Note
Go to the end to download the full example code.
Biodegradation of oil at depth
import numpy as np
from datetime import datetime, timedelta
from opendrift.readers import reader_netCDF_CF_generic
from opendrift.models.openoil import OpenOil
o = OpenOil(loglevel=0) # Set loglevel to 0 for debug information
time = datetime.now()
12:51:18 DEBUG opendrift.config:168: Adding 18 config items from __init__
12:51:18 DEBUG opendrift.config:178: Overwriting config item readers:max_number_of_fails
12:51:18 DEBUG opendrift.config:168: Adding 6 config items from __init__
12:51:18 INFO opendrift.models.basemodel:515: OpenDriftSimulation initialised (version 1.11.13 / v1.11.13-99-gd2132d3)
12:51:18 DEBUG opendrift.config:168: Adding 15 config items from oceandrift
12:51:18 DEBUG opendrift.config:178: Overwriting config item seed:z
12:51:18 DEBUG opendrift.config:168: Adding 15 config items from openoil
Current from HYCOM and wind from NCEP GFS
o.add_readers_from_list([
'https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd',
'https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd'])
o.set_config('environment:constant:ocean_mixed_layer_thickness', 20)
o.set_config('drift', {'current_uncertainty': 0, 'wind_uncertainty': 0, 'horizontal_diffusivity': 20})
12:51:18 DEBUG opendrift.readers.reader_lazy:38: Delaying initialisation of LazyReader: https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:51:18 DEBUG opendrift.readers.reader_lazy:38: Delaying initialisation of LazyReader: https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:51:18 DEBUG opendrift.models.basemodel.environment:328: Added reader LazyReader: https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:51:18 DEBUG opendrift.models.basemodel.environment:328: Added reader LazyReader: https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:51:18 INFO opendrift.config:68: set_config('drift:current_uncertainty', 0)
12:51:18 INFO opendrift.config:68: set_config('drift:wind_uncertainty', 0)
12:51:18 INFO opendrift.config:68: set_config('drift:horizontal_diffusivity', 20)
Configuration
o.set_config('drift:vertical_mixing', True)
o.set_config('drift:vertical_mixing', True)
o.set_config('processes:biodegradation', True)
o.set_config('processes:dispersion', False)
o.set_config('biodegradation:method', 'half_time')
Fast decay for droplets, and slow decay for slick
kwargs = {'biodegradation_half_time_slick': 3, # days
'biodegradation_half_time_droplet': 1, # days
'oil_type': 'GENERIC MEDIUM CRUDE', 'm3_per_hour': .5, 'diameter': 8e-5} # small droplets
Seed oil at surface and at 150m depth
time = datetime.today() - timedelta(days=5)
lon = 23.5
lat = 35.0
o.seed_elements(lon=lon, lat=lat, z=0, radius=100, number=3000, time=time, **kwargs)
o.seed_elements(lon=lon, lat=lat, z=-150, radius=100, number=3000, time=time, **kwargs)
12:51:18 INFO opendrift.models.openoil.openoil:1638: Droplet diameter is provided, and will be kept constant during simulation
12:51:18 INFO opendrift.models.openoil.adios.dirjs:90: Querying ADIOS database for oil: GENERIC MEDIUM CRUDE
12:51:18 DEBUG opendrift.models.openoil.adios.oil:76: Parsing Oil: AD04001 / GENERIC MEDIUM CRUDE
12:51:18 INFO opendrift.models.openoil.openoil:1719: Using density 880.4 and viscosity 2.230881193744365e-05 of oiltype GENERIC MEDIUM CRUDE
12:51:18 DEBUG opendrift.readers.basereader:186: Variable mapping: ['sea_floor_depth_below_sea_level'] -> ['land_binary_mask'] is not activated
12:51:18 DEBUG opendrift.models.basemodel.environment:328: Added reader constant_reader
12:51:18 INFO opendrift.models.basemodel.environment:218: Adding a dynamical landmask with max. priority based on assumed maximum speed of 1.3 m/s. Adding a customised landmask may be faster...
12:51:18 DEBUG opendrift.readers.basereader:186: Variable mapping: ['sea_floor_depth_below_sea_level'] -> ['land_binary_mask'] is not activated
12:51:23 DEBUG opendrift.models.basemodel.environment:328: Added reader global_landmask
12:51:23 INFO opendrift.models.basemodel.environment:245: Fallback values will be used for the following variables which have no readers:
12:51:23 INFO opendrift.models.basemodel.environment:248: sea_surface_height: 0.000000
12:51:23 INFO opendrift.models.basemodel.environment:248: upward_sea_water_velocity: 0.000000
12:51:23 INFO opendrift.models.basemodel.environment:248: sea_surface_wave_significant_height: 0.000000
12:51:23 INFO opendrift.models.basemodel.environment:248: sea_surface_wave_stokes_drift_x_velocity: 0.000000
12:51:23 INFO opendrift.models.basemodel.environment:248: sea_surface_wave_stokes_drift_y_velocity: 0.000000
12:51:23 INFO opendrift.models.basemodel.environment:248: sea_surface_wave_period_at_variance_spectral_density_maximum: 0.000000
12:51:23 INFO opendrift.models.basemodel.environment:248: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0.000000
12:51:23 INFO opendrift.models.basemodel.environment:248: sea_ice_area_fraction: 0.000000
12:51:23 INFO opendrift.models.basemodel.environment:248: sea_ice_x_velocity: 0.000000
12:51:23 INFO opendrift.models.basemodel.environment:248: sea_ice_y_velocity: 0.000000
12:51:23 INFO opendrift.models.basemodel.environment:248: sea_water_temperature: 10.000000
12:51:23 INFO opendrift.models.basemodel.environment:248: sea_water_salinity: 34.000000
12:51:23 INFO opendrift.models.basemodel.environment:248: sea_floor_depth_below_sea_level: 10000.000000
12:51:23 INFO opendrift.models.basemodel.environment:248: ocean_vertical_diffusivity: 0.020000
12:51:23 DEBUG opendrift.models.basemodel:95: Changed mode from Mode.Config to Mode.Ready
12:51:23 INFO opendrift.models.openoil.openoil:1638: Droplet diameter is provided, and will be kept constant during simulation
12:51:23 INFO opendrift.models.openoil.adios.dirjs:90: Querying ADIOS database for oil: GENERIC MEDIUM CRUDE
12:51:23 DEBUG opendrift.models.openoil.adios.oil:76: Parsing Oil: AD04001 / GENERIC MEDIUM CRUDE
12:51:23 INFO opendrift.models.openoil.openoil:1719: Using density 880.4 and viscosity 2.230881193744365e-05 of oiltype GENERIC MEDIUM CRUDE
Running model
o.run(duration=timedelta(days=5), time_step=3600, outfile='oil.nc')
12:51:23 DEBUG opendrift.models.basemodel:95: Changed mode from Mode.Ready to Mode.Run
12:51:25 DEBUG opendrift.models.basemodel:1778:
------------------------------------------------------
Software and hardware:
OpenDrift version 1.11.13
Platform: Linux, 5.15.0-1057-aws
68.56775665283203 GB memory
36 processors (x86_64)
NumPy version 1.26.4
SciPy version 1.14.1
Matplotlib version 3.9.1
NetCDF4 version 1.6.1
Xarray version 2024.10.0
ADIOS (adios_db) version 1.1.1
Copernicusmarine version 1.3.4
Python version 3.11.6 | packaged by conda-forge | (main, Oct 3 2023, 10:40:35) [GCC 12.3.0]
------------------------------------------------------
12:51:25 DEBUG opendrift.models.basemodel:1910: Finalizing environment and preparing readers for simulation coverage ([17.320116865357402, 29.937168348157726, 29.680066240111348, 40.06288505760399]) and time (2024-11-06 12:51:18.915878 to 2024-11-11 12:51:18.915878)
12:51:25 DEBUG opendrift.models.basemodel.environment:180: Preparing LazyReader: https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd for extent [17.320116865357402, 29.937168348157726, 29.680066240111348, 40.06288505760399]
12:51:25 DEBUG opendrift.models.basemodel.environment:180: Preparing LazyReader: https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd for extent [17.320116865357402, 29.937168348157726, 29.680066240111348, 40.06288505760399]
12:51:25 DEBUG opendrift.models.basemodel.environment:180: Preparing constant_reader for extent [17.320116865357402, 29.937168348157726, 29.680066240111348, 40.06288505760399]
12:51:25 DEBUG opendrift.readers.basereader.variables:549: Nothing more to prepare for constant_reader
12:51:25 DEBUG opendrift.models.basemodel.environment:180: Preparing global_landmask for extent [17.320116865357402, 29.937168348157726, 29.680066240111348, 40.06288505760399]
12:51:25 DEBUG opendrift.readers.basereader.variables:549: Nothing more to prepare for global_landmask
12:51:25 INFO opendrift.models.basemodel:936: Using existing reader for land_binary_mask
12:51:25 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:51:25 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:51:25 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:51:25 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:51:25 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:51:25 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:51:25 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:51:25 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:51:25 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:51:25 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:51:25 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:51:25 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:51:25 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:51:25 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:51:25 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:51:25 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:51:25 INFO opendrift.models.basemodel:947: All points are in ocean
12:51:25 INFO opendrift.models.openoil.openoil:687: Oil-water surface tension is 0.031402 Nm
12:51:25 INFO opendrift.models.openoil.openoil:700: Max water fraction not available for GENERIC MEDIUM CRUDE, using default
12:51:25 DEBUG opendrift.models.basemodel:891: to be seeded: 6000, already seeded 0
12:51:25 DEBUG opendrift.models.basemodel:909: Released 6000 new elements.
12:51:25 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:51:25 INFO opendrift.models.basemodel:2038: 2024-11-06 12:51:18.915878 - step 1 of 120 - 6000 active elements (0 deactivated)
12:51:25 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:51:25 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:51:25 DEBUG opendrift.models.basemodel:2057: 34.996628 <- latitude -> 35.003426
12:51:25 DEBUG opendrift.models.basemodel:2062: 23.496576 <- longitude -> 23.503607
12:51:25 DEBUG opendrift.models.basemodel:2067: -150.0 <- z -> 0.0
12:51:25 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:51:25 DEBUG opendrift.models.basemodel.environment:581: Variables not covered by any reader: ['sea_water_temperature', 'y_sea_water_velocity', 'x_wind', 'sea_water_salinity', 'sea_surface_height', 'sea_floor_depth_below_sea_level', 'y_wind', 'x_sea_water_velocity']
12:51:25 DEBUG opendrift.readers.reader_lazy:57: Initialising: LazyReader: https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:51:25 DEBUG opendrift.readers:84: Testing reader <module 'opendrift.readers.reader_netCDF_CF_generic' from '/root/project/opendrift/readers/reader_netCDF_CF_generic.py'>
12:51:25 INFO opendrift.readers.reader_netCDF_CF_generic:102: Opening dataset: https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:51:26 DEBUG opendrift.readers.reader_netCDF_CF_generic:119: Finding coordinate variables.
12:51:26 INFO opendrift.readers.reader_netCDF_CF_generic:292: Grid coordinates are detected, but proj4 string not given: assuming latlong
12:51:26 INFO opendrift.readers.reader_netCDF_CF_generic:325: Detected dimensions: {'z': 'depth', 'y': 'lat', 'x': 'lon', 'time': 'time'}
12:51:26 DEBUG opendrift.readers.basereader.variables:608: Setting buffer size 9 for reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd, assuming a maximum average speed of 5 m/s and time span of 3:00:00
12:51:26 INFO opendrift.readers.basereader:176: Variable y_sea_water_velocity will be rotated from northward_sea_water_velocity
12:51:26 INFO opendrift.readers.basereader:176: Variable x_sea_water_velocity will be rotated from eastward_sea_water_velocity
12:51:26 DEBUG opendrift.readers.basereader:186: Variable mapping: ['sea_floor_depth_below_sea_level'] -> ['land_binary_mask'] is not activated
12:51:26 DEBUG opendrift.readers.basereader.variables:563: Adding variable mapping: ['x_sea_water_velocity', 'y_sea_water_velocity'] -> sea_water_speed
12:51:26 DEBUG opendrift.readers.basereader.structured:153: Clearing cache for reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd before starting new simulation
12:51:26 DEBUG opendrift.readers.basereader.variables:608: Setting buffer size 4 for reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd, assuming a maximum average speed of 1.3 m/s and time span of 3:00:00
12:51:26 DEBUG opendrift.readers.basereader.variables:549: Nothing more to prepare for https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:51:26 DEBUG opendrift.readers.reader_lazy:72: Reader initialised: https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:51:26 DEBUG opendrift.readers.basereader.variables:608: Setting buffer size 4 for reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd, assuming a maximum average speed of 1.3 m/s and time span of 3:00:00
12:51:26 DEBUG opendrift.readers.reader_lazy:57: Initialising: LazyReader: https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:51:26 DEBUG opendrift.readers:84: Testing reader <module 'opendrift.readers.reader_netCDF_CF_generic' from '/root/project/opendrift/readers/reader_netCDF_CF_generic.py'>
12:51:26 INFO opendrift.readers.reader_netCDF_CF_generic:102: Opening dataset: https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:51:27 DEBUG opendrift.readers.reader_netCDF_CF_generic:119: Finding coordinate variables.
12:51:27 DEBUG opendrift.readers.reader_netCDF_CF_generic:255: Lon and lat are 1D arrays - using as projection coordinates
12:51:27 INFO opendrift.readers.reader_netCDF_CF_generic:325: Detected dimensions: {'time': 'time', 'x': 'longitude', 'y': 'latitude'}
12:51:27 DEBUG opendrift.readers.basereader.variables:608: Setting buffer size 944747 for reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd, assuming a maximum average speed of 5 m/s and time span of 121373 days, 9:44:45.959999
12:51:27 INFO opendrift.readers.basereader:176: Variable x_wind will be rotated from eastward_wind
12:51:27 INFO opendrift.readers.basereader:176: Variable y_wind will be rotated from northward_wind
12:51:27 DEBUG opendrift.readers.basereader:186: Variable mapping: ['sea_floor_depth_below_sea_level'] -> ['land_binary_mask'] is not activated
12:51:27 DEBUG opendrift.readers.basereader.variables:563: Adding variable mapping: ['x_wind', 'y_wind'] -> wind_speed
12:51:27 DEBUG opendrift.readers.basereader.structured:153: Clearing cache for reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd before starting new simulation
12:51:27 DEBUG opendrift.readers.basereader.variables:608: Setting buffer size 245636 for reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd, assuming a maximum average speed of 1.3 m/s and time span of 121373 days, 9:44:45.959999
12:51:27 DEBUG opendrift.readers.basereader.variables:549: Nothing more to prepare for https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:51:27 DEBUG opendrift.readers.reader_lazy:72: Reader initialised: https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:51:27 DEBUG opendrift.readers.basereader.variables:608: Setting buffer size 245636 for reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd, assuming a maximum average speed of 1.3 m/s and time span of 121373 days, 9:44:45.959999
12:51:27 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:51:27 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:51:27 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:51:27 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:51:27 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:51:27 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:51:27 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:51:27 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:51:27 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:51:27 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:51:27 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:51:27 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:51:27 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:51:27 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:51:27 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:51:27 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:51:27 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:51:27 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:51:27 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:51:27 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:51:27 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:51:27 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:51:27 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:51:27 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:51:27 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:51:27 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:51:27 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:51:27 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:51:27 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:51:27 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-06 12:00:00 (before)
2024-11-06 15:00:00 (after)
12:51:27 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
12:51:27 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
12:51:28 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:51:28 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:51:28 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:51:28 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 8x9x23) for time before (2024-11-06 12:00:00)
12:51:28 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
12:51:28 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
12:51:28 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:51:28 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:51:28 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:51:28 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 8x9x23) for time after (2024-11-06 15:00:00)
12:51:28 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-06 12:00:00) in space (linearNDFast)
12:51:28 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:51:28 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-06 15:00:00) in space (linearNDFast)
12:51:28 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:51:28 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-06 12:00:00, weight 0.71) and
after (2024-11-06 15:00:00, weight 0.29) in time
12:51:28 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:51:28 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:51:28 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:51:28 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:51:28 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:51:28 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:51:28 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:51:28 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:51:28 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:51:28 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:51:28 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-06 12:00:00 (before)
2024-11-06 15:00:00 (after)
12:51:28 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_wind to retrieve x_wind
12:51:31 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_wind to retrieve y_wind
12:51:35 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:51:35 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:51:35 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:51:35 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 720x361x1) for time before (2024-11-06 12:00:00)
12:51:35 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_wind to retrieve x_wind
12:51:38 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_wind to retrieve y_wind
12:51:40 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:51:40 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:51:40 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:51:40 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 720x361x1) for time after (2024-11-06 15:00:00)
12:51:40 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-06 12:00:00) in space (linearNDFast)
12:51:40 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:51:40 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-06 15:00:00) in space (linearNDFast)
12:51:40 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:51:40 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-06 12:00:00, weight 0.71) and
after (2024-11-06 15:00:00, weight 0.29) in time
12:51:40 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:51:40 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:51:40 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:51:40 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:51:40 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:51:40 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:51:40 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:51:40 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:51:40 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:51:40 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.101809 (min) 0.179826 (max)
12:51:40 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0177597 (min) 0.0296777 (max)
12:51:40 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0.675366 (min) 0.762602 (max)
12:51:40 DEBUG opendrift.models.basemodel.environment:905: y_wind: -0.179573 (min) -0.0825003 (max)
12:51:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:51:40 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:51:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:51:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:51:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:51:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:51:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:51:40 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:51:40 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:51:40 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:51:40 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:51:40 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:51:40 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:51:40 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:51:40 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:51:40 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:51:40 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:51:40 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.012014, mean: 0.013947, max: 0.014515
12:51:40 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:51:40 DEBUG opendrift.models.physics_methods:1061: min: 0.597131, mean: 0.643307, max: 0.656352
12:51:40 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 0.597131, mean: 0.643307, max: 0.656352
12:51:40 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:51:40 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:51:40 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:51:40 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:51:40 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:51:40 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:51:40 DEBUG opendrift.models.openoil.openoil:861: Emulsification not yet started
12:51:40 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:51:40 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:51:40 DEBUG opendrift.models.physics_methods:1061: min: 0.597131, mean: 0.643307, max: 0.656352
12:51:40 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:51:40 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.012945, dN_50: 0.001016
12:51:40 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:51:40 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.0002170939324000877
12:51:40 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:51:40 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:51:40 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:51:41 DEBUG opendrift.models.physics_methods:915: Advecting 3000 of 6000 elements above 0.100m with wind-sheared ocean current (0.020965 m/s - 0.023040 m/s)
12:51:41 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:51:41 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0022460362237508094 and 0.48135814700051643 m/s
12:51:41 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:51:41 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:51:41 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:51:41 INFO opendrift.models.basemodel:2038: 2024-11-06 13:51:18.915878 - step 2 of 120 - 6000 active elements (0 deactivated)
12:51:41 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:51:41 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:51:41 DEBUG opendrift.models.basemodel:2057: 34.986616852648 <- latitude -> 35.01379331748806
12:51:41 DEBUG opendrift.models.basemodel:2062: 23.488690312448988 <- longitude -> 23.52525935122943
12:51:41 DEBUG opendrift.models.basemodel:2067: -150.17516672978425 <- z -> 0.0
12:51:41 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:51:41 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:51:41 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:51:41 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:51:41 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:51:41 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:51:41 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:51:41 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:51:41 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:51:41 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:51:41 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:51:41 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:51:41 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:51:41 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:51:41 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:51:41 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:51:41 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:51:41 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:51:41 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:51:41 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:51:41 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:51:41 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:51:41 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:51:41 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:51:41 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:51:41 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:51:41 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:51:41 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:51:41 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:51:41 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:51:41 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-06 12:00:00 (before)
2024-11-06 15:00:00 (after)
12:51:41 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-06 12:00:00) in space (linearNDFast)
12:51:41 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:51:41 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-06 15:00:00) in space (linearNDFast)
12:51:41 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:51:41 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-06 12:00:00, weight 0.38) and
after (2024-11-06 15:00:00, weight 0.62) in time
12:51:41 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:51:41 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:51:41 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:51:41 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:51:41 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:51:41 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:51:41 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:51:41 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:51:41 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:51:41 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:51:41 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-06 12:00:00 (before)
2024-11-06 15:00:00 (after)
12:51:41 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-06 12:00:00) in space (linearNDFast)
12:51:41 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:51:41 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-06 15:00:00) in space (linearNDFast)
12:51:41 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:51:41 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-06 12:00:00, weight 0.38) and
after (2024-11-06 15:00:00, weight 0.62) in time
12:51:41 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:51:41 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:51:41 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:51:41 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:51:41 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:51:41 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:51:41 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:51:41 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:51:41 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:51:41 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0920644 (min) 0.213289 (max)
12:51:41 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0342784 (min) 0.0286333 (max)
12:51:41 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.0244133 (min) 0.258684 (max)
12:51:41 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0.414957 (min) 0.753428 (max)
12:51:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:51:41 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:51:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:51:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:51:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:51:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:51:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:51:41 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:51:41 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:51:41 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:51:41 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:51:41 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:51:41 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:51:41 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:51:41 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:51:41 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:51:41 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:51:41 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.004251, mean: 0.012768, max: 0.015323
12:51:41 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:51:41 DEBUG opendrift.models.physics_methods:1061: min: 0.355182, mean: 0.613948, max: 0.674378
12:51:41 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 0.355182, mean: 0.613948, max: 0.674378
12:51:41 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:51:41 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:51:41 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:51:41 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:51:41 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:51:41 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:51:41 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:51:41 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:51:41 DEBUG opendrift.models.physics_methods:1061: min: 0.355182, mean: 0.613948, max: 0.674378
12:51:41 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:51:41 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.013818, dN_50: 0.001084
12:51:41 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:51:41 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.00022894856424003558
12:51:41 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:51:41 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:51:41 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:51:41 DEBUG opendrift.models.physics_methods:915: Advecting 3000 of 6000 elements above 0.100m with wind-sheared ocean current (0.013462 m/s - 0.023677 m/s)
12:51:41 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:51:41 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0006187029221147531 and 0.45730975932548934 m/s
12:51:41 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:51:41 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:51:41 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:51:41 INFO opendrift.models.basemodel:2038: 2024-11-06 14:51:18.915878 - step 3 of 120 - 6000 active elements (0 deactivated)
12:51:41 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:51:41 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:51:41 DEBUG opendrift.models.basemodel:2057: 34.984246794370925 <- latitude -> 35.01811909210327
12:51:41 DEBUG opendrift.models.basemodel:2062: 23.487771054234514 <- longitude -> 23.541366920477405
12:51:41 DEBUG opendrift.models.basemodel:2067: -149.71929421800513 <- z -> 0.0
12:51:41 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:51:41 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:51:41 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:51:41 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:51:41 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:51:41 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:51:41 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:51:41 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:51:41 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:51:41 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:51:41 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:51:41 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:51:41 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:51:41 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:51:41 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:51:41 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:51:41 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:51:41 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:51:41 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:51:41 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:51:41 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:51:41 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:51:41 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:51:41 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:51:41 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:51:41 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:51:41 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:51:41 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:51:41 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:51:41 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:51:41 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-06 12:00:00 (before)
2024-11-06 15:00:00 (after)
12:51:41 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-06 12:00:00) in space (linearNDFast)
12:51:41 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:51:41 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-06 15:00:00) in space (linearNDFast)
12:51:41 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:51:41 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-06 12:00:00, weight 0.05) and
after (2024-11-06 15:00:00, weight 0.95) in time
12:51:41 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:51:41 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:51:41 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:51:41 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:51:41 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:51:41 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:51:41 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:51:41 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:51:41 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:51:41 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:51:41 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-06 12:00:00 (before)
2024-11-06 15:00:00 (after)
12:51:41 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-06 12:00:00) in space (linearNDFast)
12:51:41 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:51:41 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-06 15:00:00) in space (linearNDFast)
12:51:41 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:51:41 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-06 12:00:00, weight 0.05) and
after (2024-11-06 15:00:00, weight 0.95) in time
12:51:41 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:51:41 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:51:41 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:51:41 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:51:41 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:51:41 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:51:41 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:51:41 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:51:41 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:51:41 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0888873 (min) 0.236102 (max)
12:51:41 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0628417 (min) 0.0270669 (max)
12:51:41 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.626366 (min) -0.24465 (max)
12:51:41 DEBUG opendrift.models.basemodel.environment:905: y_wind: 1.10588 (min) 1.5669 (max)
12:51:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:51:41 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:51:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:51:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:51:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:51:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:51:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:51:41 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:51:41 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:51:41 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:51:41 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:51:41 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:51:41 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:51:41 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:51:41 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:51:41 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:51:41 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:51:41 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.039721, mean: 0.055148, max: 0.061957
12:51:41 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:51:41 DEBUG opendrift.models.physics_methods:1061: min: 1.085771, mean: 1.278659, max: 1.356045
12:51:41 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.085771, mean: 1.278659, max: 1.356045
12:51:41 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:51:41 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:51:41 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:51:41 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:51:41 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:51:41 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:51:41 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:51:41 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:51:41 DEBUG opendrift.models.physics_methods:1061: min: 1.085771, mean: 1.278659, max: 1.356045
12:51:41 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:51:41 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.005747, dN_50: 0.000451
12:51:41 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:51:41 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.0009129370730841468
12:51:41 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:51:41 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:51:41 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:51:41 DEBUG opendrift.models.physics_methods:915: Advecting 3000 of 6000 elements above 0.100m with wind-sheared ocean current (0.038708 m/s - 0.047551 m/s)
12:51:41 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:51:41 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0017541404241848248 and 0.49065842250043995 m/s
12:51:41 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:51:41 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:51:41 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:51:41 INFO opendrift.models.basemodel:2038: 2024-11-06 15:51:18.915878 - step 4 of 120 - 6000 active elements (0 deactivated)
12:51:41 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:51:41 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:51:41 DEBUG opendrift.models.basemodel:2057: 34.97860097393403 <- latitude -> 35.02679017720848
12:51:41 DEBUG opendrift.models.basemodel:2062: 23.487119340243016 <- longitude -> 23.55154619812824
12:51:41 DEBUG opendrift.models.basemodel:2067: -149.09442657248135 <- z -> 0.0
12:51:41 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:51:41 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:51:41 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:51:41 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:51:41 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:51:41 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:51:41 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:51:41 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:51:41 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:51:41 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:51:41 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:51:41 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:51:41 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:51:41 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:51:41 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:51:41 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:51:41 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:51:41 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:51:41 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:51:41 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:51:41 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:51:41 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:51:41 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:51:41 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:51:41 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:51:41 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:51:41 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:51:41 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:51:41 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:51:41 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:51:41 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-06 15:00:00 (before)
2024-11-06 18:00:00 (after)
12:51:41 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
12:51:41 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
12:51:42 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:51:42 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:51:42 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:51:42 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 9x9x23) for time after (2024-11-06 18:00:00)
12:51:42 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-06 15:00:00) in space (linearNDFast)
12:51:42 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:51:42 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-06 18:00:00) in space (linearNDFast)
12:51:42 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:51:42 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-06 15:00:00, weight 0.71) and
after (2024-11-06 18:00:00, weight 0.29) in time
12:51:42 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:51:42 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:51:42 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:51:42 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:51:42 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:51:42 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:51:42 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:51:42 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:51:42 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:51:42 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:51:42 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-06 15:00:00 (before)
2024-11-06 18:00:00 (after)
12:51:42 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_wind to retrieve x_wind
12:51:45 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_wind to retrieve y_wind
12:51:48 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:51:48 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:51:48 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:51:48 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 720x361x1) for time after (2024-11-06 18:00:00)
12:51:48 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-06 15:00:00) in space (linearNDFast)
12:51:48 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:51:48 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-06 18:00:00) in space (linearNDFast)
12:51:48 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:51:48 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-06 15:00:00, weight 0.71) and
after (2024-11-06 18:00:00, weight 0.29) in time
12:51:48 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:51:48 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:51:48 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:51:48 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:51:48 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:51:48 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:51:48 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:51:48 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:51:48 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:51:48 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0727223 (min) 0.234935 (max)
12:51:48 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0743259 (min) 0.0265768 (max)
12:51:48 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.15566 (min) -0.582395 (max)
12:51:48 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0.223209 (min) 0.84984 (max)
12:51:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:51:48 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:51:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:51:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:51:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:51:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:51:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:51:48 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:51:48 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:51:48 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:51:48 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:51:48 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:51:48 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:51:48 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:51:48 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:51:48 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:51:48 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:51:48 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.023193, mean: 0.024605, max: 0.034080
12:51:48 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:51:48 DEBUG opendrift.models.physics_methods:1061: min: 0.829681, mean: 0.854511, max: 1.005732
12:51:48 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 0.829681, mean: 0.854511, max: 1.005732
12:51:48 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:51:48 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:51:48 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:51:48 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:51:48 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:51:48 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:51:48 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:51:48 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:51:48 DEBUG opendrift.models.physics_methods:1061: min: 0.829681, mean: 0.854511, max: 1.005732
12:51:48 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:51:48 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.009284, dN_50: 0.000729
12:51:48 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:51:48 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.0005040671738411064
12:51:48 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:51:48 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:51:48 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:51:48 DEBUG opendrift.models.physics_methods:915: Advecting 3000 of 6000 elements above 0.100m with wind-sheared ocean current (0.029510 m/s - 0.035311 m/s)
12:51:48 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:51:48 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0015934277674514868 and 0.4558445351294395 m/s
12:51:48 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:51:48 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:51:48 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:51:48 INFO opendrift.models.basemodel:2038: 2024-11-06 16:51:18.915878 - step 5 of 120 - 6000 active elements (0 deactivated)
12:51:48 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:51:48 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:51:48 DEBUG opendrift.models.basemodel:2057: 34.97253825200329 <- latitude -> 35.035204894039346
12:51:48 DEBUG opendrift.models.basemodel:2062: 23.487201510675213 <- longitude -> 23.56040334121696
12:51:48 DEBUG opendrift.models.basemodel:2067: -148.1223754816824 <- z -> 0.0
12:51:48 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:51:48 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:51:48 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:51:48 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:51:48 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:51:48 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:51:48 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:51:48 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:51:48 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:51:48 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:51:48 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:51:48 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:51:48 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:51:48 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:51:48 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:51:48 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:51:48 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:51:48 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:51:48 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:51:48 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:51:48 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:51:48 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:51:48 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:51:48 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:51:48 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:51:48 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:51:48 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:51:48 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:51:48 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:51:48 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:51:48 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-06 15:00:00 (before)
2024-11-06 18:00:00 (after)
12:51:48 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-06 15:00:00) in space (linearNDFast)
12:51:48 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:51:48 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-06 18:00:00) in space (linearNDFast)
12:51:48 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:51:48 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-06 15:00:00, weight 0.38) and
after (2024-11-06 18:00:00, weight 0.62) in time
12:51:48 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:51:48 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:51:48 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:51:48 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:51:48 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:51:48 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:51:48 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:51:48 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:51:48 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:51:48 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:51:48 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-06 15:00:00 (before)
2024-11-06 18:00:00 (after)
12:51:48 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-06 15:00:00) in space (linearNDFast)
12:51:48 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:51:48 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-06 18:00:00) in space (linearNDFast)
12:51:48 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:51:48 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-06 15:00:00, weight 0.38) and
after (2024-11-06 18:00:00, weight 0.62) in time
12:51:48 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:51:48 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:51:48 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:51:48 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:51:48 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:51:48 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:51:48 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:51:48 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:51:48 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:51:48 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.00193756 (min) 0.226538 (max)
12:51:48 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0821125 (min) 0.0209655 (max)
12:51:48 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.61 (min) -0.893595 (max)
12:51:48 DEBUG opendrift.models.basemodel.environment:905: y_wind: -0.825093 (min) -0.116418 (max)
12:51:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:51:48 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:51:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:51:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:51:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:51:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:51:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:51:48 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:51:48 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:51:48 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:51:48 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:51:48 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:51:48 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:51:48 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:51:48 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:51:48 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:51:48 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:51:48 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.020187, mean: 0.028755, max: 0.080513
12:51:48 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:51:48 DEBUG opendrift.models.physics_methods:1061: min: 0.774040, mean: 0.920395, max: 1.545835
12:51:48 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 0.774040, mean: 0.920395, max: 1.545835
12:51:48 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:51:48 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:51:48 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:51:48 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:51:48 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:51:48 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:51:48 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:51:48 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:51:48 DEBUG opendrift.models.physics_methods:1061: min: 0.774040, mean: 0.920395, max: 1.545835
12:51:48 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:51:48 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.008587, dN_50: 0.000674
12:51:48 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:51:48 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.0011851093016148997
12:51:48 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:51:48 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:51:48 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:51:48 DEBUG opendrift.models.physics_methods:915: Advecting 3000 of 6000 elements above 0.100m with wind-sheared ocean current (0.028140 m/s - 0.054273 m/s)
12:51:48 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:51:48 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0036340290906254212 and 0.465993503464017 m/s
12:51:48 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:51:48 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:51:48 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:51:48 INFO opendrift.models.basemodel:2038: 2024-11-06 17:51:18.915878 - step 6 of 120 - 6000 active elements (0 deactivated)
12:51:48 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:51:48 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:51:48 DEBUG opendrift.models.basemodel:2057: 34.97007733047352 <- latitude -> 35.03675760751565
12:51:48 DEBUG opendrift.models.basemodel:2062: 23.484900660569185 <- longitude -> 23.565808365740423
12:51:48 DEBUG opendrift.models.basemodel:2067: -147.5108683519258 <- z -> 0.0
12:51:48 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:51:48 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:51:48 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:51:48 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:51:48 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:51:48 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:51:48 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:51:48 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:51:48 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:51:48 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:51:48 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:51:48 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:51:48 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:51:48 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:51:48 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:51:48 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:51:48 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:51:48 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:51:48 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:51:48 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:51:48 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:51:48 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:51:48 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:51:48 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:51:48 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:51:48 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:51:48 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:51:48 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:51:48 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:51:48 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:51:48 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-06 15:00:00 (before)
2024-11-06 18:00:00 (after)
12:51:48 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-06 15:00:00) in space (linearNDFast)
12:51:48 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:51:48 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-06 18:00:00) in space (linearNDFast)
12:51:48 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:51:48 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-06 15:00:00, weight 0.05) and
after (2024-11-06 18:00:00, weight 0.95) in time
12:51:48 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:51:49 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:51:49 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:51:49 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:51:49 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:51:49 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:51:49 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:51:49 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:51:49 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:51:49 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:51:49 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-06 15:00:00 (before)
2024-11-06 18:00:00 (after)
12:51:49 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-06 15:00:00) in space (linearNDFast)
12:51:49 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:51:49 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-06 18:00:00) in space (linearNDFast)
12:51:49 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:51:49 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-06 15:00:00, weight 0.05) and
after (2024-11-06 18:00:00, weight 0.95) in time
12:51:49 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:51:49 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:51:49 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:51:49 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:51:49 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:51:49 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:51:49 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:51:49 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:51:49 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:51:49 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0433637 (min) 0.221584 (max)
12:51:49 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0983841 (min) 0.00459335 (max)
12:51:49 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.92262 (min) -1.2094 (max)
12:51:49 DEBUG opendrift.models.basemodel.environment:905: y_wind: -1.71755 (min) -1.08196 (max)
12:51:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:51:49 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:51:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:51:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:51:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:51:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:51:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:51:49 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:51:49 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:51:49 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:51:49 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:51:49 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:51:49 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:51:49 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:51:49 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:51:49 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:51:49 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:51:49 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.066343, mean: 0.085118, max: 0.163503
12:51:49 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:51:49 DEBUG opendrift.models.physics_methods:1061: min: 1.403227, mean: 1.586958, max: 2.202886
12:51:49 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.403227, mean: 1.586958, max: 2.202886
12:51:49 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:51:49 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:51:49 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:51:49 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:51:49 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:51:49 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:51:49 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:51:49 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:51:49 DEBUG opendrift.models.physics_methods:1061: min: 1.403227, mean: 1.586958, max: 2.202886
12:51:49 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:51:49 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.004489, dN_50: 0.000352
12:51:49 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:51:49 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.002402338605402985
12:51:49 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:51:49 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:51:49 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:51:49 DEBUG opendrift.models.physics_methods:915: Advecting 3000 of 6000 elements above 0.100m with wind-sheared ocean current (0.051179 m/s - 0.077342 m/s)
12:51:49 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:51:49 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.004161841768363598 and 0.445781632089122 m/s
12:51:49 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:51:49 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:51:49 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:51:49 INFO opendrift.models.basemodel:2038: 2024-11-06 18:51:18.915878 - step 7 of 120 - 6000 active elements (0 deactivated)
12:51:49 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:51:49 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:51:49 DEBUG opendrift.models.basemodel:2057: 34.9598444056592 <- latitude -> 35.03620098472288
12:51:49 DEBUG opendrift.models.basemodel:2062: 23.48662767036381 <- longitude -> 23.574460619015102
12:51:49 DEBUG opendrift.models.basemodel:2067: -146.53316508425527 <- z -> 0.0
12:51:49 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:51:49 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:51:49 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:51:49 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:51:49 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:51:49 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:51:49 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:51:49 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:51:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:51:49 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:51:49 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:51:49 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:51:49 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:51:49 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:51:49 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:51:49 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:51:49 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:51:49 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:51:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:51:49 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:51:49 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:51:49 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:51:49 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:51:49 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:51:49 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:51:49 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:51:49 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:51:49 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:51:49 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:51:49 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:51:49 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-06 18:00:00 (before)
2024-11-06 21:00:00 (after)
12:51:49 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
12:51:49 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
12:51:49 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:51:49 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:51:49 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:51:49 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 9x10x23) for time after (2024-11-06 21:00:00)
12:51:49 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-06 18:00:00) in space (linearNDFast)
12:51:49 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:51:49 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-06 21:00:00) in space (linearNDFast)
12:51:49 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:51:49 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-06 18:00:00, weight 0.71) and
after (2024-11-06 21:00:00, weight 0.29) in time
12:51:49 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:51:49 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:51:49 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:51:49 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:51:49 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:51:49 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:51:49 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:51:49 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:51:49 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:51:49 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:51:49 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-06 18:00:00 (before)
2024-11-06 21:00:00 (after)
12:51:49 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_wind to retrieve x_wind
12:51:52 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_wind to retrieve y_wind
12:51:55 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:51:55 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:51:55 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:51:55 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 720x361x1) for time after (2024-11-06 21:00:00)
12:51:55 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-06 18:00:00) in space (linearNDFast)
12:51:55 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:51:55 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-06 21:00:00) in space (linearNDFast)
12:51:55 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:51:55 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-06 18:00:00, weight 0.71) and
after (2024-11-06 21:00:00, weight 0.29) in time
12:51:55 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:51:55 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:51:55 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:51:55 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:51:55 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:51:55 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:51:55 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:51:55 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:51:55 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:51:55 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0655573 (min) 0.191629 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0980649 (min) 0.0246606 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.70185 (min) -0.968859 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.29889 (min) -1.72458 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:51:55 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.103691, mean: 0.123443, max: 0.201257
12:51:55 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:51:55 DEBUG opendrift.models.physics_methods:1061: min: 1.754282, mean: 1.912790, max: 2.444023
12:51:55 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.754282, mean: 1.912790, max: 2.444023
12:51:55 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:51:55 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:51:55 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:51:55 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:51:55 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:51:55 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:51:55 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:51:55 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:51:55 DEBUG opendrift.models.physics_methods:1061: min: 1.754282, mean: 1.912790, max: 2.444023
12:51:55 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:51:55 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.003599, dN_50: 0.000282
12:51:55 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:51:55 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.0029560965641319235
12:51:55 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:51:55 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:51:55 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:51:55 DEBUG opendrift.models.physics_methods:915: Advecting 3000 of 6000 elements above 0.100m with wind-sheared ocean current (0.063779 m/s - 0.085808 m/s)
12:51:55 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:51:55 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0010419219817162152 and 0.4256272139196229 m/s
12:51:55 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:51:55 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:51:55 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:51:55 INFO opendrift.models.basemodel:2038: 2024-11-06 19:51:18.915878 - step 8 of 120 - 6000 active elements (0 deactivated)
12:51:55 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:51:55 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:51:55 DEBUG opendrift.models.basemodel:2057: 34.95315351760472 <- latitude -> 35.038013239056305
12:51:55 DEBUG opendrift.models.basemodel:2062: 23.483574903690197 <- longitude -> 23.578309320898185
12:51:55 DEBUG opendrift.models.basemodel:2067: -146.1128916451689 <- z -> 0.0
12:51:55 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:51:55 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:51:55 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:51:55 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:51:55 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:51:55 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:51:55 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:51:55 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:51:55 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:51:55 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:51:55 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:51:55 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:51:55 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:51:55 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:51:55 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:51:55 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:51:55 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:51:55 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:51:55 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:51:55 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:51:55 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:51:55 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:51:55 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:51:55 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:51:55 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:51:55 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:51:55 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:51:55 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:51:55 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:51:55 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:51:55 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-06 18:00:00 (before)
2024-11-06 21:00:00 (after)
12:51:55 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-06 18:00:00) in space (linearNDFast)
12:51:55 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:51:55 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-06 21:00:00) in space (linearNDFast)
12:51:55 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:51:55 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-06 18:00:00, weight 0.38) and
after (2024-11-06 21:00:00, weight 0.62) in time
12:51:55 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:51:55 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:51:55 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:51:55 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:51:55 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:51:55 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:51:55 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:51:55 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:51:55 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:51:55 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:51:55 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-06 18:00:00 (before)
2024-11-06 21:00:00 (after)
12:51:55 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-06 18:00:00) in space (linearNDFast)
12:51:55 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:51:55 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-06 21:00:00) in space (linearNDFast)
12:51:55 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:51:55 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-06 18:00:00, weight 0.38) and
after (2024-11-06 21:00:00, weight 0.62) in time
12:51:55 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:51:55 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:51:55 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:51:55 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:51:55 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:51:55 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:51:55 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:51:55 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:51:55 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:51:55 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0941011 (min) 0.161863 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0965782 (min) 0.0482958 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.39537 (min) -0.635915 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.85448 (min) -2.36116 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:51:55 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.155404, mean: 0.174362, max: 0.248339
12:51:55 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:51:55 DEBUG opendrift.models.physics_methods:1061: min: 2.147635, mean: 2.274374, max: 2.714891
12:51:55 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.147635, mean: 2.274374, max: 2.714891
12:51:55 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:51:55 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:51:55 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:51:55 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:51:55 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:51:55 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:51:55 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:51:55 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:51:55 DEBUG opendrift.models.physics_methods:1061: min: 2.147635, mean: 2.274374, max: 2.714891
12:51:55 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:51:55 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.002937, dN_50: 0.000230
12:51:55 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:51:55 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.0036466635809654525
12:51:55 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:51:55 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:51:55 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:51:55 DEBUG opendrift.models.physics_methods:915: Advecting 3000 of 6000 elements above 0.100m with wind-sheared ocean current (0.077390 m/s - 0.095318 m/s)
12:51:55 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:51:55 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0018810156762602741 and 0.40454949578149074 m/s
12:51:55 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:51:55 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:51:55 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:51:55 INFO opendrift.models.basemodel:2038: 2024-11-06 20:51:18.915878 - step 9 of 120 - 6000 active elements (0 deactivated)
12:51:55 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:51:55 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:51:55 DEBUG opendrift.models.basemodel:2057: 34.944822364895174 <- latitude -> 35.03026308245333
12:51:55 DEBUG opendrift.models.basemodel:2062: 23.484188739204022 <- longitude -> 23.581711064874483
12:51:55 DEBUG opendrift.models.basemodel:2067: -145.24482834189752 <- z -> 0.0
12:51:55 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:51:55 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:51:55 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:51:55 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:51:55 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:51:55 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:51:55 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:51:55 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:51:55 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:51:55 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:51:55 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:51:55 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:51:55 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:51:55 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:51:55 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:51:55 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:51:55 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:51:55 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:51:55 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:51:55 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:51:55 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:51:55 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:51:55 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:51:55 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:51:55 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:51:55 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:51:55 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:51:55 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:51:55 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:51:55 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:51:55 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-06 18:00:00 (before)
2024-11-06 21:00:00 (after)
12:51:55 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-06 18:00:00) in space (linearNDFast)
12:51:55 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:51:55 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-06 21:00:00) in space (linearNDFast)
12:51:55 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:51:55 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-06 18:00:00, weight 0.05) and
after (2024-11-06 21:00:00, weight 0.95) in time
12:51:55 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:51:55 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:51:55 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:51:55 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:51:55 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:51:55 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:51:55 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:51:55 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:51:55 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:51:55 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:51:55 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-06 18:00:00 (before)
2024-11-06 21:00:00 (after)
12:51:55 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-06 18:00:00) in space (linearNDFast)
12:51:55 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:51:55 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-06 21:00:00) in space (linearNDFast)
12:51:55 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:51:55 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-06 18:00:00, weight 0.05) and
after (2024-11-06 21:00:00, weight 0.95) in time
12:51:55 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:51:55 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:51:55 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:51:55 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:51:55 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:51:55 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:51:55 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:51:55 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:51:55 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:51:55 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0710402 (min) 0.132104 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.108284 (min) 0.0800661 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.98114 (min) -0.309675 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.46459 (min) -2.93776 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:51:55 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:51:55 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.232461, mean: 0.246507, max: 0.306913
12:51:55 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:51:55 DEBUG opendrift.models.physics_methods:1061: min: 2.626664, mean: 2.704575, max: 3.018126
12:51:55 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.626664, mean: 2.704575, max: 3.018126
12:51:55 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:51:55 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:51:55 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:51:55 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:51:55 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:51:56 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:51:56 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:51:56 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:51:56 DEBUG opendrift.models.physics_methods:1061: min: 2.626664, mean: 2.704575, max: 3.018126
12:51:56 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:51:56 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.002401, dN_50: 0.000188
12:51:56 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:51:56 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.004505780765866946
12:51:56 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:51:56 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:51:56 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:51:56 DEBUG opendrift.models.physics_methods:915: Advecting 3000 of 6000 elements above 0.100m with wind-sheared ocean current (0.092221 m/s - 0.104892 m/s)
12:51:56 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:51:56 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.00030840357758222376 and 0.4484315123261148 m/s
12:51:56 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:51:56 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:51:56 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:51:56 INFO opendrift.models.basemodel:2038: 2024-11-06 21:51:18.915878 - step 10 of 120 - 6000 active elements (0 deactivated)
12:51:56 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:51:56 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:51:56 DEBUG opendrift.models.basemodel:2057: 34.947210067737466 <- latitude -> 35.03130925368629
12:51:56 DEBUG opendrift.models.basemodel:2062: 23.48184110257983 <- longitude -> 23.58106754802608
12:51:56 DEBUG opendrift.models.basemodel:2067: -144.12307823955237 <- z -> 0.0
12:51:56 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:51:56 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:51:56 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:51:56 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:51:56 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:51:56 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:51:56 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:51:56 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:51:56 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:51:56 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:51:56 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:51:56 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:51:56 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:51:56 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:51:56 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:51:56 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:51:56 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:51:56 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:51:56 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:51:56 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:51:56 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:51:56 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:51:56 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:51:56 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:51:56 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:51:56 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:51:56 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:51:56 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:51:56 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:51:56 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:51:56 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-06 21:00:00 (before)
2024-11-07 00:00:00 (after)
12:51:56 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
12:51:56 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
12:51:56 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:51:56 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:51:56 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:51:56 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 9x10x23) for time after (2024-11-07 00:00:00)
12:51:56 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-06 21:00:00) in space (linearNDFast)
12:51:56 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:51:56 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-07 00:00:00) in space (linearNDFast)
12:51:56 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:51:56 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-06 21:00:00, weight 0.71) and
after (2024-11-07 00:00:00, weight 0.29) in time
12:51:56 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:51:56 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:51:56 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:51:56 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:51:56 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:51:56 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:51:56 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:51:56 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:51:56 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:51:56 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:51:56 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-06 21:00:00 (before)
2024-11-07 00:00:00 (after)
12:51:56 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_wind to retrieve x_wind
12:52:00 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_wind to retrieve y_wind
12:52:02 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:52:02 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:52:02 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:52:02 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 720x361x1) for time after (2024-11-07 00:00:00)
12:52:02 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-06 21:00:00) in space (linearNDFast)
12:52:02 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:02 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-07 00:00:00) in space (linearNDFast)
12:52:02 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:02 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-06 21:00:00, weight 0.71) and
after (2024-11-07 00:00:00, weight 0.29) in time
12:52:02 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:02 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:02 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:52:02 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:52:02 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:52:02 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:52:02 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:52:02 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:52:02 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:52:02 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0660116 (min) 0.09586 (max)
12:52:02 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0712494 (min) 0.106605 (max)
12:52:02 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.15441 (min) -0.569817 (max)
12:52:02 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.89762 (min) -3.29924 (max)
12:52:02 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:52:02 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:52:02 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:52:02 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:52:02 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:52:02 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:52:02 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:52:02 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:52:02 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:52:02 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:52:02 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:52:02 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:52:02 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:52:02 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:52:02 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:52:02 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:52:02 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:52:02 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.294963, mean: 0.314380, max: 0.398833
12:52:02 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:52:02 DEBUG opendrift.models.physics_methods:1061: min: 2.958782, mean: 3.054174, max: 3.440529
12:52:02 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.958782, mean: 3.054174, max: 3.440529
12:52:02 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:52:02 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:52:03 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:52:03 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:52:03 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:52:03 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:52:03 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:52:03 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:52:03 DEBUG opendrift.models.physics_methods:1061: min: 2.958782, mean: 3.054174, max: 3.440529
12:52:03 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:52:03 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.002090, dN_50: 0.000164
12:52:03 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:52:03 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.005853997897037832
12:52:03 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:52:03 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:52:03 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:52:03 DEBUG opendrift.models.physics_methods:915: Advecting 3000 of 6000 elements above 0.100m with wind-sheared ocean current (0.103881 m/s - 0.116300 m/s)
12:52:03 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:52:03 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0018802227484577855 and 0.4732995180827738 m/s
12:52:03 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:52:03 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:52:03 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:52:03 INFO opendrift.models.basemodel:2038: 2024-11-06 22:51:18.915878 - step 11 of 120 - 6000 active elements (0 deactivated)
12:52:03 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:52:03 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:52:03 DEBUG opendrift.models.basemodel:2057: 34.93634382944402 <- latitude -> 35.028333098027204
12:52:03 DEBUG opendrift.models.basemodel:2062: 23.48232284919789 <- longitude -> 23.582447388517508
12:52:03 DEBUG opendrift.models.basemodel:2067: -143.2463195170896 <- z -> 0.0
