.49506707409589 degrees.
20:11:31 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.50547356643384 and -65.49506707409589 degrees.
20:11:31 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:11:31 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:11:31 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:11:31 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:11:31 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:11:31 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:11:31 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:11:31 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:11:31 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:11:31 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:11:31 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:11:31 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:11:31 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:11:31 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:11:31 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:11:31 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:11:31 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0636311 (min) 0.0868608 (max)
20:11:31 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.13082 (min) 0.171825 (max)
20:11:31 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.498931 (min) -0.49627 (max)
20:11:31 DEBUG opendrift.models.basemodel.environment:905: x_wind: -2.22237 (min) -2.21359 (max)
20:11:31 DEBUG opendrift.models.basemodel.environment:905: y_wind: -4.82698 (min) -4.78051 (max)
20:11:31 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -2.06035e-07 (min) -1.19372e-07 (max)
20:11:31 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:11:31 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:11:31 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:11:31 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:11:31 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:11:31 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:11:31 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:11:31 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:11:31 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:11:31 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:11:31 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:11:31 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:11:31 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:11:31 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:11:31 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:11:31 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:11:31 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:11:31 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.369 (min) 298.632 (max)
20:11:31 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:11:31 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:11:31 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.682730, mean: 0.688796, max: 0.694671
20:11:31 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:11:31 DEBUG opendrift.models.physics_methods:1061: min: 4.501469, mean: 4.521418, max: 4.540660
20:11:31 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 4.501469, mean: 4.521418, max: 4.540660
20:11:31 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:11:31 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:11:31 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:11:31 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:11:31 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.105363 m/s - 0.106280 m/s)
20:11:31 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:11:31 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:11:31 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:11:31 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:11:31 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:11:31 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:11:31 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:11:31 INFO opendrift.models.basemodel:2035: 2024-12-09 01:00:00 - step 2 of 71 - 1000 active elements (0 deactivated)
20:11:31 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:11:31 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:11:31 DEBUG opendrift.models.basemodel:2054: 59.99877833511116 <- latitude -> 60.004880615268405
20:11:31 DEBUG opendrift.models.basemodel:2059: 4.496512215103492 <- longitude -> 4.507347810270389
20:11:31 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:11:31 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:11:31 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:11:31 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:11:31 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:11:31 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:11:31 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:11:31 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:11:31 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:11:31 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-09 01:00:00 (before)
2024-12-09 02:00:00 (after)
20:11:43 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:11:43 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:11:43 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:11:43 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:11:43 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:11:43 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:11:43 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 23x23x7) for time before (2024-12-09 01:00:00)
20:11:43 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-09 01:00:00) in space (linearNDFast)
20:11:43 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:11:43 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:11:43 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.5034987086908 and -65.49266310750974 degrees.
20:11:43 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.5034987086908 and -65.49266310750974 degrees.
20:11:43 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:11:43 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:11:43 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:11:43 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:11:43 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:11:43 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:11:43 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:11:43 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:11:43 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:11:43 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:11:43 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:11:43 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:11:43 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:11:43 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:11:43 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:11:43 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:11:43 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0576887 (min) 0.0829642 (max)
20:11:43 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.178474 (min) 0.223884 (max)
20:11:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.355291 (min) -0.352205 (max)
20:11:43 DEBUG opendrift.models.basemodel.environment:905: x_wind: -2.46911 (min) -2.42724 (max)
20:11:43 DEBUG opendrift.models.basemodel.environment:905: y_wind: -4.41677 (min) -4.38233 (max)
20:11:43 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 3.98623e-05 (min) 4.0016e-05 (max)
20:11:43 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:11:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:11:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:11:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:11:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:11:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:11:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:11:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:11:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:11:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:11:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:11:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:11:43 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:11:43 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:11:43 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:11:43 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:11:43 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:11:43 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.485 (min) 298.718 (max)
20:11:43 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:11:43 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:11:43 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.617369, mean: 0.623941, max: 0.629801
20:11:43 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:11:43 DEBUG opendrift.models.physics_methods:1061: min: 4.280575, mean: 4.303293, max: 4.323457
20:11:43 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 4.280575, mean: 4.303293, max: 4.323457
20:11:43 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:11:43 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:11:43 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:11:43 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:11:43 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.100192 m/s - 0.101196 m/s)
20:11:43 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:11:43 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:11:43 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:11:43 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:11:43 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:11:43 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:11:43 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:11:43 INFO opendrift.models.basemodel:2035: 2024-12-09 02:00:00 - step 3 of 71 - 1000 active elements (0 deactivated)
20:11:43 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:11:43 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:11:43 DEBUG opendrift.models.basemodel:2054: 60.001874670094665 <- latitude -> 60.00884911829958
20:11:43 DEBUG opendrift.models.basemodel:2059: 4.497672933677941 <- longitude -> 4.509356268674435
20:11:43 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:11:43 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:11:43 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:11:43 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:11:43 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:11:43 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:11:43 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:11:43 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:11:43 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:11:43 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-09 02:00:00 (before)
2024-12-09 03:00:00 (after)
20:11:57 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:11:57 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:11:57 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:11:57 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:11:57 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:11:57 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:11:57 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 23x23x7) for time before (2024-12-09 02:00:00)
20:11:57 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-09 02:00:00) in space (linearNDFast)
20:11:57 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:11:57 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:11:57 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.50233798985666 and -65.49065465669653 degrees.
20:11:57 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.50233798985666 and -65.49065465669653 degrees.
20:11:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:11:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:11:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:11:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:11:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:11:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:11:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:11:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:11:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:11:57 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:11:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:11:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:11:57 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:11:57 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:11:57 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:11:57 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:11:57 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0579977 (min) 0.0883811 (max)
20:11:57 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.211589 (min) 0.259497 (max)
20:11:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.231026 (min) -0.227762 (max)
20:11:57 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.71464 (min) -1.69599 (max)
20:11:57 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.82932 (min) -3.80628 (max)
20:11:57 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 6.19455e-05 (min) 6.40052e-05 (max)
20:11:57 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:11:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:11:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:11:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:11:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:11:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:11:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:11:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:11:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:11:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:11:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:11:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:11:57 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:11:57 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:11:57 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:11:57 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:11:57 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:11:57 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.659 (min) 298.837 (max)
20:11:57 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:11:57 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:11:57 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.428525, mean: 0.429824, max: 0.431547
20:11:57 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:11:57 DEBUG opendrift.models.physics_methods:1061: min: 3.566297, mean: 3.571697, max: 3.578851
20:11:57 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.566297, mean: 3.571697, max: 3.578851
20:11:57 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:11:57 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:11:57 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:11:57 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:11:57 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.083474 m/s - 0.083768 m/s)
20:11:57 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:11:57 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:11:57 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:11:57 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:11:57 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:11:57 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:11:57 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:11:57 INFO opendrift.models.basemodel:2035: 2024-12-09 03:00:00 - step 4 of 71 - 1000 active elements (0 deactivated)
20:11:57 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:11:57 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:11:57 DEBUG opendrift.models.basemodel:2054: 60.006427186729354 <- latitude -> 60.01456012067315
20:11:57 DEBUG opendrift.models.basemodel:2059: 4.499535062480698 <- longitude -> 4.512666472955501
20:11:57 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:11:57 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:11:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:11:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:11:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:11:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:11:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:11:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:11:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:11:57 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-09 03:00:00 (before)
2024-12-09 04:00:00 (after)
20:12:09 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:12:09 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:12:09 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:12:09 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:12:09 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:12:09 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:12:09 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 23x23x7) for time before (2024-12-09 03:00:00)
20:12:09 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-09 03:00:00) in space (linearNDFast)
20:12:09 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:12:09 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:12:09 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.50047585185229 and -65.48734445583686 degrees.
20:12:09 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.50047585185229 and -65.48734445583686 degrees.
20:12:09 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:12:09 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:12:09 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:12:09 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:12:09 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:12:09 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:12:09 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:12:09 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:12:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:12:09 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:12:09 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:12:09 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:12:09 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:12:09 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:12:09 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:12:09 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:12:09 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0716486 (min) 0.117673 (max)
20:12:09 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.227704 (min) 0.282803 (max)
20:12:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.155143 (min) -0.150649 (max)
20:12:09 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.571639 (min) -0.545044 (max)
20:12:09 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.74355 (min) -3.68962 (max)
20:12:09 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 2.62006e-05 (min) 2.72465e-05 (max)
20:12:09 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:12:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:12:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:12:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:12:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:12:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:12:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:12:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:12:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:12:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:12:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:12:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:12:09 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:12:09 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:12:09 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:12:09 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:12:09 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:12:09 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.871 (min) 299.007 (max)
20:12:09 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:12:09 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:12:09 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.342195, mean: 0.347382, max: 0.352787
20:12:09 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:12:09 DEBUG opendrift.models.physics_methods:1061: min: 3.186885, mean: 3.210939, max: 3.235832
20:12:09 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.186885, mean: 3.210939, max: 3.235832
20:12:09 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:12:09 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:12:09 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:12:09 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:12:09 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.074593 m/s - 0.075739 m/s)
20:12:09 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:12:09 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:12:09 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:12:09 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:12:09 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:12:09 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:12:09 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:12:09 INFO opendrift.models.basemodel:2035: 2024-12-09 04:00:00 - step 5 of 71 - 1000 active elements (0 deactivated)
20:12:09 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:12:09 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:12:09 DEBUG opendrift.models.basemodel:2054: 60.01148450007494 <- latitude -> 60.021110137860376
20:12:09 DEBUG opendrift.models.basemodel:2059: 4.503608843525559 <- longitude -> 4.519484932178915
20:12:09 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:12:09 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:12:09 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:12:09 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:12:09 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:12:09 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:12:09 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:12:09 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:12:09 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:12:09 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-09 04:00:00 (before)
2024-12-09 05:00:00 (after)
20:12:21 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:12:21 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:12:21 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:12:21 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:12:21 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:12:21 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:12:21 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 23x23x7) for time before (2024-12-09 04:00:00)
20:12:21 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-09 04:00:00) in space (linearNDFast)
20:12:21 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:12:21 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:12:21 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.49640206906183 and -65.4805259821425 degrees.
20:12:21 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.49640206906183 and -65.4805259821425 degrees.
20:12:21 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:12:21 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:12:21 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:12:21 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:12:21 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:12:21 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:12:21 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:12:21 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:12:21 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:12:21 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:12:21 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:12:21 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:12:21 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:12:21 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:12:21 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:12:21 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:12:21 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0718497 (min) 0.135422 (max)
20:12:21 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.21375 (min) 0.283709 (max)
20:12:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.134602 (min) -0.129465 (max)
20:12:21 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.302421 (min) -0.218299 (max)
20:12:21 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.87818 (min) -3.80904 (max)
20:12:21 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -6.4234e-06 (min) -6.10086e-06 (max)
20:12:21 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:12:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:12:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:12:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:12:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:12:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:12:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:12:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:12:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:12:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:12:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:12:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:12:21 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:12:21 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:12:21 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:12:21 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:12:21 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:12:21 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 299.074 (min) 299.214 (max)
20:12:21 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:12:21 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:12:21 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.358088, mean: 0.365750, max: 0.372241
20:12:21 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:12:21 DEBUG opendrift.models.physics_methods:1061: min: 3.260050, mean: 3.294732, max: 3.323854
20:12:21 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.260050, mean: 3.294732, max: 3.323854
20:12:21 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:12:21 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:12:21 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:12:21 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:12:21 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.076306 m/s - 0.077799 m/s)
20:12:21 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:12:21 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:12:21 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:12:21 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:12:21 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:12:21 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:12:21 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:12:21 INFO opendrift.models.basemodel:2035: 2024-12-09 05:00:00 - step 6 of 71 - 1000 active elements (0 deactivated)
20:12:21 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:12:21 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:12:21 DEBUG opendrift.models.basemodel:2054: 60.015929384286046 <- latitude -> 60.0276231942277
20:12:21 DEBUG opendrift.models.basemodel:2059: 4.5079461556790745 <- longitude -> 4.527942814831888
20:12:21 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:12:21 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:12:21 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:12:21 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:12:21 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:12:21 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:12:21 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:12:21 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:12:21 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:12:21 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-09 05:00:00 (before)
2024-12-09 06:00:00 (after)
20:12:37 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:12:37 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:12:37 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:12:37 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:12:37 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:12:37 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:12:37 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 23x24x7) for time before (2024-12-09 05:00:00)
20:12:37 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-09 05:00:00) in space (linearNDFast)
20:12:37 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:12:37 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:12:37 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.4920647467938 and -65.47206809900207 degrees.
20:12:37 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.4920647467938 and -65.47206809900207 degrees.
20:12:37 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:12:37 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:12:37 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:12:37 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:12:37 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:12:37 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:12:37 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:12:37 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:12:37 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:12:37 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:12:37 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:12:37 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:12:37 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:12:37 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:12:37 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:12:37 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:12:37 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0378795 (min) 0.123987 (max)
20:12:37 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.187982 (min) 0.27097 (max)
20:12:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.182955 (min) -0.175751 (max)
20:12:37 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.361006 (min) -0.266699 (max)
20:12:37 DEBUG opendrift.models.basemodel.environment:905: y_wind: -4.39219 (min) -4.38151 (max)
20:12:37 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -2.25407e-05 (min) -2.21367e-05 (max)
20:12:37 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:12:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:12:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:12:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:12:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:12:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:12:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:12:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:12:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:12:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:12:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:12:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:12:37 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:12:37 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:12:37 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:12:37 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:12:37 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:12:37 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 299.218 (min) 299.415 (max)
20:12:37 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:12:37 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:12:37 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.474616, mean: 0.476364, max: 0.477088
20:12:37 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:12:37 DEBUG opendrift.models.physics_methods:1061: min: 3.753192, mean: 3.760098, max: 3.762955
20:12:37 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.753192, mean: 3.760098, max: 3.762955
20:12:37 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:12:37 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:12:37 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:12:37 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:12:37 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.087848 m/s - 0.088077 m/s)
20:12:37 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:12:37 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:12:37 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:12:37 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:12:37 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:12:37 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:12:37 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:12:37 INFO opendrift.models.basemodel:2035: 2024-12-09 06:00:00 - step 7 of 71 - 1000 active elements (0 deactivated)
20:12:37 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:12:37 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:12:37 DEBUG opendrift.models.basemodel:2054: 60.01916994352439 <- latitude -> 60.033403003734065
20:12:37 DEBUG opendrift.models.basemodel:2059: 4.509926378651621 <- longitude -> 4.5356029437962455
20:12:37 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:12:37 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:12:37 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:12:37 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:12:37 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:12:37 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:12:37 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:12:37 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:12:37 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:12:37 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-09 06:00:00 (before)
2024-12-09 07:00:00 (after)
20:12:53 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:12:53 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:12:53 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:12:53 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:12:53 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:12:53 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:12:53 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 24x25x7) for time before (2024-12-09 06:00:00)
20:12:53 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-09 06:00:00) in space (linearNDFast)
20:12:53 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:12:53 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:12:53 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.49008452969287 and -65.46440797634776 degrees.
20:12:53 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.49008452969287 and -65.46440797634776 degrees.
20:12:53 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:12:53 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:12:53 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:12:53 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:12:53 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:12:53 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:12:53 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:12:53 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:12:53 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:12:53 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:12:53 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:12:53 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:12:53 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:12:53 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:12:53 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:12:53 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:12:53 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.02829 (min) 0.0993821 (max)
20:12:53 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.182652 (min) 0.26924 (max)
20:12:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.30122 (min) -0.292731 (max)
20:12:53 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.26032 (min) -1.23703 (max)
20:12:53 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.40988 (min) -3.32579 (max)
20:12:53 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -3.99289e-05 (min) -3.93504e-05 (max)
20:12:53 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:12:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:12:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:12:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:12:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:12:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:12:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:12:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:12:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:12:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:12:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:12:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:12:53 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:12:53 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:12:53 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:12:53 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:12:53 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:12:53 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 299.28 (min) 299.571 (max)
20:12:53 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:12:53 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:12:53 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.311172, mean: 0.318760, max: 0.323871
20:12:53 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:12:53 DEBUG opendrift.models.physics_methods:1061: min: 3.038996, mean: 3.075808, max: 3.100384
20:12:53 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.038996, mean: 3.075808, max: 3.100384
20:12:53 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:12:53 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:12:53 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:12:53 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:12:53 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.071132 m/s - 0.072569 m/s)
20:12:53 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:12:53 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:12:53 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:12:53 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:12:53 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:12:53 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:12:53 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:12:53 INFO opendrift.models.basemodel:2035: 2024-12-09 07:00:00 - step 8 of 71 - 1000 active elements (0 deactivated)
20:12:53 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:12:53 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:12:53 DEBUG opendrift.models.basemodel:2054: 60.02292241188671 <- latitude -> 60.03978450514933
20:12:53 DEBUG opendrift.models.basemodel:2059: 4.506496678273472 <- longitude -> 4.5403922047790015
20:12:53 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:12:53 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:12:53 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:12:53 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:12:53 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:12:53 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:12:53 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:12:53 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:12:53 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:12:53 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-09 07:00:00 (before)
2024-12-09 08:00:00 (after)
20:13:08 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:13:08 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:08 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:08 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:13:08 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:08 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:08 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 23x25x7) for time before (2024-12-09 07:00:00)
20:13:08 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-09 07:00:00) in space (linearNDFast)
20:13:08 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:13:08 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:13:08 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.49351423194543 and -65.45961871523568 degrees.
20:13:08 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.49351423194543 and -65.45961871523568 degrees.
20:13:08 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:08 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:08 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:08 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:08 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:08 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:08 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:08 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:08 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:08 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:08 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:08 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:08 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:08 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:08 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:13:08 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:08 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.114132 (min) 0.0813691 (max)
20:13:08 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.20515 (min) 0.297217 (max)
20:13:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.456912 (min) -0.443962 (max)
20:13:08 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.536833 (min) -0.502157 (max)
20:13:08 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.73607 (min) -2.41245 (max)
20:13:08 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -4.62868e-05 (min) -4.43121e-05 (max)
20:13:08 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:13:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:13:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:13:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:13:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:13:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:13:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:13:08 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:13:08 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:13:08 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:13:08 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:13:08 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:08 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 299.189 (min) 299.675 (max)
20:13:08 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:08 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:08 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.150259, mean: 0.171899, max: 0.190382
20:13:08 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:08 DEBUG opendrift.models.physics_methods:1061: min: 2.111789, mean: 2.258367, max: 2.377073
20:13:08 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.111789, mean: 2.258367, max: 2.377073
20:13:08 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:08 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:13:09 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:09 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:13:09 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.049429 m/s - 0.055638 m/s)
20:13:09 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:09 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:13:09 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:13:09 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:09 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:09 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:13:09 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:09 INFO opendrift.models.basemodel:2035: 2024-12-09 08:00:00 - step 9 of 71 - 1000 active elements (0 deactivated)
20:13:09 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:09 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:09 DEBUG opendrift.models.basemodel:2054: 60.02799214484793 <- latitude -> 60.047619854943314
20:13:09 DEBUG opendrift.models.basemodel:2059: 4.4984723218978875 <- longitude -> 4.544953235167174
20:13:09 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:13:09 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:09 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:09 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:13:09 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:09 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:13:09 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:09 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:09 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:13:09 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-09 08:00:00 (before)
2024-12-09 09:00:00 (after)
20:13:24 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:13:24 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:24 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:24 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:13:24 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:24 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:24 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 23x26x7) for time before (2024-12-09 08:00:00)
20:13:24 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-09 08:00:00) in space (linearNDFast)
20:13:24 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:13:24 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:13:24 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.50153859133619 and -65.45505768921286 degrees.
20:13:24 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.50153859133619 and -65.45505768921286 degrees.
20:13:24 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:24 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:24 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:24 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:24 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:24 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:24 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:24 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:24 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:24 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:24 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:24 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:24 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:24 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:24 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:13:24 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:24 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.226995 (min) 0.0568298 (max)
20:13:24 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.240466 (min) 0.335084 (max)
20:13:24 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.611017 (min) -0.590327 (max)
20:13:24 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0.479521 (min) 0.582402 (max)
20:13:24 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.90338 (min) -2.59179 (max)
20:13:24 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -3.68284e-05 (min) -3.47561e-05 (max)
20:13:24 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:13:24 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:24 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:24 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:24 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:24 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:24 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:13:24 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:13:24 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:13:24 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:13:24 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:13:24 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:13:24 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:13:24 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:13:24 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:13:24 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:13:24 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:24 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.949 (min) 299.775 (max)
20:13:24 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:24 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:24 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.173592, mean: 0.195752, max: 0.213025
20:13:24 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:24 DEBUG opendrift.models.physics_methods:1061: min: 2.269835, mean: 2.410055, max: 2.514463
20:13:24 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.269835, mean: 2.410055, max: 2.514463
20:13:24 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:24 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:13:24 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:24 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:13:24 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.053128 m/s - 0.058854 m/s)
20:13:24 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:24 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:13:24 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:13:24 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:24 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:24 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:13:24 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:24 INFO opendrift.models.basemodel:2035: 2024-12-09 09:00:00 - step 10 of 71 - 1000 active elements (0 deactivated)
20:13:24 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:24 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:24 DEBUG opendrift.models.basemodel:2054: 60.03408715970647 <- latitude -> 60.05651020776513
20:13:24 DEBUG opendrift.models.basemodel:2059: 4.4844214358680645 <- longitude -> 4.549375898263872
20:13:24 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:13:24 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:24 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:24 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:13:24 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:24 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:13:24 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:24 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:24 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:13:24 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-09 09:00:00 (before)
2024-12-09 10:00:00 (after)
20:13:40 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:13:40 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:40 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:40 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:13:40 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:40 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:40 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 23x27x7) for time before (2024-12-09 09:00:00)
20:13:40 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-09 09:00:00) in space (linearNDFast)
20:13:40 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:13:40 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:13:40 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.51558947238051 and -65.45063501802926 degrees.
20:13:40 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.51558947238051 and -65.45063501802926 degrees.
20:13:40 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:40 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:40 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:40 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:40 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:40 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:40 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:40 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:40 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:40 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:40 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:40 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:40 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:40 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:40 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:13:40 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:40 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.367678 (min) 0.0181515 (max)
20:13:40 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.284566 (min) 0.3677 (max)
20:13:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.706917 (min) -0.675723 (max)
20:13:40 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0.868346 (min) 0.874854 (max)
20:13:40 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.65082 (min) -3.52415 (max)
20:13:40 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -1.78681e-05 (min) -1.43911e-05 (max)
20:13:40 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:13:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:13:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:13:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:13:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:13:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:13:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:13:40 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:13:40 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:13:40 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:13:40 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:13:40 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:40 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.465 (min) 299.861 (max)
20:13:40 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:40 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:40 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.324226, mean: 0.343157, max: 0.346448
20:13:40 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:40 DEBUG opendrift.models.physics_methods:1061: min: 3.102084, mean: 3.191319, max: 3.206627
20:13:40 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.102084, mean: 3.191319, max: 3.206627
20:13:40 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:40 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:13:40 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:40 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:13:40 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.072608 m/s - 0.075055 m/s)
20:13:40 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:40 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:13:40 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:13:40 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:40 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:40 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:13:40 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:40 INFO opendrift.models.basemodel:2035: 2024-12-09 10:00:00 - step 11 of 71 - 1000 active elements (0 deactivated)
20:13:40 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:40 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:40 DEBUG opendrift.models.basemodel:2054: 60.04100464611879 <- latitude -> 60.0652354867284
20:13:40 DEBUG opendrift.models.basemodel:2059: 4.46177444744454 <- longitude -> 4.551674976781974
20:13:40 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:13:40 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:40 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:40 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:13:40 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:40 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:13:40 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:40 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:40 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:13:40 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-09 10:00:00 (before)
2024-12-09 11:00:00 (after)
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:57 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 22x29x7) for time before (2024-12-09 10:00:00)
20:13:57 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-09 10:00:00) in space (linearNDFast)
20:13:57 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:13:57 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:13:57 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.53823646014548 and -65.4483359298045 degrees.
20:13:57 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.53823646014548 and -65.4483359298045 degrees.
20:13:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:57 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:57 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:13:57 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:57 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.489423 (min) -0.0602175 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.325246 (min) 0.412649 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.725769 (min) -0.681969 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0.188668 (min) 0.312312 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: y_wind: -4.1284 (min) -3.91107 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 1.00006e-06 (min) 3.90455e-06 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 297.603 (min) 299.915 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:57 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.377169, mean: 0.399094, max: 0.421675
20:13:57 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:57 DEBUG opendrift.models.physics_methods:1061: min: 3.345779, mean: 3.441536, max: 3.537678
20:13:57 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.345779, mean: 3.441536, max: 3.537678
20:13:57 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:57 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:13:57 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:57 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:13:57 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.078312 m/s - 0.082804 m/s)
20:13:57 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:57 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:13:57 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:13:57 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:57 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:57 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:13:57 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:57 INFO opendrift.models.basemodel:2035: 2024-12-09 11:00:00 - step 12 of 71 - 1000 active elements (0 deactivated)
20:13:57 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:57 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:57 DEBUG opendrift.models.basemodel:2054: 60.04964035331626 <- latitude -> 60.073204780719664
20:13:57 DEBUG opendrift.models.basemodel:2059: 4.430530274326379 <- longitude -> 4.548027655440524
20:13:57 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:13:57 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:13:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:13:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-09 11:00:00 (before)
2024-12-09 12:00:00 (after)
20:14:09 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:14:09 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:14:09 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:14:09 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:14:09 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:14:09 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:14:09 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 23x31x7) for time before (2024-12-09 11:00:00)
20:14:09 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-09 11:00:00) in space (linearNDFast)
20:14:09 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:14:09 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:14:09 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.56948063756609 and -65.45198324961974 degrees.
20:14:09 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.56948063756609 and -65.45198324961974 degrees.
20:14:09 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:14:09 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:14:09 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:14:09 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:14:09 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:14:09 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:14:09 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:14:09 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:14:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:14:09 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:14:09 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:14:09 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:14:09 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:14:09 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:14:09 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:14:09 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:14:09 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.560076 (min) -0.183748 (max)
20:14:09 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.25724 (min) 0.440957 (max)
20:14:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.669651 (min) -0.618415 (max)
20:14:09 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.295479 (min) -0.092748 (max)
20:14:09 DEBUG opendrift.models.basemodel.environment:905: y_wind: -4.34287 (min) -3.86708 (max)
20:14:09 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 2.44294e-05 (min) 2.65007e-05 (max)
20:14:09 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:14:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:14:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:14:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:14:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:14:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:14:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:14:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:14:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:14:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:14:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:14:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:14:09 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:14:09 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:14:09 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:14:09 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:14:09 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:14:09 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 296.252 (min) 299.884 (max)
20:14:09 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:14:09 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:14:09 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.370024, mean: 0.417240, max: 0.464180
20:14:09 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:14:09 DEBUG opendrift.models.physics_methods:1061: min: 3.313940, mean: 3.518429, max: 3.711701
20:14:09 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.313940, mean: 3.518429, max: 3.711701
20:14:09 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:14:09 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:14:09 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:14:09 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:14:09 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.077567 m/s - 0.086877 m/s)
20:14:09 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:14:09 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:14:09 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:14:09 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:14:09 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:14:09 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:14:09 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:14:09 INFO opendrift.models.basemodel:2035: 2024-12-09 12:00:00 - step 13 of 71 - 1000 active elements (0 deactivated)
20:14:09 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:14:09 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:14:09 DEBUG opendrift.models.basemodel:2054: 60.0595298874734 <- latitude -> 60.08069036084018
20:14:09 DEBUG opendrift.models.basemodel:2059: 4.394629551279647 <- longitude -> 4.535768736108945
20:14:09 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:14:09 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:14:09 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:14:09 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:14:09 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:14:09 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:14:09 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:14:09 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:14:09 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:14:09 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-09 12:00:00 (before)
2024-12-09 13:00:00 (after)
20:14:21 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:14:21 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:14:21 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:14:21 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:14:21 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:14:21 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:14:21 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 24x32x7) for time before (2024-12-09 12:00:00)
20:14:21 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-09 12:00:00) in space (linearNDFast)
20:14:21 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:14:21 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:14:21 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.60538135266174 and -65.4642421729941 degrees.
20:14:21 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.60538135266174 and -65.4642421729941 degrees.
20:14:21 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:14:21 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:14:21 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:14:21 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:14:21 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:14:21 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:14:21 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:14:21 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:14:21 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:14:21 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:14:21 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:14:21 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:14:21 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:14:21 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:14:21 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:14:21 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:14:21 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.615595 (min) -0.328291 (max)
20:14:21 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.110906 (min) 0.452548 (max)
20:14:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.553224 (min) -0.505182 (max)
20:14:21 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.688847 (min) -0.402382 (max)
20:14:21 DEBUG opendrift.models.basemodel.environment:905: y_wind: -4.78395 (min) -3.94696 (max)
20:14:21 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 3.67868e-05 (min) 4.03213e-05 (max)
20:14:21 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:14:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:14:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:14:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:14:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:14:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:14:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:14:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:14:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:14:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:14:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:14:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:14:21 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:14:21 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:14:21 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:14:21 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:14:21 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:14:21 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 294.502 (min) 299.69 (max)
20:14:21 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:14:21 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:14:21 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.394904, mean: 0.504410, max: 0.566983
20:14:21 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:14:21 DEBUG opendrift.models.physics_methods:1061: min: 3.423540, mean: 3.867703, max: 4.102179
20:14:21 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.423540, mean: 3.867703, max: 4.102179
20:14:21 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:14:21 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:14:21 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:14:21 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:14:21 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.080132 m/s - 0.096017 m/s)
20:14:21 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:14:21 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:14:21 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:14:21 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:14:21 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:14:21 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:14:21 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:14:21 INFO opendrift.models.basemodel:2035: 2024-12-09 13:00:00 - step 14 of 71 - 1000 active elements (0 deactivated)
20:14:21 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:14:21 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:14:21 DEBUG opendrift.models.basemodel:2054: 60.070610746358085 <- latitude -> 60.08803527530129
20:14:21 DEBUG opendrift.models.basemodel:2059: 4.361083753911169 <- longitude -> 4.51365105716442
20:14:21 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:14:21 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:14:21 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:14:21 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:14:21 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:14:21 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:14:21 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:14:21 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:14:21 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:14:21 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-09 13:00:00 (before)
2024-12-09 14:00:00 (after)
20:14:37 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:14:37 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:14:37 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:14:37 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:14:37 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:14:37 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:14:37 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 25x33x7) for time before (2024-12-09 13:00:00)
20:14:37 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-09 13:00:00) in space (linearNDFast)
20:14:37 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:14:37 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:14:37 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.63892715251441 and -65.4863598504384 degrees.
20:14:37 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.63892715251441 and -65.4863598504384 degrees.
20:14:37 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:14:37 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:14:37 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:14:37 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:14:37 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:14:37 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:14:37 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:14:37 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:14:37 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:14:37 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:14:37 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:14:37 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:14:37 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:14:37 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:14:37 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:14:37 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:14:37 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.654576 (min) -0.447853 (max)
20:14:37 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0409028 (min) 0.458163 (max)
20:14:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.415787 (min) -0.375261 (max)
20:14:37 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.22207 (min) -0.365829 (max)
20:14:37 DEBUG opendrift.models.basemodel.environment:905: y_wind: -4.93673 (min) -3.40006 (max)
20:14:37 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 3.66902e-05 (min) 4.06481e-05 (max)
20:14:37 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:14:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:14:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:14:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:14:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:14:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:14:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:14:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:14:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:14:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:14:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:14:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:14:37 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:14:37 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:14:37 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:14:37 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:14:37 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:14:37 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 293.16 (min) 299.138 (max)
20:14:37 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:14:37 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:14:37 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.321124, mean: 0.548503, max: 0.602827
20:14:37 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:14:37 DEBUG opendrift.models.physics_methods:1061: min: 3.087210, mean: 4.032085, max: 4.229860
20:14:37 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.087210, mean: 4.032085, max: 4.229860
20:14:37 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:14:37 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:14:37 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:14:37 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:14:37 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.072260 m/s - 0.099005 m/s)
20:14:37 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:14:37 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:14:37 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:14:37 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:14:37 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:14:37 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:14:37 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:14:37 INFO opendrift.models.basemodel:2035: 2024-12-09 14:00:00 - step 15 of 71 - 1000 active elements (0 deactivated)
20:14:37 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:14:37 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:14:37 DEBUG opendrift.models.basemodel:2054: 60.074546473582274 <- latitude -> 60.094343884755204
20:14:37 DEBUG opendrift.models.basemodel:2059: 4.331648911231125 <- longitude -> 4.480432855845975
20:14:37 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:14:37 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:14:37 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:14:37 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:14:37 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:14:37 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:14:37 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:14:37 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:14:37 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:14:37 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-09 14:00:00 (before)
2024-12-09 15:00:00 (after)
20:14:54 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:14:54 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:14:54 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:14:54 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:14:54 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:14:54 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:14:54 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 27x31x7) for time before (2024-12-09 14:00:00)
20:14:54 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-09 14:00:00) in space (linearNDFast)
20:14:54 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:14:54 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:14:54 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.66836200070512 and -65.51957805126895 degrees.
20:14:54 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.66836200070512 and -65.51957805126895 degrees.
20:14:54 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:14:54 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:14:54 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:14:54 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:14:54 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:14:54 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:14:54 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:14:54 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:14:54 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:14:54 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:14:54 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:14:54 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:14:54 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:14:54 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:14:54 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:14:54 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:14:54 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.681198 (min) -0.348572 (max)
20:14:54 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.195769 (min) 0.463803 (max)
20:14:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.290911 (min) -0.261681 (max)
20:14:54 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.778796 (min) 0.223257 (max)
20:14:54 DEBUG opendrift.models.basemodel.environment:905: y_wind: -4.82516 (min) -3.65577 (max)
20:14:54 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 2.84579e-05 (min) 3.15829e-05 (max)
20:14:54 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:14:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:14:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:14:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:14:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:14:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:14:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:14:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:14:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:14:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:14:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:14:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:14:54 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:14:54 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:14:54 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:14:54 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:14:54 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:14:54 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 291.824 (min) 297.862 (max)
20:14:54 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:14:54 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:14:54 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.343690, mean: 0.567957, max: 0.573319
20:14:54 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:14:54 DEBUG opendrift.models.physics_methods:1061: min: 3.193839, mean: 4.105217, max: 4.125035
20:14:54 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.193839, mean: 4.105217, max: 4.125035
20:14:54 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:14:54 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:14:54 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:14:54 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:14:54 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.074756 m/s - 0.096552 m/s)
20:14:54 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:14:54 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:14:54 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:14:54 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:14:54 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:14:54 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:14:54 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:14:54 INFO opendrift.models.basemodel:2035: 2024-12-09 15:00:00 - step 16 of 71 - 1000 active elements (0 deactivated)
20:14:54 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:14:54 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:14:54 DEBUG opendrift.models.basemodel:2054: 60.065119826756444 <- latitude -> 60.10027738966282
20:14:54 DEBUG opendrift.models.basemodel:2059: 4.30940262425346 <- longitude -> 4.438517198576622
20:14:54 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:14:54 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:14:54 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:14:54 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:14:54 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:14:54 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:14:54 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:14:54 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:14:54 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:14:54 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-09 15:00:00 (before)
2024-12-09 16:00:00 (after)
20:15:10 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:15:10 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:15:10 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:15:10 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:15:10 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:15:10 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:15:10 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 29x28x7) for time before (2024-12-09 15:00:00)
20:15:10 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-09 15:00:00) in space (linearNDFast)
20:15:10 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:15:10 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:15:10 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.69060829766286 and -65.56149369686497 degrees.
20:15:10 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.69060829766286 and -65.56149369686497 degrees.
20:15:10 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:15:10 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:15:10 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:15:10 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:15:10 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:15:10 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:15:10 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:15:10 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:15:10 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:15:10 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:15:10 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:15:10 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:15:10 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:15:10 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:15:10 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:15:10 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:15:10 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.6834 (min) -0.252529 (max)
20:15:10 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.324246 (min) 0.394982 (max)
20:15:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.203593 (min) -0.182319 (max)
20:15:10 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0.277435 (min) 0.373005 (max)
20:15:10 DEBUG opendrift.models.basemodel.environment:905: y_wind: -5.29401 (min) -5.05972 (max)
20:15:10 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 1.68841e-05 (min) 1.89463e-05 (max)
20:15:10 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:15:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:15:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:15:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:15:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:15:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:15:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:15:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:15:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:15:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:15:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:15:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:15:10 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:15:10 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:15:10 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:15:10 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:15:10 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:15:10 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 290.625 (min) 295.402 (max)
20:15:10 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:15:10 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:15:10 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.632825, mean: 0.638099, max: 0.691347
20:15:10 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:15:10 DEBUG opendrift.models.physics_methods:1061: min: 4.333823, mean: 4.351773, max: 4.529784
20:15:10 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 4.333823, mean: 4.351773, max: 4.529784
20:15:10 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:15:10 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:15:10 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:15:10 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:15:10 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.101439 m/s - 0.106026 m/s)
20:15:10 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:15:10 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:15:10 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:15:10 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:15:10 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:15:10 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:15:10 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:15:10 INFO opendrift.models.basemodel:2035: 2024-12-09 16:00:00 - step 17 of 71 - 1000 active elements (0 deactivated)
20:15:10 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:15:10 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:15:10 DEBUG opendrift.models.basemodel:2054: 60.0513639327341 <- latitude -> 60.10538962015637
20:15:10 DEBUG opendrift.models.basemodel:2059: 4.29354746714102 <- longitude -> 4.394640986114139
20:15:10 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:15:10 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:15:10 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:15:10 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:15:10 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:15:10 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:15:10 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:15:10 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:15:10 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:15:10 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-09 16:00:00 (before)
2024-12-09 17:00:00 (after)
20:15:26 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:15:26 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:15:26 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:15:26 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:15:26 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:15:26 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:15:26 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 31x26x7) for time before (2024-12-09 16:00:00)
20:15:26 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-09 16:00:00) in space (linearNDFast)
20:15:26 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:15:26 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:15:26 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.7064634611654 and -65.60536991003036 degrees.
20:15:26 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.7064634611654 and -65.60536991003036 degrees.
20:15:26 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:15:26 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:15:26 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:15:26 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:15:26 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:15:26 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:15:26 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:15:26 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:15:26 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:15:26 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:15:26 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:15:26 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:15:26 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:15:26 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:15:26 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:15:26 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:15:26 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.629556 (min) -0.178619 (max)
20:15:26 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.402534 (min) 0.190883 (max)
20:15:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.165292 (min) -0.14841 (max)
20:15:26 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.146268 (min) 0.0526836 (max)
20:15:26 DEBUG opendrift.models.basemodel.environment:905: y_wind: -4.64684 (min) -4.61659 (max)
20:15:26 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -2.24402e-07 (min) 1.63394e-06 (max)
20:15:26 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:15:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:15:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:15:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:15:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:15:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:15:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:15:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:15:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:15:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:15:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:15:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:15:26 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:15:26 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:15:26 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:15:26 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:15:26 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:15:26 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 289.77 (min) 295.49 (max)
20:15:26 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:15:26 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:15:26 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.524359, mean: 0.526897, max: 0.531196
20:15:26 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:15:26 DEBUG opendrift.models.physics_methods:1061: min: 3.944973, mean: 3.954501, max: 3.970608
20:15:26 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.944973, mean: 3.954501, max: 3.970608
20:15:26 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:15:26 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:15:26 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:15:26 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:15:26 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.092337 m/s - 0.092937 m/s)
20:15:26 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:15:26 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:15:26 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:15:26 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:15:26 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:15:26 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:15:26 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:15:26 INFO opendrift.models.basemodel:2035: 2024-12-09 17:00:00 - step 18 of 71 - 1000 active elements (0 deactivated)
20:15:26 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:15:26 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:15:26 DEBUG opendrift.models.basemodel:2054: 60.035367668527314 <- latitude -> 60.10832894945364
20:15:26 DEBUG opendrift.models.basemodel:2059: 4.282005857547621 <- longitude -> 4.353698131468008
20:15:26 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:15:26 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:15:26 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:15:26 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:15:26 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:15:26 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:15:26 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:15:26 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:15:26 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:15:26 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-09 17:00:00 (before)
2024-12-09 18:00:00 (after)
20:15:42 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:15:42 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:15:42 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:15:42 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:15:42 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:15:42 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:15:42 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 33x23x7) for time before (2024-12-09 17:00:00)
20:15:42 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-09 17:00:00) in space (linearNDFast)
20:15:42 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:15:42 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:15:42 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.71800508581168 and -65.64631277307059 degrees.
20:15:42 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.71800508581168 and -65.64631277307059 degrees.
20:15:42 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:15:42 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:15:42 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:15:42 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:15:42 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:15:42 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:15:42 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:15:42 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:15:42 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:15:42 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:15:42 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:15:42 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:15:42 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:15:42 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:15:42 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:15:42 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:15:42 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.509633 (min) -0.103359 (max)
20:15:42 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.436623 (min) -0.0739309 (max)
20:15:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.196666 (min) -0.18333 (max)
20:15:42 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0.0575094 (min) 0.256275 (max)
20:15:42 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.90776 (min) -3.85778 (max)
20:15:42 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -2.27928e-05 (min) -1.98179e-05 (max)
20:15:42 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:15:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:15:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:15:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:15:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:15:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:15:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:15:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:15:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:15:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:15:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:15:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:15:42 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:15:42 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:15:42 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:15:42 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:15:42 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:15:42 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 288.994 (min) 296.966 (max)
20:15:42 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:15:42 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:15:42 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.366191, mean: 0.372809, max: 0.377273
20:15:42 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:15:42 DEBUG opendrift.models.physics_methods:1061: min: 3.296730, mean: 3.326382, max: 3.346242
20:15:42 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.296730, mean: 3.326382, max: 3.346242
20:15:42 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:15:42 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:15:42 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:15:42 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:15:42 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.077164 m/s - 0.078323 m/s)
20:15:42 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:15:42 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:15:42 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:15:42 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:15:42 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:15:42 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:15:42 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:15:42 INFO opendrift.models.basemodel:2035: 2024-12-09 18:00:00 - step 19 of 71 - 1000 active elements (0 deactivated)
20:15:42 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:15:42 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:15:42 DEBUG opendrift.models.basemodel:2054: 60.019695460790814 <- latitude -> 60.1034429448826
20:15:42 DEBUG opendrift.models.basemodel:2059: 4.275576944974855 <- longitude -> 4.320787523767518
20:15:42 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:15:42 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:15:42 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:15:42 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:15:42 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:15:42 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:15:42 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:15:42 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:15:42 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:15:42 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-09 18:00:00 (before)
2024-12-09 19:00:00 (after)
20:15:58 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:15:58 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:15:58 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:15:58 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:15:58 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:15:58 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:15:58 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 34x24x7) for time before (2024-12-09 18:00:00)
20:15:58 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-09 18:00:00) in space (linearNDFast)
20:15:58 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:15:58 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:15:58 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.72443398717321 and -65.67922337879419 degrees.
20:15:58 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.72443398717321 and -65.67922337879419 degrees.
20:15:58 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:15:58 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:15:58 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:15:58 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:15:58 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:15:58 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:15:58 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:15:58 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:15:58 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:15:58 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:15:58 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:15:58 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:15:58 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:15:58 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:15:58 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:15:58 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:15:58 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.365616 (min) -0.0505865 (max)
20:15:58 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.439361 (min) -0.256415 (max)
20:15:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.301402 (min) -0.294077 (max)
20:15:58 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0.608526 (min) 0.668137 (max)
20:15:58 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.4376 (min) -3.40617 (max)
20:15:58 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -4.17909e-05 (min) -3.86806e-05 (max)
20:15:58 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:15:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:15:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:15:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:15:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:15:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:15:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:15:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:15:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:15:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:15:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:15:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:15:58 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:15:58 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:15:58 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:15:58 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:15:58 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:15:58 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 288.832 (min) 298.109 (max)
20:15:58 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:15:58 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:15:58 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.295707, mean: 0.296616, max: 0.301655
20:15:58 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:15:58 DEBUG opendrift.models.physics_methods:1061: min: 2.962515, mean: 2.967063, max: 2.992159
20:15:58 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.962515, mean: 2.967063, max: 2.992159
20:15:58 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:15:58 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:15:58 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:15:58 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:15:58 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.069342 m/s - 0.070035 m/s)
20:15:58 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:15:58 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:15:58 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:15:58 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:15:58 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:15:58 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:15:58 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:15:58 INFO opendrift.models.basemodel:2035: 2024-12-09 19:00:00 - step 20 of 71 - 1000 active elements (0 deactivated)
20:15:58 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:15:58 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:15:58 DEBUG opendrift.models.basemodel:2054: 60.00513343455227 <- latitude -> 60.09293505736659
20:15:58 DEBUG opendrift.models.basemodel:2059: 4.273097891955547 <- longitude -> 4.297982581519966
20:15:58 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:15:58 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:15:58 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:15:58 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:15:58 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:15:58 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:15:58 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:15:58 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:15:58 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:15:58 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-09 19:00:00 (before)
2024-12-09 20:00:00 (after)
20:16:16 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:16:16 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:16:16 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:16:16 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:16:16 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:16:16 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:16:16 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 34x25x7) for time before (2024-12-09 19:00:00)
20:16:16 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-09 19:00:00) in space (linearNDFast)
20:16:16 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:16:16 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:16:16 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.72691305078001 and -65.70202833116032 degrees.
20:16:16 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.72691305078001 and -65.70202833116032 degrees.
20:16:16 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:16:16 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:16:16 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:16:16 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:16:16 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:16:16 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:16:16 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:16:16 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:16:16 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:16:16 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:16:16 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:16:16 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:16:16 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:16:16 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:16:16 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:16:16 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:16:16 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.252151 (min) 0.015247 (max)
20:16:16 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.434084 (min) -0.303039 (max)
20:16:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.469314 (min) -0.460141 (max)
20:16:16 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.04555 (min) 1.10492 (max)
20:16:16 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.19084 (min) -3.07594 (max)
20:16:16 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -5.36334e-05 (min) -5.07347e-05 (max)
20:16:16 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:16:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:16:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:16:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:16:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:16:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:16:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:16:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:16:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:16:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:16:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:16:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:16:16 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:16:16 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:16:16 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:16:16 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:16:16 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:16:16 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 290.259 (min) 298.627 (max)
20:16:16 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:16:16 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:16:16 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.261484, mean: 0.267591, max: 0.277356
20:16:16 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:16:16 DEBUG opendrift.models.physics_methods:1061: min: 2.785814, mean: 2.818099, max: 2.869120
20:16:16 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.785814, mean: 2.818099, max: 2.869120
20:16:16 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:16:16 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:16:16 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:16:16 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:16:16 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.065206 m/s - 0.067155 m/s)
20:16:16 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:16:16 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:16:16 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:16:16 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:16:16 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:16:16 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:16:16 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:16:16 INFO opendrift.models.basemodel:2035: 2024-12-09 20:00:00 - step 21 of 71 - 1000 active elements (0 deactivated)
20:16:16 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:16:16 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:16:16 DEBUG opendrift.models.basemodel:2054: 59.99327941885326 <- latitude -> 60.079665509897204
20:16:16 DEBUG opendrift.models.basemodel:2059: 4.273613163141585 <- longitude -> 4.2831036927215616
20:16:16 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:16:16 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:16:16 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:16:16 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:16:16 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:16:16 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:16:16 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:16:16 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:16:16 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:16:16 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-09 20:00:00 (before)
2024-12-09 21:00:00 (after)
20:16:37 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:16:37 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:16:37 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:16:37 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:16:37 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:16:37 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:16:37 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 33x27x7) for time before (2024-12-09 20:00:00)
20:16:37 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-09 20:00:00) in space (linearNDFast)
20:16:37 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:16:37 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:16:37 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.7263977624324 and -65.71690722198349 degrees.
20:16:37 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.7263977624324 and -65.71690722198349 degrees.
20:16:37 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:16:37 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:16:37 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:16:37 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:16:37 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:16:37 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:16:37 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:16:37 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:16:37 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:16:37 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:16:37 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:16:37 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:16:37 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:16:37 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:16:37 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:16:37 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:16:37 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.136505 (min) 0.0818955 (max)
20:16:37 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.404399 (min) -0.218741 (max)
20:16:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.659389 (min) -0.642823 (max)
20:16:37 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.53236 (min) 1.71922 (max)
20:16:37 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.14954 (min) -2.80597 (max)
20:16:37 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -5.12586e-05 (min) -4.8961e-05 (max)
20:16:37 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:16:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:16:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:16:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:16:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:16:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:16:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:16:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:16:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:16:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:16:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:16:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:16:37 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:16:37 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:16:37 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:16:37 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:16:37 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:16:37 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 291.984 (min) 298.663 (max)
20:16:37 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:16:37 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:16:37 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.266397, mean: 0.287038, max: 0.301786
20:16:37 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:16:37 DEBUG opendrift.models.physics_methods:1061: min: 2.811864, mean: 2.918637, max: 2.992810
20:16:37 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.811864, mean: 2.918637, max: 2.992810
20:16:37 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:16:37 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:16:37 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:16:37 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:16:37 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.065815 m/s - 0.070051 m/s)
20:16:37 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:16:37 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:16:37 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:16:37 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:16:37 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:16:37 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:16:37 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:16:37 INFO opendrift.models.basemodel:2035: 2024-12-09 21:00:00 - step 22 of 71 - 1000 active elements (0 deactivated)
20:16:37 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:16:37 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:16:37 DEBUG opendrift.models.basemodel:2054: 59.98417584043732 <- latitude -> 60.06559626083046
20:16:37 DEBUG opendrift.models.basemodel:2059: 4.275370004591793 <- longitude -> 4.282687935188694
20:16:37 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:16:37 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:16:37 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:16:37 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:16:37 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:16:37 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:16:37 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:16:37 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:16:37 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:16:37 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-09 21:00:00 (before)
2024-12-09 22:00:00 (after)
20:16:53 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:16:53 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:16:53 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:16:53 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:16:53 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:16:53 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:16:53 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 32x27x7) for time before (2024-12-09 21:00:00)
20:16:53 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-09 21:00:00) in space (linearNDFast)
20:16:53 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:16:53 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:16:53 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.72464093325453 and -65.71732303038976 degrees.
20:16:53 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.72464093325453 and -65.71732303038976 degrees.
20:16:53 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:16:53 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:16:53 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:16:53 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:16:53 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:16:53 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:16:53 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:16:53 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:16:53 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:16:53 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:16:53 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:16:53 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:16:53 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:16:53 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:16:53 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:16:53 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:16:53 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0139631 (min) 0.140172 (max)
20:16:53 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.366237 (min) -0.142342 (max)
20:16:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.820714 (min) -0.797607 (max)
20:16:53 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.93749 (min) 2.03135 (max)
20:16:53 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.14737 (min) -2.60954 (max)
20:16:53 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -3.70092e-05 (min) -3.54072e-05 (max)
20:16:53 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:16:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:16:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:16:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:16:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:16:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:16:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:16:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:16:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:16:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:16:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:16:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:16:53 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:16:53 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:16:53 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:16:53 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:16:53 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:16:53 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 293.625 (min) 298.675 (max)
20:16:53 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:16:53 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:16:53 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.259863, mean: 0.317184, max: 0.345068
20:16:53 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:16:53 DEBUG opendrift.models.physics_methods:1061: min: 2.777167, mean: 3.067641, max: 3.200235
20:16:53 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.777167, mean: 3.067641, max: 3.200235
20:16:53 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:16:53 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:16:53 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:16:53 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:16:53 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.065003 m/s - 0.074906 m/s)
20:16:53 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:16:53 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:16:53 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:16:53 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:16:53 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:16:53 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:16:53 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:16:53 INFO opendrift.models.basemodel:2035: 2024-12-09 22:00:00 - step 23 of 71 - 1000 active elements (0 deactivated)
20:16:53 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:16:53 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:16:53 DEBUG opendrift.models.basemodel:2054: 59.977542102220404 <- latitude -> 60.0525799425688
20:16:53 DEBUG opendrift.models.basemodel:2059: 4.278103343244821 <- longitude -> 4.294343382144437
20:16:53 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:16:53 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:16:53 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:16:53 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:16:53 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:16:53 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:16:53 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:16:53 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:16:53 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:16:53 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-09 22:00:00 (before)
2024-12-09 23:00:00 (after)
20:17:13 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:17:13 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:17:13 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:17:13 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:17:13 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:17:13 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:17:13 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 31x27x7) for time before (2024-12-09 22:00:00)
20:17:13 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-09 22:00:00) in space (linearNDFast)
20:17:13 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:17:13 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:17:13 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.72190758264487 and -65.7056675601461 degrees.
20:17:13 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.72190758264487 and -65.7056675601461 degrees.
20:17:13 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:17:13 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:17:13 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:17:13 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:17:13 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:17:13 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:17:13 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:17:13 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:17:13 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:17:13 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:17:13 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:17:13 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:17:13 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:17:13 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:17:13 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:17:13 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:17:13 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0900059 (min) 0.172615 (max)
20:17:13 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.320323 (min) -0.0458037 (max)
20:17:13 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.909422 (min) -0.882194 (max)
20:17:13 DEBUG opendrift.models.basemodel.environment:905: x_wind: 2.01209 (min) 2.19797 (max)
20:17:13 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.72774 (min) -2.50868 (max)
20:17:13 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -1.20299e-05 (min) -1.10979e-05 (max)
20:17:13 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:17:13 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:17:13 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:17:13 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:17:13 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:17:13 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:17:13 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:17:13 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:17:13 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:17:13 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:17:13 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:17:13 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:17:13 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:17:13 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:17:13 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:17:13 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:17:13 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:17:13 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 295.116 (min) 298.694 (max)
20:17:13 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:17:13 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:17:13 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.273664, mean: 0.280858, max: 0.282631
20:17:13 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:17:13 DEBUG opendrift.models.physics_methods:1061: min: 2.849957, mean: 2.887173, max: 2.896273
20:17:13 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.849957, mean: 2.887173, max: 2.896273
20:17:13 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:17:13 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:17:13 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:17:13 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:17:13 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.066707 m/s - 0.067791 m/s)
20:17:13 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:17:13 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:17:13 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:17:13 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:17:13 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:17:13 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:17:13 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:17:13 INFO opendrift.models.basemodel:2035: 2024-12-09 23:00:00 - step 24 of 71 - 1000 active elements (0 deactivated)
20:17:13 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:17:13 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:17:13 DEBUG opendrift.models.basemodel:2054: 59.974298769127074 <- latitude -> 60.040768753316485
20:17:13 DEBUG opendrift.models.basemodel:2059: 4.286757204021537 <- longitude -> 4.308066017242352
20:17:13 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:17:13 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:17:13 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:17:13 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:17:13 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:17:13 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:17:13 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:17:13 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:17:13 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:17:13 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-09 23:00:00 (before)
2024-12-10 00:00:00 (after)
20:17:30 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:17:30 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:17:30 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:17:30 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:17:30 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:17:30 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:17:30 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 30x27x7) for time before (2024-12-09 23:00:00)
20:17:30 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-09 23:00:00) in space (linearNDFast)
20:17:30 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:17:30 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:17:30 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.71325372210507 and -65.6919449293655 degrees.
20:17:30 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.71325372210507 and -65.6919449293655 degrees.
20:17:30 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:17:30 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:17:30 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:17:30 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:17:30 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:17:30 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:17:30 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:17:30 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:17:30 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:17:30 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:17:30 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:17:30 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:17:30 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:17:30 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:17:30 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:17:30 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:17:30 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.130225 (min) 0.174327 (max)
20:17:30 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.257371 (min) 0.0677776 (max)
20:17:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.901312 (min) -0.873856 (max)
20:17:30 DEBUG opendrift.models.basemodel.environment:905: x_wind: 2.18691 (min) 2.36558 (max)
20:17:30 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.49071 (min) -2.11509 (max)
20:17:30 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 1.37792e-05 (min) 1.46503e-05 (max)
20:17:30 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:17:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:17:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:17:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:17:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:17:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:17:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:17:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:17:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:17:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:17:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:17:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:17:30 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:17:30 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:17:30 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:17:30 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:17:30 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:17:30 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 296.518 (min) 298.728 (max)
20:17:30 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:17:30 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:17:30 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.247712, mean: 0.259007, max: 0.270260
20:17:30 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:17:30 DEBUG opendrift.models.physics_methods:1061: min: 2.711458, mean: 2.772530, max: 2.832179
20:17:30 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.711458, mean: 2.772530, max: 2.832179
20:17:30 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:17:30 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:17:30 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:17:30 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:17:30 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.063465 m/s - 0.066291 m/s)
20:17:30 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:17:30 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:17:30 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:17:30 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:17:30 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:17:30 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:17:30 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:17:30 INFO opendrift.models.basemodel:2035: 2024-12-10 00:00:00 - step 25 of 71 - 1000 active elements (0 deactivated)
20:17:30 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:17:30 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:17:30 DEBUG opendrift.models.basemodel:2054: 59.974878705325665 <- latitude -> 60.03108528301439
20:17:30 DEBUG opendrift.models.basemodel:2059: 4.299097419999761 <- longitude -> 4.32212461088948
20:17:30 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:17:30 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:17:30 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:17:30 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:17:30 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:17:30 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:17:30 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:17:30 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:17:30 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:17:30 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-10 00:00:00 (before)
2024-12-10 01:00:00 (after)
20:17:50 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:17:50 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:17:50 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:17:50 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:17:50 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:17:50 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:17:50 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 28x27x7) for time before (2024-12-10 00:00:00)
20:17:50 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-10 00:00:00) in space (linearNDFast)
20:17:50 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:17:50 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:17:50 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.7009135022708 and -65.67788633381994 degrees.
20:17:50 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.7009135022708 and -65.67788633381994 degrees.
20:17:50 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:17:50 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:17:50 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:17:50 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:17:50 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:17:50 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:17:50 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:17:50 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:17:50 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:17:50 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:17:50 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:17:50 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:17:50 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:17:50 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:17:50 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:17:50 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:17:50 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.124033 (min) 0.226261 (max)
20:17:50 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.177946 (min) 0.214245 (max)
20:17:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.813544 (min) -0.789659 (max)
20:17:50 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.84841 (min) 2.16592 (max)
20:17:50 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.3419 (min) -1.68622 (max)
20:17:50 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -4.25239e-07 (min) 3.10015e-07 (max)
20:17:50 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:17:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:17:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:17:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:17:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:17:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:17:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:17:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:17:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:17:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:17:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:17:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:17:50 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:17:50 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:17:50 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:17:50 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:17:50 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:17:50 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 296.738 (min) 298.75 (max)
20:17:50 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:17:50 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:17:50 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.153994, mean: 0.219858, max: 0.250323
20:17:50 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:17:50 DEBUG opendrift.models.physics_methods:1061: min: 2.137873, mean: 2.553486, max: 2.725711
20:17:50 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.137873, mean: 2.553486, max: 2.725711
20:17:50 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:17:50 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:17:50 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:17:50 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:17:50 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.050040 m/s - 0.063799 m/s)
20:17:50 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:17:50 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:17:50 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:17:50 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:17:50 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:17:50 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:17:50 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:17:50 INFO opendrift.models.basemodel:2035: 2024-12-10 01:00:00 - step 26 of 71 - 1000 active elements (0 deactivated)
20:17:50 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:17:50 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:17:50 DEBUG opendrift.models.basemodel:2054: 59.9793511592338 <- latitude -> 60.02424535563048
20:17:50 DEBUG opendrift.models.basemodel:2059: 4.311108233717329 <- longitude -> 4.339507128058975
20:17:50 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:17:50 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:17:50 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:17:50 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:17:50 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:17:50 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:17:50 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:17:50 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:17:50 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:17:50 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-10 01:00:00 (before)
2024-12-10 02:00:00 (after)
20:18:09 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:18:09 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:18:09 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:18:09 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:18:09 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:18:09 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:18:09 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 28x27x7) for time before (2024-12-10 01:00:00)
20:18:09 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-10 01:00:00) in space (linearNDFast)
20:18:09 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:18:09 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:18:09 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.68890269298761 and -65.6605038188033 degrees.
20:18:09 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.68890269298761 and -65.6605038188033 degrees.
20:18:09 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:18:09 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:18:09 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:18:09 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:18:09 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:18:09 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:18:09 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:18:09 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:18:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:18:09 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:18:09 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:18:09 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:18:09 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:18:09 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:18:09 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:18:09 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:18:09 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.114802 (min) 0.307224 (max)
20:18:09 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.114516 (min) 0.324113 (max)
20:18:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.678351 (min) -0.660441 (max)
20:18:09 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.61089 (min) 1.90993 (max)
20:18:09 DEBUG opendrift.models.basemodel.environment:905: y_wind: -0.970712 (min) -0.73051 (max)
20:18:09 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 3.77604e-05 (min) 4.01355e-05 (max)
20:18:09 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:18:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:18:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:18:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:18:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:18:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:18:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:18:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:18:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:18:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:18:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:18:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:18:09 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:18:09 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:18:09 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:18:09 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:18:09 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:18:09 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 297.196 (min) 298.803 (max)
20:18:09 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:18:09 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:18:09 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.087016, mean: 0.092604, max: 0.102864
20:18:09 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:18:09 DEBUG opendrift.models.physics_methods:1061: min: 1.607049, mean: 1.657745, max: 1.747276
20:18:09 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.607049, mean: 1.657745, max: 1.747276
20:18:09 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:18:09 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:18:09 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:18:09 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:18:09 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.037615 m/s - 0.040897 m/s)
20:18:09 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:18:09 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:18:09 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:18:09 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:18:09 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:18:09 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:18:09 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:18:09 INFO opendrift.models.basemodel:2035: 2024-12-10 02:00:00 - step 27 of 71 - 1000 active elements (0 deactivated)
20:18:09 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:18:09 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:18:09 DEBUG opendrift.models.basemodel:2054: 59.98680535612286 <- latitude -> 60.02007265364336
20:18:09 DEBUG opendrift.models.basemodel:2059: 4.322807863960096 <- longitude -> 4.361400479757478
20:18:09 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:18:09 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:18:09 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:18:09 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:18:09 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:18:09 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:18:09 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:18:09 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:18:09 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:18:09 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-10 02:00:00 (before)
2024-12-10 03:00:00 (after)
20:18:26 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:18:26 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:18:26 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:18:26 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:18:26 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:18:26 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:18:26 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 26x27x7) for time before (2024-12-10 02:00:00)
20:18:26 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-10 02:00:00) in space (linearNDFast)
20:18:26 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:18:26 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:18:26 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.67720306491766 and -65.63861046429945 degrees.
20:18:26 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.67720306491766 and -65.63861046429945 degrees.
20:18:26 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:18:26 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:18:26 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:18:26 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:18:26 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:18:26 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:18:26 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:18:26 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:18:26 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:18:26 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:18:26 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:18:26 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:18:26 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:18:26 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:18:26 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:18:26 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:18:26 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.123399 (min) 0.345228 (max)
20:18:26 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0288631 (min) 0.354477 (max)
20:18:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.534909 (min) -0.52137 (max)
20:18:26 DEBUG opendrift.models.basemodel.environment:905: x_wind: 3.04105 (min) 3.52605 (max)
20:18:26 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.48737 (min) -2.13674 (max)
20:18:26 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 6.0152e-05 (min) 6.21826e-05 (max)
20:18:26 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:18:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:18:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:18:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:18:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:18:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:18:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:18:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:18:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:18:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:18:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:18:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:18:26 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:18:26 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:18:26 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:18:26 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:18:26 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:18:26 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 297.802 (min) 298.818 (max)
20:18:26 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:18:26 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:18:26 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.339815, mean: 0.408599, max: 0.455902
20:18:26 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:18:26 DEBUG opendrift.models.physics_methods:1061: min: 3.175784, mean: 3.481446, max: 3.678453
20:18:26 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.175784, mean: 3.481446, max: 3.678453
20:18:26 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:18:26 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:18:26 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:18:26 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:18:26 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.074333 m/s - 0.086099 m/s)
20:18:26 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:18:26 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:18:26 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:18:26 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:18:26 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:18:26 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:18:26 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:18:26 INFO opendrift.models.basemodel:2035: 2024-12-10 03:00:00 - step 28 of 71 - 1000 active elements (0 deactivated)
20:18:26 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:18:26 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:18:26 DEBUG opendrift.models.basemodel:2054: 59.99043994739135 <- latitude -> 60.017543634878606
20:18:26 DEBUG opendrift.models.basemodel:2059: 4.3355184215861975 <- longitude -> 4.387598285663547
20:18:26 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:18:26 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:18:26 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:18:26 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:18:26 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:18:26 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:18:26 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:18:26 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:18:26 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:18:26 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-10 03:00:00 (before)
2024-12-10 04:00:00 (after)
20:18:38 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:18:38 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:18:38 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:18:38 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:18:38 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:18:38 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:18:38 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 25x27x7) for time before (2024-12-10 03:00:00)
20:18:38 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-10 03:00:00) in space (linearNDFast)
20:18:38 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:18:38 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:18:38 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.66449251221015 and -65.61241264538614 degrees.
20:18:38 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.66449251221015 and -65.61241264538614 degrees.
20:18:38 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:18:38 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:18:38 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:18:38 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:18:38 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:18:38 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:18:38 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:18:38 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:18:38 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:18:38 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:18:38 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:18:38 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:18:38 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:18:38 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:18:38 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:18:38 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:18:38 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.1342 (min) 0.386588 (max)
20:18:38 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.00270481 (min) 0.382203 (max)
20:18:38 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.396113 (min) -0.383951 (max)
20:18:38 DEBUG opendrift.models.basemodel.environment:905: x_wind: 3.08978 (min) 3.29803 (max)
20:18:38 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.08992 (min) -2.9539 (max)
20:18:38 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 3.01918e-05 (min) 3.50487e-05 (max)
20:18:38 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:18:38 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:18:38 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:18:38 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:18:38 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:18:38 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:18:38 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:18:38 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:18:38 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:18:38 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:18:38 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:18:38 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:18:38 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:18:38 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:18:38 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:18:38 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:18:38 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:18:38 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.062 (min) 298.852 (max)
20:18:38 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:18:38 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:18:38 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.461545, mean: 0.479489, max: 0.484699
20:18:38 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:18:38 DEBUG opendrift.models.physics_methods:1061: min: 3.701150, mean: 3.772377, max: 3.792850
20:18:38 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.701150, mean: 3.772377, max: 3.792850
20:18:38 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:18:38 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:18:38 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:18:38 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:18:38 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.086630 m/s - 0.088777 m/s)
20:18:38 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:18:38 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:18:38 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:18:38 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:18:38 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:18:38 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:18:38 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:18:38 INFO opendrift.models.basemodel:2035: 2024-12-10 04:00:00 - step 29 of 71 - 1000 active elements (0 deactivated)
20:18:38 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:18:38 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:18:38 DEBUG opendrift.models.basemodel:2054: 59.99424741822976 <- latitude -> 60.015721692082984
20:18:38 DEBUG opendrift.models.basemodel:2059: 4.348996889215472 <- longitude -> 4.416536988947098
20:18:38 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:18:38 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:18:38 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:18:38 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:18:38 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:18:38 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:18:38 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:18:38 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:18:38 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:18:38 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-10 04:00:00 (before)
2024-12-10 05:00:00 (after)
20:18:52 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:18:52 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:18:52 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:18:52 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:18:52 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:18:52 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:18:52 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 26x27x7) for time before (2024-12-10 04:00:00)
20:18:52 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-10 04:00:00) in space (linearNDFast)
20:18:52 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:18:52 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:18:52 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.65101404285664 and -65.58347393719373 degrees.
20:18:52 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.65101404285664 and -65.58347393719373 degrees.
20:18:52 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:18:52 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:18:52 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:18:52 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:18:52 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:18:52 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:18:52 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:18:52 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:18:52 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:18:52 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:18:52 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:18:52 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:18:52 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:18:52 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:18:52 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:18:52 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:18:52 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.123905 (min) 0.409918 (max)
20:18:52 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0147759 (min) 0.386383 (max)
20:18:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.296308 (min) -0.284338 (max)
20:18:52 DEBUG opendrift.models.basemodel.environment:905: x_wind: 2.28006 (min) 2.651 (max)
20:18:52 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.61629 (min) -2.47296 (max)
20:18:52 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 1.16455e-05 (min) 1.38074e-05 (max)
20:18:52 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:18:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:18:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:18:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:18:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:18:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:18:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:18:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:18:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:18:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:18:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:18:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:18:52 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:18:52 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:18:52 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:18:52 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:18:52 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:18:52 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.264 (min) 298.86 (max)
20:18:52 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:18:52 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:18:52 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.278329, mean: 0.325814, max: 0.339030
20:18:52 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:18:52 DEBUG opendrift.models.physics_methods:1061: min: 2.874147, mean: 3.108919, max: 3.172114
20:18:52 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.874147, mean: 3.108919, max: 3.172114
20:18:52 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:18:52 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:18:52 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:18:52 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:18:52 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.067273 m/s - 0.074247 m/s)
20:18:52 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:18:52 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:18:52 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:18:52 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:18:52 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:18:52 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:18:52 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:18:52 INFO opendrift.models.basemodel:2035: 2024-12-10 05:00:00 - step 30 of 71 - 1000 active elements (0 deactivated)
20:18:52 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:18:52 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:18:52 DEBUG opendrift.models.basemodel:2054: 59.99981548248573 <- latitude -> 60.02147041994341
20:18:52 DEBUG opendrift.models.basemodel:2059: 4.3615234658409605 <- longitude -> 4.445945656802899
20:18:52 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:18:52 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:18:52 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:18:52 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:18:52 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:18:52 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:18:52 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:18:52 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:18:52 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:18:52 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-10 05:00:00 (before)
2024-12-10 06:00:00 (after)
20:19:04 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:19:04 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:19:04 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:19:04 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:19:04 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:19:04 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:19:04 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 27x27x7) for time before (2024-12-10 05:00:00)
20:19:04 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-10 05:00:00) in space (linearNDFast)
20:19:04 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:19:04 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:19:04 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.63848747596046 and -65.55406526034983 degrees.
20:19:04 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.63848747596046 and -65.55406526034983 degrees.
20:19:04 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:19:04 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:19:04 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:19:04 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:19:04 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:19:04 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:19:04 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:19:04 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:19:04 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:19:04 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:19:04 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:19:04 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:19:04 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:19:04 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:19:04 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:19:04 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:19:04 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.117139 (min) 0.417376 (max)
20:19:04 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.032908 (min) 0.360615 (max)
20:19:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.249652 (min) -0.237356 (max)
20:19:04 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.93392 (min) 2.1091 (max)
20:19:04 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.21253 (min) -1.77501 (max)
20:19:04 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 9.7704e-07 (min) 1.99527e-06 (max)
20:19:04 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:19:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:19:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:19:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:19:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:19:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:19:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:19:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:19:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:19:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:19:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:19:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:19:04 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:19:04 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:19:04 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:19:04 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:19:04 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:19:04 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.242 (min) 298.839 (max)
20:19:04 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:19:04 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:19:04 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.183527, mean: 0.196418, max: 0.229853
20:19:04 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:19:04 DEBUG opendrift.models.physics_methods:1061: min: 2.333888, mean: 2.414254, max: 2.611887
20:19:04 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.333888, mean: 2.414254, max: 2.611887
20:19:04 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:19:04 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:19:04 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:19:04 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:19:04 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.054628 m/s - 0.061135 m/s)
20:19:04 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:19:04 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:19:04 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:19:04 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:19:04 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:19:04 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:19:04 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:19:04 INFO opendrift.models.basemodel:2035: 2024-12-10 06:00:00 - step 31 of 71 - 1000 active elements (0 deactivated)
20:19:04 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:19:04 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:19:04 DEBUG opendrift.models.basemodel:2054: 60.005708886695665 <- latitude -> 60.031585672314066
20:19:04 DEBUG opendrift.models.basemodel:2059: 4.37219928164921 <- longitude -> 4.4756240161557965
20:19:04 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:19:04 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:19:04 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:19:04 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:19:04 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:19:04 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:19:04 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:19:04 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:19:04 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:19:04 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-10 06:00:00 (before)
2024-12-10 07:00:00 (after)
20:19:17 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:19:17 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:19:17 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:19:17 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:19:17 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:19:17 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:19:17 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 28x27x7) for time before (2024-12-10 06:00:00)
20:19:17 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-10 06:00:00) in space (linearNDFast)
20:19:17 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:19:17 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:19:17 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.62781164938293 and -65.5243868992785 degrees.
20:19:17 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.62781164938293 and -65.5243868992785 degrees.
20:19:17 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:19:17 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:19:17 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:19:17 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:19:17 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:19:17 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:19:17 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:19:17 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:19:17 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:19:17 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:19:17 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:19:17 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:19:17 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:19:17 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:19:17 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:19:17 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:19:17 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.126458 (min) 0.415877 (max)
20:19:17 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0751941 (min) 0.321115 (max)
20:19:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.276541 (min) -0.264006 (max)
20:19:17 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.94167 (min) 2.14933 (max)
20:19:17 DEBUG opendrift.models.basemodel.environment:905: y_wind: -1.13646 (min) -0.731193 (max)
20:19:17 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -1.58763e-05 (min) -1.28124e-05 (max)
20:19:17 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:19:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:19:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:19:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:19:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:19:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:19:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:19:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:19:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:19:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:19:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:19:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:19:17 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:19:17 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:19:17 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:19:17 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:19:17 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:19:17 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.239 (min) 298.79 (max)
20:19:17 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:19:17 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:19:17 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.105896, mean: 0.127868, max: 0.145414
20:19:17 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:19:17 DEBUG opendrift.models.physics_methods:1061: min: 1.772841, mean: 1.947653, max: 2.077463
20:19:17 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.772841, mean: 1.947653, max: 2.077463
20:19:17 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:19:17 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:19:17 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:19:17 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:19:17 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.041496 m/s - 0.048626 m/s)
20:19:17 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:19:17 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:19:17 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:19:17 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:19:17 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:19:17 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:19:17 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:19:17 INFO opendrift.models.basemodel:2035: 2024-12-10 07:00:00 - step 32 of 71 - 1000 active elements (0 deactivated)
20:19:17 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:19:17 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:19:17 DEBUG opendrift.models.basemodel:2054: 60.012168069293814 <- latitude -> 60.041068949328576
20:19:17 DEBUG opendrift.models.basemodel:2059: 4.3828686543724915 <- longitude -> 4.50518606370071
20:19:17 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:19:17 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:19:17 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:19:17 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:19:17 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:19:17 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:19:17 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:19:17 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:19:17 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:19:17 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-10 07:00:00 (before)
2024-12-10 08:00:00 (after)
20:19:29 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:19:29 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:19:29 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:19:29 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:19:29 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:19:29 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:19:29 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 29x29x7) for time before (2024-12-10 07:00:00)
20:19:29 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-10 07:00:00) in space (linearNDFast)
20:19:29 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:19:29 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:19:29 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.61714226842405 and -65.49482484702143 degrees.
20:19:29 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.61714226842405 and -65.49482484702143 degrees.
20:19:29 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:19:29 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:19:29 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:19:29 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:19:29 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:19:29 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:19:29 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:19:29 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:19:29 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:19:29 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:19:29 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:19:29 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:19:29 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:19:29 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:19:29 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:19:29 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:19:29 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.144221 (min) 0.39086 (max)
20:19:29 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.107455 (min) 0.360086 (max)
20:19:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.367257 (min) -0.341688 (max)
20:19:29 DEBUG opendrift.models.basemodel.environment:905: x_wind: 2.51725 (min) 2.93827 (max)
20:19:29 DEBUG opendrift.models.basemodel.environment:905: y_wind: -0.998838 (min) -0.816655 (max)
20:19:29 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -3.62938e-05 (min) -3.29528e-05 (max)
20:19:29 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:19:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:19:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:19:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:19:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:19:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:19:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:19:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:19:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:19:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:19:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:19:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:19:29 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:19:29 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:19:29 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:19:29 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:19:29 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:19:29 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.235 (min) 299.157 (max)
20:19:29 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:19:29 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:19:29 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.180422, mean: 0.203745, max: 0.231021
20:19:29 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:19:29 DEBUG opendrift.models.physics_methods:1061: min: 2.314061, mean: 2.458793, max: 2.618519
20:19:29 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.314061, mean: 2.458793, max: 2.618519
20:19:29 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:19:29 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:19:29 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:19:29 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:19:29 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.054164 m/s - 0.061290 m/s)
20:19:29 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:19:29 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:19:29 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:19:29 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:19:29 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:19:29 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:19:29 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:19:29 INFO opendrift.models.basemodel:2035: 2024-12-10 08:00:00 - step 33 of 71 - 1000 active elements (0 deactivated)
20:19:29 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:19:29 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:19:29 DEBUG opendrift.models.basemodel:2054: 60.01802827350505 <- latitude -> 60.05205816370942
20:19:29 DEBUG opendrift.models.basemodel:2059: 4.39597233990864 <- longitude -> 4.518810283004871
20:19:29 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:19:29 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:19:29 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:19:29 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:19:29 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:19:29 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:19:29 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:19:29 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:19:29 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:19:29 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-10 08:00:00 (before)
2024-12-10 09:00:00 (after)
20:19:42 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:19:42 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:19:42 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:19:42 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:19:42 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:19:42 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:19:42 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 30x28x7) for time before (2024-12-10 08:00:00)
20:19:42 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-10 08:00:00) in space (linearNDFast)
20:19:42 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:19:42 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:19:42 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.60403859405187 and -65.481200627268 degrees.
20:19:42 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.60403859405187 and -65.481200627268 degrees.
20:19:42 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:19:42 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:19:42 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:19:42 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:19:42 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:19:42 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:19:42 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:19:42 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:19:42 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:19:42 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:19:42 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:19:42 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:19:42 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:19:42 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:19:42 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:19:42 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:19:42 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.149453 (min) 0.363515 (max)
20:19:42 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.140621 (min) 0.43446 (max)
20:19:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.519666 (min) -0.496805 (max)
20:19:42 DEBUG opendrift.models.basemodel.environment:905: x_wind: 3.99085 (min) 4.2612 (max)
20:19:42 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.10288 (min) -2.00474 (max)
20:19:42 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -4.53861e-05 (min) -4.15196e-05 (max)
20:19:42 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:19:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:19:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:19:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:19:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:19:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:19:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:19:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:19:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:19:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:19:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:19:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:19:42 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:19:42 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:19:42 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:19:42 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:19:42 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:19:42 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.227 (min) 299.379 (max)
20:19:42 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:19:42 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:19:42 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.500344, mean: 0.532257, max: 0.545973
20:19:42 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:19:42 DEBUG opendrift.models.physics_methods:1061: min: 3.853578, mean: 3.974378, max: 4.025458
20:19:42 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.853578, mean: 3.974378, max: 4.025458
20:19:42 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:19:42 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:19:42 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:19:42 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:19:42 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.090198 m/s - 0.094221 m/s)
20:19:42 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:19:42 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:19:42 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:19:42 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:19:42 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:19:42 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:19:42 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:19:42 INFO opendrift.models.basemodel:2035: 2024-12-10 09:00:00 - step 34 of 71 - 1000 active elements (0 deactivated)
20:19:42 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:19:42 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:19:42 DEBUG opendrift.models.basemodel:2054: 60.02255868558001 <- latitude -> 60.06470890564614
20:19:42 DEBUG opendrift.models.basemodel:2059: 4.414313789873339 <- longitude -> 4.533631274781601
20:19:42 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:19:42 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:19:42 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:19:42 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:19:42 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:19:42 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:19:42 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:19:42 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:19:42 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:19:42 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-10 09:00:00 (before)
2024-12-10 10:00:00 (after)
20:19:55 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:19:55 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:19:55 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:19:55 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:19:55 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:19:55 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:19:55 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 31x27x7) for time before (2024-12-10 09:00:00)
20:19:55 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-10 09:00:00) in space (linearNDFast)
20:19:55 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:19:55 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:19:55 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.58569712757637 and -65.46637961898219 degrees.
20:19:55 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.58569712757637 and -65.46637961898219 degrees.
20:19:55 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:19:55 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:19:55 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:19:55 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:19:55 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:19:55 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:19:55 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:19:55 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:19:55 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:19:55 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:19:55 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:19:55 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:19:55 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:19:55 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:19:55 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:19:55 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:19:55 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0794902 (min) 0.325051 (max)
20:19:55 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.230286 (min) 0.493686 (max)
20:19:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.670837 (min) -0.650926 (max)
20:19:55 DEBUG opendrift.models.basemodel.environment:905: x_wind: 4.30638 (min) 4.41469 (max)
20:19:55 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.51468 (min) -2.37602 (max)
20:19:55 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -4.24072e-05 (min) -3.95573e-05 (max)
20:19:55 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:19:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:19:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:19:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:19:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:19:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:19:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:19:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:19:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:19:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:19:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:19:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:19:55 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:19:55 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:19:55 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:19:55 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:19:55 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:19:55 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.22 (min) 299.637 (max)
20:19:55 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:19:55 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:19:55 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.595084, mean: 0.623844, max: 0.630336
20:19:55 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:19:55 DEBUG opendrift.models.physics_methods:1061: min: 4.202605, mean: 4.302954, max: 4.325293
20:19:55 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 4.202605, mean: 4.302954, max: 4.325293
20:19:55 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:19:55 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:19:55 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:19:55 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:19:55 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.098367 m/s - 0.101239 m/s)
20:19:55 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:19:55 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:19:55 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:19:55 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:19:55 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:19:55 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:19:55 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:19:55 INFO opendrift.models.basemodel:2035: 2024-12-10 10:00:00 - step 35 of 71 - 1000 active elements (0 deactivated)
20:19:55 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:19:55 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:19:55 DEBUG opendrift.models.basemodel:2054: 60.02846307734788 <- latitude -> 60.07863792043379
20:19:55 DEBUG opendrift.models.basemodel:2059: 4.43651642149999 <- longitude -> 4.544402541384059
20:19:55 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:19:55 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:19:55 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:19:55 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:19:55 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:19:55 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:19:55 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:19:55 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:19:55 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:19:55 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-10 10:00:00 (before)
2024-12-10 11:00:00 (after)
20:20:08 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:20:08 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:20:08 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:20:08 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:20:08 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:20:08 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:20:08 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 31x26x7) for time before (2024-12-10 10:00:00)
20:20:08 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-10 10:00:00) in space (linearNDFast)
20:20:08 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:20:08 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:20:08 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.56349451054544 and -65.45560836431413 degrees.
20:20:08 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.56349451054544 and -65.45560836431413 degrees.
20:20:08 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:20:08 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:20:08 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:20:08 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:20:08 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:20:08 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:20:08 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:20:08 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:20:08 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:20:08 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:20:08 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:20:08 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:20:08 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:20:08 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:20:08 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:20:08 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:20:08 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.00397832 (min) 0.325076 (max)
20:20:08 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.428211 (min) 0.527728 (max)
20:20:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.766231 (min) -0.75199 (max)
20:20:08 DEBUG opendrift.models.basemodel.environment:905: x_wind: 3.21631 (min) 3.41231 (max)
20:20:08 DEBUG opendrift.models.basemodel.environment:905: y_wind: -1.90683 (min) -1.53569 (max)
20:20:08 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -2.2486e-05 (min) -1.99962e-05 (max)
20:20:08 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:20:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:20:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:20:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:20:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:20:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:20:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:20:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:20:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:20:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:20:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:20:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:20:08 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:20:08 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:20:08 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:20:08 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:20:08 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:20:08 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.188 (min) 299.846 (max)
20:20:08 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:20:08 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:20:08 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.328029, mean: 0.334757, max: 0.361352
20:20:08 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:20:08 DEBUG opendrift.models.physics_methods:1061: min: 3.120222, mean: 3.151887, max: 3.274878
20:20:08 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.120222, mean: 3.151887, max: 3.274878
20:20:08 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:20:08 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:20:08 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:20:08 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:20:08 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.073033 m/s - 0.076653 m/s)
20:20:08 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:20:08 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:20:08 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:20:08 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:20:08 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:20:08 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:20:08 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:20:08 INFO opendrift.models.basemodel:2035: 2024-12-10 11:00:00 - step 36 of 71 - 1000 active elements (0 deactivated)
20:20:08 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:20:08 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:20:08 DEBUG opendrift.models.basemodel:2054: 60.04130597584144 <- latitude -> 60.0939784617543
20:20:08 DEBUG opendrift.models.basemodel:2059: 4.457620355772076 <- longitude -> 4.550969082091244
20:20:08 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:20:08 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:20:08 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:20:08 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:20:08 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:20:08 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:20:08 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:20:08 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:20:08 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:20:08 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-10 11:00:00 (before)
2024-12-10 12:00:00 (after)
20:20:22 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:20:22 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:20:22 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:20:22 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:20:22 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:20:22 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:20:22 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 31x25x7) for time before (2024-12-10 11:00:00)
20:20:22 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-10 11:00:00) in space (linearNDFast)
20:20:22 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:20:22 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:20:22 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.5423905756832 and -65.44904180593517 degrees.
20:20:22 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.5423905756832 and -65.44904180593517 degrees.
20:20:22 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:20:22 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:20:22 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:20:22 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:20:22 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:20:22 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:20:22 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:20:22 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:20:22 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:20:22 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:20:22 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:20:22 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:20:22 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:20:22 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:20:22 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:20:22 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:20:22 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0984531 (min) 0.384307 (max)
20:20:22 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.486115 (min) 0.543302 (max)
20:20:22 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.789716 (min) -0.768426 (max)
20:20:22 DEBUG opendrift.models.basemodel.environment:905: x_wind: 3.71297 (min) 3.91695 (max)
20:20:22 DEBUG opendrift.models.basemodel.environment:905: y_wind: -1.54822 (min) -1.34348 (max)
20:20:22 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 4.89487e-06 (min) 7.93408e-06 (max)
20:20:22 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:20:22 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:20:22 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:20:22 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:20:22 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:20:22 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:20:22 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:20:22 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:20:22 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:20:22 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:20:22 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:20:22 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:20:22 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:20:22 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:20:22 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:20:22 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:20:22 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:20:22 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.135 (min) 299.951 (max)
20:20:22 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:20:22 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:20:22 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.385772, mean: 0.412818, max: 0.434608
20:20:22 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:20:22 DEBUG opendrift.models.physics_methods:1061: min: 3.383724, mean: 3.499767, max: 3.591520
20:20:22 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.383724, mean: 3.499767, max: 3.591520
20:20:22 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:20:22 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:20:22 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:20:22 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:20:22 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.079200 m/s - 0.084064 m/s)
20:20:22 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:20:22 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:20:22 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:20:22 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:20:22 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:20:22 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:20:22 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:20:22 INFO opendrift.models.basemodel:2035: 2024-12-10 12:00:00 - step 37 of 71 - 1000 active elements (0 deactivated)
20:20:22 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:20:22 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:20:22 DEBUG opendrift.models.basemodel:2054: 60.0572267688344 <- latitude -> 60.10966619623835
20:20:22 DEBUG opendrift.models.basemodel:2059: 4.4842677711209555 <- longitude -> 4.556415004139574
20:20:22 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:20:22 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:20:22 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:20:22 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:20:22 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:20:22 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:20:22 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:20:22 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:20:22 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:20:22 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-10 12:00:00 (before)
2024-12-10 13:00:00 (after)
20:20:34 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:20:34 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:20:34 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:20:34 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:20:34 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:20:34 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:20:34 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 30x25x7) for time before (2024-12-10 12:00:00)
20:20:34 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-10 12:00:00) in space (linearNDFast)
20:20:34 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:20:34 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:20:34 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.51574314230834 and -65.44359587914164 degrees.
20:20:34 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.51574314230834 and -65.44359587914164 degrees.
20:20:34 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:20:34 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:20:34 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:20:34 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:20:34 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:20:34 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:20:34 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:20:34 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:20:34 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:20:34 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:20:34 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:20:34 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:20:34 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:20:34 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:20:34 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:20:34 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:20:34 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.23923 (min) 0.409904 (max)
20:20:34 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.467579 (min) 0.595917 (max)
20:20:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.724665 (min) -0.697361 (max)
20:20:34 DEBUG opendrift.models.basemodel.environment:905: x_wind: 3.6091 (min) 3.73438 (max)
20:20:34 DEBUG opendrift.models.basemodel.environment:905: y_wind: -1.16066 (min) -0.958793 (max)
20:20:34 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 2.80434e-05 (min) 3.16675e-05 (max)
20:20:34 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:20:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:20:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:20:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:20:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:20:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:20:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:20:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:20:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:20:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:20:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:20:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:20:34 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:20:34 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:20:34 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:20:34 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:20:34 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:20:34 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.449 (min) 299.992 (max)
20:20:34 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:20:34 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:20:34 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.347511, mean: 0.351059, max: 0.376201
20:20:34 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:20:34 DEBUG opendrift.models.physics_methods:1061: min: 3.211543, mean: 3.227863, max: 3.341484
20:20:34 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.211543, mean: 3.227863, max: 3.341484
20:20:34 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:20:34 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:20:34 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:20:34 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:20:34 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.075170 m/s - 0.078212 m/s)
20:20:34 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:20:34 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:20:34 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:20:34 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:20:34 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:20:34 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:20:34 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:20:34 INFO opendrift.models.basemodel:2035: 2024-12-10 13:00:00 - step 38 of 71 - 1000 active elements (0 deactivated)
20:20:34 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:20:34 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:20:34 DEBUG opendrift.models.basemodel:2054: 60.07397554360061 <- latitude -> 60.128300780092076
20:20:34 DEBUG opendrift.models.basemodel:2059: 4.5156023014834075 <- longitude -> 4.561763314634309
20:20:34 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:20:34 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:20:34 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:20:34 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:20:34 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:20:34 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:20:34 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:20:34 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:20:34 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:20:34 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-10 13:00:00 (before)
2024-12-10 14:00:00 (after)
20:20:46 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:20:46 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:20:46 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:20:46 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:20:46 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:20:46 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:20:46 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 29x25x7) for time before (2024-12-10 13:00:00)
20:20:46 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-10 13:00:00) in space (linearNDFast)
20:20:46 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:20:46 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:20:46 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.48440858624413 and -65.43824757085049 degrees.
20:20:46 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.48440858624413 and -65.43824757085049 degrees.