12:52:03 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:52:03 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:03 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:52:03 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:03 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:52:03 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:03 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:03 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:52:03 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:52:03 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:03 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:03 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:03 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:52:03 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:03 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:52:03 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:03 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:03 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:52:03 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:52:03 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:52:03 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:03 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:03 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:03 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:52:03 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:03 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:52:03 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:03 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:03 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:52:03 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:52:03 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-06 21:00:00 (before)
2024-11-07 00:00:00 (after)
12:52:03 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-06 21:00:00) in space (linearNDFast)
12:52:03 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:03 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-07 00:00:00) in space (linearNDFast)
12:52:03 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:03 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-06 21:00:00, weight 0.38) and
after (2024-11-07 00:00:00, weight 0.62) in time
12:52:03 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:03 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:03 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:03 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:52:03 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:03 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:52:03 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:03 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:03 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:52:03 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:52:03 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-06 21:00:00 (before)
2024-11-07 00:00:00 (after)
12:52:03 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-06 21:00:00) in space (linearNDFast)
12:52:03 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:03 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-07 00:00:00) in space (linearNDFast)
12:52:03 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:03 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-06 21:00:00, weight 0.38) and
after (2024-11-07 00:00:00, weight 0.62) in time
12:52:03 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:03 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:03 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:52:03 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:52:03 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:52:03 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:52:03 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:52:03 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:52:03 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:52:03 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.076558 (min) 0.0669211 (max)
12:52:03 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0395001 (min) 0.142148 (max)
12:52:03 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.39907 (min) -0.937805 (max)
12:52:03 DEBUG opendrift.models.basemodel.environment:905: y_wind: -4.27903 (min) -3.58812 (max)
12:52:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:52:03 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:52:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:52:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:52:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:52:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:52:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:52:03 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:52:03 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:52:03 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:52:03 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:52:03 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:52:03 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:52:03 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:52:03 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:52:03 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:52:03 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:52:03 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.351289, mean: 0.388889, max: 0.495608
12:52:03 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:52:03 DEBUG opendrift.models.physics_methods:1061: min: 3.228954, mean: 3.396530, max: 3.835294
12:52:03 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.228954, mean: 3.396530, max: 3.835294
12:52:03 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:52:03 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:52:03 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:52:03 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:52:03 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:52:03 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:52:03 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:52:03 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:52:03 DEBUG opendrift.models.physics_methods:1061: min: 3.228954, mean: 3.396530, max: 3.835294
12:52:03 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:52:03 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.001856, dN_50: 0.000146
12:52:03 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:52:03 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.007273418785229604
12:52:03 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:52:03 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:52:03 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:52:03 DEBUG opendrift.models.physics_methods:915: Advecting 3000 of 6000 elements above 0.100m with wind-sheared ocean current (0.113367 m/s - 0.129037 m/s)
12:52:03 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:52:03 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.002096687969625632 and 0.46700195144406736 m/s
12:52:03 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:52:03 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:52:03 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:52:03 INFO opendrift.models.basemodel:2038: 2024-11-06 23:51:18.915878 - step 12 of 120 - 6000 active elements (0 deactivated)
12:52:03 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:52:03 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:52:03 DEBUG opendrift.models.basemodel:2057: 34.935119731671534 <- latitude -> 35.03076393801556
12:52:03 DEBUG opendrift.models.basemodel:2062: 23.47697335969472 <- longitude -> 23.585330106351094
12:52:03 DEBUG opendrift.models.basemodel:2067: -142.47849670050897 <- z -> 0.0
12:52:03 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:52:03 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:03 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:52:03 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:03 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:52:03 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:03 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:03 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:52:03 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:52:03 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:03 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:03 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:03 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:52:03 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:03 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:52:03 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:03 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:03 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:52:03 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:52:03 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:52:03 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:03 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:03 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:03 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:52:03 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:03 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:52:03 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:03 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:03 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:52:03 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:52:03 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-06 21:00:00 (before)
2024-11-07 00:00:00 (after)
12:52:03 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-06 21:00:00) in space (linearNDFast)
12:52:03 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:03 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-07 00:00:00) in space (linearNDFast)
12:52:03 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:03 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-06 21:00:00, weight 0.05) and
after (2024-11-07 00:00:00, weight 0.95) in time
12:52:03 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:03 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:03 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:03 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:52:03 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:03 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:52:03 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:03 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:03 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:52:03 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:52:03 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-06 21:00:00 (before)
2024-11-07 00:00:00 (after)
12:52:03 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-06 21:00:00) in space (linearNDFast)
12:52:03 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:03 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-07 00:00:00) in space (linearNDFast)
12:52:03 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:03 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-06 21:00:00, weight 0.05) and
after (2024-11-07 00:00:00, weight 0.95) in time
12:52:03 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:03 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:03 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:52:03 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:52:03 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:52:03 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:52:03 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:52:03 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:52:03 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:52:03 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0899616 (min) 0.0371263 (max)
12:52:03 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0198968 (min) 0.186452 (max)
12:52:03 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.85172 (min) -1.25241 (max)
12:52:03 DEBUG opendrift.models.basemodel.environment:905: y_wind: -4.76026 (min) -3.86143 (max)
12:52:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:52:03 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:52:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:52:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:52:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:52:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:52:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:52:03 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:52:03 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:52:03 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:52:03 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:52:03 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:52:03 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:52:03 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:52:03 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:52:03 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:52:03 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:52:03 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.410642, mean: 0.474262, max: 0.641789
12:52:03 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:52:03 DEBUG opendrift.models.physics_methods:1061: min: 3.491091, mean: 3.750294, max: 4.364411
12:52:03 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.491091, mean: 3.750294, max: 4.364411
12:52:03 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:52:03 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:52:03 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:52:03 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:52:03 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:52:03 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:52:03 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:52:03 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:52:03 DEBUG opendrift.models.physics_methods:1061: min: 3.491091, mean: 3.750294, max: 4.364411
12:52:03 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:52:03 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.001667, dN_50: 0.000131
12:52:03 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:52:03 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.009417494537563539
12:52:03 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:52:03 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:52:03 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:52:03 DEBUG opendrift.models.physics_methods:915: Advecting 3000 of 6000 elements above 0.100m with wind-sheared ocean current (0.122570 m/s - 0.142271 m/s)
12:52:03 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:52:03 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0016584042501132461 and 0.41051135467426275 m/s
12:52:03 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:52:03 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:52:03 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:52:03 INFO opendrift.models.basemodel:2038: 2024-11-07 00:51:18.915878 - step 13 of 120 - 6000 active elements (0 deactivated)
12:52:03 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:52:03 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:52:03 DEBUG opendrift.models.basemodel:2057: 34.93163814462001 <- latitude -> 35.03476203471817
12:52:03 DEBUG opendrift.models.basemodel:2062: 23.474130003229273 <- longitude -> 23.58662765978335
12:52:03 DEBUG opendrift.models.basemodel:2067: -141.91973385005608 <- z -> 0.0
12:52:03 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:52:03 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:03 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:52:03 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:03 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:52:03 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:03 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:03 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:52:03 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:52:03 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:03 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:03 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:03 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:52:03 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:03 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:52:03 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:03 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:03 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:52:03 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:52:03 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:52:03 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:03 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:03 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:03 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:52:03 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:03 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:52:03 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:03 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:03 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:52:03 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:52:03 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-07 00:00:00 (before)
2024-11-07 03:00:00 (after)
12:52:03 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
12:52:04 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
12:52:04 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:52:04 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:52:04 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:52:04 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 9x10x23) for time after (2024-11-07 03:00:00)
12:52:04 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-07 00:00:00) in space (linearNDFast)
12:52:04 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:04 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-07 03:00:00) in space (linearNDFast)
12:52:04 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:04 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-07 00:00:00, weight 0.71) and
after (2024-11-07 03:00:00, weight 0.29) in time
12:52:04 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:04 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:04 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:04 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:52:04 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:04 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:52:04 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:04 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:04 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:52:04 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:52:04 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-07 00:00:00 (before)
2024-11-07 03:00:00 (after)
12:52:04 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_wind to retrieve x_wind
12:52:07 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_wind to retrieve y_wind
12:52:09 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:52:09 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:52:09 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:52:09 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 720x361x1) for time after (2024-11-07 03:00:00)
12:52:09 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-07 00:00:00) in space (linearNDFast)
12:52:09 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:09 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-07 03:00:00) in space (linearNDFast)
12:52:09 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:09 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-07 00:00:00, weight 0.71) and
after (2024-11-07 03:00:00, weight 0.29) in time
12:52:09 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:09 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:09 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:52:09 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:52:09 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:52:09 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:52:09 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:52:09 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:52:09 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:52:09 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0926449 (min) 0.0470288 (max)
12:52:09 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.00904759 (min) 0.192127 (max)
12:52:09 DEBUG opendrift.models.basemodel.environment:905: x_wind: -2.17865 (min) -1.44607 (max)
12:52:09 DEBUG opendrift.models.basemodel.environment:905: y_wind: -4.43999 (min) -3.55718 (max)
12:52:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:52:09 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:52:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:52:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:52:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:52:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:52:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:52:09 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:52:09 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:52:09 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:52:09 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:52:09 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:52:09 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:52:09 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:52:09 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:52:09 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:52:09 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:52:09 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.371601, mean: 0.420516, max: 0.601717
12:52:09 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:52:09 DEBUG opendrift.models.physics_methods:1061: min: 3.320993, mean: 3.531457, max: 4.225963
12:52:09 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.320993, mean: 3.531457, max: 4.225963
12:52:09 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:52:09 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:52:09 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:52:09 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:52:09 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:52:10 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:52:10 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:52:10 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:52:10 DEBUG opendrift.models.physics_methods:1061: min: 3.320993, mean: 3.531456, max: 4.225964
12:52:10 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:52:10 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.001801, dN_50: 0.000141
12:52:10 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:52:10 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.008829751635407678
12:52:10 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:52:10 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:52:10 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:52:10 DEBUG opendrift.models.physics_methods:915: Advecting 3000 of 6000 elements above 0.100m with wind-sheared ocean current (0.116598 m/s - 0.134353 m/s)
12:52:10 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:52:10 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.002381379748994719 and 0.42621304252069175 m/s
12:52:10 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:52:10 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:52:10 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:52:10 INFO opendrift.models.basemodel:2038: 2024-11-07 01:51:18.915878 - step 14 of 120 - 6000 active elements (0 deactivated)
12:52:10 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:52:10 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:52:10 DEBUG opendrift.models.basemodel:2057: 34.93149842203971 <- latitude -> 35.040458154706386
12:52:10 DEBUG opendrift.models.basemodel:2062: 23.471471144482837 <- longitude -> 23.588148151950406
12:52:10 DEBUG opendrift.models.basemodel:2067: -140.9804357689741 <- z -> 0.0
12:52:10 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:52:10 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:10 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:52:10 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:10 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:52:10 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:10 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:10 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:52:10 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:52:10 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:10 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:10 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:10 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:52:10 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:10 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:52:10 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:10 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:10 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:52:10 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:52:10 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:52:10 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:10 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:10 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:10 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:52:10 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:10 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:52:10 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:10 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:10 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:52:10 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:52:10 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-07 00:00:00 (before)
2024-11-07 03:00:00 (after)
12:52:10 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-07 00:00:00) in space (linearNDFast)
12:52:10 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:10 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-07 03:00:00) in space (linearNDFast)
12:52:10 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:10 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-07 00:00:00, weight 0.38) and
after (2024-11-07 03:00:00, weight 0.62) in time
12:52:10 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:10 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:10 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:10 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:52:10 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:10 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:52:10 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:10 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:10 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:52:10 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:52:10 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-07 00:00:00 (before)
2024-11-07 03:00:00 (after)
12:52:10 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-07 00:00:00) in space (linearNDFast)
12:52:10 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:10 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-07 03:00:00) in space (linearNDFast)
12:52:10 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:10 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-07 00:00:00, weight 0.38) and
after (2024-11-07 03:00:00, weight 0.62) in time
12:52:10 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:10 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:10 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:52:10 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:52:10 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:52:10 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:52:10 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:52:10 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:52:10 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:52:10 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0929065 (min) 0.058832 (max)
12:52:10 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0312795 (min) 0.206268 (max)
12:52:10 DEBUG opendrift.models.basemodel.environment:905: x_wind: -2.4852 (min) -1.64411 (max)
12:52:10 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.97913 (min) -3.12855 (max)
12:52:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:52:10 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:52:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:52:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:52:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:52:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:52:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:52:10 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:52:10 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:52:10 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:52:10 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:52:10 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:52:10 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:52:10 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:52:10 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:52:10 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:52:10 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:52:10 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.315778, mean: 0.356442, max: 0.541438
12:52:10 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:52:10 DEBUG opendrift.models.physics_methods:1061: min: 3.061405, mean: 3.251232, max: 4.008705
12:52:10 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.061405, mean: 3.251232, max: 4.008705
12:52:10 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:52:10 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:52:10 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:52:10 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:52:10 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:52:10 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:52:10 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:52:10 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:52:10 DEBUG opendrift.models.physics_methods:1061: min: 3.061405, mean: 3.251232, max: 4.008705
12:52:10 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:52:10 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.001996, dN_50: 0.000157
12:52:10 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:52:10 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.007945627044442696
12:52:10 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:52:10 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:52:10 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:52:10 DEBUG opendrift.models.physics_methods:915: Advecting 3000 of 6000 elements above 0.100m with wind-sheared ocean current (0.107484 m/s - 0.123441 m/s)
12:52:10 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:52:10 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0010137294541979057 and 0.46543462203736635 m/s
12:52:10 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:52:10 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:52:10 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:52:10 INFO opendrift.models.basemodel:2038: 2024-11-07 02:51:18.915878 - step 15 of 120 - 6000 active elements (0 deactivated)
12:52:10 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:52:10 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:52:10 DEBUG opendrift.models.basemodel:2057: 34.92724787969773 <- latitude -> 35.043022525716744
12:52:10 DEBUG opendrift.models.basemodel:2062: 23.472478513881544 <- longitude -> 23.592930886751315
12:52:10 DEBUG opendrift.models.basemodel:2067: -140.49910507230817 <- z -> 0.0
12:52:10 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:52:10 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:10 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:52:10 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:10 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:52:10 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:10 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:10 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:52:10 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:52:10 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:10 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:10 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:10 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:52:10 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:10 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:52:10 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:10 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:10 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:52:10 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:52:10 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:52:10 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:10 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:10 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:10 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:52:10 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:10 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:52:10 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:10 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:10 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:52:10 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:52:10 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-07 00:00:00 (before)
2024-11-07 03:00:00 (after)
12:52:10 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-07 00:00:00) in space (linearNDFast)
12:52:10 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:10 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-07 03:00:00) in space (linearNDFast)
12:52:10 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:10 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-07 00:00:00, weight 0.05) and
after (2024-11-07 03:00:00, weight 0.95) in time
12:52:10 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:10 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:10 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:10 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:52:10 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:10 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:52:10 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:10 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:10 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:52:10 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:52:10 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-07 00:00:00 (before)
2024-11-07 03:00:00 (after)
12:52:10 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-07 00:00:00) in space (linearNDFast)
12:52:10 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:10 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-07 03:00:00) in space (linearNDFast)
12:52:10 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:10 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-07 00:00:00, weight 0.05) and
after (2024-11-07 03:00:00, weight 0.95) in time
12:52:10 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:10 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:10 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:52:10 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:52:10 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:52:10 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:52:10 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:52:10 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:52:10 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:52:10 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0953431 (min) 0.0755235 (max)
12:52:10 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0448522 (min) 0.21006 (max)
12:52:10 DEBUG opendrift.models.basemodel.environment:905: x_wind: -2.71814 (min) -1.84629 (max)
12:52:10 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.44925 (min) -2.67133 (max)
12:52:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:52:10 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:52:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:52:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:52:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:52:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:52:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:52:10 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:52:10 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:52:10 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:52:10 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:52:10 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:52:10 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:52:10 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:52:10 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:52:10 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:52:10 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:52:10 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.259402, mean: 0.307890, max: 0.470792
12:52:10 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:52:10 DEBUG opendrift.models.physics_methods:1061: min: 2.774702, mean: 3.021287, max: 3.738043
12:52:10 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.774702, mean: 3.021287, max: 3.738043
12:52:10 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:52:10 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:52:10 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:52:10 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:52:10 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:52:10 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:52:10 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:52:10 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:52:10 DEBUG opendrift.models.physics_methods:1061: min: 2.774702, mean: 3.021287, max: 3.738043
12:52:10 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:52:10 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.002187, dN_50: 0.000172
12:52:10 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:52:10 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.00690944312824373
12:52:10 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:52:10 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:52:10 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:52:10 DEBUG opendrift.models.physics_methods:915: Advecting 3000 of 6000 elements above 0.100m with wind-sheared ocean current (0.097418 m/s - 0.117770 m/s)
12:52:10 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:52:10 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0005100047373104568 and 0.448816599910058 m/s
12:52:10 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:52:10 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:52:10 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:52:10 INFO opendrift.models.basemodel:2038: 2024-11-07 03:51:18.915878 - step 16 of 120 - 6000 active elements (0 deactivated)
12:52:10 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:52:10 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:52:10 DEBUG opendrift.models.basemodel:2057: 34.92676845351632 <- latitude -> 35.04409759059299
12:52:10 DEBUG opendrift.models.basemodel:2062: 23.469054604496964 <- longitude -> 23.594447585477642
12:52:10 DEBUG opendrift.models.basemodel:2067: -140.01050389410264 <- z -> 0.0
12:52:10 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:52:10 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:10 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:52:10 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:10 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:52:10 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:10 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:10 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:52:10 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:52:10 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:10 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:10 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:10 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:52:10 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:10 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:52:10 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:10 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:10 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:52:10 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:52:10 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:52:10 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:10 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:10 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:10 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:52:10 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:10 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:52:10 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:10 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:10 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:52:10 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:52:10 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-07 03:00:00 (before)
2024-11-07 06:00:00 (after)
12:52:10 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
12:52:11 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
12:52:11 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:52:11 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:52:11 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:52:11 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 10x11x23) for time after (2024-11-07 06:00:00)
12:52:11 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-07 03:00:00) in space (linearNDFast)
12:52:11 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:11 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-07 06:00:00) in space (linearNDFast)
12:52:11 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:11 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-07 03:00:00, weight 0.71) and
after (2024-11-07 06:00:00, weight 0.29) in time
12:52:11 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:11 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:11 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:11 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:52:11 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:11 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:52:11 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:11 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:11 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:52:11 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:52:11 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-07 03:00:00 (before)
2024-11-07 06:00:00 (after)
12:52:11 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_wind to retrieve x_wind
12:52:14 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_wind to retrieve y_wind
12:52:17 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:52:17 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:52:17 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:52:17 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 720x361x1) for time after (2024-11-07 06:00:00)
12:52:17 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-07 03:00:00) in space (linearNDFast)
12:52:17 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:17 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-07 06:00:00) in space (linearNDFast)
12:52:17 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:17 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-07 03:00:00, weight 0.71) and
after (2024-11-07 06:00:00, weight 0.29) in time
12:52:17 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:17 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:17 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:52:17 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:52:17 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:52:17 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:52:17 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:52:17 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:52:17 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:52:17 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.118666 (min) 0.0962955 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0439134 (min) 0.211977 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: x_wind: -2.6214 (min) -1.72587 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.58498 (min) -2.66254 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:52:17 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.247667, mean: 0.295551, max: 0.485207
12:52:17 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:52:17 DEBUG opendrift.models.physics_methods:1061: min: 2.711211, mean: 2.959851, max: 3.794837
12:52:17 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.711211, mean: 2.959851, max: 3.794837
12:52:17 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:52:17 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:52:17 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:52:17 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:52:17 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:52:17 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:52:17 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:52:17 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:52:17 DEBUG opendrift.models.physics_methods:1061: min: 2.711211, mean: 2.959851, max: 3.794837
12:52:17 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:52:17 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.002255, dN_50: 0.000177
12:52:17 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:52:17 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.007120864992521181
12:52:17 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:52:17 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:52:17 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:52:17 DEBUG opendrift.models.physics_methods:915: Advecting 3000 of 6000 elements above 0.100m with wind-sheared ocean current (0.095189 m/s - 0.118282 m/s)
12:52:17 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:52:17 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0004169399674894707 and 0.4502459559015394 m/s
12:52:17 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:52:17 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:52:17 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:52:17 INFO opendrift.models.basemodel:2038: 2024-11-07 04:51:18.915878 - step 17 of 120 - 6000 active elements (0 deactivated)
12:52:17 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:52:17 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:52:17 DEBUG opendrift.models.basemodel:2057: 34.926035751124836 <- latitude -> 35.05301436359296
12:52:17 DEBUG opendrift.models.basemodel:2062: 23.467835039552465 <- longitude -> 23.590752950356386
12:52:17 DEBUG opendrift.models.basemodel:2067: -138.5308209826548 <- z -> 0.0
12:52:17 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:52:17 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:17 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:52:17 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:17 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:52:17 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:17 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:17 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:52:17 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:52:17 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:17 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:17 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:17 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:52:17 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:17 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:52:17 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:17 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:17 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:52:17 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:52:17 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:52:17 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:17 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:17 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:17 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:52:17 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:17 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:52:17 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:17 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:17 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:52:17 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:52:17 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-07 03:00:00 (before)
2024-11-07 06:00:00 (after)
12:52:17 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-07 03:00:00) in space (linearNDFast)
12:52:17 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:17 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-07 06:00:00) in space (linearNDFast)
12:52:17 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:17 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-07 03:00:00, weight 0.38) and
after (2024-11-07 06:00:00, weight 0.62) in time
12:52:17 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:17 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:17 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:17 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:52:17 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:17 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:52:17 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:17 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:17 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:52:17 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:52:17 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-07 03:00:00 (before)
2024-11-07 06:00:00 (after)
12:52:17 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-07 03:00:00) in space (linearNDFast)
12:52:17 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:17 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-07 06:00:00) in space (linearNDFast)
12:52:17 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:17 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-07 03:00:00, weight 0.38) and
after (2024-11-07 06:00:00, weight 0.62) in time
12:52:17 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:17 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:17 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:52:17 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:52:17 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:52:17 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:52:17 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:52:17 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:52:17 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:52:17 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.112151 (min) 0.121531 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0410788 (min) 0.21471 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: x_wind: -2.3927 (min) -1.5436 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.71684 (min) -2.74283 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:52:17 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.248633, mean: 0.291550, max: 0.480683
12:52:17 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:52:17 DEBUG opendrift.models.physics_methods:1061: min: 2.716493, mean: 2.939526, max: 3.777104
12:52:17 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.716493, mean: 2.939526, max: 3.777104
12:52:17 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:52:17 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:52:17 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:52:17 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:52:17 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:52:17 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:52:17 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:52:17 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:52:17 DEBUG opendrift.models.physics_methods:1061: min: 2.716493, mean: 2.939526, max: 3.777104
12:52:17 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:52:17 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.002289, dN_50: 0.000180
12:52:17 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:52:17 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.007054509396539768
12:52:17 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:52:17 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:52:17 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:52:17 DEBUG opendrift.models.physics_methods:915: Advecting 3000 of 6000 elements above 0.100m with wind-sheared ocean current (0.095375 m/s - 0.117825 m/s)
12:52:17 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:52:17 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0036448558374618917 and 0.43510560648902263 m/s
12:52:17 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:52:17 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:52:17 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:52:17 INFO opendrift.models.basemodel:2038: 2024-11-07 05:51:18.915878 - step 18 of 120 - 6000 active elements (0 deactivated)
12:52:17 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:52:17 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:52:17 DEBUG opendrift.models.basemodel:2057: 34.92702372874069 <- latitude -> 35.05814572257176
12:52:17 DEBUG opendrift.models.basemodel:2062: 23.47019392017243 <- longitude -> 23.59260092948562
12:52:17 DEBUG opendrift.models.basemodel:2067: -137.3135987157782 <- z -> 0.0
12:52:17 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:52:17 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:17 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:52:17 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:17 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:52:17 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:17 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:17 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:52:17 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:52:17 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:17 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:17 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:17 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:52:17 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:17 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:52:17 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:17 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:17 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:52:17 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:52:17 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:52:17 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:17 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:17 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:17 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:52:17 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:17 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:52:17 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:17 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:17 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:52:17 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:52:17 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-07 03:00:00 (before)
2024-11-07 06:00:00 (after)
12:52:17 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-07 03:00:00) in space (linearNDFast)
12:52:17 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:17 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-07 06:00:00) in space (linearNDFast)
12:52:17 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:17 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-07 03:00:00, weight 0.05) and
after (2024-11-07 06:00:00, weight 0.95) in time
12:52:17 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:17 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:17 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:17 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:52:17 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:17 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:52:17 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:17 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:17 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:52:17 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:52:17 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-07 03:00:00 (before)
2024-11-07 06:00:00 (after)
12:52:17 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-07 03:00:00) in space (linearNDFast)
12:52:17 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:17 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-07 06:00:00) in space (linearNDFast)
12:52:17 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:17 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-07 03:00:00, weight 0.05) and
after (2024-11-07 06:00:00, weight 0.95) in time
12:52:17 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:17 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:17 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:52:17 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:52:17 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:52:17 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:52:17 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:52:17 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:52:17 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:52:17 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.127571 (min) 0.157566 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0354934 (min) 0.21709 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: x_wind: -2.2276 (min) -1.26997 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.90217 (min) -2.82795 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:52:17 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:52:17 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.247458, mean: 0.291511, max: 0.495335
12:52:17 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:52:17 DEBUG opendrift.models.physics_methods:1061: min: 2.710070, mean: 2.939148, max: 3.834239
12:52:17 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.710070, mean: 2.939148, max: 3.834239
12:52:17 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:52:17 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:52:17 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:52:17 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:52:17 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:52:17 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:52:17 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:52:17 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:52:17 DEBUG opendrift.models.physics_methods:1061: min: 2.710070, mean: 2.939148, max: 3.834239
12:52:17 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:52:17 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.002307, dN_50: 0.000181
12:52:18 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:52:18 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.007269418534547365
12:52:18 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:52:18 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:52:18 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:52:18 DEBUG opendrift.models.physics_methods:915: Advecting 3000 of 6000 elements above 0.100m with wind-sheared ocean current (0.095149 m/s - 0.123028 m/s)
12:52:18 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:52:18 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0013611490288181931 and 0.4236016440884586 m/s
12:52:18 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:52:18 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:52:18 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:52:18 INFO opendrift.models.basemodel:2038: 2024-11-07 06:51:18.915878 - step 19 of 120 - 6000 active elements (0 deactivated)
12:52:18 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:52:18 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:52:18 DEBUG opendrift.models.basemodel:2057: 34.92532498054074 <- latitude -> 35.0605538781076
12:52:18 DEBUG opendrift.models.basemodel:2062: 23.46878366048699 <- longitude -> 23.590386004133663
12:52:18 DEBUG opendrift.models.basemodel:2067: -136.56450765878526 <- z -> 0.0
12:52:18 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:52:18 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:18 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:52:18 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:18 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:52:18 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:18 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:18 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:52:18 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:52:18 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:18 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:18 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:18 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:52:18 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:18 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:52:18 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:18 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:18 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:52:18 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:52:18 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:52:18 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:18 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:18 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:18 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:52:18 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:18 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:52:18 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:18 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:18 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:52:18 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:52:18 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-07 06:00:00 (before)
2024-11-07 09:00:00 (after)
12:52:18 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
12:52:18 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
12:52:18 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:52:18 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:52:18 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:52:18 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 10x11x23) for time after (2024-11-07 09:00:00)
12:52:18 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-07 06:00:00) in space (linearNDFast)
12:52:18 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:18 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-07 09:00:00) in space (linearNDFast)
12:52:18 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:18 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-07 06:00:00, weight 0.71) and
after (2024-11-07 09:00:00, weight 0.29) in time
12:52:18 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:18 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:18 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:18 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:52:18 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:18 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:52:18 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:18 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:18 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:52:18 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:52:18 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-07 06:00:00 (before)
2024-11-07 09:00:00 (after)
12:52:18 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_wind to retrieve x_wind
12:52:22 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_wind to retrieve y_wind
12:52:25 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:52:25 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:52:25 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:52:25 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 720x361x1) for time after (2024-11-07 09:00:00)
12:52:25 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-07 06:00:00) in space (linearNDFast)
12:52:25 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:25 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-07 09:00:00) in space (linearNDFast)
12:52:25 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:25 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-07 06:00:00, weight 0.71) and
after (2024-11-07 09:00:00, weight 0.29) in time
12:52:25 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:25 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:25 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:52:25 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:52:25 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:52:25 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:52:25 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:52:25 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:52:25 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:52:25 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.136744 (min) 0.164098 (max)
12:52:25 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0242669 (min) 0.205831 (max)
12:52:25 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.87023 (min) -0.890447 (max)
12:52:25 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.37728 (min) -2.26423 (max)
12:52:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:52:25 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:52:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:52:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:52:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:52:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:52:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:52:25 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:52:25 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:52:25 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:52:25 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:52:25 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:52:25 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:52:25 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:52:25 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:52:25 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:52:25 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:52:25 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.159332, mean: 0.188209, max: 0.366633
12:52:25 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:52:25 DEBUG opendrift.models.physics_methods:1061: min: 2.174608, mean: 2.360196, max: 3.298718
12:52:25 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.174608, mean: 2.360196, max: 3.298718
12:52:25 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:52:25 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:52:25 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:52:25 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:52:25 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:52:25 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:52:25 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:52:25 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:52:25 DEBUG opendrift.models.physics_methods:1061: min: 2.174608, mean: 2.360196, max: 3.298718
12:52:25 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:52:25 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.002981, dN_50: 0.000234
12:52:25 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:52:25 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.005381704336126213
12:52:25 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:52:25 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:52:25 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:52:25 DEBUG opendrift.models.physics_methods:915: Advecting 3000 of 6000 elements above 0.100m with wind-sheared ocean current (0.076349 m/s - 0.102756 m/s)
12:52:25 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:52:25 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.001790071247880411 and 0.4718204805265167 m/s
12:52:25 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:52:25 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:52:25 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:52:25 INFO opendrift.models.basemodel:2038: 2024-11-07 07:51:18.915878 - step 20 of 120 - 6000 active elements (0 deactivated)
12:52:25 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:52:25 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:52:25 DEBUG opendrift.models.basemodel:2057: 34.92559593173059 <- latitude -> 35.06241316364871
12:52:25 DEBUG opendrift.models.basemodel:2062: 23.468110271274583 <- longitude -> 23.593805767623323
12:52:25 DEBUG opendrift.models.basemodel:2067: -135.75801891396907 <- z -> 0.0
12:52:25 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:52:25 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:25 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:52:25 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:25 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:52:25 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:25 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:25 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:52:25 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:52:25 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:25 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:25 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:25 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:52:25 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:25 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:52:25 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:25 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:25 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:52:25 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:52:25 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:52:25 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:25 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:25 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:25 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:52:25 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:25 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:52:25 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:25 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:25 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:52:25 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:52:25 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-07 06:00:00 (before)
2024-11-07 09:00:00 (after)
12:52:25 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-07 06:00:00) in space (linearNDFast)
12:52:25 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:25 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-07 09:00:00) in space (linearNDFast)
12:52:25 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:25 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-07 06:00:00, weight 0.38) and
after (2024-11-07 09:00:00, weight 0.62) in time
12:52:25 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:25 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:25 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:25 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:52:25 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:25 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:52:25 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:25 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:25 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:52:25 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:52:25 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-07 06:00:00 (before)
2024-11-07 09:00:00 (after)
12:52:25 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-07 06:00:00) in space (linearNDFast)
12:52:25 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:25 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-07 09:00:00) in space (linearNDFast)
12:52:25 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:25 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-07 06:00:00, weight 0.38) and
after (2024-11-07 09:00:00, weight 0.62) in time
12:52:25 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:25 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:25 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:52:25 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:52:25 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:52:25 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:52:25 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:52:25 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:52:25 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:52:25 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.129277 (min) 0.163767 (max)
12:52:25 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.00202772 (min) 0.197243 (max)
12:52:25 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.66883 (min) -0.496876 (max)
12:52:25 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.90424 (min) -1.61033 (max)
12:52:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:52:25 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:52:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:52:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:52:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:52:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:52:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:52:25 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:52:25 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:52:25 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:52:25 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:52:25 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:52:25 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:52:25 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:52:25 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:52:25 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:52:25 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:52:25 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.080115, mean: 0.096170, max: 0.276002
12:52:25 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:52:25 DEBUG opendrift.models.physics_methods:1061: min: 1.542009, mean: 1.683970, max: 2.862105
12:52:25 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.542009, mean: 1.683970, max: 2.862105
12:52:25 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:52:25 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:52:25 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:52:25 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:52:25 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:52:25 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:52:25 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:52:25 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:52:25 DEBUG opendrift.models.physics_methods:1061: min: 1.542009, mean: 1.683970, max: 2.862105
12:52:25 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:52:25 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.004410, dN_50: 0.000346
12:52:25 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:52:25 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.004052395958483159
12:52:25 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:52:25 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:52:25 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:52:26 DEBUG opendrift.models.physics_methods:915: Advecting 3000 of 6000 elements above 0.100m with wind-sheared ocean current (0.054139 m/s - 0.078744 m/s)
12:52:26 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:52:26 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0015699985914477657 and 0.4584055201733749 m/s
12:52:26 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:52:26 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:52:26 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:52:26 INFO opendrift.models.basemodel:2038: 2024-11-07 08:51:18.915878 - step 21 of 120 - 6000 active elements (0 deactivated)
12:52:26 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:52:26 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:52:26 DEBUG opendrift.models.basemodel:2057: 34.92350421617329 <- latitude -> 35.06931721714877
12:52:26 DEBUG opendrift.models.basemodel:2062: 23.47213420698274 <- longitude -> 23.596987672037887
12:52:26 DEBUG opendrift.models.basemodel:2067: -134.93684562405832 <- z -> 0.0
12:52:26 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:52:26 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:26 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:52:26 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:26 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:52:26 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:26 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:26 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:52:26 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:52:26 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:26 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:26 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:26 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:52:26 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:26 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:52:26 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:26 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:26 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:52:26 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:52:26 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:52:26 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:26 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:26 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:26 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:52:26 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:26 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:52:26 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:26 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:26 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:52:26 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:52:26 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-07 06:00:00 (before)
2024-11-07 09:00:00 (after)
12:52:26 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-07 06:00:00) in space (linearNDFast)
12:52:26 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:26 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-07 09:00:00) in space (linearNDFast)
12:52:26 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:26 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-07 06:00:00, weight 0.05) and
after (2024-11-07 09:00:00, weight 0.95) in time
12:52:26 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:26 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:26 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:26 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:52:26 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:26 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:52:26 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:26 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:26 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:52:26 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:52:26 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-07 06:00:00 (before)
2024-11-07 09:00:00 (after)
12:52:26 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-07 06:00:00) in space (linearNDFast)
12:52:26 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:26 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-07 09:00:00) in space (linearNDFast)
12:52:26 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:26 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-07 06:00:00, weight 0.05) and
after (2024-11-07 09:00:00, weight 0.95) in time
12:52:26 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:26 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:26 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:52:26 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:52:26 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:52:26 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:52:26 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:52:26 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:52:26 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:52:26 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.165987 (min) 0.171576 (max)
12:52:26 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.032268 (min) 0.193212 (max)
12:52:26 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.32749 (min) -0.105305 (max)
12:52:26 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.29582 (min) -0.953622 (max)
12:52:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:52:26 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:52:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:52:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:52:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:52:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:52:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:52:26 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:52:26 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:52:26 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:52:26 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:52:26 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:52:26 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:52:26 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:52:26 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:52:26 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:52:26 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:52:26 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.025330, mean: 0.035417, max: 0.173012
12:52:26 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:52:26 DEBUG opendrift.models.physics_methods:1061: min: 0.867057, mean: 1.014844, max: 2.266041
12:52:26 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 0.867057, mean: 1.014844, max: 2.266041
12:52:26 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:52:26 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:52:26 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:52:26 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:52:26 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:52:26 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:52:26 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:52:26 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:52:26 DEBUG opendrift.models.physics_methods:1061: min: 0.867057, mean: 1.014844, max: 2.266041
12:52:26 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:52:26 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.008012, dN_50: 0.000629
12:52:26 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:52:26 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.002541816113202514
12:52:26 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:52:26 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:52:26 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:52:26 DEBUG opendrift.models.physics_methods:915: Advecting 3000 of 6000 elements above 0.100m with wind-sheared ocean current (0.030442 m/s - 0.058194 m/s)
12:52:26 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:52:26 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0025886005479159218 and 0.4620561572740499 m/s
12:52:26 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:52:26 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:52:26 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:52:26 INFO opendrift.models.basemodel:2038: 2024-11-07 09:51:18.915878 - step 22 of 120 - 6000 active elements (0 deactivated)
12:52:26 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:52:26 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:52:26 DEBUG opendrift.models.basemodel:2057: 34.9241505147121 <- latitude -> 35.07075089061683
12:52:26 DEBUG opendrift.models.basemodel:2062: 23.470225392164643 <- longitude -> 23.60309227416791
12:52:26 DEBUG opendrift.models.basemodel:2067: -134.18488792203757 <- z -> 0.0
12:52:26 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:52:26 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:26 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:52:26 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:26 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:52:26 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:26 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:26 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:52:26 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:52:26 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:26 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:26 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:26 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:52:26 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:26 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:52:26 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:26 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:26 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:52:26 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:52:26 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:52:26 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:26 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:26 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:26 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:52:26 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:26 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:52:26 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:26 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:26 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:52:26 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:52:26 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-07 09:00:00 (before)
2024-11-07 12:00:00 (after)
12:52:26 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
12:52:27 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
12:52:27 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:52:27 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:52:27 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:52:27 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 10x11x23) for time after (2024-11-07 12:00:00)
12:52:27 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-07 09:00:00) in space (linearNDFast)
12:52:27 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:27 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-07 12:00:00) in space (linearNDFast)
12:52:27 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:27 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-07 09:00:00, weight 0.71) and
after (2024-11-07 12:00:00, weight 0.29) in time
12:52:27 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:27 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:27 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:27 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:52:27 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:27 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:52:27 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:27 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:27 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:52:27 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:52:27 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-07 09:00:00 (before)
2024-11-07 12:00:00 (after)
12:52:27 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_wind to retrieve x_wind
12:52:30 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_wind to retrieve y_wind
12:52:32 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:52:32 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:52:32 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:52:32 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 720x361x1) for time after (2024-11-07 12:00:00)
12:52:32 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-07 09:00:00) in space (linearNDFast)
12:52:32 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:32 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-07 12:00:00) in space (linearNDFast)
12:52:32 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:32 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-07 09:00:00, weight 0.71) and
after (2024-11-07 12:00:00, weight 0.29) in time
12:52:32 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:32 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:32 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:52:32 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:52:32 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:52:32 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:52:32 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:52:32 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:52:32 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:52:32 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.181515 (min) 0.145172 (max)
12:52:32 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0403907 (min) 0.188611 (max)
12:52:32 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.857899 (min) 0.432989 (max)
12:52:32 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.22031 (min) -0.83513 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:52:33 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.017823, mean: 0.030245, max: 0.139378
12:52:33 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:52:33 DEBUG opendrift.models.physics_methods:1061: min: 0.727318, mean: 0.939382, max: 2.033884
12:52:33 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 0.727318, mean: 0.939382, max: 2.033884
12:52:33 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:52:33 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:52:33 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:52:33 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:52:33 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:52:33 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:52:33 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:52:33 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:52:33 DEBUG opendrift.models.physics_methods:1061: min: 0.727318, mean: 0.939382, max: 2.033884
12:52:33 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:52:33 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.008746, dN_50: 0.000686
12:52:33 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:52:33 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.0020484904972551837
12:52:33 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:52:33 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:52:33 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:52:33 DEBUG opendrift.models.physics_methods:915: Advecting 3000 of 6000 elements above 0.100m with wind-sheared ocean current (0.025536 m/s - 0.053491 m/s)
12:52:33 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:52:33 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0022526256461819017 and 0.47493229902157874 m/s
12:52:33 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:52:33 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:52:33 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:52:33 INFO opendrift.models.basemodel:2038: 2024-11-07 10:51:18.915878 - step 23 of 120 - 6000 active elements (0 deactivated)
12:52:33 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:52:33 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:52:33 DEBUG opendrift.models.basemodel:2057: 34.92755450414757 <- latitude -> 35.072338163159955
12:52:33 DEBUG opendrift.models.basemodel:2062: 23.466916950137342 <- longitude -> 23.608027432885773
12:52:33 DEBUG opendrift.models.basemodel:2067: -133.57436868548893 <- z -> 0.0
12:52:33 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:52:33 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:33 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:52:33 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:33 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:52:33 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:33 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:33 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:52:33 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:52:33 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:33 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:33 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:33 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:52:33 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:33 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:52:33 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:33 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:33 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:52:33 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:52:33 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:52:33 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:33 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:33 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:33 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:52:33 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:33 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:52:33 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:33 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:33 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:52:33 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:52:33 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-07 09:00:00 (before)
2024-11-07 12:00:00 (after)
12:52:33 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-07 09:00:00) in space (linearNDFast)
12:52:33 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:33 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-07 12:00:00) in space (linearNDFast)
12:52:33 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:33 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-07 09:00:00, weight 0.38) and
after (2024-11-07 12:00:00, weight 0.62) in time
12:52:33 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:33 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:33 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:33 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:52:33 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:33 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:52:33 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:33 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:33 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:52:33 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:52:33 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-07 09:00:00 (before)
2024-11-07 12:00:00 (after)
12:52:33 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-07 09:00:00) in space (linearNDFast)
12:52:33 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:33 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-07 12:00:00) in space (linearNDFast)
12:52:33 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:33 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-07 09:00:00, weight 0.38) and
after (2024-11-07 12:00:00, weight 0.62) in time