20:20:46 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:20:46 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:20:46 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:20:46 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:20:46 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:20:46 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:20:46 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:20:46 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:20:46 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:20:46 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:20:46 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:20:46 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:20:46 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:20:46 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:20:46 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:20:46 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:20:46 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.364422 (min) 0.33825 (max)
20:20:46 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.433698 (min) 0.704238 (max)
20:20:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.582835 (min) -0.560916 (max)
20:20:46 DEBUG opendrift.models.basemodel.environment:905: x_wind: 3.13391 (min) 3.20433 (max)
20:20:46 DEBUG opendrift.models.basemodel.environment:905: y_wind: -1.10382 (min) -0.791648 (max)
20:20:46 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 3.96728e-05 (min) 4.47109e-05 (max)
20:20:46 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:20:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:20:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:20:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:20:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:20:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:20:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:20:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:20:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:20:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:20:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:20:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:20:46 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:20:46 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:20:46 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:20:46 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:20:46 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:20:46 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 299.178 (min) 299.997 (max)
20:20:46 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:20:46 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:20:46 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.258999, mean: 0.275365, max: 0.279261
20:20:46 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:20:46 DEBUG opendrift.models.physics_methods:1061: min: 2.772547, mean: 2.858709, max: 2.878956
20:20:46 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.772547, mean: 2.858709, max: 2.878956
20:20:46 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:20:46 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:20:46 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:20:46 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:20:46 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.064895 m/s - 0.067386 m/s)
20:20:46 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:20:46 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:20:46 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:20:46 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:20:46 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:20:46 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:20:46 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:20:46 INFO opendrift.models.basemodel:2035: 2024-12-10 14:00:00 - step 39 of 71 - 1000 active elements (0 deactivated)
20:20:46 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:20:46 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:20:46 DEBUG opendrift.models.basemodel:2054: 60.08962042351297 <- latitude -> 60.15054218192198
20:20:46 DEBUG opendrift.models.basemodel:2059: 4.516663203486504 <- longitude -> 4.564013181895774
20:20:46 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:20:46 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:20:46 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:20:46 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:20:46 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:20:46 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:20:46 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:20:46 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:20:46 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:20:46 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-10 14:00:00 (before)
2024-12-10 15:00:00 (after)
20:21:01 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:21:01 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:21:01 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:21:01 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:21:01 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:21:01 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:21:01 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 28x28x7) for time before (2024-12-10 14:00:00)
20:21:01 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-10 14:00:00) in space (linearNDFast)
20:21:01 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:21:01 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:21:01 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.48334766586996 and -65.4359977017566 degrees.
20:21:01 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.48334766586996 and -65.4359977017566 degrees.
20:21:01 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:21:01 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:21:01 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:21:01 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:21:01 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:21:01 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:21:01 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:21:01 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:21:01 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:21:01 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:21:01 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:21:01 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:21:01 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:21:01 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:21:01 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:21:01 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:21:01 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.476914 (min) 0.142527 (max)
20:21:01 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.391026 (min) 0.797094 (max)
20:21:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.431311 (min) -0.413079 (max)
20:21:01 DEBUG opendrift.models.basemodel.environment:905: x_wind: 2.6174 (min) 2.7386 (max)
20:21:01 DEBUG opendrift.models.basemodel.environment:905: y_wind: -1.20794 (min) -0.338356 (max)
20:21:01 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 3.81322e-05 (min) 4.25088e-05 (max)
20:21:01 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:21:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:21:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:21:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:21:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:21:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:21:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:21:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:21:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:21:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:21:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:21:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:21:01 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:21:01 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:21:01 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:21:01 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:21:01 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:21:01 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 299.46 (min) 299.973 (max)
20:21:01 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:21:01 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:21:01 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.171345, mean: 0.207202, max: 0.218295
20:21:01 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:21:01 DEBUG opendrift.models.physics_methods:1061: min: 2.255100, mean: 2.479077, max: 2.545375
20:21:01 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.255100, mean: 2.479077, max: 2.545375
20:21:01 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:21:01 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:21:01 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:21:01 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:21:01 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.052784 m/s - 0.059578 m/s)
20:21:01 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:21:01 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:21:01 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:21:01 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:21:01 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:21:01 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:21:01 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:21:01 INFO opendrift.models.basemodel:2035: 2024-12-10 15:00:00 - step 40 of 71 - 1000 active elements (0 deactivated)
20:21:01 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:21:01 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:21:01 DEBUG opendrift.models.basemodel:2054: 60.10240998023001 <- latitude -> 60.176075260153986
20:21:01 DEBUG opendrift.models.basemodel:2059: 4.489125208413175 <- longitude -> 4.5659857859006
20:21:01 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:21:01 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:21:01 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:21:01 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:21:01 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:21:01 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:21:01 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:21:01 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:21:01 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:21:01 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-10 15:00:00 (before)
2024-12-10 16:00:00 (after)
20:21:17 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:21:17 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:21:17 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:21:17 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:21:17 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:21:17 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:21:17 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 30x31x7) for time before (2024-12-10 15:00:00)
20:21:17 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-10 15:00:00) in space (linearNDFast)
20:21:17 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:21:17 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:21:17 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.51088566004117 and -65.43402509936809 degrees.
20:21:17 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.51088566004117 and -65.43402509936809 degrees.
20:21:17 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:21:17 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:21:17 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:21:17 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:21:17 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:21:17 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:21:17 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:21:17 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:21:17 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:21:17 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:21:17 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:21:17 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:21:17 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:21:17 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:21:17 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:21:17 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:21:17 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.55643 (min) 0.0240458 (max)
20:21:17 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.361015 (min) 0.827809 (max)
20:21:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.296413 (min) -0.273912 (max)
20:21:17 DEBUG opendrift.models.basemodel.environment:905: x_wind: 2.37137 (min) 2.61874 (max)
20:21:17 DEBUG opendrift.models.basemodel.environment:905: y_wind: -1.24355 (min) -0.487112 (max)
20:21:17 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 3.35541e-05 (min) 3.65664e-05 (max)
20:21:17 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:21:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:21:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:21:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:21:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:21:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:21:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:21:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:21:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:21:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:21:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:21:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:21:17 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:21:17 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:21:17 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:21:17 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:21:17 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:21:17 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 299.401 (min) 299.903 (max)
20:21:17 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:21:17 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:21:17 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.168124, mean: 0.172466, max: 0.177468
20:21:17 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:21:17 DEBUG opendrift.models.physics_methods:1061: min: 2.233800, mean: 2.262409, max: 2.295039
20:21:17 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.233800, mean: 2.262409, max: 2.295039
20:21:17 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:21:17 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:21:17 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:21:17 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:21:17 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.052285 m/s - 0.053718 m/s)
20:21:17 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:21:17 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:21:17 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:21:17 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:21:17 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:21:17 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:21:17 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:21:17 INFO opendrift.models.basemodel:2035: 2024-12-10 16:00:00 - step 41 of 71 - 1000 active elements (0 deactivated)
20:21:17 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:21:17 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:21:17 DEBUG opendrift.models.basemodel:2054: 60.11349702156193 <- latitude -> 60.20250325501734
20:21:17 DEBUG opendrift.models.basemodel:2059: 4.456404968713745 <- longitude -> 4.563694536922246
20:21:17 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:21:17 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:21:17 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:21:17 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:21:17 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:21:17 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:21:17 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:21:17 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:21:17 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:21:17 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-10 16:00:00 (before)
2024-12-10 17:00:00 (after)
20:21:37 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:21:37 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:21:37 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:21:37 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:21:37 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:21:37 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:21:37 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 30x34x7) for time before (2024-12-10 16:00:00)
20:21:37 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-10 16:00:00) in space (linearNDFast)
20:21:37 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:21:37 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:21:37 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.54360589628752 and -65.43631633360309 degrees.
20:21:37 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.54360589628752 and -65.43631633360309 degrees.
20:21:37 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:21:37 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:21:37 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:21:37 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:21:37 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:21:37 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:21:37 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:21:37 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:21:37 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:21:37 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:21:37 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:21:37 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:21:37 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:21:37 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:21:37 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:21:37 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:21:37 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.642204 (min) -0.0892383 (max)
20:21:37 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.342067 (min) 0.8061 (max)
20:21:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.178665 (min) -0.160726 (max)
20:21:37 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.38586 (min) 1.6532 (max)
20:21:37 DEBUG opendrift.models.basemodel.environment:905: y_wind: -0.19558 (min) 1.07386 (max)
20:21:37 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 2.40509e-05 (min) 2.68239e-05 (max)
20:21:37 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:21:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:21:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:21:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:21:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:21:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:21:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:21:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:21:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:21:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:21:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:21:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:21:37 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:21:37 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:21:37 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:21:37 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:21:37 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:21:37 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 299.537 (min) 299.865 (max)
20:21:37 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:21:37 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:21:37 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.047831, mean: 0.055260, max: 0.095602
20:21:37 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:21:37 DEBUG opendrift.models.physics_methods:1061: min: 1.191469, mean: 1.278333, max: 1.684467
20:21:37 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.191469, mean: 1.278333, max: 1.684467
20:21:37 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:21:37 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:21:37 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:21:37 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:21:37 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.027888 m/s - 0.039427 m/s)
20:21:37 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:21:37 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:21:37 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:21:37 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:21:37 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:21:37 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:21:37 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:21:37 INFO opendrift.models.basemodel:2035: 2024-12-10 17:00:00 - step 42 of 71 - 1000 active elements (0 deactivated)
20:21:37 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:21:37 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:21:37 DEBUG opendrift.models.basemodel:2054: 60.12442328929056 <- latitude -> 60.22908390932727
20:21:37 DEBUG opendrift.models.basemodel:2059: 4.416832563636195 <- longitude -> 4.555015882872593
20:21:37 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:21:37 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:21:37 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:21:37 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:21:37 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:21:37 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:21:37 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:21:37 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:21:37 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:21:37 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-10 17:00:00 (before)
2024-12-10 18:00:00 (after)
20:21:54 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:21:54 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:21:54 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:21:54 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:21:54 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:21:54 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:21:54 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 32x37x7) for time before (2024-12-10 17:00:00)
20:21:54 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-10 17:00:00) in space (linearNDFast)
20:21:54 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:21:54 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:21:54 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.58317829562706 and -65.444994998391 degrees.
20:21:54 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.58317829562706 and -65.444994998391 degrees.
20:21:54 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:21:54 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:21:54 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:21:54 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:21:54 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:21:54 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:21:54 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:21:54 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:21:54 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:21:54 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:21:54 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:21:54 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:21:54 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:21:54 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:21:54 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:21:54 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:21:54 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.732284 (min) -0.253937 (max)
20:21:54 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.375493 (min) 0.765525 (max)
20:21:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.10539 (min) -0.0958278 (max)
20:21:54 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0.532808 (min) 1.86179 (max)
20:21:54 DEBUG opendrift.models.basemodel.environment:905: y_wind: 1.25967 (min) 1.91578 (max)
20:21:54 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 7.16759e-06 (min) 1.02335e-05 (max)
20:21:54 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:21:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:21:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:21:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:21:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:21:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:21:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:21:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:21:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:21:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:21:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:21:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:21:54 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:21:54 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:21:54 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:21:54 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:21:54 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:21:54 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 299.553 (min) 300.486 (max)
20:21:54 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:21:54 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:21:54 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.046029, mean: 0.085869, max: 0.168295
20:21:54 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:21:54 DEBUG opendrift.models.physics_methods:1061: min: 1.168810, mean: 1.586794, max: 2.234938
20:21:54 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.168810, mean: 1.586794, max: 2.234938
20:21:54 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:21:54 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:21:54 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:21:54 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:21:54 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.027358 m/s - 0.052312 m/s)
20:21:54 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:21:54 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:21:54 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:21:54 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:21:54 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:21:54 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:21:54 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:21:54 INFO opendrift.models.basemodel:2035: 2024-12-10 18:00:00 - step 43 of 71 - 1000 active elements (0 deactivated)
20:21:54 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:21:54 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:21:54 DEBUG opendrift.models.basemodel:2054: 60.137369158815524 <- latitude -> 60.25351620697329
20:21:54 DEBUG opendrift.models.basemodel:2059: 4.371646275538914 <- longitude -> 4.539255919765918
20:21:54 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:21:54 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:21:54 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:21:54 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:21:54 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:21:54 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:21:54 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:21:54 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:21:54 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:21:54 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-10 18:00:00 (before)
2024-12-10 19:00:00 (after)
20:22:11 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:22:11 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:22:11 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:22:11 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:22:11 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:22:11 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:22:11 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 32x39x7) for time before (2024-12-10 18:00:00)
20:22:11 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-10 18:00:00) in space (linearNDFast)
20:22:11 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:22:11 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:22:11 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.62836457873628 and -65.46075494477975 degrees.
20:22:11 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.62836457873628 and -65.46075494477975 degrees.
20:22:11 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:22:11 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:22:11 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:22:11 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:22:11 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:22:11 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:22:11 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:22:11 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:22:11 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:22:11 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:22:11 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:22:11 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:22:11 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:22:11 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:22:11 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:22:11 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:22:11 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.817679 (min) -0.397725 (max)
20:22:11 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.451862 (min) 0.736211 (max)
20:22:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.109813 (min) -0.10191 (max)
20:22:11 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0.8719 (min) 3.44887 (max)
20:22:11 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0.436231 (min) 2.55932 (max)
20:22:11 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -1.51756e-05 (min) -1.4024e-05 (max)
20:22:11 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:22:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:22:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:22:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:22:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:22:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:22:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:22:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:22:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:22:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:22:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:22:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:22:11 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:22:11 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:22:11 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:22:11 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:22:11 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:22:11 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.551 (min) 300.657 (max)
20:22:11 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:22:11 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:22:11 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.179834, mean: 0.199174, max: 0.297291
20:22:11 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:22:11 DEBUG opendrift.models.physics_methods:1061: min: 2.310282, mean: 2.429078, max: 2.970435
20:22:11 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.310282, mean: 2.429078, max: 2.970435
20:22:11 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:22:11 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:22:11 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:22:11 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:22:11 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.054075 m/s - 0.069527 m/s)
20:22:11 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:22:11 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:22:11 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:22:11 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:22:11 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:22:11 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:22:11 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:22:11 INFO opendrift.models.basemodel:2035: 2024-12-10 19:00:00 - step 44 of 71 - 1000 active elements (0 deactivated)
20:22:11 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:22:11 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:22:11 DEBUG opendrift.models.basemodel:2054: 60.1536209955198 <- latitude -> 60.27206757050479
20:22:11 DEBUG opendrift.models.basemodel:2059: 4.32294297418525 <- longitude -> 4.514608203969906
20:22:11 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:22:11 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:22:11 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:22:11 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:22:11 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:22:11 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:22:11 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:22:11 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:22:11 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:22:11 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-10 19:00:00 (before)
2024-12-10 20:00:00 (after)
20:22:27 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:22:27 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:22:27 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:22:27 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:22:27 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:22:27 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:22:27 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 31x41x7) for time before (2024-12-10 19:00:00)
20:22:27 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-10 19:00:00) in space (linearNDFast)
20:22:27 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:22:27 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:22:27 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.677067872465 and -65.48540266921395 degrees.
20:22:27 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.677067872465 and -65.48540266921395 degrees.
20:22:27 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:22:27 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:22:27 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:22:27 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:22:27 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:22:27 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:22:27 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:22:27 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:22:27 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:22:27 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:22:27 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:22:27 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:22:27 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:22:27 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:22:27 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:22:27 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:22:27 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.859444 (min) -0.454394 (max)
20:22:27 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.372896 (min) 0.715085 (max)
20:22:27 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.199916 (min) -0.185976 (max)
20:22:27 DEBUG opendrift.models.basemodel.environment:905: x_wind: 2.75743 (min) 5.27401 (max)
20:22:27 DEBUG opendrift.models.basemodel.environment:905: y_wind: -1.76379 (min) 0.043502 (max)
20:22:27 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -3.93348e-05 (min) -3.8336e-05 (max)
20:22:27 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:22:27 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:22:27 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:22:27 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:22:27 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:22:27 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:22:27 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:22:27 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:22:27 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:22:27 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:22:27 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:22:27 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:22:27 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:22:27 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:22:27 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:22:27 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:22:27 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:22:27 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 296.732 (min) 300.668 (max)
20:22:27 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:22:27 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:22:27 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.187090, mean: 0.368976, max: 0.760782
20:22:27 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:22:27 DEBUG opendrift.models.physics_methods:1061: min: 2.356434, mean: 3.278492, max: 4.751817
20:22:27 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.356434, mean: 3.278492, max: 4.751817
20:22:27 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:22:27 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:22:27 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:22:27 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:22:27 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.055155 m/s - 0.111222 m/s)
20:22:27 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:22:27 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:22:27 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:22:27 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:22:27 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:22:27 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:22:27 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:22:27 INFO opendrift.models.basemodel:2035: 2024-12-10 20:00:00 - step 45 of 71 - 1000 active elements (0 deactivated)
20:22:27 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:22:27 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:22:27 DEBUG opendrift.models.basemodel:2054: 60.17074229808277 <- latitude -> 60.28296448453986
20:22:27 DEBUG opendrift.models.basemodel:2059: 4.273878596736566 <- longitude -> 4.488716930460014
20:22:27 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:22:27 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:22:27 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:22:27 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:22:27 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:22:27 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:22:27 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:22:27 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:22:27 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:22:27 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-10 20:00:00 (before)
2024-12-10 21:00:00 (after)
20:22:48 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:22:48 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:22:48 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:22:48 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:22:48 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:22:48 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:22:48 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 30x42x7) for time before (2024-12-10 20:00:00)
20:22:48 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-10 20:00:00) in space (linearNDFast)
20:22:48 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:22:48 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:22:48 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.72613225305237 and -65.5112939364269 degrees.
20:22:48 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.72613225305237 and -65.5112939364269 degrees.
20:22:48 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:22:48 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:22:48 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:22:48 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:22:48 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:22:48 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:22:48 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:22:48 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:22:48 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:22:48 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:22:48 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:22:48 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:22:48 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:22:48 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:22:48 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:22:48 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:22:48 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.914963 (min) -0.462544 (max)
20:22:48 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.244938 (min) 0.701732 (max)
20:22:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.37391 (min) -0.365966 (max)
20:22:48 DEBUG opendrift.models.basemodel.environment:905: x_wind: 3.54303 (min) 4.68014 (max)
20:22:48 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.29958 (min) -1.75445 (max)
20:22:48 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -6.03268e-05 (min) -5.67425e-05 (max)
20:22:48 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:22:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:22:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:22:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:22:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:22:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:22:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:22:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:22:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:22:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:22:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:22:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:22:48 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:22:48 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:22:48 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:22:48 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:22:48 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:22:48 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 296.313 (min) 300.507 (max)
20:22:48 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:22:48 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:22:48 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.384527, mean: 0.523956, max: 0.668917
20:22:48 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:22:48 DEBUG opendrift.models.physics_methods:1061: min: 3.378261, mean: 3.939683, max: 4.455699
20:22:48 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.378261, mean: 3.939683, max: 4.455699
20:22:48 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:22:48 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:22:48 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:22:48 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:22:48 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.079073 m/s - 0.104291 m/s)
20:22:48 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:22:48 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:22:48 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:22:48 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:22:48 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:22:48 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:22:48 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:22:48 INFO opendrift.models.basemodel:2035: 2024-12-10 21:00:00 - step 46 of 71 - 1000 active elements (0 deactivated)
20:22:48 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:22:48 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:22:48 DEBUG opendrift.models.basemodel:2054: 60.1889283395699 <- latitude -> 60.28937916702387
20:22:48 DEBUG opendrift.models.basemodel:2059: 4.220417317923425 <- longitude -> 4.463300942388142
20:22:48 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:22:48 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:22:48 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:22:48 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:22:48 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:22:48 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:22:48 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:22:48 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:22:48 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:22:48 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-10 21:00:00 (before)
2024-12-10 22:00:00 (after)
20:23:09 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:23:09 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:23:09 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:23:09 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:23:09 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:23:09 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:23:09 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 28x44x7) for time before (2024-12-10 21:00:00)
20:23:09 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-10 21:00:00) in space (linearNDFast)
20:23:09 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:23:09 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:23:09 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.77959352814685 and -65.53670991560423 degrees.
20:23:09 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.77959352814685 and -65.53670991560423 degrees.
20:23:09 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:23:09 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:23:09 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:23:09 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:23:09 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:23:09 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:23:09 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:23:09 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:23:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:23:09 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:23:09 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:23:09 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:23:09 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:23:09 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:23:09 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:23:09 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:23:09 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.945526 (min) -0.476767 (max)
20:23:09 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.176997 (min) 0.676017 (max)
20:23:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.590875 (min) -0.581832 (max)
20:23:09 DEBUG opendrift.models.basemodel.environment:905: x_wind: 3.00679 (min) 3.60885 (max)
20:23:09 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.00321 (min) -1.81896 (max)
20:23:09 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -6.31162e-05 (min) -5.85568e-05 (max)
20:23:09 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:23:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:23:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:23:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:23:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:23:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:23:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:23:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:23:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:23:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:23:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:23:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:23:09 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:23:09 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:23:09 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:23:09 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:23:09 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:23:09 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 296.22 (min) 300.242 (max)
20:23:09 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:23:09 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:23:09 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.303795, mean: 0.359979, max: 0.408547
20:23:09 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:23:09 DEBUG opendrift.models.physics_methods:1061: min: 3.002756, mean: 3.268287, max: 3.482177
20:23:09 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.002756, mean: 3.268287, max: 3.482177
20:23:09 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:23:09 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:23:09 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:23:09 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:23:09 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.070283 m/s - 0.081505 m/s)
20:23:09 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:23:09 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:23:09 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:23:09 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:23:09 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:23:09 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:23:09 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:23:09 INFO opendrift.models.basemodel:2035: 2024-12-10 22:00:00 - step 47 of 71 - 1000 active elements (0 deactivated)
20:23:09 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:23:09 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:23:09 DEBUG opendrift.models.basemodel:2054: 60.208561931497705 <- latitude -> 60.29386109664894
20:23:09 DEBUG opendrift.models.basemodel:2059: 4.165063119591799 <- longitude -> 4.436249947730537
20:23:09 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:23:09 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:23:09 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:23:09 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:23:09 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:23:09 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:23:09 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:23:09 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:23:09 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:23:09 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-10 22:00:00 (before)
2024-12-10 23:00:00 (after)
20:23:29 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:23:29 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:23:29 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:23:29 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:23:29 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:23:29 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:23:29 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 26x44x7) for time before (2024-12-10 22:00:00)
20:23:29 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-10 22:00:00) in space (linearNDFast)
20:23:29 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:23:29 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:23:29 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.83494773903618 and -65.56376091439978 degrees.
20:23:29 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.83494773903618 and -65.56376091439978 degrees.
20:23:29 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:23:29 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:23:29 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:23:29 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:23:29 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:23:29 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:23:29 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:23:29 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:23:29 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:23:29 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:23:29 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:23:29 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:23:29 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:23:29 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:23:29 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:23:29 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:23:29 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.947282 (min) -0.523363 (max)
20:23:29 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.094631 (min) 0.669663 (max)
20:23:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.786116 (min) -0.767408 (max)
20:23:29 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0.225458 (min) 2.94041 (max)
20:23:29 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.26062 (min) -1.36505 (max)
20:23:29 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -4.5027e-05 (min) -4.0954e-05 (max)
20:23:29 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:23:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:23:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:23:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:23:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:23:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:23:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:23:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:23:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:23:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:23:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:23:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:23:29 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:23:29 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:23:29 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:23:29 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:23:29 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:23:29 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 296.232 (min) 299.907 (max)
20:23:29 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:23:29 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:23:29 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.235919, mean: 0.263252, max: 0.279577
20:23:29 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:23:29 DEBUG opendrift.models.physics_methods:1061: min: 2.646130, mean: 2.794574, max: 2.880580
20:23:29 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.646130, mean: 2.794574, max: 2.880580
20:23:29 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:23:29 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:23:29 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:23:29 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:23:29 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.061936 m/s - 0.067424 m/s)
20:23:29 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:23:29 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:23:29 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:23:29 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:23:29 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:23:29 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:23:29 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:23:29 INFO opendrift.models.basemodel:2035: 2024-12-10 23:00:00 - step 48 of 71 - 1000 active elements (0 deactivated)
20:23:29 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:23:29 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:23:29 DEBUG opendrift.models.basemodel:2054: 60.229313111811244 <- latitude -> 60.29662462959209
20:23:29 DEBUG opendrift.models.basemodel:2059: 4.1096975845328645 <- longitude -> 4.405859842789515
20:23:29 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:23:29 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:23:29 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:23:29 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:23:29 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:23:29 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:23:29 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:23:29 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:23:29 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:23:29 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-10 23:00:00 (before)
2024-12-11 00:00:00 (after)
20:23:47 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:23:47 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:23:47 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:23:47 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:23:47 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:23:47 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:23:47 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 25x45x7) for time before (2024-12-10 23:00:00)
20:23:47 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-10 23:00:00) in space (linearNDFast)
20:23:47 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:23:47 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:23:47 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.89031327731342 and -65.59415100003532 degrees.
20:23:47 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.89031327731342 and -65.59415100003532 degrees.
20:23:47 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:23:47 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:23:47 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:23:47 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:23:47 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:23:47 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:23:47 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:23:47 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:23:47 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:23:47 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:23:47 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:23:47 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:23:47 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:23:47 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:23:47 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:23:47 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:23:47 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.938174 (min) -0.599236 (max)
20:23:47 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0587228 (min) 0.672398 (max)
20:23:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.890407 (min) -0.864192 (max)
20:23:47 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.751955 (min) 1.45417 (max)
20:23:47 DEBUG opendrift.models.basemodel.environment:905: y_wind: -1.55529 (min) -1.12152 (max)
20:23:47 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -1.80869e-05 (min) -1.3424e-05 (max)
20:23:47 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:23:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:23:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:23:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:23:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:23:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:23:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:23:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:23:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:23:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:23:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:23:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:23:47 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:23:47 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:23:47 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:23:47 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:23:47 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:23:47 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 296.31 (min) 300.688 (max)
20:23:47 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:23:47 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:23:47 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.043756, mean: 0.054065, max: 0.111525
20:23:47 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:23:47 DEBUG opendrift.models.physics_methods:1061: min: 1.139594, mean: 1.263656, max: 1.819349
20:23:47 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.139594, mean: 1.263656, max: 1.819349
20:23:47 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:23:47 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:23:47 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:23:47 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:23:47 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.026674 m/s - 0.042584 m/s)
20:23:47 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:23:47 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:23:47 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:23:47 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:23:47 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:23:47 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:23:47 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:23:47 INFO opendrift.models.basemodel:2035: 2024-12-11 00:00:00 - step 49 of 71 - 1000 active elements (0 deactivated)
20:23:47 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:23:47 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:23:47 DEBUG opendrift.models.basemodel:2054: 60.25002833296831 <- latitude -> 60.304925053276264
20:23:47 DEBUG opendrift.models.basemodel:2059: 4.060435287437407 <- longitude -> 4.368794602453429
20:23:47 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:23:47 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:23:47 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:23:47 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:23:47 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:23:47 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:23:47 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:23:47 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:23:47 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:23:47 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-11 00:00:00 (before)
2024-12-11 01:00:00 (after)
20:24:05 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:24:05 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:24:05 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:24:05 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:24:05 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:24:05 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:24:05 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 27x44x7) for time before (2024-12-11 00:00:00)
20:24:05 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-11 00:00:00) in space (linearNDFast)
20:24:05 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:24:05 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:24:05 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.93957557083176 and -65.6312162476852 degrees.
20:24:05 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.93957557083176 and -65.6312162476852 degrees.
20:24:05 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:24:05 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:24:05 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:24:05 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:24:05 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:24:05 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:24:05 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:24:05 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:24:05 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:24:05 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:24:05 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:24:05 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:24:05 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:24:05 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:24:05 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:24:05 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:24:05 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.902455 (min) -0.592336 (max)
20:24:05 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0588507 (min) 0.702311 (max)
20:24:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.88474 (min) -0.858883 (max)
20:24:05 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.109356 (min) 1.45752 (max)
20:24:05 DEBUG opendrift.models.basemodel.environment:905: y_wind: -0.850978 (min) 1.05479 (max)
20:24:05 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -3.34108e-06 (min) 4.10517e-06 (max)
20:24:05 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:24:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:24:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:24:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:24:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:24:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:24:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:24:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:24:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:24:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:24:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:24:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:24:05 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:24:05 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:24:05 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:24:05 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:24:05 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:24:05 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 296.414 (min) 300.875 (max)
20:24:05 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:24:05 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:24:05 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.008367, mean: 0.059450, max: 0.070074
20:24:05 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:24:05 DEBUG opendrift.models.physics_methods:1061: min: 0.498339, mean: 1.319870, max: 1.442143
20:24:05 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 0.498339, mean: 1.319870, max: 1.442143
20:24:05 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:24:05 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:24:05 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:24:05 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:24:05 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.011664 m/s - 0.033755 m/s)
20:24:05 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:24:05 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:24:05 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:24:05 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:24:05 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:24:05 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:24:05 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:24:05 INFO opendrift.models.basemodel:2035: 2024-12-11 01:00:00 - step 50 of 71 - 1000 active elements (0 deactivated)
20:24:05 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:24:05 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:24:05 DEBUG opendrift.models.basemodel:2054: 60.27327936932171 <- latitude -> 60.314909181654436
20:24:05 DEBUG opendrift.models.basemodel:2059: 4.023634641348323 <- longitude -> 4.325060424152566
20:24:05 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:24:05 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:24:05 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:24:05 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:24:05 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:24:05 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:24:05 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:24:05 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:24:05 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:24:05 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-11 01:00:00 (before)
2024-12-11 02:00:00 (after)
20:24:28 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:24:28 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:24:28 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:24:28 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:24:28 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:24:28 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:24:28 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 29x42x7) for time before (2024-12-11 01:00:00)
20:24:28 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-11 01:00:00) in space (linearNDFast)
20:24:28 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:24:28 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:24:28 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.97637621791291 and -65.67495042759289 degrees.
20:24:28 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.97637621791291 and -65.67495042759289 degrees.
20:24:28 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:24:28 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:24:28 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:24:28 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:24:28 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:24:28 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:24:28 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:24:28 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:24:28 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:24:28 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:24:28 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:24:28 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:24:28 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:24:28 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:24:28 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:24:28 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:24:28 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.846475 (min) -0.456547 (max)
20:24:28 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0677887 (min) 0.759253 (max)
20:24:28 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.78475 (min) -0.763402 (max)
20:24:28 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0.690156 (min) 1.77867 (max)
20:24:28 DEBUG opendrift.models.basemodel.environment:905: y_wind: -4.74595 (min) -1.36316 (max)
20:24:28 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 3.32252e-05 (min) 3.79614e-05 (max)
20:24:28 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:24:28 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:24:28 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:24:28 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:24:28 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:24:28 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:24:28 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:24:28 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:24:28 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:24:28 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:24:28 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:24:28 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:24:28 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:24:28 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:24:28 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:24:28 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:24:28 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:24:28 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 296.645 (min) 301.072 (max)
20:24:28 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:24:28 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:24:28 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.078082, mean: 0.490537, max: 0.565809
20:24:28 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:24:28 DEBUG opendrift.models.physics_methods:1061: min: 1.522313, mean: 3.808123, max: 4.097930
20:24:28 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.522313, mean: 3.808123, max: 4.097930
20:24:28 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:24:28 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:24:28 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:24:28 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:24:28 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.035632 m/s - 0.095917 m/s)
20:24:28 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:24:28 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:24:28 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:24:28 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:24:28 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:24:28 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:24:28 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:24:28 INFO opendrift.models.basemodel:2035: 2024-12-11 02:00:00 - step 51 of 71 - 1000 active elements (0 deactivated)
20:24:28 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:24:28 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:24:28 DEBUG opendrift.models.basemodel:2054: 60.296921962206454 <- latitude -> 60.323835762483824
20:24:28 DEBUG opendrift.models.basemodel:2059: 3.9948069690458388 <- longitude -> 4.278343845578291
20:24:28 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:24:28 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:24:28 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:24:28 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:24:28 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:24:28 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:24:28 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:24:28 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:24:28 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:24:28 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-11 02:00:00 (before)
2024-12-11 03:00:00 (after)
20:24:48 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:24:48 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:24:48 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:24:48 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:24:48 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:24:48 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:24:48 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 30x40x7) for time before (2024-12-11 02:00:00)
20:24:48 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-11 02:00:00) in space (linearNDFast)
20:24:48 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:24:48 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:24:48 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.00520390447426 and -65.72166699384546 degrees.
20:24:48 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.00520390447426 and -65.72166699384546 degrees.
20:24:48 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:24:48 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:24:48 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:24:48 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:24:48 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:24:48 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:24:48 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:24:48 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:24:48 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:24:48 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:24:48 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:24:48 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:24:48 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:24:48 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:24:48 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:24:48 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:24:48 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.781187 (min) -0.329068 (max)
20:24:48 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0427383 (min) 0.779425 (max)
20:24:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.637641 (min) -0.620871 (max)
20:24:48 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.373128 (min) 0.672754 (max)
20:24:48 DEBUG opendrift.models.basemodel.environment:905: y_wind: -4.87845 (min) -3.98329 (max)
20:24:48 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 4.43327e-05 (min) 4.7708e-05 (max)
20:24:48 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:24:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:24:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:24:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:24:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:24:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:24:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:24:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:24:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:24:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:24:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:24:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:24:48 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:24:48 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:24:48 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:24:48 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:24:48 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:24:48 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 297.218 (min) 301.118 (max)
20:24:48 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:24:48 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:24:48 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.401453, mean: 0.505071, max: 0.588886
20:24:48 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:24:48 DEBUG opendrift.models.physics_methods:1061: min: 3.451811, mean: 3.869897, max: 4.180664
20:24:48 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.451811, mean: 3.869897, max: 4.180664
20:24:48 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:24:48 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:24:48 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:24:48 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:24:48 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.080794 m/s - 0.097854 m/s)
20:24:48 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:24:48 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:24:48 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:24:48 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:24:48 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:24:48 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:24:48 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:24:48 INFO opendrift.models.basemodel:2035: 2024-12-11 03:00:00 - step 52 of 71 - 1000 active elements (0 deactivated)
20:24:48 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:24:48 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:24:48 DEBUG opendrift.models.basemodel:2054: 60.298346319521244 <- latitude -> 60.33335650418533
20:24:48 DEBUG opendrift.models.basemodel:2059: 3.972894024666431 <- longitude -> 4.231649564125464
20:24:48 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:24:48 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:24:48 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:24:48 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:24:48 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:24:48 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:24:48 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:24:48 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:24:48 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:24:48 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-11 03:00:00 (before)
2024-12-11 04:00:00 (after)
20:25:07 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:25:07 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:25:07 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:25:07 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:25:07 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:25:07 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:25:07 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 32x37x7) for time before (2024-12-11 03:00:00)
20:25:07 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-11 03:00:00) in space (linearNDFast)
20:25:07 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:25:07 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:25:07 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.02711685111733 and -65.76836128386392 degrees.
20:25:08 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.02711685111733 and -65.76836128386392 degrees.
20:25:08 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:25:08 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:25:08 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:25:08 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:25:08 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:25:08 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:25:08 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:25:08 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:25:08 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:25:08 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:25:08 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:25:08 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:25:08 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:25:08 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:25:08 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:25:08 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:25:08 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.723419 (min) -0.245165 (max)
20:25:08 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.00410585 (min) 0.662077 (max)
20:25:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.469795 (min) -0.452482 (max)
20:25:08 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.496553 (min) -0.0566925 (max)
20:25:08 DEBUG opendrift.models.basemodel.environment:905: y_wind: -5.93192 (min) -4.27495 (max)
20:25:08 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 4.20515e-05 (min) 5.26173e-05 (max)
20:25:08 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:25:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:25:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:25:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:25:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:25:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:25:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:25:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:25:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:25:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:25:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:25:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:25:08 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:25:08 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:25:08 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:25:08 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:25:08 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:25:08 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.845 (min) 301.032 (max)
20:25:08 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:25:08 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:25:08 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.455635, mean: 0.780789, max: 0.865873
20:25:08 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:25:08 DEBUG opendrift.models.physics_methods:1061: min: 3.677376, mean: 4.810873, max: 5.069400
20:25:08 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.677376, mean: 4.810873, max: 5.069400
20:25:08 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:25:08 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:25:08 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:25:08 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:25:08 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.086074 m/s - 0.118656 m/s)
20:25:08 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:25:08 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:25:08 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:25:08 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:25:08 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:25:08 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:25:08 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:25:08 INFO opendrift.models.basemodel:2035: 2024-12-11 04:00:00 - step 53 of 71 - 1000 active elements (0 deactivated)
20:25:08 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:25:08 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:25:08 DEBUG opendrift.models.basemodel:2054: 60.29661252512289 <- latitude -> 60.34273976554495
20:25:08 DEBUG opendrift.models.basemodel:2059: 3.9567572852854536 <- longitude -> 4.185197854496008
20:25:08 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:25:08 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:25:08 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:25:08 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:25:08 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:25:08 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:25:08 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:25:08 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:25:08 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:25:08 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-11 04:00:00 (before)
2024-12-11 05:00:00 (after)
20:25:25 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:25:25 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:25:25 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:25:25 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:25:25 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:25:25 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:25:25 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 34x34x7) for time before (2024-12-11 04:00:00)
20:25:25 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-11 04:00:00) in space (linearNDFast)
20:25:25 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:25:25 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:25:25 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.04325358551431 and -65.81481297774637 degrees.
20:25:25 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.04325358551431 and -65.81481297774637 degrees.
20:25:25 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:25:25 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:25:25 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:25:25 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:25:25 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:25:25 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:25:25 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:25:25 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:25:25 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:25:25 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:25:25 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:25:25 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:25:25 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:25:25 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:25:25 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:25:25 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:25:25 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.65914 (min) -0.184309 (max)
20:25:25 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0628039 (min) 0.467208 (max)
20:25:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.300789 (min) -0.280599 (max)
20:25:25 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.8933 (min) -0.328837 (max)
20:25:25 DEBUG opendrift.models.basemodel.environment:905: y_wind: -6.31522 (min) -5.54635 (max)
20:25:25 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 4.73449e-05 (min) 5.18177e-05 (max)
20:25:25 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:25:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:25:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:25:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:25:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:25:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:25:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:25:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:25:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:25:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:25:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:25:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:25:25 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:25:25 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:25:25 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:25:25 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:25:25 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:25:25 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 299.221 (min) 300.867 (max)
20:25:25 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:25:25 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:25:25 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.759405, mean: 0.986300, max: 0.993776
20:25:25 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:25:25 DEBUG opendrift.models.physics_methods:1061: min: 4.747516, mean: 5.410332, max: 5.430925
20:25:25 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 4.747516, mean: 5.410332, max: 5.430925
20:25:25 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:25:25 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:25:25 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:25:25 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:25:25 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.111122 m/s - 0.127118 m/s)
20:25:25 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:25:25 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:25:25 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:25:25 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:25:25 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:25:25 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:25:25 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:25:25 INFO opendrift.models.basemodel:2035: 2024-12-11 05:00:00 - step 54 of 71 - 1000 active elements (0 deactivated)
20:25:25 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:25:25 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:25:25 DEBUG opendrift.models.basemodel:2054: 60.29219069877223 <- latitude -> 60.34964992870665
20:25:25 DEBUG opendrift.models.basemodel:2059: 3.943100789481431 <- longitude -> 4.141982094407804
20:25:25 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:25:25 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:25:25 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:25:25 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:25:25 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:25:25 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:25:25 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:25:25 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:25:25 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:25:25 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-11 05:00:00 (before)
2024-12-11 06:00:00 (after)
20:25:41 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:25:41 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:25:41 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:25:41 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:25:41 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:25:41 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:25:41 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 34x32x7) for time before (2024-12-11 05:00:00)
20:25:41 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-11 05:00:00) in space (linearNDFast)
20:25:41 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:25:41 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:25:41 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.05691008305988 and -65.85802874253977 degrees.
20:25:41 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.05691008305988 and -65.85802874253977 degrees.
20:25:41 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:25:41 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:25:41 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:25:41 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:25:41 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:25:41 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:25:41 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:25:41 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:25:41 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:25:41 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:25:41 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:25:41 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:25:41 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:25:41 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:25:41 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:25:41 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:25:41 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.592285 (min) -0.156632 (max)
20:25:41 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.125622 (min) 0.219443 (max)
20:25:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.14715 (min) -0.126915 (max)
20:25:41 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.104781 (min) 0.588626 (max)
20:25:41 DEBUG opendrift.models.basemodel.environment:905: y_wind: -5.5975 (min) -5.30859 (max)
20:25:41 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 3.70078e-05 (min) 4.01758e-05 (max)
20:25:41 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:25:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:25:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:25:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:25:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:25:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:25:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:25:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:25:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:25:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:25:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:25:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:25:41 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:25:41 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:25:41 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:25:41 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:25:41 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:25:41 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 299.122 (min) 300.686 (max)
20:25:41 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:25:41 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:25:41 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.701779, mean: 0.761648, max: 0.771252
20:25:41 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:25:41 DEBUG opendrift.models.physics_methods:1061: min: 4.563832, mean: 4.754479, max: 4.784404
20:25:41 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 4.563832, mean: 4.754479, max: 4.784404
20:25:41 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:25:41 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:25:41 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:25:41 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:25:41 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.106822 m/s - 0.111985 m/s)
20:25:41 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:25:41 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:25:41 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:25:41 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:25:41 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:25:41 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:25:41 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:25:41 INFO opendrift.models.basemodel:2035: 2024-12-11 06:00:00 - step 55 of 71 - 1000 active elements (0 deactivated)
20:25:41 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:25:41 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:25:41 DEBUG opendrift.models.basemodel:2054: 60.28564794216131 <- latitude -> 60.35330420452856
20:25:41 DEBUG opendrift.models.basemodel:2059: 3.93196329078238 <- longitude -> 4.104121243589553
20:25:41 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:25:41 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:25:41 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:25:41 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:25:41 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:25:41 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:25:41 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:25:41 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:25:41 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:25:41 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-11 06:00:00 (before)
2024-12-11 07:00:00 (after)
20:25:58 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:25:58 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:25:58 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:25:58 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:25:58 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:25:58 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:25:58 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 35x30x7) for time before (2024-12-11 06:00:00)
20:25:58 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-11 06:00:00) in space (linearNDFast)
20:25:58 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:25:58 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:25:58 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.06804758712488 and -65.89588959433549 degrees.
20:25:58 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.06804758712488 and -65.89588959433549 degrees.
20:25:58 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:25:58 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:25:58 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:25:58 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:25:58 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:25:58 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:25:58 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:25:58 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:25:58 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:25:58 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:25:58 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:25:58 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:25:58 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:25:58 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:25:58 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:25:58 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:25:58 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.535251 (min) -0.155966 (max)
20:25:58 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.204306 (min) 0.0115113 (max)
20:25:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.0520127 (min) -0.0384475 (max)
20:25:58 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0.460743 (min) 0.957751 (max)
20:25:58 DEBUG opendrift.models.basemodel.environment:905: y_wind: -5.74754 (min) -5.60869 (max)
20:25:58 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 1.17546e-05 (min) 1.48495e-05 (max)
20:25:58 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:25:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:25:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:25:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:25:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:25:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:25:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:25:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:25:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:25:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:25:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:25:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:25:58 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:25:58 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:25:58 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:25:58 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:25:58 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:25:58 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 299.019 (min) 300.527 (max)
20:25:58 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:25:58 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:25:58 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.789883, mean: 0.800626, max: 0.818033
20:25:58 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:25:58 DEBUG opendrift.models.physics_methods:1061: min: 4.841846, mean: 4.874607, max: 4.927368
20:25:58 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 4.841846, mean: 4.874607, max: 4.927368
20:25:58 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:25:58 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:25:58 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:25:58 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:25:58 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.113330 m/s - 0.115331 m/s)
20:25:58 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:25:58 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:25:58 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:25:58 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:25:58 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:25:58 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:25:58 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:25:58 INFO opendrift.models.basemodel:2035: 2024-12-11 07:00:00 - step 56 of 71 - 1000 active elements (0 deactivated)
20:25:58 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:25:58 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:25:58 DEBUG opendrift.models.basemodel:2054: 60.2764593412068 <- latitude -> 60.350038473196335
20:25:58 DEBUG opendrift.models.basemodel:2059: 3.9218125359937575 <- longitude -> 4.070464704692413
20:25:58 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:25:58 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:25:58 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:25:58 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:25:58 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:25:58 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:25:58 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:25:58 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:25:58 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:25:58 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-11 07:00:00 (before)
2024-12-11 08:00:00 (after)
20:26:14 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:26:14 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:26:14 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:26:14 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:26:14 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:26:14 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:26:14 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 35x28x7) for time before (2024-12-11 07:00:00)
20:26:14 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-11 07:00:00) in space (linearNDFast)
20:26:14 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:26:14 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:26:14 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.07819833180443 and -65.9295461364842 degrees.
20:26:14 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.07819833180443 and -65.9295461364842 degrees.
20:26:14 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:26:14 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:26:14 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:26:14 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:26:14 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:26:14 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:26:14 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:26:14 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:26:14 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:26:14 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:26:14 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:26:14 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:26:14 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:26:14 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:26:14 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:26:14 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:26:14 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.431798 (min) -0.167127 (max)
20:26:14 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.278253 (min) -0.0944813 (max)
20:26:14 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.0607067 (min) -0.0536718 (max)
20:26:14 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0.591351 (min) 1.08914 (max)
20:26:14 DEBUG opendrift.models.basemodel.environment:905: y_wind: -4.52228 (min) -4.33884 (max)
20:26:14 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -1.8662e-05 (min) -1.78118e-05 (max)
20:26:14 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:26:14 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:26:14 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:26:14 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:26:14 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:26:14 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:26:14 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:26:14 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:26:14 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:26:14 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:26:14 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:26:14 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:26:14 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:26:14 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:26:14 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:26:14 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:26:14 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:26:14 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.88 (min) 300.427 (max)
20:26:14 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:26:14 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:26:14 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.472365, mean: 0.489889, max: 0.532276
20:26:14 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:26:14 DEBUG opendrift.models.physics_methods:1061: min: 3.744282, mean: 3.813009, max: 3.974644
20:26:14 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.744282, mean: 3.813009, max: 3.974644
20:26:14 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:26:14 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:26:14 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:26:14 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:26:14 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.087640 m/s - 0.093032 m/s)
20:26:14 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:26:14 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:26:14 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:26:14 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:26:14 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:26:14 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:26:14 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:26:14 INFO opendrift.models.basemodel:2035: 2024-12-11 08:00:00 - step 57 of 71 - 1000 active elements (0 deactivated)
20:26:14 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:26:14 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:26:14 DEBUG opendrift.models.basemodel:2054: 60.26660081190933 <- latitude -> 60.3419348684138
20:26:14 DEBUG opendrift.models.basemodel:2059: 3.911364474887392 <- longitude -> 4.043733287533514
20:26:14 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:26:14 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:26:14 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:26:14 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:26:14 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:26:14 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:26:14 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:26:14 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:26:14 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:26:14 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-11 08:00:00 (before)
2024-12-11 09:00:00 (after)
20:26:34 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:26:34 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:26:34 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:26:34 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:26:34 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:26:34 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:26:34 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 34x27x7) for time before (2024-12-11 08:00:00)
20:26:34 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-11 08:00:00) in space (linearNDFast)
20:26:34 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:26:34 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:26:34 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.08864640069656 and -65.95627756662104 degrees.
20:26:34 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.08864640069656 and -65.95627756662104 degrees.
20:26:34 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:26:34 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:26:34 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:26:34 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:26:34 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:26:34 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:26:34 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:26:34 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:26:34 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:26:34 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:26:34 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:26:34 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:26:34 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:26:34 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:26:34 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:26:34 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:26:34 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.357354 (min) -0.176394 (max)
20:26:34 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.33839 (min) -0.102564 (max)
20:26:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.165808 (min) -0.159859 (max)
20:26:34 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0.278048 (min) 0.370729 (max)
20:26:34 DEBUG opendrift.models.basemodel.environment:905: y_wind: -4.98193 (min) -4.74908 (max)
20:26:34 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -4.78194e-05 (min) -4.61756e-05 (max)
20:26:34 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:26:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:26:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:26:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:26:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:26:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:26:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:26:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:26:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:26:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:26:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:26:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:26:34 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:26:34 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:26:34 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:26:34 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:26:34 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:26:34 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.688 (min) 300.379 (max)
20:26:34 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:26:34 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:26:34 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.557610, mean: 0.576977, max: 0.613943
20:26:34 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:26:34 DEBUG opendrift.models.physics_methods:1061: min: 4.068129, mean: 4.138062, max: 4.268679
20:26:34 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 4.068129, mean: 4.138062, max: 4.268679
20:26:34 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:26:34 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:26:34 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:26:34 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:26:34 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.095220 m/s - 0.099914 m/s)
20:26:34 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:26:34 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:26:34 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:26:34 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:26:34 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:26:34 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:26:34 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:26:34 INFO opendrift.models.basemodel:2035: 2024-12-11 09:00:00 - step 58 of 71 - 1000 active elements (0 deactivated)
20:26:34 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:26:34 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:26:34 DEBUG opendrift.models.basemodel:2054: 60.25602488771975 <- latitude -> 60.32939773308573
20:26:34 DEBUG opendrift.models.basemodel:2059: 3.9000080297765636 <- longitude -> 4.020927165525108
20:26:34 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:26:34 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:26:34 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:26:34 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:26:34 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:26:34 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:26:34 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:26:34 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:26:34 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:26:34 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-11 09:00:00 (before)
2024-12-11 10:00:00 (after)
20:26:51 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:26:51 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:26:51 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:26:51 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:26:51 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:26:51 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:26:51 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 34x27x7) for time before (2024-12-11 09:00:00)
20:26:51 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-11 09:00:00) in space (linearNDFast)
20:26:51 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:26:51 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:26:51 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.10000286124225 and -65.97908369621815 degrees.
20:26:51 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.10000286124225 and -65.97908369621815 degrees.
20:26:51 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:26:51 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:26:51 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:26:51 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:26:51 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:26:51 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:26:51 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:26:51 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:26:51 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:26:51 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:26:51 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:26:51 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:26:51 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:26:51 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:26:51 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:26:51 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:26:51 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.30918 (min) -0.160157 (max)
20:26:51 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.38037 (min) -0.0738321 (max)
20:26:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.365299 (min) -0.352452 (max)
20:26:51 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.728477 (min) -0.506769 (max)
20:26:51 DEBUG opendrift.models.basemodel.environment:905: y_wind: -4.44507 (min) -4.25651 (max)
20:26:51 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -6.43302e-05 (min) -6.22301e-05 (max)
20:26:51 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:26:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:26:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:26:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:26:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:26:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:26:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:26:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:26:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:26:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:26:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:26:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:26:51 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:26:51 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:26:51 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:26:51 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:26:51 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:26:51 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.433 (min) 300.167 (max)
20:26:51 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:26:51 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:26:51 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.452017, mean: 0.490130, max: 0.497693
20:26:51 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:26:51 DEBUG opendrift.models.physics_methods:1061: min: 3.662746, mean: 3.813966, max: 3.843352
20:26:51 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.662746, mean: 3.813966, max: 3.843352
20:26:51 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:26:51 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:26:51 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:26:51 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:26:51 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.085731 m/s - 0.089959 m/s)
20:26:51 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:26:51 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:26:51 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:26:51 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:26:51 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:26:51 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:26:51 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:26:51 INFO opendrift.models.basemodel:2035: 2024-12-11 10:00:00 - step 59 of 71 - 1000 active elements (0 deactivated)
20:26:51 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:26:51 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:26:51 DEBUG opendrift.models.basemodel:2054: 60.24698158195913 <- latitude -> 60.31469327683935
20:26:51 DEBUG opendrift.models.basemodel:2059: 3.887407679258629 <- longitude -> 4.000126605039542
20:26:51 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:26:51 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:26:51 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:26:51 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:26:51 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:26:51 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:26:51 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:26:51 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:26:51 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:26:51 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-11 10:00:00 (before)
2024-12-11 11:00:00 (after)
20:27:08 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:27:08 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:27:08 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:27:08 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:27:08 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:27:08 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:27:08 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 34x27x7) for time before (2024-12-11 10:00:00)
20:27:08 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-11 10:00:00) in space (linearNDFast)
20:27:08 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:27:08 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:27:08 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.11260320626268 and -65.99988425271412 degrees.
20:27:08 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.11260320626268 and -65.99988425271412 degrees.
20:27:08 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:27:08 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:27:08 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:27:08 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:27:08 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:27:08 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:27:08 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:27:08 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:27:08 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:27:08 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:27:08 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:27:08 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:27:08 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:27:08 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:27:08 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:27:08 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:27:08 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.266036 (min) -0.140208 (max)
20:27:08 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.396535 (min) -0.00820025 (max)
20:27:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.570347 (min) -0.554415 (max)
20:27:08 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.949336 (min) -0.824939 (max)
20:27:08 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.33793 (min) -3.26235 (max)
20:27:08 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -5.05952e-05 (min) -4.93243e-05 (max)
20:27:08 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:27:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:27:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:27:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:27:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:27:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:27:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:27:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:27:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:27:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:27:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:27:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:27:08 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:27:08 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:27:08 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:27:08 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:27:08 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:27:08 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.229 (min) 299.807 (max)
20:27:08 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:27:08 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:27:08 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.283626, mean: 0.293276, max: 0.294942
20:27:08 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:27:08 DEBUG opendrift.models.physics_methods:1061: min: 2.901367, mean: 2.950292, max: 2.958679
20:27:08 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.901367, mean: 2.950292, max: 2.958679
20:27:08 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:27:08 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:27:08 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:27:08 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:27:08 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.067910 m/s - 0.069252 m/s)
20:27:08 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:27:08 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:27:08 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:27:08 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:27:08 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:27:08 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:27:08 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:27:08 INFO opendrift.models.basemodel:2035: 2024-12-11 11:00:00 - step 60 of 71 - 1000 active elements (0 deactivated)
20:27:08 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:27:08 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:27:08 DEBUG opendrift.models.basemodel:2054: 60.241269770976515 <- latitude -> 60.29977154385165
20:27:08 DEBUG opendrift.models.basemodel:2059: 3.8754960239432346 <- longitude -> 3.981578924839883
20:27:08 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:27:08 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:27:08 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:27:08 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:27:08 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:27:08 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:27:08 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:27:08 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:27:08 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:27:08 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-11 11:00:00 (before)
2024-12-11 12:00:00 (after)
20:27:24 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:27:24 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:27:24 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:27:24 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:27:24 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:27:24 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:27:24 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 32x27x7) for time before (2024-12-11 11:00:00)
20:27:24 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-11 11:00:00) in space (linearNDFast)
20:27:24 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:27:24 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:27:24 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.12451486045629 and -66.01843194424555 degrees.
20:27:24 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.12451486045629 and -66.01843194424555 degrees.
20:27:24 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:27:24 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:27:24 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:27:24 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:27:24 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:27:24 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:27:24 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:27:24 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:27:24 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:27:24 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:27:24 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:27:24 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:27:24 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:27:24 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:27:24 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:27:24 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:27:24 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.21667 (min) -0.124769 (max)
20:27:24 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.371453 (min) 0.0803197 (max)
20:27:24 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.70944 (min) -0.693409 (max)
20:27:24 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.962754 (min) -0.734061 (max)
20:27:24 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.53333 (min) -2.39367 (max)
20:27:24 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -2.69913e-05 (min) -2.60857e-05 (max)
20:27:24 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:27:24 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:27:24 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:27:24 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:27:24 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:27:24 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:27:24 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:27:24 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:27:24 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:27:24 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:27:24 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:27:24 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:27:24 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:27:24 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:27:24 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:27:24 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:27:24 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:27:24 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.18 (min) 299.425 (max)
20:27:24 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:27:24 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:27:24 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.158606, mean: 0.177196, max: 0.180394
20:27:24 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:27:24 DEBUG opendrift.models.physics_methods:1061: min: 2.169649, mean: 2.293235, max: 2.313880
20:27:24 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.169649, mean: 2.293235, max: 2.313880
20:27:24 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:27:24 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:27:24 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:27:24 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:27:24 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.050783 m/s - 0.054159 m/s)
20:27:24 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:27:24 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:27:24 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:27:24 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:27:24 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:27:24 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:27:24 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:27:24 INFO opendrift.models.basemodel:2035: 2024-12-11 12:00:00 - step 61 of 71 - 1000 active elements (0 deactivated)
20:27:24 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:27:24 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:27:24 DEBUG opendrift.models.basemodel:2054: 60.238784396262176 <- latitude -> 60.28619510817677
20:27:24 DEBUG opendrift.models.basemodel:2059: 3.8657740681239776 <- longitude -> 3.9665221312631207
20:27:24 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:27:24 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:27:24 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:27:24 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:27:24 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:27:24 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:27:24 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:27:24 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:27:24 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:27:24 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-11 12:00:00 (before)
2024-12-11 13:00:00 (after)
20:27:41 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:27:41 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:27:41 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:27:41 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:27:41 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:27:41 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:27:41 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 30x28x7) for time before (2024-12-11 12:00:00)
20:27:41 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-11 12:00:00) in space (linearNDFast)
20:27:41 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:27:41 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:27:41 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.13423681220267 and -66.03348875529231 degrees.
20:27:41 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.13423681220267 and -66.03348875529231 degrees.
20:27:41 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:27:41 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:27:41 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:27:41 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:27:41 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:27:41 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:27:41 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:27:41 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:27:41 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:27:41 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:27:41 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:27:41 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:27:41 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:27:41 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:27:41 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:27:41 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:27:41 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.150675 (min) -0.0882755 (max)
20:27:41 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.316291 (min) 0.170666 (max)
20:27:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.750791 (min) -0.737175 (max)
20:27:41 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.0301458 (min) 0.319299 (max)
20:27:41 DEBUG opendrift.models.basemodel.environment:905: y_wind: -1.6402 (min) -1.47001 (max)
20:27:41 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 1.80097e-06 (min) 2.9226e-06 (max)
20:27:41 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:27:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:27:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:27:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:27:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:27:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:27:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:27:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:27:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:27:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:27:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:27:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:27:41 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:27:41 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:27:41 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:27:41 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:27:41 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:27:41 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.291 (min) 299.258 (max)
20:27:41 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:27:41 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:27:41 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.054525, mean: 0.064411, max: 0.066198
20:27:41 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:27:41 DEBUG opendrift.models.physics_methods:1061: min: 1.272120, mean: 1.382607, max: 1.401693
20:27:41 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.272120, mean: 1.382607, max: 1.401693
20:27:41 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:27:41 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:27:41 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:27:41 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:27:41 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.029776 m/s - 0.032808 m/s)
20:27:41 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:27:41 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:27:41 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:27:41 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:27:41 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:27:41 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:27:41 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:27:41 INFO opendrift.models.basemodel:2035: 2024-12-11 13:00:00 - step 62 of 71 - 1000 active elements (0 deactivated)
20:27:41 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:27:41 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:27:41 DEBUG opendrift.models.basemodel:2054: 60.23994093132491 <- latitude -> 60.27549056515753
20:27:41 DEBUG opendrift.models.basemodel:2059: 3.8603375633102432 <- longitude -> 3.9571348404326487
20:27:41 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:27:41 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:27:41 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:27:41 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:27:41 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:27:41 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:27:41 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:27:41 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:27:41 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:27:41 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-11 13:00:00 (before)
2024-12-11 14:00:00 (after)
20:27:57 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:27:57 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:27:57 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:27:57 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:27:57 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:27:57 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:27:57 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 28x28x7) for time before (2024-12-11 13:00:00)
20:27:57 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-11 13:00:00) in space (linearNDFast)
20:27:57 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:27:57 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:27:57 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.13967331710408 and -66.04287604498178 degrees.
20:27:57 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.13967331710408 and -66.04287604498178 degrees.
20:27:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:27:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:27:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:27:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:27:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:27:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:27:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:27:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:27:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:27:57 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:27:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:27:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:27:57 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:27:57 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:27:57 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:27:57 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:27:57 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.10345 (min) -0.0347647 (max)
20:27:57 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.263182 (min) 0.246879 (max)
20:27:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.691376 (min) -0.681384 (max)
20:27:57 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0.914813 (min) 1.18727 (max)
20:27:57 DEBUG opendrift.models.basemodel.environment:905: y_wind: -0.772791 (min) -0.627169 (max)
20:27:57 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 2.72857e-05 (min) 2.78221e-05 (max)
20:27:57 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:27:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:27:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:27:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:27:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:27:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:27:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:27:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:27:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:27:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:27:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:27:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:27:57 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:27:57 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:27:57 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:27:57 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:27:57 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:27:57 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.15 (min) 299.36 (max)
20:27:57 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:27:57 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:27:57 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.034116, mean: 0.039071, max: 0.048254
20:27:57 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:27:57 DEBUG opendrift.models.physics_methods:1061: min: 1.006261, mean: 1.076475, max: 1.196726
20:27:57 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.006261, mean: 1.076475, max: 1.196726
20:27:57 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:27:57 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:27:57 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:27:57 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:27:57 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.023553 m/s - 0.028011 m/s)
20:27:57 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:27:57 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:27:57 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:27:57 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:27:57 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:27:57 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:27:57 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:27:57 INFO opendrift.models.basemodel:2035: 2024-12-11 14:00:00 - step 63 of 71 - 1000 active elements (0 deactivated)
20:27:57 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:27:57 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:27:57 DEBUG opendrift.models.basemodel:2054: 60.243859076130455 <- latitude -> 60.28306216206
20:27:57 DEBUG opendrift.models.basemodel:2059: 3.8595971682956147 <- longitude -> 3.952869515513068
20:27:57 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:27:57 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:27:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:27:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:27:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:27:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:27:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:27:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:27:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:27:57 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-11 14:00:00 (before)
2024-12-11 15:00:00 (after)
20:28:16 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:28:16 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:28:16 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:28:16 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:28:16 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:28:16 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:28:16 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 27x29x7) for time before (2024-12-11 14:00:00)
20:28:16 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-11 14:00:00) in space (linearNDFast)
20:28:16 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:28:16 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:28:16 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.14041372091427 and -66.0471413607194 degrees.
20:28:16 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.14041372091427 and -66.0471413607194 degrees.
20:28:16 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:28:16 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:28:16 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:28:16 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:28:16 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:28:16 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:28:16 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:28:16 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:28:16 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:28:16 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:28:16 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:28:16 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:28:16 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:28:16 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:28:16 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:28:16 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:28:16 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0620337 (min) 0.0221149 (max)
20:28:16 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.225419 (min) 0.285807 (max)
20:28:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.564217 (min) -0.556 (max)
20:28:16 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.30424 (min) 1.63626 (max)
20:28:16 DEBUG opendrift.models.basemodel.environment:905: y_wind: -0.26342 (min) -0.156463 (max)
20:28:16 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 4.08157e-05 (min) 4.10259e-05 (max)
20:28:16 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:28:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:28:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:28:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:28:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:28:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:28:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:28:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:28:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:28:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:28:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:28:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:28:16 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:28:16 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:28:16 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:28:16 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:28:16 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:28:16 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.088 (min) 299.407 (max)
20:28:16 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:28:16 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:28:16 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.042542, mean: 0.049343, max: 0.066772
20:28:16 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:28:16 DEBUG opendrift.models.physics_methods:1061: min: 1.123668, mean: 1.209557, max: 1.407754
20:28:16 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.123668, mean: 1.209557, max: 1.407754
20:28:16 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:28:16 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:28:16 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:28:16 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:28:16 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.026301 m/s - 0.032950 m/s)
20:28:16 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:28:16 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:28:16 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:28:16 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:28:16 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:28:16 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:28:16 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:28:16 INFO opendrift.models.basemodel:2035: 2024-12-11 15:00:00 - step 64 of 71 - 1000 active elements (0 deactivated)
20:28:16 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:28:16 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:28:16 DEBUG opendrift.models.basemodel:2054: 60.243456575531354 <- latitude -> 60.29217263565762
20:28:16 DEBUG opendrift.models.basemodel:2059: 3.8626520376607267 <- longitude -> 3.9530051207233137
20:28:16 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:28:16 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:28:16 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:28:16 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:28:16 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:28:16 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:28:16 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:28:16 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:28:16 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:28:16 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-11 15:00:00 (before)
2024-12-11 16:00:00 (after)
20:28:32 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:28:32 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:28:32 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:28:32 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:28:32 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:28:32 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:28:32 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 27x29x7) for time before (2024-12-11 15:00:00)
20:28:32 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-11 15:00:00) in space (linearNDFast)
20:28:32 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:28:32 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:28:32 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.1373588554738 and -66.0470057615131 degrees.
20:28:32 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.1373588554738 and -66.0470057615131 degrees.
20:28:32 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:28:32 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:28:32 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:28:32 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:28:32 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:28:32 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:28:32 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:28:32 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:28:32 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:28:32 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:28:32 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:28:32 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:28:32 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:28:32 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:28:32 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:28:32 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:28:32 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.00956874 (min) 0.0708048 (max)
20:28:32 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.217294 (min) 0.282098 (max)
20:28:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.407288 (min) -0.4 (max)
20:28:32 DEBUG opendrift.models.basemodel.environment:905: x_wind: 2.14935 (min) 2.54798 (max)
20:28:32 DEBUG opendrift.models.basemodel.environment:905: y_wind: -0.158186 (min) -0.042322 (max)
20:28:32 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 4.56358e-05 (min) 4.70922e-05 (max)
20:28:32 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:28:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:28:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:28:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:28:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:28:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:28:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:28:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:28:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:28:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:28:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:28:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:28:32 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:28:32 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:28:32 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:28:32 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:28:32 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:28:32 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 297.973 (min) 299.387 (max)
20:28:32 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:28:32 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:28:32 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.113891, mean: 0.120128, max: 0.160323
20:28:32 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:28:32 DEBUG opendrift.models.physics_methods:1061: min: 1.838548, mean: 1.888036, max: 2.181364
20:28:32 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.838548, mean: 1.888036, max: 2.181364
20:28:32 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:28:32 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:28:32 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:28:32 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:28:32 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.043034 m/s - 0.051058 m/s)
20:28:32 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:28:32 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:28:32 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:28:32 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:28:32 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:28:32 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:28:32 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:28:32 INFO opendrift.models.basemodel:2035: 2024-12-11 16:00:00 - step 65 of 71 - 1000 active elements (0 deactivated)
20:28:32 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:28:32 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:28:32 DEBUG opendrift.models.basemodel:2054: 60.24100950332016 <- latitude -> 60.301185186410656
20:28:32 DEBUG opendrift.models.basemodel:2059: 3.868898153280922 <- longitude -> 3.9577690540970547
20:28:32 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:28:32 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:28:32 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:28:32 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:28:32 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:28:32 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:28:32 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:28:32 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:28:32 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:28:32 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-11 16:00:00 (before)
2024-12-11 17:00:00 (after)
20:28:49 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:28:49 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:28:49 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:28:49 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:28:49 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:28:49 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:28:49 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 28x30x7) for time before (2024-12-11 16:00:00)
20:28:49 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-11 16:00:00) in space (linearNDFast)
20:28:49 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:28:49 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:28:49 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.13111273472065 and -66.04224183759061 degrees.
20:28:49 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.13111273472065 and -66.04224183759061 degrees.
20:28:49 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:28:49 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:28:49 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:28:49 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:28:49 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:28:49 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:28:49 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:28:49 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:28:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:28:49 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:28:49 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:28:49 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:28:49 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:28:49 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:28:49 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:28:49 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:28:49 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0342675 (min) 0.105671 (max)
20:28:49 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.252015 (min) 0.229054 (max)
20:28:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.235145 (min) -0.222058 (max)
20:28:49 DEBUG opendrift.models.basemodel.environment:905: x_wind: 2.31252 (min) 2.55642 (max)
20:28:49 DEBUG opendrift.models.basemodel.environment:905: y_wind: -0.760713 (min) -0.138792 (max)
20:28:49 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 4.74537e-05 (min) 4.96953e-05 (max)
20:28:49 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:28:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:28:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:28:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:28:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:28:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:28:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:28:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:28:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:28:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:28:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:28:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:28:49 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:28:49 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:28:49 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:28:49 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:28:49 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:28:49 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 297.869 (min) 299.316 (max)
20:28:49 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:28:49 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:28:49 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.132049, mean: 0.141859, max: 0.172067
20:28:49 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:28:49 DEBUG opendrift.models.physics_methods:1061: min: 1.979692, mean: 2.051584, max: 2.259844
20:28:49 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.979692, mean: 2.051584, max: 2.259844
20:28:49 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:28:49 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:28:49 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:28:49 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:28:49 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.046337 m/s - 0.052895 m/s)
20:28:49 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:28:49 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:28:49 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:28:49 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:28:49 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:28:49 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:28:49 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:28:49 INFO opendrift.models.basemodel:2035: 2024-12-11 17:00:00 - step 66 of 71 - 1000 active elements (0 deactivated)
20:28:49 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:28:49 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:28:49 DEBUG opendrift.models.basemodel:2054: 60.23762505957211 <- latitude -> 60.30809449936915
20:28:49 DEBUG opendrift.models.basemodel:2059: 3.8751928354886265 <- longitude -> 3.9665594348358364
20:28:49 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:28:49 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:28:49 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:28:49 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:28:49 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:28:49 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:28:49 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:28:49 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:28:49 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:28:49 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-11 17:00:00 (before)
2024-12-11 18:00:00 (after)
20:29:06 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:29:06 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:29:06 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:29:06 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:29:06 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:29:06 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:29:06 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 29x31x7) for time before (2024-12-11 17:00:00)
20:29:06 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-11 17:00:00) in space (linearNDFast)
20:29:06 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:29:06 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:29:07 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.12481803954033 and -66.03345145358492 degrees.
20:29:07 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.12481803954033 and -66.03345145358492 degrees.
20:29:07 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:29:07 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:29:07 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:29:07 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:29:07 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:29:07 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:29:07 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:29:07 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:29:07 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:29:07 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:29:07 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:29:07 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:29:07 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:29:07 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:29:07 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:29:07 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:29:07 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0120915 (min) 0.14006 (max)
20:29:07 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.319339 (min) 0.14979 (max)
20:29:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.0785903 (min) -0.0562353 (max)
20:29:07 DEBUG opendrift.models.basemodel.environment:905: x_wind: 2.13272 (min) 2.66201 (max)
20:29:07 DEBUG opendrift.models.basemodel.environment:905: y_wind: -1.50143 (min) -0.839712 (max)
20:29:07 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 3.92358e-05 (min) 4.10474e-05 (max)
20:29:07 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:29:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:29:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:29:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:29:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:29:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:29:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:29:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:29:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:29:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:29:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:29:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:29:07 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:29:07 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:29:07 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:29:07 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:29:07 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:29:07 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 297.722 (min) 299.253 (max)
20:29:07 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:29:07 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:29:07 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.167349, mean: 0.186619, max: 0.191750
20:29:07 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:29:07 DEBUG opendrift.models.physics_methods:1061: min: 2.228646, mean: 2.353391, max: 2.385600
20:29:07 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.228646, mean: 2.353391, max: 2.385600
20:29:07 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:29:07 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:29:07 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:29:07 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:29:07 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.052164 m/s - 0.055838 m/s)
20:29:07 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:29:07 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:29:07 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:29:07 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:29:07 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:29:07 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:29:07 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:29:07 INFO opendrift.models.basemodel:2035: 2024-12-11 18:00:00 - step 67 of 71 - 1000 active elements (0 deactivated)
20:29:07 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:29:07 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:29:07 DEBUG opendrift.models.basemodel:2054: 60.231172904569696 <- latitude -> 60.31196405466584
20:29:07 DEBUG opendrift.models.basemodel:2059: 3.878758478782234 <- longitude -> 3.9791187380532844
20:29:07 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:29:07 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:29:07 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:29:07 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:29:07 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:29:07 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:29:07 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:29:07 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:29:07 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:29:07 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-11 18:00:00 (before)
2024-12-11 19:00:00 (after)
20:29:23 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:29:23 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:29:23 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:29:23 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:29:23 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:29:23 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:29:23 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 31x32x7) for time before (2024-12-11 18:00:00)
20:29:23 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-11 18:00:00) in space (linearNDFast)
20:29:23 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:29:23 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:29:23 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.12125239241436 and -66.02089214798814 degrees.
20:29:23 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.12125239241436 and -66.02089214798814 degrees.
20:29:23 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:29:23 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:29:23 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:29:23 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:29:23 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:29:23 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:29:23 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:29:23 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:29:23 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:29:23 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:29:23 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:29:23 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:29:23 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:29:23 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:29:23 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:29:23 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:29:23 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0142452 (min) 0.17618 (max)
20:29:23 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.399498 (min) 0.0704574 (max)
20:29:23 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.0338341 (min) 0.0637175 (max)
20:29:23 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0.591101 (min) 1.96856 (max)
20:29:23 DEBUG opendrift.models.basemodel.environment:905: y_wind: -1.87434 (min) -1.45603 (max)
20:29:23 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 2.09305e-05 (min) 2.21717e-05 (max)
20:29:23 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:29:23 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:29:23 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:29:23 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:29:23 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:29:23 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:29:23 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:29:23 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:29:23 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:29:23 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:29:23 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:29:23 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:29:23 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:29:23 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:29:23 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:29:23 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:29:23 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:29:23 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 297.504 (min) 299.216 (max)
20:29:23 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:29:23 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:29:23 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.095019, mean: 0.129454, max: 0.147484
20:29:23 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:29:23 DEBUG opendrift.models.physics_methods:1061: min: 1.679322, mean: 1.959303, max: 2.092191
20:29:23 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.679322, mean: 1.959303, max: 2.092191
20:29:23 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:29:23 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:29:23 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:29:23 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:29:23 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.039307 m/s - 0.048970 m/s)
20:29:23 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:29:23 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:29:23 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:29:23 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:29:23 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:29:23 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:29:23 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:29:23 INFO opendrift.models.basemodel:2035: 2024-12-11 19:00:00 - step 68 of 71 - 1000 active elements (0 deactivated)
20:29:23 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:29:23 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:29:23 DEBUG opendrift.models.basemodel:2054: 60.21850926539901 <- latitude -> 60.31302936034645
20:29:23 DEBUG opendrift.models.basemodel:2059: 3.878600618706879 <- longitude -> 3.9931179971435906
20:29:23 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:29:23 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:29:23 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:29:23 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:29:23 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:29:23 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:29:23 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:29:23 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:29:23 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:29:23 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-11 19:00:00 (before)
2024-12-11 20:00:00 (after)
20:29:40 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:29:40 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:29:40 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:29:40 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:29:40 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:29:40 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:29:40 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 31x34x7) for time before (2024-12-11 19:00:00)
20:29:40 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-11 19:00:00) in space (linearNDFast)
20:29:40 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:29:40 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:29:40 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.12141024713436 and -66.00689289912097 degrees.
20:29:40 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.12141024713436 and -66.00689289912097 degrees.
20:29:40 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:29:40 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:29:40 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:29:40 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:29:40 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:29:40 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:29:40 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:29:40 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:29:40 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:29:40 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:29:40 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:29:40 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:29:40 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:29:40 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:29:40 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:29:40 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:29:40 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0477137 (min) 0.19184 (max)
20:29:40 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.468969 (min) 0.0139148 (max)
20:29:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.0533657 (min) 0.0839939 (max)
20:29:40 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0.584646 (min) 1.07708 (max)
20:29:40 DEBUG opendrift.models.basemodel.environment:905: y_wind: -1.97041 (min) -1.74576 (max)
20:29:40 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -9.50741e-06 (min) -9.07012e-06 (max)
20:29:40 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:29:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:29:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:29:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:29:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:29:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:29:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:29:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:29:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:29:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:29:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:29:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:29:40 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:29:40 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:29:40 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:29:40 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:29:40 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:29:40 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 297.227 (min) 299.213 (max)
20:29:40 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:29:40 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:29:40 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.096846, mean: 0.097618, max: 0.109657
20:29:40 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:29:40 DEBUG opendrift.models.physics_methods:1061: min: 1.695391, mean: 1.702110, max: 1.804044
20:29:40 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.695391, mean: 1.702110, max: 1.804044
20:29:40 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:29:40 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:29:40 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:29:40 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:29:40 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.039683 m/s - 0.042226 m/s)
20:29:40 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:29:40 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:29:40 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:29:40 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:29:40 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:29:40 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:29:40 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:29:40 INFO opendrift.models.basemodel:2035: 2024-12-11 20:00:00 - step 69 of 71 - 1000 active elements (0 deactivated)
20:29:40 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:29:40 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:29:40 DEBUG opendrift.models.basemodel:2054: 60.202227475328115 <- latitude -> 60.31220560308148
20:29:40 DEBUG opendrift.models.basemodel:2059: 3.8764806794933135 <- longitude -> 4.006969608172233
20:29:40 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:29:40 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:29:40 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:29:40 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:29:40 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:29:40 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:29:40 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:29:40 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:29:40 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:29:40 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-11 20:00:00 (before)
2024-12-11 21:00:00 (after)
20:29:56 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:29:56 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:29:56 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:29:56 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:29:56 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:29:56 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:29:56 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 33x36x7) for time before (2024-12-11 20:00:00)
20:29:56 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-11 20:00:00) in space (linearNDFast)
20:29:56 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:29:56 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:29:56 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.12353019346045 and -65.99304128400004 degrees.
20:29:56 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.12353019346045 and -65.99304128400004 degrees.
20:29:56 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:29:56 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:29:56 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:29:56 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:29:56 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:29:56 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:29:56 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:29:56 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:29:56 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:29:56 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:29:56 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:29:56 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:29:56 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:29:56 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:29:56 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:29:56 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:29:56 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0846201 (min) 0.198663 (max)
20:29:56 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.459784 (min) -0.0300777 (max)
20:29:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.036585 (min) -0.0122727 (max)
20:29:56 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0.608537 (min) 1.12288 (max)
20:29:56 DEBUG opendrift.models.basemodel.environment:905: y_wind: -1.69711 (min) -1.62781 (max)
20:29:56 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -4.41572e-05 (min) -4.21126e-05 (max)
20:29:56 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:29:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:29:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:29:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:29:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:29:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:29:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:29:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:29:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:29:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:29:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:29:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:29:56 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:29:56 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:29:56 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:29:56 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:29:56 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:29:56 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 296.999 (min) 299.228 (max)
20:29:56 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:29:56 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:29:56 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.078712, mean: 0.080452, max: 0.096201
20:29:56 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:29:56 DEBUG opendrift.models.physics_methods:1061: min: 1.528447, mean: 1.545186, max: 1.689742
20:29:56 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.528447, mean: 1.545186, max: 1.689742
20:29:56 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:29:56 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:29:56 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:29:56 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:29:56 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.035775 m/s - 0.039551 m/s)
20:29:56 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:29:56 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:29:56 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:29:56 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:29:56 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:29:56 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:29:56 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:29:56 INFO opendrift.models.basemodel:2035: 2024-12-11 21:00:00 - step 70 of 71 - 1000 active elements (0 deactivated)
20:29:56 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:29:56 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:29:56 DEBUG opendrift.models.basemodel:2054: 60.18629226492533 <- latitude -> 60.31018172453916
20:29:56 DEBUG opendrift.models.basemodel:2059: 3.8724320614001315 <- longitude -> 4.020790528219189
20:29:56 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:29:56 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:29:56 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:29:56 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:29:56 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:29:56 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:29:56 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:29:56 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:29:56 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:29:56 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-11 21:00:00 (before)
2024-12-11 22:00:00 (after)
20:30:13 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:30:13 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:30:13 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:30:13 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:30:13 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:30:13 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:30:13 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 33x39x7) for time before (2024-12-11 21:00:00)
20:30:13 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-11 21:00:00) in space (linearNDFast)
20:30:13 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:30:13 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:30:13 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.12757880952803 and -65.9792203731031 degrees.
20:30:13 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.12757880952803 and -65.9792203731031 degrees.
20:30:13 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:30:13 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:30:13 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:30:13 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:30:13 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:30:13 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:30:13 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:30:13 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:30:13 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:30:13 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:30:13 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:30:13 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:30:13 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:30:13 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:30:13 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:30:13 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:30:13 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.150525 (min) 0.198225 (max)
20:30:13 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.426162 (min) -0.0584945 (max)
20:30:13 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.23464 (min) -0.218406 (max)
20:30:13 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0.632522 (min) 1.37404 (max)
20:30:13 DEBUG opendrift.models.basemodel.environment:905: y_wind: -1.56719 (min) -1.4986 (max)
20:30:13 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -6.79346e-05 (min) -6.51048e-05 (max)
20:30:13 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:30:13 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:30:13 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:30:13 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:30:13 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:30:13 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:30:13 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:30:13 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:30:13 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:30:13 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:30:13 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:30:13 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:30:13 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:30:13 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:30:13 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:30:13 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:30:13 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:30:13 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 296.811 (min) 299.264 (max)
20:30:13 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:30:13 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:30:13 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.068414, mean: 0.071432, max: 0.106864
20:30:13 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:30:13 DEBUG opendrift.models.physics_methods:1061: min: 1.424956, mean: 1.455850, max: 1.780923
20:30:13 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.424956, mean: 1.455850, max: 1.780923
20:30:13 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:30:13 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:30:13 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:30:13 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:30:13 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.033353 m/s - 0.041685 m/s)
20:30:13 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:30:13 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:30:13 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:30:13 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:30:13 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:30:13 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:30:13 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:30:13 INFO opendrift.models.basemodel:2035: 2024-12-11 22:00:00 - step 71 of 71 - 1000 active elements (0 deactivated)
20:30:13 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:30:13 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:30:13 DEBUG opendrift.models.basemodel:2054: 60.171530580004514 <- latitude -> 60.30727859284619
20:30:13 DEBUG opendrift.models.basemodel:2059: 3.864418990730272 <- longitude -> 4.034529227412516
20:30:13 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:30:13 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:30:13 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:30:13 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:30:13 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:30:13 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
20:30:13 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:30:13 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:30:13 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
20:30:13 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-11 22:00:00 (before)
2024-12-11 23:00:00 (after)
20:30:29 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
20:30:29 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:30:29 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:30:29 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:30:29 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:30:29 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:30:29 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 35x40x7) for time before (2024-12-11 22:00:00)
20:30:29 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-11 22:00:00) in space (linearNDFast)
20:30:29 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:30:29 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:30:29 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.13559188896082 and -65.96548168332149 degrees.
20:30:29 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.13559188896082 and -65.96548168332149 degrees.
20:30:29 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:30:29 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:30:29 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:30:29 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:30:29 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:30:29 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:30:29 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:30:29 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:30:29 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:30:29 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:30:29 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:30:29 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:30:29 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:30:29 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:30:29 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
20:30:29 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:30:29 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.26267 (min) 0.184164 (max)
20:30:29 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.378489 (min) -0.0252513 (max)
20:30:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.47847 (min) -0.467331 (max)
20:30:29 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.62688 (min) 2.28848 (max)
20:30:29 DEBUG opendrift.models.basemodel.environment:905: y_wind: -1.12613 (min) -0.981435 (max)
20:30:29 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -7.10231e-05 (min) -6.6678e-05 (max)
20:30:29 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:30:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:30:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:30:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:30:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:30:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:30:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:30:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:30:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:30:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:30:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:30:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:30:29 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:30:29 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:30:29 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:30:29 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:30:29 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:30:29 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 296.738 (min) 299.34 (max)
20:30:29 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:30:29 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:30:29 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.092461, mean: 0.105238, max: 0.160031
20:30:29 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:30:29 DEBUG opendrift.models.physics_methods:1061: min: 1.656566, mean: 1.766517, max: 2.179375
20:30:29 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.656566, mean: 1.766517, max: 2.179375
20:30:29 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:30:29 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:30:29 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:30:29 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:30:29 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.038774 m/s - 0.051011 m/s)
20:30:29 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:30:29 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:30:29 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:30:29 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:30:29 DEBUG opendrift.models.basemodel:2152: Cleaning up
20:30:29 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:30:29 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:30:29 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:30:29 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:30:29 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:30:29 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:30:29 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:30:29 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:30:29 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:30:29 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:30:29 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:30:29 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:30:29 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:30:29 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:30:29 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:30:29 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:30:29 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:30:29 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:30:29 DEBUG opendrift.models.basemodel:95: Changed mode from Mode.Run to Mode.Result
20:30:29 DEBUG opendrift.models.oceandrift:115: No machine learning correction available.
20:30:29 DEBUG opendrift.config:168: Adding 50 config items from environment
20:30:29 DEBUG opendrift.config:168: Adding 5 config items from environment
20:30:29 DEBUG opendrift.config:168: Adding 18 config items from __init__
20:30:29 DEBUG opendrift.config:178: Overwriting config item readers:max_number_of_fails
20:30:29 DEBUG opendrift.config:168: Adding 5 config items from __init__
20:30:29 INFO opendrift.models.basemodel:515: OpenDriftSimulation initialised (version 1.12.0 / v1.12.0-26-g390e945)
20:30:29 DEBUG opendrift.config:168: Adding 15 config items from oceandrift
20:30:29 DEBUG opendrift.config:178: Overwriting config item seed:z
20:30:29 DEBUG opendrift.readers.reader_lazy:38: Delaying initialisation of LazyReader: https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:30:29 DEBUG opendrift.models.basemodel.environment:328: Added reader LazyReader: https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:30:29 INFO opendrift.models.basemodel.environment:218: Adding a dynamical landmask with max. priority based on assumed maximum speed of 2.0 m/s. Adding a customised landmask may be faster...
20:30:29 DEBUG opendrift.readers.basereader:186: Variable mapping: ['sea_floor_depth_below_sea_level'] -> ['land_binary_mask'] is not activated
20:30:29 DEBUG opendrift.models.basemodel.environment:328: Added reader global_landmask
20:30:29 INFO opendrift.models.basemodel.environment:245: Fallback values will be used for the following variables which have no readers:
20:30:29 INFO opendrift.models.basemodel.environment:248: x_sea_water_velocity: 0.000000
20:30:29 INFO opendrift.models.basemodel.environment:248: y_sea_water_velocity: 0.000000
20:30:29 INFO opendrift.models.basemodel.environment:248: sea_surface_height: 0.000000
20:30:29 INFO opendrift.models.basemodel.environment:248: x_wind: 0.000000
20:30:29 INFO opendrift.models.basemodel.environment:248: y_wind: 0.000000
20:30:29 INFO opendrift.models.basemodel.environment:248: upward_sea_water_velocity: 0.000000
20:30:29 INFO opendrift.models.basemodel.environment:248: ocean_vertical_diffusivity: 0.000000
20:30:29 INFO opendrift.models.basemodel.environment:248: sea_surface_wave_significant_height: 0.000000
20:30:29 INFO opendrift.models.basemodel.environment:248: sea_surface_wave_stokes_drift_x_velocity: 0.000000
20:30:29 INFO opendrift.models.basemodel.environment:248: sea_surface_wave_stokes_drift_y_velocity: 0.000000
20:30:29 INFO opendrift.models.basemodel.environment:248: sea_surface_wave_period_at_variance_spectral_density_maximum: 0.000000
20:30:29 INFO opendrift.models.basemodel.environment:248: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0.000000
20:30:29 INFO opendrift.models.basemodel.environment:248: sea_surface_swell_wave_to_direction: 0.000000
20:30:29 INFO opendrift.models.basemodel.environment:248: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0.000000
20:30:29 INFO opendrift.models.basemodel.environment:248: sea_surface_swell_wave_significant_height: 0.000000
20:30:29 INFO opendrift.models.basemodel.environment:248: sea_surface_wind_wave_to_direction: 0.000000
20:30:29 INFO opendrift.models.basemodel.environment:248: sea_surface_wind_wave_mean_period: 0.000000
20:30:29 INFO opendrift.models.basemodel.environment:248: sea_surface_wind_wave_significant_height: 0.000000
20:30:29 INFO opendrift.models.basemodel.environment:248: surface_downward_x_stress: 0.000000
20:30:29 INFO opendrift.models.basemodel.environment:248: surface_downward_y_stress: 0.000000
20:30:29 INFO opendrift.models.basemodel.environment:248: turbulent_kinetic_energy: 0.000000
20:30:29 INFO opendrift.models.basemodel.environment:248: turbulent_generic_length_scale: 0.000000
20:30:29 INFO opendrift.models.basemodel.environment:248: ocean_mixed_layer_thickness: 50.000000
20:30:29 INFO opendrift.models.basemodel.environment:248: sea_floor_depth_below_sea_level: 10000.000000
20:30:29 DEBUG opendrift.models.basemodel:95: Changed mode from Mode.Config to Mode.Ready
20:30:29 DEBUG opendrift.models.basemodel:95: Changed mode from Mode.Ready to Mode.Run
20:30:29 DEBUG opendrift.models.basemodel:1777:
------------------------------------------------------
Software and hardware:
OpenDrift version 1.12.0
Platform: Linux, 5.15.0-1057-aws
68.56775283813477 GB memory
36 processors (x86_64)
NumPy version 1.26.4
SciPy version 1.14.1
Matplotlib version 3.9.1
NetCDF4 version 1.6.1
Xarray version 2024.11.0
ADIOS (adios_db) version 1.2.5
Copernicusmarine version 1.3.5
Python version 3.11.6 | packaged by conda-forge | (main, Oct 3 2023, 10:40:35) [GCC 12.3.0]
------------------------------------------------------
20:30:29 DEBUG opendrift.models.basemodel:1791: No output file is specified, neglecting export_buffer_length
20:30:29 DEBUG opendrift.models.basemodel:1909: Finalizing environment and preparing readers for simulation coverage ([-4.71627286447143, 55.39190523302233, 13.715758357177728, 64.60825116956556]) and time (2024-12-09 00:00:00 to 2024-12-11 23:00:00)
20:30:29 DEBUG opendrift.models.basemodel.environment:180: Preparing LazyReader: https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be for extent [-4.71627286447143, 55.39190523302233, 13.715758357177728, 64.60825116956556]
20:30:29 DEBUG opendrift.models.basemodel.environment:180: Preparing global_landmask for extent [-4.71627286447143, 55.39190523302233, 13.715758357177728, 64.60825116956556]
20:30:29 DEBUG opendrift.readers.basereader.variables:549: Nothing more to prepare for global_landmask
20:30:29 INFO opendrift.models.basemodel:935: Using existing reader for land_binary_mask
20:30:29 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:30:29 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:30:29 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:30:29 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:30:29 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:30:29 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:30:29 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:30:29 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:30:29 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:30:29 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:30:29 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:30:29 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:30:29 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:30:29 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:30:29 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:30:29 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:30:29 INFO opendrift.models.basemodel:946: All points are in ocean
20:30:29 DEBUG opendrift.models.basemodel:890: to be seeded: 1000, already seeded 0
20:30:29 DEBUG opendrift.models.basemodel:908: Released 1000 new elements.
20:30:29 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:30:29 INFO opendrift.models.basemodel:2035: 2024-12-09 00:00:00 - step 1 of 71 - 1000 active elements (0 deactivated)
20:30:29 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:30:29 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:30:29 DEBUG opendrift.models.basemodel:2054: 59.99731 <- latitude -> 60.002846
20:30:29 DEBUG opendrift.models.basemodel:2059: 4.4945407 <- longitude -> 4.504945
20:30:29 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:30:29 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:30:29 DEBUG opendrift.models.basemodel.environment:581: Variables not covered by any reader: ['turbulent_kinetic_energy', 'upward_sea_water_velocity', 'sea_surface_wave_significant_height', 'ocean_vertical_diffusivity', 'surface_downward_y_stress', 'ocean_mixed_layer_thickness', 'sea_surface_wave_period_at_variance_spectral_density_maximum', 'y_sea_water_velocity', 'turbulent_generic_length_scale', 'sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment', 'sea_surface_wave_stokes_drift_y_velocity', 'surface_downward_x_stress', 'y_wind', 'sea_floor_depth_below_sea_level', 'sea_surface_height', 'x_sea_water_velocity', 'x_wind', 'sea_surface_wave_stokes_drift_x_velocity']
20:30:29 DEBUG opendrift.readers.reader_lazy:57: Initialising: LazyReader: https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:30:29 DEBUG opendrift.readers:127: Testing reader <module 'opendrift.readers.reader_netCDF_CF_generic' from '/root/project/opendrift/readers/reader_netCDF_CF_generic.py'>
20:30:29 INFO opendrift.readers:58: Opening file with xr.open_dataset
20:30:37 DEBUG opendrift.readers.reader_netCDF_CF_generic:128: Finding coordinate variables.