12:52:33 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:33 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:33 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:52:33 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:52:33 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:52:33 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:52:33 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:52:33 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:52:33 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:52:33 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.191501 (min) 0.114529 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0441222 (min) 0.187549 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.42429 (min) 0.998695 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.29955 (min) -0.77427 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:52:33 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.022383, mean: 0.042939, max: 0.134512
12:52:33 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:52:33 DEBUG opendrift.models.physics_methods:1061: min: 0.815053, mean: 1.125080, max: 1.998068
12:52:33 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 0.815053, mean: 1.125080, max: 1.998068
12:52:33 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:52:33 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:52:33 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:52:33 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:52:33 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:52:33 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:52:33 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:52:33 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:52:33 DEBUG opendrift.models.physics_methods:1061: min: 0.815053, mean: 1.125080, max: 1.998068
12:52:33 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:52:33 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.007039, dN_50: 0.000552
12:52:33 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:52:33 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.0019771261044103814
12:52:33 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:52:33 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:52:33 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:52:33 DEBUG opendrift.models.physics_methods:915: Advecting 3000 of 6000 elements above 0.100m with wind-sheared ocean current (0.028616 m/s - 0.054862 m/s)
12:52:33 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:52:33 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.003522229351052053 and 0.4665936127275161 m/s
12:52:33 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:52:33 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:52:33 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:52:33 INFO opendrift.models.basemodel:2038: 2024-11-07 11:51:18.915878 - step 24 of 120 - 6000 active elements (0 deactivated)
12:52:33 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:52:33 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:52:33 DEBUG opendrift.models.basemodel:2057: 34.92428306090195 <- latitude -> 35.074424578045715
12:52:33 DEBUG opendrift.models.basemodel:2062: 23.45976587428229 <- longitude -> 23.614475568254406
12:52:33 DEBUG opendrift.models.basemodel:2067: -132.46991349191566 <- z -> 0.0
12:52:33 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:52:33 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:33 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:52:33 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:33 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:52:33 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:33 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:33 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:52:33 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:52:33 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:33 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:33 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:33 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:52:33 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:33 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:52:33 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:33 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:33 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:52:33 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:52:33 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:52:33 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:33 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:33 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:33 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:52:33 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:33 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:52:33 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:33 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:33 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:52:33 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:52:33 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-07 09:00:00 (before)
2024-11-07 12:00:00 (after)
12:52:33 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-07 09:00:00) in space (linearNDFast)
12:52:33 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:33 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-07 12:00:00) in space (linearNDFast)
12:52:33 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:33 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-07 09:00:00, weight 0.05) and
after (2024-11-07 12:00:00, weight 0.95) in time
12:52:33 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:33 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:33 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:33 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:52:33 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:33 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:52:33 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:33 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:33 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:52:33 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:52:33 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-07 09:00:00 (before)
2024-11-07 12:00:00 (after)
12:52:33 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-07 09:00:00) in space (linearNDFast)
12:52:33 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:33 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-07 12:00:00) in space (linearNDFast)
12:52:33 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:33 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-07 09:00:00, weight 0.05) and
after (2024-11-07 12:00:00, weight 0.95) in time
12:52:33 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:33 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:33 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:52:33 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:52:33 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:52:33 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:52:33 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:52:33 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:52:33 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:52:33 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.252638 (min) 0.082704 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0591976 (min) 0.187144 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.0924578 (min) 1.57331 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.44556 (min) -0.697227 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:52:33 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:52:33 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.033387, mean: 0.068012, max: 0.147337
12:52:33 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:52:33 DEBUG opendrift.models.physics_methods:1061: min: 0.995444, mean: 1.417812, max: 2.091149
12:52:33 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 0.995444, mean: 1.417812, max: 2.091149
12:52:33 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:52:33 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:52:33 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:52:33 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:52:33 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:52:33 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:52:33 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:52:33 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:52:33 DEBUG opendrift.models.physics_methods:1061: min: 0.995444, mean: 1.417812, max: 2.091149
12:52:33 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:52:33 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.005395, dN_50: 0.000423
12:52:33 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:52:33 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.0021652282303546103
12:52:33 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:52:33 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:52:33 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:52:34 DEBUG opendrift.models.physics_methods:915: Advecting 3000 of 6000 elements above 0.100m with wind-sheared ocean current (0.034950 m/s - 0.059604 m/s)
12:52:34 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:52:34 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0033112070621011567 and 0.454073836509572 m/s
12:52:34 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:52:34 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:52:34 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:52:34 INFO opendrift.models.basemodel:2038: 2024-11-07 12:51:18.915878 - step 25 of 120 - 6000 active elements (0 deactivated)
12:52:34 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:52:34 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:52:34 DEBUG opendrift.models.basemodel:2057: 34.9213975151028 <- latitude -> 35.079486087757466
12:52:34 DEBUG opendrift.models.basemodel:2062: 23.46366848954585 <- longitude -> 23.608352416620058
12:52:34 DEBUG opendrift.models.basemodel:2067: -131.98205277511562 <- z -> 0.0
12:52:34 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:52:34 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:34 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:52:34 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:34 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:52:34 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:34 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:34 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:52:34 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:52:34 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:34 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:34 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:34 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:52:34 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:34 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:52:34 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:34 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:34 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:52:34 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:52:34 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:52:34 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:34 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:34 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:34 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:52:34 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:34 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:52:34 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:34 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:34 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:52:34 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:52:34 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-07 12:00:00 (before)
2024-11-07 15:00:00 (after)
12:52:34 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
12:52:37 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
12:52:37 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:52:37 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:52:37 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:52:37 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 10x12x23) for time after (2024-11-07 15:00:00)
12:52:37 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-07 12:00:00) in space (linearNDFast)
12:52:37 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:37 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-07 15:00:00) in space (linearNDFast)
12:52:37 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:37 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-07 12:00:00, weight 0.71) and
after (2024-11-07 15:00:00, weight 0.29) in time
12:52:37 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:37 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:37 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:37 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:52:37 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:37 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:52:37 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:37 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:37 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:52:37 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:52:37 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-07 12:00:00 (before)
2024-11-07 15:00:00 (after)
12:52:37 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_wind to retrieve x_wind
12:52:40 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_wind to retrieve y_wind
12:52:43 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:52:43 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:52:43 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:52:43 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 720x361x1) for time after (2024-11-07 15:00:00)
12:52:43 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-07 12:00:00) in space (linearNDFast)
12:52:43 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:43 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-07 15:00:00) in space (linearNDFast)
12:52:43 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:43 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-07 12:00:00, weight 0.71) and
after (2024-11-07 15:00:00, weight 0.29) in time
12:52:43 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:43 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:43 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:52:43 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:52:43 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:52:43 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:52:43 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:52:43 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:52:43 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:52:43 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.296907 (min) 0.0620721 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0524632 (min) 0.190553 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.272472 (min) 1.16501 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.09239 (min) -0.562526 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:52:43 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.020429, mean: 0.038880, max: 0.109527
12:52:43 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:52:43 DEBUG opendrift.models.physics_methods:1061: min: 0.778666, mean: 1.071661, max: 1.802977
12:52:43 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 0.778666, mean: 1.071661, max: 1.802977
12:52:43 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:52:43 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:52:43 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:52:43 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:52:43 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:52:43 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:52:43 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:52:43 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:52:43 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:52:43 DEBUG opendrift.models.physics_methods:1061: min: 0.778666, mean: 1.071661, max: 1.802977
12:52:43 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:52:43 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.007450, dN_50: 0.000585
12:52:43 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:52:43 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.0016106627956630703
12:52:43 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:52:43 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:52:43 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:52:43 DEBUG opendrift.models.physics_methods:915: Advecting 3000 of 6000 elements above 0.100m with wind-sheared ocean current (0.027339 m/s - 0.055346 m/s)
12:52:43 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:52:43 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.001283620157565291 and 0.4278625174534719 m/s
12:52:43 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:52:43 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:52:43 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:52:43 INFO opendrift.models.basemodel:2038: 2024-11-07 13:51:18.915878 - step 26 of 120 - 6000 active elements (0 deactivated)
12:52:43 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:52:43 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:52:43 DEBUG opendrift.models.basemodel:2057: 34.92413050105404 <- latitude -> 35.08422394872791
12:52:43 DEBUG opendrift.models.basemodel:2062: 23.461214879674014 <- longitude -> 23.61479718673402
12:52:43 DEBUG opendrift.models.basemodel:2067: -131.2979046965102 <- z -> 0.0
12:52:43 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:52:43 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:43 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:52:43 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:43 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:52:43 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:43 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:43 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:52:43 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:52:43 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:43 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:43 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:43 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:52:43 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:43 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:52:43 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:43 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:43 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:52:43 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:52:43 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:52:43 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:43 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:43 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:43 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:52:43 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:43 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:52:43 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:43 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:43 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:52:43 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:52:43 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-07 12:00:00 (before)
2024-11-07 15:00:00 (after)
12:52:43 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-07 12:00:00) in space (linearNDFast)
12:52:43 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:43 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-07 15:00:00) in space (linearNDFast)
12:52:43 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:43 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-07 12:00:00, weight 0.38) and
after (2024-11-07 15:00:00, weight 0.62) in time
12:52:43 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:43 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:43 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:43 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:52:43 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:43 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:52:43 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:43 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:43 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:52:43 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:52:43 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-07 12:00:00 (before)
2024-11-07 15:00:00 (after)
12:52:43 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-07 12:00:00) in space (linearNDFast)
12:52:43 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:43 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-07 15:00:00) in space (linearNDFast)
12:52:43 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:43 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-07 12:00:00, weight 0.38) and
after (2024-11-07 15:00:00, weight 0.62) in time
12:52:43 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:43 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:43 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:52:43 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:52:43 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:52:43 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:52:43 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:52:43 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:52:43 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:52:43 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.324834 (min) 0.0453316 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0436764 (min) 0.19731 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.834939 (min) 0.592164 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: y_wind: -1.90878 (min) -0.364474 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:52:43 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.007735, mean: 0.014646, max: 0.106778
12:52:43 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:52:43 DEBUG opendrift.models.physics_methods:1061: min: 0.479129, mean: 0.652870, max: 1.780204
12:52:43 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 0.479129, mean: 0.652870, max: 1.780204
12:52:43 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:52:43 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:52:43 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:52:43 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:52:43 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:52:43 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:52:43 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:52:43 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:52:43 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:52:43 DEBUG opendrift.models.physics_methods:1061: min: 0.479129, mean: 0.652870, max: 1.780204
12:52:43 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:52:43 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.013402, dN_50: 0.001052
12:52:43 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:52:43 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.0015703373645609222
12:52:43 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:52:43 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:52:43 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:52:43 DEBUG opendrift.models.physics_methods:915: Advecting 3000 of 6000 elements above 0.100m with wind-sheared ocean current (0.016822 m/s - 0.056243 m/s)
12:52:43 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:52:43 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0002914579376952525 and 0.431620491407613 m/s
12:52:43 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:52:43 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:52:43 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:52:43 INFO opendrift.models.basemodel:2038: 2024-11-07 14:51:18.915878 - step 27 of 120 - 6000 active elements (0 deactivated)
12:52:43 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:52:43 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:52:43 DEBUG opendrift.models.basemodel:2057: 34.92969715467056 <- latitude -> 35.08909191272835
12:52:43 DEBUG opendrift.models.basemodel:2062: 23.45436886879702 <- longitude -> 23.618461502868808
12:52:43 DEBUG opendrift.models.basemodel:2067: -130.54408642487167 <- z -> 0.0
12:52:43 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:52:43 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:43 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:52:43 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:43 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:52:43 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:43 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:43 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:52:43 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:52:43 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:43 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:43 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:43 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:52:43 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:43 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:52:43 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:43 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:43 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:52:43 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:52:43 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:52:43 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:43 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:43 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:43 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:52:43 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:43 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:52:43 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:43 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:43 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:52:43 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:52:43 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-07 12:00:00 (before)
2024-11-07 15:00:00 (after)
12:52:43 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-07 12:00:00) in space (linearNDFast)
12:52:43 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:43 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-07 15:00:00) in space (linearNDFast)
12:52:43 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:43 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-07 12:00:00, weight 0.05) and
after (2024-11-07 15:00:00, weight 0.95) in time
12:52:43 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:43 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:43 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:43 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:52:43 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:43 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:52:43 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:43 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:43 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:52:43 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:52:43 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-07 12:00:00 (before)
2024-11-07 15:00:00 (after)
12:52:43 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-07 12:00:00) in space (linearNDFast)
12:52:43 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:43 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-07 15:00:00) in space (linearNDFast)
12:52:43 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:43 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-07 12:00:00, weight 0.05) and
after (2024-11-07 15:00:00, weight 0.95) in time
12:52:43 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:43 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:43 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:52:43 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:52:43 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:52:43 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:52:43 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:52:43 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:52:43 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:52:43 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.368277 (min) 0.0279908 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0334878 (min) 0.202533 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.32777 (min) 0.0577514 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: y_wind: -1.70727 (min) -0.174775 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:52:43 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:52:44 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000751, mean: 0.006639, max: 0.115072
12:52:44 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:52:44 DEBUG opendrift.models.physics_methods:1061: min: 0.149340, mean: 0.397076, max: 1.848057
12:52:44 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 0.149340, mean: 0.397076, max: 1.848057
12:52:44 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:52:44 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:52:44 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:52:44 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:52:44 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:52:44 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:52:44 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:52:44 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:52:44 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:52:44 DEBUG opendrift.models.physics_methods:1061: min: 0.149340, mean: 0.397076, max: 1.848057
12:52:44 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:52:44 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.030557, dN_50: 0.002398
12:52:44 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:52:44 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.0016919991695932917
12:52:44 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:52:44 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:52:44 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:52:44 DEBUG opendrift.models.physics_methods:915: Advecting 3000 of 6000 elements above 0.100m with wind-sheared ocean current (0.005243 m/s - 0.062176 m/s)
12:52:44 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:52:44 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0022859984016221605 and 0.49811742001960796 m/s
12:52:44 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:52:44 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:52:44 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:52:44 INFO opendrift.models.basemodel:2038: 2024-11-07 15:51:18.915878 - step 28 of 120 - 6000 active elements (0 deactivated)
12:52:44 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:52:44 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:52:44 DEBUG opendrift.models.basemodel:2057: 34.92869950492819 <- latitude -> 35.092242514378256
12:52:44 DEBUG opendrift.models.basemodel:2062: 23.442696905578646 <- longitude -> 23.6154205684722
12:52:44 DEBUG opendrift.models.basemodel:2067: -129.45275017087042 <- z -> 0.0
12:52:44 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:52:44 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:44 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:52:44 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:44 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:52:44 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:44 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:44 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:52:44 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:52:44 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:44 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:44 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:44 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:52:44 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:44 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:52:44 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:44 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:44 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:52:44 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:52:44 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:52:44 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:44 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:44 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:44 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:52:44 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:44 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:52:44 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:44 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:44 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:52:44 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:52:44 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-07 15:00:00 (before)
2024-11-07 18:00:00 (after)
12:52:44 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
12:52:44 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
12:52:44 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:52:44 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:52:44 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:52:44 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 10x12x23) for time after (2024-11-07 18:00:00)
12:52:44 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-07 15:00:00) in space (linearNDFast)
12:52:44 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:44 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-07 18:00:00) in space (linearNDFast)
12:52:44 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:44 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-07 15:00:00, weight 0.71) and
after (2024-11-07 18:00:00, weight 0.29) in time
12:52:44 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:44 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:44 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:44 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:52:44 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:44 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:52:44 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:44 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:44 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:52:44 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:52:44 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-07 15:00:00 (before)
2024-11-07 18:00:00 (after)
12:52:44 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_wind to retrieve x_wind
12:52:47 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_wind to retrieve y_wind
12:52:50 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:52:50 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:52:50 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:52:50 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 720x361x1) for time after (2024-11-07 18:00:00)
12:52:50 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-07 15:00:00) in space (linearNDFast)
12:52:50 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:50 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-07 18:00:00) in space (linearNDFast)
12:52:50 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:50 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-07 15:00:00, weight 0.71) and
after (2024-11-07 18:00:00, weight 0.29) in time
12:52:50 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:50 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:50 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:52:50 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:52:50 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:52:50 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:52:50 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:52:50 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:52:50 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:52:50 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.409398 (min) 0.027701 (max)
12:52:50 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.012976 (min) 0.206736 (max)
12:52:50 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.79684 (min) -0.250611 (max)
12:52:50 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.06509 (min) -0.488883 (max)
12:52:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:52:50 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:52:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:52:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:52:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:52:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:52:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:52:50 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:52:50 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:52:50 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:52:50 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:52:50 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:52:50 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:52:50 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:52:50 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:52:50 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:52:50 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:52:50 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.009540, mean: 0.023281, max: 0.184226
12:52:50 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:52:50 DEBUG opendrift.models.physics_methods:1061: min: 0.532126, mean: 0.801348, max: 2.338325
12:52:50 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 0.532126, mean: 0.801348, max: 2.338325
12:52:50 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:52:50 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:52:50 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:52:50 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:52:50 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:52:50 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:52:50 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:52:50 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:52:50 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:52:50 DEBUG opendrift.models.physics_methods:1061: min: 0.532126, mean: 0.801348, max: 2.338326
12:52:50 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:52:50 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.011299, dN_50: 0.000887
12:52:50 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:52:50 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.0027062926537304017
12:52:50 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:52:50 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:52:50 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:52:50 DEBUG opendrift.models.physics_methods:915: Advecting 3000 of 6000 elements above 0.100m with wind-sheared ocean current (0.019194 m/s - 0.082097 m/s)
12:52:50 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:52:50 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0020135087636341314 and 0.429206887794728 m/s
12:52:50 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:52:50 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:52:50 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:52:50 INFO opendrift.models.basemodel:2038: 2024-11-07 16:51:18.915878 - step 29 of 120 - 6000 active elements (0 deactivated)
12:52:50 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:52:50 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:52:50 DEBUG opendrift.models.basemodel:2057: 34.928161501809974 <- latitude -> 35.09534677982401
12:52:50 DEBUG opendrift.models.basemodel:2062: 23.430989976535503 <- longitude -> 23.613729474983103
12:52:50 DEBUG opendrift.models.basemodel:2067: -128.62222041879298 <- z -> 0.0
12:52:50 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:52:50 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:50 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:52:50 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:50 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:52:50 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:50 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:50 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:52:50 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:52:50 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:50 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:50 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:50 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:52:50 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:50 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:52:50 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:50 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:50 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:52:50 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:52:50 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:52:50 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:50 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:50 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:50 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:52:50 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:50 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:52:50 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:50 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:50 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:52:50 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:52:50 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-07 15:00:00 (before)
2024-11-07 18:00:00 (after)
12:52:50 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-07 15:00:00) in space (linearNDFast)
12:52:50 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:50 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-07 18:00:00) in space (linearNDFast)
12:52:50 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:50 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-07 15:00:00, weight 0.38) and
after (2024-11-07 18:00:00, weight 0.62) in time
12:52:50 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:50 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:50 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:50 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:52:50 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:50 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:52:50 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:50 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:50 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:52:50 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:52:50 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-07 15:00:00 (before)
2024-11-07 18:00:00 (after)
12:52:50 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-07 15:00:00) in space (linearNDFast)
12:52:50 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:50 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-07 18:00:00) in space (linearNDFast)
12:52:50 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:50 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-07 15:00:00, weight 0.38) and
after (2024-11-07 18:00:00, weight 0.62) in time
12:52:50 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:50 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:50 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:52:50 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:52:50 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:52:50 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:52:50 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:52:50 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:52:50 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:52:50 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.401051 (min) 0.0202834 (max)
12:52:50 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.00365749 (min) 0.199483 (max)
12:52:50 DEBUG opendrift.models.basemodel.environment:905: x_wind: -2.32247 (min) -0.601902 (max)
12:52:50 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.41898 (min) -0.838632 (max)
12:52:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:52:50 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:52:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:52:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:52:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:52:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:52:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:52:50 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:52:50 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:52:50 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:52:50 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:52:50 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:52:50 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:52:50 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:52:50 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:52:50 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:52:50 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:52:50 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.026213, mean: 0.056858, max: 0.276635
12:52:50 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:52:50 DEBUG opendrift.models.physics_methods:1061: min: 0.882047, mean: 1.279837, max: 2.865388
12:52:50 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 0.882047, mean: 1.279837, max: 2.865388
12:52:50 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:52:50 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:52:50 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:52:50 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:52:50 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:52:50 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:52:50 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:52:50 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:52:50 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:52:50 DEBUG opendrift.models.physics_methods:1061: min: 0.882047, mean: 1.279837, max: 2.865388
12:52:50 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:52:50 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.006286, dN_50: 0.000493
12:52:50 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:52:50 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.004061686394159293
12:52:50 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:52:50 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:52:50 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:52:51 DEBUG opendrift.models.physics_methods:915: Advecting 3000 of 6000 elements above 0.100m with wind-sheared ocean current (0.032296 m/s - 0.100602 m/s)
12:52:51 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:52:51 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.002351111542610649 and 0.5013286994422927 m/s
12:52:51 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:52:51 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:52:51 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:52:51 INFO opendrift.models.basemodel:2038: 2024-11-07 17:51:18.915878 - step 30 of 120 - 6000 active elements (0 deactivated)
12:52:51 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:52:51 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:52:51 DEBUG opendrift.models.basemodel:2057: 34.930023279944734 <- latitude -> 35.092278329608654
12:52:51 DEBUG opendrift.models.basemodel:2062: 23.42076397214454 <- longitude -> 23.607178061869654
12:52:51 DEBUG opendrift.models.basemodel:2067: -127.36632072018037 <- z -> 0.0
12:52:51 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:52:51 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:51 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:52:51 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:51 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:52:51 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:51 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:51 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:52:51 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:52:51 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:51 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:51 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:51 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:52:51 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:51 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:52:51 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:51 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:51 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:52:51 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:52:51 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:52:51 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:51 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:51 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:51 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:52:51 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:51 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:52:51 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:51 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:51 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:52:51 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:52:51 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-07 15:00:00 (before)
2024-11-07 18:00:00 (after)
12:52:51 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-07 15:00:00) in space (linearNDFast)
12:52:51 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:51 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-07 18:00:00) in space (linearNDFast)
12:52:51 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:51 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-07 15:00:00, weight 0.05) and
after (2024-11-07 18:00:00, weight 0.95) in time
12:52:51 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:51 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:51 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:51 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:52:51 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:51 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:52:51 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:51 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:51 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:52:51 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:52:51 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-07 15:00:00 (before)
2024-11-07 18:00:00 (after)
12:52:51 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-07 15:00:00) in space (linearNDFast)
12:52:51 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:51 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-07 18:00:00) in space (linearNDFast)
12:52:51 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:51 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-07 15:00:00, weight 0.05) and
after (2024-11-07 18:00:00, weight 0.95) in time
12:52:51 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:51 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:51 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:52:51 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:52:51 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:52:51 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:52:51 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:52:51 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:52:51 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:52:51 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.460162 (min) 0.0211085 (max)
12:52:51 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.00967461 (min) 0.197854 (max)
12:52:51 DEBUG opendrift.models.basemodel.environment:905: x_wind: -2.96435 (min) -0.930517 (max)
12:52:51 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.01868 (min) -1.10347 (max)
12:52:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:52:51 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:52:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:52:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:52:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:52:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:52:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:52:51 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:52:51 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:52:51 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:52:51 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:52:51 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:52:51 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:52:51 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:52:51 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:52:51 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:52:51 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:52:51 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.051254, mean: 0.105484, max: 0.440336
12:52:51 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:52:51 DEBUG opendrift.models.physics_methods:1061: min: 1.233375, mean: 1.755152, max: 3.615112
12:52:51 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.233375, mean: 1.755152, max: 3.615112
12:52:51 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:52:51 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:52:51 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:52:51 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:52:51 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:52:51 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:52:51 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:52:51 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:52:51 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:52:51 DEBUG opendrift.models.physics_methods:1061: min: 1.233375, mean: 1.755152, max: 3.615112
12:52:51 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:52:51 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.004335, dN_50: 0.000340
12:52:51 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:52:51 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.006462731868957457
12:52:51 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:52:51 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:52:51 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:52:51 DEBUG opendrift.models.physics_methods:915: Advecting 3000 of 6000 elements above 0.100m with wind-sheared ocean current (0.045640 m/s - 0.126925 m/s)
12:52:51 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:52:51 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0006626867173019304 and 0.46358617690543624 m/s
12:52:51 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:52:51 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:52:51 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:52:51 INFO opendrift.models.basemodel:2038: 2024-11-07 18:51:18.915878 - step 31 of 120 - 6000 active elements (0 deactivated)
12:52:51 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:52:51 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:52:51 DEBUG opendrift.models.basemodel:2057: 34.93506144701366 <- latitude -> 35.09512181661593
12:52:51 DEBUG opendrift.models.basemodel:2062: 23.401572697171577 <- longitude -> 23.60180934725091
12:52:51 DEBUG opendrift.models.basemodel:2067: -126.23638025817564 <- z -> 0.0
12:52:51 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:52:51 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:51 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:52:51 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:51 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:52:51 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:51 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:51 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:52:51 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:52:51 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:51 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:51 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:51 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:52:51 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:51 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:52:51 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:51 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:51 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:52:51 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:52:51 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:52:51 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:51 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:51 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:51 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:52:51 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:51 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:52:51 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:51 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:51 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:52:51 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:52:51 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-07 18:00:00 (before)
2024-11-07 21:00:00 (after)
12:52:51 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
12:52:51 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
12:52:51 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:52:51 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:52:51 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:52:51 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 11x12x23) for time after (2024-11-07 21:00:00)
12:52:51 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-07 18:00:00) in space (linearNDFast)
12:52:51 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:51 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-07 21:00:00) in space (linearNDFast)
12:52:51 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:51 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-07 18:00:00, weight 0.71) and
after (2024-11-07 21:00:00, weight 0.29) in time
12:52:51 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:51 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:51 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:51 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:52:51 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:51 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:52:51 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:51 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:51 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:52:51 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:52:51 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-07 18:00:00 (before)
2024-11-07 21:00:00 (after)
12:52:51 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_wind to retrieve x_wind
12:52:54 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_wind to retrieve y_wind
12:52:57 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:52:57 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:52:57 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:52:57 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 720x361x1) for time after (2024-11-07 21:00:00)
12:52:57 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-07 18:00:00) in space (linearNDFast)
12:52:57 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:57 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-07 21:00:00) in space (linearNDFast)
12:52:57 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:57 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-07 18:00:00, weight 0.71) and
after (2024-11-07 21:00:00, weight 0.29) in time
12:52:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:57 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:52:57 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:52:57 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:52:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:52:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:52:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:52:57 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:52:57 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.448494 (min) 0.0202736 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0390426 (min) 0.208286 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: x_wind: -3.0043 (min) -0.849746 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.40355 (min) -1.41903 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:52:57 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.069845, mean: 0.129285, max: 0.507005
12:52:57 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:52:57 DEBUG opendrift.models.physics_methods:1061: min: 1.439780, mean: 1.944614, max: 3.879143
12:52:57 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.439780, mean: 1.944614, max: 3.879143
12:52:57 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:52:57 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:52:57 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:52:57 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:52:57 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:52:57 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:52:57 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:52:57 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:52:57 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:52:57 DEBUG opendrift.models.physics_methods:1061: min: 1.439780, mean: 1.944615, max: 3.879143
12:52:57 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:52:57 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.003868, dN_50: 0.000304
12:52:57 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:52:57 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.007440587132650421
12:52:57 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:52:57 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:52:57 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:52:57 DEBUG opendrift.models.physics_methods:915: Advecting 3000 of 6000 elements above 0.100m with wind-sheared ocean current (0.052211 m/s - 0.136195 m/s)
12:52:57 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:52:57 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0009598150619966209 and 0.4716859862045055 m/s
12:52:57 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:52:57 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:52:57 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:52:57 INFO opendrift.models.basemodel:2038: 2024-11-07 19:51:18.915878 - step 32 of 120 - 6000 active elements (0 deactivated)
12:52:57 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:52:57 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:52:57 DEBUG opendrift.models.basemodel:2057: 34.93387289229711 <- latitude -> 35.098878329210436
12:52:57 DEBUG opendrift.models.basemodel:2062: 23.379330716395906 <- longitude -> 23.596054491704848
12:52:57 DEBUG opendrift.models.basemodel:2067: -125.72961779610344 <- z -> 0.0
12:52:57 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:52:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:52:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:52:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:52:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:52:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:52:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:52:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:52:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:52:57 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:52:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:52:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:52:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:52:57 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:52:57 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-07 18:00:00 (before)
2024-11-07 21:00:00 (after)
12:52:57 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-07 18:00:00) in space (linearNDFast)
12:52:57 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:57 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-07 21:00:00) in space (linearNDFast)
12:52:57 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:57 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-07 18:00:00, weight 0.38) and
after (2024-11-07 21:00:00, weight 0.62) in time
12:52:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:52:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:52:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:52:57 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:52:57 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-07 18:00:00 (before)
2024-11-07 21:00:00 (after)
12:52:57 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-07 18:00:00) in space (linearNDFast)
12:52:57 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:57 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-07 21:00:00) in space (linearNDFast)
12:52:57 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:57 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-07 18:00:00, weight 0.38) and
after (2024-11-07 21:00:00, weight 0.62) in time
12:52:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:57 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:52:57 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:52:57 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:52:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:52:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:52:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:52:57 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:52:57 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.45042 (min) 0.0441153 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0816712 (min) 0.216248 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: x_wind: -3.04666 (min) -0.691208 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.80242 (min) -1.71645 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:52:57 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.088305, mean: 0.155160, max: 0.584017
12:52:57 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:52:57 DEBUG opendrift.models.physics_methods:1061: min: 1.618909, mean: 2.131501, max: 4.163344
12:52:57 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.618909, mean: 2.131501, max: 4.163344
12:52:57 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:52:57 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:52:57 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:52:57 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:52:57 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:52:57 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:52:57 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:52:57 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:52:57 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:52:57 DEBUG opendrift.models.physics_methods:1061: min: 1.618909, mean: 2.131501, max: 4.163344
12:52:57 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:52:57 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.003500, dN_50: 0.000275
12:52:57 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:52:57 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.0085701377018502
12:52:57 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:52:57 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:52:57 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:52:57 DEBUG opendrift.models.physics_methods:915: Advecting 3000 of 6000 elements above 0.100m with wind-sheared ocean current (0.058037 m/s - 0.146173 m/s)
12:52:57 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:52:57 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0017010292993552808 and 0.5117220625509399 m/s
12:52:57 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:52:57 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:52:57 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:52:57 INFO opendrift.models.basemodel:2038: 2024-11-07 20:51:18.915878 - step 33 of 120 - 6000 active elements (0 deactivated)
12:52:57 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:52:57 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:52:57 DEBUG opendrift.models.basemodel:2057: 34.93727870622 <- latitude -> 35.1020442653734
12:52:57 DEBUG opendrift.models.basemodel:2062: 23.361825587003178 <- longitude -> 23.596342653723323
12:52:57 DEBUG opendrift.models.basemodel:2067: -124.82022242728782 <- z -> 0.0
12:52:57 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:52:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:52:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:52:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:52:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:52:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:52:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:52:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:52:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:52:57 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:52:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:52:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:52:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:52:57 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:52:57 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-07 18:00:00 (before)
2024-11-07 21:00:00 (after)
12:52:57 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-07 18:00:00) in space (linearNDFast)
12:52:57 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:57 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-07 21:00:00) in space (linearNDFast)
12:52:57 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:57 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-07 18:00:00, weight 0.05) and
after (2024-11-07 21:00:00, weight 0.95) in time
12:52:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:52:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:52:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:52:57 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:52:57 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-07 18:00:00 (before)
2024-11-07 21:00:00 (after)
12:52:57 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-07 18:00:00) in space (linearNDFast)
12:52:57 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:57 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-07 21:00:00) in space (linearNDFast)
12:52:57 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:57 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-07 18:00:00, weight 0.05) and
after (2024-11-07 21:00:00, weight 0.95) in time
12:52:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:57 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:52:57 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:52:57 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:52:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:52:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:52:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:52:57 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:52:57 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.444265 (min) 0.0546537 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0968213 (min) 0.225449 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: x_wind: -3.04989 (min) -0.520462 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: y_wind: -4.09158 (min) -2.02996 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:52:57 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:52:57 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.113531, mean: 0.188807, max: 0.640653
12:52:57 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:52:57 DEBUG opendrift.models.physics_methods:1061: min: 1.835637, mean: 2.352952, max: 4.360548
12:52:57 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.835637, mean: 2.352952, max: 4.360548
12:52:57 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:52:57 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:52:57 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:52:57 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:52:57 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:52:57 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:52:57 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:52:57 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:52:57 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:52:57 DEBUG opendrift.models.physics_methods:1061: min: 1.835637, mean: 2.352952, max: 4.360548
12:52:57 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:52:57 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.003147, dN_50: 0.000247
12:52:57 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:52:57 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.00940083688907976
12:52:57 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:52:57 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:52:57 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:52:58 DEBUG opendrift.models.physics_methods:915: Advecting 3000 of 6000 elements above 0.100m with wind-sheared ocean current (0.064955 m/s - 0.153096 m/s)
12:52:58 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:52:58 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0018283509639090813 and 0.4167800170994464 m/s
12:52:58 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:52:58 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:52:58 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:52:58 INFO opendrift.models.basemodel:2038: 2024-11-07 21:51:18.915878 - step 34 of 120 - 6000 active elements (0 deactivated)
12:52:58 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:52:58 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:52:58 DEBUG opendrift.models.basemodel:2057: 34.94042502739797 <- latitude -> 35.11045409593698
12:52:58 DEBUG opendrift.models.basemodel:2062: 23.34755617556419 <- longitude -> 23.59785121586893
12:52:58 DEBUG opendrift.models.basemodel:2067: -123.53796077031197 <- z -> 0.0
12:52:58 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:52:58 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:58 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:52:58 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:58 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:52:58 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:58 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:58 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:52:58 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:52:58 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:58 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:58 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:58 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:52:58 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:58 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:52:58 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:58 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:58 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:52:58 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:52:58 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:52:58 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:58 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:58 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:58 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:52:58 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:58 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:52:58 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:58 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:58 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:52:58 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:52:58 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-07 21:00:00 (before)
2024-11-08 00:00:00 (after)
12:52:58 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
12:52:58 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
12:52:58 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:52:58 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:52:58 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:52:58 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 11x12x22) for time after (2024-11-08 00:00:00)
12:52:58 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-07 21:00:00) in space (linearNDFast)
12:52:58 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:58 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-08 00:00:00) in space (linearNDFast)
12:52:58 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:52:58 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-07 21:00:00, weight 0.71) and
after (2024-11-08 00:00:00, weight 0.29) in time
12:52:58 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:52:58 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:52:58 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:52:58 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:52:58 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:52:58 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:52:58 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:52:58 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:52:58 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:52:58 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:52:58 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-07 21:00:00 (before)
2024-11-08 00:00:00 (after)
12:52:58 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_wind to retrieve x_wind
12:53:00 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_wind to retrieve y_wind
12:53:02 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:53:02 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:53:02 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:53:02 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 720x361x1) for time after (2024-11-08 00:00:00)
12:53:02 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-07 21:00:00) in space (linearNDFast)
12:53:02 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:02 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-08 00:00:00) in space (linearNDFast)
12:53:02 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:02 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-07 21:00:00, weight 0.71) and
after (2024-11-08 00:00:00, weight 0.29) in time
12:53:02 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:02 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:02 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:53:02 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:53:02 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:53:02 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:53:02 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:53:02 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:53:02 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:53:02 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.472723 (min) 0.0704075 (max)
12:53:02 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0696212 (min) 0.225616 (max)
12:53:02 DEBUG opendrift.models.basemodel.environment:905: x_wind: -3.05594 (min) -0.414681 (max)
12:53:02 DEBUG opendrift.models.basemodel.environment:905: y_wind: -4.4315 (min) -2.21209 (max)
12:53:02 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:53:02 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:53:02 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:53:02 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:53:02 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:53:02 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:53:02 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:53:02 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:53:02 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:53:02 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:53:02 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:53:02 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:53:02 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:53:02 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:53:02 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:53:02 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:53:02 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:53:02 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.131099, mean: 0.215865, max: 0.712833
12:53:02 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:53:02 DEBUG opendrift.models.physics_methods:1061: min: 1.972558, mean: 2.513913, max: 4.599637
12:53:02 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.972558, mean: 2.513913, max: 4.599637
12:53:02 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:53:02 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:53:02 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:53:02 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:53:02 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:53:02 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:53:02 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:53:02 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:53:02 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:53:02 DEBUG opendrift.models.physics_methods:1061: min: 1.972558, mean: 2.513913, max: 4.599637
12:53:02 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:53:02 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.002948, dN_50: 0.000231
12:53:02 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:53:02 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.010459524363787833
12:53:02 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:53:02 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:53:02 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:53:02 DEBUG opendrift.models.physics_methods:915: Advecting 3000 of 6000 elements above 0.100m with wind-sheared ocean current (0.069867 m/s - 0.161491 m/s)
12:53:02 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:53:02 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0019520467603742014 and 0.46510201652138533 m/s
12:53:02 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:53:02 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:53:02 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:53:02 INFO opendrift.models.basemodel:2038: 2024-11-07 22:51:18.915878 - step 35 of 120 - 6000 active elements (0 deactivated)
12:53:02 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:53:02 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:53:02 DEBUG opendrift.models.basemodel:2057: 34.94475556562942 <- latitude -> 35.12120754845366
12:53:02 DEBUG opendrift.models.basemodel:2062: 23.333333035619162 <- longitude -> 23.604182455981988
12:53:02 DEBUG opendrift.models.basemodel:2067: -122.69419317006043 <- z -> 0.0
12:53:02 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:53:02 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:02 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:53:02 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:02 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:53:02 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:02 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:02 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:53:02 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:02 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:02 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:02 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:02 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:53:02 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:02 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:53:02 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:02 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:02 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:53:02 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:02 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:53:02 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:02 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:02 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:02 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:53:02 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:02 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:53:02 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:02 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:02 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:53:02 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:02 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-07 21:00:00 (before)
2024-11-08 00:00:00 (after)
12:53:02 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-07 21:00:00) in space (linearNDFast)
12:53:02 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:02 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-08 00:00:00) in space (linearNDFast)
12:53:02 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:02 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-07 21:00:00, weight 0.38) and
after (2024-11-08 00:00:00, weight 0.62) in time
12:53:02 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:02 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:02 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:02 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:53:02 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:02 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:53:02 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:02 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:02 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:53:02 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:02 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-07 21:00:00 (before)
2024-11-08 00:00:00 (after)
12:53:02 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-07 21:00:00) in space (linearNDFast)
12:53:02 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:02 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-08 00:00:00) in space (linearNDFast)
12:53:02 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:02 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-07 21:00:00, weight 0.38) and
after (2024-11-08 00:00:00, weight 0.62) in time
12:53:02 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:02 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:02 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:53:02 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:53:02 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:53:02 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:53:02 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:53:02 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:53:02 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:53:02 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.478086 (min) 0.0886078 (max)
12:53:02 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0389142 (min) 0.221087 (max)
12:53:02 DEBUG opendrift.models.basemodel.environment:905: x_wind: -3.04239 (min) -0.329703 (max)
12:53:02 DEBUG opendrift.models.basemodel.environment:905: y_wind: -4.75009 (min) -2.3584 (max)
12:53:02 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:53:02 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:53:02 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:53:02 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:53:02 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:53:02 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:53:02 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:53:02 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:53:02 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:53:02 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:53:02 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:53:02 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:53:02 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:53:02 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:53:02 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:53:02 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:53:02 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:53:02 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.147669, mean: 0.244543, max: 0.782758
12:53:02 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:53:02 DEBUG opendrift.models.physics_methods:1061: min: 2.093507, mean: 2.672906, max: 4.819958
12:53:02 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.093507, mean: 2.672906, max: 4.819958
12:53:02 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:53:02 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:53:02 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:53:02 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:53:02 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:53:02 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:53:02 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:53:02 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:53:02 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:53:02 DEBUG opendrift.models.physics_methods:1061: min: 2.093507, mean: 2.672906, max: 4.819958
12:53:02 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:53:02 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.002783, dN_50: 0.000218
12:53:03 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:53:03 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.011485123943682368
12:53:03 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:53:03 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:53:03 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:53:03 DEBUG opendrift.models.physics_methods:915: Advecting 3000 of 6000 elements above 0.100m with wind-sheared ocean current (0.073676 m/s - 0.169226 m/s)
12:53:03 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:53:03 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0029872099773988617 and 0.4788246250206879 m/s
12:53:03 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:53:03 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:53:03 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:53:03 INFO opendrift.models.basemodel:2038: 2024-11-07 23:51:18.915878 - step 36 of 120 - 6000 active elements (0 deactivated)
12:53:03 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:53:03 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:53:03 DEBUG opendrift.models.basemodel:2057: 34.94160384108324 <- latitude -> 35.13057853284105
12:53:03 DEBUG opendrift.models.basemodel:2062: 23.323717524368327 <- longitude -> 23.607385510280515
12:53:03 DEBUG opendrift.models.basemodel:2067: -121.70304318020246 <- z -> 0.0
12:53:03 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:53:03 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:03 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:53:03 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:03 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:53:03 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:03 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:03 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:53:03 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:03 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:03 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:03 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:03 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:53:03 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:03 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:53:03 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:03 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:03 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:53:03 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:03 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:53:03 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:03 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:03 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:03 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:53:03 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:03 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:53:03 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:03 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:03 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:53:03 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:03 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-07 21:00:00 (before)
2024-11-08 00:00:00 (after)
12:53:03 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-07 21:00:00) in space (linearNDFast)
12:53:03 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:03 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-08 00:00:00) in space (linearNDFast)
12:53:03 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:03 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-07 21:00:00, weight 0.05) and
after (2024-11-08 00:00:00, weight 0.95) in time
12:53:03 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:03 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:03 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:03 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:53:03 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:03 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:53:03 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:03 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:03 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:53:03 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:03 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-07 21:00:00 (before)
2024-11-08 00:00:00 (after)
12:53:03 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-07 21:00:00) in space (linearNDFast)
12:53:03 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:03 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-08 00:00:00) in space (linearNDFast)
12:53:03 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:03 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-07 21:00:00, weight 0.05) and
after (2024-11-08 00:00:00, weight 0.95) in time
12:53:03 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:03 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:03 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:53:03 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:53:03 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:53:03 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:53:03 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:53:03 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:53:03 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:53:03 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.520921 (min) 0.108833 (max)
12:53:03 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0178334 (min) 0.217847 (max)
12:53:03 DEBUG opendrift.models.basemodel.environment:905: x_wind: -3.01351 (min) -0.246862 (max)
12:53:03 DEBUG opendrift.models.basemodel.environment:905: y_wind: -5.03606 (min) -2.50159 (max)