20:30:37 DEBUG opendrift.readers.reader_netCDF_CF_generic:143: Parsing CF grid mapping dictionary: {'grid_mapping_name': 'polar_stereographic', 'straight_vertical_longitude_from_pole': 70.0, 'latitude_of_projection_origin': 90.0, 'standard_parallel': 60.0, 'false_easting': 3192800.0, 'false_northing': 1784000.0, 'semi_major_axis': 6378137.0, 'semi_minor_axis': 6356752.3142, 'proj4': '+proj=stere +lat_0=90 +lat_ts=60 +lon_0=70 +x_0=3192800 +y_0=1784000 +a=6378137 +b=6356752.3142 +units=m +no_defs +type=crs'}
20:30:38 INFO opendrift.readers.reader_netCDF_CF_generic:337: Detected dimensions: {'x': 'X', 'y': 'Y', 'z': 'depth', 'time': 'time'}
20:30:38 DEBUG opendrift.readers.reader_netCDF_CF_generic:373: Skipped variables without standard_name: ['angle', 'tke', 'ubar', 'vbar']
20:30:38 DEBUG opendrift.readers.basereader.variables:608: Setting buffer size 25 for reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be, assuming a maximum average speed of 5 m/s and time span of 1:00:00
20:30:38 DEBUG opendrift.readers.basereader:186: Variable mapping: ['sea_floor_depth_below_sea_level'] -> ['land_binary_mask'] is not activated
20:30:38 DEBUG opendrift.readers.basereader.variables:563: Adding variable mapping: ['x_wind', 'y_wind'] -> wind_speed
20:30:38 DEBUG opendrift.readers.basereader.variables:563: Adding variable mapping: ['x_sea_water_velocity', 'y_sea_water_velocity'] -> sea_water_speed
20:30:38 DEBUG opendrift.readers.basereader.structured:153: Clearing cache for reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be before starting new simulation
20:30:38 DEBUG opendrift.readers.basereader.variables:608: Setting buffer size 11 for reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be, assuming a maximum average speed of 2 m/s and time span of 1:00:00
20:30:38 DEBUG opendrift.readers.basereader.variables:549: Nothing more to prepare for https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:30:38 DEBUG opendrift.readers.reader_lazy:72: Reader initialised: https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:30:38 DEBUG opendrift.readers.basereader.variables:608: Setting buffer size 11 for reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be, assuming a maximum average speed of 2 m/s and time span of 1:00:00
20:30:38 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:30:38 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:30:38 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:30:38 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:30:38 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:30:38 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:30:38 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:30:38 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:30:38 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:30:38 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:30:38 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:30:38 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:30:38 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:30:38 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:30:38 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:30:38 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:30:38 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:30:38 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:30:38 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-09 00:00:00 (before)
2024-12-09 01:00:00 (after)
20:30:39 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:30:39 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:30:39 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:30:39 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:30:39 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:30:39 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 22x23x2) for time before (2024-12-09 00:00:00)
20:30:39 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-09 00:00:00) in space (linearNDFast)
20:30:39 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:30:39 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:30:39 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.50547356643384 and -65.49506707409589 degrees.
20:30:39 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.50547356643384 and -65.49506707409589 degrees.
20:30:39 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:30:39 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:30:39 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:30:39 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:30:39 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:30:39 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:30:39 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0636311 (min) 0.0868608 (max)
20:30:39 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.13082 (min) 0.171825 (max)
20:30:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.498931 (min) -0.49627 (max)
20:30:39 DEBUG opendrift.models.basemodel.environment:905: x_wind: -2.22237 (min) -2.21359 (max)
20:30:39 DEBUG opendrift.models.basemodel.environment:905: y_wind: -4.82698 (min) -4.78051 (max)
20:30:39 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -2.06035e-07 (min) -1.19372e-07 (max)
20:30:39 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:30:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:30:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:30:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:30:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:30:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:30:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:30:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:30:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:30:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:30:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:30:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:30:39 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:30:39 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:30:39 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:30:39 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:30:39 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:30:39 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.369 (min) 298.632 (max)
20:30:39 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:30:39 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:30:39 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.682730, mean: 0.688796, max: 0.694671
20:30:39 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:30:39 DEBUG opendrift.models.physics_methods:1061: min: 4.501469, mean: 4.521418, max: 4.540660
20:30:39 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 4.501469, mean: 4.521418, max: 4.540660
20:30:39 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:30:39 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:30:39 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:30:39 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:30:39 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.105363 m/s - 0.106280 m/s)
20:30:39 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:30:39 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:30:39 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:30:39 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:30:39 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:30:39 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:30:39 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:30:39 INFO opendrift.models.basemodel:2035: 2024-12-09 01:00:00 - step 2 of 71 - 1000 active elements (0 deactivated)
20:30:39 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:30:39 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:30:39 DEBUG opendrift.models.basemodel:2054: 59.99877833511116 <- latitude -> 60.004880615268405
20:30:39 DEBUG opendrift.models.basemodel:2059: 4.496512215103492 <- longitude -> 4.507347810270389
20:30:39 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:30:39 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:30:39 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:30:39 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:30:39 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:30:39 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:30:39 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:30:39 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:30:39 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:30:39 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:30:39 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:30:39 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:30:39 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:30:39 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:30:39 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:30:39 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:30:39 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:30:39 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:30:39 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:30:39 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:30:39 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-09 01:00:00 (before)
2024-12-09 02:00:00 (after)
20:30:41 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:30:41 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:30:41 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:30:41 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:30:41 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:30:41 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 23x23x2) for time before (2024-12-09 01:00:00)
20:30:41 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-09 01:00:00) in space (linearNDFast)
20:30:41 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:30:41 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:30:41 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.5034987086908 and -65.49266310750974 degrees.
20:30:41 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.5034987086908 and -65.49266310750974 degrees.
20:30:41 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:30:41 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:30:41 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:30:41 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:30:41 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:30:41 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:30:41 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0576887 (min) 0.0829642 (max)
20:30:41 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.178474 (min) 0.223884 (max)
20:30:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.355291 (min) -0.352205 (max)
20:30:41 DEBUG opendrift.models.basemodel.environment:905: x_wind: -2.46911 (min) -2.42724 (max)
20:30:41 DEBUG opendrift.models.basemodel.environment:905: y_wind: -4.41677 (min) -4.38233 (max)
20:30:41 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 3.98623e-05 (min) 4.0016e-05 (max)
20:30:41 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:30:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:30:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:30:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:30:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:30:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:30:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:30:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:30:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:30:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:30:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:30:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:30:41 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:30:41 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:30:41 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:30:41 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:30:41 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:30:41 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.485 (min) 298.718 (max)
20:30:41 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:30:41 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:30:41 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.617369, mean: 0.623941, max: 0.629801
20:30:41 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:30:41 DEBUG opendrift.models.physics_methods:1061: min: 4.280575, mean: 4.303293, max: 4.323457
20:30:41 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 4.280575, mean: 4.303293, max: 4.323457
20:30:41 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:30:41 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:30:41 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:30:41 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:30:41 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.100192 m/s - 0.101196 m/s)
20:30:41 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:30:41 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:30:41 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:30:41 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:30:41 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:30:41 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:30:41 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:30:41 INFO opendrift.models.basemodel:2035: 2024-12-09 02:00:00 - step 3 of 71 - 1000 active elements (0 deactivated)
20:30:41 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:30:41 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:30:41 DEBUG opendrift.models.basemodel:2054: 60.001874670094665 <- latitude -> 60.00884911829958
20:30:41 DEBUG opendrift.models.basemodel:2059: 4.497672933677941 <- longitude -> 4.509356268674435
20:30:41 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:30:41 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:30:41 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:30:41 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:30:41 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:30:41 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:30:41 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:30:41 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:30:41 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:30:41 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:30:41 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:30:41 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:30:41 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:30:41 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:30:41 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:30:41 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:30:41 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:30:41 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:30:41 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:30:41 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:30:41 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-09 02:00:00 (before)
2024-12-09 03:00:00 (after)
20:30:42 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:30:42 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:30:42 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:30:42 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:30:42 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:30:42 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 23x23x2) for time before (2024-12-09 02:00:00)
20:30:42 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-09 02:00:00) in space (linearNDFast)
20:30:42 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:30:42 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:30:42 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.50233798985666 and -65.49065465669653 degrees.
20:30:42 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.50233798985666 and -65.49065465669653 degrees.
20:30:42 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:30:42 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:30:42 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:30:42 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:30:42 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:30:42 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:30:42 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0579977 (min) 0.0883811 (max)
20:30:42 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.211589 (min) 0.259497 (max)
20:30:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.231026 (min) -0.227762 (max)
20:30:42 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.71464 (min) -1.69599 (max)
20:30:42 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.82932 (min) -3.80628 (max)
20:30:42 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 6.19455e-05 (min) 6.40052e-05 (max)
20:30:42 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:30:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:30:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:30:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:30:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:30:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:30:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:30:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:30:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:30:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:30:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:30:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:30:42 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:30:42 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:30:42 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:30:42 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:30:42 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:30:42 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.659 (min) 298.837 (max)
20:30:42 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:30:42 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:30:42 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.428525, mean: 0.429824, max: 0.431547
20:30:42 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:30:42 DEBUG opendrift.models.physics_methods:1061: min: 3.566297, mean: 3.571697, max: 3.578851
20:30:42 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.566297, mean: 3.571697, max: 3.578851
20:30:42 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:30:42 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:30:42 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:30:42 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:30:42 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.083474 m/s - 0.083768 m/s)
20:30:42 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:30:42 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:30:42 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:30:42 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:30:42 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:30:42 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:30:42 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:30:42 INFO opendrift.models.basemodel:2035: 2024-12-09 03:00:00 - step 4 of 71 - 1000 active elements (0 deactivated)
20:30:42 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:30:42 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:30:42 DEBUG opendrift.models.basemodel:2054: 60.006427186729354 <- latitude -> 60.01456012067315
20:30:42 DEBUG opendrift.models.basemodel:2059: 4.499535062480698 <- longitude -> 4.512666472955501
20:30:42 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:30:42 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:30:42 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:30:42 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:30:42 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:30:42 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:30:42 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:30:42 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:30:42 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:30:42 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:30:42 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:30:42 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:30:42 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:30:42 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:30:42 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:30:42 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:30:42 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:30:42 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:30:42 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:30:42 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:30:42 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-09 03:00:00 (before)
2024-12-09 04:00:00 (after)
20:30:43 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:30:43 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:30:43 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:30:43 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:30:43 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:30:43 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 23x23x2) for time before (2024-12-09 03:00:00)
20:30:43 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-09 03:00:00) in space (linearNDFast)
20:30:43 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:30:43 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:30:43 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.50047585185229 and -65.48734445583686 degrees.
20:30:43 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.50047585185229 and -65.48734445583686 degrees.
20:30:43 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:30:43 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:30:43 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:30:43 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:30:43 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:30:43 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:30:43 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0716486 (min) 0.117673 (max)
20:30:43 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.227704 (min) 0.282803 (max)
20:30:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.155143 (min) -0.150649 (max)
20:30:43 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.571639 (min) -0.545044 (max)
20:30:43 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.74355 (min) -3.68962 (max)
20:30:43 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 2.62006e-05 (min) 2.72465e-05 (max)
20:30:43 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:30:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:30:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:30:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:30:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:30:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:30:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:30:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:30:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:30:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:30:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:30:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:30:43 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:30:43 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:30:43 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:30:43 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:30:43 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:30:43 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.871 (min) 299.007 (max)
20:30:43 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:30:43 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:30:43 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.342195, mean: 0.347382, max: 0.352787
20:30:43 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:30:43 DEBUG opendrift.models.physics_methods:1061: min: 3.186885, mean: 3.210939, max: 3.235832
20:30:43 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.186885, mean: 3.210939, max: 3.235832
20:30:43 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:30:43 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:30:43 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:30:43 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:30:43 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.074593 m/s - 0.075739 m/s)
20:30:43 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:30:43 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:30:43 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:30:43 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:30:43 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:30:43 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:30:43 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:30:43 INFO opendrift.models.basemodel:2035: 2024-12-09 04:00:00 - step 5 of 71 - 1000 active elements (0 deactivated)
20:30:43 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:30:43 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:30:43 DEBUG opendrift.models.basemodel:2054: 60.01148450007494 <- latitude -> 60.021110137860376
20:30:43 DEBUG opendrift.models.basemodel:2059: 4.503608843525559 <- longitude -> 4.519484932178915
20:30:43 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:30:43 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:30:43 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:30:43 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:30:43 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:30:43 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:30:43 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:30:43 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:30:43 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:30:43 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:30:43 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:30:43 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:30:43 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:30:43 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:30:43 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:30:43 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:30:43 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:30:43 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:30:43 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:30:43 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:30:43 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-09 04:00:00 (before)
2024-12-09 05:00:00 (after)
20:30:44 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:30:44 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:30:44 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:30:44 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:30:44 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:30:44 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 23x23x2) for time before (2024-12-09 04:00:00)
20:30:44 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-09 04:00:00) in space (linearNDFast)
20:30:44 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:30:44 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:30:44 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.49640206906183 and -65.4805259821425 degrees.
20:30:44 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.49640206906183 and -65.4805259821425 degrees.
20:30:44 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:30:44 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:30:44 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:30:44 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:30:44 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:30:44 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:30:44 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0718497 (min) 0.135422 (max)
20:30:44 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.21375 (min) 0.283709 (max)
20:30:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.134602 (min) -0.129465 (max)
20:30:44 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.302421 (min) -0.218299 (max)
20:30:44 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.87818 (min) -3.80904 (max)
20:30:44 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -6.4234e-06 (min) -6.10086e-06 (max)
20:30:44 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:30:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:30:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:30:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:30:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:30:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:30:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:30:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:30:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:30:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:30:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:30:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:30:44 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:30:44 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:30:44 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:30:44 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:30:44 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:30:44 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 299.074 (min) 299.214 (max)
20:30:44 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:30:44 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:30:44 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.358088, mean: 0.365750, max: 0.372241
20:30:44 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:30:44 DEBUG opendrift.models.physics_methods:1061: min: 3.260050, mean: 3.294732, max: 3.323854
20:30:44 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.260050, mean: 3.294732, max: 3.323854
20:30:44 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:30:44 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:30:44 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:30:44 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:30:44 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.076306 m/s - 0.077799 m/s)
20:30:44 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:30:44 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:30:44 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:30:44 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:30:44 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:30:44 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:30:44 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:30:44 INFO opendrift.models.basemodel:2035: 2024-12-09 05:00:00 - step 6 of 71 - 1000 active elements (0 deactivated)
20:30:44 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:30:44 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:30:44 DEBUG opendrift.models.basemodel:2054: 60.015929384286046 <- latitude -> 60.0276231942277
20:30:44 DEBUG opendrift.models.basemodel:2059: 4.5079461556790745 <- longitude -> 4.527942814831888
20:30:44 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:30:44 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:30:44 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:30:44 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:30:44 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:30:44 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:30:44 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:30:44 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:30:44 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:30:44 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:30:44 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:30:44 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:30:44 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:30:44 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:30:44 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:30:44 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:30:44 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:30:44 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:30:44 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:30:44 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:30:44 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-09 05:00:00 (before)
2024-12-09 06:00:00 (after)
20:30:45 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:30:45 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:30:45 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:30:45 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:30:45 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:30:45 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 23x24x2) for time before (2024-12-09 05:00:00)
20:30:45 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-09 05:00:00) in space (linearNDFast)
20:30:45 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:30:45 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:30:45 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.4920647467938 and -65.47206809900207 degrees.
20:30:45 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.4920647467938 and -65.47206809900207 degrees.
20:30:45 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:30:45 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:30:45 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:30:45 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:30:45 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:30:45 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:30:45 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0378795 (min) 0.123987 (max)
20:30:45 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.187982 (min) 0.27097 (max)
20:30:45 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.182955 (min) -0.175751 (max)
20:30:45 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.361006 (min) -0.266699 (max)
20:30:45 DEBUG opendrift.models.basemodel.environment:905: y_wind: -4.39219 (min) -4.38151 (max)
20:30:45 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -2.25407e-05 (min) -2.21367e-05 (max)
20:30:45 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:30:45 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:30:45 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:30:45 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:30:45 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:30:45 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:30:45 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:30:45 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:30:45 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:30:45 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:30:45 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:30:45 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:30:45 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:30:45 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:30:45 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:30:45 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:30:45 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:30:45 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 299.218 (min) 299.415 (max)
20:30:45 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:30:45 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:30:45 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.474616, mean: 0.476364, max: 0.477088
20:30:45 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:30:45 DEBUG opendrift.models.physics_methods:1061: min: 3.753192, mean: 3.760098, max: 3.762955
20:30:45 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.753192, mean: 3.760098, max: 3.762955
20:30:45 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:30:45 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:30:46 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:30:46 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:30:46 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.087848 m/s - 0.088077 m/s)
20:30:46 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:30:46 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:30:46 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:30:46 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:30:46 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:30:46 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:30:46 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:30:46 INFO opendrift.models.basemodel:2035: 2024-12-09 06:00:00 - step 7 of 71 - 1000 active elements (0 deactivated)
20:30:46 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:30:46 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:30:46 DEBUG opendrift.models.basemodel:2054: 60.01916994352439 <- latitude -> 60.033403003734065
20:30:46 DEBUG opendrift.models.basemodel:2059: 4.509926378651621 <- longitude -> 4.5356029437962455
20:30:46 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:30:46 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:30:46 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:30:46 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:30:46 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:30:46 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:30:46 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:30:46 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:30:46 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:30:46 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:30:46 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:30:46 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:30:46 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:30:46 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:30:46 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:30:46 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:30:46 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:30:46 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:30:46 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:30:46 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:30:46 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-09 06:00:00 (before)
2024-12-09 07:00:00 (after)
20:30:47 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:30:47 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:30:47 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:30:47 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:30:47 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:30:47 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 24x25x2) for time before (2024-12-09 06:00:00)
20:30:47 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-09 06:00:00) in space (linearNDFast)
20:30:47 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:30:47 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:30:47 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.49008452969287 and -65.46440797634776 degrees.
20:30:47 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.49008452969287 and -65.46440797634776 degrees.
20:30:47 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:30:47 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:30:47 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:30:47 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:30:47 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:30:47 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:30:47 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.02829 (min) 0.0993821 (max)
20:30:47 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.182652 (min) 0.26924 (max)
20:30:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.30122 (min) -0.292731 (max)
20:30:47 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.26032 (min) -1.23703 (max)
20:30:47 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.40988 (min) -3.32579 (max)
20:30:47 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -3.99289e-05 (min) -3.93504e-05 (max)
20:30:47 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:30:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:30:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:30:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:30:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:30:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:30:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:30:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:30:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:30:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:30:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:30:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:30:47 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:30:47 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:30:47 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:30:47 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:30:47 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:30:47 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 299.28 (min) 299.571 (max)
20:30:47 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:30:47 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:30:47 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.311172, mean: 0.318760, max: 0.323871
20:30:47 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:30:47 DEBUG opendrift.models.physics_methods:1061: min: 3.038996, mean: 3.075808, max: 3.100384
20:30:47 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.038996, mean: 3.075808, max: 3.100384
20:30:47 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:30:47 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:30:47 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:30:47 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:30:47 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.071132 m/s - 0.072569 m/s)
20:30:47 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:30:47 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:30:47 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:30:47 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:30:47 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:30:47 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:30:47 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:30:47 INFO opendrift.models.basemodel:2035: 2024-12-09 07:00:00 - step 8 of 71 - 1000 active elements (0 deactivated)
20:30:47 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:30:47 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:30:47 DEBUG opendrift.models.basemodel:2054: 60.02292241188671 <- latitude -> 60.03978450514933
20:30:47 DEBUG opendrift.models.basemodel:2059: 4.506496678273472 <- longitude -> 4.5403922047790015
20:30:47 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:30:47 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:30:47 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:30:47 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:30:47 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:30:47 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:30:47 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:30:47 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:30:47 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:30:47 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:30:47 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:30:47 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:30:47 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:30:47 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:30:47 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:30:47 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:30:47 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:30:47 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:30:47 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:30:47 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:30:47 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-09 07:00:00 (before)
2024-12-09 08:00:00 (after)
20:30:48 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:30:48 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:30:48 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:30:48 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:30:48 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:30:48 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 23x25x2) for time before (2024-12-09 07:00:00)
20:30:48 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-09 07:00:00) in space (linearNDFast)
20:30:48 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:30:48 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:30:48 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.49351423194543 and -65.45961871523568 degrees.
20:30:48 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.49351423194543 and -65.45961871523568 degrees.
20:30:48 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:30:48 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:30:48 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:30:48 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:30:48 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:30:48 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:30:48 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.114132 (min) 0.0813691 (max)
20:30:48 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.20515 (min) 0.297217 (max)
20:30:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.456912 (min) -0.443962 (max)
20:30:48 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.536833 (min) -0.502157 (max)
20:30:48 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.73607 (min) -2.41245 (max)
20:30:48 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -4.62868e-05 (min) -4.43121e-05 (max)
20:30:48 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:30:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:30:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:30:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:30:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:30:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:30:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:30:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:30:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:30:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:30:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:30:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:30:48 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:30:48 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:30:48 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:30:48 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:30:48 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:30:48 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 299.189 (min) 299.675 (max)
20:30:48 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:30:48 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:30:48 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.150259, mean: 0.171899, max: 0.190382
20:30:48 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:30:48 DEBUG opendrift.models.physics_methods:1061: min: 2.111789, mean: 2.258367, max: 2.377073
20:30:48 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.111789, mean: 2.258367, max: 2.377073
20:30:48 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:30:48 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:30:48 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:30:48 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:30:48 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.049429 m/s - 0.055638 m/s)
20:30:48 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:30:48 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:30:48 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:30:48 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:30:48 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:30:48 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:30:48 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:30:48 INFO opendrift.models.basemodel:2035: 2024-12-09 08:00:00 - step 9 of 71 - 1000 active elements (0 deactivated)
20:30:48 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:30:48 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:30:48 DEBUG opendrift.models.basemodel:2054: 60.02799214484793 <- latitude -> 60.047619854943314
20:30:48 DEBUG opendrift.models.basemodel:2059: 4.4984723218978875 <- longitude -> 4.544953235167174
20:30:48 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:30:48 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:30:48 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:30:48 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:30:48 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:30:48 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:30:48 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:30:48 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:30:48 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:30:48 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:30:48 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:30:48 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:30:48 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:30:48 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:30:48 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:30:48 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:30:48 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:30:48 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:30:48 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:30:48 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:30:48 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-09 08:00:00 (before)
2024-12-09 09:00:00 (after)
20:30:49 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:30:49 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:30:49 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:30:49 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:30:49 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:30:49 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 23x26x2) for time before (2024-12-09 08:00:00)
20:30:49 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-09 08:00:00) in space (linearNDFast)
20:30:49 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:30:49 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:30:49 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.50153859133619 and -65.45505768921286 degrees.
20:30:49 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.50153859133619 and -65.45505768921286 degrees.
20:30:49 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:30:49 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:30:49 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:30:49 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:30:49 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:30:49 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:30:49 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.226995 (min) 0.0568298 (max)
20:30:49 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.240466 (min) 0.335084 (max)
20:30:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.611017 (min) -0.590327 (max)
20:30:49 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0.479521 (min) 0.582402 (max)
20:30:49 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.90338 (min) -2.59179 (max)
20:30:49 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -3.68284e-05 (min) -3.47561e-05 (max)
20:30:49 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:30:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:30:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:30:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:30:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:30:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:30:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:30:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:30:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:30:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:30:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:30:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:30:49 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:30:49 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:30:49 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:30:49 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:30:49 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:30:49 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.949 (min) 299.775 (max)
20:30:49 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:30:49 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:30:49 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.173592, mean: 0.195752, max: 0.213025
20:30:49 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:30:49 DEBUG opendrift.models.physics_methods:1061: min: 2.269835, mean: 2.410055, max: 2.514463
20:30:49 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.269835, mean: 2.410055, max: 2.514463
20:30:49 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:30:49 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:30:49 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:30:49 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:30:49 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.053128 m/s - 0.058854 m/s)
20:30:49 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:30:49 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:30:49 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:30:49 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:30:49 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:30:49 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:30:49 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:30:49 INFO opendrift.models.basemodel:2035: 2024-12-09 09:00:00 - step 10 of 71 - 1000 active elements (0 deactivated)
20:30:49 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:30:49 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:30:49 DEBUG opendrift.models.basemodel:2054: 60.03408715970647 <- latitude -> 60.05651020776513
20:30:49 DEBUG opendrift.models.basemodel:2059: 4.4844214358680645 <- longitude -> 4.549375898263872
20:30:49 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:30:49 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:30:49 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:30:49 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:30:49 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:30:49 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:30:49 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:30:49 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:30:49 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:30:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:30:49 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:30:49 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:30:49 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:30:49 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:30:49 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:30:49 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:30:49 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:30:49 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:30:49 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:30:49 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:30:49 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-09 09:00:00 (before)
2024-12-09 10:00:00 (after)
20:30:50 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:30:50 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:30:50 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:30:50 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:30:50 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:30:50 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 23x27x2) for time before (2024-12-09 09:00:00)
20:30:50 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-09 09:00:00) in space (linearNDFast)
20:30:50 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:30:50 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:30:50 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.51558947238051 and -65.45063501802926 degrees.
20:30:50 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.51558947238051 and -65.45063501802926 degrees.
20:30:50 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:30:50 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:30:50 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:30:50 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:30:50 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:30:50 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:30:50 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.367678 (min) 0.0181515 (max)
20:30:50 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.284566 (min) 0.3677 (max)
20:30:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.706917 (min) -0.675723 (max)
20:30:50 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0.868346 (min) 0.874854 (max)
20:30:50 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.65082 (min) -3.52415 (max)
20:30:50 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -1.78681e-05 (min) -1.43911e-05 (max)
20:30:50 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:30:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:30:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:30:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:30:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:30:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:30:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:30:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:30:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:30:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:30:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:30:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:30:50 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:30:50 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:30:50 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:30:50 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:30:50 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:30:50 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.465 (min) 299.861 (max)
20:30:50 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:30:50 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:30:50 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.324226, mean: 0.343157, max: 0.346448
20:30:50 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:30:50 DEBUG opendrift.models.physics_methods:1061: min: 3.102084, mean: 3.191319, max: 3.206627
20:30:50 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.102084, mean: 3.191319, max: 3.206627
20:30:50 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:30:50 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:30:50 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:30:50 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:30:50 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.072608 m/s - 0.075055 m/s)
20:30:50 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:30:50 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:30:50 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:30:50 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:30:50 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:30:50 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:30:50 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:30:50 INFO opendrift.models.basemodel:2035: 2024-12-09 10:00:00 - step 11 of 71 - 1000 active elements (0 deactivated)
20:30:50 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:30:50 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:30:50 DEBUG opendrift.models.basemodel:2054: 60.04100464611879 <- latitude -> 60.0652354867284
20:30:50 DEBUG opendrift.models.basemodel:2059: 4.46177444744454 <- longitude -> 4.551674976781974
20:30:50 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:30:50 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:30:50 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:30:50 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:30:50 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:30:50 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:30:50 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:30:50 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:30:50 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:30:50 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:30:50 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:30:50 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:30:50 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:30:50 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:30:50 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:30:50 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:30:50 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:30:50 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:30:50 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:30:50 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:30:50 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-09 10:00:00 (before)
2024-12-09 11:00:00 (after)
20:30:52 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:30:52 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:30:52 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:30:52 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:30:52 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:30:52 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 22x29x2) for time before (2024-12-09 10:00:00)
20:30:52 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-09 10:00:00) in space (linearNDFast)
20:30:52 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:30:52 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:30:52 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.53823646014548 and -65.4483359298045 degrees.
20:30:52 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.53823646014548 and -65.4483359298045 degrees.
20:30:52 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:30:52 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:30:52 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:30:52 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:30:52 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:30:52 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:30:52 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.489423 (min) -0.0602175 (max)
20:30:52 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.325246 (min) 0.412649 (max)
20:30:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.725769 (min) -0.681969 (max)
20:30:52 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0.188668 (min) 0.312312 (max)
20:30:52 DEBUG opendrift.models.basemodel.environment:905: y_wind: -4.1284 (min) -3.91107 (max)
20:30:52 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 1.00006e-06 (min) 3.90455e-06 (max)
20:30:52 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:30:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:30:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:30:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:30:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:30:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:30:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:30:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:30:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:30:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:30:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:30:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:30:52 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:30:52 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:30:52 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:30:52 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:30:52 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:30:52 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 297.603 (min) 299.915 (max)
20:30:52 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:30:52 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:30:52 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.377169, mean: 0.399094, max: 0.421675
20:30:52 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:30:52 DEBUG opendrift.models.physics_methods:1061: min: 3.345779, mean: 3.441536, max: 3.537678
20:30:52 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.345779, mean: 3.441536, max: 3.537678
20:30:52 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:30:52 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:30:52 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:30:52 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:30:52 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.078312 m/s - 0.082804 m/s)
20:30:52 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:30:52 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:30:52 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:30:52 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:30:52 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:30:52 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:30:52 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:30:52 INFO opendrift.models.basemodel:2035: 2024-12-09 11:00:00 - step 12 of 71 - 1000 active elements (0 deactivated)
20:30:52 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:30:52 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:30:52 DEBUG opendrift.models.basemodel:2054: 60.04964035331626 <- latitude -> 60.073204780719664
20:30:52 DEBUG opendrift.models.basemodel:2059: 4.430530274326379 <- longitude -> 4.548027655440524
20:30:52 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:30:52 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:30:52 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:30:52 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:30:52 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:30:52 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:30:52 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:30:52 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:30:52 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:30:52 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:30:52 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:30:52 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:30:52 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:30:52 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:30:52 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:30:52 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:30:52 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:30:52 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:30:52 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:30:52 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:30:52 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-09 11:00:00 (before)
2024-12-09 12:00:00 (after)
20:30:53 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:30:53 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:30:53 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:30:53 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:30:53 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:30:53 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 23x31x2) for time before (2024-12-09 11:00:00)
20:30:53 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-09 11:00:00) in space (linearNDFast)
20:30:53 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:30:53 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:30:53 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.56948063756609 and -65.45198324961974 degrees.
20:30:53 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.56948063756609 and -65.45198324961974 degrees.
20:30:53 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:30:53 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:30:53 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:30:53 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:30:53 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:30:53 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:30:53 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.560076 (min) -0.183748 (max)
20:30:53 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.25724 (min) 0.440957 (max)
20:30:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.669651 (min) -0.618415 (max)
20:30:53 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.295479 (min) -0.092748 (max)
20:30:53 DEBUG opendrift.models.basemodel.environment:905: y_wind: -4.34287 (min) -3.86708 (max)
20:30:53 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 2.44294e-05 (min) 2.65007e-05 (max)
20:30:53 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:30:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:30:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:30:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:30:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:30:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:30:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:30:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:30:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:30:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:30:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:30:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:30:53 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:30:53 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:30:53 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:30:53 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:30:53 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:30:53 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 296.252 (min) 299.884 (max)
20:30:53 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:30:53 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:30:53 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.370024, mean: 0.417240, max: 0.464180
20:30:53 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:30:53 DEBUG opendrift.models.physics_methods:1061: min: 3.313940, mean: 3.518429, max: 3.711701
20:30:53 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.313940, mean: 3.518429, max: 3.711701
20:30:53 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:30:53 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:30:53 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:30:53 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:30:53 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.077567 m/s - 0.086877 m/s)
20:30:53 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:30:53 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:30:53 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:30:53 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:30:53 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:30:53 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:30:53 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:30:53 INFO opendrift.models.basemodel:2035: 2024-12-09 12:00:00 - step 13 of 71 - 1000 active elements (0 deactivated)
20:30:53 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:30:53 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:30:53 DEBUG opendrift.models.basemodel:2054: 60.0595298874734 <- latitude -> 60.08069036084018
20:30:53 DEBUG opendrift.models.basemodel:2059: 4.394629551279647 <- longitude -> 4.535768736108945
20:30:53 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:30:53 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:30:53 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:30:53 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:30:53 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:30:53 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:30:53 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:30:53 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:30:53 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:30:53 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:30:53 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:30:53 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:30:53 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:30:53 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:30:53 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:30:53 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:30:53 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:30:53 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:30:53 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:30:53 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:30:53 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-09 12:00:00 (before)
2024-12-09 13:00:00 (after)
20:30:55 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:30:55 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:30:55 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:30:55 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:30:55 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:30:55 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 24x32x2) for time before (2024-12-09 12:00:00)
20:30:55 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-09 12:00:00) in space (linearNDFast)
20:30:55 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:30:55 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:30:55 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.60538135266174 and -65.4642421729941 degrees.
20:30:55 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.60538135266174 and -65.4642421729941 degrees.
20:30:55 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:30:55 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:30:55 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:30:55 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:30:55 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:30:55 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:30:55 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.615595 (min) -0.328291 (max)
20:30:55 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.110906 (min) 0.452548 (max)
20:30:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.553224 (min) -0.505182 (max)
20:30:55 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.688847 (min) -0.402382 (max)
20:30:55 DEBUG opendrift.models.basemodel.environment:905: y_wind: -4.78395 (min) -3.94696 (max)
20:30:55 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 3.67868e-05 (min) 4.03213e-05 (max)
20:30:55 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:30:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:30:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:30:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:30:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:30:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:30:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:30:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:30:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:30:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:30:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:30:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:30:55 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:30:55 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:30:55 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:30:55 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:30:55 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:30:55 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 294.502 (min) 299.69 (max)
20:30:55 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:30:55 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:30:55 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.394904, mean: 0.504410, max: 0.566983
20:30:55 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:30:55 DEBUG opendrift.models.physics_methods:1061: min: 3.423540, mean: 3.867703, max: 4.102179
20:30:55 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.423540, mean: 3.867703, max: 4.102179
20:30:55 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:30:55 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:30:55 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:30:55 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:30:55 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.080132 m/s - 0.096017 m/s)
20:30:55 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:30:55 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:30:55 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:30:55 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:30:55 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:30:55 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:30:55 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:30:55 INFO opendrift.models.basemodel:2035: 2024-12-09 13:00:00 - step 14 of 71 - 1000 active elements (0 deactivated)
20:30:55 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:30:55 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:30:55 DEBUG opendrift.models.basemodel:2054: 60.070610746358085 <- latitude -> 60.08803527530129
20:30:55 DEBUG opendrift.models.basemodel:2059: 4.361083753911169 <- longitude -> 4.51365105716442
20:30:55 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:30:55 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:30:55 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:30:55 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:30:55 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:30:55 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:30:55 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:30:55 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:30:55 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:30:55 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:30:55 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:30:55 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:30:55 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:30:55 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:30:55 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:30:55 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:30:55 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:30:55 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:30:55 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:30:55 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:30:55 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-09 13:00:00 (before)
2024-12-09 14:00:00 (after)
20:30:56 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:30:56 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:30:56 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:30:56 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:30:56 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:30:56 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 25x33x2) for time before (2024-12-09 13:00:00)
20:30:56 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-09 13:00:00) in space (linearNDFast)
20:30:56 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:30:56 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:30:56 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.63892715251441 and -65.4863598504384 degrees.
20:30:56 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.63892715251441 and -65.4863598504384 degrees.
20:30:56 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:30:56 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:30:56 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:30:56 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:30:56 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:30:56 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:30:56 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.654576 (min) -0.447853 (max)
20:30:56 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0409028 (min) 0.458163 (max)
20:30:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.415787 (min) -0.375261 (max)
20:30:56 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.22207 (min) -0.365829 (max)
20:30:56 DEBUG opendrift.models.basemodel.environment:905: y_wind: -4.93673 (min) -3.40006 (max)
20:30:56 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 3.66902e-05 (min) 4.06481e-05 (max)
20:30:56 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:30:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:30:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:30:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:30:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:30:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:30:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:30:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:30:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:30:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:30:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:30:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:30:56 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:30:56 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:30:56 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:30:56 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:30:56 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:30:56 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 293.16 (min) 299.138 (max)
20:30:56 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:30:56 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:30:56 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.321124, mean: 0.548503, max: 0.602827
20:30:56 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:30:56 DEBUG opendrift.models.physics_methods:1061: min: 3.087210, mean: 4.032085, max: 4.229860
20:30:56 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.087210, mean: 4.032085, max: 4.229860
20:30:56 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:30:56 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:30:56 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:30:56 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:30:56 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.072260 m/s - 0.099005 m/s)
20:30:56 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:30:56 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:30:56 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:30:56 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:30:56 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:30:56 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:30:56 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:30:56 INFO opendrift.models.basemodel:2035: 2024-12-09 14:00:00 - step 15 of 71 - 1000 active elements (0 deactivated)
20:30:56 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:30:56 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:30:56 DEBUG opendrift.models.basemodel:2054: 60.074546473582274 <- latitude -> 60.094343884755204
20:30:56 DEBUG opendrift.models.basemodel:2059: 4.331648911231125 <- longitude -> 4.480432855845975
20:30:56 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:30:56 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:30:56 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:30:56 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:30:56 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:30:56 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:30:56 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:30:56 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:30:56 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:30:56 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:30:56 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:30:56 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:30:56 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:30:56 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:30:56 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:30:56 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:30:56 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:30:56 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:30:56 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:30:56 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:30:56 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-09 14:00:00 (before)
2024-12-09 15:00:00 (after)
20:30:58 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:30:58 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:30:58 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:30:58 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:30:58 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:30:58 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 27x31x2) for time before (2024-12-09 14:00:00)
20:30:58 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-09 14:00:00) in space (linearNDFast)
20:30:58 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:30:58 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:30:58 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.66836200070512 and -65.51957805126895 degrees.
20:30:58 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.66836200070512 and -65.51957805126895 degrees.
20:30:58 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:30:58 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:30:58 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:30:58 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:30:58 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:30:58 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:30:58 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.681198 (min) -0.348572 (max)
20:30:58 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.195769 (min) 0.463803 (max)
20:30:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.290911 (min) -0.261681 (max)
20:30:58 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.778796 (min) 0.223257 (max)
20:30:58 DEBUG opendrift.models.basemodel.environment:905: y_wind: -4.82516 (min) -3.65577 (max)
20:30:58 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 2.84579e-05 (min) 3.15829e-05 (max)
20:30:58 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:30:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:30:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:30:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:30:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:30:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:30:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:30:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:30:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:30:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:30:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:30:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:30:58 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:30:58 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:30:58 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:30:58 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:30:58 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:30:58 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 291.824 (min) 297.862 (max)
20:30:58 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:30:58 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:30:58 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.343690, mean: 0.567957, max: 0.573319
20:30:58 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:30:58 DEBUG opendrift.models.physics_methods:1061: min: 3.193839, mean: 4.105217, max: 4.125035
20:30:58 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.193839, mean: 4.105217, max: 4.125035
20:30:58 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:30:58 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:30:58 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:30:58 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:30:58 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.074756 m/s - 0.096552 m/s)
20:30:58 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:30:58 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:30:58 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:30:58 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:30:58 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:30:58 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:30:58 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:30:58 INFO opendrift.models.basemodel:2035: 2024-12-09 15:00:00 - step 16 of 71 - 1000 active elements (0 deactivated)
20:30:58 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:30:58 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:30:58 DEBUG opendrift.models.basemodel:2054: 60.065119826756444 <- latitude -> 60.10027738966282
20:30:58 DEBUG opendrift.models.basemodel:2059: 4.30940262425346 <- longitude -> 4.438517198576622
20:30:58 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:30:58 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:30:58 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:30:58 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:30:58 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:30:58 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:30:58 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:30:58 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:30:58 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:30:58 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:30:58 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:30:58 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:30:58 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:30:58 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:30:58 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:30:58 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:30:58 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:30:58 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:30:58 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:30:58 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:30:58 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-09 15:00:00 (before)
2024-12-09 16:00:00 (after)
20:30:59 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:30:59 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:30:59 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:30:59 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:30:59 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:30:59 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 29x28x2) for time before (2024-12-09 15:00:00)
20:30:59 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-09 15:00:00) in space (linearNDFast)
20:30:59 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:30:59 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:30:59 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.69060829766286 and -65.56149369686497 degrees.
20:30:59 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.69060829766286 and -65.56149369686497 degrees.
20:30:59 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:30:59 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:30:59 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:30:59 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:30:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:30:59 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:30:59 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.6834 (min) -0.252529 (max)
20:30:59 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.324246 (min) 0.394982 (max)
20:30:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.203593 (min) -0.182319 (max)
20:30:59 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0.277435 (min) 0.373005 (max)
20:30:59 DEBUG opendrift.models.basemodel.environment:905: y_wind: -5.29401 (min) -5.05972 (max)
20:30:59 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 1.68841e-05 (min) 1.89463e-05 (max)
20:30:59 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:30:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:30:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:30:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:30:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:30:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:30:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:30:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:30:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:30:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:30:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:30:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:30:59 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:30:59 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:30:59 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:30:59 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:30:59 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:30:59 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 290.625 (min) 295.402 (max)
20:30:59 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:30:59 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:30:59 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.632825, mean: 0.638099, max: 0.691347
20:30:59 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:30:59 DEBUG opendrift.models.physics_methods:1061: min: 4.333823, mean: 4.351773, max: 4.529784
20:30:59 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 4.333823, mean: 4.351773, max: 4.529784
20:30:59 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:30:59 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:30:59 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:30:59 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:30:59 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.101439 m/s - 0.106026 m/s)
20:30:59 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:30:59 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:30:59 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:30:59 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:30:59 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:30:59 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:30:59 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:30:59 INFO opendrift.models.basemodel:2035: 2024-12-09 16:00:00 - step 17 of 71 - 1000 active elements (0 deactivated)
20:30:59 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:30:59 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:30:59 DEBUG opendrift.models.basemodel:2054: 60.0513639327341 <- latitude -> 60.10538962015637
20:30:59 DEBUG opendrift.models.basemodel:2059: 4.29354746714102 <- longitude -> 4.394640986114139
20:30:59 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:30:59 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:30:59 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:30:59 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:30:59 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:30:59 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:30:59 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:30:59 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:30:59 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:30:59 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:30:59 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:30:59 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:30:59 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:30:59 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:30:59 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:30:59 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:30:59 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:30:59 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:30:59 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:30:59 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:30:59 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-09 16:00:00 (before)
2024-12-09 17:00:00 (after)
20:31:01 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:31:01 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:31:01 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:31:01 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:31:01 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:31:01 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 31x26x2) for time before (2024-12-09 16:00:00)
20:31:01 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-09 16:00:00) in space (linearNDFast)
20:31:01 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:31:01 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:31:01 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.7064634611654 and -65.60536991003036 degrees.
20:31:01 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.7064634611654 and -65.60536991003036 degrees.
20:31:01 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:01 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:31:01 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:31:01 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:31:01 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:31:01 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:31:01 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.629556 (min) -0.178619 (max)
20:31:01 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.402534 (min) 0.190883 (max)
20:31:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.165292 (min) -0.14841 (max)
20:31:01 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.146268 (min) 0.0526836 (max)
20:31:01 DEBUG opendrift.models.basemodel.environment:905: y_wind: -4.64684 (min) -4.61659 (max)
20:31:01 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -2.24402e-07 (min) 1.63394e-06 (max)
20:31:01 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:31:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:31:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:31:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:31:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:31:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:31:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:31:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:31:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:31:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:31:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:31:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:31:01 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:31:01 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:31:01 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:31:01 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:31:01 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:31:01 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 289.77 (min) 295.49 (max)
20:31:01 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:31:01 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:31:01 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.524359, mean: 0.526897, max: 0.531196
20:31:01 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:31:01 DEBUG opendrift.models.physics_methods:1061: min: 3.944973, mean: 3.954501, max: 3.970608
20:31:01 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.944973, mean: 3.954501, max: 3.970608
20:31:01 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:31:01 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:01 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:31:01 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:31:01 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.092337 m/s - 0.092937 m/s)
20:31:01 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:31:01 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:31:01 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:31:01 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:31:01 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:31:01 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:01 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:31:01 INFO opendrift.models.basemodel:2035: 2024-12-09 17:00:00 - step 18 of 71 - 1000 active elements (0 deactivated)
20:31:01 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:31:01 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:31:01 DEBUG opendrift.models.basemodel:2054: 60.035367668527314 <- latitude -> 60.10832894945364
20:31:01 DEBUG opendrift.models.basemodel:2059: 4.282005857547621 <- longitude -> 4.353698131468008
20:31:01 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:31:01 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:31:01 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:01 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:31:01 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:01 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:31:01 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:01 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:01 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:31:01 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:31:01 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:31:01 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:31:01 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:01 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:01 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:31:01 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:01 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:31:01 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:01 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:01 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:31:01 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-09 17:00:00 (before)
2024-12-09 18:00:00 (after)
20:31:02 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:31:02 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:31:02 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:31:02 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:31:02 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:31:02 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 33x23x2) for time before (2024-12-09 17:00:00)
20:31:02 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-09 17:00:00) in space (linearNDFast)
20:31:02 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:31:02 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:31:02 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.71800508581168 and -65.64631277307059 degrees.
20:31:02 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.71800508581168 and -65.64631277307059 degrees.
20:31:02 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:02 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:31:02 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:31:02 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:31:02 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:31:02 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:31:02 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.509633 (min) -0.103359 (max)
20:31:02 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.436623 (min) -0.0739309 (max)
20:31:02 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.196666 (min) -0.18333 (max)
20:31:02 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0.0575094 (min) 0.256275 (max)
20:31:02 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.90776 (min) -3.85778 (max)
20:31:02 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -2.27928e-05 (min) -1.98179e-05 (max)
20:31:02 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:31:02 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:31:02 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:31:02 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:31:02 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:31:02 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:31:02 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:31:02 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:31:02 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:31:02 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:31:02 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:31:02 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:31:02 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:31:02 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:31:02 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:31:02 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:31:02 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:31:02 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 288.994 (min) 296.966 (max)
20:31:02 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:31:02 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:31:02 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.366191, mean: 0.372809, max: 0.377273
20:31:02 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:31:02 DEBUG opendrift.models.physics_methods:1061: min: 3.296730, mean: 3.326382, max: 3.346242
20:31:02 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.296730, mean: 3.326382, max: 3.346242
20:31:02 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:31:02 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:02 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:31:02 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:31:02 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.077164 m/s - 0.078323 m/s)
20:31:02 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:31:02 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:31:02 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:31:02 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:31:02 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:31:02 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:02 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:31:02 INFO opendrift.models.basemodel:2035: 2024-12-09 18:00:00 - step 19 of 71 - 1000 active elements (0 deactivated)
20:31:02 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:31:02 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:31:02 DEBUG opendrift.models.basemodel:2054: 60.019695460790814 <- latitude -> 60.1034429448826
20:31:02 DEBUG opendrift.models.basemodel:2059: 4.275576944974855 <- longitude -> 4.320787523767518
20:31:02 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:31:02 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:31:02 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:02 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:31:02 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:02 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:31:02 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:02 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:02 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:31:02 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:31:02 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:31:02 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:31:02 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:02 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:02 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:31:02 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:02 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:31:02 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:02 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:02 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:31:02 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-09 18:00:00 (before)
2024-12-09 19:00:00 (after)
20:31:04 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:31:04 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:31:04 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:31:04 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:31:04 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:31:04 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 34x24x2) for time before (2024-12-09 18:00:00)
20:31:04 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-09 18:00:00) in space (linearNDFast)
20:31:04 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:31:04 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:31:04 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.72443398717321 and -65.67922337879419 degrees.
20:31:04 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.72443398717321 and -65.67922337879419 degrees.
20:31:04 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:04 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:31:04 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:31:04 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:31:04 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:31:04 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:31:04 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.365616 (min) -0.0505865 (max)
20:31:04 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.439361 (min) -0.256415 (max)
20:31:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.301402 (min) -0.294077 (max)
20:31:04 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0.608526 (min) 0.668137 (max)
20:31:04 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.4376 (min) -3.40617 (max)
20:31:04 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -4.17909e-05 (min) -3.86806e-05 (max)
20:31:04 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:31:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:31:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:31:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:31:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:31:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:31:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:31:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:31:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:31:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:31:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:31:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:31:04 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:31:04 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:31:04 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:31:04 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:31:04 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:31:04 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 288.832 (min) 298.109 (max)
20:31:04 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:31:04 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:31:04 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.295707, mean: 0.296616, max: 0.301655
20:31:04 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:31:04 DEBUG opendrift.models.physics_methods:1061: min: 2.962515, mean: 2.967063, max: 2.992159
20:31:04 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.962515, mean: 2.967063, max: 2.992159
20:31:04 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:31:04 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:04 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:31:04 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:31:04 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.069342 m/s - 0.070035 m/s)
20:31:04 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:31:04 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:31:04 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:31:04 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:31:04 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:31:04 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:04 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:31:04 INFO opendrift.models.basemodel:2035: 2024-12-09 19:00:00 - step 20 of 71 - 1000 active elements (0 deactivated)
20:31:04 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:31:04 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:31:04 DEBUG opendrift.models.basemodel:2054: 60.00513343455227 <- latitude -> 60.09293505736659
20:31:04 DEBUG opendrift.models.basemodel:2059: 4.273097891955547 <- longitude -> 4.297982581519966
20:31:04 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:31:04 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:31:04 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:04 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:31:04 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:04 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:31:04 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:04 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:04 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:31:04 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:31:04 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:31:04 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:31:04 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:04 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:04 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:31:04 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:04 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:31:04 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:04 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:04 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:31:04 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-09 19:00:00 (before)
2024-12-09 20:00:00 (after)
20:31:05 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:31:05 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:31:05 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:31:05 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:31:05 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:31:05 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 34x25x2) for time before (2024-12-09 19:00:00)
20:31:05 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-09 19:00:00) in space (linearNDFast)
20:31:05 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:31:05 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:31:05 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.72691305078001 and -65.70202833116032 degrees.
20:31:05 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.72691305078001 and -65.70202833116032 degrees.
20:31:05 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:05 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:31:05 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:31:05 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:31:05 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:31:05 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:31:05 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.252151 (min) 0.015247 (max)
20:31:05 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.434084 (min) -0.303039 (max)
20:31:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.469314 (min) -0.460141 (max)
20:31:05 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.04555 (min) 1.10492 (max)
20:31:05 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.19084 (min) -3.07594 (max)
20:31:05 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -5.36334e-05 (min) -5.07347e-05 (max)
20:31:05 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:31:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:31:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:31:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:31:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:31:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:31:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:31:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:31:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:31:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:31:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:31:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:31:05 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:31:05 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:31:05 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:31:05 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:31:05 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:31:05 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 290.259 (min) 298.627 (max)
20:31:05 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:31:05 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:31:05 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.261484, mean: 0.267591, max: 0.277356
20:31:05 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:31:05 DEBUG opendrift.models.physics_methods:1061: min: 2.785814, mean: 2.818099, max: 2.869120
20:31:05 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.785814, mean: 2.818099, max: 2.869120
20:31:05 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:31:05 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:05 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:31:05 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:31:05 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.065206 m/s - 0.067155 m/s)
20:31:05 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:31:05 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:31:05 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:31:05 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:31:05 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:31:05 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:05 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:31:05 INFO opendrift.models.basemodel:2035: 2024-12-09 20:00:00 - step 21 of 71 - 1000 active elements (0 deactivated)
20:31:05 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:31:05 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:31:05 DEBUG opendrift.models.basemodel:2054: 59.99327941885326 <- latitude -> 60.079665509897204
20:31:05 DEBUG opendrift.models.basemodel:2059: 4.273613163141585 <- longitude -> 4.2831036927215616
20:31:05 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:31:05 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:31:05 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:05 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:31:05 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:05 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:31:05 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:05 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:05 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:31:05 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:31:05 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:31:05 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:31:05 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:05 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:05 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:31:05 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:05 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:31:05 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:05 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:05 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:31:05 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-09 20:00:00 (before)
2024-12-09 21:00:00 (after)
20:31:06 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:31:06 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:31:06 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:31:06 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:31:06 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:31:06 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 33x27x2) for time before (2024-12-09 20:00:00)
20:31:06 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-09 20:00:00) in space (linearNDFast)
20:31:06 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:31:06 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:31:06 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.7263977624324 and -65.71690722198349 degrees.
20:31:06 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.7263977624324 and -65.71690722198349 degrees.
20:31:06 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:06 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:31:06 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:31:06 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:31:06 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:31:06 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:31:06 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.136505 (min) 0.0818955 (max)
20:31:06 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.404399 (min) -0.218741 (max)
20:31:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.659389 (min) -0.642823 (max)
20:31:06 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.53236 (min) 1.71922 (max)
20:31:06 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.14954 (min) -2.80597 (max)
20:31:06 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -5.12586e-05 (min) -4.8961e-05 (max)
20:31:06 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:31:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:31:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:31:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:31:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:31:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:31:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:31:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:31:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:31:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:31:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:31:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:31:06 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:31:06 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:31:06 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:31:06 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:31:06 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:31:06 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 291.984 (min) 298.663 (max)
20:31:06 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:31:06 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:31:06 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.266397, mean: 0.287038, max: 0.301786
20:31:06 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:31:06 DEBUG opendrift.models.physics_methods:1061: min: 2.811864, mean: 2.918637, max: 2.992810
20:31:06 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.811864, mean: 2.918637, max: 2.992810
20:31:06 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:31:06 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:06 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:31:06 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:31:06 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.065815 m/s - 0.070051 m/s)
20:31:06 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:31:06 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:31:06 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:31:06 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:31:06 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:31:06 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:06 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:31:06 INFO opendrift.models.basemodel:2035: 2024-12-09 21:00:00 - step 22 of 71 - 1000 active elements (0 deactivated)
20:31:06 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:31:06 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:31:06 DEBUG opendrift.models.basemodel:2054: 59.98417584043732 <- latitude -> 60.06559626083046
20:31:06 DEBUG opendrift.models.basemodel:2059: 4.275370004591793 <- longitude -> 4.282687935188694
20:31:06 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:31:06 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:31:06 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:06 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:31:06 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:06 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:31:06 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:06 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:06 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:31:06 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:31:06 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:31:06 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:31:06 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:06 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:06 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:31:06 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:06 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:31:06 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:06 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:06 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:31:06 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-09 21:00:00 (before)
2024-12-09 22:00:00 (after)
20:31:07 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:31:07 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:31:07 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:31:07 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:31:07 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:31:07 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 32x27x2) for time before (2024-12-09 21:00:00)
20:31:07 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-09 21:00:00) in space (linearNDFast)
20:31:07 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:31:07 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:31:07 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.72464093325453 and -65.71732303038976 degrees.
20:31:07 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.72464093325453 and -65.71732303038976 degrees.
20:31:07 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:07 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:31:07 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:31:07 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:31:07 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:31:07 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:31:07 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0139631 (min) 0.140172 (max)
20:31:07 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.366237 (min) -0.142342 (max)
20:31:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.820714 (min) -0.797607 (max)
20:31:07 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.93749 (min) 2.03135 (max)
20:31:07 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.14737 (min) -2.60954 (max)
20:31:07 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -3.70092e-05 (min) -3.54072e-05 (max)
20:31:07 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:31:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:31:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:31:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:31:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:31:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:31:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:31:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:31:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:31:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:31:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:31:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:31:07 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:31:07 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:31:07 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:31:07 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:31:07 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:31:07 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 293.625 (min) 298.675 (max)
20:31:07 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:31:07 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:31:07 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.259863, mean: 0.317184, max: 0.345068
20:31:07 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:31:07 DEBUG opendrift.models.physics_methods:1061: min: 2.777167, mean: 3.067641, max: 3.200235
20:31:07 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.777167, mean: 3.067641, max: 3.200235
20:31:07 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:31:07 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:07 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:31:07 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:31:07 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.065003 m/s - 0.074906 m/s)
20:31:07 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:31:07 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:31:07 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:31:07 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:31:07 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:31:07 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:07 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:31:07 INFO opendrift.models.basemodel:2035: 2024-12-09 22:00:00 - step 23 of 71 - 1000 active elements (0 deactivated)
20:31:07 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:31:07 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:31:07 DEBUG opendrift.models.basemodel:2054: 59.977542102220404 <- latitude -> 60.0525799425688
20:31:07 DEBUG opendrift.models.basemodel:2059: 4.278103343244821 <- longitude -> 4.294343382144437
20:31:07 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:31:07 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:31:07 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:07 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:31:07 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:07 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:31:07 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:07 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:07 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:31:07 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:31:07 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:31:07 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:31:07 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:07 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:07 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:31:07 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:07 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:31:07 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:07 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:07 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:31:07 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-09 22:00:00 (before)
2024-12-09 23:00:00 (after)
20:31:08 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:31:08 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:31:08 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:31:08 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:31:08 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:31:08 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 31x27x2) for time before (2024-12-09 22:00:00)
20:31:08 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-09 22:00:00) in space (linearNDFast)
20:31:08 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:31:08 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:31:08 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.72190758264487 and -65.7056675601461 degrees.
20:31:08 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.72190758264487 and -65.7056675601461 degrees.
20:31:08 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:08 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:31:08 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:31:08 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:31:08 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:31:08 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:31:08 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0900059 (min) 0.172615 (max)
20:31:08 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.320323 (min) -0.0458037 (max)
20:31:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.909422 (min) -0.882194 (max)
20:31:08 DEBUG opendrift.models.basemodel.environment:905: x_wind: 2.01209 (min) 2.19797 (max)
20:31:08 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.72774 (min) -2.50868 (max)
20:31:08 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -1.20299e-05 (min) -1.10979e-05 (max)
20:31:08 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:31:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:31:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:31:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:31:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:31:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:31:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:31:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:31:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:31:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:31:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:31:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:31:08 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:31:08 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:31:08 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:31:08 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:31:08 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:31:08 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 295.116 (min) 298.694 (max)
20:31:08 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:31:08 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:31:08 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.273664, mean: 0.280858, max: 0.282631
20:31:08 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:31:08 DEBUG opendrift.models.physics_methods:1061: min: 2.849957, mean: 2.887173, max: 2.896273
20:31:08 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.849957, mean: 2.887173, max: 2.896273
20:31:08 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:31:08 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:08 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:31:08 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:31:08 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.066707 m/s - 0.067791 m/s)
20:31:08 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:31:08 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:31:08 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:31:08 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:31:08 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:31:08 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:08 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:31:08 INFO opendrift.models.basemodel:2035: 2024-12-09 23:00:00 - step 24 of 71 - 1000 active elements (0 deactivated)
20:31:08 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:31:08 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:31:08 DEBUG opendrift.models.basemodel:2054: 59.974298769127074 <- latitude -> 60.040768753316485
20:31:08 DEBUG opendrift.models.basemodel:2059: 4.286757204021537 <- longitude -> 4.308066017242352
20:31:08 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:31:08 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:31:08 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:08 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:31:08 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:08 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:31:08 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:08 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:08 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:31:08 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:31:08 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:31:08 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:31:08 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:08 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:08 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:31:08 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:08 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:31:08 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:08 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:08 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:31:08 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-09 23:00:00 (before)
2024-12-10 00:00:00 (after)
20:31:10 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:31:10 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:31:10 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:31:10 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:31:10 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:31:10 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 30x27x2) for time before (2024-12-09 23:00:00)
20:31:10 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-09 23:00:00) in space (linearNDFast)
20:31:10 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:31:10 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:31:10 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.71325372210507 and -65.6919449293655 degrees.
20:31:10 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.71325372210507 and -65.6919449293655 degrees.
20:31:10 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:10 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:31:10 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:31:10 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:31:10 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:31:10 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:31:10 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.130225 (min) 0.174327 (max)
20:31:10 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.257371 (min) 0.0677776 (max)
20:31:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.901312 (min) -0.873856 (max)
20:31:10 DEBUG opendrift.models.basemodel.environment:905: x_wind: 2.18691 (min) 2.36558 (max)
20:31:10 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.49071 (min) -2.11509 (max)
20:31:10 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 1.37792e-05 (min) 1.46503e-05 (max)
20:31:10 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:31:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:31:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:31:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:31:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:31:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:31:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:31:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:31:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:31:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:31:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:31:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:31:10 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:31:10 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:31:10 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:31:10 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:31:10 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:31:10 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 296.518 (min) 298.728 (max)
20:31:10 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:31:10 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:31:10 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.247712, mean: 0.259007, max: 0.270260
20:31:10 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:31:10 DEBUG opendrift.models.physics_methods:1061: min: 2.711458, mean: 2.772530, max: 2.832179
20:31:10 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.711458, mean: 2.772530, max: 2.832179
20:31:10 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:31:10 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:10 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:31:10 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:31:10 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.063465 m/s - 0.066291 m/s)
20:31:10 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:31:10 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:31:10 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:31:10 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:31:10 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:31:10 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:10 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:31:10 INFO opendrift.models.basemodel:2035: 2024-12-10 00:00:00 - step 25 of 71 - 1000 active elements (0 deactivated)
20:31:10 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:31:10 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:31:10 DEBUG opendrift.models.basemodel:2054: 59.974878705325665 <- latitude -> 60.03108528301439
20:31:10 DEBUG opendrift.models.basemodel:2059: 4.299097419999761 <- longitude -> 4.32212461088948
20:31:10 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:31:10 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:31:10 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:10 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:31:10 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:10 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:31:10 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:10 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:10 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:31:10 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:31:10 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:31:10 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:31:10 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:10 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:10 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:31:10 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:10 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:31:10 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:10 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:10 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:31:10 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-10 00:00:00 (before)
2024-12-10 01:00:00 (after)
20:31:11 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:31:11 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:31:11 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:31:11 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:31:11 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:31:11 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 28x27x2) for time before (2024-12-10 00:00:00)
20:31:11 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-10 00:00:00) in space (linearNDFast)
20:31:11 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:31:11 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:31:11 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.7009135022708 and -65.67788633381994 degrees.
20:31:11 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.7009135022708 and -65.67788633381994 degrees.
20:31:11 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:11 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:31:11 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:31:11 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:31:11 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:31:11 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:31:11 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.124033 (min) 0.226261 (max)
20:31:11 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.177946 (min) 0.214245 (max)
20:31:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.813544 (min) -0.789659 (max)
20:31:11 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.84841 (min) 2.16592 (max)
20:31:11 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.3419 (min) -1.68622 (max)
20:31:11 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -4.25239e-07 (min) 3.10015e-07 (max)
20:31:11 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:31:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:31:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:31:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:31:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:31:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:31:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:31:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:31:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:31:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:31:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:31:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:31:11 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:31:11 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:31:11 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:31:11 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:31:11 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:31:11 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 296.738 (min) 298.75 (max)
20:31:11 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:31:11 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:31:11 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.153994, mean: 0.219858, max: 0.250323
20:31:11 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:31:11 DEBUG opendrift.models.physics_methods:1061: min: 2.137873, mean: 2.553486, max: 2.725711
20:31:11 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.137873, mean: 2.553486, max: 2.725711
20:31:11 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:31:11 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:11 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:31:11 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:31:11 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.050040 m/s - 0.063799 m/s)
20:31:11 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:31:11 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:31:11 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:31:11 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:31:11 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:31:11 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:11 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:31:11 INFO opendrift.models.basemodel:2035: 2024-12-10 01:00:00 - step 26 of 71 - 1000 active elements (0 deactivated)
20:31:11 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:31:11 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:31:11 DEBUG opendrift.models.basemodel:2054: 59.9793511592338 <- latitude -> 60.02424535563048
20:31:11 DEBUG opendrift.models.basemodel:2059: 4.311108233717329 <- longitude -> 4.339507128058975
20:31:11 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:31:11 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:31:11 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:11 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:31:11 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:11 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:31:11 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:11 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:11 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:31:11 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:31:11 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:31:11 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:31:11 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:11 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:11 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:31:11 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:11 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:31:11 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:11 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:11 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:31:11 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-10 01:00:00 (before)
2024-12-10 02:00:00 (after)
20:31:12 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:31:12 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:31:12 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:31:12 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:31:12 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:31:12 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 28x27x2) for time before (2024-12-10 01:00:00)
20:31:12 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-10 01:00:00) in space (linearNDFast)
20:31:12 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:31:12 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:31:12 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.68890269298761 and -65.6605038188033 degrees.
20:31:12 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.68890269298761 and -65.6605038188033 degrees.
20:31:12 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:12 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:31:12 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:31:12 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:31:12 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:31:12 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:31:12 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.114802 (min) 0.307224 (max)
20:31:12 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.114516 (min) 0.324113 (max)
20:31:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.678351 (min) -0.660441 (max)
20:31:12 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.61089 (min) 1.90993 (max)
20:31:12 DEBUG opendrift.models.basemodel.environment:905: y_wind: -0.970712 (min) -0.73051 (max)
20:31:12 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 3.77604e-05 (min) 4.01355e-05 (max)
20:31:12 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:31:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:31:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:31:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:31:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:31:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:31:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:31:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:31:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:31:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:31:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:31:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:31:12 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:31:12 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:31:12 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:31:12 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:31:12 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:31:12 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 297.196 (min) 298.803 (max)
20:31:12 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:31:12 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:31:12 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.087016, mean: 0.092604, max: 0.102864
20:31:12 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:31:12 DEBUG opendrift.models.physics_methods:1061: min: 1.607049, mean: 1.657745, max: 1.747276
20:31:12 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.607049, mean: 1.657745, max: 1.747276
20:31:12 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:31:12 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:12 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:31:12 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:31:12 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.037615 m/s - 0.040897 m/s)
20:31:12 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:31:12 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:31:12 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:31:12 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:31:12 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:31:12 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:12 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:31:12 INFO opendrift.models.basemodel:2035: 2024-12-10 02:00:00 - step 27 of 71 - 1000 active elements (0 deactivated)
20:31:12 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:31:12 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:31:12 DEBUG opendrift.models.basemodel:2054: 59.98680535612286 <- latitude -> 60.02007265364336
20:31:12 DEBUG opendrift.models.basemodel:2059: 4.322807863960096 <- longitude -> 4.361400479757478
20:31:12 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:31:12 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:31:12 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:12 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:31:12 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:12 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:31:12 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:12 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:12 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:31:12 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:31:12 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:31:12 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:31:12 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:12 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:12 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:31:12 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:12 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:31:12 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:12 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:12 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:31:12 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-10 02:00:00 (before)
2024-12-10 03:00:00 (after)
20:31:14 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:31:14 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:31:14 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:31:14 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:31:14 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:31:14 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 26x27x2) for time before (2024-12-10 02:00:00)
20:31:14 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-10 02:00:00) in space (linearNDFast)
20:31:14 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:31:14 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:31:14 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.67720306491766 and -65.63861046429945 degrees.
20:31:14 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.67720306491766 and -65.63861046429945 degrees.
20:31:14 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:14 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:31:14 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:31:14 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:31:14 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:31:14 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:31:14 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.123399 (min) 0.345228 (max)
20:31:14 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0288631 (min) 0.354477 (max)
20:31:14 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.534909 (min) -0.52137 (max)
20:31:14 DEBUG opendrift.models.basemodel.environment:905: x_wind: 3.04105 (min) 3.52605 (max)
20:31:14 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.48737 (min) -2.13674 (max)
20:31:14 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 6.0152e-05 (min) 6.21826e-05 (max)
20:31:14 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:31:14 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:31:14 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:31:14 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:31:14 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:31:14 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:31:14 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:31:14 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:31:14 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:31:14 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:31:14 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:31:14 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:31:14 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:31:14 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:31:14 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:31:14 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:31:14 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:31:14 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 297.802 (min) 298.818 (max)
20:31:14 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:31:14 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:31:14 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.339815, mean: 0.408599, max: 0.455902
20:31:14 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:31:14 DEBUG opendrift.models.physics_methods:1061: min: 3.175784, mean: 3.481446, max: 3.678453
20:31:14 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.175784, mean: 3.481446, max: 3.678453
20:31:14 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:31:14 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:14 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:31:14 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:31:14 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.074333 m/s - 0.086099 m/s)
20:31:14 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:31:14 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:31:14 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:31:14 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:31:14 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:31:14 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:14 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:31:14 INFO opendrift.models.basemodel:2035: 2024-12-10 03:00:00 - step 28 of 71 - 1000 active elements (0 deactivated)
20:31:14 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:31:14 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:31:14 DEBUG opendrift.models.basemodel:2054: 59.99043994739135 <- latitude -> 60.017543634878606
20:31:14 DEBUG opendrift.models.basemodel:2059: 4.3355184215861975 <- longitude -> 4.387598285663547
20:31:14 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:31:14 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:31:14 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:14 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:31:14 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:14 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:31:14 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:14 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:14 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:31:14 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:31:14 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:31:14 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:31:14 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:14 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:14 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:31:14 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:14 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:31:14 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:14 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:14 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:31:14 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-10 03:00:00 (before)
2024-12-10 04:00:00 (after)
20:31:15 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:31:15 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:31:15 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:31:15 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:31:15 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:31:15 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 25x27x2) for time before (2024-12-10 03:00:00)
20:31:15 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-10 03:00:00) in space (linearNDFast)
20:31:15 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:31:15 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:31:15 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.66449251221015 and -65.61241264538614 degrees.
20:31:15 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.66449251221015 and -65.61241264538614 degrees.
20:31:15 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:15 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:31:15 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:31:15 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:31:15 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:31:15 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:31:15 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.1342 (min) 0.386588 (max)
20:31:15 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.00270481 (min) 0.382203 (max)
20:31:15 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.396113 (min) -0.383951 (max)
20:31:15 DEBUG opendrift.models.basemodel.environment:905: x_wind: 3.08978 (min) 3.29803 (max)
20:31:15 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.08992 (min) -2.9539 (max)
20:31:15 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 3.01918e-05 (min) 3.50487e-05 (max)
20:31:15 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:31:15 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:31:15 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:31:15 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:31:15 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:31:15 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:31:15 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:31:15 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:31:15 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:31:15 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:31:15 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:31:15 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:31:15 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:31:15 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:31:15 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:31:15 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:31:15 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:31:15 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.062 (min) 298.852 (max)
20:31:15 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:31:15 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:31:15 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.461545, mean: 0.479489, max: 0.484699
20:31:15 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:31:15 DEBUG opendrift.models.physics_methods:1061: min: 3.701150, mean: 3.772377, max: 3.792850
20:31:15 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.701150, mean: 3.772377, max: 3.792850
20:31:15 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:31:15 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:15 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:31:15 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:31:15 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.086630 m/s - 0.088777 m/s)
20:31:15 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:31:15 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:31:15 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:31:15 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:31:15 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:31:15 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:15 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:31:15 INFO opendrift.models.basemodel:2035: 2024-12-10 04:00:00 - step 29 of 71 - 1000 active elements (0 deactivated)
20:31:15 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:31:15 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:31:15 DEBUG opendrift.models.basemodel:2054: 59.99424741822976 <- latitude -> 60.015721692082984
20:31:15 DEBUG opendrift.models.basemodel:2059: 4.348996889215472 <- longitude -> 4.416536988947098
20:31:15 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:31:15 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:31:15 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:15 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:31:15 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:15 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:31:15 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:15 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:15 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:31:15 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:31:15 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:31:15 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:31:15 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:15 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:15 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:31:15 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:15 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:31:15 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:15 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:15 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:31:15 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-10 04:00:00 (before)
2024-12-10 05:00:00 (after)
20:31:16 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:31:16 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:31:16 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:31:16 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:31:16 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:31:16 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 26x27x2) for time before (2024-12-10 04:00:00)
20:31:16 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-10 04:00:00) in space (linearNDFast)
20:31:16 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:31:16 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:31:16 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.65101404285664 and -65.58347393719373 degrees.
20:31:16 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.65101404285664 and -65.58347393719373 degrees.
20:31:16 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:16 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:31:16 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:31:16 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:31:16 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:31:16 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:31:16 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.123905 (min) 0.409918 (max)
20:31:16 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0147759 (min) 0.386383 (max)
20:31:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.296308 (min) -0.284338 (max)
20:31:16 DEBUG opendrift.models.basemodel.environment:905: x_wind: 2.28006 (min) 2.651 (max)
20:31:16 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.61629 (min) -2.47296 (max)
20:31:16 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 1.16455e-05 (min) 1.38074e-05 (max)
20:31:16 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:31:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:31:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:31:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:31:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:31:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:31:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:31:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:31:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:31:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:31:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:31:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:31:16 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:31:16 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:31:16 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:31:16 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:31:16 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:31:16 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.264 (min) 298.86 (max)
20:31:16 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:31:16 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:31:16 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.278329, mean: 0.325814, max: 0.339030
20:31:16 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:31:16 DEBUG opendrift.models.physics_methods:1061: min: 2.874147, mean: 3.108919, max: 3.172114
20:31:16 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.874147, mean: 3.108919, max: 3.172114
20:31:16 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:31:16 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:16 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:31:16 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:31:16 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.067273 m/s - 0.074247 m/s)
20:31:16 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:31:16 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:31:16 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:31:16 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:31:16 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:31:16 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:16 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:31:16 INFO opendrift.models.basemodel:2035: 2024-12-10 05:00:00 - step 30 of 71 - 1000 active elements (0 deactivated)
20:31:16 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:31:16 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:31:16 DEBUG opendrift.models.basemodel:2054: 59.99981548248573 <- latitude -> 60.02147041994341
20:31:16 DEBUG opendrift.models.basemodel:2059: 4.3615234658409605 <- longitude -> 4.445945656802899
20:31:16 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:31:16 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:31:16 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:16 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:31:16 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:16 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:31:16 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:16 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:16 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:31:16 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:31:16 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:31:16 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:31:16 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:16 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:16 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:31:16 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:16 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:31:16 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:16 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:16 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:31:16 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-10 05:00:00 (before)
2024-12-10 06:00:00 (after)
20:31:18 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:31:18 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:31:18 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:31:18 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:31:18 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:31:18 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 27x27x2) for time before (2024-12-10 05:00:00)
20:31:18 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-10 05:00:00) in space (linearNDFast)
20:31:18 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:31:18 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:31:18 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.63848747596046 and -65.55406526034983 degrees.
20:31:18 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.63848747596046 and -65.55406526034983 degrees.
20:31:18 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:18 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:31:18 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:31:18 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:31:18 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:31:18 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:31:18 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.117139 (min) 0.417376 (max)
20:31:18 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.032908 (min) 0.360615 (max)
20:31:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.249652 (min) -0.237356 (max)
20:31:18 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.93392 (min) 2.1091 (max)
20:31:18 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.21253 (min) -1.77501 (max)
20:31:18 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 9.7704e-07 (min) 1.99527e-06 (max)
20:31:18 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:31:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:31:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:31:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:31:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:31:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:31:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:31:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:31:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:31:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:31:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:31:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:31:18 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:31:18 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:31:18 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:31:18 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:31:18 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:31:18 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.242 (min) 298.839 (max)
20:31:18 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:31:18 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:31:18 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.183527, mean: 0.196418, max: 0.229853
20:31:18 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:31:18 DEBUG opendrift.models.physics_methods:1061: min: 2.333888, mean: 2.414254, max: 2.611887
20:31:18 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.333888, mean: 2.414254, max: 2.611887
20:31:18 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:31:18 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:18 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:31:18 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:31:18 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.054628 m/s - 0.061135 m/s)
20:31:18 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:31:18 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:31:18 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:31:18 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:31:18 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:31:18 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:18 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:31:18 INFO opendrift.models.basemodel:2035: 2024-12-10 06:00:00 - step 31 of 71 - 1000 active elements (0 deactivated)
20:31:18 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:31:18 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:31:18 DEBUG opendrift.models.basemodel:2054: 60.005708886695665 <- latitude -> 60.031585672314066
20:31:18 DEBUG opendrift.models.basemodel:2059: 4.37219928164921 <- longitude -> 4.4756240161557965
20:31:18 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:31:18 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:31:18 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:18 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:31:18 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:18 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:31:18 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:18 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:18 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:31:18 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:31:18 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:31:18 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:31:18 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:18 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:18 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:31:18 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:18 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:31:18 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:18 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:18 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:31:18 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-10 06:00:00 (before)
2024-12-10 07:00:00 (after)
20:31:19 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:31:19 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:31:19 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:31:19 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:31:19 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:31:19 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 28x27x2) for time before (2024-12-10 06:00:00)
20:31:19 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-10 06:00:00) in space (linearNDFast)
20:31:19 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:31:19 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:31:19 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.62781164938293 and -65.5243868992785 degrees.
20:31:19 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.62781164938293 and -65.5243868992785 degrees.
20:31:19 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:19 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:31:19 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:31:19 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:31:19 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:31:19 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:31:19 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.126458 (min) 0.415877 (max)
20:31:19 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0751941 (min) 0.321115 (max)
20:31:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.276541 (min) -0.264006 (max)
20:31:19 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.94167 (min) 2.14933 (max)
20:31:19 DEBUG opendrift.models.basemodel.environment:905: y_wind: -1.13646 (min) -0.731193 (max)
20:31:19 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -1.58763e-05 (min) -1.28124e-05 (max)
20:31:19 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:31:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:31:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:31:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:31:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:31:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:31:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:31:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:31:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:31:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:31:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:31:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:31:19 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:31:19 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:31:19 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:31:19 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:31:19 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:31:19 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.239 (min) 298.79 (max)
20:31:19 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:31:19 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:31:19 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.105896, mean: 0.127868, max: 0.145414
20:31:19 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:31:19 DEBUG opendrift.models.physics_methods:1061: min: 1.772841, mean: 1.947653, max: 2.077463
20:31:19 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.772841, mean: 1.947653, max: 2.077463
20:31:19 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:31:19 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:19 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:31:19 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:31:19 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.041496 m/s - 0.048626 m/s)
20:31:19 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:31:19 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:31:19 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:31:19 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:31:19 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:31:19 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:19 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:31:19 INFO opendrift.models.basemodel:2035: 2024-12-10 07:00:00 - step 32 of 71 - 1000 active elements (0 deactivated)
20:31:19 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:31:19 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:31:19 DEBUG opendrift.models.basemodel:2054: 60.012168069293814 <- latitude -> 60.041068949328576
20:31:19 DEBUG opendrift.models.basemodel:2059: 4.3828686543724915 <- longitude -> 4.50518606370071
20:31:19 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:31:19 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:31:19 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:19 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:31:19 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:19 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:31:19 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:19 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:19 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:31:19 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:31:19 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:31:19 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:31:19 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:19 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:19 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:31:19 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:19 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:31:19 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:19 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:19 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:31:19 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-10 07:00:00 (before)
2024-12-10 08:00:00 (after)
20:31:20 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:31:20 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:31:20 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:31:20 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:31:20 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:31:20 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 29x29x2) for time before (2024-12-10 07:00:00)
20:31:20 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-10 07:00:00) in space (linearNDFast)
20:31:20 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:31:20 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:31:20 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.61714226842405 and -65.49482484702143 degrees.
20:31:20 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.61714226842405 and -65.49482484702143 degrees.
20:31:20 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:20 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:31:20 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:31:20 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:31:20 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:31:20 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:31:20 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.144221 (min) 0.39086 (max)
20:31:20 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.107455 (min) 0.360086 (max)
20:31:20 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.367257 (min) -0.341688 (max)
20:31:20 DEBUG opendrift.models.basemodel.environment:905: x_wind: 2.51725 (min) 2.93827 (max)
20:31:20 DEBUG opendrift.models.basemodel.environment:905: y_wind: -0.998838 (min) -0.816655 (max)
20:31:20 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -3.62938e-05 (min) -3.29528e-05 (max)
20:31:20 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:31:20 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:31:20 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:31:20 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:31:20 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:31:20 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:31:20 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:31:20 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:31:20 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:31:20 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:31:20 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:31:20 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:31:20 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:31:20 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:31:20 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:31:20 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:31:20 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:31:20 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.235 (min) 299.157 (max)
20:31:20 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:31:20 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:31:20 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.180422, mean: 0.203745, max: 0.231021
20:31:20 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:31:20 DEBUG opendrift.models.physics_methods:1061: min: 2.314061, mean: 2.458793, max: 2.618519
20:31:20 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.314061, mean: 2.458793, max: 2.618519
20:31:20 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:31:20 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:20 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:31:20 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:31:20 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.054164 m/s - 0.061290 m/s)
20:31:20 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:31:20 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:31:20 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:31:20 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:31:20 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:31:20 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:20 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:31:20 INFO opendrift.models.basemodel:2035: 2024-12-10 08:00:00 - step 33 of 71 - 1000 active elements (0 deactivated)
20:31:20 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:31:20 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:31:20 DEBUG opendrift.models.basemodel:2054: 60.01802827350505 <- latitude -> 60.05205816370942
20:31:20 DEBUG opendrift.models.basemodel:2059: 4.39597233990864 <- longitude -> 4.518810283004871
20:31:20 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:31:20 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:31:20 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:20 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:31:20 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:20 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:31:20 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:20 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:20 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:31:20 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:31:20 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:31:20 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:31:20 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:20 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:20 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:31:20 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:20 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:31:20 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:20 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:20 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:31:20 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-10 08:00:00 (before)
2024-12-10 09:00:00 (after)
20:31:21 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:31:21 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:31:21 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:31:21 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:31:21 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:31:21 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 30x28x2) for time before (2024-12-10 08:00:00)
20:31:21 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-10 08:00:00) in space (linearNDFast)
20:31:21 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:31:21 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:31:21 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.60403859405187 and -65.481200627268 degrees.
20:31:21 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.60403859405187 and -65.481200627268 degrees.
20:31:21 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:21 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:31:21 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:31:21 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:31:21 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:31:21 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:31:21 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.149453 (min) 0.363515 (max)
20:31:21 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.140621 (min) 0.43446 (max)
20:31:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.519666 (min) -0.496805 (max)
20:31:21 DEBUG opendrift.models.basemodel.environment:905: x_wind: 3.99085 (min) 4.2612 (max)
20:31:21 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.10288 (min) -2.00474 (max)
20:31:21 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -4.53861e-05 (min) -4.15196e-05 (max)
20:31:21 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:31:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:31:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:31:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:31:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:31:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:31:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:31:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:31:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:31:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:31:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:31:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:31:21 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:31:21 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:31:21 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:31:21 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:31:21 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:31:21 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.227 (min) 299.379 (max)
20:31:21 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:31:21 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:31:21 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.500344, mean: 0.532257, max: 0.545973
20:31:21 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:31:21 DEBUG opendrift.models.physics_methods:1061: min: 3.853578, mean: 3.974378, max: 4.025458
20:31:21 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.853578, mean: 3.974378, max: 4.025458
20:31:21 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:31:21 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:21 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:31:21 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:31:21 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.090198 m/s - 0.094221 m/s)
20:31:21 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:31:21 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:31:21 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:31:21 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:31:21 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:31:21 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:21 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:31:21 INFO opendrift.models.basemodel:2035: 2024-12-10 09:00:00 - step 34 of 71 - 1000 active elements (0 deactivated)
20:31:21 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:31:21 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:31:21 DEBUG opendrift.models.basemodel:2054: 60.02255868558001 <- latitude -> 60.06470890564614
20:31:21 DEBUG opendrift.models.basemodel:2059: 4.414313789873339 <- longitude -> 4.533631274781601
20:31:21 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:31:21 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:31:21 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:21 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:31:21 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:21 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:31:21 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:21 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:21 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:31:21 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:31:21 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:31:21 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:31:21 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:21 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:21 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:31:21 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:21 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:31:21 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:21 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:21 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:31:21 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-10 09:00:00 (before)
2024-12-10 10:00:00 (after)
20:31:23 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:31:23 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:31:23 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:31:23 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:31:23 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:31:23 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 31x27x2) for time before (2024-12-10 09:00:00)
20:31:23 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-10 09:00:00) in space (linearNDFast)
20:31:23 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:31:23 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:31:23 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.58569712757637 and -65.46637961898219 degrees.
20:31:23 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.58569712757637 and -65.46637961898219 degrees.
20:31:23 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:23 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:31:23 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:31:23 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:31:23 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:31:23 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:31:23 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0794902 (min) 0.325051 (max)
20:31:23 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.230286 (min) 0.493686 (max)
20:31:23 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.670837 (min) -0.650926 (max)
20:31:23 DEBUG opendrift.models.basemodel.environment:905: x_wind: 4.30638 (min) 4.41469 (max)
20:31:23 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.51468 (min) -2.37602 (max)
20:31:23 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -4.24072e-05 (min) -3.95573e-05 (max)
20:31:23 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:31:23 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:31:23 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:31:23 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:31:23 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:31:23 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:31:23 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:31:23 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:31:23 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:31:23 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:31:23 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:31:23 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:31:23 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:31:23 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:31:23 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:31:23 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:31:23 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:31:23 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.22 (min) 299.637 (max)
20:31:23 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:31:23 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:31:23 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.595084, mean: 0.623844, max: 0.630336
20:31:23 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:31:23 DEBUG opendrift.models.physics_methods:1061: min: 4.202605, mean: 4.302954, max: 4.325293
20:31:23 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 4.202605, mean: 4.302954, max: 4.325293
20:31:23 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:31:23 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:23 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:31:23 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:31:23 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.098367 m/s - 0.101239 m/s)
20:31:23 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:31:23 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:31:23 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:31:23 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:31:23 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:31:23 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:23 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:31:23 INFO opendrift.models.basemodel:2035: 2024-12-10 10:00:00 - step 35 of 71 - 1000 active elements (0 deactivated)
20:31:23 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:31:23 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:31:23 DEBUG opendrift.models.basemodel:2054: 60.02846307734788 <- latitude -> 60.07863792043379
20:31:23 DEBUG opendrift.models.basemodel:2059: 4.43651642149999 <- longitude -> 4.544402541384059
20:31:23 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:31:23 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:31:23 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:23 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:31:23 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:23 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:31:23 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:23 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:23 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:31:23 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:31:23 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:31:23 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:31:23 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:23 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:23 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:31:23 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:23 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:31:23 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:23 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:23 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:31:23 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-10 10:00:00 (before)
2024-12-10 11:00:00 (after)
20:31:25 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:31:25 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:31:25 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:31:25 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:31:25 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:31:25 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 31x26x2) for time before (2024-12-10 10:00:00)
20:31:25 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-10 10:00:00) in space (linearNDFast)
20:31:25 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:31:25 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:31:25 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.56349451054544 and -65.45560836431413 degrees.
20:31:25 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.56349451054544 and -65.45560836431413 degrees.
20:31:25 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:25 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:31:25 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:31:25 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:31:25 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:31:25 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:31:25 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.00397832 (min) 0.325076 (max)
20:31:25 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.428211 (min) 0.527728 (max)
20:31:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.766231 (min) -0.75199 (max)
20:31:25 DEBUG opendrift.models.basemodel.environment:905: x_wind: 3.21631 (min) 3.41231 (max)
20:31:25 DEBUG opendrift.models.basemodel.environment:905: y_wind: -1.90683 (min) -1.53569 (max)
20:31:25 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -2.2486e-05 (min) -1.99962e-05 (max)
20:31:25 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:31:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:31:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:31:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:31:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:31:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:31:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:31:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:31:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:31:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:31:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:31:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:31:25 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:31:25 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:31:25 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:31:25 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:31:25 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:31:25 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.188 (min) 299.846 (max)
20:31:25 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:31:25 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:31:25 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.328029, mean: 0.334757, max: 0.361352
20:31:25 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:31:25 DEBUG opendrift.models.physics_methods:1061: min: 3.120222, mean: 3.151887, max: 3.274878
20:31:25 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.120222, mean: 3.151887, max: 3.274878
20:31:25 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:31:25 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:25 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:31:25 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:31:25 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.073033 m/s - 0.076653 m/s)
20:31:25 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:31:25 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:31:25 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:31:25 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:31:25 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:31:25 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:25 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:31:25 INFO opendrift.models.basemodel:2035: 2024-12-10 11:00:00 - step 36 of 71 - 1000 active elements (0 deactivated)
20:31:25 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:31:25 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:31:25 DEBUG opendrift.models.basemodel:2054: 60.04130597584144 <- latitude -> 60.0939784617543
20:31:25 DEBUG opendrift.models.basemodel:2059: 4.457620355772076 <- longitude -> 4.550969082091244
20:31:25 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:31:25 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:31:25 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:25 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:31:25 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:25 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:31:25 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:25 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:25 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:31:25 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:31:25 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:31:25 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:31:25 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:25 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:25 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:31:25 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:25 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:31:25 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:25 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:25 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:31:25 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-10 11:00:00 (before)
2024-12-10 12:00:00 (after)
20:31:26 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:31:26 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:31:26 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:31:26 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:31:26 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:31:26 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 31x25x2) for time before (2024-12-10 11:00:00)
20:31:26 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-10 11:00:00) in space (linearNDFast)
20:31:26 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:31:26 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:31:26 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.5423905756832 and -65.44904180593517 degrees.
20:31:26 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.5423905756832 and -65.44904180593517 degrees.
20:31:26 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:26 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:31:26 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:31:26 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:31:26 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:31:26 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:31:26 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0984531 (min) 0.384307 (max)
20:31:26 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.486115 (min) 0.543302 (max)
20:31:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.789716 (min) -0.768426 (max)
20:31:26 DEBUG opendrift.models.basemodel.environment:905: x_wind: 3.71297 (min) 3.91695 (max)
20:31:26 DEBUG opendrift.models.basemodel.environment:905: y_wind: -1.54822 (min) -1.34348 (max)
20:31:26 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 4.89487e-06 (min) 7.93408e-06 (max)
20:31:26 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:31:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:31:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:31:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:31:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:31:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:31:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:31:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:31:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:31:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:31:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:31:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:31:26 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:31:26 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:31:26 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:31:26 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:31:26 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:31:26 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.135 (min) 299.951 (max)
20:31:26 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:31:26 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:31:26 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.385772, mean: 0.412818, max: 0.434608
20:31:26 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:31:26 DEBUG opendrift.models.physics_methods:1061: min: 3.383724, mean: 3.499767, max: 3.591520
20:31:26 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.383724, mean: 3.499767, max: 3.591520
20:31:26 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:31:26 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:26 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:31:26 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:31:26 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.079200 m/s - 0.084064 m/s)
20:31:26 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:31:26 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:31:26 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:31:26 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:31:26 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:31:26 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:26 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:31:26 INFO opendrift.models.basemodel:2035: 2024-12-10 12:00:00 - step 37 of 71 - 1000 active elements (0 deactivated)
20:31:26 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:31:26 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:31:26 DEBUG opendrift.models.basemodel:2054: 60.0572267688344 <- latitude -> 60.10966619623835
20:31:26 DEBUG opendrift.models.basemodel:2059: 4.4842677711209555 <- longitude -> 4.556415004139574
20:31:26 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:31:26 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:31:26 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:26 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:31:26 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:26 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:31:26 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:26 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:26 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:31:26 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:31:26 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:31:26 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:31:26 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:26 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:26 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:31:26 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:26 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:31:26 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:26 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:26 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:31:26 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-10 12:00:00 (before)
2024-12-10 13:00:00 (after)
20:31:27 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:31:27 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:31:27 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:31:27 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:31:27 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:31:27 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 30x25x2) for time before (2024-12-10 12:00:00)
20:31:27 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-10 12:00:00) in space (linearNDFast)
20:31:27 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:31:27 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:31:27 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.51574314230834 and -65.44359587914164 degrees.