12:53:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:53:03 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:53:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:53:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:53:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:53:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:53:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:53:03 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:53:03 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:53:03 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:53:03 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:53:03 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:53:03 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:53:03 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:53:03 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:53:03 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:53:03 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:53:03 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.162067, mean: 0.276884, max: 0.847301
12:53:03 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:53:03 DEBUG opendrift.models.physics_methods:1061: min: 2.193190, mean: 2.841271, max: 5.014739
12:53:03 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.193190, mean: 2.841271, max: 5.014739
12:53:03 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:53:03 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:53:03 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:53:03 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:53:03 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:53:03 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:53:03 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:53:03 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:53:03 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:53:03 DEBUG opendrift.models.physics_methods:1061: min: 2.193191, mean: 2.841271, max: 5.014740
12:53:03 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:53:03 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.002632, dN_50: 0.000207
12:53:03 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:53:03 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.012431794703847088
12:53:03 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:53:03 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:53:03 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:53:03 DEBUG opendrift.models.physics_methods:915: Advecting 3000 of 6000 elements above 0.100m with wind-sheared ocean current (0.077670 m/s - 0.176065 m/s)
12:53:03 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:53:03 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0014505774505427333 and 0.4498663622548493 m/s
12:53:03 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:53:03 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:53:03 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:53:03 INFO opendrift.models.basemodel:2038: 2024-11-08 00:51:18.915878 - step 37 of 120 - 6000 active elements (0 deactivated)
12:53:03 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:53:03 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:53:03 DEBUG opendrift.models.basemodel:2057: 34.941520436892795 <- latitude -> 35.12727866482797
12:53:03 DEBUG opendrift.models.basemodel:2062: 23.31244285493947 <- longitude -> 23.608442018666054
12:53:03 DEBUG opendrift.models.basemodel:2067: -121.03119987368329 <- z -> 0.0
12:53:03 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:53:03 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:03 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:53:03 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:03 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:53:03 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:03 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:03 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:53:03 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:03 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:03 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:03 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:03 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:53:03 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:03 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:53:03 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:03 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:03 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:53:03 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:03 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:53:03 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:03 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:03 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:03 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:53:03 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:03 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:53:03 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:03 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:03 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:53:03 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:03 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-08 00:00:00 (before)
2024-11-08 03:00:00 (after)
12:53:03 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
12:53:03 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
12:53:03 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:53:03 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:53:03 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:53:03 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
12:53:03 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 12x13x22) for time after (2024-11-08 03:00:00)
12:53:03 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-08 00:00:00) in space (linearNDFast)
12:53:03 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:03 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-08 03:00:00) in space (linearNDFast)
12:53:03 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:04 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-08 00:00:00, weight 0.71) and
after (2024-11-08 03:00:00, weight 0.29) in time
12:53:04 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:04 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:04 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:04 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:53:04 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:04 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:53:04 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:04 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:04 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:53:04 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:04 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-08 00:00:00 (before)
2024-11-08 03:00:00 (after)
12:53:04 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_wind to retrieve x_wind
12:53:07 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_wind to retrieve y_wind
12:53:09 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:53:09 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:53:09 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:53:09 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 720x361x1) for time after (2024-11-08 03:00:00)
12:53:09 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-08 00:00:00) in space (linearNDFast)
12:53:09 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:09 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-08 03:00:00) in space (linearNDFast)
12:53:09 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:09 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-08 00:00:00, weight 0.71) and
after (2024-11-08 03:00:00, weight 0.29) in time
12:53:09 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:09 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:09 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:53:09 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:53:09 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:53:09 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:53:09 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:53:09 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:53:09 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:53:09 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.46014 (min) 0.119319 (max)
12:53:09 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0130726 (min) 0.207684 (max)
12:53:09 DEBUG opendrift.models.basemodel.environment:905: x_wind: -3.30276 (min) -0.509493 (max)
12:53:09 DEBUG opendrift.models.basemodel.environment:905: y_wind: -5.41048 (min) -2.73766 (max)
12:53:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:53:09 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:53:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:53:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:53:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:53:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:53:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:53:09 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:53:09 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:53:09 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:53:09 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:53:09 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:53:09 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:53:09 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:53:09 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:53:09 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:53:09 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:53:09 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.198900, mean: 0.354051, max: 0.988465
12:53:09 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:53:09 DEBUG opendrift.models.physics_methods:1061: min: 2.429671, mean: 3.211768, max: 5.416395
12:53:09 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.429671, mean: 3.211768, max: 5.416395
12:53:09 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:53:09 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:53:09 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:53:09 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:53:09 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:53:09 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:53:09 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:53:09 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:53:09 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:53:09 DEBUG opendrift.models.physics_methods:1061: min: 2.429671, mean: 3.211768, max: 5.416395
12:53:09 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:53:09 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.002332, dN_50: 0.000183
12:53:09 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:53:09 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.014502295723213189
12:53:09 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:53:09 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:53:09 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:53:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 3000 surface elements
12:53:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2999 surface elements
12:53:09 DEBUG opendrift.models.physics_methods:915: Advecting 2998 of 6000 elements above 0.100m with wind-sheared ocean current (0.086435 m/s - 0.190167 m/s)
12:53:09 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:53:09 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0006054092429790289 and 0.4478466550348009 m/s
12:53:09 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:53:09 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:53:09 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:53:09 INFO opendrift.models.basemodel:2038: 2024-11-08 01:51:18.915878 - step 38 of 120 - 6000 active elements (0 deactivated)
12:53:09 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:53:09 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:53:09 DEBUG opendrift.models.basemodel:2057: 34.93888792562747 <- latitude -> 35.13361188701932
12:53:09 DEBUG opendrift.models.basemodel:2062: 23.300850721997637 <- longitude -> 23.615199295527226
12:53:09 DEBUG opendrift.models.basemodel:2067: -119.95185181105387 <- z -> 0.0
12:53:09 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:53:09 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:09 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:53:09 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:09 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:53:09 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:09 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:09 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:53:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:09 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:09 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:09 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:09 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:53:09 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:09 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:53:09 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:09 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:09 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:53:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:09 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:53:09 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:09 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:09 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:09 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:53:09 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:09 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:53:09 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:09 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:09 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:53:09 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:09 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-08 00:00:00 (before)
2024-11-08 03:00:00 (after)
12:53:09 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-08 00:00:00) in space (linearNDFast)
12:53:09 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:09 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-08 03:00:00) in space (linearNDFast)
12:53:09 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:09 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-08 00:00:00, weight 0.38) and
after (2024-11-08 03:00:00, weight 0.62) in time
12:53:09 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:09 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:09 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:09 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:53:09 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:09 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:53:09 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:09 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:09 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:53:09 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:09 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-08 00:00:00 (before)
2024-11-08 03:00:00 (after)
12:53:09 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-08 00:00:00) in space (linearNDFast)
12:53:09 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:09 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-08 03:00:00) in space (linearNDFast)
12:53:09 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:09 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-08 00:00:00, weight 0.38) and
after (2024-11-08 03:00:00, weight 0.62) in time
12:53:09 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:09 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:09 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:53:09 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:53:10 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:53:10 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:53:10 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:53:10 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:53:10 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:53:10 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.455315 (min) 0.122612 (max)
12:53:10 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0138241 (min) 0.195175 (max)
12:53:10 DEBUG opendrift.models.basemodel.environment:905: x_wind: -3.73575 (min) -0.82131 (max)
12:53:10 DEBUG opendrift.models.basemodel.environment:905: y_wind: -5.79233 (min) -2.99074 (max)
12:53:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:53:10 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:53:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:53:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:53:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:53:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:53:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:53:10 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:53:10 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:53:10 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:53:10 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:53:10 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:53:10 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:53:10 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:53:10 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:53:10 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:53:10 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:53:10 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.243495, mean: 0.449936, max: 1.165870
12:53:10 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:53:10 DEBUG opendrift.models.physics_methods:1061: min: 2.688279, mean: 3.620682, max: 5.882400
12:53:10 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.688279, mean: 3.620682, max: 5.882400
12:53:10 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:53:10 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:53:10 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:53:10 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:53:10 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:53:10 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:53:10 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:53:10 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:53:10 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:53:10 DEBUG opendrift.models.physics_methods:1061: min: 2.688279, mean: 3.620682, max: 5.882401
12:53:10 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:53:10 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.002082, dN_50: 0.000163
12:53:10 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:53:10 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.017104330768122233
12:53:10 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:53:10 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:53:10 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:53:10 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2998 surface elements
12:53:10 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2997 surface elements
12:53:10 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2996 surface elements
12:53:10 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2994 surface elements
12:53:10 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2993 surface elements
12:53:10 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2992 surface elements
12:53:10 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2990 surface elements
12:53:10 DEBUG opendrift.models.physics_methods:915: Advecting 2989 of 6000 elements above 0.100m with wind-sheared ocean current (0.096391 m/s - 0.206528 m/s)
12:53:10 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:53:10 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0006931579280825805 and 0.4368256885859911 m/s
12:53:10 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:53:10 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:53:10 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:53:10 INFO opendrift.models.basemodel:2038: 2024-11-08 02:51:18.915878 - step 39 of 120 - 6000 active elements (0 deactivated)
12:53:10 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:53:10 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:53:10 DEBUG opendrift.models.basemodel:2057: 34.94361771203837 <- latitude -> 35.13132537379084
12:53:10 DEBUG opendrift.models.basemodel:2062: 23.29188830995564 <- longitude -> 23.613143119228294
12:53:10 DEBUG opendrift.models.basemodel:2067: -119.0143730853019 <- z -> 0.0
12:53:10 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:53:10 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:10 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:53:10 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:10 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:53:10 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:10 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:10 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:53:10 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:10 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:10 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:10 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:10 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:53:10 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:10 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:53:10 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:10 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:10 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:53:10 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:10 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:53:10 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:10 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:10 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:10 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:53:10 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:10 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:53:10 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:10 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:10 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:53:10 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:10 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-08 00:00:00 (before)
2024-11-08 03:00:00 (after)
12:53:10 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-08 00:00:00) in space (linearNDFast)
12:53:10 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:10 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-08 03:00:00) in space (linearNDFast)
12:53:10 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:10 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-08 00:00:00, weight 0.05) and
after (2024-11-08 03:00:00, weight 0.95) in time
12:53:10 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:10 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:10 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:10 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:53:10 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:10 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:53:10 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:10 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:10 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:53:10 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:10 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-08 00:00:00 (before)
2024-11-08 03:00:00 (after)
12:53:10 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-08 00:00:00) in space (linearNDFast)
12:53:10 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:10 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-08 03:00:00) in space (linearNDFast)
12:53:10 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:10 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-08 00:00:00, weight 0.05) and
after (2024-11-08 03:00:00, weight 0.95) in time
12:53:10 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:10 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:10 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:53:10 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:53:10 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:53:10 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:53:10 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:53:10 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:53:10 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:53:10 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.436787 (min) 0.121983 (max)
12:53:10 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0124335 (min) 0.192549 (max)
12:53:10 DEBUG opendrift.models.basemodel.environment:905: x_wind: -4.13437 (min) -1.06655 (max)
12:53:10 DEBUG opendrift.models.basemodel.environment:905: y_wind: -6.20001 (min) -3.27727 (max)
12:53:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:53:10 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:53:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:53:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:53:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:53:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:53:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:53:10 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:53:10 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:53:10 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:53:10 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:53:10 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:53:10 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:53:10 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:53:10 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:53:10 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:53:10 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:53:10 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.297628, mean: 0.557016, max: 1.362699
12:53:10 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:53:10 DEBUG opendrift.models.physics_methods:1061: min: 2.972120, mean: 4.029430, max: 6.359596
12:53:10 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.972120, mean: 4.029430, max: 6.359596
12:53:10 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:53:10 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:53:10 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:53:10 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:53:10 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:53:10 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:53:10 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:53:10 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:53:10 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:53:10 DEBUG opendrift.models.physics_methods:1061: min: 2.972120, mean: 4.029430, max: 6.359596
12:53:10 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:53:10 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.001896, dN_50: 0.000149
12:53:10 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:53:10 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.019991282202540067
12:53:10 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:53:10 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:53:10 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:53:10 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2989 surface elements
12:53:10 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2988 surface elements
12:53:10 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2986 surface elements
12:53:10 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2984 surface elements
12:53:10 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2983 surface elements
12:53:10 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2982 surface elements
12:53:10 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2980 surface elements
12:53:10 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2976 surface elements
12:53:10 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2975 surface elements
12:53:10 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2974 surface elements
12:53:10 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2973 surface elements
12:53:10 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2971 surface elements
12:53:10 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2969 surface elements
12:53:10 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2968 surface elements
12:53:10 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2968 surface elements
12:53:10 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2967 surface elements
12:53:10 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2966 surface elements
12:53:10 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2965 surface elements
12:53:10 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2964 surface elements
12:53:10 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2963 surface elements
12:53:10 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2962 surface elements
12:53:10 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2961 surface elements
12:53:10 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2960 surface elements
12:53:10 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2959 surface elements
12:53:10 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2957 surface elements
12:53:10 DEBUG opendrift.models.physics_methods:915: Advecting 2957 of 6000 elements above 0.100m with wind-sheared ocean current (0.065511 m/s - 0.223282 m/s)
12:53:10 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:53:10 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.00032499714442314347 and 0.4872856938316968 m/s
12:53:10 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:53:10 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:53:10 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:53:10 INFO opendrift.models.basemodel:2038: 2024-11-08 03:51:18.915878 - step 40 of 120 - 6000 active elements (0 deactivated)
12:53:10 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:53:10 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:53:10 DEBUG opendrift.models.basemodel:2057: 34.9445464706478 <- latitude -> 35.132821621021904
12:53:10 DEBUG opendrift.models.basemodel:2062: 23.285647152603936 <- longitude -> 23.614459618430683
12:53:10 DEBUG opendrift.models.basemodel:2067: -118.25801503874274 <- z -> 0.0
12:53:10 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:53:10 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:10 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:53:10 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:10 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:53:10 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:10 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:10 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:53:10 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:10 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:10 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:10 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:10 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:53:10 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:10 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:53:10 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:10 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:10 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:53:10 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:10 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:53:10 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:10 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:10 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:10 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:53:10 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:10 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:53:10 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:10 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:10 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:53:10 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:10 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-08 03:00:00 (before)
2024-11-08 06:00:00 (after)
12:53:10 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
12:53:10 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
12:53:10 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:53:10 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:53:10 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:53:10 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
12:53:10 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 12x13x22) for time after (2024-11-08 06:00:00)
12:53:10 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-08 03:00:00) in space (linearNDFast)
12:53:10 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:11 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-08 06:00:00) in space (linearNDFast)
12:53:11 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:11 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-08 03:00:00, weight 0.71) and
after (2024-11-08 06:00:00, weight 0.29) in time
12:53:11 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:11 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:11 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:11 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:53:11 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:11 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:53:11 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:11 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:11 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:53:11 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:11 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-08 03:00:00 (before)
2024-11-08 06:00:00 (after)
12:53:11 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_wind to retrieve x_wind
12:53:14 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_wind to retrieve y_wind
12:53:16 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:53:16 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:53:16 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:53:16 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 720x361x1) for time after (2024-11-08 06:00:00)
12:53:16 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-08 03:00:00) in space (linearNDFast)
12:53:16 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:16 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-08 06:00:00) in space (linearNDFast)
12:53:16 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:16 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-08 03:00:00, weight 0.71) and
after (2024-11-08 06:00:00, weight 0.29) in time
12:53:16 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:16 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:16 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:53:16 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:53:16 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:53:16 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:53:16 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:53:16 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:53:16 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:53:16 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.383899 (min) 0.0943126 (max)
12:53:16 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.027248 (min) 0.195791 (max)
12:53:16 DEBUG opendrift.models.basemodel.environment:905: x_wind: -4.34462 (min) -1.2504 (max)
12:53:16 DEBUG opendrift.models.basemodel.environment:905: y_wind: -6.55216 (min) -3.29583 (max)
12:53:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:53:16 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:53:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:53:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:53:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:53:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:53:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:53:16 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:53:16 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:53:16 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:53:16 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:53:16 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:53:16 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:53:16 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:53:16 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:53:16 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:53:16 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:53:16 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.309039, mean: 0.612757, max: 1.519108
12:53:16 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:53:16 DEBUG opendrift.models.physics_methods:1061: min: 3.028562, mean: 4.222477, max: 6.714659
12:53:16 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.028562, mean: 4.222477, max: 6.714659
12:53:16 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:53:16 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:53:16 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:53:16 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:53:16 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:53:16 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:53:16 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:53:16 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:53:16 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:53:16 DEBUG opendrift.models.physics_methods:1061: min: 3.028562, mean: 4.222476, max: 6.714659
12:53:16 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:53:16 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.001853, dN_50: 0.000145
12:53:16 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:53:16 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.022285378593355092
12:53:16 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:53:16 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:53:16 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:53:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2956 surface elements
12:53:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2955 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2954 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2952 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2951 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2950 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2949 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2946 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2945 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2944 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2943 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2940 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2939 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 2937 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2932 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2931 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2930 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2928 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2927 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2926 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2925 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2924 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2923 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2922 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2920 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2919 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2918 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2915 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2912 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2910 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2909 surface elements
12:53:17 DEBUG opendrift.models.physics_methods:915: Advecting 2908 of 6000 elements above 0.100m with wind-sheared ocean current (0.108638 m/s - 0.230740 m/s)
12:53:17 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:53:17 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0013780232825027809 and 0.43004160851500345 m/s
12:53:17 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:53:17 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:53:17 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:53:17 INFO opendrift.models.basemodel:2038: 2024-11-08 04:51:18.915878 - step 41 of 120 - 6000 active elements (0 deactivated)
12:53:17 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:53:17 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:53:17 DEBUG opendrift.models.basemodel:2057: 34.94009637768984 <- latitude -> 35.1320748200186
12:53:17 DEBUG opendrift.models.basemodel:2062: 23.281580984750263 <- longitude -> 23.61432240991379
12:53:17 DEBUG opendrift.models.basemodel:2067: -117.16177247171676 <- z -> 0.0
12:53:17 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:53:17 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:17 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:53:17 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:17 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:53:17 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:17 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:17 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:53:17 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:17 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:17 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:17 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:17 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:53:17 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:17 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:53:17 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:17 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:17 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:53:17 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:17 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:53:17 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:17 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:17 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:17 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:53:17 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:17 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:53:17 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:17 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:17 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:53:17 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:17 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-08 03:00:00 (before)
2024-11-08 06:00:00 (after)
12:53:17 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-08 03:00:00) in space (linearNDFast)
12:53:17 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:17 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-08 06:00:00) in space (linearNDFast)
12:53:17 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:17 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-08 03:00:00, weight 0.38) and
after (2024-11-08 06:00:00, weight 0.62) in time
12:53:17 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:17 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:17 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:17 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:53:17 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:17 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:53:17 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:17 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:17 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:53:17 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:17 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-08 03:00:00 (before)
2024-11-08 06:00:00 (after)
12:53:17 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-08 03:00:00) in space (linearNDFast)
12:53:17 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:17 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-08 06:00:00) in space (linearNDFast)
12:53:17 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:17 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-08 03:00:00, weight 0.38) and
after (2024-11-08 06:00:00, weight 0.62) in time
12:53:17 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:17 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:17 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:53:17 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:53:17 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:53:17 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:53:17 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:53:17 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:53:17 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:53:17 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.378563 (min) 0.100042 (max)
12:53:17 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0541638 (min) 0.175369 (max)
12:53:17 DEBUG opendrift.models.basemodel.environment:905: x_wind: -4.46074 (min) -1.42666 (max)
12:53:17 DEBUG opendrift.models.basemodel.environment:905: y_wind: -6.8506 (min) -3.2427 (max)
12:53:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:53:17 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:53:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:53:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:53:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:53:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:53:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:53:17 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:53:17 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:53:17 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:53:17 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:53:17 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:53:17 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:53:17 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:53:17 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:53:17 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:53:17 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:53:17 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.309079, mean: 0.661955, max: 1.643990
12:53:17 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:53:17 DEBUG opendrift.models.physics_methods:1061: min: 3.028758, mean: 4.384644, max: 6.985206
12:53:17 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.028758, mean: 4.384644, max: 6.985206
12:53:17 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:53:17 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:53:17 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:53:17 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:53:17 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:53:17 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:53:17 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:53:17 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:53:17 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:53:17 DEBUG opendrift.models.physics_methods:1061: min: 3.028758, mean: 4.384644, max: 6.985206
12:53:17 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:53:17 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.001836, dN_50: 0.000144
12:53:17 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:53:17 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.024117062399412127
12:53:17 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:53:17 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:53:17 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2908 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2908 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2905 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2905 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2903 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2902 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2901 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2900 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2899 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2898 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2897 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2897 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2895 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2894 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2893 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2892 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2889 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2887 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2886 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2884 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2883 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2882 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2880 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2879 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2876 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2875 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2874 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2872 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2869 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2867 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2865 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2864 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2862 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2858 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2857 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2856 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2855 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2854 surface elements
12:53:17 DEBUG opendrift.models.physics_methods:915: Advecting 2853 of 6000 elements above 0.100m with wind-sheared ocean current (0.109534 m/s - 0.240264 m/s)
12:53:17 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:53:17 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0007222447785961865 and 0.4220613910967817 m/s
12:53:17 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:53:17 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:53:17 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:53:17 INFO opendrift.models.basemodel:2038: 2024-11-08 05:51:18.915878 - step 42 of 120 - 6000 active elements (0 deactivated)
12:53:17 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:53:17 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:53:17 DEBUG opendrift.models.basemodel:2057: 34.93473316439298 <- latitude -> 35.132759029305596
12:53:17 DEBUG opendrift.models.basemodel:2062: 23.273654763438476 <- longitude -> 23.6093984893254
12:53:17 DEBUG opendrift.models.basemodel:2067: -116.22413306475296 <- z -> 0.0
12:53:17 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:53:17 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:17 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:53:17 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:17 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:53:17 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:17 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:17 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:53:17 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:17 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:17 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:17 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:17 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:53:17 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:17 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:53:17 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:17 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:17 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:53:17 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:17 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:53:17 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:17 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:17 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:17 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:53:17 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:17 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:53:17 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:17 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:17 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:53:17 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:17 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-08 03:00:00 (before)
2024-11-08 06:00:00 (after)
12:53:17 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-08 03:00:00) in space (linearNDFast)
12:53:17 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:17 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-08 06:00:00) in space (linearNDFast)
12:53:17 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:17 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-08 03:00:00, weight 0.05) and
after (2024-11-08 06:00:00, weight 0.95) in time
12:53:17 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:17 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:17 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:17 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:53:17 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:17 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:53:17 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:17 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:17 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:53:17 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:17 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-08 03:00:00 (before)
2024-11-08 06:00:00 (after)
12:53:17 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-08 03:00:00) in space (linearNDFast)
12:53:17 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:17 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-08 06:00:00) in space (linearNDFast)
12:53:17 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:17 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-08 03:00:00, weight 0.05) and
after (2024-11-08 06:00:00, weight 0.95) in time
12:53:17 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:17 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:17 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:53:17 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:53:17 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:53:17 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:53:17 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:53:17 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:53:17 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:53:17 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.370462 (min) 0.114524 (max)
12:53:17 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0762943 (min) 0.145012 (max)
12:53:17 DEBUG opendrift.models.basemodel.environment:905: x_wind: -4.58601 (min) -1.54669 (max)
12:53:17 DEBUG opendrift.models.basemodel.environment:905: y_wind: -7.18996 (min) -3.17157 (max)
12:53:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:53:17 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:53:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:53:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:53:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:53:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:53:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:53:17 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:53:17 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:53:17 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:53:17 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:53:17 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:53:17 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:53:17 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:53:17 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:53:17 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:53:17 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:53:17 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.306298, mean: 0.713591, max: 1.789082
12:53:17 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:53:17 DEBUG opendrift.models.physics_methods:1061: min: 3.015097, mean: 4.549837, max: 7.286933
12:53:17 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.015097, mean: 4.549837, max: 7.286933
12:53:17 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:53:17 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:53:17 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:53:17 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:53:17 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:53:17 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:53:17 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:53:17 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:53:17 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:53:17 DEBUG opendrift.models.physics_methods:1061: min: 3.015097, mean: 4.549837, max: 7.286932
12:53:17 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:53:17 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.001827, dN_50: 0.000143
12:53:17 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:53:17 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.02624516755744468
12:53:17 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:53:17 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:53:17 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2853 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2852 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2849 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2846 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 7 of 2845 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2838 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2837 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2836 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2834 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2832 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2831 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2830 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2829 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2827 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2824 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2823 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2821 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2820 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2816 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2813 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2812 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2811 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2809 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2807 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2806 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2805 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2804 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2802 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2799 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2795 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 6 of 2793 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2787 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2785 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2782 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2781 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2781 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2779 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2778 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2777 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2776 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2774 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2770 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2768 surface elements
12:53:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2767 surface elements
12:53:17 DEBUG opendrift.models.physics_methods:915: Advecting 2769 of 6000 elements above 0.100m with wind-sheared ocean current (0.076885 m/s - 0.247279 m/s)
12:53:17 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:53:17 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0014377826745921476 and 0.4493327482454887 m/s
12:53:17 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:53:17 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:53:17 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:53:17 INFO opendrift.models.basemodel:2038: 2024-11-08 06:51:18.915878 - step 43 of 120 - 6000 active elements (0 deactivated)
12:53:17 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:53:17 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:53:17 DEBUG opendrift.models.basemodel:2057: 34.93692166022805 <- latitude -> 35.13913793491222
12:53:17 DEBUG opendrift.models.basemodel:2062: 23.264621998349288 <- longitude -> 23.6130885897597
12:53:17 DEBUG opendrift.models.basemodel:2067: -115.21172801589726 <- z -> 0.0
12:53:17 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:53:17 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:17 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:53:17 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:17 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:53:17 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:17 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:17 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:53:17 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:17 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:17 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:17 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:17 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:53:17 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:17 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:53:17 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:17 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:17 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:53:17 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:17 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:53:17 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:17 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:17 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:17 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:53:17 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:17 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:53:17 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:17 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:17 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:53:17 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:17 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-08 06:00:00 (before)
2024-11-08 09:00:00 (after)
12:53:17 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
12:53:17 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
12:53:17 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:53:17 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:53:17 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:53:17 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
12:53:17 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 13x13x22) for time after (2024-11-08 09:00:00)
12:53:17 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-08 06:00:00) in space (linearNDFast)
12:53:17 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:17 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-08 09:00:00) in space (linearNDFast)
12:53:17 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:17 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-08 06:00:00, weight 0.71) and
after (2024-11-08 09:00:00, weight 0.29) in time
12:53:17 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:17 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:17 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:17 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:53:17 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:17 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:53:17 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:17 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:17 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:53:17 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:17 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-08 06:00:00 (before)
2024-11-08 09:00:00 (after)
12:53:17 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_wind to retrieve x_wind
12:53:20 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_wind to retrieve y_wind
12:53:21 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:53:21 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:53:21 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:53:21 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 720x361x1) for time after (2024-11-08 09:00:00)
12:53:21 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-08 06:00:00) in space (linearNDFast)
12:53:21 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:21 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-08 09:00:00) in space (linearNDFast)
12:53:21 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:21 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-08 06:00:00, weight 0.71) and
after (2024-11-08 09:00:00, weight 0.29) in time
12:53:21 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:21 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:21 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:53:21 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:53:21 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:53:21 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:53:21 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:53:21 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:53:21 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:53:21 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.378276 (min) 0.127186 (max)
12:53:21 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0985749 (min) 0.15634 (max)
12:53:21 DEBUG opendrift.models.basemodel.environment:905: x_wind: -4.75892 (min) -1.49777 (max)
12:53:21 DEBUG opendrift.models.basemodel.environment:905: y_wind: -6.81631 (min) -2.18599 (max)
12:53:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:53:21 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:53:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:53:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:53:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:53:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:53:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:53:21 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:53:21 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:53:21 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:53:21 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:53:21 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:53:21 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:53:21 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:53:21 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:53:21 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:53:21 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:53:21 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.172738, mean: 0.513670, max: 1.700091
12:53:21 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:53:21 DEBUG opendrift.models.physics_methods:1061: min: 2.264244, mean: 3.824830, max: 7.103390
12:53:21 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.264244, mean: 3.824830, max: 7.103390
12:53:21 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:53:21 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:53:21 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:53:21 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:53:21 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:53:21 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:53:21 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:53:21 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:53:21 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:53:21 DEBUG opendrift.models.physics_methods:1061: min: 2.264244, mean: 3.824830, max: 7.103390
12:53:21 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:53:21 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.002276, dN_50: 0.000179
12:53:21 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:53:21 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.024939903872676247
12:53:21 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:53:21 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:53:21 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:53:21 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2766 surface elements
12:53:21 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2765 surface elements
12:53:21 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2764 surface elements
12:53:21 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2763 surface elements
12:53:21 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2762 surface elements
12:53:21 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2761 surface elements
12:53:21 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2760 surface elements
12:53:21 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2761 surface elements
12:53:21 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2759 surface elements
12:53:21 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2758 surface elements
12:53:21 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2757 surface elements
12:53:21 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2756 surface elements
12:53:21 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2755 surface elements
12:53:21 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2754 surface elements
12:53:21 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2753 surface elements
12:53:21 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2752 surface elements
12:53:21 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2750 surface elements
12:53:21 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2750 surface elements
12:53:21 DEBUG opendrift.models.physics_methods:915: Advecting 2750 of 6000 elements above 0.100m with wind-sheared ocean current (0.048006 m/s - 0.235157 m/s)
12:53:21 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:53:21 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0014411247040008923 and 0.4312068457340787 m/s
12:53:21 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:53:21 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:53:21 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:53:21 INFO opendrift.models.basemodel:2038: 2024-11-08 07:51:18.915878 - step 44 of 120 - 6000 active elements (0 deactivated)
12:53:21 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:53:21 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:53:21 DEBUG opendrift.models.basemodel:2057: 34.94370280541635 <- latitude -> 35.13842062251159
12:53:21 DEBUG opendrift.models.basemodel:2062: 23.256608648790007 <- longitude -> 23.615475764633732
12:53:21 DEBUG opendrift.models.basemodel:2067: -114.75249283544915 <- z -> 0.0
12:53:21 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:53:21 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:21 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:53:21 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:21 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:53:21 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:21 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:21 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:53:21 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:21 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:21 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:21 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:21 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:53:21 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:21 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:53:21 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:21 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:21 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:53:21 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:21 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:53:21 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:21 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:21 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:21 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:53:21 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:21 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:53:21 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:21 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:21 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:53:21 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:21 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-08 06:00:00 (before)
2024-11-08 09:00:00 (after)
12:53:21 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-08 06:00:00) in space (linearNDFast)
12:53:21 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:21 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-08 09:00:00) in space (linearNDFast)
12:53:21 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:21 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-08 06:00:00, weight 0.38) and
after (2024-11-08 09:00:00, weight 0.62) in time
12:53:21 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:21 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:21 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:21 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:53:21 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:21 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:53:21 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:21 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:21 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:53:21 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:21 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-08 06:00:00 (before)
2024-11-08 09:00:00 (after)
12:53:21 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-08 06:00:00) in space (linearNDFast)
12:53:21 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:21 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-08 09:00:00) in space (linearNDFast)
12:53:21 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:21 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-08 06:00:00, weight 0.38) and
after (2024-11-08 09:00:00, weight 0.62) in time
12:53:21 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:21 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:21 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:53:21 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:53:21 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:53:21 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:53:21 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:53:21 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:53:21 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:53:21 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.404162 (min) 0.18511 (max)
12:53:21 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.138192 (min) 0.176952 (max)
12:53:21 DEBUG opendrift.models.basemodel.environment:905: x_wind: -4.87001 (min) -1.41524 (max)
12:53:21 DEBUG opendrift.models.basemodel.environment:905: y_wind: -6.27386 (min) -1.0109 (max)
12:53:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:53:21 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:53:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:53:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:53:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:53:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:53:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:53:21 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:53:21 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:53:21 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:53:21 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:53:21 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:53:21 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:53:21 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:53:21 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:53:21 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:53:21 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:53:21 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.074411, mean: 0.339408, max: 1.551726
12:53:21 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:53:21 DEBUG opendrift.models.physics_methods:1061: min: 1.486098, mean: 3.051968, max: 6.786364
12:53:21 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.486098, mean: 3.051968, max: 6.786364
12:53:21 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:53:21 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:53:21 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:53:21 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:53:21 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:53:21 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:53:21 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:53:21 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:53:21 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:53:21 DEBUG opendrift.models.physics_methods:1061: min: 1.486098, mean: 3.051967, max: 6.786364
12:53:21 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:53:21 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.003023, dN_50: 0.000237
12:53:21 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:53:21 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.02276379570645683
12:53:21 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:53:21 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:53:21 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:53:21 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2748 surface elements
12:53:21 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2747 surface elements
12:53:21 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2746 surface elements
12:53:21 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2746 surface elements
12:53:21 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2745 surface elements
12:53:21 DEBUG opendrift.models.physics_methods:915: Advecting 2745 of 6000 elements above 0.100m with wind-sheared ocean current (0.053971 m/s - 0.221453 m/s)
12:53:21 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:53:22 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.004101206223366215 and 0.45764769220468826 m/s
12:53:22 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:53:22 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:53:22 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:53:22 INFO opendrift.models.basemodel:2038: 2024-11-08 08:51:18.915878 - step 45 of 120 - 6000 active elements (0 deactivated)
12:53:22 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:53:22 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:53:22 DEBUG opendrift.models.basemodel:2057: 34.944254266587535 <- latitude -> 35.14132432770913
12:53:22 DEBUG opendrift.models.basemodel:2062: 23.253354951465706 <- longitude -> 23.61129575027325
12:53:22 DEBUG opendrift.models.basemodel:2067: -114.16295417318814 <- z -> 0.0
12:53:22 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:53:22 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:22 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:53:22 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:22 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:53:22 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:22 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:22 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:53:22 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:22 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:22 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:22 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:22 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:53:22 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:22 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:53:22 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:22 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:22 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:53:22 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:22 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:53:22 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:22 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:22 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:22 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:53:22 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:22 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:53:22 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:22 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:22 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:53:22 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:22 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-08 06:00:00 (before)
2024-11-08 09:00:00 (after)
12:53:22 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-08 06:00:00) in space (linearNDFast)
12:53:22 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:22 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-08 09:00:00) in space (linearNDFast)
12:53:22 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:22 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-08 06:00:00, weight 0.05) and
after (2024-11-08 09:00:00, weight 0.95) in time
12:53:22 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:22 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:22 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:22 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:53:22 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:22 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:53:22 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:22 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:22 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:53:22 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:22 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-08 06:00:00 (before)
2024-11-08 09:00:00 (after)
12:53:22 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-08 06:00:00) in space (linearNDFast)
12:53:22 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:22 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-08 09:00:00) in space (linearNDFast)
12:53:22 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:22 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-08 06:00:00, weight 0.05) and
after (2024-11-08 09:00:00, weight 0.95) in time
12:53:22 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:22 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:22 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:53:22 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:53:22 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:53:22 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:53:22 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:53:22 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:53:22 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:53:22 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.448227 (min) 0.165444 (max)
12:53:22 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.183692 (min) 0.198427 (max)
12:53:22 DEBUG opendrift.models.basemodel.environment:905: x_wind: -4.92497 (min) -1.31607 (max)
12:53:22 DEBUG opendrift.models.basemodel.environment:905: y_wind: -5.66555 (min) 0.219158 (max)
12:53:22 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:53:22 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:53:22 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:53:22 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:53:22 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:53:22 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:53:22 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:53:22 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:53:22 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:53:22 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:53:22 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:53:22 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:53:22 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:53:22 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:53:22 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:53:22 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:53:22 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:53:22 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.043402, mean: 0.235945, max: 1.386305
12:53:22 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:53:22 DEBUG opendrift.models.physics_methods:1061: min: 1.134965, mean: 2.498200, max: 6.414443
12:53:22 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.134965, mean: 2.498200, max: 6.414443
12:53:22 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:53:22 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:53:22 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:53:22 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:53:22 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:53:22 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:53:22 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:53:22 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:53:22 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:53:22 DEBUG opendrift.models.physics_methods:1061: min: 1.134965, mean: 2.498200, max: 6.414443
12:53:22 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:53:22 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.003888, dN_50: 0.000305
12:53:22 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:53:22 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.02033751696589763
12:53:22 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:53:22 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:53:22 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:53:22 DEBUG opendrift.models.physics_methods:915: Advecting 2748 of 6000 elements above 0.100m with wind-sheared ocean current (0.007561 m/s - 0.207260 m/s)
12:53:22 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:53:22 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0017908188912365063 and 0.41383519308694083 m/s
12:53:22 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:53:22 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:53:22 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:53:22 INFO opendrift.models.basemodel:2038: 2024-11-08 09:51:18.915878 - step 46 of 120 - 6000 active elements (0 deactivated)
12:53:22 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:53:22 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:53:22 DEBUG opendrift.models.basemodel:2057: 34.940624944604416 <- latitude -> 35.143565484053326
12:53:22 DEBUG opendrift.models.basemodel:2062: 23.2523178697637 <- longitude -> 23.614870258517357
12:53:22 DEBUG opendrift.models.basemodel:2067: -112.82106316149553 <- z -> 0.0
12:53:22 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:53:22 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:22 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:53:22 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:22 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:53:22 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:22 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:22 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:53:22 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:22 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:22 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:22 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:22 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:53:22 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:22 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:53:22 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:22 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:22 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:53:22 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:22 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:53:22 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:22 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:22 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:22 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:53:22 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:22 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:53:22 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:22 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:22 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:53:22 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:22 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-08 09:00:00 (before)
2024-11-08 12:00:00 (after)
12:53:22 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
12:53:22 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
12:53:22 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:53:22 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:53:22 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:53:22 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
12:53:22 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 13x13x22) for time after (2024-11-08 12:00:00)
12:53:22 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-08 09:00:00) in space (linearNDFast)
12:53:22 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:22 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-08 12:00:00) in space (linearNDFast)
12:53:22 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:22 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-08 09:00:00, weight 0.71) and
after (2024-11-08 12:00:00, weight 0.29) in time
12:53:22 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:22 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:22 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:22 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:53:22 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:22 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:53:22 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:22 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:22 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:53:22 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:22 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-08 09:00:00 (before)
2024-11-08 12:00:00 (after)
12:53:22 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_wind to retrieve x_wind
12:53:25 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_wind to retrieve y_wind
12:53:28 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:53:28 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:53:28 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:53:28 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 720x361x1) for time after (2024-11-08 12:00:00)
12:53:28 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-08 09:00:00) in space (linearNDFast)
12:53:28 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:28 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-08 12:00:00) in space (linearNDFast)
12:53:28 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:28 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-08 09:00:00, weight 0.71) and
after (2024-11-08 12:00:00, weight 0.29) in time
12:53:28 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:28 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:28 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:53:28 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:53:28 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:53:28 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:53:28 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:53:28 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:53:28 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:53:28 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.486254 (min) 0.113411 (max)
12:53:28 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.168808 (min) 0.233472 (max)
12:53:28 DEBUG opendrift.models.basemodel.environment:905: x_wind: -4.69728 (min) -0.754112 (max)
12:53:28 DEBUG opendrift.models.basemodel.environment:905: y_wind: -5.52418 (min) 0.113555 (max)
12:53:28 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:53:28 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:53:28 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:53:28 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:53:28 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:53:28 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:53:28 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:53:28 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:53:28 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:53:28 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:53:28 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:53:28 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:53:28 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:53:28 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:53:28 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:53:28 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:53:28 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:53:28 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.014179, mean: 0.192559, max: 1.293492
12:53:28 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:53:28 DEBUG opendrift.models.physics_methods:1061: min: 0.648702, mean: 2.202851, max: 6.196001
12:53:28 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 0.648702, mean: 2.202851, max: 6.196001
12:53:28 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:53:28 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:53:28 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:53:28 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:53:28 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:53:28 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:53:28 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:53:28 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:53:28 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:53:28 DEBUG opendrift.models.physics_methods:1061: min: 0.648702, mean: 2.202851, max: 6.196001
12:53:28 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:53:28 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.004825, dN_50: 0.000379
12:53:28 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:53:28 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.018976203057181878
12:53:28 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:53:28 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:53:28 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:53:28 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2745 surface elements
12:53:28 DEBUG opendrift.models.physics_methods:915: Advecting 2745 of 6000 elements above 0.100m with wind-sheared ocean current (0.024756 m/s - 0.201835 m/s)
12:53:28 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:53:28 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0028618454278339133 and 0.4373111265937053 m/s
12:53:28 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:53:28 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:53:28 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:53:28 INFO opendrift.models.basemodel:2038: 2024-11-08 10:51:18.915878 - step 47 of 120 - 6000 active elements (0 deactivated)
12:53:28 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:53:28 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:53:28 DEBUG opendrift.models.basemodel:2057: 34.94089992501259 <- latitude -> 35.149240514706236
12:53:28 DEBUG opendrift.models.basemodel:2062: 23.240965301690334 <- longitude -> 23.612979087707764
12:53:28 DEBUG opendrift.models.basemodel:2067: -112.11074098725234 <- z -> 0.0
12:53:28 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:53:28 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:28 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:53:28 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:28 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:53:28 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:28 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:28 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:53:28 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:28 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:28 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:28 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:28 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:53:28 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:28 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:53:28 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:28 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:28 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:53:29 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:29 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:53:29 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:29 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:29 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:29 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:53:29 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:29 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:53:29 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:29 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:29 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:53:29 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:29 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-08 09:00:00 (before)
2024-11-08 12:00:00 (after)
12:53:29 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-08 09:00:00) in space (linearNDFast)
12:53:29 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:29 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-08 12:00:00) in space (linearNDFast)
12:53:29 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:29 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-08 09:00:00, weight 0.38) and
after (2024-11-08 12:00:00, weight 0.62) in time
12:53:29 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:29 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:29 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:29 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:53:29 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:29 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:53:29 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:29 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:29 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:53:29 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:29 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-08 09:00:00 (before)
2024-11-08 12:00:00 (after)
12:53:29 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-08 09:00:00) in space (linearNDFast)
12:53:29 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:29 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-08 12:00:00) in space (linearNDFast)
12:53:29 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:29 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-08 09:00:00, weight 0.38) and
after (2024-11-08 12:00:00, weight 0.62) in time
12:53:29 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:29 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:29 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:53:29 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:53:29 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:53:29 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:53:29 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:53:29 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:53:29 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:53:29 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.508346 (min) 0.0866571 (max)
12:53:29 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.148356 (min) 0.261737 (max)
12:53:29 DEBUG opendrift.models.basemodel.environment:905: x_wind: -4.45776 (min) -0.0884007 (max)
12:53:29 DEBUG opendrift.models.basemodel.environment:905: y_wind: -5.47324 (min) -0.176182 (max)
12:53:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:53:29 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:53:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:53:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:53:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:53:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:53:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:53:29 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:53:29 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:53:29 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:53:29 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:53:29 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:53:29 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:53:29 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:53:29 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:53:29 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:53:29 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:53:29 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.001730, mean: 0.173083, max: 1.225768
12:53:29 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:53:29 DEBUG opendrift.models.physics_methods:1061: min: 0.226616, mean: 2.061496, max: 6.031617
12:53:29 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 0.226616, mean: 2.061496, max: 6.031617
12:53:29 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:53:29 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:53:29 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:53:29 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:53:29 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:53:29 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:53:29 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:53:29 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:53:29 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:53:29 DEBUG opendrift.models.physics_methods:1061: min: 0.226616, mean: 2.061496, max: 6.031617
12:53:29 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:53:29 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.005784, dN_50: 0.000454
12:53:29 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:53:29 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.017982876955084156
12:53:29 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:53:29 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:53:29 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:53:29 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2744 surface elements
12:53:29 DEBUG opendrift.models.physics_methods:915: Advecting 2744 of 6000 elements above 0.100m with wind-sheared ocean current (0.009235 m/s - 0.186385 m/s)
12:53:29 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:53:29 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.002014528692394443 and 0.47529544875288304 m/s
12:53:29 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:53:29 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:53:29 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:53:29 INFO opendrift.models.basemodel:2038: 2024-11-08 11:51:18.915878 - step 48 of 120 - 6000 active elements (0 deactivated)
12:53:29 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:53:29 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:53:29 DEBUG opendrift.models.basemodel:2057: 34.938246081686664 <- latitude -> 35.152497037526366
12:53:29 DEBUG opendrift.models.basemodel:2062: 23.224128114040813 <- longitude -> 23.609482304068816
12:53:29 DEBUG opendrift.models.basemodel:2067: -111.15273372564577 <- z -> 0.0
12:53:29 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:53:29 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:29 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:53:29 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:29 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:53:29 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:29 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:29 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:53:29 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:29 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:29 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:29 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:29 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:53:29 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:29 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:53:29 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:29 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:29 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:53:29 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:29 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:53:29 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:29 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:29 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:29 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:53:29 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:29 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:53:29 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:29 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:29 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:53:29 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:29 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-08 09:00:00 (before)
2024-11-08 12:00:00 (after)
12:53:29 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-08 09:00:00) in space (linearNDFast)
12:53:29 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:29 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-08 12:00:00) in space (linearNDFast)
12:53:29 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:29 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-08 09:00:00, weight 0.05) and
after (2024-11-08 12:00:00, weight 0.95) in time
12:53:29 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:29 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:29 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:29 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:53:29 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:29 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:53:29 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:29 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:29 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:53:29 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:29 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-08 09:00:00 (before)
2024-11-08 12:00:00 (after)
12:53:29 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-08 09:00:00) in space (linearNDFast)
12:53:29 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:29 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-08 12:00:00) in space (linearNDFast)
12:53:29 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:29 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-08 09:00:00, weight 0.05) and
after (2024-11-08 12:00:00, weight 0.95) in time
12:53:29 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:29 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:29 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:53:29 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:53:29 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:53:29 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:53:29 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:53:29 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:53:29 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:53:29 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.574862 (min) 0.0332657 (max)
12:53:29 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.162052 (min) 0.295061 (max)
12:53:29 DEBUG opendrift.models.basemodel.environment:905: x_wind: -4.29552 (min) 0.628417 (max)
12:53:29 DEBUG opendrift.models.basemodel.environment:905: y_wind: -5.43498 (min) -0.481081 (max)
12:53:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:53:29 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:53:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:53:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:53:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:53:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:53:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:53:29 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:53:29 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:53:29 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:53:29 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:53:29 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:53:29 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:53:29 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:53:29 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:53:29 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:53:29 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:53:29 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.009790, mean: 0.173725, max: 1.180568
12:53:29 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:53:29 DEBUG opendrift.models.physics_methods:1061: min: 0.539031, mean: 2.117366, max: 5.919365
12:53:29 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 0.539031, mean: 2.117366, max: 5.919365
12:53:29 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:53:29 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:53:29 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:53:29 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:53:29 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:53:29 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:53:29 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:53:29 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:53:29 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:53:29 DEBUG opendrift.models.physics_methods:1061: min: 0.539031, mean: 2.117367, max: 5.919365
12:53:29 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:53:29 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.005000, dN_50: 0.000392
12:53:29 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:53:29 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.017319922112591892
12:53:29 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:53:29 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:53:29 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:53:29 DEBUG opendrift.models.physics_methods:915: Advecting 2745 of 6000 elements above 0.100m with wind-sheared ocean current (0.022867 m/s - 0.173107 m/s)
12:53:29 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:53:29 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0003291284235230825 and 0.4203852057432633 m/s
12:53:29 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:53:29 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:53:29 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:53:29 INFO opendrift.models.basemodel:2038: 2024-11-08 12:51:18.915878 - step 49 of 120 - 6000 active elements (0 deactivated)
12:53:29 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:53:29 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:53:29 DEBUG opendrift.models.basemodel:2057: 34.937321288699096 <- latitude -> 35.16576256853261
12:53:29 DEBUG opendrift.models.basemodel:2062: 23.21108738808145 <- longitude -> 23.612935868702813
12:53:29 DEBUG opendrift.models.basemodel:2067: -110.49903802780376 <- z -> 0.0
12:53:29 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:53:29 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:29 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:53:29 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:29 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:53:29 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:29 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:29 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:53:29 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:29 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:29 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:29 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:29 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:53:29 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:29 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:53:29 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:29 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:29 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:53:29 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:29 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:53:29 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:29 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:29 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:29 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:53:29 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:29 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:53:29 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:29 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:29 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:53:29 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:29 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-08 12:00:00 (before)
2024-11-08 15:00:00 (after)
12:53:29 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
12:53:29 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
12:53:29 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:53:29 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:53:29 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:53:29 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
12:53:29 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 13x14x22) for time after (2024-11-08 15:00:00)
12:53:29 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-08 12:00:00) in space (linearNDFast)
12:53:29 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:29 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-08 15:00:00) in space (linearNDFast)
12:53:29 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:29 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-08 12:00:00, weight 0.71) and
after (2024-11-08 15:00:00, weight 0.29) in time
12:53:29 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:29 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:29 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:29 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:53:29 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:29 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:53:29 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:29 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:29 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:53:29 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:29 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-08 12:00:00 (before)
2024-11-08 15:00:00 (after)
12:53:29 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_wind to retrieve x_wind
12:53:32 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_wind to retrieve y_wind
12:53:34 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:53:34 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:53:34 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:53:34 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 720x361x1) for time after (2024-11-08 15:00:00)
12:53:34 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-08 12:00:00) in space (linearNDFast)
12:53:34 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:34 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-08 15:00:00) in space (linearNDFast)
12:53:34 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:34 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-08 12:00:00, weight 0.71) and
after (2024-11-08 15:00:00, weight 0.29) in time
12:53:34 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:34 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:34 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:53:34 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:53:34 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:53:34 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:53:34 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:53:34 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:53:34 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:53:34 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.548519 (min) 0.044525 (max)
12:53:34 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.136247 (min) 0.286178 (max)
12:53:34 DEBUG opendrift.models.basemodel.environment:905: x_wind: -4.15481 (min) 0.959319 (max)
12:53:34 DEBUG opendrift.models.basemodel.environment:905: y_wind: -5.6262 (min) -0.201289 (max)
12:53:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:53:34 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:53:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:53:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:53:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:53:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:53:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:53:34 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:53:34 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:53:34 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:53:34 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:53:34 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:53:34 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:53:34 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:53:34 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:53:34 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:53:34 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:53:34 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.011376, mean: 0.149465, max: 1.203347
12:53:34 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:53:34 DEBUG opendrift.models.physics_methods:1061: min: 0.581057, mean: 1.927340, max: 5.976199
12:53:34 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 0.581057, mean: 1.927340, max: 5.976199
12:53:34 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:53:35 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:53:35 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:53:35 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:53:35 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:53:35 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:53:35 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:53:35 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:53:35 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:53:35 DEBUG opendrift.models.physics_methods:1061: min: 0.581057, mean: 1.927340, max: 5.976199
12:53:35 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:53:35 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.005785, dN_50: 0.000454
12:53:35 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:53:35 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.017654028761068412
12:53:35 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:53:35 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:53:35 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:53:35 DEBUG opendrift.models.physics_methods:915: Advecting 2746 of 6000 elements above 0.100m with wind-sheared ocean current (0.020401 m/s - 0.167679 m/s)
12:53:35 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:53:35 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.002091914266446171 and 0.469272119667634 m/s
12:53:35 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:53:35 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:53:35 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:53:35 INFO opendrift.models.basemodel:2038: 2024-11-08 13:51:18.915878 - step 50 of 120 - 6000 active elements (0 deactivated)
12:53:35 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:53:35 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:53:35 DEBUG opendrift.models.basemodel:2057: 34.940279225132194 <- latitude -> 35.17437567020887
12:53:35 DEBUG opendrift.models.basemodel:2062: 23.19965186371223 <- longitude -> 23.605881283129904
12:53:35 DEBUG opendrift.models.basemodel:2067: -109.41788334952949 <- z -> 0.0
12:53:35 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:53:35 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:35 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:53:35 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:35 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:53:35 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:35 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:35 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:53:35 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:35 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:35 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:35 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:35 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:53:35 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:35 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:53:35 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:35 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:35 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:53:35 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:35 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:53:35 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:35 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:35 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:35 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:53:35 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:35 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:53:35 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:35 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:35 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:53:35 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:35 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-08 12:00:00 (before)
2024-11-08 15:00:00 (after)
12:53:35 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-08 12:00:00) in space (linearNDFast)
12:53:35 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:35 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-08 15:00:00) in space (linearNDFast)
12:53:35 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:35 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-08 12:00:00, weight 0.38) and
after (2024-11-08 15:00:00, weight 0.62) in time
12:53:35 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:35 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:35 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:35 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:53:35 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:35 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:53:35 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:35 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:35 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:53:35 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:35 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-08 12:00:00 (before)
2024-11-08 15:00:00 (after)
12:53:35 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-08 12:00:00) in space (linearNDFast)
12:53:35 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:35 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-08 15:00:00) in space (linearNDFast)
12:53:35 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:35 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-08 12:00:00, weight 0.38) and
after (2024-11-08 15:00:00, weight 0.62) in time
12:53:35 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:35 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:35 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:53:35 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:53:35 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:53:35 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:53:35 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:53:35 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:53:35 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:53:35 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.6172 (min) 0.0724661 (max)
12:53:35 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.057119 (min) 0.249055 (max)
12:53:35 DEBUG opendrift.models.basemodel.environment:905: x_wind: -3.94365 (min) 1.22578 (max)
12:53:35 DEBUG opendrift.models.basemodel.environment:905: y_wind: -5.85583 (min) 0.19501 (max)
12:53:35 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:53:35 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:53:35 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:53:35 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:53:35 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:53:35 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:53:35 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:53:35 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:53:35 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:53:35 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:53:35 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:53:35 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:53:35 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:53:35 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:53:35 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:53:35 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:53:35 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:53:35 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.008042, mean: 0.125543, max: 1.226140
12:53:35 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:53:35 DEBUG opendrift.models.physics_methods:1061: min: 0.488557, mean: 1.709646, max: 6.032533
12:53:35 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 0.488557, mean: 1.709646, max: 6.032533
12:53:35 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:53:35 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:53:35 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:53:35 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:53:35 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:53:35 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:53:35 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:53:35 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:53:35 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:53:35 DEBUG opendrift.models.physics_methods:1061: min: 0.488557, mean: 1.709646, max: 6.032533
12:53:35 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:53:35 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.007144, dN_50: 0.000561
12:53:35 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:53:35 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.017988338132395414
12:53:35 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:53:35 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:53:35 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:53:35 DEBUG opendrift.models.physics_methods:915: Advecting 2747 of 6000 elements above 0.100m with wind-sheared ocean current (0.017153 m/s - 0.165710 m/s)
12:53:35 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:53:35 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0014462115866222427 and 0.465928923038023 m/s
12:53:35 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:53:35 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:53:35 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:53:35 INFO opendrift.models.basemodel:2038: 2024-11-08 14:51:18.915878 - step 51 of 120 - 6000 active elements (0 deactivated)
12:53:35 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:53:35 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:53:35 DEBUG opendrift.models.basemodel:2057: 34.94061281976963 <- latitude -> 35.17904034570789
12:53:35 DEBUG opendrift.models.basemodel:2062: 23.191951039037686 <- longitude -> 23.60331855380021
12:53:35 DEBUG opendrift.models.basemodel:2067: -108.8976029892506 <- z -> 0.0
12:53:35 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:53:35 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:35 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:53:35 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:35 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:53:35 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:35 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:35 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:53:35 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:35 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:35 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:35 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:35 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:53:35 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:35 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:53:35 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:35 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:35 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:53:35 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:35 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:53:35 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:35 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:35 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:35 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:53:35 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:35 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:53:35 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:35 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:35 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:53:35 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:35 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-08 12:00:00 (before)
2024-11-08 15:00:00 (after)
12:53:35 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-08 12:00:00) in space (linearNDFast)
12:53:35 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:35 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-08 15:00:00) in space (linearNDFast)
12:53:35 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:35 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-08 12:00:00, weight 0.05) and
after (2024-11-08 15:00:00, weight 0.95) in time
12:53:35 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:35 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:35 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:35 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:53:35 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:35 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:53:35 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:35 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:35 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:53:35 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:35 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-08 12:00:00 (before)
2024-11-08 15:00:00 (after)
12:53:35 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-08 12:00:00) in space (linearNDFast)
12:53:35 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:35 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-08 15:00:00) in space (linearNDFast)
12:53:35 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:35 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-08 12:00:00, weight 0.05) and
after (2024-11-08 15:00:00, weight 0.95) in time
12:53:35 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:35 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:35 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:53:35 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:53:35 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:53:35 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:53:35 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:53:35 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:53:35 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:53:35 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.66171 (min) 0.0851542 (max)
12:53:35 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0428121 (min) 0.241502 (max)
12:53:35 DEBUG opendrift.models.basemodel.environment:905: x_wind: -3.73418 (min) 1.49871 (max)
12:53:35 DEBUG opendrift.models.basemodel.environment:905: y_wind: -6.09965 (min) 0.673567 (max)
12:53:35 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:53:35 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:53:35 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:53:35 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:53:35 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:53:35 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:53:35 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:53:35 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:53:35 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:53:35 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:53:35 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:53:35 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:53:35 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:53:35 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:53:35 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:53:35 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:53:35 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:53:35 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.009271, mean: 0.107548, max: 1.258286
12:53:35 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:53:35 DEBUG opendrift.models.physics_methods:1061: min: 0.524554, mean: 1.533000, max: 6.111098
12:53:35 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 0.524554, mean: 1.533000, max: 6.111098
12:53:35 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:53:35 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:53:35 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:53:35 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:53:35 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:53:35 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:53:35 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:53:35 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:53:35 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:53:35 DEBUG opendrift.models.physics_methods:1061: min: 0.524554, mean: 1.533000, max: 6.111098
12:53:35 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:53:35 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.008708, dN_50: 0.000683
12:53:35 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:53:35 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.018459832700855022
12:53:35 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:53:35 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:53:35 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:53:35 DEBUG opendrift.models.physics_methods:915: Advecting 2747 of 6000 elements above 0.100m with wind-sheared ocean current (0.019817 m/s - 0.166156 m/s)
12:53:35 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:53:35 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0022427665561321874 and 0.4905003502028899 m/s
12:53:35 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:53:35 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:53:35 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:53:35 INFO opendrift.models.basemodel:2038: 2024-11-08 15:51:18.915878 - step 52 of 120 - 6000 active elements (0 deactivated)
12:53:35 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:53:35 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:53:35 DEBUG opendrift.models.basemodel:2057: 34.938140175836715 <- latitude -> 35.18095577612054
12:53:35 DEBUG opendrift.models.basemodel:2062: 23.176641796705844 <- longitude -> 23.604075493463768
12:53:35 DEBUG opendrift.models.basemodel:2067: -107.91755056215723 <- z -> 0.0
12:53:35 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:53:35 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:35 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:53:35 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:35 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:53:35 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:35 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:35 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:53:35 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:35 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:35 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:35 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:35 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:53:35 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:35 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:53:35 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:35 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:35 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:53:35 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:35 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:53:35 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:35 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:35 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:35 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:53:35 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:35 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:53:35 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:35 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:35 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:53:35 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:35 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-08 15:00:00 (before)
2024-11-08 18:00:00 (after)
12:53:35 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
12:53:35 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
12:53:35 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:53:35 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:53:35 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:53:35 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
12:53:35 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 14x14x22) for time after (2024-11-08 18:00:00)
12:53:35 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-08 15:00:00) in space (linearNDFast)
12:53:35 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:35 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-08 18:00:00) in space (linearNDFast)
12:53:35 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:35 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-08 15:00:00, weight 0.71) and
after (2024-11-08 18:00:00, weight 0.29) in time
12:53:35 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:35 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:35 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:35 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:53:35 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:35 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:53:35 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:35 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:35 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:53:35 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:35 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-08 15:00:00 (before)
2024-11-08 18:00:00 (after)
12:53:35 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_wind to retrieve x_wind
12:53:38 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_wind to retrieve y_wind
12:53:40 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:53:40 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:53:40 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:53:40 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 720x361x1) for time after (2024-11-08 18:00:00)
12:53:40 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-08 15:00:00) in space (linearNDFast)
12:53:40 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:40 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-08 18:00:00) in space (linearNDFast)
12:53:40 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:40 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-08 15:00:00, weight 0.71) and
after (2024-11-08 18:00:00, weight 0.29) in time
12:53:40 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:40 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:40 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:53:40 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:53:40 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:53:40 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:53:40 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:53:40 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:53:40 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:53:40 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.605955 (min) 0.103948 (max)
12:53:40 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0453489 (min) 0.21997 (max)
12:53:40 DEBUG opendrift.models.basemodel.environment:905: x_wind: -4.16743 (min) 0.842885 (max)
12:53:40 DEBUG opendrift.models.basemodel.environment:905: y_wind: -6.64048 (min) -0.000121951 (max)
12:53:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:53:40 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:53:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:53:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:53:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:53:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:53:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:53:40 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:53:40 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:53:40 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:53:40 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:53:40 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:53:40 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:53:40 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:53:40 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:53:40 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:53:40 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:53:40 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.008865, mean: 0.174610, max: 1.512001
12:53:40 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:53:40 DEBUG opendrift.models.physics_methods:1061: min: 0.512939, mean: 2.026185, max: 6.698933
12:53:40 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 0.512939, mean: 2.026185, max: 6.698933
12:53:40 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:53:41 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:53:41 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:53:41 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:53:41 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:53:41 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:53:41 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:53:41 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:53:41 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:53:41 DEBUG opendrift.models.physics_methods:1061: min: 0.512939, mean: 2.026185, max: 6.698933
12:53:41 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:53:41 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.006330, dN_50: 0.000497
12:53:41 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:53:41 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.022181140266444386
12:53:41 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:53:41 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:53:41 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:53:41 DEBUG opendrift.models.physics_methods:915: Advecting 2749 of 6000 elements above 0.100m with wind-sheared ocean current (0.018338 m/s - 0.182817 m/s)
12:53:41 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:53:41 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.002407567007829294 and 0.4717615359087869 m/s
12:53:41 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:53:41 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:53:41 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:53:41 INFO opendrift.models.basemodel:2038: 2024-11-08 16:51:18.915878 - step 53 of 120 - 6000 active elements (0 deactivated)
12:53:41 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:53:41 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:53:41 DEBUG opendrift.models.basemodel:2057: 34.930279936258614 <- latitude -> 35.18621238152831
12:53:41 DEBUG opendrift.models.basemodel:2062: 23.172193542091893 <- longitude -> 23.605146846693092
12:53:41 DEBUG opendrift.models.basemodel:2067: -107.1586118848641 <- z -> 0.0
12:53:41 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:53:41 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:41 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:53:41 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:41 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:53:41 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:41 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:41 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:53:41 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:41 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:41 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:41 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:41 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:53:41 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:41 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:53:41 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:41 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:41 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:53:41 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:41 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:53:41 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:41 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:41 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:41 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:53:41 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:41 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:53:41 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:41 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:41 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:53:41 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:41 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-08 15:00:00 (before)
2024-11-08 18:00:00 (after)
12:53:41 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-08 15:00:00) in space (linearNDFast)
12:53:41 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:41 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-08 18:00:00) in space (linearNDFast)
12:53:41 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:41 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-08 15:00:00, weight 0.38) and
after (2024-11-08 18:00:00, weight 0.62) in time
12:53:41 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:41 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:41 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:41 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:53:41 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:41 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:53:41 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:41 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:41 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:53:41 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:41 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-08 15:00:00 (before)
2024-11-08 18:00:00 (after)
12:53:41 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-08 15:00:00) in space (linearNDFast)
12:53:41 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:41 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-08 18:00:00) in space (linearNDFast)
12:53:41 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:41 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-08 15:00:00, weight 0.38) and
after (2024-11-08 18:00:00, weight 0.62) in time
12:53:41 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:41 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:41 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:53:41 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:53:41 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:53:41 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:53:41 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:53:41 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:53:41 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:53:41 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.63487 (min) 0.139191 (max)
12:53:41 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0702849 (min) 0.195534 (max)
12:53:41 DEBUG opendrift.models.basemodel.environment:905: x_wind: -4.6469 (min) 0.0530545 (max)
12:53:41 DEBUG opendrift.models.basemodel.environment:905: y_wind: -7.0011 (min) -0.83181 (max)
12:53:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:53:41 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:53:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:53:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:53:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:53:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:53:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:53:41 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:53:41 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:53:41 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:53:41 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:53:41 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:53:41 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:53:41 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:53:41 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:53:41 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:53:41 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:53:41 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.017024, mean: 0.294854, max: 1.716982
12:53:41 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:53:41 DEBUG opendrift.models.physics_methods:1061: min: 0.710817, mean: 2.778510, max: 7.138589
12:53:41 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 0.710817, mean: 2.778510, max: 7.138589
12:53:41 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:53:41 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:53:41 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:53:41 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:53:41 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:53:41 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:53:41 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:53:41 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:53:41 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:53:41 DEBUG opendrift.models.physics_methods:1061: min: 0.710817, mean: 2.778510, max: 7.138589
12:53:41 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:53:41 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.003964, dN_50: 0.000311
12:53:41 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:53:41 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.025187643535617636
12:53:41 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:53:41 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:53:41 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:53:41 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2750 surface elements
12:53:41 DEBUG opendrift.models.physics_methods:915: Advecting 2750 of 6000 elements above 0.100m with wind-sheared ocean current (0.025665 m/s - 0.223825 m/s)
12:53:41 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:53:41 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.00040337052940982333 and 0.4791670141124311 m/s
12:53:41 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:53:41 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:53:41 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:53:41 INFO opendrift.models.basemodel:2038: 2024-11-08 17:51:18.915878 - step 54 of 120 - 6000 active elements (0 deactivated)
12:53:41 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:53:41 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:53:41 DEBUG opendrift.models.basemodel:2057: 34.927595112333876 <- latitude -> 35.18691155894208
12:53:41 DEBUG opendrift.models.basemodel:2062: 23.16070332452475 <- longitude -> 23.59604740483601
12:53:41 DEBUG opendrift.models.basemodel:2067: -105.98493489966876 <- z -> 0.0
12:53:41 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:53:41 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:41 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:53:41 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:41 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:53:41 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:41 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:41 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:53:41 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:41 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:41 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:41 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:41 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:53:41 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:41 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:53:41 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:41 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:41 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:53:41 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:41 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:53:41 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:41 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:41 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:41 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:53:41 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:41 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:53:41 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:41 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:41 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:53:41 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:41 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-08 15:00:00 (before)
2024-11-08 18:00:00 (after)
12:53:41 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-08 15:00:00) in space (linearNDFast)
12:53:41 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:41 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-08 18:00:00) in space (linearNDFast)
12:53:41 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:41 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-08 15:00:00, weight 0.05) and
after (2024-11-08 18:00:00, weight 0.95) in time
12:53:41 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:41 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:41 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:41 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:53:41 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:41 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:53:41 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:41 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:41 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:53:41 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:41 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-08 15:00:00 (before)
2024-11-08 18:00:00 (after)
12:53:41 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-08 15:00:00) in space (linearNDFast)
12:53:41 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:41 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-08 18:00:00) in space (linearNDFast)
12:53:41 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:41 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-08 15:00:00, weight 0.05) and
after (2024-11-08 18:00:00, weight 0.95) in time
12:53:41 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:41 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:41 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:53:41 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:53:41 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:53:41 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:53:41 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:53:41 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:53:41 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:53:41 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.569941 (min) 0.15612 (max)
12:53:41 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0617921 (min) 0.177317 (max)
12:53:41 DEBUG opendrift.models.basemodel.environment:905: x_wind: -5.14436 (min) -0.680735 (max)
12:53:41 DEBUG opendrift.models.basemodel.environment:905: y_wind: -7.49062 (min) -1.67526 (max)
12:53:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:53:41 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:53:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:53:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:53:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:53:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:53:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:53:41 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:53:41 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:53:41 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:53:41 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:53:41 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:53:41 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:53:41 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:53:41 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:53:41 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:53:41 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:53:41 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.082870, mean: 0.452524, max: 2.031317
12:53:41 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:53:41 DEBUG opendrift.models.physics_methods:1061: min: 1.568293, mean: 3.539143, max: 7.764587
12:53:41 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.568293, mean: 3.539143, max: 7.764587
12:53:41 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:53:41 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:53:41 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:53:41 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:53:41 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:53:41 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:53:41 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:53:41 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:53:41 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:53:41 DEBUG opendrift.models.physics_methods:1061: min: 1.568293, mean: 3.539143, max: 7.764587
12:53:41 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:53:41 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.003026, dN_50: 0.000237
12:53:41 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:53:41 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.029798081183771502
12:53:41 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:53:41 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:53:41 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:53:41 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2749 surface elements
12:53:41 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2748 surface elements
12:53:41 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2748 surface elements
12:53:41 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2747 surface elements
12:53:41 DEBUG opendrift.models.physics_methods:915: Advecting 2747 of 6000 elements above 0.100m with wind-sheared ocean current (0.055446 m/s - 0.245213 m/s)
12:53:41 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:53:41 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0016007632476944386 and 0.43005744645727206 m/s
12:53:41 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:53:41 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:53:41 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:53:41 INFO opendrift.models.basemodel:2038: 2024-11-08 18:51:18.915878 - step 55 of 120 - 6000 active elements (0 deactivated)
12:53:41 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:53:41 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:53:41 DEBUG opendrift.models.basemodel:2057: 34.925004504661025 <- latitude -> 35.18698953219759
12:53:41 DEBUG opendrift.models.basemodel:2062: 23.142779947093917 <- longitude -> 23.596851080298098
12:53:41 DEBUG opendrift.models.basemodel:2067: -105.03421869351122 <- z -> 0.0
12:53:41 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:53:41 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:41 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:53:41 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:41 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:53:41 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:41 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:41 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:53:41 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:41 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:41 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:41 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:41 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:53:41 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:41 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:53:41 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:41 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:41 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:53:41 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:41 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:53:41 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:41 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:41 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:41 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:53:41 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:41 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:53:41 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:41 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:41 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:53:41 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:41 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-08 18:00:00 (before)
2024-11-08 21:00:00 (after)
12:53:41 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
12:53:41 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
12:53:41 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:53:41 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:53:41 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:53:41 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
12:53:41 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 14x14x22) for time after (2024-11-08 21:00:00)
12:53:41 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-08 18:00:00) in space (linearNDFast)
12:53:41 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:41 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-08 21:00:00) in space (linearNDFast)
12:53:41 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:42 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-08 18:00:00, weight 0.71) and
after (2024-11-08 21:00:00, weight 0.29) in time
12:53:42 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:42 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:42 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:42 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:53:42 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:42 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:53:42 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:42 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:42 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:53:42 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:42 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-08 18:00:00 (before)
2024-11-08 21:00:00 (after)
12:53:42 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_wind to retrieve x_wind
12:53:44 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_wind to retrieve y_wind
12:53:46 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:53:46 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:53:46 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:53:46 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 720x361x1) for time after (2024-11-08 21:00:00)
12:53:46 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-08 18:00:00) in space (linearNDFast)
12:53:46 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:46 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-08 21:00:00) in space (linearNDFast)
12:53:46 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:46 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-08 18:00:00, weight 0.71) and
after (2024-11-08 21:00:00, weight 0.29) in time
12:53:46 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:46 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:46 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:53:46 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:53:46 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:53:46 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:53:46 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:53:46 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:53:46 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:53:46 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.589643 (min) 0.15487 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0995629 (min) 0.13901 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: x_wind: -5.42142 (min) -0.907163 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: y_wind: -7.86461 (min) -2.33497 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:53:46 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.157124, mean: 0.586825, max: 2.234965
12:53:46 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:53:46 DEBUG opendrift.models.physics_methods:1061: min: 2.159492, mean: 4.081149, max: 8.144510
12:53:46 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.159492, mean: 4.081149, max: 8.144510
12:53:46 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:53:46 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:53:46 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:53:46 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:53:46 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:53:46 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:53:46 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:53:46 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:53:46 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:53:46 DEBUG opendrift.models.physics_methods:1061: min: 2.159492, mean: 4.081149, max: 8.144509
12:53:46 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:53:46 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.002695, dN_50: 0.000211
12:53:46 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:53:46 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.032785049021387826
12:53:46 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:53:46 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:53:46 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2746 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2746 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2745 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2744 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2743 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2742 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2741 surface elements
12:53:46 DEBUG opendrift.models.physics_methods:915: Advecting 2740 of 6000 elements above 0.100m with wind-sheared ocean current (0.077596 m/s - 0.255438 m/s)
12:53:46 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:53:46 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.001834535048097319 and 0.4299819225068871 m/s
12:53:46 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:53:46 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:53:46 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:53:46 INFO opendrift.models.basemodel:2038: 2024-11-08 19:51:18.915878 - step 56 of 120 - 6000 active elements (0 deactivated)
12:53:46 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:53:46 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:53:46 DEBUG opendrift.models.basemodel:2057: 34.92111038126937 <- latitude -> 35.183513113644416
12:53:46 DEBUG opendrift.models.basemodel:2062: 23.121680460532318 <- longitude -> 23.600462094193045
12:53:46 DEBUG opendrift.models.basemodel:2067: -104.13289087828163 <- z -> 0.0
12:53:46 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:53:46 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:46 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:53:46 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:46 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:53:46 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:46 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:46 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:53:46 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:46 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:46 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:46 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:46 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:53:46 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:46 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:53:46 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:46 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:46 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:53:46 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:46 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:53:46 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:46 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:46 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:46 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:53:46 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:46 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:53:46 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:46 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:46 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:53:46 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:46 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-08 18:00:00 (before)
2024-11-08 21:00:00 (after)
12:53:46 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-08 18:00:00) in space (linearNDFast)
12:53:46 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:46 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-08 21:00:00) in space (linearNDFast)
12:53:46 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:46 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-08 18:00:00, weight 0.38) and
after (2024-11-08 21:00:00, weight 0.62) in time
12:53:46 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:46 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:46 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:46 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:53:46 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:46 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:53:46 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:46 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:46 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:53:46 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:46 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-08 18:00:00 (before)
2024-11-08 21:00:00 (after)
12:53:46 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-08 18:00:00) in space (linearNDFast)
12:53:46 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:46 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-08 21:00:00) in space (linearNDFast)
12:53:46 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:46 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-08 18:00:00, weight 0.38) and
after (2024-11-08 21:00:00, weight 0.62) in time
12:53:46 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:46 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:46 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:53:46 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:53:46 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:53:46 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:53:46 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:53:46 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:53:46 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:53:46 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.584901 (min) 0.148234 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.139201 (min) 0.105351 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: x_wind: -5.6286 (min) -1.07008 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: y_wind: -8.19633 (min) -2.93667 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:53:46 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.241363, mean: 0.729321, max: 2.423769
12:53:46 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:53:46 DEBUG opendrift.models.physics_methods:1061: min: 2.676487, mean: 4.584726, max: 8.481549
12:53:46 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.676487, mean: 4.584726, max: 8.481549
12:53:46 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:53:46 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:53:46 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:53:46 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:53:46 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:53:46 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:53:46 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:53:46 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:53:46 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:53:46 DEBUG opendrift.models.physics_methods:1061: min: 2.676487, mean: 4.584725, max: 8.481549
12:53:46 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:53:46 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.002510, dN_50: 0.000197
12:53:46 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:53:46 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.03555428899197115
12:53:46 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:53:46 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:53:46 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2740 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2739 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2738 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2736 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2733 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2732 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2729 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2728 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2727 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2726 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2725 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2724 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2722 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2721 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2720 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2719 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2718 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2716 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2715 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2714 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2713 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2712 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2711 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2710 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2709 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2707 surface elements
12:53:46 DEBUG opendrift.models.physics_methods:915: Advecting 2707 of 6000 elements above 0.100m with wind-sheared ocean current (0.099929 m/s - 0.263485 m/s)
12:53:46 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:53:46 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0036060686880969956 and 0.41123880626060894 m/s
12:53:46 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:53:46 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:53:46 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:53:46 INFO opendrift.models.basemodel:2038: 2024-11-08 20:51:18.915878 - step 57 of 120 - 6000 active elements (0 deactivated)
12:53:46 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:53:46 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:53:46 DEBUG opendrift.models.basemodel:2057: 34.914176191841825 <- latitude -> 35.1811438553888
12:53:46 DEBUG opendrift.models.basemodel:2062: 23.10404167719851 <- longitude -> 23.604123888881613
12:53:46 DEBUG opendrift.models.basemodel:2067: -103.75950312949419 <- z -> 0.0
12:53:46 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:53:46 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:46 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:53:46 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:46 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:53:46 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:46 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:46 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:53:46 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:46 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:46 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:46 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:46 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:53:46 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:46 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:53:46 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:46 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:46 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:53:46 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:46 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:53:46 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:46 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:46 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:46 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:53:46 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:46 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:53:46 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:46 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:46 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:53:46 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:46 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-08 18:00:00 (before)
2024-11-08 21:00:00 (after)
12:53:46 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-08 18:00:00) in space (linearNDFast)
12:53:46 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:46 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-08 21:00:00) in space (linearNDFast)
12:53:46 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:46 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-08 18:00:00, weight 0.05) and
after (2024-11-08 21:00:00, weight 0.95) in time
12:53:46 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:46 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:46 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:46 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:53:46 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:46 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:53:46 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:46 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:46 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:53:46 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:46 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-08 18:00:00 (before)
2024-11-08 21:00:00 (after)
12:53:46 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-08 18:00:00) in space (linearNDFast)
12:53:46 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:46 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-08 21:00:00) in space (linearNDFast)
12:53:46 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:46 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-08 18:00:00, weight 0.05) and
after (2024-11-08 21:00:00, weight 0.95) in time
12:53:46 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:46 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:46 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:53:46 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:53:46 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:53:46 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:53:46 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:53:46 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:53:46 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:53:46 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.56938 (min) 0.130665 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.19687 (min) 0.0691757 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: x_wind: -5.81335 (min) -1.18961 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: y_wind: -8.43761 (min) -3.54046 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:53:46 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:53:46 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.344018, mean: 0.886827, max: 2.581680
12:53:46 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:53:46 DEBUG opendrift.models.physics_methods:1061: min: 3.195362, mean: 5.080695, max: 8.753481
12:53:46 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.195362, mean: 5.080695, max: 8.753481
12:53:46 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:53:46 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:53:46 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:53:46 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:53:46 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:53:46 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:53:46 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:53:46 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:53:46 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:53:46 DEBUG opendrift.models.physics_methods:1061: min: 3.195362, mean: 5.080695, max: 8.753481
12:53:46 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:53:46 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.002400, dN_50: 0.000188
12:53:46 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:53:46 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.03787041342400034
12:53:46 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:53:46 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:53:46 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2706 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2705 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2702 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2700 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2699 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2697 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2696 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2695 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2694 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2692 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2690 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2689 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2687 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2683 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2682 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2681 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2681 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2680 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2679 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2678 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2677 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2676 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2675 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2674 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2672 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2671 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2670 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2668 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2667 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2666 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2665 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2664 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2663 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2662 surface elements
12:53:46 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2661 surface elements
12:53:46 DEBUG opendrift.models.physics_methods:915: Advecting 2662 of 6000 elements above 0.100m with wind-sheared ocean current (0.016029 m/s - 0.270300 m/s)
12:53:46 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:53:46 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0005246252744592852 and 0.4440053909665622 m/s
12:53:47 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:53:47 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:53:47 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:53:47 INFO opendrift.models.basemodel:2038: 2024-11-08 21:51:18.915878 - step 58 of 120 - 6000 active elements (0 deactivated)
12:53:47 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:53:47 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:53:47 DEBUG opendrift.models.basemodel:2057: 34.911225961799026 <- latitude -> 35.18148675865277
12:53:47 DEBUG opendrift.models.basemodel:2062: 23.094559908270742 <- longitude -> 23.608286165640504
12:53:47 DEBUG opendrift.models.basemodel:2067: -102.61160282343413 <- z -> 0.0
12:53:47 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:53:47 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:47 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:53:47 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:47 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:53:47 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:47 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:47 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:53:47 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:47 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:47 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:47 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:47 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:53:47 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:47 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:53:47 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:47 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:47 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:53:47 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:47 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:53:47 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:47 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:47 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:47 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:53:47 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:47 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:53:47 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:47 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:47 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:53:47 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:47 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-08 21:00:00 (before)
2024-11-09 00:00:00 (after)
12:53:47 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
12:53:47 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
12:53:47 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:53:47 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:53:47 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:53:47 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
12:53:47 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 15x15x22) for time after (2024-11-09 00:00:00)
12:53:47 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-08 21:00:00) in space (linearNDFast)
12:53:47 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:47 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-09 00:00:00) in space (linearNDFast)
12:53:47 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:47 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-08 21:00:00, weight 0.71) and
after (2024-11-09 00:00:00, weight 0.29) in time
12:53:47 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:47 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:47 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:47 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:53:47 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:47 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:53:47 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:47 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:47 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:53:47 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:47 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-08 21:00:00 (before)
2024-11-09 00:00:00 (after)
12:53:47 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_wind to retrieve x_wind
12:53:50 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_wind to retrieve y_wind
12:53:52 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:53:52 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:53:52 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:53:52 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 720x361x1) for time after (2024-11-09 00:00:00)
12:53:52 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-08 21:00:00) in space (linearNDFast)
12:53:52 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:52 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-09 00:00:00) in space (linearNDFast)
12:53:52 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:52 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-08 21:00:00, weight 0.71) and
after (2024-11-09 00:00:00, weight 0.29) in time
12:53:52 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:52 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:52 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:53:52 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:53:52 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:53:52 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:53:52 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:53:52 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:53:52 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:53:52 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.589103 (min) 0.110233 (max)