20:31:27 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.51574314230834 and -65.44359587914164 degrees.
20:31:27 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:27 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:31:27 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:31:27 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:31:27 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:31:27 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:31:27 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.23923 (min) 0.409904 (max)
20:31:27 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.467579 (min) 0.595917 (max)
20:31:27 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.724665 (min) -0.697361 (max)
20:31:27 DEBUG opendrift.models.basemodel.environment:905: x_wind: 3.6091 (min) 3.73438 (max)
20:31:27 DEBUG opendrift.models.basemodel.environment:905: y_wind: -1.16066 (min) -0.958793 (max)
20:31:27 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 2.80434e-05 (min) 3.16675e-05 (max)
20:31:27 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:31:27 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:31:27 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:31:27 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:31:27 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:31:27 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:31:27 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:31:27 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:31:27 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:31:27 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:31:27 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:31:27 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:31:27 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:31:27 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:31:27 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:31:27 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:31:27 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:31:27 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.449 (min) 299.992 (max)
20:31:27 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:31:27 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:31:27 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.347511, mean: 0.351059, max: 0.376201
20:31:27 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:31:27 DEBUG opendrift.models.physics_methods:1061: min: 3.211543, mean: 3.227863, max: 3.341484
20:31:27 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.211543, mean: 3.227863, max: 3.341484
20:31:27 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:31:27 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:27 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:31:27 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:31:27 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.075170 m/s - 0.078212 m/s)
20:31:27 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:31:27 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:31:27 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:31:27 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:31:27 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:31:27 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:27 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:31:27 INFO opendrift.models.basemodel:2035: 2024-12-10 13:00:00 - step 38 of 71 - 1000 active elements (0 deactivated)
20:31:27 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:31:27 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:31:27 DEBUG opendrift.models.basemodel:2054: 60.07397554360061 <- latitude -> 60.128300780092076
20:31:27 DEBUG opendrift.models.basemodel:2059: 4.5156023014834075 <- longitude -> 4.561763314634309
20:31:27 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:31:27 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:31:27 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:27 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:31:27 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:27 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:31:27 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:27 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:27 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:31:27 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:31:27 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:31:27 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:31:27 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:27 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:27 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:31:27 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:27 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:31:27 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:27 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:27 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:31:27 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-10 13:00:00 (before)
2024-12-10 14:00:00 (after)
20:31:28 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:31:28 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:31:28 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:31:28 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:31:28 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:31:28 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 29x25x2) for time before (2024-12-10 13:00:00)
20:31:28 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-10 13:00:00) in space (linearNDFast)
20:31:28 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:31:28 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:31:28 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.48440858624413 and -65.43824757085049 degrees.
20:31:28 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.48440858624413 and -65.43824757085049 degrees.
20:31:28 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:28 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:31:28 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:31:28 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:31:28 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:31:28 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:31:29 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.364422 (min) 0.33825 (max)
20:31:29 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.433698 (min) 0.704238 (max)
20:31:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.582835 (min) -0.560916 (max)
20:31:29 DEBUG opendrift.models.basemodel.environment:905: x_wind: 3.13391 (min) 3.20433 (max)
20:31:29 DEBUG opendrift.models.basemodel.environment:905: y_wind: -1.10382 (min) -0.791648 (max)
20:31:29 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 3.96728e-05 (min) 4.47109e-05 (max)
20:31:29 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:31:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:31:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:31:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:31:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:31:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:31:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:31:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:31:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:31:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:31:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:31:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:31:29 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:31:29 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:31:29 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:31:29 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:31:29 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:31:29 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 299.178 (min) 299.997 (max)
20:31:29 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:31:29 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:31:29 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.258999, mean: 0.275365, max: 0.279261
20:31:29 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:31:29 DEBUG opendrift.models.physics_methods:1061: min: 2.772547, mean: 2.858709, max: 2.878956
20:31:29 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.772547, mean: 2.858709, max: 2.878956
20:31:29 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:31:29 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:29 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:31:29 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:31:29 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.064895 m/s - 0.067386 m/s)
20:31:29 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:31:29 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:31:29 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:31:29 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:31:29 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:31:29 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:29 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:31:29 INFO opendrift.models.basemodel:2035: 2024-12-10 14:00:00 - step 39 of 71 - 1000 active elements (0 deactivated)
20:31:29 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:31:29 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:31:29 DEBUG opendrift.models.basemodel:2054: 60.08962042351297 <- latitude -> 60.15054218192198
20:31:29 DEBUG opendrift.models.basemodel:2059: 4.516663203486504 <- longitude -> 4.564013181895774
20:31:29 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:31:29 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:31:29 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:29 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:31:29 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:29 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:31:29 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:29 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:29 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:31:29 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:31:29 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:31:29 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:31:29 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:29 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:29 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:31:29 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:29 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:31:29 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:29 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:29 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:31:29 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-10 14:00:00 (before)
2024-12-10 15:00:00 (after)
20:31:30 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:31:30 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:31:30 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:31:30 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:31:30 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:31:30 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 28x28x2) for time before (2024-12-10 14:00:00)
20:31:30 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-10 14:00:00) in space (linearNDFast)
20:31:30 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:31:30 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:31:30 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.48334766586996 and -65.4359977017566 degrees.
20:31:30 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.48334766586996 and -65.4359977017566 degrees.
20:31:30 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:30 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:31:30 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:31:30 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:31:30 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:31:30 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:31:30 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.476914 (min) 0.142527 (max)
20:31:30 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.391026 (min) 0.797094 (max)
20:31:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.431311 (min) -0.413079 (max)
20:31:30 DEBUG opendrift.models.basemodel.environment:905: x_wind: 2.6174 (min) 2.7386 (max)
20:31:30 DEBUG opendrift.models.basemodel.environment:905: y_wind: -1.20794 (min) -0.338356 (max)
20:31:30 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 3.81322e-05 (min) 4.25088e-05 (max)
20:31:30 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:31:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:31:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:31:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:31:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:31:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:31:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:31:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:31:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:31:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:31:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:31:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:31:30 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:31:30 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:31:30 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:31:30 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:31:30 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:31:30 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 299.46 (min) 299.973 (max)
20:31:30 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:31:30 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:31:30 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.171345, mean: 0.207202, max: 0.218295
20:31:30 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:31:30 DEBUG opendrift.models.physics_methods:1061: min: 2.255100, mean: 2.479077, max: 2.545375
20:31:30 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.255100, mean: 2.479077, max: 2.545375
20:31:30 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:31:30 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:30 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:31:30 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:31:30 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.052784 m/s - 0.059578 m/s)
20:31:30 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:31:30 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:31:30 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:31:30 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:31:30 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:31:30 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:30 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:31:30 INFO opendrift.models.basemodel:2035: 2024-12-10 15:00:00 - step 40 of 71 - 1000 active elements (0 deactivated)
20:31:30 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:31:30 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:31:30 DEBUG opendrift.models.basemodel:2054: 60.10240998023001 <- latitude -> 60.176075260153986
20:31:30 DEBUG opendrift.models.basemodel:2059: 4.489125208413175 <- longitude -> 4.5659857859006
20:31:30 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:31:30 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:31:30 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:30 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:31:30 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:30 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:31:30 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:30 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:30 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:31:30 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:31:30 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:31:30 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:31:30 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:30 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:30 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:31:30 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:30 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:31:30 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:30 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:30 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:31:30 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-10 15:00:00 (before)
2024-12-10 16:00:00 (after)
20:31:31 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:31:31 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:31:31 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:31:31 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:31:31 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:31:31 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 30x31x2) for time before (2024-12-10 15:00:00)
20:31:31 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-10 15:00:00) in space (linearNDFast)
20:31:31 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:31:31 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:31:31 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.51088566004117 and -65.43402509936809 degrees.
20:31:31 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.51088566004117 and -65.43402509936809 degrees.
20:31:31 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:31 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:31:31 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:31:31 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:31:31 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:31:31 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:31:31 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.55643 (min) 0.0240458 (max)
20:31:31 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.361015 (min) 0.827809 (max)
20:31:31 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.296413 (min) -0.273912 (max)
20:31:31 DEBUG opendrift.models.basemodel.environment:905: x_wind: 2.37137 (min) 2.61874 (max)
20:31:31 DEBUG opendrift.models.basemodel.environment:905: y_wind: -1.24355 (min) -0.487112 (max)
20:31:31 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 3.35541e-05 (min) 3.65664e-05 (max)
20:31:31 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:31:31 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:31:31 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:31:31 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:31:31 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:31:31 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:31:31 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:31:31 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:31:31 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:31:31 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:31:31 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:31:31 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:31:31 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:31:31 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:31:31 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:31:31 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:31:31 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:31:31 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 299.401 (min) 299.903 (max)
20:31:31 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:31:31 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:31:31 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.168124, mean: 0.172466, max: 0.177468
20:31:31 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:31:31 DEBUG opendrift.models.physics_methods:1061: min: 2.233800, mean: 2.262409, max: 2.295039
20:31:31 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.233800, mean: 2.262409, max: 2.295039
20:31:31 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:31:31 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:31 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:31:31 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:31:31 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.052285 m/s - 0.053718 m/s)
20:31:31 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:31:31 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:31:31 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:31:31 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:31:31 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:31:31 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:31 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:31:31 INFO opendrift.models.basemodel:2035: 2024-12-10 16:00:00 - step 41 of 71 - 1000 active elements (0 deactivated)
20:31:31 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:31:31 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:31:31 DEBUG opendrift.models.basemodel:2054: 60.11349702156193 <- latitude -> 60.20250325501734
20:31:31 DEBUG opendrift.models.basemodel:2059: 4.456404968713745 <- longitude -> 4.563694536922246
20:31:31 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:31:31 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:31:31 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:31 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:31:31 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:31 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:31:31 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:31 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:31 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:31:31 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:31:31 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:31:31 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:31:31 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:31 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:31 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:31:31 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:31 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:31:31 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:31 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:31 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:31:31 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-10 16:00:00 (before)
2024-12-10 17:00:00 (after)
20:31:33 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:31:33 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:31:33 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:31:33 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:31:33 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:31:33 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 30x34x2) for time before (2024-12-10 16:00:00)
20:31:33 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-10 16:00:00) in space (linearNDFast)
20:31:33 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:31:33 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:31:33 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.54360589628752 and -65.43631633360309 degrees.
20:31:33 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.54360589628752 and -65.43631633360309 degrees.
20:31:33 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:33 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:31:33 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:31:33 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:31:33 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:31:33 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:31:33 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.642204 (min) -0.0892383 (max)
20:31:33 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.342067 (min) 0.8061 (max)
20:31:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.178665 (min) -0.160726 (max)
20:31:33 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.38586 (min) 1.6532 (max)
20:31:33 DEBUG opendrift.models.basemodel.environment:905: y_wind: -0.19558 (min) 1.07386 (max)
20:31:33 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 2.40509e-05 (min) 2.68239e-05 (max)
20:31:33 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:31:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:31:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:31:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:31:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:31:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:31:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:31:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:31:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:31:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:31:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:31:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:31:33 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:31:33 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:31:33 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:31:33 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:31:33 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:31:33 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 299.537 (min) 299.865 (max)
20:31:33 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:31:33 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:31:33 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.047831, mean: 0.055260, max: 0.095602
20:31:33 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:31:33 DEBUG opendrift.models.physics_methods:1061: min: 1.191469, mean: 1.278333, max: 1.684467
20:31:33 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.191469, mean: 1.278333, max: 1.684467
20:31:33 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:31:33 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:33 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:31:33 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:31:33 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.027888 m/s - 0.039427 m/s)
20:31:33 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:31:33 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:31:33 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:31:33 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:31:33 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:31:33 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:33 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:31:33 INFO opendrift.models.basemodel:2035: 2024-12-10 17:00:00 - step 42 of 71 - 1000 active elements (0 deactivated)
20:31:33 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:31:33 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:31:33 DEBUG opendrift.models.basemodel:2054: 60.12442328929056 <- latitude -> 60.22908390932727
20:31:33 DEBUG opendrift.models.basemodel:2059: 4.416832563636195 <- longitude -> 4.555015882872593
20:31:33 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:31:33 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:31:33 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:33 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:31:33 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:33 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:31:33 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:33 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:33 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:31:33 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:31:33 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:31:33 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:31:33 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:33 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:33 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:31:33 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:33 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:31:33 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:33 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:33 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:31:33 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-10 17:00:00 (before)
2024-12-10 18:00:00 (after)
20:31:34 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:31:34 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:31:34 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:31:34 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:31:34 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:31:34 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 32x37x2) for time before (2024-12-10 17:00:00)
20:31:34 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-10 17:00:00) in space (linearNDFast)
20:31:34 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:31:34 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:31:34 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.58317829562706 and -65.444994998391 degrees.
20:31:34 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.58317829562706 and -65.444994998391 degrees.
20:31:34 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:34 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:31:34 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:31:34 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:31:34 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:31:34 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:31:34 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.732284 (min) -0.253937 (max)
20:31:34 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.375493 (min) 0.765525 (max)
20:31:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.10539 (min) -0.0958278 (max)
20:31:34 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0.532808 (min) 1.86179 (max)
20:31:34 DEBUG opendrift.models.basemodel.environment:905: y_wind: 1.25967 (min) 1.91578 (max)
20:31:34 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 7.16759e-06 (min) 1.02335e-05 (max)
20:31:34 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:31:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:31:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:31:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:31:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:31:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:31:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:31:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:31:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:31:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:31:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:31:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:31:34 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:31:34 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:31:34 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:31:34 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:31:34 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:31:34 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 299.553 (min) 300.486 (max)
20:31:34 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:31:34 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:31:34 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.046029, mean: 0.085869, max: 0.168295
20:31:34 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:31:34 DEBUG opendrift.models.physics_methods:1061: min: 1.168810, mean: 1.586794, max: 2.234938
20:31:34 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.168810, mean: 1.586794, max: 2.234938
20:31:34 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:31:34 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:34 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:31:34 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:31:34 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.027358 m/s - 0.052312 m/s)
20:31:34 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:31:34 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:31:34 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:31:34 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:31:34 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:31:34 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:34 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:31:34 INFO opendrift.models.basemodel:2035: 2024-12-10 18:00:00 - step 43 of 71 - 1000 active elements (0 deactivated)
20:31:34 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:31:34 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:31:34 DEBUG opendrift.models.basemodel:2054: 60.137369158815524 <- latitude -> 60.25351620697329
20:31:34 DEBUG opendrift.models.basemodel:2059: 4.371646275538914 <- longitude -> 4.539255919765918
20:31:34 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:31:34 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:31:34 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:34 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:31:34 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:34 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:31:34 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:34 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:34 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:31:34 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:31:34 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:31:34 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:31:34 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:34 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:34 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:31:34 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:34 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:31:34 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:34 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:34 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:31:34 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-10 18:00:00 (before)
2024-12-10 19:00:00 (after)
20:31:36 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:31:36 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:31:36 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:31:36 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:31:36 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:31:36 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 32x39x2) for time before (2024-12-10 18:00:00)
20:31:36 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-10 18:00:00) in space (linearNDFast)
20:31:36 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:31:36 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:31:36 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.62836457873628 and -65.46075494477975 degrees.
20:31:36 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.62836457873628 and -65.46075494477975 degrees.
20:31:36 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:36 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:31:36 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:31:36 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:31:36 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:31:36 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:31:36 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.817679 (min) -0.397725 (max)
20:31:36 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.451862 (min) 0.736211 (max)
20:31:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.109813 (min) -0.10191 (max)
20:31:36 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0.8719 (min) 3.44887 (max)
20:31:36 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0.436231 (min) 2.55932 (max)
20:31:36 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -1.51756e-05 (min) -1.4024e-05 (max)
20:31:36 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:31:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:31:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:31:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:31:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:31:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:31:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:31:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:31:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:31:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:31:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:31:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:31:36 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:31:36 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:31:36 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:31:36 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:31:36 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:31:36 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.551 (min) 300.657 (max)
20:31:36 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:31:36 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:31:36 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.179834, mean: 0.199174, max: 0.297291
20:31:36 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:31:36 DEBUG opendrift.models.physics_methods:1061: min: 2.310282, mean: 2.429078, max: 2.970435
20:31:36 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.310282, mean: 2.429078, max: 2.970435
20:31:36 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:31:36 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:36 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:31:36 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:31:36 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.054075 m/s - 0.069527 m/s)
20:31:36 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:31:36 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:31:36 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:31:36 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:31:36 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:31:36 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:36 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:31:36 INFO opendrift.models.basemodel:2035: 2024-12-10 19:00:00 - step 44 of 71 - 1000 active elements (0 deactivated)
20:31:36 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:31:36 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:31:36 DEBUG opendrift.models.basemodel:2054: 60.1536209955198 <- latitude -> 60.27206757050479
20:31:36 DEBUG opendrift.models.basemodel:2059: 4.32294297418525 <- longitude -> 4.514608203969906
20:31:36 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:31:36 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:31:36 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:36 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:31:36 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:36 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:31:36 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:36 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:36 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:31:36 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:31:36 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:31:36 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:31:36 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:36 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:36 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:31:36 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:36 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:31:36 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:36 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:36 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:31:36 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-10 19:00:00 (before)
2024-12-10 20:00:00 (after)
20:31:37 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:31:37 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:31:37 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:31:37 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:31:37 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:31:37 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 31x41x2) for time before (2024-12-10 19:00:00)
20:31:37 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-10 19:00:00) in space (linearNDFast)
20:31:37 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:31:37 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:31:37 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.677067872465 and -65.48540266921395 degrees.
20:31:37 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.677067872465 and -65.48540266921395 degrees.
20:31:37 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:37 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:31:37 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:31:37 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:31:37 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:31:37 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:31:37 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.859444 (min) -0.454394 (max)
20:31:37 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.372896 (min) 0.715085 (max)
20:31:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.199916 (min) -0.185976 (max)
20:31:37 DEBUG opendrift.models.basemodel.environment:905: x_wind: 2.75743 (min) 5.27401 (max)
20:31:37 DEBUG opendrift.models.basemodel.environment:905: y_wind: -1.76379 (min) 0.043502 (max)
20:31:37 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -3.93348e-05 (min) -3.8336e-05 (max)
20:31:37 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:31:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:31:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:31:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:31:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:31:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:31:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:31:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:31:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:31:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:31:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:31:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:31:37 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:31:37 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:31:37 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:31:37 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:31:37 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:31:37 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 296.732 (min) 300.668 (max)
20:31:37 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:31:37 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:31:37 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.187090, mean: 0.368976, max: 0.760782
20:31:37 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:31:37 DEBUG opendrift.models.physics_methods:1061: min: 2.356434, mean: 3.278492, max: 4.751817
20:31:37 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.356434, mean: 3.278492, max: 4.751817
20:31:37 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:31:37 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:37 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:31:37 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:31:37 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.055155 m/s - 0.111222 m/s)
20:31:37 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:31:37 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:31:37 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:31:37 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:31:37 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:31:37 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:37 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:31:37 INFO opendrift.models.basemodel:2035: 2024-12-10 20:00:00 - step 45 of 71 - 1000 active elements (0 deactivated)
20:31:37 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:31:37 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:31:37 DEBUG opendrift.models.basemodel:2054: 60.17074229808277 <- latitude -> 60.28296448453986
20:31:37 DEBUG opendrift.models.basemodel:2059: 4.273878596736566 <- longitude -> 4.488716930460014
20:31:37 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:31:37 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:31:37 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:37 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:31:37 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:37 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:31:37 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:37 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:37 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:31:37 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:31:37 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:31:37 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:31:37 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:37 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:37 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:31:37 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:37 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:31:37 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:37 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:37 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:31:37 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-10 20:00:00 (before)
2024-12-10 21:00:00 (after)
20:31:38 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:31:38 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:31:38 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:31:38 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:31:38 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:31:38 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 30x42x2) for time before (2024-12-10 20:00:00)
20:31:38 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-10 20:00:00) in space (linearNDFast)
20:31:38 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:31:38 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:31:38 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.72613225305237 and -65.5112939364269 degrees.
20:31:38 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.72613225305237 and -65.5112939364269 degrees.
20:31:38 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:38 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:31:38 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:31:38 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:31:38 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:31:38 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:31:38 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.914963 (min) -0.462544 (max)
20:31:38 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.244938 (min) 0.701732 (max)
20:31:38 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.37391 (min) -0.365966 (max)
20:31:38 DEBUG opendrift.models.basemodel.environment:905: x_wind: 3.54303 (min) 4.68014 (max)
20:31:38 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.29958 (min) -1.75445 (max)
20:31:38 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -6.03268e-05 (min) -5.67425e-05 (max)
20:31:38 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:31:38 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:31:38 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:31:38 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:31:38 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:31:38 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:31:38 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:31:38 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:31:38 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:31:38 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:31:38 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:31:38 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:31:38 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:31:38 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:31:38 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:31:38 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:31:38 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:31:38 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 296.313 (min) 300.507 (max)
20:31:38 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:31:38 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:31:38 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.384527, mean: 0.523956, max: 0.668917
20:31:38 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:31:38 DEBUG opendrift.models.physics_methods:1061: min: 3.378261, mean: 3.939683, max: 4.455699
20:31:38 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.378261, mean: 3.939683, max: 4.455699
20:31:38 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:31:38 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:38 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:31:38 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:31:38 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.079073 m/s - 0.104291 m/s)
20:31:38 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:31:38 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:31:38 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:31:38 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:31:38 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:31:38 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:38 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:31:38 INFO opendrift.models.basemodel:2035: 2024-12-10 21:00:00 - step 46 of 71 - 1000 active elements (0 deactivated)
20:31:38 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:31:38 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:31:38 DEBUG opendrift.models.basemodel:2054: 60.1889283395699 <- latitude -> 60.28937916702387
20:31:38 DEBUG opendrift.models.basemodel:2059: 4.220417317923425 <- longitude -> 4.463300942388142
20:31:38 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:31:38 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:31:38 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:38 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:31:38 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:38 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:31:38 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:38 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:38 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:31:38 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:31:38 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:31:38 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:31:38 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:38 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:38 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:31:38 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:38 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:31:38 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:38 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:38 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:31:38 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-10 21:00:00 (before)
2024-12-10 22:00:00 (after)
20:31:40 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:31:40 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:31:40 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:31:40 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:31:40 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:31:40 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 28x44x2) for time before (2024-12-10 21:00:00)
20:31:40 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-10 21:00:00) in space (linearNDFast)
20:31:40 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:31:40 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:31:40 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.77959352814685 and -65.53670991560423 degrees.
20:31:40 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.77959352814685 and -65.53670991560423 degrees.
20:31:40 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:40 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:31:40 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:31:40 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:31:40 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:31:40 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:31:40 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.945526 (min) -0.476767 (max)
20:31:40 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.176997 (min) 0.676017 (max)
20:31:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.590875 (min) -0.581832 (max)
20:31:40 DEBUG opendrift.models.basemodel.environment:905: x_wind: 3.00679 (min) 3.60885 (max)
20:31:40 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.00321 (min) -1.81896 (max)
20:31:40 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -6.31162e-05 (min) -5.85568e-05 (max)
20:31:40 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:31:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:31:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:31:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:31:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:31:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:31:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:31:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:31:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:31:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:31:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:31:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:31:40 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:31:40 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:31:40 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:31:40 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:31:40 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:31:40 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 296.22 (min) 300.242 (max)
20:31:40 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:31:40 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:31:40 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.303795, mean: 0.359979, max: 0.408547
20:31:40 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:31:40 DEBUG opendrift.models.physics_methods:1061: min: 3.002756, mean: 3.268287, max: 3.482177
20:31:40 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.002756, mean: 3.268287, max: 3.482177
20:31:40 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:31:40 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:40 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:31:40 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:31:40 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.070283 m/s - 0.081505 m/s)
20:31:40 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:31:40 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:31:40 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:31:40 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:31:40 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:31:40 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:40 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:31:40 INFO opendrift.models.basemodel:2035: 2024-12-10 22:00:00 - step 47 of 71 - 1000 active elements (0 deactivated)
20:31:40 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:31:40 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:31:40 DEBUG opendrift.models.basemodel:2054: 60.208561931497705 <- latitude -> 60.29386109664894
20:31:40 DEBUG opendrift.models.basemodel:2059: 4.165063119591799 <- longitude -> 4.436249947730537
20:31:40 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:31:40 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:31:40 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:40 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:31:40 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:40 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:31:40 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:40 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:40 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:31:40 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:31:40 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:31:40 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:31:40 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:40 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:40 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:31:40 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:40 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:31:40 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:40 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:40 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:31:40 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-10 22:00:00 (before)
2024-12-10 23:00:00 (after)
20:31:41 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:31:41 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:31:41 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:31:41 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:31:41 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:31:41 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 26x44x2) for time before (2024-12-10 22:00:00)
20:31:41 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-10 22:00:00) in space (linearNDFast)
20:31:41 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:31:41 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:31:41 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.83494773903618 and -65.56376091439978 degrees.
20:31:41 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.83494773903618 and -65.56376091439978 degrees.
20:31:41 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:41 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:31:41 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:31:41 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:31:41 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:31:41 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:31:41 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.947282 (min) -0.523363 (max)
20:31:41 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.094631 (min) 0.669663 (max)
20:31:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.786116 (min) -0.767408 (max)
20:31:41 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0.225458 (min) 2.94041 (max)
20:31:41 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.26062 (min) -1.36505 (max)
20:31:41 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -4.5027e-05 (min) -4.0954e-05 (max)
20:31:41 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:31:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:31:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:31:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:31:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:31:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:31:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:31:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:31:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:31:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:31:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:31:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:31:41 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:31:41 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:31:41 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:31:41 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:31:41 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:31:41 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 296.232 (min) 299.907 (max)
20:31:41 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:31:41 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:31:41 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.235919, mean: 0.263252, max: 0.279577
20:31:41 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:31:41 DEBUG opendrift.models.physics_methods:1061: min: 2.646130, mean: 2.794574, max: 2.880580
20:31:41 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.646130, mean: 2.794574, max: 2.880580
20:31:41 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:31:41 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:41 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:31:41 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:31:41 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.061936 m/s - 0.067424 m/s)
20:31:41 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:31:41 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:31:41 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:31:41 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:31:41 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:31:41 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:41 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:31:41 INFO opendrift.models.basemodel:2035: 2024-12-10 23:00:00 - step 48 of 71 - 1000 active elements (0 deactivated)
20:31:41 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:31:41 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:31:41 DEBUG opendrift.models.basemodel:2054: 60.229313111811244 <- latitude -> 60.29662462959209
20:31:41 DEBUG opendrift.models.basemodel:2059: 4.1096975845328645 <- longitude -> 4.405859842789515
20:31:41 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:31:41 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:31:41 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:41 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:31:41 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:41 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:31:41 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:41 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:41 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:31:41 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:31:41 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:31:41 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:31:41 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:41 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:41 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:31:41 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:41 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:31:41 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:41 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:41 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:31:41 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-10 23:00:00 (before)
2024-12-11 00:00:00 (after)
20:31:42 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:31:42 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:31:42 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:31:42 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:31:42 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:31:42 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 25x45x2) for time before (2024-12-10 23:00:00)
20:31:42 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-10 23:00:00) in space (linearNDFast)
20:31:42 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:31:42 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:31:42 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.89031327731342 and -65.59415100003532 degrees.
20:31:42 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.89031327731342 and -65.59415100003532 degrees.
20:31:42 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:42 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:31:42 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:31:42 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:31:42 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:31:42 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:31:42 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.938174 (min) -0.599236 (max)
20:31:42 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0587228 (min) 0.672398 (max)
20:31:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.890407 (min) -0.864192 (max)
20:31:42 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.751955 (min) 1.45417 (max)
20:31:42 DEBUG opendrift.models.basemodel.environment:905: y_wind: -1.55529 (min) -1.12152 (max)
20:31:42 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -1.80869e-05 (min) -1.3424e-05 (max)
20:31:42 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:31:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:31:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:31:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:31:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:31:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:31:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:31:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:31:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:31:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:31:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:31:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:31:42 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:31:42 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:31:42 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:31:42 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:31:42 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:31:42 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 296.31 (min) 300.688 (max)
20:31:42 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:31:42 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:31:42 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.043756, mean: 0.054065, max: 0.111525
20:31:42 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:31:42 DEBUG opendrift.models.physics_methods:1061: min: 1.139594, mean: 1.263656, max: 1.819349
20:31:42 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.139594, mean: 1.263656, max: 1.819349
20:31:42 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:31:42 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:42 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:31:42 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:31:42 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.026674 m/s - 0.042584 m/s)
20:31:42 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:31:42 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:31:42 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:31:42 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:31:42 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:31:42 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:42 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:31:42 INFO opendrift.models.basemodel:2035: 2024-12-11 00:00:00 - step 49 of 71 - 1000 active elements (0 deactivated)
20:31:42 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:31:42 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:31:42 DEBUG opendrift.models.basemodel:2054: 60.25002833296831 <- latitude -> 60.304925053276264
20:31:42 DEBUG opendrift.models.basemodel:2059: 4.060435287437407 <- longitude -> 4.368794602453429
20:31:42 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:31:42 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:31:42 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:42 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:31:42 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:42 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:31:42 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:42 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:42 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:31:42 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:31:42 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:31:42 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:31:42 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:42 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:42 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:31:42 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:42 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:31:42 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:42 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:42 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:31:42 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-11 00:00:00 (before)
2024-12-11 01:00:00 (after)
20:31:43 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:31:43 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:31:43 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:31:43 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:31:43 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:31:43 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 27x44x2) for time before (2024-12-11 00:00:00)
20:31:43 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-11 00:00:00) in space (linearNDFast)
20:31:43 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:31:43 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:31:43 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.93957557083176 and -65.6312162476852 degrees.
20:31:43 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.93957557083176 and -65.6312162476852 degrees.
20:31:43 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:43 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:31:43 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:31:43 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:31:43 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:31:43 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:31:43 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.902455 (min) -0.592336 (max)
20:31:43 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0588507 (min) 0.702311 (max)
20:31:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.88474 (min) -0.858883 (max)
20:31:43 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.109356 (min) 1.45752 (max)
20:31:43 DEBUG opendrift.models.basemodel.environment:905: y_wind: -0.850978 (min) 1.05479 (max)
20:31:43 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -3.34108e-06 (min) 4.10517e-06 (max)
20:31:43 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:31:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:31:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:31:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:31:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:31:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:31:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:31:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:31:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:31:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:31:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:31:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:31:43 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:31:43 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:31:43 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:31:43 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:31:43 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:31:43 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 296.414 (min) 300.875 (max)
20:31:43 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:31:43 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:31:43 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.008367, mean: 0.059450, max: 0.070074
20:31:43 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:31:43 DEBUG opendrift.models.physics_methods:1061: min: 0.498339, mean: 1.319870, max: 1.442143
20:31:43 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 0.498339, mean: 1.319870, max: 1.442143
20:31:43 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:31:43 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:43 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:31:43 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:31:43 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.011664 m/s - 0.033755 m/s)
20:31:43 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:31:43 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:31:43 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:31:43 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:31:43 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:31:43 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:43 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:31:43 INFO opendrift.models.basemodel:2035: 2024-12-11 01:00:00 - step 50 of 71 - 1000 active elements (0 deactivated)
20:31:43 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:31:43 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:31:43 DEBUG opendrift.models.basemodel:2054: 60.27327936932171 <- latitude -> 60.314909181654436
20:31:43 DEBUG opendrift.models.basemodel:2059: 4.023634641348323 <- longitude -> 4.325060424152566
20:31:43 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:31:43 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:31:43 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:43 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:31:43 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:43 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:31:43 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:43 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:43 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:31:43 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:31:43 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:31:43 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:31:43 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:43 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:43 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:31:43 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:43 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:31:43 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:43 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:43 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:31:43 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-11 01:00:00 (before)
2024-12-11 02:00:00 (after)
20:31:44 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:31:44 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:31:44 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:31:44 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:31:44 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:31:44 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 29x42x2) for time before (2024-12-11 01:00:00)
20:31:44 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-11 01:00:00) in space (linearNDFast)
20:31:44 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:31:44 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:31:44 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.97637621791291 and -65.67495042759289 degrees.
20:31:44 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.97637621791291 and -65.67495042759289 degrees.
20:31:44 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:44 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:31:44 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:31:44 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:31:44 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:31:44 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:31:44 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.846475 (min) -0.456547 (max)
20:31:44 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0677887 (min) 0.759253 (max)
20:31:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.78475 (min) -0.763402 (max)
20:31:44 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0.690156 (min) 1.77867 (max)
20:31:44 DEBUG opendrift.models.basemodel.environment:905: y_wind: -4.74595 (min) -1.36316 (max)
20:31:44 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 3.32252e-05 (min) 3.79614e-05 (max)
20:31:44 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:31:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:31:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:31:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:31:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:31:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:31:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:31:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:31:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:31:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:31:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:31:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:31:44 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:31:44 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:31:44 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:31:44 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:31:44 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:31:44 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 296.645 (min) 301.072 (max)
20:31:44 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:31:44 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:31:44 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.078082, mean: 0.490537, max: 0.565809
20:31:44 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:31:44 DEBUG opendrift.models.physics_methods:1061: min: 1.522313, mean: 3.808123, max: 4.097930
20:31:44 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.522313, mean: 3.808123, max: 4.097930
20:31:44 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:31:44 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:44 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:31:44 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:31:44 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.035632 m/s - 0.095917 m/s)
20:31:44 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:31:44 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:31:44 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:31:44 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:31:44 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:31:44 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:44 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:31:44 INFO opendrift.models.basemodel:2035: 2024-12-11 02:00:00 - step 51 of 71 - 1000 active elements (0 deactivated)
20:31:44 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:31:44 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:31:44 DEBUG opendrift.models.basemodel:2054: 60.296921962206454 <- latitude -> 60.323835762483824
20:31:44 DEBUG opendrift.models.basemodel:2059: 3.9948069690458388 <- longitude -> 4.278343845578291
20:31:44 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:31:44 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:31:44 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:44 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:31:44 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:44 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:31:44 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:44 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:44 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:31:44 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:31:44 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:31:44 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:31:44 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:44 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:44 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:31:44 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:44 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:31:44 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:44 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:44 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:31:44 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-11 02:00:00 (before)
2024-12-11 03:00:00 (after)
20:31:46 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:31:46 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:31:46 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:31:46 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:31:46 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:31:46 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 30x40x2) for time before (2024-12-11 02:00:00)
20:31:46 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-11 02:00:00) in space (linearNDFast)
20:31:46 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:31:46 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:31:46 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.00520390447426 and -65.72166699384546 degrees.
20:31:46 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.00520390447426 and -65.72166699384546 degrees.
20:31:46 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:46 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:31:46 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:31:46 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:31:46 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:31:46 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:31:46 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.781187 (min) -0.329068 (max)
20:31:46 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0427383 (min) 0.779425 (max)
20:31:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.637641 (min) -0.620871 (max)
20:31:46 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.373128 (min) 0.672754 (max)
20:31:46 DEBUG opendrift.models.basemodel.environment:905: y_wind: -4.87845 (min) -3.98329 (max)
20:31:46 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 4.43327e-05 (min) 4.7708e-05 (max)
20:31:46 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:31:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:31:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:31:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:31:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:31:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:31:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:31:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:31:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:31:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:31:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:31:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:31:46 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:31:46 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:31:46 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:31:46 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:31:46 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:31:46 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 297.218 (min) 301.118 (max)
20:31:46 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:31:46 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:31:46 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.401453, mean: 0.505071, max: 0.588886
20:31:46 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:31:46 DEBUG opendrift.models.physics_methods:1061: min: 3.451811, mean: 3.869897, max: 4.180664
20:31:46 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.451811, mean: 3.869897, max: 4.180664
20:31:46 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:31:46 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:46 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:31:46 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:31:46 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.080794 m/s - 0.097854 m/s)
20:31:46 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:31:46 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:31:46 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:31:46 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:31:46 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:31:46 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:46 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:31:46 INFO opendrift.models.basemodel:2035: 2024-12-11 03:00:00 - step 52 of 71 - 1000 active elements (0 deactivated)
20:31:46 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:31:46 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:31:46 DEBUG opendrift.models.basemodel:2054: 60.298346319521244 <- latitude -> 60.33335650418533
20:31:46 DEBUG opendrift.models.basemodel:2059: 3.972894024666431 <- longitude -> 4.231649564125464
20:31:46 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:31:46 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:31:46 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:46 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:31:46 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:46 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:31:46 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:46 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:46 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:31:46 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:31:46 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:31:46 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:31:46 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:46 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:46 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:31:46 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:46 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:31:46 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:46 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:46 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:31:46 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-11 03:00:00 (before)
2024-12-11 04:00:00 (after)
20:31:47 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:31:47 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:31:47 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:31:47 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:31:47 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:31:47 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 32x37x2) for time before (2024-12-11 03:00:00)
20:31:47 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-11 03:00:00) in space (linearNDFast)
20:31:47 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:31:47 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:31:47 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.02711685111733 and -65.76836128386392 degrees.
20:31:47 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.02711685111733 and -65.76836128386392 degrees.
20:31:47 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:47 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:31:47 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:31:47 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:31:47 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:31:47 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:31:47 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.723419 (min) -0.245165 (max)
20:31:47 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.00410585 (min) 0.662077 (max)
20:31:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.469795 (min) -0.452482 (max)
20:31:47 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.496553 (min) -0.0566925 (max)
20:31:47 DEBUG opendrift.models.basemodel.environment:905: y_wind: -5.93192 (min) -4.27495 (max)
20:31:47 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 4.20515e-05 (min) 5.26173e-05 (max)
20:31:47 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:31:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:31:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:31:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:31:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:31:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:31:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:31:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:31:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:31:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:31:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:31:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:31:47 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:31:47 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:31:47 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:31:47 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:31:47 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:31:47 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.845 (min) 301.032 (max)
20:31:47 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:31:47 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:31:47 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.455635, mean: 0.780789, max: 0.865873
20:31:47 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:31:47 DEBUG opendrift.models.physics_methods:1061: min: 3.677376, mean: 4.810873, max: 5.069400
20:31:47 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.677376, mean: 4.810873, max: 5.069400
20:31:47 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:31:47 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:47 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:31:47 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:31:47 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.086074 m/s - 0.118656 m/s)
20:31:47 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:31:47 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:31:47 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:31:47 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:31:47 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:31:47 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:47 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:31:47 INFO opendrift.models.basemodel:2035: 2024-12-11 04:00:00 - step 53 of 71 - 1000 active elements (0 deactivated)
20:31:47 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:31:47 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:31:47 DEBUG opendrift.models.basemodel:2054: 60.29661252512289 <- latitude -> 60.34273976554495
20:31:47 DEBUG opendrift.models.basemodel:2059: 3.9567572852854536 <- longitude -> 4.185197854496008
20:31:47 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:31:47 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:31:47 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:47 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:31:47 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:47 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:31:47 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:47 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:47 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:31:47 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:31:47 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:31:47 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:31:47 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:47 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:47 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:31:47 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:47 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:31:47 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:47 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:47 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:31:47 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-11 04:00:00 (before)
2024-12-11 05:00:00 (after)
20:31:48 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:31:48 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:31:48 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:31:48 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:31:48 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:31:48 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 34x34x2) for time before (2024-12-11 04:00:00)
20:31:48 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-11 04:00:00) in space (linearNDFast)
20:31:48 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:31:48 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:31:48 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.04325358551431 and -65.81481297774637 degrees.
20:31:48 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.04325358551431 and -65.81481297774637 degrees.
20:31:48 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:48 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:31:48 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:31:48 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:31:48 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:31:48 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:31:48 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.65914 (min) -0.184309 (max)
20:31:48 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0628039 (min) 0.467208 (max)
20:31:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.300789 (min) -0.280599 (max)
20:31:48 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.8933 (min) -0.328837 (max)
20:31:48 DEBUG opendrift.models.basemodel.environment:905: y_wind: -6.31522 (min) -5.54635 (max)
20:31:48 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 4.73449e-05 (min) 5.18177e-05 (max)
20:31:48 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:31:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:31:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:31:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:31:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:31:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:31:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:31:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:31:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:31:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:31:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:31:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:31:48 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:31:48 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:31:48 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:31:48 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:31:48 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:31:48 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 299.221 (min) 300.867 (max)
20:31:48 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:31:48 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:31:48 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.759405, mean: 0.986300, max: 0.993776
20:31:48 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:31:48 DEBUG opendrift.models.physics_methods:1061: min: 4.747516, mean: 5.410332, max: 5.430925
20:31:48 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 4.747516, mean: 5.410332, max: 5.430925
20:31:48 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:31:48 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:48 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:31:48 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:31:48 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.111122 m/s - 0.127118 m/s)
20:31:48 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:31:48 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:31:48 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:31:48 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:31:48 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:31:48 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:48 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:31:48 INFO opendrift.models.basemodel:2035: 2024-12-11 05:00:00 - step 54 of 71 - 1000 active elements (0 deactivated)
20:31:48 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:31:48 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:31:48 DEBUG opendrift.models.basemodel:2054: 60.29219069877223 <- latitude -> 60.34964992870665
20:31:48 DEBUG opendrift.models.basemodel:2059: 3.943100789481431 <- longitude -> 4.141982094407804
20:31:48 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:31:48 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:31:48 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:48 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:31:48 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:48 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:31:48 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:48 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:48 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:31:48 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:31:48 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:31:48 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:31:48 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:48 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:48 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:31:48 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:48 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:31:48 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:48 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:48 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:31:48 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-11 05:00:00 (before)
2024-12-11 06:00:00 (after)
20:31:49 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:31:49 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:31:49 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:31:49 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:31:49 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:31:49 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 34x32x2) for time before (2024-12-11 05:00:00)
20:31:49 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-11 05:00:00) in space (linearNDFast)
20:31:49 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:31:49 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:31:49 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.05691008305988 and -65.85802874253977 degrees.
20:31:49 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.05691008305988 and -65.85802874253977 degrees.
20:31:49 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:49 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:31:49 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:31:49 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:31:49 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:31:49 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:31:49 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.592285 (min) -0.156632 (max)
20:31:49 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.125622 (min) 0.219443 (max)
20:31:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.14715 (min) -0.126915 (max)
20:31:49 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.104781 (min) 0.588626 (max)
20:31:49 DEBUG opendrift.models.basemodel.environment:905: y_wind: -5.5975 (min) -5.30859 (max)
20:31:49 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 3.70078e-05 (min) 4.01758e-05 (max)
20:31:49 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:31:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:31:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:31:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:31:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:31:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:31:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:31:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:31:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:31:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:31:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:31:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:31:49 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:31:49 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:31:49 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:31:49 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:31:49 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:31:49 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 299.122 (min) 300.686 (max)
20:31:49 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:31:49 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:31:49 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.701779, mean: 0.761648, max: 0.771252
20:31:49 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:31:49 DEBUG opendrift.models.physics_methods:1061: min: 4.563832, mean: 4.754479, max: 4.784404
20:31:49 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 4.563832, mean: 4.754479, max: 4.784404
20:31:49 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:31:49 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:49 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:31:49 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:31:49 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.106822 m/s - 0.111985 m/s)
20:31:49 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:31:49 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:31:49 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:31:49 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:31:49 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:31:49 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:49 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:31:49 INFO opendrift.models.basemodel:2035: 2024-12-11 06:00:00 - step 55 of 71 - 1000 active elements (0 deactivated)
20:31:49 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:31:49 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:31:49 DEBUG opendrift.models.basemodel:2054: 60.28564794216131 <- latitude -> 60.35330420452856
20:31:49 DEBUG opendrift.models.basemodel:2059: 3.93196329078238 <- longitude -> 4.104121243589553
20:31:49 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:31:49 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:31:49 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:49 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:31:49 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:49 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:31:49 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:49 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:49 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:31:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:31:49 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:31:49 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:31:49 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:49 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:49 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:31:49 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:49 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:31:49 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:49 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:49 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:31:49 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-11 06:00:00 (before)
2024-12-11 07:00:00 (after)
20:31:50 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:31:50 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:31:50 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:31:50 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:31:50 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:31:50 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 35x30x2) for time before (2024-12-11 06:00:00)
20:31:50 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-11 06:00:00) in space (linearNDFast)
20:31:50 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:31:50 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:31:50 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.06804758712488 and -65.89588959433549 degrees.
20:31:50 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.06804758712488 and -65.89588959433549 degrees.
20:31:50 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:50 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:31:50 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:31:50 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:31:50 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:31:50 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:31:50 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.535251 (min) -0.155966 (max)
20:31:50 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.204306 (min) 0.0115113 (max)
20:31:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.0520127 (min) -0.0384475 (max)
20:31:50 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0.460743 (min) 0.957751 (max)
20:31:50 DEBUG opendrift.models.basemodel.environment:905: y_wind: -5.74754 (min) -5.60869 (max)
20:31:50 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 1.17546e-05 (min) 1.48495e-05 (max)
20:31:50 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:31:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:31:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:31:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:31:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:31:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:31:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:31:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:31:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:31:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:31:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:31:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:31:50 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:31:50 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:31:50 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:31:50 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:31:50 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:31:50 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 299.019 (min) 300.527 (max)
20:31:50 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:31:50 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:31:50 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.789883, mean: 0.800626, max: 0.818033
20:31:50 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:31:50 DEBUG opendrift.models.physics_methods:1061: min: 4.841846, mean: 4.874607, max: 4.927368
20:31:50 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 4.841846, mean: 4.874607, max: 4.927368
20:31:50 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:31:50 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:50 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:31:50 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:31:50 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.113330 m/s - 0.115331 m/s)
20:31:50 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:31:50 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:31:50 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:31:50 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:31:50 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:31:50 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:50 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:31:50 INFO opendrift.models.basemodel:2035: 2024-12-11 07:00:00 - step 56 of 71 - 1000 active elements (0 deactivated)
20:31:50 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:31:50 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:31:50 DEBUG opendrift.models.basemodel:2054: 60.2764593412068 <- latitude -> 60.350038473196335
20:31:50 DEBUG opendrift.models.basemodel:2059: 3.9218125359937575 <- longitude -> 4.070464704692413
20:31:50 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:31:50 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:31:50 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:50 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:31:50 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:50 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:31:50 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:50 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:50 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:31:50 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:31:50 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:31:50 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:31:50 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:50 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:50 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:31:50 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:50 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:31:50 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:50 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:50 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:31:50 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-11 07:00:00 (before)
2024-12-11 08:00:00 (after)
20:31:51 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:31:51 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:31:51 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:31:51 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:31:51 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:31:51 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 35x28x2) for time before (2024-12-11 07:00:00)
20:31:51 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-11 07:00:00) in space (linearNDFast)
20:31:51 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:31:51 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:31:51 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.07819833180443 and -65.9295461364842 degrees.
20:31:51 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.07819833180443 and -65.9295461364842 degrees.
20:31:51 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:51 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:31:51 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:31:51 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:31:51 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:31:51 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:31:51 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.431798 (min) -0.167127 (max)
20:31:51 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.278253 (min) -0.0944813 (max)
20:31:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.0607067 (min) -0.0536718 (max)
20:31:51 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0.591351 (min) 1.08914 (max)
20:31:51 DEBUG opendrift.models.basemodel.environment:905: y_wind: -4.52228 (min) -4.33884 (max)
20:31:51 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -1.8662e-05 (min) -1.78118e-05 (max)
20:31:51 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:31:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:31:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:31:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:31:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:31:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:31:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:31:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:31:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:31:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:31:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:31:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:31:51 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:31:51 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:31:51 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:31:51 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:31:51 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:31:51 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.88 (min) 300.427 (max)
20:31:51 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:31:51 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:31:51 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.472365, mean: 0.489889, max: 0.532276
20:31:51 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:31:51 DEBUG opendrift.models.physics_methods:1061: min: 3.744282, mean: 3.813009, max: 3.974644
20:31:51 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.744282, mean: 3.813009, max: 3.974644
20:31:51 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:31:51 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:51 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:31:51 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:31:51 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.087640 m/s - 0.093032 m/s)
20:31:51 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:31:51 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:31:51 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:31:51 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:31:51 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:31:51 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:51 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:31:51 INFO opendrift.models.basemodel:2035: 2024-12-11 08:00:00 - step 57 of 71 - 1000 active elements (0 deactivated)
20:31:51 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:31:51 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:31:51 DEBUG opendrift.models.basemodel:2054: 60.26660081190933 <- latitude -> 60.3419348684138
20:31:51 DEBUG opendrift.models.basemodel:2059: 3.911364474887392 <- longitude -> 4.043733287533514
20:31:51 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:31:51 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:31:51 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:51 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:31:51 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:51 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:31:51 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:51 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:51 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:31:51 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:31:51 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:31:51 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:31:51 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:51 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:51 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:31:51 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:51 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:31:51 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:51 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:51 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:31:51 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-11 08:00:00 (before)
2024-12-11 09:00:00 (after)
20:31:53 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:31:53 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:31:53 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:31:53 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:31:53 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:31:53 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 34x27x2) for time before (2024-12-11 08:00:00)
20:31:53 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-11 08:00:00) in space (linearNDFast)
20:31:53 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:31:53 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:31:53 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.08864640069656 and -65.95627756662104 degrees.
20:31:53 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.08864640069656 and -65.95627756662104 degrees.
20:31:53 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:53 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:31:53 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:31:53 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:31:53 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:31:53 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:31:53 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.357354 (min) -0.176394 (max)
20:31:53 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.33839 (min) -0.102564 (max)
20:31:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.165808 (min) -0.159859 (max)
20:31:53 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0.278048 (min) 0.370729 (max)
20:31:53 DEBUG opendrift.models.basemodel.environment:905: y_wind: -4.98193 (min) -4.74908 (max)
20:31:53 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -4.78194e-05 (min) -4.61756e-05 (max)
20:31:53 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:31:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:31:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:31:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:31:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:31:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:31:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:31:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:31:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:31:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:31:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:31:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:31:53 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:31:53 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:31:53 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:31:53 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:31:53 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:31:53 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.688 (min) 300.379 (max)
20:31:53 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:31:53 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:31:53 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.557610, mean: 0.576977, max: 0.613943
20:31:53 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:31:53 DEBUG opendrift.models.physics_methods:1061: min: 4.068129, mean: 4.138062, max: 4.268679
20:31:53 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 4.068129, mean: 4.138062, max: 4.268679
20:31:53 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:31:53 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:53 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:31:53 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:31:53 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.095220 m/s - 0.099914 m/s)
20:31:53 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:31:53 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:31:53 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:31:53 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:31:53 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:31:53 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:53 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:31:53 INFO opendrift.models.basemodel:2035: 2024-12-11 09:00:00 - step 58 of 71 - 1000 active elements (0 deactivated)
20:31:53 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:31:53 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:31:53 DEBUG opendrift.models.basemodel:2054: 60.25602488771975 <- latitude -> 60.32939773308573
20:31:53 DEBUG opendrift.models.basemodel:2059: 3.9000080297765636 <- longitude -> 4.020927165525108
20:31:53 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:31:53 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:31:53 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:53 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:31:53 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:53 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:31:53 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:53 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:53 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:31:53 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:31:53 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:31:53 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:31:53 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:53 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:53 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:31:53 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:53 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:31:53 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:53 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:53 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:31:53 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-11 09:00:00 (before)
2024-12-11 10:00:00 (after)
20:31:54 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:31:54 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:31:54 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:31:54 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:31:54 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:31:54 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 34x27x2) for time before (2024-12-11 09:00:00)
20:31:54 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-11 09:00:00) in space (linearNDFast)
20:31:54 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:31:54 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:31:54 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.10000286124225 and -65.97908369621815 degrees.
20:31:54 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.10000286124225 and -65.97908369621815 degrees.
20:31:54 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:54 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:31:54 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:31:54 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:31:54 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:31:54 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:31:54 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.30918 (min) -0.160157 (max)
20:31:54 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.38037 (min) -0.0738321 (max)
20:31:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.365299 (min) -0.352452 (max)
20:31:54 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.728477 (min) -0.506769 (max)
20:31:54 DEBUG opendrift.models.basemodel.environment:905: y_wind: -4.44507 (min) -4.25651 (max)
20:31:54 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -6.43302e-05 (min) -6.22301e-05 (max)
20:31:54 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:31:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:31:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:31:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:31:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:31:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:31:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:31:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:31:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:31:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:31:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:31:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:31:54 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:31:54 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:31:54 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:31:54 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:31:54 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:31:54 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.433 (min) 300.167 (max)
20:31:54 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:31:54 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:31:54 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.452017, mean: 0.490130, max: 0.497693
20:31:54 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:31:54 DEBUG opendrift.models.physics_methods:1061: min: 3.662746, mean: 3.813966, max: 3.843352
20:31:54 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.662746, mean: 3.813966, max: 3.843352
20:31:54 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:31:54 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:54 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:31:54 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:31:54 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.085731 m/s - 0.089959 m/s)
20:31:54 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:31:54 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:31:54 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:31:54 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:31:54 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:31:54 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:54 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:31:54 INFO opendrift.models.basemodel:2035: 2024-12-11 10:00:00 - step 59 of 71 - 1000 active elements (0 deactivated)
20:31:54 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:31:54 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:31:54 DEBUG opendrift.models.basemodel:2054: 60.24698158195913 <- latitude -> 60.31469327683935
20:31:54 DEBUG opendrift.models.basemodel:2059: 3.887407679258629 <- longitude -> 4.000126605039542
20:31:54 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:31:54 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:31:54 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:54 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:31:54 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:54 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:31:54 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:54 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:54 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:31:54 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:31:54 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:31:54 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:31:54 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:54 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:54 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:31:54 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:54 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:31:54 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:54 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:54 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:31:54 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-11 10:00:00 (before)
2024-12-11 11:00:00 (after)
20:31:55 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:31:55 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:31:55 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:31:55 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:31:55 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:31:55 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 34x27x2) for time before (2024-12-11 10:00:00)
20:31:55 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-11 10:00:00) in space (linearNDFast)
20:31:55 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:31:55 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:31:55 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.11260320626268 and -65.99988425271412 degrees.
20:31:55 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.11260320626268 and -65.99988425271412 degrees.
20:31:55 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:55 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:31:55 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:31:55 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:31:55 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:31:55 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:31:55 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.266036 (min) -0.140208 (max)
20:31:55 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.396535 (min) -0.00820025 (max)
20:31:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.570347 (min) -0.554415 (max)
20:31:55 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.949336 (min) -0.824939 (max)
20:31:55 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.33793 (min) -3.26235 (max)
20:31:55 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -5.05952e-05 (min) -4.93243e-05 (max)
20:31:55 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:31:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:31:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:31:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:31:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:31:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:31:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:31:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:31:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:31:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:31:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:31:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:31:55 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:31:55 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:31:55 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:31:55 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:31:55 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:31:55 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.229 (min) 299.807 (max)
20:31:55 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:31:55 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:31:55 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.283626, mean: 0.293276, max: 0.294942
20:31:55 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:31:55 DEBUG opendrift.models.physics_methods:1061: min: 2.901367, mean: 2.950292, max: 2.958679
20:31:55 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.901367, mean: 2.950292, max: 2.958679
20:31:55 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:31:55 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:55 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:31:55 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:31:55 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.067910 m/s - 0.069252 m/s)
20:31:55 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:31:55 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:31:55 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:31:55 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:31:55 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:31:55 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:55 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:31:55 INFO opendrift.models.basemodel:2035: 2024-12-11 11:00:00 - step 60 of 71 - 1000 active elements (0 deactivated)
20:31:55 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:31:55 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:31:55 DEBUG opendrift.models.basemodel:2054: 60.241269770976515 <- latitude -> 60.29977154385165
20:31:55 DEBUG opendrift.models.basemodel:2059: 3.8754960239432346 <- longitude -> 3.981578924839883
20:31:55 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:31:55 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:31:55 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:55 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:31:55 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:55 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:31:55 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:55 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:55 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:31:55 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:31:55 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:31:55 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:31:55 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:55 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:55 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:31:55 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:55 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:31:55 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:55 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:55 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:31:55 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-11 11:00:00 (before)
2024-12-11 12:00:00 (after)
20:31:56 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:31:56 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:31:56 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:31:56 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:31:56 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:31:56 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 32x27x2) for time before (2024-12-11 11:00:00)
20:31:56 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-11 11:00:00) in space (linearNDFast)
20:31:56 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:31:56 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:31:56 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.12451486045629 and -66.01843194424555 degrees.
20:31:56 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.12451486045629 and -66.01843194424555 degrees.
20:31:56 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:56 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:31:56 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:31:56 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:31:56 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:31:56 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:31:56 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.21667 (min) -0.124769 (max)
20:31:56 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.371453 (min) 0.0803197 (max)
20:31:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.70944 (min) -0.693409 (max)
20:31:56 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.962754 (min) -0.734061 (max)
20:31:56 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.53333 (min) -2.39367 (max)
20:31:56 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -2.69913e-05 (min) -2.60857e-05 (max)
20:31:56 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:31:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:31:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:31:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:31:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:31:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:31:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:31:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:31:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:31:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:31:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:31:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:31:56 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:31:56 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:31:56 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:31:56 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:31:56 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:31:56 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.18 (min) 299.425 (max)
20:31:56 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:31:56 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:31:56 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.158606, mean: 0.177196, max: 0.180394
20:31:56 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:31:56 DEBUG opendrift.models.physics_methods:1061: min: 2.169649, mean: 2.293235, max: 2.313880
20:31:56 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.169649, mean: 2.293235, max: 2.313880
20:31:56 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:31:56 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:56 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:31:56 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:31:56 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.050783 m/s - 0.054159 m/s)
20:31:56 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:31:56 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:31:56 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:31:56 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:31:56 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:31:56 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:56 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:31:56 INFO opendrift.models.basemodel:2035: 2024-12-11 12:00:00 - step 61 of 71 - 1000 active elements (0 deactivated)
20:31:56 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:31:56 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:31:56 DEBUG opendrift.models.basemodel:2054: 60.238784396262176 <- latitude -> 60.28619510817677
20:31:56 DEBUG opendrift.models.basemodel:2059: 3.8657740681239776 <- longitude -> 3.9665221312631207
20:31:56 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:31:56 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:31:56 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:56 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:31:56 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:56 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:31:56 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:56 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:56 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:31:56 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:31:56 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:31:56 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:31:56 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:56 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:56 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:31:56 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:56 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:31:56 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:56 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:56 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:31:56 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-11 12:00:00 (before)
2024-12-11 13:00:00 (after)
20:31:57 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:31:57 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:31:57 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:31:57 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:31:57 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:31:57 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 30x28x2) for time before (2024-12-11 12:00:00)
20:31:57 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-11 12:00:00) in space (linearNDFast)
20:31:57 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:31:57 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:31:57 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.13423681220267 and -66.03348875529231 degrees.
20:31:57 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.13423681220267 and -66.03348875529231 degrees.
20:31:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:57 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:31:57 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:31:57 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:31:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:31:57 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:31:57 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.150675 (min) -0.0882755 (max)
20:31:57 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.316291 (min) 0.170666 (max)
20:31:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.750791 (min) -0.737175 (max)
20:31:57 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.0301458 (min) 0.319299 (max)
20:31:57 DEBUG opendrift.models.basemodel.environment:905: y_wind: -1.6402 (min) -1.47001 (max)
20:31:57 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 1.80097e-06 (min) 2.9226e-06 (max)
20:31:57 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:31:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:31:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:31:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:31:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:31:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:31:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:31:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:31:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:31:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:31:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:31:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:31:57 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:31:57 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:31:57 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:31:57 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:31:57 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:31:57 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.291 (min) 299.258 (max)
20:31:57 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:31:57 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:31:57 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.054525, mean: 0.064411, max: 0.066198
20:31:57 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:31:57 DEBUG opendrift.models.physics_methods:1061: min: 1.272120, mean: 1.382607, max: 1.401693
20:31:57 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.272120, mean: 1.382607, max: 1.401693
20:31:57 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:31:57 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:57 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:31:57 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:31:57 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.029776 m/s - 0.032808 m/s)
20:31:57 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:31:57 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:31:57 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:31:57 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:31:57 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:31:57 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:57 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:31:57 INFO opendrift.models.basemodel:2035: 2024-12-11 13:00:00 - step 62 of 71 - 1000 active elements (0 deactivated)
20:31:57 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:31:57 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:31:57 DEBUG opendrift.models.basemodel:2054: 60.23994093132491 <- latitude -> 60.27549056515753
20:31:57 DEBUG opendrift.models.basemodel:2059: 3.8603375633102432 <- longitude -> 3.9571348404326487
20:31:57 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:31:57 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:31:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:31:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:31:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:31:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:31:57 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:31:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:31:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:31:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:31:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:31:57 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-11 13:00:00 (before)
2024-12-11 14:00:00 (after)
20:31:58 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:31:58 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:31:58 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:31:58 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:31:58 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:31:58 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 28x28x2) for time before (2024-12-11 13:00:00)
20:31:58 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-11 13:00:00) in space (linearNDFast)
20:31:58 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:31:58 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:31:58 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.13967331710408 and -66.04287604498178 degrees.
20:31:58 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.13967331710408 and -66.04287604498178 degrees.
20:31:58 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:58 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:31:58 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:31:58 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:31:58 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:31:58 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:31:58 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.10345 (min) -0.0347647 (max)
20:31:58 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.263182 (min) 0.246879 (max)
20:31:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.691376 (min) -0.681384 (max)
20:31:58 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0.914813 (min) 1.18727 (max)
20:31:58 DEBUG opendrift.models.basemodel.environment:905: y_wind: -0.772791 (min) -0.627169 (max)
20:31:58 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 2.72857e-05 (min) 2.78221e-05 (max)
20:31:58 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:31:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:31:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:31:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:31:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:31:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:31:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:31:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:31:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:31:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:31:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:31:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:31:58 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:31:58 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:31:58 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:31:58 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:31:58 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:31:58 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.15 (min) 299.36 (max)
20:31:58 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:31:58 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:31:58 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.034116, mean: 0.039071, max: 0.048254
20:31:58 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:31:58 DEBUG opendrift.models.physics_methods:1061: min: 1.006261, mean: 1.076475, max: 1.196726
20:31:58 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.006261, mean: 1.076475, max: 1.196726
20:31:58 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:31:58 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:58 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:31:58 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:31:58 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.023553 m/s - 0.028011 m/s)
20:31:58 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:31:58 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:31:58 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:31:58 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:31:58 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:31:58 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:58 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:31:58 INFO opendrift.models.basemodel:2035: 2024-12-11 14:00:00 - step 63 of 71 - 1000 active elements (0 deactivated)
20:31:58 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:31:58 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:31:58 DEBUG opendrift.models.basemodel:2054: 60.243859076130455 <- latitude -> 60.28306216206
20:31:58 DEBUG opendrift.models.basemodel:2059: 3.8595971682956147 <- longitude -> 3.952869515513068
20:31:58 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:31:58 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:31:58 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:58 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:31:58 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:58 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:31:58 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:58 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:58 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:31:58 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:31:58 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:31:58 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:31:58 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:58 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:58 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:31:58 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:58 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:31:58 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:58 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:58 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:31:58 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-11 14:00:00 (before)
2024-12-11 15:00:00 (after)
20:31:59 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:31:59 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:31:59 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:31:59 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:31:59 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:31:59 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 27x29x2) for time before (2024-12-11 14:00:00)
20:31:59 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-11 14:00:00) in space (linearNDFast)
20:31:59 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:31:59 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:31:59 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.14041372091427 and -66.0471413607194 degrees.
20:31:59 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.14041372091427 and -66.0471413607194 degrees.
20:31:59 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:59 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:31:59 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:31:59 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:31:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:31:59 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:31:59 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0620337 (min) 0.0221149 (max)
20:31:59 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.225419 (min) 0.285807 (max)
20:31:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.564217 (min) -0.556 (max)
20:31:59 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.30424 (min) 1.63626 (max)
20:31:59 DEBUG opendrift.models.basemodel.environment:905: y_wind: -0.26342 (min) -0.156463 (max)
20:31:59 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 4.08157e-05 (min) 4.10259e-05 (max)
20:31:59 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:31:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:31:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:31:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:31:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:31:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:31:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:31:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:31:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:31:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:31:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:31:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:31:59 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:31:59 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:31:59 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:31:59 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:31:59 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:31:59 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.088 (min) 299.407 (max)
20:31:59 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:31:59 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:31:59 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.042542, mean: 0.049343, max: 0.066772
20:31:59 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:31:59 DEBUG opendrift.models.physics_methods:1061: min: 1.123668, mean: 1.209557, max: 1.407754
20:31:59 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.123668, mean: 1.209557, max: 1.407754
20:31:59 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:31:59 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:59 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:31:59 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:31:59 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.026301 m/s - 0.032950 m/s)
20:31:59 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:31:59 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:31:59 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:31:59 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:31:59 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:31:59 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:31:59 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:31:59 INFO opendrift.models.basemodel:2035: 2024-12-11 15:00:00 - step 64 of 71 - 1000 active elements (0 deactivated)
20:31:59 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:31:59 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:31:59 DEBUG opendrift.models.basemodel:2054: 60.243456575531354 <- latitude -> 60.29217263565762
20:31:59 DEBUG opendrift.models.basemodel:2059: 3.8626520376607267 <- longitude -> 3.9530051207233137
20:31:59 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:31:59 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:31:59 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:59 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:31:59 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:59 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:31:59 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:59 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:59 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:31:59 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:31:59 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:31:59 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:31:59 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:31:59 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:31:59 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:31:59 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:31:59 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:31:59 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:31:59 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:31:59 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:31:59 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-11 15:00:00 (before)
2024-12-11 16:00:00 (after)
20:32:00 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:32:00 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:32:00 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:32:00 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:32:00 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:32:00 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 27x29x2) for time before (2024-12-11 15:00:00)
20:32:00 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-11 15:00:00) in space (linearNDFast)
20:32:00 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:32:00 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:32:00 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.1373588554738 and -66.0470057615131 degrees.
20:32:00 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.1373588554738 and -66.0470057615131 degrees.
20:32:00 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:32:00 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:32:00 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:32:00 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:32:00 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:32:00 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:32:00 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.00956874 (min) 0.0708048 (max)
20:32:00 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.217294 (min) 0.282098 (max)
20:32:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.407288 (min) -0.4 (max)
20:32:00 DEBUG opendrift.models.basemodel.environment:905: x_wind: 2.14935 (min) 2.54798 (max)
20:32:00 DEBUG opendrift.models.basemodel.environment:905: y_wind: -0.158186 (min) -0.042322 (max)
20:32:00 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 4.56358e-05 (min) 4.70922e-05 (max)
20:32:00 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:32:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:32:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:32:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:32:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:32:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:32:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:32:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:32:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:32:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:32:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:32:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:32:00 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:32:00 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:32:00 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:32:00 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:32:00 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:32:00 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 297.973 (min) 299.387 (max)
20:32:00 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:32:00 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:32:00 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.113891, mean: 0.120128, max: 0.160323
20:32:00 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:32:00 DEBUG opendrift.models.physics_methods:1061: min: 1.838548, mean: 1.888036, max: 2.181364
20:32:00 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.838548, mean: 1.888036, max: 2.181364
20:32:00 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:32:00 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:32:00 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:32:00 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:32:00 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.043034 m/s - 0.051058 m/s)
20:32:00 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:32:00 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:32:00 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:32:00 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:32:00 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:32:00 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:32:00 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:32:00 INFO opendrift.models.basemodel:2035: 2024-12-11 16:00:00 - step 65 of 71 - 1000 active elements (0 deactivated)
20:32:00 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:32:00 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:32:00 DEBUG opendrift.models.basemodel:2054: 60.24100950332016 <- latitude -> 60.301185186410656
20:32:00 DEBUG opendrift.models.basemodel:2059: 3.868898153280922 <- longitude -> 3.9577690540970547
20:32:00 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:32:00 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:32:00 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:32:00 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:32:00 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:32:00 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:32:00 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:32:00 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:32:00 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:32:00 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:32:00 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:32:00 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:32:00 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:32:00 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:32:00 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:32:00 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:32:00 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:32:00 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:32:00 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:32:00 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:32:00 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-11 16:00:00 (before)
2024-12-11 17:00:00 (after)
20:32:01 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:32:01 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:32:01 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:32:01 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:32:01 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:32:01 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 28x30x2) for time before (2024-12-11 16:00:00)
20:32:01 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-11 16:00:00) in space (linearNDFast)
20:32:01 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:32:01 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:32:01 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.13111273472065 and -66.04224183759061 degrees.
20:32:01 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.13111273472065 and -66.04224183759061 degrees.
20:32:01 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:32:01 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:32:01 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:32:01 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:32:01 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:32:01 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:32:01 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0342675 (min) 0.105671 (max)
20:32:01 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.252015 (min) 0.229054 (max)
20:32:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.235145 (min) -0.222058 (max)
20:32:01 DEBUG opendrift.models.basemodel.environment:905: x_wind: 2.31252 (min) 2.55642 (max)
20:32:01 DEBUG opendrift.models.basemodel.environment:905: y_wind: -0.760713 (min) -0.138792 (max)
20:32:01 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 4.74537e-05 (min) 4.96953e-05 (max)
20:32:01 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:32:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:32:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:32:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:32:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:32:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:32:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:32:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:32:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:32:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:32:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:32:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:32:01 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:32:01 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:32:01 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:32:01 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:32:01 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:32:01 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 297.869 (min) 299.316 (max)
20:32:01 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:32:01 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:32:01 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.132049, mean: 0.141859, max: 0.172067
20:32:01 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:32:01 DEBUG opendrift.models.physics_methods:1061: min: 1.979692, mean: 2.051584, max: 2.259844
20:32:01 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.979692, mean: 2.051584, max: 2.259844
20:32:01 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:32:01 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:32:01 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:32:01 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:32:01 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.046337 m/s - 0.052895 m/s)
20:32:01 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:32:01 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:32:01 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:32:01 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:32:01 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:32:01 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:32:01 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:32:01 INFO opendrift.models.basemodel:2035: 2024-12-11 17:00:00 - step 66 of 71 - 1000 active elements (0 deactivated)
20:32:01 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:32:01 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:32:01 DEBUG opendrift.models.basemodel:2054: 60.23762505957211 <- latitude -> 60.30809449936915
20:32:01 DEBUG opendrift.models.basemodel:2059: 3.8751928354886265 <- longitude -> 3.9665594348358364
20:32:01 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:32:01 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:32:01 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:32:01 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:32:01 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:32:01 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:32:01 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:32:01 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:32:01 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:32:01 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:32:01 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:32:01 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:32:01 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:32:01 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:32:01 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:32:01 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:32:01 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:32:01 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:32:01 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:32:01 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:32:01 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-11 17:00:00 (before)
2024-12-11 18:00:00 (after)
20:32:03 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:32:03 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:32:03 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:32:03 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:32:03 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:32:03 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 29x31x2) for time before (2024-12-11 17:00:00)
20:32:03 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-11 17:00:00) in space (linearNDFast)
20:32:03 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:32:03 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:32:03 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.12481803954033 and -66.03345145358492 degrees.
20:32:03 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.12481803954033 and -66.03345145358492 degrees.
20:32:03 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:32:03 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:32:03 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:32:03 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:32:03 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:32:03 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:32:03 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0120915 (min) 0.14006 (max)
20:32:03 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.319339 (min) 0.14979 (max)
20:32:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.0785903 (min) -0.0562353 (max)
20:32:03 DEBUG opendrift.models.basemodel.environment:905: x_wind: 2.13272 (min) 2.66201 (max)
20:32:03 DEBUG opendrift.models.basemodel.environment:905: y_wind: -1.50143 (min) -0.839712 (max)
20:32:03 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 3.92358e-05 (min) 4.10474e-05 (max)
20:32:03 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:32:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:32:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:32:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:32:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:32:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:32:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:32:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:32:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:32:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:32:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:32:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:32:03 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:32:03 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:32:03 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:32:03 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:32:03 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:32:03 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 297.722 (min) 299.253 (max)
20:32:03 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:32:03 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:32:03 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.167349, mean: 0.186619, max: 0.191750
20:32:03 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:32:03 DEBUG opendrift.models.physics_methods:1061: min: 2.228646, mean: 2.353391, max: 2.385600
20:32:03 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.228646, mean: 2.353391, max: 2.385600
20:32:03 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:32:03 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:32:03 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:32:03 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:32:03 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.052164 m/s - 0.055838 m/s)
20:32:03 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:32:03 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:32:03 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:32:03 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:32:03 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:32:03 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:32:03 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:32:03 INFO opendrift.models.basemodel:2035: 2024-12-11 18:00:00 - step 67 of 71 - 1000 active elements (0 deactivated)
20:32:03 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:32:03 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:32:03 DEBUG opendrift.models.basemodel:2054: 60.231172904569696 <- latitude -> 60.31196405466584
20:32:03 DEBUG opendrift.models.basemodel:2059: 3.878758478782234 <- longitude -> 3.9791187380532844
20:32:03 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:32:03 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:32:03 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:32:03 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:32:03 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:32:03 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:32:03 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:32:03 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:32:03 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:32:03 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:32:03 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:32:03 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:32:03 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:32:03 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:32:03 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:32:03 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:32:03 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:32:03 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:32:03 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:32:03 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:32:03 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-11 18:00:00 (before)
2024-12-11 19:00:00 (after)
20:32:03 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:32:03 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:32:03 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:32:03 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:32:03 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:32:03 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 31x32x2) for time before (2024-12-11 18:00:00)
20:32:03 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-11 18:00:00) in space (linearNDFast)
20:32:03 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:32:03 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:32:03 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.12125239241436 and -66.02089214798814 degrees.
20:32:03 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.12125239241436 and -66.02089214798814 degrees.
20:32:03 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:32:03 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:32:03 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:32:03 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:32:03 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:32:03 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:32:03 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0142452 (min) 0.17618 (max)
20:32:03 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.399498 (min) 0.0704574 (max)
20:32:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.0338341 (min) 0.0637175 (max)
20:32:03 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0.591101 (min) 1.96856 (max)
20:32:03 DEBUG opendrift.models.basemodel.environment:905: y_wind: -1.87434 (min) -1.45603 (max)
20:32:03 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 2.09305e-05 (min) 2.21717e-05 (max)
20:32:03 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:32:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:32:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:32:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:32:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:32:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:32:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:32:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:32:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:32:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:32:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:32:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:32:03 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:32:03 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:32:03 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:32:03 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:32:03 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:32:03 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 297.504 (min) 299.216 (max)
20:32:03 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:32:03 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:32:04 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.095019, mean: 0.129454, max: 0.147484
20:32:04 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:32:04 DEBUG opendrift.models.physics_methods:1061: min: 1.679322, mean: 1.959303, max: 2.092191
20:32:04 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.679322, mean: 1.959303, max: 2.092191
20:32:04 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:32:04 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:32:04 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:32:04 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:32:04 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.039307 m/s - 0.048970 m/s)
20:32:04 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:32:04 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:32:04 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:32:04 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:32:04 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:32:04 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:32:04 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:32:04 INFO opendrift.models.basemodel:2035: 2024-12-11 19:00:00 - step 68 of 71 - 1000 active elements (0 deactivated)
20:32:04 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:32:04 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:32:04 DEBUG opendrift.models.basemodel:2054: 60.21850926539901 <- latitude -> 60.31302936034645
20:32:04 DEBUG opendrift.models.basemodel:2059: 3.878600618706879 <- longitude -> 3.9931179971435906
20:32:04 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:32:04 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:32:04 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:32:04 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:32:04 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:32:04 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:32:04 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:32:04 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:32:04 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:32:04 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:32:04 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:32:04 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:32:04 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:32:04 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:32:04 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:32:04 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:32:04 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:32:04 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:32:04 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:32:04 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:32:04 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-11 19:00:00 (before)
2024-12-11 20:00:00 (after)
20:32:05 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:32:05 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:32:05 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:32:05 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:32:05 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:32:05 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 31x34x2) for time before (2024-12-11 19:00:00)
20:32:05 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-11 19:00:00) in space (linearNDFast)
20:32:05 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:32:05 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:32:05 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.12141024713436 and -66.00689289912097 degrees.
20:32:05 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.12141024713436 and -66.00689289912097 degrees.
20:32:05 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:32:05 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:32:05 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:32:05 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:32:05 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:32:05 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:32:05 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0477137 (min) 0.19184 (max)
20:32:05 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.468969 (min) 0.0139148 (max)
20:32:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.0533657 (min) 0.0839939 (max)
20:32:05 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0.584646 (min) 1.07708 (max)
20:32:05 DEBUG opendrift.models.basemodel.environment:905: y_wind: -1.97041 (min) -1.74576 (max)
20:32:05 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -9.50741e-06 (min) -9.07012e-06 (max)
20:32:05 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:32:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:32:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:32:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:32:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:32:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:32:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:32:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:32:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:32:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:32:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:32:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:32:05 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:32:05 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:32:05 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:32:05 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:32:05 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:32:05 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 297.227 (min) 299.213 (max)
20:32:05 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:32:05 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:32:05 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.096846, mean: 0.097618, max: 0.109657
20:32:05 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:32:05 DEBUG opendrift.models.physics_methods:1061: min: 1.695391, mean: 1.702110, max: 1.804044
20:32:05 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.695391, mean: 1.702110, max: 1.804044
20:32:05 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:32:05 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:32:05 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:32:05 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:32:05 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.039683 m/s - 0.042226 m/s)
20:32:05 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:32:05 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:32:05 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:32:05 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:32:05 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:32:05 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:32:05 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:32:05 INFO opendrift.models.basemodel:2035: 2024-12-11 20:00:00 - step 69 of 71 - 1000 active elements (0 deactivated)
20:32:05 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:32:05 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:32:05 DEBUG opendrift.models.basemodel:2054: 60.202227475328115 <- latitude -> 60.31220560308148
20:32:05 DEBUG opendrift.models.basemodel:2059: 3.8764806794933135 <- longitude -> 4.006969608172233
20:32:05 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:32:05 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:32:05 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:32:05 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:32:05 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:32:05 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:32:05 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:32:05 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:32:05 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:32:05 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:32:05 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:32:05 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:32:05 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:32:05 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:32:05 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:32:05 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:32:05 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:32:05 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:32:05 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:32:05 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:32:05 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-11 20:00:00 (before)
2024-12-11 21:00:00 (after)
20:32:06 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:32:06 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:32:06 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:32:06 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:32:06 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:32:06 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 33x36x2) for time before (2024-12-11 20:00:00)
20:32:06 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-11 20:00:00) in space (linearNDFast)
20:32:06 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:32:06 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:32:06 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.12353019346045 and -65.99304128400004 degrees.
20:32:06 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.12353019346045 and -65.99304128400004 degrees.
20:32:06 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:32:06 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:32:06 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:32:06 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:32:06 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:32:06 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:32:06 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0846201 (min) 0.198663 (max)
20:32:06 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.459784 (min) -0.0300777 (max)
20:32:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.036585 (min) -0.0122727 (max)
20:32:06 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0.608537 (min) 1.12288 (max)
20:32:06 DEBUG opendrift.models.basemodel.environment:905: y_wind: -1.69711 (min) -1.62781 (max)
20:32:06 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -4.41572e-05 (min) -4.21126e-05 (max)
20:32:06 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:32:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:32:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:32:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:32:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:32:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:32:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:32:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:32:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:32:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:32:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:32:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:32:06 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:32:06 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:32:06 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:32:06 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:32:06 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:32:06 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 296.999 (min) 299.228 (max)
20:32:06 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:32:06 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:32:06 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.078712, mean: 0.080452, max: 0.096201
20:32:06 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:32:06 DEBUG opendrift.models.physics_methods:1061: min: 1.528447, mean: 1.545186, max: 1.689742
20:32:06 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.528447, mean: 1.545186, max: 1.689742
20:32:06 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:32:06 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:32:06 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:32:06 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:32:06 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.035775 m/s - 0.039551 m/s)
20:32:06 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:32:06 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:32:06 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:32:06 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:32:06 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:32:06 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:32:06 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:32:06 INFO opendrift.models.basemodel:2035: 2024-12-11 21:00:00 - step 70 of 71 - 1000 active elements (0 deactivated)
20:32:06 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:32:06 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:32:06 DEBUG opendrift.models.basemodel:2054: 60.18629226492533 <- latitude -> 60.31018172453916
20:32:06 DEBUG opendrift.models.basemodel:2059: 3.8724320614001315 <- longitude -> 4.020790528219189
20:32:06 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:32:06 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:32:06 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:32:06 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:32:06 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:32:06 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:32:06 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:32:06 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:32:06 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:32:06 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:32:06 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:32:06 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:32:06 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:32:06 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:32:06 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:32:06 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:32:06 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:32:06 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:32:06 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:32:06 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:32:06 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-11 21:00:00 (before)
2024-12-11 22:00:00 (after)
20:32:07 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:32:07 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:32:07 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:32:07 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:32:07 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:32:07 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 33x39x2) for time before (2024-12-11 21:00:00)
20:32:07 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-11 21:00:00) in space (linearNDFast)
20:32:07 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:32:07 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:32:07 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.12757880952803 and -65.9792203731031 degrees.
20:32:07 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.12757880952803 and -65.9792203731031 degrees.
20:32:07 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:32:07 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:32:07 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:32:07 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:32:07 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:32:07 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:32:07 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.150525 (min) 0.198225 (max)
20:32:07 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.426162 (min) -0.0584945 (max)
20:32:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.23464 (min) -0.218406 (max)
20:32:07 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0.632522 (min) 1.37404 (max)
20:32:07 DEBUG opendrift.models.basemodel.environment:905: y_wind: -1.56719 (min) -1.4986 (max)
20:32:07 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -6.79346e-05 (min) -6.51048e-05 (max)
20:32:07 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:32:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:32:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:32:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:32:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:32:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:32:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:32:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:32:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:32:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:32:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:32:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:32:07 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:32:07 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:32:07 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:32:07 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:32:07 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:32:07 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 296.811 (min) 299.264 (max)
20:32:07 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:32:07 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:32:07 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.068414, mean: 0.071432, max: 0.106864
20:32:07 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:32:07 DEBUG opendrift.models.physics_methods:1061: min: 1.424956, mean: 1.455850, max: 1.780923
20:32:07 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.424956, mean: 1.455850, max: 1.780923
20:32:07 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:32:07 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:32:07 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:32:07 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:32:07 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.033353 m/s - 0.041685 m/s)
20:32:07 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:32:07 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:32:07 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:32:07 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:32:07 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:32:07 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:32:07 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:32:07 INFO opendrift.models.basemodel:2035: 2024-12-11 22:00:00 - step 71 of 71 - 1000 active elements (0 deactivated)
20:32:07 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:32:07 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:32:07 DEBUG opendrift.models.basemodel:2054: 60.171530580004514 <- latitude -> 60.30727859284619
20:32:07 DEBUG opendrift.models.basemodel:2059: 3.864418990730272 <- longitude -> 4.034529227412516
20:32:07 DEBUG opendrift.models.basemodel:2062: z = 0.0
20:32:07 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:32:07 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:32:07 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:32:07 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:32:07 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:32:07 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:32:07 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:32:07 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:32:07 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:32:07 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:32:07 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:32:07 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:32:07 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:32:07 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
20:32:07 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:32:07 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
20:32:07 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:32:07 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:32:07 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
20:32:07 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-12-11 22:00:00 (before)
2024-12-11 23:00:00 (after)
20:32:08 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
20:32:08 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:32:08 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:32:08 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:32:08 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:32:08 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 35x40x2) for time before (2024-12-11 22:00:00)
20:32:08 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-12-11 22:00:00) in space (linearNDFast)
20:32:08 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
20:32:08 DEBUG opendrift.readers.basereader.structured:388: No time interpolation needed - right on time.
20:32:08 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.13559188896082 and -65.96548168332149 degrees.
20:32:08 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -66.13559188896082 and -65.96548168332149 degrees.
20:32:08 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:32:08 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:32:08 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:32:08 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
20:32:08 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
20:32:08 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:32:08 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.26267 (min) 0.184164 (max)
20:32:08 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.378489 (min) -0.0252513 (max)
20:32:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.47847 (min) -0.467331 (max)
20:32:08 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.62688 (min) 2.28848 (max)
20:32:08 DEBUG opendrift.models.basemodel.environment:905: y_wind: -1.12613 (min) -0.981435 (max)
20:32:08 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -7.10231e-05 (min) -6.6678e-05 (max)
20:32:08 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
20:32:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:32:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:32:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:32:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:32:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:32:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
20:32:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
20:32:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
20:32:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
20:32:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
20:32:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
20:32:08 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
20:32:08 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
20:32:08 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
20:32:08 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
20:32:08 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:32:08 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 296.738 (min) 299.34 (max)
20:32:08 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:32:08 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:32:08 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.092461, mean: 0.105238, max: 0.160031
20:32:08 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:32:08 DEBUG opendrift.models.physics_methods:1061: min: 1.656566, mean: 1.766517, max: 2.179375
20:32:08 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.656566, mean: 1.766517, max: 2.179375
20:32:08 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:32:08 DEBUG opendrift.models.basemodel:749: No elements hit seafloor.
20:32:08 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:32:08 DEBUG opendrift.models.basemodel:2108: Calling OceanDrift.update()
20:32:08 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.038774 m/s - 0.051011 m/s)
20:32:08 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:32:08 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
20:32:08 DEBUG opendrift.models.basemodel:1660: Horizontal diffusivity is 0, no random walk.
20:32:08 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:32:08 DEBUG opendrift.models.basemodel:2152: Cleaning up
20:32:08 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:32:08 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:32:08 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:32:08 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:32:08 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:32:08 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:32:08 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:32:08 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:32:08 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:32:08 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:32:08 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:32:08 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:32:08 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:32:08 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:32:08 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:32:08 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:32:08 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:32:08 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:32:08 DEBUG opendrift.models.basemodel:95: Changed mode from Mode.Run to Mode.Result
20:32:08 DEBUG opendrift.models.basemodel:2364: Setting up map: corners=None, fast=False, lscale=None
20:32:08 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
/opt/conda/envs/opendrift/lib/python3.11/site-packages/cartopy/mpl/geoaxes.py:1692: UserWarning: No data for colormapping provided via 'c'. Parameters 'cmap' will be ignored
result = super().scatter(*args, **kwargs)
20:32:21 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:32:22 DEBUG opendrift.models.basemodel:3044: Saving animation..
20:32:22 INFO opendrift.models.basemodel:4613: Saving animation to /root/project/docs/source/gallery/animations/example_manual_aggregate_0.gif...
20:32:23 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:32:24 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:32:25 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:32:27 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:32:28 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:32:30 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:32:31 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:32:33 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:32:34 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:32:36 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:32:37 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:32:39 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:32:40 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:32:41 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:32:43 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:32:44 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:32:46 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:32:47 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:32:49 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:32:50 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:32:52 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:32:53 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:32:55 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:32:56 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:32:58 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:32:59 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:33:01 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:33:02 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:33:04 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:33:05 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:33:07 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:33:08 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:33:10 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:33:11 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:33:12 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:33:14 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:33:15 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:33:17 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:33:18 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:33:20 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:33:21 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:33:22 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:33:24 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:33:25 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:33:27 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:33:28 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:33:30 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:33:31 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:33:33 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:33:34 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:33:36 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:33:37 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:33:39 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:33:40 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:33:42 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:33:43 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:33:45 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:33:46 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:33:48 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:33:49 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:33:51 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:33:53 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:33:54 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:33:56 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:33:57 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:33:59 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:34:00 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:34:02 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:34:04 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:34:05 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:34:07 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:34:09 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:34:10 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:34:12 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:34:13 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:34:14 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:34:16 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:34:17 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:34:19 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:34:20 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:34:22 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:34:23 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:34:25 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:34:26 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:34:28 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:34:29 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:34:31 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:34:32 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:34:34 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:34:35 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:34:36 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:34:38 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:34:39 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:34:41 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:34:42 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:34:44 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:34:45 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:34:47 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:34:48 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:34:50 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:34:51 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:34:53 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:34:54 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:34:55 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:34:57 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:34:58 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:35:00 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:35:01 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:35:03 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:35:04 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:35:06 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:35:07 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:35:09 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:35:10 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:35:11 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:35:13 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:35:14 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:35:16 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:35:17 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:35:19 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:35:20 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:35:22 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:35:23 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:35:25 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:35:26 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:35:28 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:35:30 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:35:31 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:35:33 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:35:34 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:35:36 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:35:37 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:35:39 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:35:40 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:35:42 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:35:43 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:35:45 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:35:46 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:35:48 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:35:50 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:35:51 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:35:53 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:35:55 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:35:56 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (3.450294589996338, 4.965985679626466, 59.77430038452148, 60.55330581665038)..
20:36:00 DEBUG opendrift.models.basemodel:4651: MPLBACKEND = agg
20:36:00 DEBUG opendrift.models.basemodel:4652: DISPLAY = None
20:36:00 DEBUG opendrift.models.basemodel:4653: Time to save animation: 0:03:37.432515
20:36:00 INFO opendrift.models.basemodel:3037: Time to make animation: 0:03:51.702304
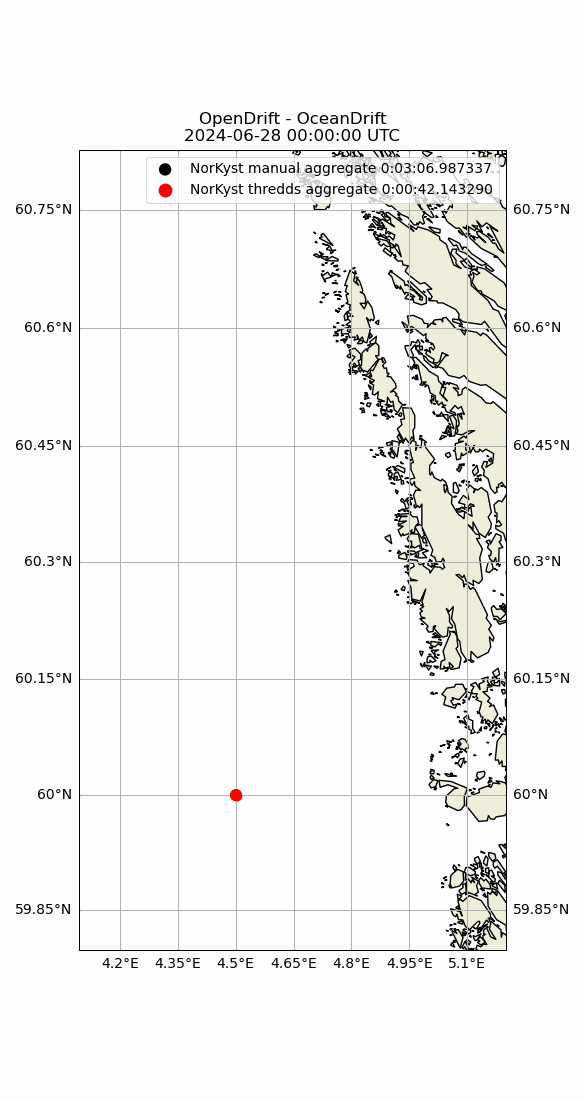
Total running time of the script: (24 minutes 54.462 seconds)