12:53:52 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.301143 (min) 0.0607354 (max)
12:53:52 DEBUG opendrift.models.basemodel.environment:905: x_wind: -6.0488 (min) -1.3457 (max)
12:53:52 DEBUG opendrift.models.basemodel.environment:905: y_wind: -8.77185 (min) -3.87192 (max)
12:53:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:53:52 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:53:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:53:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:53:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:53:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:53:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:53:52 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:53:52 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:53:52 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:53:52 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:53:52 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:53:52 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:53:52 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:53:52 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:53:52 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:53:52 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:53:52 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.419193, mean: 1.018943, max: 2.792585
12:53:52 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:53:52 DEBUG opendrift.models.physics_methods:1061: min: 3.527253, mean: 5.454482, max: 9.104012
12:53:52 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.527253, mean: 5.454482, max: 9.104012
12:53:52 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:53:52 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:53:52 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:53:52 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:53:52 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:53:52 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:53:52 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:53:52 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:53:52 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:53:52 DEBUG opendrift.models.physics_methods:1061: min: 3.527253, mean: 5.454482, max: 9.104012
12:53:52 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:53:52 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.002383, dN_50: 0.000187
12:53:52 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:53:53 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.040963823097771745
12:53:53 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:53:53 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:53:53 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2659 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2657 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2656 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2655 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2653 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2650 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2648 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2647 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2646 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2644 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2643 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2642 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2640 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2637 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2635 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2633 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2631 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2630 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2628 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2626 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2624 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2623 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2621 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2620 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2619 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2616 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2615 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2614 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2612 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2610 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2609 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2608 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2607 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2606 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2605 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 6 of 2602 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2596 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2594 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2593 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2591 surface elements
12:53:53 DEBUG opendrift.models.physics_methods:915: Advecting 2596 of 6000 elements above 0.100m with wind-sheared ocean current (0.021790 m/s - 0.279416 m/s)
12:53:53 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:53:53 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0027616545580589754 and 0.4070695727740572 m/s
12:53:53 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:53:53 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:53:53 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:53:53 INFO opendrift.models.basemodel:2038: 2024-11-08 22:51:18.915878 - step 59 of 120 - 6000 active elements (0 deactivated)
12:53:53 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:53:53 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:53:53 DEBUG opendrift.models.basemodel:2057: 34.904516568191454 <- latitude -> 35.18594755918781
12:53:53 DEBUG opendrift.models.basemodel:2062: 23.076922540148335 <- longitude -> 23.60040740569207
12:53:53 DEBUG opendrift.models.basemodel:2067: -101.29053842892905 <- z -> 0.0
12:53:53 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:53:53 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:53 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:53:53 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:53 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:53:53 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:53 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:53 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:53:53 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:53 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:53 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:53 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:53 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:53:53 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:53 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:53:53 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:53 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:53 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:53:53 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:53 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:53:53 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:53 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:53 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:53 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:53:53 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:53 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:53:53 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:53 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:53 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:53:53 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:53 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-08 21:00:00 (before)
2024-11-09 00:00:00 (after)
12:53:53 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-08 21:00:00) in space (linearNDFast)
12:53:53 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:53 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-09 00:00:00) in space (linearNDFast)
12:53:53 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:53 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-08 21:00:00, weight 0.38) and
after (2024-11-09 00:00:00, weight 0.62) in time
12:53:53 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:53 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:53 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:53 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:53:53 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:53 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:53:53 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:53 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:53 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:53:53 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:53 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-08 21:00:00 (before)
2024-11-09 00:00:00 (after)
12:53:53 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-08 21:00:00) in space (linearNDFast)
12:53:53 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:53 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-09 00:00:00) in space (linearNDFast)
12:53:53 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:53 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-08 21:00:00, weight 0.38) and
after (2024-11-09 00:00:00, weight 0.62) in time
12:53:53 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:53 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:53 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:53:53 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:53:53 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:53:53 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:53:53 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:53:53 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:53:53 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:53:53 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.61289 (min) 0.11056 (max)
12:53:53 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.372917 (min) 0.0645016 (max)
12:53:53 DEBUG opendrift.models.basemodel.environment:905: x_wind: -6.29443 (min) -1.58151 (max)
12:53:53 DEBUG opendrift.models.basemodel.environment:905: y_wind: -9.21706 (min) -4.06503 (max)
12:53:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:53:53 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:53:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:53:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:53:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:53:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:53:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:53:53 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:53:53 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:53:53 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:53:53 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:53:53 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:53:53 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:53:53 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:53:53 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:53:53 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:53:53 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:53:53 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.473117, mean: 1.155298, max: 3.064519
12:53:53 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:53:53 DEBUG opendrift.models.physics_methods:1061: min: 3.747258, mean: 5.812975, max: 9.536977
12:53:53 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.747258, mean: 5.812975, max: 9.536977
12:53:53 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:53:53 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:53:53 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:53:53 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:53:53 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:53:53 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:53:53 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:53:53 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:53:53 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:53:53 DEBUG opendrift.models.physics_methods:1061: min: 3.747258, mean: 5.812975, max: 9.536978
12:53:53 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:53:53 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.002397, dN_50: 0.000188
12:53:53 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:53:53 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.044952351110830166
12:53:53 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:53:53 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:53:53 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2590 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2589 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2586 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2583 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2581 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2579 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2578 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2576 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2575 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2574 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2572 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2570 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2566 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2565 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2563 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2560 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2558 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2555 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2554 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2551 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2550 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2549 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2548 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2546 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2542 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2541 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2540 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2537 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2536 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2535 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2533 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2532 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2530 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2528 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2527 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2524 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2523 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2522 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 2520 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2515 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2512 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2509 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2508 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2507 surface elements
12:53:53 DEBUG opendrift.models.physics_methods:915: Advecting 2507 of 6000 elements above 0.100m with wind-sheared ocean current (0.136230 m/s - 0.292505 m/s)
12:53:53 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:53:53 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0030709311183357633 and 0.4937170103328958 m/s
12:53:53 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:53:53 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:53:53 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:53:53 INFO opendrift.models.basemodel:2038: 2024-11-08 23:51:18.915878 - step 60 of 120 - 6000 active elements (0 deactivated)
12:53:53 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:53:53 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:53:53 DEBUG opendrift.models.basemodel:2057: 34.893434229172435 <- latitude -> 35.182519951509875
12:53:53 DEBUG opendrift.models.basemodel:2062: 23.057387181282255 <- longitude -> 23.600396870621758
12:53:53 DEBUG opendrift.models.basemodel:2067: -101.06177922898497 <- z -> 0.0
12:53:53 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:53:53 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:53 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:53:53 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:53 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:53:53 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:53 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:53 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:53:53 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:53 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:53 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:53 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:53 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:53:53 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:53 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:53:53 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:53 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:53 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:53:53 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:53 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:53:53 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:53 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:53 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:53 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:53:53 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:53 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:53:53 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:53 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:53 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:53:53 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:53 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-08 21:00:00 (before)
2024-11-09 00:00:00 (after)
12:53:53 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-08 21:00:00) in space (linearNDFast)
12:53:53 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:53 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-09 00:00:00) in space (linearNDFast)
12:53:53 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:53 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-08 21:00:00, weight 0.05) and
after (2024-11-09 00:00:00, weight 0.95) in time
12:53:53 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:53 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:53 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:53 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:53:53 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:53 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:53:53 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:53 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:53 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:53:53 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:53 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-08 21:00:00 (before)
2024-11-09 00:00:00 (after)
12:53:53 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-08 21:00:00) in space (linearNDFast)
12:53:53 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:53 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-09 00:00:00) in space (linearNDFast)
12:53:53 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:53 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-08 21:00:00, weight 0.05) and
after (2024-11-09 00:00:00, weight 0.95) in time
12:53:53 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:53 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:53 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:53:53 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:53:53 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:53:53 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:53:53 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:53:53 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:53:53 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:53:53 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.638337 (min) 0.10463 (max)
12:53:53 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.479522 (min) 0.0695881 (max)
12:53:53 DEBUG opendrift.models.basemodel.environment:905: x_wind: -6.53799 (min) -1.83558 (max)
12:53:53 DEBUG opendrift.models.basemodel.environment:905: y_wind: -9.67502 (min) -4.31368 (max)
12:53:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:53:53 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:53:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:53:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:53:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:53:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:53:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:53:53 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:53:53 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:53:53 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:53:53 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:53:53 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:53:53 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:53:53 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:53:53 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:53:53 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:53:53 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:53:53 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.544796, mean: 1.301773, max: 3.354244
12:53:53 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:53:53 DEBUG opendrift.models.physics_methods:1061: min: 4.021114, mean: 6.174400, max: 9.977618
12:53:53 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 4.021114, mean: 6.174400, max: 9.977618
12:53:53 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:53:53 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:53:53 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:53:53 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:53:53 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:53:53 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:53:53 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:53:53 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:53:53 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:53:53 DEBUG opendrift.models.physics_methods:1061: min: 4.021114, mean: 6.174400, max: 9.977618
12:53:53 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:53:53 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.002433, dN_50: 0.000191
12:53:53 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:53:53 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.049201820451414885
12:53:53 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:53:53 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:53:53 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2506 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2503 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2501 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2500 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2498 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2497 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2494 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2492 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2488 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2486 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2484 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 2482 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2477 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2475 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2474 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2473 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2472 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2470 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2467 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2466 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2465 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2463 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2461 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2459 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2458 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2456 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2454 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2451 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2450 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2449 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2447 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2444 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2441 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2440 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2439 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2438 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2437 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2434 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2432 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2431 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2428 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2426 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2423 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 2421 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2416 surface elements
12:53:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2414 surface elements
12:53:53 DEBUG opendrift.models.physics_methods:915: Advecting 2412 of 6000 elements above 0.100m with wind-sheared ocean current (0.146613 m/s - 0.307267 m/s)
12:53:53 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:53:53 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0012621703933467497 and 0.4992845709343615 m/s
12:53:53 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:53:53 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:53:53 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:53:53 INFO opendrift.models.basemodel:2038: 2024-11-09 00:51:18.915878 - step 61 of 120 - 6000 active elements (0 deactivated)
12:53:53 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:53:53 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:53:53 DEBUG opendrift.models.basemodel:2057: 34.87972804521162 <- latitude -> 35.187731671238154
12:53:53 DEBUG opendrift.models.basemodel:2062: 23.028668977204894 <- longitude -> 23.602702896823654
12:53:53 DEBUG opendrift.models.basemodel:2067: -100.37579887700659 <- z -> 0.0
12:53:53 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:53:53 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:53 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:53:53 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:53 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:53:53 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:53 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:53 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:53:53 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:53 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:53 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:53 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:53 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:53:53 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:53 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:53:53 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:53 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:53 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:53:53 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:53 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:53:53 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:53 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:53 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:53 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:53:53 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:53 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:53:53 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:53 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:53 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:53:53 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:53 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-09 00:00:00 (before)
2024-11-09 03:00:00 (after)
12:53:53 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
12:53:53 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
12:53:53 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:53:53 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:53:53 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:53:53 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
12:53:53 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 15x16x22) for time after (2024-11-09 03:00:00)
12:53:53 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-09 00:00:00) in space (linearNDFast)
12:53:53 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:53 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-09 03:00:00) in space (linearNDFast)
12:53:53 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:53 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-09 00:00:00, weight 0.71) and
after (2024-11-09 03:00:00, weight 0.29) in time
12:53:53 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:53 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:53 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:53 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:53:53 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:53 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:53:53 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:53 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:53 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:53:53 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:53 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-09 00:00:00 (before)
2024-11-09 03:00:00 (after)
12:53:53 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_wind to retrieve x_wind
12:53:56 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_wind to retrieve y_wind
12:53:59 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:53:59 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:53:59 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:53:59 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 720x361x1) for time after (2024-11-09 03:00:00)
12:53:59 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-09 00:00:00) in space (linearNDFast)
12:53:59 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:59 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-09 03:00:00) in space (linearNDFast)
12:53:59 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:59 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-09 00:00:00, weight 0.71) and
after (2024-11-09 03:00:00, weight 0.29) in time
12:53:59 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:59 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:59 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:53:59 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:53:59 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:53:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:53:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:53:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:53:59 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:53:59 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.65621 (min) 0.0506933 (max)
12:53:59 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.439623 (min) 0.101315 (max)
12:53:59 DEBUG opendrift.models.basemodel.environment:905: x_wind: -6.82107 (min) -1.89971 (max)
12:53:59 DEBUG opendrift.models.basemodel.environment:905: y_wind: -10.0858 (min) -4.53298 (max)
12:53:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:53:59 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:53:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:53:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:53:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:53:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:53:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:53:59 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:53:59 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:53:59 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:53:59 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:53:59 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:53:59 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:53:59 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:53:59 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:53:59 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:53:59 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:53:59 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.594256, mean: 1.391533, max: 3.646934
12:53:59 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:53:59 DEBUG opendrift.models.physics_methods:1061: min: 4.199683, mean: 6.385519, max: 10.403838
12:53:59 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 4.199683, mean: 6.385519, max: 10.403838
12:53:59 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:53:59 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:53:59 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:53:59 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:53:59 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:53:59 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:53:59 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:53:59 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:53:59 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:53:59 DEBUG opendrift.models.physics_methods:1061: min: 4.199683, mean: 6.385519, max: 10.403837
12:53:59 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:53:59 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.002530, dN_50: 0.000199
12:53:59 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:53:59 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.05349479940413533
12:53:59 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:53:59 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:53:59 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2412 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2411 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2410 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2407 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2406 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2405 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2404 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2402 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 2401 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2396 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2393 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2389 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2387 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2385 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2383 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2380 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2380 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2379 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2377 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2376 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2374 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2374 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2373 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2370 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2367 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2366 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2365 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2364 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2361 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2358 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2357 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2354 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2352 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2350 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2349 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2347 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2346 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2344 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2343 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 2341 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2336 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2334 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2332 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2328 surface elements
12:53:59 DEBUG opendrift.models.physics_methods:915: Advecting 2328 of 6000 elements above 0.100m with wind-sheared ocean current (0.152984 m/s - 0.316481 m/s)
12:53:59 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:53:59 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.001994767225313018 and 0.4297921360434717 m/s
12:53:59 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:53:59 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:53:59 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:53:59 INFO opendrift.models.basemodel:2038: 2024-11-09 01:51:18.915878 - step 62 of 120 - 6000 active elements (0 deactivated)
12:53:59 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:53:59 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:53:59 DEBUG opendrift.models.basemodel:2057: 34.864456825551265 <- latitude -> 35.186157971259846
12:53:59 DEBUG opendrift.models.basemodel:2062: 23.00578807195421 <- longitude -> 23.5975204700427
12:53:59 DEBUG opendrift.models.basemodel:2067: -99.31119801110366 <- z -> 0.0
12:53:59 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:53:59 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:59 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:53:59 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:59 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:53:59 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:59 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:59 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:53:59 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:59 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:59 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:59 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:59 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:53:59 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:59 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:53:59 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:59 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:59 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:53:59 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:53:59 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:53:59 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:59 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:59 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:59 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:53:59 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:59 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:53:59 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:59 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:59 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:53:59 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:59 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-09 00:00:00 (before)
2024-11-09 03:00:00 (after)
12:53:59 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-09 00:00:00) in space (linearNDFast)
12:53:59 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:59 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-09 03:00:00) in space (linearNDFast)
12:53:59 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:59 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-09 00:00:00, weight 0.38) and
after (2024-11-09 03:00:00, weight 0.62) in time
12:53:59 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:59 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:59 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:53:59 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:53:59 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:53:59 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:53:59 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:53:59 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:53:59 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:53:59 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:53:59 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-09 00:00:00 (before)
2024-11-09 03:00:00 (after)
12:53:59 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-09 00:00:00) in space (linearNDFast)
12:53:59 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:59 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-09 03:00:00) in space (linearNDFast)
12:53:59 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:53:59 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-09 00:00:00, weight 0.38) and
after (2024-11-09 03:00:00, weight 0.62) in time
12:53:59 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:53:59 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:53:59 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:53:59 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:53:59 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:53:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:53:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:53:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:53:59 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:53:59 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.761913 (min) -0.00998611 (max)
12:53:59 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.470446 (min) 0.137638 (max)
12:53:59 DEBUG opendrift.models.basemodel.environment:905: x_wind: -7.06781 (min) -1.9352 (max)
12:53:59 DEBUG opendrift.models.basemodel.environment:905: y_wind: -10.4207 (min) -4.66203 (max)
12:53:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:53:59 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:53:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:53:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:53:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:53:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:53:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:53:59 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:53:59 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:53:59 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:53:59 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:53:59 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:53:59 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:53:59 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:53:59 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:53:59 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:53:59 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:53:59 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.627201, mean: 1.476157, max: 3.900192
12:53:59 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:53:59 DEBUG opendrift.models.physics_methods:1061: min: 4.314524, mean: 6.578302, max: 10.759016
12:53:59 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 4.314524, mean: 6.578302, max: 10.759016
12:53:59 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:53:59 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:53:59 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:53:59 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:53:59 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:53:59 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:53:59 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:53:59 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:53:59 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:53:59 DEBUG opendrift.models.physics_methods:1061: min: 4.314524, mean: 6.578302, max: 10.759016
12:53:59 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:53:59 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.002644, dN_50: 0.000208
12:53:59 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:53:59 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.05720939419916263
12:53:59 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:53:59 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:53:59 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2326 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2324 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2323 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2321 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 2319 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2314 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 2313 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2309 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2308 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2305 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2304 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2302 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2300 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2299 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2298 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2296 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2295 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2293 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2292 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2291 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2289 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2286 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2283 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2281 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2279 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2277 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2274 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2270 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2268 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2266 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2265 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2263 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2261 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2259 surface elements
12:53:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2255 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2252 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 2252 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2247 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2244 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2243 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2242 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2241 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2239 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2238 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2237 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2235 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2231 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2228 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2225 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2224 surface elements
12:54:00 DEBUG opendrift.models.physics_methods:915: Advecting 2224 of 6000 elements above 0.100m with wind-sheared ocean current (0.067637 m/s - 0.327085 m/s)
12:54:00 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:54:00 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.002026610875415199 and 0.4846622357247117 m/s
12:54:00 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:54:00 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:54:00 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:00 INFO opendrift.models.basemodel:2038: 2024-11-09 02:51:18.915878 - step 63 of 120 - 6000 active elements (0 deactivated)
12:54:00 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:00 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:00 DEBUG opendrift.models.basemodel:2057: 34.85174908422672 <- latitude -> 35.18759017556657
12:54:00 DEBUG opendrift.models.basemodel:2062: 22.983678196935106 <- longitude -> 23.5913069636282
12:54:00 DEBUG opendrift.models.basemodel:2067: -98.63549844755251 <- z -> 0.0
12:54:00 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:00 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:00 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:00 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:00 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:00 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:00 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:00 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:54:00 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:00 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:00 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:00 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:00 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:00 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:00 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:00 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:00 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:00 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:54:00 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:00 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:00 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:00 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:00 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:00 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:54:00 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:00 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:54:00 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:00 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:00 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:54:00 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:54:00 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-09 00:00:00 (before)
2024-11-09 03:00:00 (after)
12:54:00 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-09 00:00:00) in space (linearNDFast)
12:54:00 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:00 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-09 03:00:00) in space (linearNDFast)
12:54:00 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:00 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-09 00:00:00, weight 0.05) and
after (2024-11-09 03:00:00, weight 0.95) in time
12:54:00 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:00 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:00 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:00 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:54:00 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:00 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:54:00 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:00 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:00 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:54:00 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:54:00 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-09 00:00:00 (before)
2024-11-09 03:00:00 (after)
12:54:00 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-09 00:00:00) in space (linearNDFast)
12:54:00 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:00 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-09 03:00:00) in space (linearNDFast)
12:54:00 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:00 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-09 00:00:00, weight 0.05) and
after (2024-11-09 03:00:00, weight 0.95) in time
12:54:00 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:00 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:00 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:00 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:00 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:54:00 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:54:00 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:54:00 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:54:00 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:00 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.803886 (min) -0.0501462 (max)
12:54:00 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.454762 (min) 0.165025 (max)
12:54:00 DEBUG opendrift.models.basemodel.environment:905: x_wind: -7.20909 (min) -1.945 (max)
12:54:00 DEBUG opendrift.models.basemodel.environment:905: y_wind: -10.5572 (min) -4.8533 (max)
12:54:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:54:00 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:54:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:54:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:54:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:54:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:54:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:54:00 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:54:00 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:54:00 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:54:00 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:54:00 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:54:00 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:54:00 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:54:00 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:00 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:54:00 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:00 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.672503, mean: 1.568931, max: 4.000967
12:54:00 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:54:00 DEBUG opendrift.models.physics_methods:1061: min: 4.467624, mean: 6.783256, max: 10.897128
12:54:00 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 4.467624, mean: 6.783256, max: 10.897128
12:54:00 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:54:00 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:00 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:54:00 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:54:00 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:54:00 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:54:00 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:54:00 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:54:00 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:54:00 DEBUG opendrift.models.physics_methods:1061: min: 4.467624, mean: 6.783256, max: 10.897128
12:54:00 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:54:00 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.002766, dN_50: 0.000217
12:54:00 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:54:00 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.0586874799953711
12:54:00 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:00 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:54:00 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2221 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2218 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2217 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2215 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2211 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2210 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2206 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2205 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2204 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2202 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2200 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2197 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2195 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2193 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2192 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2191 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2189 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2187 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2184 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2181 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2179 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 2178 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2173 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2170 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2169 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2166 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2165 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2164 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2162 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2160 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2158 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2157 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2155 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2154 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2153 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2153 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2151 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2150 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 2149 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2144 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2141 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2140 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2139 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2138 surface elements
12:54:00 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2136 surface elements
12:54:00 DEBUG opendrift.models.physics_methods:915: Advecting 2139 of 6000 elements above 0.100m with wind-sheared ocean current (0.020988 m/s - 0.335569 m/s)
12:54:00 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:54:00 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.003418578226658486 and 0.5079824958354799 m/s
12:54:00 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:54:00 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:54:00 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:00 INFO opendrift.models.basemodel:2038: 2024-11-09 03:51:18.915878 - step 64 of 120 - 6000 active elements (0 deactivated)
12:54:00 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:00 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:00 DEBUG opendrift.models.basemodel:2057: 34.83652471939648 <- latitude -> 35.193151108436446
12:54:00 DEBUG opendrift.models.basemodel:2062: 22.952845686297902 <- longitude -> 23.590873310563566
12:54:00 DEBUG opendrift.models.basemodel:2067: -97.46391061697865 <- z -> 0.0
12:54:00 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:00 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:00 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:00 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:00 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:00 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:00 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:00 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:54:00 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:00 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:00 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:00 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:00 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:00 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:00 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:00 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:00 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:00 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:54:00 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:00 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:00 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:00 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:00 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:00 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:54:00 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:00 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:54:00 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:00 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:00 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:54:00 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:54:00 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-09 03:00:00 (before)
2024-11-09 06:00:00 (after)
12:54:00 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
12:54:00 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
12:54:00 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:54:00 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:54:00 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:54:00 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
12:54:00 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 16x17x21) for time after (2024-11-09 06:00:00)
12:54:00 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-09 03:00:00) in space (linearNDFast)
12:54:00 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:00 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-09 06:00:00) in space (linearNDFast)
12:54:00 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:00 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-09 03:00:00, weight 0.71) and
after (2024-11-09 06:00:00, weight 0.29) in time
12:54:00 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:00 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:00 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:00 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:54:00 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:00 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:54:00 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:00 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:00 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:54:00 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:54:00 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-09 03:00:00 (before)
2024-11-09 06:00:00 (after)
12:54:00 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_wind to retrieve x_wind
12:54:03 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_wind to retrieve y_wind
12:54:05 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:54:05 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:54:05 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:54:05 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 720x361x1) for time after (2024-11-09 06:00:00)
12:54:05 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-09 03:00:00) in space (linearNDFast)
12:54:05 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:05 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-09 06:00:00) in space (linearNDFast)
12:54:05 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:05 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-09 03:00:00, weight 0.71) and
after (2024-11-09 06:00:00, weight 0.29) in time
12:54:05 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:05 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:05 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:05 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:05 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:54:05 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:54:05 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:54:05 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:54:05 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:05 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.808519 (min) -0.0532917 (max)
12:54:05 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.431388 (min) 0.188878 (max)
12:54:05 DEBUG opendrift.models.basemodel.environment:905: x_wind: -7.23407 (min) -2.24159 (max)
12:54:05 DEBUG opendrift.models.basemodel.environment:905: y_wind: -10.6106 (min) -5.27264 (max)
12:54:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:54:05 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:54:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:54:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:54:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:54:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:54:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:54:05 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:54:05 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:54:05 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:54:05 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:54:05 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:54:05 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:54:05 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:54:05 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:05 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:54:05 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:05 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.807507, mean: 1.779576, max: 4.027911
12:54:05 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:54:05 DEBUG opendrift.models.physics_methods:1061: min: 4.895564, mean: 7.233740, max: 10.933759
12:54:05 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 4.895564, mean: 7.233740, max: 10.933759
12:54:05 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:54:05 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:05 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:54:05 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:54:05 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:54:05 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:54:05 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:54:05 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:54:05 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:54:05 DEBUG opendrift.models.physics_methods:1061: min: 4.895565, mean: 7.233739, max: 10.933759
12:54:05 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:54:05 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.002830, dN_50: 0.000222
12:54:05 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:54:05 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.05908268025975346
12:54:05 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:05 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:54:05 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2134 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2130 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2127 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2126 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2124 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2123 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2121 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2119 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2118 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2117 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2113 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2112 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2110 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2108 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 2106 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2101 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2098 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2096 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2095 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2093 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2092 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2091 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2089 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2087 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2084 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2082 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2081 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2077 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2075 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2073 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2071 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2069 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2066 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2065 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2063 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2063 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2062 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2059 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2058 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 2057 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2053 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2052 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2051 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2050 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2048 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2045 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2044 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2041 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2038 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2036 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2034 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2033 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2030 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2029 surface elements
12:54:05 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2028 surface elements
12:54:05 DEBUG opendrift.models.physics_methods:915: Advecting 2030 of 6000 elements above 0.100m with wind-sheared ocean current (0.050275 m/s - 0.344648 m/s)
12:54:05 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:54:05 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.00183339485427714 and 0.46673968621477696 m/s
12:54:05 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:54:05 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:54:05 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:05 INFO opendrift.models.basemodel:2038: 2024-11-09 04:51:18.915878 - step 65 of 120 - 6000 active elements (0 deactivated)
12:54:05 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:05 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:05 DEBUG opendrift.models.basemodel:2057: 34.822261380002075 <- latitude -> 35.19250549499338
12:54:05 DEBUG opendrift.models.basemodel:2062: 22.920481634840165 <- longitude -> 23.58098140142575
12:54:05 DEBUG opendrift.models.basemodel:2067: -96.42401190305586 <- z -> 0.0
12:54:05 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:05 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:05 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:05 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:05 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:05 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:05 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:05 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:54:05 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:05 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:05 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:05 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:05 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:05 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:05 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:05 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:05 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:05 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:54:05 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:05 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:05 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:05 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:05 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:05 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:54:05 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:05 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:54:05 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:05 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:05 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:54:05 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:54:05 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-09 03:00:00 (before)
2024-11-09 06:00:00 (after)
12:54:05 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-09 03:00:00) in space (linearNDFast)
12:54:05 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:06 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-09 06:00:00) in space (linearNDFast)
12:54:06 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:06 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-09 03:00:00, weight 0.38) and
after (2024-11-09 06:00:00, weight 0.62) in time
12:54:06 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:06 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:06 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:06 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:54:06 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:06 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:54:06 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:06 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:06 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:54:06 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:54:06 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-09 03:00:00 (before)
2024-11-09 06:00:00 (after)
12:54:06 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-09 03:00:00) in space (linearNDFast)
12:54:06 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:06 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-09 06:00:00) in space (linearNDFast)
12:54:06 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:06 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-09 03:00:00, weight 0.38) and
after (2024-11-09 06:00:00, weight 0.62) in time
12:54:06 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:06 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:06 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:06 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:06 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:54:06 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:54:06 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:54:06 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:54:06 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:06 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.838344 (min) -0.0515531 (max)
12:54:06 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.401609 (min) 0.200426 (max)
12:54:06 DEBUG opendrift.models.basemodel.environment:905: x_wind: -7.26968 (min) -2.61354 (max)
12:54:06 DEBUG opendrift.models.basemodel.environment:905: y_wind: -10.6664 (min) -5.8527 (max)
12:54:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:54:06 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:54:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:54:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:54:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:54:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:54:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:54:06 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:54:06 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:54:06 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:54:06 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:54:06 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:54:06 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:54:06 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:54:06 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:06 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:54:06 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:06 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 1.010684, mean: 2.019733, max: 4.046090
12:54:06 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:54:06 DEBUG opendrift.models.physics_methods:1061: min: 5.476931, mean: 7.715695, max: 10.958405
12:54:06 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 5.476931, mean: 7.715695, max: 10.958405
12:54:06 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:54:06 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:06 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:54:06 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:54:06 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:54:06 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:54:06 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:54:06 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:54:06 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:54:06 DEBUG opendrift.models.physics_methods:1061: min: 5.476932, mean: 7.715695, max: 10.958405
12:54:06 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:54:06 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.002906, dN_50: 0.000228
12:54:06 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:54:06 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.05934932955762638
12:54:06 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:06 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:54:06 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2027 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2026 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2024 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2022 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2020 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2019 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2016 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2014 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2013 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2010 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2009 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 2007 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2002 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2001 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1998 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1996 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1995 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1994 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1992 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1989 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1985 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1984 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1982 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1980 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1976 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1974 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1971 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1968 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1965 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1965 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1961 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1959 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1957 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1955 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1952 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1950 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1949 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1948 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1945 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1944 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1943 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1940 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1937 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 1937 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1932 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1930 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1927 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1924 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1920 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1918 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1916 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1915 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1914 surface elements
12:54:06 DEBUG opendrift.models.physics_methods:915: Advecting 1919 of 6000 elements above 0.100m with wind-sheared ocean current (0.031630 m/s - 0.355434 m/s)
12:54:06 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:54:06 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0024343442443554505 and 0.45456002520331 m/s
12:54:06 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:54:06 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:54:06 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:06 INFO opendrift.models.basemodel:2038: 2024-11-09 05:51:18.915878 - step 66 of 120 - 6000 active elements (0 deactivated)
12:54:06 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:06 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:06 DEBUG opendrift.models.basemodel:2057: 34.81000604521436 <- latitude -> 35.194435719864416
12:54:06 DEBUG opendrift.models.basemodel:2062: 22.892690254714786 <- longitude -> 23.576534881276437
12:54:06 DEBUG opendrift.models.basemodel:2067: -95.65775900807178 <- z -> 0.0
12:54:06 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:06 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:06 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:06 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:06 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:06 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:06 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:06 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:54:06 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:06 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:06 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:06 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:06 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:06 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:06 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:06 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:06 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:06 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:54:06 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:06 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:06 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:06 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:06 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:06 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:54:06 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:06 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:54:06 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:06 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:06 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:54:06 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:54:06 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-09 03:00:00 (before)
2024-11-09 06:00:00 (after)
12:54:06 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-09 03:00:00) in space (linearNDFast)
12:54:06 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:06 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-09 06:00:00) in space (linearNDFast)
12:54:06 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:06 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-09 03:00:00, weight 0.05) and
after (2024-11-09 06:00:00, weight 0.95) in time
12:54:06 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:06 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:06 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:06 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:54:06 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:06 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:54:06 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:06 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:06 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:54:06 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:54:06 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-09 03:00:00 (before)
2024-11-09 06:00:00 (after)
12:54:06 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-09 03:00:00) in space (linearNDFast)
12:54:06 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:06 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-09 06:00:00) in space (linearNDFast)
12:54:06 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:06 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-09 03:00:00, weight 0.05) and
after (2024-11-09 06:00:00, weight 0.95) in time
12:54:06 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:06 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:06 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:06 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:06 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:54:06 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:54:06 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:54:06 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:54:06 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:06 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.824982 (min) -0.0486309 (max)
12:54:06 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.373373 (min) 0.208893 (max)
12:54:06 DEBUG opendrift.models.basemodel.environment:905: x_wind: -7.31471 (min) -2.96308 (max)
12:54:06 DEBUG opendrift.models.basemodel.environment:905: y_wind: -10.6969 (min) -6.37878 (max)
12:54:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:54:06 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:54:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:54:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:54:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:54:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:54:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:54:06 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:54:06 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:54:06 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:54:06 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:54:06 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:54:06 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:54:06 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:54:06 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:06 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:54:06 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:06 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 1.216930, mean: 2.268833, max: 4.057024
12:54:06 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:54:06 DEBUG opendrift.models.physics_methods:1061: min: 6.009832, mean: 8.185416, max: 10.973203
12:54:06 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 6.009832, mean: 8.185416, max: 10.973203
12:54:06 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:54:06 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:06 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:54:06 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:54:06 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:54:06 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:54:06 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:54:06 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:54:06 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:54:06 DEBUG opendrift.models.physics_methods:1061: min: 6.009831, mean: 8.185416, max: 10.973203
12:54:06 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:54:06 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.003004, dN_50: 0.000236
12:54:06 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:54:06 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.05950969567200981
12:54:06 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:06 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:54:06 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1912 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1909 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1907 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1906 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1902 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1898 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1895 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1893 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1892 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1891 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 1888 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1883 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1880 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1879 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1878 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1874 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 1872 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1868 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 1864 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1859 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1858 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1857 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1856 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1853 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1851 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1848 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 6 of 1844 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1838 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1836 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1834 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1832 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1831 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1830 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1826 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 6 of 1825 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1819 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1818 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1814 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1811 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1807 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1806 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1803 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 1801 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1796 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1794 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1792 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1790 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1788 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1787 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1783 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 6 of 1782 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1776 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1773 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1771 surface elements
12:54:06 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1768 surface elements
12:54:06 DEBUG opendrift.models.physics_methods:915: Advecting 1773 of 6000 elements above 0.100m with wind-sheared ocean current (0.044452 m/s - 0.366426 m/s)
12:54:06 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:54:06 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0011189816542274192 and 0.4291704441292908 m/s
12:54:06 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:54:06 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:54:06 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:06 INFO opendrift.models.basemodel:2038: 2024-11-09 06:51:18.915878 - step 67 of 120 - 6000 active elements (0 deactivated)
12:54:06 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:06 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:06 DEBUG opendrift.models.basemodel:2057: 34.80056327937697 <- latitude -> 35.19127775098221
12:54:06 DEBUG opendrift.models.basemodel:2062: 22.865286161583317 <- longitude -> 23.572854789041248
12:54:06 DEBUG opendrift.models.basemodel:2067: -94.48554601584664 <- z -> 0.0
12:54:06 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:06 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:06 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:06 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:06 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:06 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:06 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:06 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:54:06 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:06 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:06 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:06 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:06 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:06 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:06 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:06 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:06 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:06 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:54:06 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:06 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:06 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:06 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:06 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:06 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:54:06 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:06 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:54:06 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:06 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:06 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:54:06 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:54:06 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-09 06:00:00 (before)
2024-11-09 09:00:00 (after)
12:54:06 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
12:54:06 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
12:54:06 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:54:06 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:54:06 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:54:06 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
12:54:06 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 17x18x21) for time after (2024-11-09 09:00:00)
12:54:06 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-09 06:00:00) in space (linearNDFast)
12:54:06 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:06 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-09 09:00:00) in space (linearNDFast)
12:54:06 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:06 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-09 06:00:00, weight 0.71) and
after (2024-11-09 09:00:00, weight 0.29) in time
12:54:06 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:06 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:06 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:06 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:54:06 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:06 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:54:06 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:06 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:06 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:54:06 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:54:06 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-09 06:00:00 (before)
2024-11-09 09:00:00 (after)
12:54:06 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_wind to retrieve x_wind
12:54:09 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_wind to retrieve y_wind
12:54:11 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:54:11 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:54:11 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:54:11 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 720x361x1) for time after (2024-11-09 09:00:00)
12:54:11 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-09 06:00:00) in space (linearNDFast)
12:54:11 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:11 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-09 09:00:00) in space (linearNDFast)
12:54:11 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:11 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-09 06:00:00, weight 0.71) and
after (2024-11-09 09:00:00, weight 0.29) in time
12:54:11 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:11 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:11 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:11 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:11 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:54:11 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:54:11 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:54:11 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:54:11 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:11 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.805629 (min) -0.026571 (max)
12:54:11 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.321189 (min) 0.186409 (max)
12:54:11 DEBUG opendrift.models.basemodel.environment:905: x_wind: -7.0992 (min) -2.89429 (max)
12:54:11 DEBUG opendrift.models.basemodel.environment:905: y_wind: -10.6552 (min) -5.95664 (max)
12:54:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:54:11 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:54:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:54:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:54:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:54:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:54:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:54:11 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:54:11 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:54:11 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:54:11 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:54:11 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:54:11 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:54:11 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:54:11 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:11 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:54:11 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:11 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 1.078918, mean: 2.158237, max: 3.921650
12:54:11 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:54:11 DEBUG opendrift.models.physics_methods:1061: min: 5.658792, mean: 7.979681, max: 10.788572
12:54:11 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 5.658792, mean: 7.979681, max: 10.788572
12:54:11 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:54:11 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:11 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:54:11 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:54:11 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:54:11 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:54:11 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:54:11 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:54:11 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:54:11 DEBUG opendrift.models.physics_methods:1061: min: 5.658792, mean: 7.979681, max: 10.788571
12:54:11 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:54:11 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.003291, dN_50: 0.000258
12:54:11 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:54:11 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.057524122305940416
12:54:11 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:11 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:54:11 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:54:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1765 surface elements
12:54:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1764 surface elements
12:54:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 1762 surface elements
12:54:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1757 surface elements
12:54:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1755 surface elements
12:54:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1754 surface elements
12:54:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1752 surface elements
12:54:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1750 surface elements
12:54:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1749 surface elements
12:54:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1746 surface elements
12:54:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1744 surface elements
12:54:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1741 surface elements
12:54:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1738 surface elements
12:54:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1737 surface elements
12:54:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1734 surface elements
12:54:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1730 surface elements
12:54:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1729 surface elements
12:54:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1728 surface elements
12:54:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1726 surface elements
12:54:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1725 surface elements
12:54:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1721 surface elements
12:54:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1720 surface elements
12:54:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1718 surface elements
12:54:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1717 surface elements
12:54:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1715 surface elements
12:54:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1713 surface elements
12:54:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1711 surface elements
12:54:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1708 surface elements
12:54:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1706 surface elements
12:54:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1704 surface elements
12:54:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1703 surface elements
12:54:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1702 surface elements
12:54:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1700 surface elements
12:54:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1698 surface elements
12:54:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1694 surface elements
12:54:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1691 surface elements
12:54:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1689 surface elements
12:54:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1687 surface elements
12:54:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1685 surface elements
12:54:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1682 surface elements
12:54:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1681 surface elements
12:54:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1679 surface elements
12:54:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1675 surface elements
12:54:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1673 surface elements
12:54:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1669 surface elements
12:54:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1666 surface elements
12:54:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1664 surface elements
12:54:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1661 surface elements
12:54:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1659 surface elements
12:54:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1657 surface elements
12:54:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1657 surface elements
12:54:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 1655 surface elements
12:54:11 DEBUG opendrift.models.physics_methods:915: Advecting 1655 of 6000 elements above 0.100m with wind-sheared ocean current (0.010825 m/s - 0.360114 m/s)
12:54:11 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:54:11 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.002490704636796676 and 0.46934487437515293 m/s
12:54:11 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:54:11 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:54:11 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:11 INFO opendrift.models.basemodel:2038: 2024-11-09 07:51:18.915878 - step 68 of 120 - 6000 active elements (0 deactivated)
12:54:11 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:11 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:11 DEBUG opendrift.models.basemodel:2057: 34.782719991996764 <- latitude -> 35.18427452289563
12:54:11 DEBUG opendrift.models.basemodel:2062: 22.841382298276912 <- longitude -> 23.57414010078703
12:54:11 DEBUG opendrift.models.basemodel:2067: -93.20969435400293 <- z -> 0.0
12:54:11 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:11 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:11 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:11 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:11 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:11 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:11 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:11 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:54:11 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:11 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:11 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:11 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:11 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:11 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:11 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:11 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:11 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:11 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:54:11 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:11 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:11 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:11 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:11 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:11 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:54:11 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:11 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:54:11 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:11 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:11 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:54:11 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:54:11 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-09 06:00:00 (before)
2024-11-09 09:00:00 (after)
12:54:11 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-09 06:00:00) in space (linearNDFast)
12:54:11 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:11 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-09 09:00:00) in space (linearNDFast)
12:54:11 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:12 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-09 06:00:00, weight 0.38) and
after (2024-11-09 09:00:00, weight 0.62) in time
12:54:12 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:12 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:12 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:54:12 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:54:12 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:12 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:54:12 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:54:12 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-09 06:00:00 (before)
2024-11-09 09:00:00 (after)
12:54:12 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-09 06:00:00) in space (linearNDFast)
12:54:12 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:12 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-09 09:00:00) in space (linearNDFast)
12:54:12 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:12 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-09 06:00:00, weight 0.38) and
after (2024-11-09 09:00:00, weight 0.62) in time
12:54:12 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:12 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:12 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:12 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:54:12 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:54:12 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:54:12 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:54:12 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:12 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.787087 (min) -0.00246894 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.304675 (min) 0.184176 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: x_wind: -6.84153 (min) -2.69115 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: y_wind: -10.609 (min) -5.28573 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:12 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.865458, mean: 1.998160, max: 3.781818
12:54:12 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:54:12 DEBUG opendrift.models.physics_methods:1061: min: 5.068188, mean: 7.671852, max: 10.594487
12:54:12 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 5.068188, mean: 7.671852, max: 10.594487
12:54:12 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:54:12 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:12 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:54:12 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:54:12 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:54:12 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:54:12 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:54:12 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:54:12 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:54:12 DEBUG opendrift.models.physics_methods:1061: min: 5.068188, mean: 7.671852, max: 10.594486
12:54:12 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:54:12 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.003621, dN_50: 0.000284
12:54:12 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:54:12 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.05547318051603109
12:54:12 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:12 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:54:12 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1650 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1648 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1646 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1645 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1644 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 8 of 1642 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1635 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1634 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1632 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1631 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1630 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1628 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1626 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1625 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1624 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1623 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1622 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1621 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1619 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1616 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1615 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1613 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1611 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1611 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1609 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1607 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1606 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1603 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1604 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1603 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1601 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1599 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1598 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1597 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1594 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1592 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1590 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1588 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1586 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1584 surface elements
12:54:12 DEBUG opendrift.models.physics_methods:915: Advecting 1585 of 6000 elements above 0.100m with wind-sheared ocean current (0.078703 m/s - 0.360423 m/s)
12:54:12 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:54:12 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0021983966686998676 and 0.4543026735095814 m/s
12:54:12 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:54:12 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:54:12 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:12 INFO opendrift.models.basemodel:2038: 2024-11-09 08:51:18.915878 - step 69 of 120 - 6000 active elements (0 deactivated)
12:54:12 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:12 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:12 DEBUG opendrift.models.basemodel:2057: 34.767142477727376 <- latitude -> 35.19215234988573
12:54:12 DEBUG opendrift.models.basemodel:2062: 22.825245331034967 <- longitude -> 23.567395857750896
12:54:12 DEBUG opendrift.models.basemodel:2067: -92.44884562747401 <- z -> 0.0
12:54:12 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:12 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:12 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:12 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:54:12 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:12 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:12 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:12 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:12 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:12 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:12 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:54:12 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:12 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:12 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:12 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:12 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:54:12 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:54:12 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:12 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:54:12 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:54:12 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-09 06:00:00 (before)
2024-11-09 09:00:00 (after)
12:54:12 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-09 06:00:00) in space (linearNDFast)
12:54:12 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:12 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-09 09:00:00) in space (linearNDFast)
12:54:12 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:12 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-09 06:00:00, weight 0.05) and
after (2024-11-09 09:00:00, weight 0.95) in time
12:54:12 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:12 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:12 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:54:12 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:54:12 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:12 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:54:12 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:54:12 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-09 06:00:00 (before)
2024-11-09 09:00:00 (after)
12:54:12 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-09 06:00:00) in space (linearNDFast)
12:54:12 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:12 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-09 09:00:00) in space (linearNDFast)
12:54:12 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:12 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-09 06:00:00, weight 0.05) and
after (2024-11-09 09:00:00, weight 0.95) in time
12:54:12 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:12 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:12 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:12 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:54:12 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:54:12 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:54:12 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:54:12 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:12 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.731097 (min) 0.0241372 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.3238 (min) 0.191848 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: x_wind: -6.56501 (min) -2.56335 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: y_wind: -10.5424 (min) -4.78905 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:54:12 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:12 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.725843, mean: 1.849269, max: 3.644351
12:54:12 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:54:12 DEBUG opendrift.models.physics_methods:1061: min: 4.641419, mean: 7.373248, max: 10.400152
12:54:12 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 4.641419, mean: 7.373248, max: 10.400152
12:54:12 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:54:12 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:12 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:54:12 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:54:12 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:54:12 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:54:12 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:54:12 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:54:12 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:54:12 DEBUG opendrift.models.physics_methods:1061: min: 4.641419, mean: 7.373249, max: 10.400151
12:54:12 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:54:12 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.003966, dN_50: 0.000311
12:54:12 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:54:12 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.053456902570746874
12:54:12 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:12 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:54:12 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1583 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1582 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1578 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1577 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1576 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1575 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1574 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1573 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1572 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1571 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1568 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1566 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1565 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1564 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1563 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1561 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1559 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1557 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1556 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1555 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1554 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1553 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1551 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1549 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1548 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1546 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1544 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1541 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1539 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1537 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1536 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1533 surface elements
12:54:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1531 surface elements
12:54:12 DEBUG opendrift.models.physics_methods:915: Advecting 1537 of 6000 elements above 0.100m with wind-sheared ocean current (0.030927 m/s - 0.362908 m/s)
12:54:12 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:54:12 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.001693953406194447 and 0.472667798760554 m/s
12:54:12 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:54:12 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:54:12 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:12 INFO opendrift.models.basemodel:2038: 2024-11-09 09:51:18.915878 - step 70 of 120 - 6000 active elements (0 deactivated)
12:54:12 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:12 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:12 DEBUG opendrift.models.basemodel:2057: 34.755449065025296 <- latitude -> 35.18918573047909
12:54:12 DEBUG opendrift.models.basemodel:2062: 22.80302762451328 <- longitude -> 23.56685875957656
12:54:12 DEBUG opendrift.models.basemodel:2067: -91.73165918450407 <- z -> 0.0
12:54:12 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:12 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:12 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:12 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:54:12 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:12 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:12 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:12 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:12 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:12 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:12 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:54:12 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:12 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:12 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:12 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:12 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:54:12 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:54:12 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:12 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:54:12 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:54:12 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-09 09:00:00 (before)
2024-11-09 12:00:00 (after)
12:54:12 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
12:54:12 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
12:54:12 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:54:12 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:54:12 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:54:12 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
12:54:12 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 17x19x21) for time after (2024-11-09 12:00:00)
12:54:12 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-09 09:00:00) in space (linearNDFast)
12:54:12 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:12 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-09 12:00:00) in space (linearNDFast)
12:54:12 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:12 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-09 09:00:00, weight 0.71) and
after (2024-11-09 12:00:00, weight 0.29) in time
12:54:12 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:12 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:12 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:54:12 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:54:12 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:12 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:12 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:54:12 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:54:12 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-09 09:00:00 (before)
2024-11-09 12:00:00 (after)
12:54:12 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_wind to retrieve x_wind
12:54:15 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_wind to retrieve y_wind
12:54:18 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:54:18 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:54:18 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:54:18 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 720x361x1) for time after (2024-11-09 12:00:00)
12:54:18 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-09 09:00:00) in space (linearNDFast)
12:54:18 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:18 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-09 12:00:00) in space (linearNDFast)
12:54:18 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:18 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-09 09:00:00, weight 0.71) and
after (2024-11-09 12:00:00, weight 0.29) in time
12:54:18 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:18 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:18 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:18 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:18 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:54:18 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:54:18 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:54:18 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:54:18 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:18 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.709068 (min) 0.040569 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.344998 (min) 0.160585 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: x_wind: -6.3057 (min) -2.00239 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: y_wind: -10.6915 (min) -5.19813 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:18 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.763340, mean: 1.901907, max: 3.617378
12:54:18 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:54:18 DEBUG opendrift.models.physics_methods:1061: min: 4.759799, mean: 7.479903, max: 10.361593
12:54:18 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 4.759799, mean: 7.479903, max: 10.361593
12:54:18 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:54:18 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:18 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:54:18 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:54:18 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:54:18 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:54:18 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:54:18 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:54:18 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:54:18 DEBUG opendrift.models.physics_methods:1061: min: 4.759799, mean: 7.479903, max: 10.361592
12:54:18 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:54:18 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.004160, dN_50: 0.000326
12:54:18 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:54:18 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.053061282758204376
12:54:18 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:18 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:54:18 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1530 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1529 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1528 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1527 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1524 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1521 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1520 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1519 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1518 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1517 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1515 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1514 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1513 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1510 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1509 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1508 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1506 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1506 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1506 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1504 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1503 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1502 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1501 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1500 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1498 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1497 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1494 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1491 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1489 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1489 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1488 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1488 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1486 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1482 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1481 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1480 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1479 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1477 surface elements
12:54:18 DEBUG opendrift.models.physics_methods:915: Advecting 1486 of 6000 elements above 0.100m with wind-sheared ocean current (0.003057 m/s - 0.363456 m/s)
12:54:18 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:54:18 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0009626169875346165 and 0.4311712109253261 m/s
12:54:18 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:54:18 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:54:18 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:18 INFO opendrift.models.basemodel:2038: 2024-11-09 10:51:18.915878 - step 71 of 120 - 6000 active elements (0 deactivated)
12:54:18 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:18 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:18 DEBUG opendrift.models.basemodel:2057: 34.745754885904724 <- latitude -> 35.18084485560645
12:54:18 DEBUG opendrift.models.basemodel:2062: 22.78657537977277 <- longitude -> 23.569956832066236
12:54:18 DEBUG opendrift.models.basemodel:2067: -90.75178547801174 <- z -> 0.0
12:54:18 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:18 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:18 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:18 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:18 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:18 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:18 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:18 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:54:18 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:18 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:18 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:18 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:18 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:18 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:18 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:18 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:18 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:18 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:54:18 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:18 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:18 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:18 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:18 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:18 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:54:18 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:18 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:54:18 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:18 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:18 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:54:18 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:54:18 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-09 09:00:00 (before)
2024-11-09 12:00:00 (after)
12:54:18 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-09 09:00:00) in space (linearNDFast)
12:54:18 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:18 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-09 12:00:00) in space (linearNDFast)
12:54:18 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:18 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-09 09:00:00, weight 0.38) and
after (2024-11-09 12:00:00, weight 0.62) in time
12:54:18 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:18 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:18 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:18 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:54:18 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:18 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:54:18 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:18 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:18 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:54:18 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:54:18 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-09 09:00:00 (before)
2024-11-09 12:00:00 (after)
12:54:18 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-09 09:00:00) in space (linearNDFast)
12:54:18 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:18 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-09 12:00:00) in space (linearNDFast)
12:54:18 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:18 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-09 09:00:00, weight 0.38) and
after (2024-11-09 12:00:00, weight 0.62) in time
12:54:18 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:18 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:18 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:18 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:18 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:54:18 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:54:18 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:54:18 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:54:18 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:18 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.697188 (min) 0.0562771 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.433994 (min) 0.149671 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: x_wind: -6.04825 (min) -1.32516 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: y_wind: -10.8111 (min) -5.66747 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:18 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.833356, mean: 1.999760, max: 3.592421
12:54:18 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:54:18 DEBUG opendrift.models.physics_methods:1061: min: 4.973301, mean: 7.674681, max: 10.325788
12:54:18 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 4.973301, mean: 7.674681, max: 10.325788
12:54:18 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:54:18 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:18 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:54:18 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:54:18 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:54:18 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:54:18 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:54:18 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:54:18 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:54:18 DEBUG opendrift.models.physics_methods:1061: min: 4.973301, mean: 7.674681, max: 10.325788
12:54:18 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:54:18 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.004314, dN_50: 0.000339
12:54:18 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:54:18 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.05269524285375837
12:54:18 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:18 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:54:18 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1476 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1475 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1474 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1472 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1471 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1469 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1468 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1468 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1467 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1466 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1466 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1465 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1462 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1461 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1458 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1456 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1454 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1453 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1452 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1451 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1447 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1446 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1445 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1444 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1443 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1442 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1441 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1440 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1440 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1441 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1440 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1439 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1437 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1435 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1434 surface elements
12:54:18 DEBUG opendrift.models.physics_methods:915: Advecting 1445 of 6000 elements above 0.100m with wind-sheared ocean current (0.000700 m/s - 0.361341 m/s)
12:54:18 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:54:18 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0021707159344100634 and 0.47709524736647557 m/s
12:54:18 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:54:18 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:54:18 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:18 INFO opendrift.models.basemodel:2038: 2024-11-09 11:51:18.915878 - step 72 of 120 - 6000 active elements (0 deactivated)
12:54:18 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:18 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:18 DEBUG opendrift.models.basemodel:2057: 34.73588216205062 <- latitude -> 35.18059012719688
12:54:18 DEBUG opendrift.models.basemodel:2062: 22.76535006470634 <- longitude -> 23.562747493190088
12:54:18 DEBUG opendrift.models.basemodel:2067: -89.99411286044845 <- z -> 0.0
12:54:18 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:18 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:18 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:18 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:18 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:18 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:18 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:18 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:54:18 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:18 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:18 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:18 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:18 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:18 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:18 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:18 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:18 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:18 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:54:18 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:18 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:18 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:18 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:18 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:18 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:54:18 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:18 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:54:18 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:18 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:18 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:54:18 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:54:18 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-09 09:00:00 (before)
2024-11-09 12:00:00 (after)
12:54:18 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-09 09:00:00) in space (linearNDFast)
12:54:18 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:18 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-09 12:00:00) in space (linearNDFast)
12:54:18 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:18 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-09 09:00:00, weight 0.05) and
after (2024-11-09 12:00:00, weight 0.95) in time
12:54:18 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:18 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:18 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:18 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:54:18 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:18 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:54:18 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:18 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:18 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:54:18 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:54:18 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-09 09:00:00 (before)
2024-11-09 12:00:00 (after)
12:54:18 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-09 09:00:00) in space (linearNDFast)
12:54:18 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:18 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-09 12:00:00) in space (linearNDFast)
12:54:18 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:18 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-09 09:00:00, weight 0.05) and
after (2024-11-09 12:00:00, weight 0.95) in time
12:54:18 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:18 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:18 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:18 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:18 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:54:18 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:54:18 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:54:18 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:54:18 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:18 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.723103 (min) 0.0721716 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.542007 (min) 0.144727 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: x_wind: -5.81111 (min) -0.68354 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: y_wind: -10.9262 (min) -6.13362 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:54:18 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:18 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.936977, mean: 2.108772, max: 3.573645
12:54:18 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:54:18 DEBUG opendrift.models.physics_methods:1061: min: 5.273440, mean: 7.885978, max: 10.298767
12:54:18 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 5.273440, mean: 7.885978, max: 10.298767
12:54:18 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:54:18 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:18 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:54:18 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:54:18 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:54:18 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:54:18 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:54:18 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:54:18 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:54:18 DEBUG opendrift.models.physics_methods:1061: min: 5.273440, mean: 7.885978, max: 10.298767
12:54:18 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:54:18 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.004450, dN_50: 0.000349
12:54:18 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:54:18 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.05241983592874461
12:54:18 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:18 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:54:18 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1433 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1432 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1430 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1429 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1428 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1424 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1424 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1423 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1421 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1419 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1415 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1415 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1414 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1412 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1410 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1409 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1408 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1405 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1404 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1403 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1402 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1401 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1399 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1398 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1397 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1396 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1395 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1393 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1391 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1390 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1386 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1384 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1380 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1379 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1378 surface elements
12:54:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1376 surface elements
12:54:19 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1374 surface elements
12:54:19 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1372 surface elements
12:54:19 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1369 surface elements
12:54:19 DEBUG opendrift.models.physics_methods:915: Advecting 1369 of 6000 elements above 0.100m with wind-sheared ocean current (0.202575 m/s - 0.358971 m/s)
12:54:19 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:54:19 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.002566562302116032 and 0.44765924373920785 m/s
12:54:19 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:54:19 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:54:19 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:19 INFO opendrift.models.basemodel:2038: 2024-11-09 12:51:18.915878 - step 73 of 120 - 6000 active elements (0 deactivated)
12:54:19 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:19 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:19 DEBUG opendrift.models.basemodel:2057: 34.72725131650778 <- latitude -> 35.181641826480735
12:54:19 DEBUG opendrift.models.basemodel:2062: 22.751958612640394 <- longitude -> 23.560074120584638
12:54:19 DEBUG opendrift.models.basemodel:2067: -89.40412936772373 <- z -> 0.0
12:54:19 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:19 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:19 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:19 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:19 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:19 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:19 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:19 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:54:19 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:19 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:19 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:19 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:19 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:19 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:19 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:19 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:19 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:19 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:54:19 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:19 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:19 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:19 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:19 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:19 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:54:19 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:19 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:54:19 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:19 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:19 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:54:19 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:54:19 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-09 12:00:00 (before)
2024-11-09 15:00:00 (after)
12:54:19 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
12:54:19 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
12:54:19 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:54:19 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:54:19 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:54:19 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
12:54:19 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 18x19x20) for time after (2024-11-09 15:00:00)
12:54:19 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-09 12:00:00) in space (linearNDFast)
12:54:19 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:19 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-09 15:00:00) in space (linearNDFast)
12:54:19 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:19 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-09 12:00:00, weight 0.71) and
after (2024-11-09 15:00:00, weight 0.29) in time
12:54:19 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:19 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:19 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:19 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:54:19 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:19 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:54:19 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:19 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:19 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:54:19 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:54:19 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-09 12:00:00 (before)
2024-11-09 15:00:00 (after)
12:54:19 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_wind to retrieve x_wind
12:54:22 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_wind to retrieve y_wind
12:54:25 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:54:25 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:54:25 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:54:25 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 720x361x1) for time after (2024-11-09 15:00:00)
12:54:25 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-09 12:00:00) in space (linearNDFast)
12:54:25 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:25 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-09 15:00:00) in space (linearNDFast)
12:54:25 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:25 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-09 12:00:00, weight 0.71) and
after (2024-11-09 15:00:00, weight 0.29) in time
12:54:25 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:25 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:25 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:25 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:25 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:54:25 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:54:25 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:54:25 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:54:25 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:25 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.666216 (min) 0.0800711 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.534752 (min) 0.0999697 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:905: x_wind: -6.0033 (min) -1.11609 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:905: y_wind: -11.4008 (min) -5.56097 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:54:25 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:25 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.791383, mean: 2.110736, max: 3.929362
12:54:25 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:54:25 DEBUG opendrift.models.physics_methods:1061: min: 4.846441, mean: 7.876088, max: 10.799175
12:54:25 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 4.846441, mean: 7.876088, max: 10.799175
12:54:25 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:54:25 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:25 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:54:25 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:54:25 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:54:25 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:54:25 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:54:25 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:54:25 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:54:25 DEBUG opendrift.models.physics_methods:1061: min: 4.846441, mean: 7.876088, max: 10.799175
12:54:25 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:54:25 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.004627, dN_50: 0.000363
12:54:26 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:54:26 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.05763724165650966
12:54:26 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:26 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:54:26 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1366 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1366 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1363 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1361 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1360 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1361 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1360 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1359 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1358 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1356 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1354 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1351 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1350 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1349 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1348 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1347 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1345 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1344 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1343 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1342 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1340 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1339 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1338 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 1337 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1332 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1331 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1330 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1329 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1328 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1327 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1326 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1325 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1322 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1321 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1319 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1319 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1316 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1315 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1314 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1313 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1312 surface elements
12:54:26 DEBUG opendrift.models.physics_methods:915: Advecting 1318 of 6000 elements above 0.100m with wind-sheared ocean current (0.099733 m/s - 0.379153 m/s)
12:54:26 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:54:26 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0012912174670883531 and 0.4690303199160773 m/s
12:54:26 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:54:26 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:54:26 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:26 INFO opendrift.models.basemodel:2038: 2024-11-09 13:51:18.915878 - step 74 of 120 - 6000 active elements (0 deactivated)
12:54:26 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:26 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:26 DEBUG opendrift.models.basemodel:2057: 34.7178213174307 <- latitude -> 35.1747240159708
12:54:26 DEBUG opendrift.models.basemodel:2062: 22.73288887376349 <- longitude -> 23.565136716280474
12:54:26 DEBUG opendrift.models.basemodel:2067: -88.54198307743853 <- z -> 0.0
12:54:26 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:26 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:26 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:26 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:54:26 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:26 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:26 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:26 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:26 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:26 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:26 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:54:26 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:26 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:26 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:26 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:26 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:54:26 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:54:26 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:26 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:54:26 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:54:26 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-09 12:00:00 (before)
2024-11-09 15:00:00 (after)
12:54:26 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-09 12:00:00) in space (linearNDFast)
12:54:26 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:26 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-09 15:00:00) in space (linearNDFast)
12:54:26 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:26 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-09 12:00:00, weight 0.38) and
after (2024-11-09 15:00:00, weight 0.62) in time
12:54:26 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:26 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:26 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:54:26 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:54:26 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:26 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:54:26 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:54:26 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-09 12:00:00 (before)
2024-11-09 15:00:00 (after)
12:54:26 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-09 12:00:00) in space (linearNDFast)
12:54:26 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:26 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-09 15:00:00) in space (linearNDFast)
12:54:26 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:26 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-09 12:00:00, weight 0.38) and
after (2024-11-09 15:00:00, weight 0.62) in time
12:54:26 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:26 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:26 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:26 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:54:26 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:54:26 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:54:26 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:54:26 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:26 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.685309 (min) 0.0865732 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.560688 (min) 0.07253 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: x_wind: -6.329 (min) -1.73674 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: y_wind: -11.9389 (min) -4.76055 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:26 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.631707, mean: 2.122199, max: 4.365903
12:54:26 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:54:26 DEBUG opendrift.models.physics_methods:1061: min: 4.329995, mean: 7.876807, max: 11.383260
12:54:26 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 4.329995, mean: 7.876807, max: 11.383260
12:54:26 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:54:26 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:26 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:54:26 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:54:26 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:54:26 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:54:26 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:54:26 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:54:26 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:54:26 DEBUG opendrift.models.physics_methods:1061: min: 4.329995, mean: 7.876807, max: 11.383260
12:54:26 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:54:26 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.004789, dN_50: 0.000376
12:54:26 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:54:26 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.06404011772967452
12:54:26 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:26 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:54:26 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1310 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1309 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1308 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1307 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1306 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1305 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1304 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1303 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1302 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1301 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1299 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1298 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1296 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1294 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1292 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1291 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1287 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1285 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1283 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1282 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1280 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1279 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1278 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1277 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1276 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1274 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1273 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1271 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1270 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1269 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1268 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1267 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1266 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1264 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1262 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1261 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1260 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1259 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1257 surface elements
12:54:26 DEBUG opendrift.models.physics_methods:915: Advecting 1259 of 6000 elements above 0.100m with wind-sheared ocean current (0.010942 m/s - 0.397199 m/s)
12:54:26 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:54:26 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0014750869169321947 and 0.4488142497854939 m/s
12:54:26 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:54:26 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:54:26 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:26 INFO opendrift.models.basemodel:2038: 2024-11-09 14:51:18.915878 - step 75 of 120 - 6000 active elements (0 deactivated)
12:54:26 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:26 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:26 DEBUG opendrift.models.basemodel:2057: 34.703391284405996 <- latitude -> 35.17498124721661
12:54:26 DEBUG opendrift.models.basemodel:2062: 22.71417564598748 <- longitude -> 23.567240903078947
12:54:26 DEBUG opendrift.models.basemodel:2067: -87.8766209403388 <- z -> 0.0
12:54:26 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:26 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:26 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:26 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:54:26 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:26 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:26 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:26 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:26 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:26 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:26 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:54:26 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:26 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:26 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:26 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:26 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:54:26 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:54:26 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:26 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:54:26 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:54:26 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-09 12:00:00 (before)
2024-11-09 15:00:00 (after)
12:54:26 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-09 12:00:00) in space (linearNDFast)
12:54:26 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:26 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-09 15:00:00) in space (linearNDFast)
12:54:26 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:26 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-09 12:00:00, weight 0.05) and
after (2024-11-09 15:00:00, weight 0.95) in time
12:54:26 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:26 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:26 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:54:26 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:54:26 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:26 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:54:26 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:54:26 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-09 12:00:00 (before)
2024-11-09 15:00:00 (after)
12:54:26 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-09 12:00:00) in space (linearNDFast)
12:54:26 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:26 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-09 15:00:00) in space (linearNDFast)
12:54:26 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:26 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-09 12:00:00, weight 0.05) and
after (2024-11-09 15:00:00, weight 0.95) in time
12:54:26 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:26 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:26 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:26 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:54:26 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:54:26 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:54:26 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:54:26 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:26 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.65964 (min) 0.126878 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.603562 (min) 0.0532963 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: x_wind: -6.67109 (min) -2.35411 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: y_wind: -12.5054 (min) -3.9984 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:54:26 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:26 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.529615, mean: 2.162484, max: 4.862945
12:54:26 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:54:26 DEBUG opendrift.models.physics_methods:1061: min: 3.964693, mean: 7.928572, max: 12.013769
12:54:26 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.964693, mean: 7.928572, max: 12.013769
12:54:26 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:54:26 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:26 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:54:26 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:54:26 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:54:26 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:54:26 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:54:26 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:54:26 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:54:26 DEBUG opendrift.models.physics_methods:1061: min: 3.964693, mean: 7.928572, max: 12.013769
12:54:26 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:54:26 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.004934, dN_50: 0.000387
12:54:26 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:54:26 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.07133036284081581
12:54:26 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:26 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:54:26 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1254 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1252 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1250 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1249 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1248 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1245 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1244 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1243 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1242 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1241 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1240 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1238 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1237 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1235 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1233 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1232 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1231 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1229 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1228 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1227 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1226 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1226 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1223 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1225 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1223 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1222 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1221 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1219 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1218 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1217 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1215 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1215 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1213 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1211 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1211 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1211 surface elements
12:54:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1210 surface elements
12:54:26 DEBUG opendrift.models.physics_methods:915: Advecting 1214 of 6000 elements above 0.100m with wind-sheared ocean current (0.018462 m/s - 0.412202 m/s)
12:54:26 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:54:26 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0015542638682502035 and 0.4258198347663545 m/s
12:54:26 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:54:26 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:54:26 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:26 INFO opendrift.models.basemodel:2038: 2024-11-09 15:51:18.915878 - step 76 of 120 - 6000 active elements (0 deactivated)
12:54:26 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:26 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:26 DEBUG opendrift.models.basemodel:2057: 34.69136487164987 <- latitude -> 35.17195801293596
12:54:26 DEBUG opendrift.models.basemodel:2062: 22.69559324877139 <- longitude -> 23.56871930257381
12:54:26 DEBUG opendrift.models.basemodel:2067: -87.63795963587265 <- z -> 0.0
12:54:26 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:26 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:26 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:26 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:54:26 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:26 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:26 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:26 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:26 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:26 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:26 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:54:26 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:26 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:26 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:26 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:26 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:54:26 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:54:26 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:26 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:26 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:54:26 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:54:26 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-09 15:00:00 (before)
2024-11-09 18:00:00 (after)
12:54:26 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
12:54:27 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
12:54:27 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:54:27 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:54:27 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:54:27 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
12:54:27 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 19x20x20) for time after (2024-11-09 18:00:00)
12:54:27 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-09 15:00:00) in space (linearNDFast)
12:54:27 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:27 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-09 18:00:00) in space (linearNDFast)
12:54:27 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:27 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-09 15:00:00, weight 0.71) and
after (2024-11-09 18:00:00, weight 0.29) in time
12:54:27 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:27 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:27 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:27 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:54:27 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:27 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:54:27 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:27 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:27 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:54:27 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:54:27 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-09 15:00:00 (before)
2024-11-09 18:00:00 (after)
12:54:27 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_wind to retrieve x_wind
12:54:30 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_wind to retrieve y_wind
12:54:32 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:54:32 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:54:32 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:54:32 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 720x361x1) for time after (2024-11-09 18:00:00)
12:54:32 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-09 15:00:00) in space (linearNDFast)
12:54:32 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:32 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-09 18:00:00) in space (linearNDFast)
12:54:32 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:32 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-09 15:00:00, weight 0.71) and
after (2024-11-09 18:00:00, weight 0.29) in time
12:54:32 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:32 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:32 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:32 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:32 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:54:32 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:54:32 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:54:32 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:54:32 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:32 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.689328 (min) 0.113796 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.576138 (min) 0.0368298 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:905: x_wind: -6.58811 (min) -2.22245 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:905: y_wind: -12.1839 (min) -3.94323 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:54:32 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:32 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.504013, mean: 2.074123, max: 4.590906
12:54:32 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:54:32 DEBUG opendrift.models.physics_methods:1061: min: 3.867679, mean: 7.758026, max: 11.672900
12:54:32 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.867679, mean: 7.758026, max: 11.672900
12:54:32 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:54:32 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:32 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:54:32 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:54:32 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:54:32 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:54:32 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:54:32 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:54:32 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:54:32 DEBUG opendrift.models.physics_methods:1061: min: 3.867679, mean: 7.758026, max: 11.672900
12:54:32 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:54:32 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.005191, dN_50: 0.000407
12:54:32 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:54:32 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.06734029060847893
12:54:32 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:32 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:54:32 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:54:32 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1208 surface elements
12:54:32 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1207 surface elements
12:54:32 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1206 surface elements
12:54:32 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1204 surface elements
12:54:32 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1203 surface elements
12:54:32 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1202 surface elements
12:54:32 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1202 surface elements
12:54:32 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1201 surface elements
12:54:32 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1198 surface elements
12:54:32 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1197 surface elements
12:54:32 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1194 surface elements
12:54:32 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1192 surface elements
12:54:32 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1191 surface elements
12:54:32 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1190 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1189 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1188 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1186 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1186 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1185 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1184 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1181 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1179 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1178 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1177 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1177 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1176 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1174 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1173 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1173 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1172 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1171 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1170 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1169 surface elements
12:54:33 DEBUG opendrift.models.physics_methods:915: Advecting 1177 of 6000 elements above 0.100m with wind-sheared ocean current (0.003236 m/s - 0.408558 m/s)
12:54:33 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:54:33 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0008637224357893708 and 0.4341260629575916 m/s
12:54:33 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:54:33 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:54:33 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:33 INFO opendrift.models.basemodel:2038: 2024-11-09 16:51:18.915878 - step 77 of 120 - 6000 active elements (0 deactivated)
12:54:33 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:33 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:33 DEBUG opendrift.models.basemodel:2057: 34.68521396026948 <- latitude -> 35.16366509710659
12:54:33 DEBUG opendrift.models.basemodel:2062: 22.667165827744924 <- longitude -> 23.57314408014561
12:54:33 DEBUG opendrift.models.basemodel:2067: -86.56671291014446 <- z -> 0.0
12:54:33 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:33 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:33 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:33 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:33 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:33 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:33 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:33 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:54:33 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:33 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:33 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:33 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:33 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:33 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:33 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:33 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:33 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:33 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:54:33 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:33 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:33 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:33 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:33 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:33 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:54:33 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:33 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:54:33 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:33 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:33 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:54:33 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:54:33 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-09 15:00:00 (before)
2024-11-09 18:00:00 (after)
12:54:33 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-09 15:00:00) in space (linearNDFast)
12:54:33 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:33 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-09 18:00:00) in space (linearNDFast)
12:54:33 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:33 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-09 15:00:00, weight 0.38) and
after (2024-11-09 18:00:00, weight 0.62) in time
12:54:33 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:33 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:33 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:33 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:54:33 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:33 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:54:33 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:33 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:33 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:54:33 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:54:33 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-09 15:00:00 (before)
2024-11-09 18:00:00 (after)
12:54:33 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-09 15:00:00) in space (linearNDFast)
12:54:33 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:33 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-09 18:00:00) in space (linearNDFast)
12:54:33 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:33 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-09 15:00:00, weight 0.38) and
after (2024-11-09 18:00:00, weight 0.62) in time
12:54:33 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:33 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:33 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:33 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:33 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:54:33 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:54:33 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:54:33 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:54:33 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:33 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.687416 (min) 0.0991373 (max)
12:54:33 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.560181 (min) 0.0303435 (max)
12:54:33 DEBUG opendrift.models.basemodel.environment:905: x_wind: -6.61553 (min) -1.91122 (max)
12:54:33 DEBUG opendrift.models.basemodel.environment:905: y_wind: -11.8505 (min) -3.95891 (max)
12:54:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:54:33 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:54:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:54:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:54:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:54:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:54:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:54:33 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:54:33 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:54:33 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:54:33 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:54:33 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:54:33 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:54:33 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:54:33 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:33 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:54:33 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:33 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.477243, mean: 1.967755, max: 4.295849
12:54:33 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:54:33 DEBUG opendrift.models.physics_methods:1061: min: 3.763563, mean: 7.552387, max: 11.291564
12:54:33 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.763563, mean: 7.552387, max: 11.291564
12:54:33 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:54:33 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:33 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:54:33 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:54:33 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:54:33 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:54:33 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:54:33 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:54:33 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:54:33 DEBUG opendrift.models.physics_methods:1061: min: 3.763563, mean: 7.552387, max: 11.291564
12:54:33 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:54:33 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.005479, dN_50: 0.000430
12:54:33 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:54:33 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.06301260550375587
12:54:33 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:33 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:54:33 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1168 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1167 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1166 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1165 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1165 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1164 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1162 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1160 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1160 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1160 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1158 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1157 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1156 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1156 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1155 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1154 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1154 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1153 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1152 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1150 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1150 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1149 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1148 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1147 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1145 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1144 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1143 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1141 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1139 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1137 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1135 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1134 surface elements
12:54:33 DEBUG opendrift.models.physics_methods:915: Advecting 1140 of 6000 elements above 0.100m with wind-sheared ocean current (0.029091 m/s - 0.396408 m/s)
12:54:33 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:54:33 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0007227125603531762 and 0.4518652933650653 m/s
12:54:33 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:54:33 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:54:33 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:33 INFO opendrift.models.basemodel:2038: 2024-11-09 17:51:18.915878 - step 78 of 120 - 6000 active elements (0 deactivated)
12:54:33 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:33 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:33 DEBUG opendrift.models.basemodel:2057: 34.665271091790615 <- latitude -> 35.15694645026779
12:54:33 DEBUG opendrift.models.basemodel:2062: 22.645625147655 <- longitude -> 23.57157304441592
12:54:33 DEBUG opendrift.models.basemodel:2067: -85.50772328084057 <- z -> 0.0
12:54:33 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:33 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:33 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:33 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:33 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:33 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:33 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:33 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:54:33 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:33 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:33 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:33 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:33 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:33 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:33 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:33 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:33 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:33 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:54:33 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:33 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:33 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:33 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:33 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:33 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:54:33 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:33 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:54:33 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:33 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:33 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:54:33 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:54:33 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-09 15:00:00 (before)
2024-11-09 18:00:00 (after)
12:54:33 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-09 15:00:00) in space (linearNDFast)
12:54:33 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:33 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-09 18:00:00) in space (linearNDFast)
12:54:33 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:33 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-09 15:00:00, weight 0.05) and
after (2024-11-09 18:00:00, weight 0.95) in time
12:54:33 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:33 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:33 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:33 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:54:33 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:33 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:54:33 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:33 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:33 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:54:33 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:54:33 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-09 15:00:00 (before)
2024-11-09 18:00:00 (after)
12:54:33 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-09 15:00:00) in space (linearNDFast)
12:54:33 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:33 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-09 18:00:00) in space (linearNDFast)
12:54:33 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:33 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-09 15:00:00, weight 0.05) and
after (2024-11-09 18:00:00, weight 0.95) in time
12:54:33 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:33 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:33 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:33 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:33 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:54:33 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:54:33 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:54:33 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:54:33 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:33 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.763 (min) 0.0983244 (max)
12:54:33 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.590653 (min) 0.0434064 (max)
12:54:33 DEBUG opendrift.models.basemodel.environment:905: x_wind: -6.8006 (min) -1.68229 (max)
12:54:33 DEBUG opendrift.models.basemodel.environment:905: y_wind: -11.5151 (min) -4.03195 (max)
12:54:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:54:33 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:54:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:54:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:54:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:54:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:54:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:54:33 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:54:33 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:54:33 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:54:33 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:54:33 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:54:33 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:54:33 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:54:33 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:33 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:54:33 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:33 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.469532, mean: 1.867713, max: 4.065307
12:54:33 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:54:33 DEBUG opendrift.models.physics_methods:1061: min: 3.733038, mean: 7.353568, max: 10.984398
12:54:33 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.733038, mean: 7.353568, max: 10.984398
12:54:33 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:54:33 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:33 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:54:33 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:54:33 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:54:33 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:54:33 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:54:33 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:54:33 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:54:33 DEBUG opendrift.models.physics_methods:1061: min: 3.733037, mean: 7.353568, max: 10.984398
12:54:33 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:54:33 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.005781, dN_50: 0.000454
12:54:33 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:54:33 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.0596311813064781
12:54:33 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:33 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:54:33 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1133 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1132 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1130 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1128 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1127 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1126 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1125 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1124 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1123 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1121 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1121 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1120 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1119 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1118 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1116 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1115 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1114 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1113 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1112 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1112 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1112 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1112 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1111 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1110 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1109 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1108 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1107 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1106 surface elements
12:54:33 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1105 surface elements
12:54:33 DEBUG opendrift.models.physics_methods:915: Advecting 1112 of 6000 elements above 0.100m with wind-sheared ocean current (0.039932 m/s - 0.383033 m/s)
12:54:33 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:54:33 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.002087991725624819 and 0.43864849271270356 m/s
12:54:33 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:54:33 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:54:33 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:33 INFO opendrift.models.basemodel:2038: 2024-11-09 18:51:18.915878 - step 79 of 120 - 6000 active elements (0 deactivated)
12:54:33 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:33 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:33 DEBUG opendrift.models.basemodel:2057: 34.64891594642566 <- latitude -> 35.1509542718089
12:54:33 DEBUG opendrift.models.basemodel:2062: 22.62236539608536 <- longitude -> 23.564752479253386
12:54:33 DEBUG opendrift.models.basemodel:2067: -84.64996072471797 <- z -> 0.0
12:54:33 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:33 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:33 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:33 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:33 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:33 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:33 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:33 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:54:33 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:33 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:33 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:33 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:33 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:33 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:33 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:33 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:33 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:33 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:54:33 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:33 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:33 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:33 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:33 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:33 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:54:33 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:33 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:54:33 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:33 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:33 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:54:33 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:54:33 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-09 18:00:00 (before)
2024-11-09 21:00:00 (after)
12:54:33 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
12:54:34 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
12:54:35 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:54:35 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:54:35 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:54:35 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
12:54:35 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 20x20x20) for time after (2024-11-09 21:00:00)
12:54:35 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-09 18:00:00) in space (linearNDFast)
12:54:35 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:35 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-09 21:00:00) in space (linearNDFast)
12:54:35 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:35 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-09 18:00:00, weight 0.71) and
after (2024-11-09 21:00:00, weight 0.29) in time
12:54:35 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:35 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:35 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:35 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:54:35 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:35 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:54:35 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:35 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:35 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:54:35 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:54:35 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-09 18:00:00 (before)
2024-11-09 21:00:00 (after)
12:54:35 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_wind to retrieve x_wind
12:54:39 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_wind to retrieve y_wind
12:54:41 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:54:41 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:54:41 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:54:41 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 720x361x1) for time after (2024-11-09 21:00:00)
12:54:41 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-09 18:00:00) in space (linearNDFast)
12:54:41 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:41 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-09 21:00:00) in space (linearNDFast)
12:54:41 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:41 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-09 18:00:00, weight 0.71) and
after (2024-11-09 21:00:00, weight 0.29) in time
12:54:41 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:41 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:41 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:41 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:41 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:54:41 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:54:41 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:54:41 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:54:41 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:41 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.752193 (min) 0.038851 (max)
12:54:41 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.513905 (min) 0.048928 (max)
12:54:41 DEBUG opendrift.models.basemodel.environment:905: x_wind: -6.79012 (min) -1.75337 (max)
12:54:41 DEBUG opendrift.models.basemodel.environment:905: y_wind: -10.7396 (min) -4.39732 (max)
12:54:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:54:41 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:54:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:54:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:54:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:54:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:54:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:54:41 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:54:41 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:54:41 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:54:41 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:54:41 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:54:41 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:54:41 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:54:41 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:41 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:54:41 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:41 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.555425, mean: 1.830652, max: 3.702751
12:54:41 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:54:41 DEBUG opendrift.models.physics_methods:1061: min: 4.060152, mean: 7.292999, max: 10.483151
12:54:41 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 4.060152, mean: 7.292999, max: 10.483151
12:54:41 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:54:42 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:42 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:54:42 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:54:42 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:54:42 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:54:42 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:54:42 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:54:42 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:54:42 DEBUG opendrift.models.physics_methods:1061: min: 4.060152, mean: 7.292999, max: 10.483151
12:54:42 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:54:42 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.006075, dN_50: 0.000477
12:54:42 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:54:42 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.054313475363523885
12:54:42 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:42 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:54:42 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:54:42 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1104 surface elements
12:54:42 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1103 surface elements
12:54:42 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1103 surface elements
12:54:42 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1101 surface elements
12:54:42 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1100 surface elements
12:54:42 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1099 surface elements
12:54:42 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1098 surface elements
12:54:42 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1098 surface elements
12:54:42 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1097 surface elements
12:54:42 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1096 surface elements
12:54:42 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1095 surface elements
12:54:42 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1093 surface elements
12:54:42 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1093 surface elements
12:54:42 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1093 surface elements
12:54:42 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1092 surface elements
12:54:42 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1091 surface elements
12:54:42 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1090 surface elements
12:54:42 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1089 surface elements
12:54:42 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1088 surface elements
12:54:42 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1088 surface elements
12:54:42 DEBUG opendrift.models.physics_methods:915: Advecting 1098 of 6000 elements above 0.100m with wind-sheared ocean current (0.006716 m/s - 0.363503 m/s)
12:54:42 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:54:42 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0013312670992847201 and 0.4207266504580065 m/s
12:54:42 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:54:42 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:54:42 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:42 INFO opendrift.models.basemodel:2038: 2024-11-09 19:51:18.915878 - step 80 of 120 - 6000 active elements (0 deactivated)
12:54:42 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:42 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:42 DEBUG opendrift.models.basemodel:2057: 34.63500629835017 <- latitude -> 35.147573413531
12:54:42 DEBUG opendrift.models.basemodel:2062: 22.59895839127616 <- longitude -> 23.55283237485196
12:54:42 DEBUG opendrift.models.basemodel:2067: -83.4656021044308 <- z -> 0.0
12:54:42 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:42 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:42 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:42 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:54:42 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:42 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:42 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:42 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:42 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:42 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:42 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:54:42 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:42 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:42 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:42 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:42 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:54:42 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:54:42 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:42 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:54:42 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:54:42 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-09 18:00:00 (before)
2024-11-09 21:00:00 (after)
12:54:42 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-09 18:00:00) in space (linearNDFast)
12:54:42 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:42 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-09 21:00:00) in space (linearNDFast)
12:54:42 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:42 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-09 18:00:00, weight 0.38) and
after (2024-11-09 21:00:00, weight 0.62) in time
12:54:42 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:42 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:42 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:54:42 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:54:42 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:42 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:54:42 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:54:42 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-09 18:00:00 (before)
2024-11-09 21:00:00 (after)
12:54:42 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-09 18:00:00) in space (linearNDFast)
12:54:42 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:42 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-09 21:00:00) in space (linearNDFast)
12:54:42 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:42 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-09 18:00:00, weight 0.38) and
after (2024-11-09 21:00:00, weight 0.62) in time
12:54:42 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:42 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:42 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:42 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:54:42 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:54:42 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:54:42 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:54:42 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:42 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.805887 (min) 0.00739422 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.514482 (min) 0.059413 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: x_wind: -6.77014 (min) -1.93085 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: y_wind: -9.99605 (min) -4.5875 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:42 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.646568, mean: 1.797901, max: 3.424560
12:54:42 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:54:42 DEBUG opendrift.models.physics_methods:1061: min: 4.380630, mean: 7.233840, max: 10.081658
12:54:42 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 4.380630, mean: 7.233840, max: 10.081658
12:54:42 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:54:42 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:42 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:54:42 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:54:42 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:54:42 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:54:42 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:54:42 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:54:42 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:54:42 DEBUG opendrift.models.physics_methods:1061: min: 4.380630, mean: 7.233840, max: 10.081657
12:54:42 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:54:42 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.006394, dN_50: 0.000502
12:54:42 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:54:42 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.050233172071840936
12:54:42 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:42 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:54:42 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:54:42 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1087 surface elements
12:54:42 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1085 surface elements
12:54:42 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1085 surface elements
12:54:42 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1084 surface elements
12:54:42 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1083 surface elements
12:54:42 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1083 surface elements
12:54:42 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1083 surface elements
12:54:42 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1082 surface elements
12:54:42 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1081 surface elements
12:54:42 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1081 surface elements
12:54:42 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1080 surface elements
12:54:42 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1079 surface elements
12:54:42 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1078 surface elements
12:54:42 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1077 surface elements
12:54:42 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1076 surface elements
12:54:42 DEBUG opendrift.models.physics_methods:915: Advecting 1081 of 6000 elements above 0.100m with wind-sheared ocean current (0.042900 m/s - 0.344666 m/s)
12:54:42 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:54:42 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.002637255255343778 and 0.4141488635302691 m/s
12:54:42 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:54:42 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:54:42 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:42 INFO opendrift.models.basemodel:2038: 2024-11-09 20:51:18.915878 - step 81 of 120 - 6000 active elements (0 deactivated)
12:54:42 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:42 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:42 DEBUG opendrift.models.basemodel:2057: 34.622818591245014 <- latitude -> 35.14660022602508
12:54:42 DEBUG opendrift.models.basemodel:2062: 22.57620388445755 <- longitude -> 23.55018543476602
12:54:42 DEBUG opendrift.models.basemodel:2067: -82.59616164769103 <- z -> 0.0
12:54:42 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:42 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:42 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:42 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:54:42 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:42 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:42 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:42 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:42 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:42 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:42 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:54:42 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:42 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:42 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:42 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:42 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:54:42 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:54:42 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:42 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:54:42 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:54:42 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-09 18:00:00 (before)
2024-11-09 21:00:00 (after)
12:54:42 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-09 18:00:00) in space (linearNDFast)
12:54:42 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:42 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-09 21:00:00) in space (linearNDFast)
12:54:42 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:42 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-09 18:00:00, weight 0.05) and
after (2024-11-09 21:00:00, weight 0.95) in time
12:54:42 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:42 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:42 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:54:42 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:54:42 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:42 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:54:42 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:54:42 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-09 18:00:00 (before)
2024-11-09 21:00:00 (after)
12:54:42 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-09 18:00:00) in space (linearNDFast)
12:54:42 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:42 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-09 21:00:00) in space (linearNDFast)
12:54:42 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:42 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-09 18:00:00, weight 0.05) and
after (2024-11-09 21:00:00, weight 0.95) in time
12:54:42 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:42 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:42 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:42 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:54:42 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:54:42 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:54:42 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:54:42 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:42 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.866005 (min) -0.00515147 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.477462 (min) 0.0681788 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: x_wind: -6.74173 (min) -2.07414 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: y_wind: -9.21662 (min) -4.56038 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:54:42 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:42 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.623584, mean: 1.758065, max: 3.146477
12:54:42 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:54:42 DEBUG opendrift.models.physics_methods:1061: min: 4.302067, mean: 7.150568, max: 9.663665
12:54:42 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 4.302067, mean: 7.150568, max: 9.663665
12:54:42 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:54:42 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:42 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:54:42 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:54:42 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:54:42 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:54:42 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:54:42 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:54:42 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:54:42 DEBUG opendrift.models.physics_methods:1061: min: 4.302067, mean: 7.150568, max: 9.663665
12:54:42 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:54:42 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.006747, dN_50: 0.000530
12:54:42 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:54:42 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.04615444472996957
12:54:42 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:42 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:54:42 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:54:42 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1075 surface elements
12:54:42 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1074 surface elements
12:54:42 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1073 surface elements
12:54:42 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1072 surface elements
12:54:42 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1071 surface elements
12:54:42 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1071 surface elements
12:54:42 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1070 surface elements
12:54:42 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1068 surface elements
12:54:42 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1069 surface elements
12:54:42 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1068 surface elements
12:54:42 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1066 surface elements
12:54:42 DEBUG opendrift.models.physics_methods:915: Advecting 1076 of 6000 elements above 0.100m with wind-sheared ocean current (0.052141 m/s - 0.331815 m/s)
12:54:42 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:54:42 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0035705042667924917 and 0.43627284119716353 m/s
12:54:42 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:54:42 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:54:42 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:42 INFO opendrift.models.basemodel:2038: 2024-11-09 21:51:18.915878 - step 82 of 120 - 6000 active elements (0 deactivated)
12:54:42 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:42 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:42 DEBUG opendrift.models.basemodel:2057: 34.6130511235376 <- latitude -> 35.14360786709575
12:54:42 DEBUG opendrift.models.basemodel:2062: 22.553041459881616 <- longitude -> 23.542451387270226
12:54:42 DEBUG opendrift.models.basemodel:2067: -81.39704084527958 <- z -> 0.0
12:54:42 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:42 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:42 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:42 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:54:42 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:42 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:42 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:42 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:42 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:42 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:42 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:54:42 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:42 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:42 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:42 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:42 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:54:42 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:54:42 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:42 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:42 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:54:42 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:54:42 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-09 21:00:00 (before)
2024-11-10 00:00:00 (after)
12:54:42 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
12:54:42 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
12:54:43 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:54:43 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:54:43 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:54:43 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
12:54:43 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 20x21x20) for time after (2024-11-10 00:00:00)
12:54:43 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-09 21:00:00) in space (linearNDFast)
12:54:43 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:43 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-10 00:00:00) in space (linearNDFast)
12:54:43 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:43 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-09 21:00:00, weight 0.71) and
after (2024-11-10 00:00:00, weight 0.29) in time
12:54:43 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:43 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:43 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:43 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:54:43 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:43 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:54:43 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:43 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:43 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:54:43 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:54:43 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-09 21:00:00 (before)
2024-11-10 00:00:00 (after)
12:54:43 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_wind to retrieve x_wind
12:54:47 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_wind to retrieve y_wind
12:54:50 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:54:50 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:54:50 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:54:50 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 720x361x1) for time after (2024-11-10 00:00:00)
12:54:50 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-09 21:00:00) in space (linearNDFast)
12:54:50 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:50 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-10 00:00:00) in space (linearNDFast)
12:54:50 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:50 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-09 21:00:00, weight 0.71) and
after (2024-11-10 00:00:00, weight 0.29) in time
12:54:50 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:50 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:50 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:50 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:50 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:54:50 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:54:50 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:54:50 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:54:50 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:50 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.772655 (min) -0.0168033 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.406507 (min) 0.0851335 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: x_wind: -6.72893 (min) -2.13979 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: y_wind: -9.39081 (min) -4.4188 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:50 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.592971, mean: 1.757619, max: 3.254700
12:54:50 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:54:50 DEBUG opendrift.models.physics_methods:1061: min: 4.195139, mean: 7.140493, max: 9.828451
12:54:50 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 4.195139, mean: 7.140493, max: 9.828451
12:54:50 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:54:50 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:50 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:54:50 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:54:50 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:54:50 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:54:50 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:54:50 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:54:50 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:54:50 DEBUG opendrift.models.physics_methods:1061: min: 4.195139, mean: 7.140493, max: 9.828451
12:54:50 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:54:50 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.006978, dN_50: 0.000548
12:54:50 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:54:50 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.04774178932855113
12:54:50 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:50 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:54:50 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:54:50 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1068 surface elements
12:54:50 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1066 surface elements
12:54:50 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1065 surface elements
12:54:50 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1064 surface elements
12:54:50 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1063 surface elements
12:54:50 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1063 surface elements
12:54:50 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1061 surface elements
12:54:50 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1060 surface elements
12:54:50 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1060 surface elements
12:54:50 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1059 surface elements
12:54:50 DEBUG opendrift.models.physics_methods:915: Advecting 1066 of 6000 elements above 0.100m with wind-sheared ocean current (0.031242 m/s - 0.337919 m/s)
12:54:50 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:54:50 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0028659116843620467 and 0.46893661348350435 m/s
12:54:50 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:54:50 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:54:50 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:50 INFO opendrift.models.basemodel:2038: 2024-11-09 22:51:18.915878 - step 83 of 120 - 6000 active elements (0 deactivated)
12:54:50 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:50 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:50 DEBUG opendrift.models.basemodel:2057: 34.60215471336929 <- latitude -> 35.14427737549678
12:54:50 DEBUG opendrift.models.basemodel:2062: 22.533163787053883 <- longitude -> 23.545832503491177
12:54:50 DEBUG opendrift.models.basemodel:2067: -80.5515090686377 <- z -> 0.0
12:54:50 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:50 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:50 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:50 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:50 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:50 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:50 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:50 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:54:50 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:50 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:50 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:50 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:50 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:50 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:50 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:50 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:50 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:50 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:54:50 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:50 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:50 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:50 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:50 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:50 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:54:50 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:50 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:54:50 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:50 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:50 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:54:50 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:54:50 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-09 21:00:00 (before)
2024-11-10 00:00:00 (after)
12:54:50 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-09 21:00:00) in space (linearNDFast)
12:54:50 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:50 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-10 00:00:00) in space (linearNDFast)
12:54:50 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:50 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-09 21:00:00, weight 0.38) and
after (2024-11-10 00:00:00, weight 0.62) in time
12:54:50 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:50 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:50 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:50 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:54:50 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:50 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:54:50 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:50 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:50 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:54:50 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:54:50 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-09 21:00:00 (before)
2024-11-10 00:00:00 (after)
12:54:50 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-09 21:00:00) in space (linearNDFast)
12:54:50 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:50 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-10 00:00:00) in space (linearNDFast)
12:54:50 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:50 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-09 21:00:00, weight 0.38) and
after (2024-11-10 00:00:00, weight 0.62) in time
12:54:50 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:50 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:50 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:50 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:50 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:54:50 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:54:50 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:54:50 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:54:50 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:50 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.756026 (min) -0.01433 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.361037 (min) 0.115848 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: x_wind: -6.80993 (min) -2.15585 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: y_wind: -9.77896 (min) -3.6768 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:50 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.475199, mean: 1.788471, max: 3.407135
12:54:50 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:54:50 DEBUG opendrift.models.physics_methods:1061: min: 3.755495, mean: 7.191849, max: 10.055976
12:54:50 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.755495, mean: 7.191849, max: 10.055976
12:54:50 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:54:50 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:50 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:54:50 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:54:50 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:54:50 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:54:50 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:54:50 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:54:50 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:54:50 DEBUG opendrift.models.physics_methods:1061: min: 3.755495, mean: 7.191849, max: 10.055977
12:54:50 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:54:50 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.007165, dN_50: 0.000562
12:54:50 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:54:50 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.049977589678796966
12:54:50 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:50 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:54:50 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:54:50 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1061 surface elements
12:54:50 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1062 surface elements
12:54:50 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1061 surface elements
12:54:50 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1061 surface elements
12:54:50 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1060 surface elements
12:54:50 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1059 surface elements
12:54:50 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1058 surface elements
12:54:50 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1057 surface elements
12:54:50 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1057 surface elements
12:54:50 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1056 surface elements
12:54:50 DEBUG opendrift.models.physics_methods:915: Advecting 1063 of 6000 elements above 0.100m with wind-sheared ocean current (0.019153 m/s - 0.342575 m/s)
12:54:50 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:54:50 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0019060043800467334 and 0.43008807980567504 m/s
12:54:50 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:54:50 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:54:50 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:50 INFO opendrift.models.basemodel:2038: 2024-11-09 23:51:18.915878 - step 84 of 120 - 6000 active elements (0 deactivated)
12:54:50 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:50 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:50 DEBUG opendrift.models.basemodel:2057: 34.591056915138 <- latitude -> 35.14274451607251
12:54:50 DEBUG opendrift.models.basemodel:2062: 22.511096048451485 <- longitude -> 23.542403001005415
12:54:50 DEBUG opendrift.models.basemodel:2067: -79.47615200762493 <- z -> 0.0
12:54:50 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:50 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:50 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:50 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:50 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:50 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:50 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:50 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:54:50 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:50 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:50 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:50 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:50 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:50 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:50 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:50 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:50 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:50 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:54:50 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:50 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:50 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:50 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:50 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:50 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:54:50 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:50 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:54:50 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:50 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:50 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:54:50 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:54:50 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-09 21:00:00 (before)
2024-11-10 00:00:00 (after)
12:54:50 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-09 21:00:00) in space (linearNDFast)
12:54:50 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:50 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-10 00:00:00) in space (linearNDFast)
12:54:50 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:50 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-09 21:00:00, weight 0.05) and
after (2024-11-10 00:00:00, weight 0.95) in time
12:54:50 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:50 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:50 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:50 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:54:50 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:50 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:54:50 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:50 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:50 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:54:50 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:54:50 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-09 21:00:00 (before)
2024-11-10 00:00:00 (after)
12:54:50 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-09 21:00:00) in space (linearNDFast)
12:54:50 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:50 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-10 00:00:00) in space (linearNDFast)
12:54:50 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:50 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-09 21:00:00, weight 0.05) and
after (2024-11-10 00:00:00, weight 0.95) in time
12:54:50 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:50 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:50 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:50 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:50 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:54:50 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:54:50 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:54:50 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:54:50 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:50 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.752432 (min) -0.0416244 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.306461 (min) 0.16685 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: x_wind: -6.9387 (min) -2.14345 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: y_wind: -10.2103 (min) -2.84562 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:54:50 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:50 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.368320, mean: 1.845360, max: 3.598118
12:54:50 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:54:50 DEBUG opendrift.models.physics_methods:1061: min: 3.306301, mean: 7.292623, max: 10.333973
12:54:50 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.306301, mean: 7.292623, max: 10.333973
12:54:50 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:54:50 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:50 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:54:50 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:54:50 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:54:50 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:54:50 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:54:50 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:54:50 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:54:50 DEBUG opendrift.models.physics_methods:1061: min: 3.306301, mean: 7.292623, max: 10.333973
12:54:50 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:54:50 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.007333, dN_50: 0.000576
12:54:50 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:54:50 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.052778796398690285
12:54:50 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:50 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:54:50 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:54:50 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1056 surface elements
12:54:50 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1055 surface elements
12:54:50 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1054 surface elements
12:54:50 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1054 surface elements
12:54:50 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1053 surface elements
12:54:50 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1052 surface elements
12:54:50 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1051 surface elements
12:54:50 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1050 surface elements
12:54:50 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1049 surface elements
12:54:50 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1048 surface elements
12:54:50 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1047 surface elements
12:54:50 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1046 surface elements
12:54:50 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1045 surface elements
12:54:50 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1044 surface elements
12:54:50 DEBUG opendrift.models.physics_methods:915: Advecting 1055 of 6000 elements above 0.100m with wind-sheared ocean current (0.000115 m/s - 0.344055 m/s)
12:54:50 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:54:50 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0007745766548517118 and 0.423865926119671 m/s
12:54:50 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:54:50 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:54:50 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:50 INFO opendrift.models.basemodel:2038: 2024-11-10 00:51:18.915878 - step 85 of 120 - 6000 active elements (0 deactivated)
12:54:50 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:50 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:50 DEBUG opendrift.models.basemodel:2057: 34.57851951029867 <- latitude -> 35.14121793537882
12:54:50 DEBUG opendrift.models.basemodel:2062: 22.486224238368557 <- longitude -> 23.535194663756496
12:54:50 DEBUG opendrift.models.basemodel:2067: -78.9281544474305 <- z -> 0.0
12:54:50 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:50 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:50 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:50 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:50 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:50 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:50 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:50 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:54:50 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:50 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:50 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:50 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:50 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:50 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:50 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:50 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:50 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:50 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:54:50 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:50 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:50 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:50 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:50 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:50 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:54:50 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:50 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:54:50 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:50 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:50 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:54:50 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:54:50 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-10 00:00:00 (before)
2024-11-10 03:00:00 (after)
12:54:50 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
12:54:51 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
12:54:51 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:54:51 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:54:51 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:54:51 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
12:54:51 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 21x22x19) for time after (2024-11-10 03:00:00)
12:54:51 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-10 00:00:00) in space (linearNDFast)
12:54:51 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:51 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-10 03:00:00) in space (linearNDFast)
12:54:51 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:51 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-10 00:00:00, weight 0.71) and
after (2024-11-10 03:00:00, weight 0.29) in time
12:54:51 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:51 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:51 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:51 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:54:51 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:51 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:54:51 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:51 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:51 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:54:51 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:54:51 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-10 00:00:00 (before)
2024-11-10 03:00:00 (after)
12:54:51 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_wind to retrieve x_wind
12:54:54 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_wind to retrieve y_wind
12:54:57 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:54:57 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:54:57 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:54:57 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 720x361x1) for time after (2024-11-10 03:00:00)
12:54:57 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-10 00:00:00) in space (linearNDFast)
12:54:57 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:57 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-10 03:00:00) in space (linearNDFast)
12:54:57 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:57 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-10 00:00:00, weight 0.71) and
after (2024-11-10 03:00:00, weight 0.29) in time
12:54:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:57 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:57 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:57 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:54:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:54:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:54:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:54:57 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:57 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.748194 (min) -0.0211755 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.296289 (min) 0.171993 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: x_wind: -6.99652 (min) -2.14667 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: y_wind: -10.1821 (min) -3.19184 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:57 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.369544, mean: 1.897654, max: 3.577108
12:54:57 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:54:57 DEBUG opendrift.models.physics_methods:1061: min: 3.311787, mean: 7.394999, max: 10.303757
12:54:57 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.311787, mean: 7.394999, max: 10.303757
12:54:57 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:54:57 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:57 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:54:57 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:54:57 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:54:57 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:54:57 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:54:57 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:54:57 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:54:57 DEBUG opendrift.models.physics_methods:1061: min: 3.311787, mean: 7.394999, max: 10.303757
12:54:57 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:54:57 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.007526, dN_50: 0.000591
12:54:57 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:54:57 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.05247063298665334
12:54:57 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:57 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:54:57 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:54:57 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1042 surface elements
12:54:57 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1042 surface elements
12:54:57 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1041 surface elements
12:54:57 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1040 surface elements
12:54:57 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1040 surface elements
12:54:57 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1039 surface elements
12:54:57 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1037 surface elements
12:54:57 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1036 surface elements
12:54:57 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1035 surface elements
12:54:57 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1034 surface elements
12:54:57 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1033 surface elements
12:54:57 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1032 surface elements
12:54:57 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1032 surface elements
12:54:57 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1031 surface elements
12:54:57 DEBUG opendrift.models.physics_methods:915: Advecting 1041 of 6000 elements above 0.100m with wind-sheared ocean current (0.022493 m/s - 0.339449 m/s)
12:54:57 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:54:57 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0016859481881622174 and 0.4697775072729364 m/s
12:54:57 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:54:57 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:54:57 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:57 INFO opendrift.models.basemodel:2038: 2024-11-10 01:51:18.915878 - step 86 of 120 - 6000 active elements (0 deactivated)
12:54:57 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:57 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:57 DEBUG opendrift.models.basemodel:2057: 34.56504758347415 <- latitude -> 35.138038847755546
12:54:57 DEBUG opendrift.models.basemodel:2062: 22.461625007914765 <- longitude -> 23.537548117317893
12:54:57 DEBUG opendrift.models.basemodel:2067: -77.93563040844377 <- z -> 0.0
12:54:57 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:54:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:54:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:57 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:54:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:54:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:54:57 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:54:57 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-10 00:00:00 (before)
2024-11-10 03:00:00 (after)
12:54:57 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-10 00:00:00) in space (linearNDFast)
12:54:57 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:57 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-10 03:00:00) in space (linearNDFast)
12:54:57 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:57 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-10 00:00:00, weight 0.38) and
after (2024-11-10 03:00:00, weight 0.62) in time
12:54:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:54:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:54:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:54:57 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:54:57 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-10 00:00:00 (before)
2024-11-10 03:00:00 (after)
12:54:57 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-10 00:00:00) in space (linearNDFast)
12:54:57 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:57 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-10 03:00:00) in space (linearNDFast)
12:54:57 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:57 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-10 00:00:00, weight 0.38) and
after (2024-11-10 03:00:00, weight 0.62) in time
12:54:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:57 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:57 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:57 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:54:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:54:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:54:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:54:57 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:57 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.733757 (min) -0.00356066 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.28587 (min) 0.177515 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: x_wind: -7.11256 (min) -1.66618 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: y_wind: -10.057 (min) -3.73605 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:54:57 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:57 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.411662, mean: 1.942923, max: 3.537952
12:54:57 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:54:57 DEBUG opendrift.models.physics_methods:1061: min: 3.495423, mean: 7.483970, max: 10.247208
12:54:57 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.495423, mean: 7.483970, max: 10.247208
12:54:57 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:54:57 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:57 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:54:57 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:54:57 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:54:57 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:54:57 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:54:57 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:54:57 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:54:57 DEBUG opendrift.models.physics_methods:1061: min: 3.495423, mean: 7.483970, max: 10.247208
12:54:57 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:54:57 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.007740, dN_50: 0.000607
12:54:57 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:54:57 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.051896327965273374
12:54:57 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:57 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:54:57 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:54:57 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1030 surface elements
12:54:57 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1030 surface elements
12:54:57 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1030 surface elements
12:54:57 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1029 surface elements
12:54:57 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1027 surface elements
12:54:57 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1026 surface elements
12:54:57 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1025 surface elements
12:54:57 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1026 surface elements
12:54:57 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1025 surface elements
12:54:58 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1024 surface elements
12:54:58 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1023 surface elements
12:54:58 DEBUG opendrift.models.physics_methods:915: Advecting 1028 of 6000 elements above 0.100m with wind-sheared ocean current (0.071403 m/s - 0.341885 m/s)
12:54:58 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:54:58 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0021991614125434046 and 0.4069238431919135 m/s
12:54:58 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:54:58 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:54:58 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:58 INFO opendrift.models.basemodel:2038: 2024-11-10 02:51:18.915878 - step 87 of 120 - 6000 active elements (0 deactivated)
12:54:58 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:58 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:58 DEBUG opendrift.models.basemodel:2057: 34.55802596339361 <- latitude -> 35.13645783725233
12:54:58 DEBUG opendrift.models.basemodel:2062: 22.441712858172394 <- longitude -> 23.533930168912995
12:54:58 DEBUG opendrift.models.basemodel:2067: -76.89755344998342 <- z -> 0.0
12:54:58 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:58 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:58 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:58 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:58 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:58 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:58 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:58 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:54:58 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:58 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:58 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:58 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:58 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:58 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:58 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:58 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:58 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:58 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:54:58 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:58 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:58 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:58 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:58 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:58 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:54:58 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:58 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:54:58 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:58 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:58 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:54:58 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:54:58 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-10 00:00:00 (before)
2024-11-10 03:00:00 (after)
12:54:58 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-10 00:00:00) in space (linearNDFast)
12:54:58 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:58 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-10 03:00:00) in space (linearNDFast)
12:54:58 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:58 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-10 00:00:00, weight 0.05) and
after (2024-11-10 03:00:00, weight 0.95) in time
12:54:58 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:58 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:58 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:58 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:54:58 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:58 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:54:58 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:58 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:58 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:54:58 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:54:58 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-10 00:00:00 (before)
2024-11-10 03:00:00 (after)
12:54:58 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-10 00:00:00) in space (linearNDFast)
12:54:58 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:58 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-10 03:00:00) in space (linearNDFast)
12:54:58 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:58 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-10 00:00:00, weight 0.05) and
after (2024-11-10 03:00:00, weight 0.95) in time
12:54:58 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:58 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:58 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:54:58 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:54:58 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:54:58 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:54:58 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:54:58 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:54:58 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:54:58 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.708431 (min) 0.0138729 (max)
12:54:58 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.27102 (min) 0.182844 (max)
12:54:58 DEBUG opendrift.models.basemodel.environment:905: x_wind: -7.20976 (min) -1.20274 (max)
12:54:58 DEBUG opendrift.models.basemodel.environment:905: y_wind: -9.94666 (min) -4.31713 (max)
12:54:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:54:58 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:54:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:54:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:54:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:54:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:54:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:54:58 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:54:58 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:54:58 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:54:58 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:54:58 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:54:58 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:54:58 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:54:58 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:54:58 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:54:58 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:54:58 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.494070, mean: 1.984517, max: 3.493959
12:54:58 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:54:58 DEBUG opendrift.models.physics_methods:1061: min: 3.829341, mean: 7.563850, max: 10.183299
12:54:58 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.829341, mean: 7.563850, max: 10.183299
12:54:58 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:54:58 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:54:58 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:54:58 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:54:58 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:54:58 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:54:58 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:54:58 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:54:58 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:54:58 DEBUG opendrift.models.physics_methods:1061: min: 3.829341, mean: 7.563850, max: 10.183299
12:54:58 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:54:58 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.007968, dN_50: 0.000625
12:54:58 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:54:58 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.05125106994622453
12:54:58 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:54:58 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:54:58 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:54:58 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1023 surface elements
12:54:58 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1022 surface elements
12:54:58 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1022 surface elements
12:54:58 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1021 surface elements
12:54:58 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1020 surface elements
12:54:58 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1019 surface elements
12:54:58 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1020 surface elements
12:54:58 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1024 surface elements
12:54:58 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1022 surface elements
12:54:58 DEBUG opendrift.models.physics_methods:915: Advecting 1025 of 6000 elements above 0.100m with wind-sheared ocean current (0.002466 m/s - 0.339542 m/s)
12:54:58 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:54:58 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.002379579192403369 and 0.5041930911674757 m/s
12:54:58 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:54:58 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:54:58 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:54:58 INFO opendrift.models.basemodel:2038: 2024-11-10 03:51:18.915878 - step 88 of 120 - 6000 active elements (0 deactivated)
12:54:58 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:54:58 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:54:58 DEBUG opendrift.models.basemodel:2057: 34.5412843758595 <- latitude -> 35.13099293612016
12:54:58 DEBUG opendrift.models.basemodel:2062: 22.41405175202071 <- longitude -> 23.53093543361146
12:54:58 DEBUG opendrift.models.basemodel:2067: -75.70240394539164 <- z -> 0.0
12:54:58 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:54:58 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:58 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:54:58 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:58 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:54:58 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:58 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:58 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:54:58 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:58 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:58 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:58 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:58 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:54:58 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:58 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:54:58 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:58 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:58 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:54:58 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:54:58 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:54:58 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:58 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:58 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:58 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:54:58 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:58 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:54:58 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:58 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:58 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:54:58 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:54:58 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-10 03:00:00 (before)
2024-11-10 06:00:00 (after)
12:54:58 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
12:54:58 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
12:54:58 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:54:58 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:54:58 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:54:58 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
12:54:58 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 22x23x19) for time after (2024-11-10 06:00:00)
12:54:58 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-10 03:00:00) in space (linearNDFast)
12:54:58 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:58 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-10 06:00:00) in space (linearNDFast)
12:54:58 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:54:58 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-10 03:00:00, weight 0.71) and
after (2024-11-10 06:00:00, weight 0.29) in time
12:54:58 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:54:58 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:54:58 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:54:58 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:54:58 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:54:58 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:54:58 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:54:58 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:54:58 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:54:58 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:54:58 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-10 03:00:00 (before)
2024-11-10 06:00:00 (after)
12:54:58 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_wind to retrieve x_wind
12:55:01 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_wind to retrieve y_wind
12:55:04 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:55:04 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:55:04 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:55:04 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 720x361x1) for time after (2024-11-10 06:00:00)
12:55:04 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-10 03:00:00) in space (linearNDFast)
12:55:04 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:04 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-10 06:00:00) in space (linearNDFast)
12:55:04 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:04 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-10 03:00:00, weight 0.71) and
after (2024-11-10 06:00:00, weight 0.29) in time
12:55:04 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:04 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:04 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:04 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:55:04 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:55:04 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:55:04 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:55:04 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:04 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.756381 (min) 0.0101105 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.31367 (min) 0.162613 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: x_wind: -7.43796 (min) -1.30743 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: y_wind: -10.0087 (min) -4.33318 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:04 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.503951, mean: 2.055786, max: 3.511965
12:55:04 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:55:04 DEBUG opendrift.models.physics_methods:1061: min: 3.867441, mean: 7.703390, max: 10.209503
12:55:04 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.867441, mean: 7.703390, max: 10.209503
12:55:04 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:55:04 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:04 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:55:04 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:55:04 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:55:04 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:55:04 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:55:04 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:55:04 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:55:04 DEBUG opendrift.models.physics_methods:1061: min: 3.867441, mean: 7.703391, max: 10.209503
12:55:04 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:55:04 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.008130, dN_50: 0.000638
12:55:04 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:55:04 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.0515151561221444
12:55:04 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:04 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:55:04 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:55:04 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1021 surface elements
12:55:04 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1021 surface elements
12:55:04 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1020 surface elements
12:55:04 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1018 surface elements
12:55:04 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1017 surface elements
12:55:04 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1016 surface elements
12:55:04 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1015 surface elements
12:55:04 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1014 surface elements
12:55:04 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1012 surface elements
12:55:04 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1011 surface elements
12:55:04 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1010 surface elements
12:55:04 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1010 surface elements
12:55:04 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1009 surface elements
12:55:04 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1008 surface elements
12:55:04 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1007 surface elements
12:55:04 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1006 surface elements
12:55:04 DEBUG opendrift.models.physics_methods:915: Advecting 1011 of 6000 elements above 0.100m with wind-sheared ocean current (0.041035 m/s - 0.342827 m/s)
12:55:04 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:55:04 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.00010955146658270836 and 0.42017592004148496 m/s
12:55:04 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:55:04 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:55:04 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:04 INFO opendrift.models.basemodel:2038: 2024-11-10 04:51:18.915878 - step 89 of 120 - 6000 active elements (0 deactivated)
12:55:04 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:04 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:04 DEBUG opendrift.models.basemodel:2057: 34.52525563659224 <- latitude -> 35.12857381160087
12:55:04 DEBUG opendrift.models.basemodel:2062: 22.381721486142553 <- longitude -> 23.52490373490963
12:55:04 DEBUG opendrift.models.basemodel:2067: -75.11533772462604 <- z -> 0.0
12:55:04 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:04 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:04 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:04 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:55:04 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:04 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:04 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:04 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:04 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:04 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:04 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:55:04 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:04 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:04 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:04 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:04 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:55:04 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:55:04 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:04 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:55:04 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:55:04 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-10 03:00:00 (before)
2024-11-10 06:00:00 (after)
12:55:04 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-10 03:00:00) in space (linearNDFast)
12:55:04 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:04 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-10 06:00:00) in space (linearNDFast)
12:55:04 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:04 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-10 03:00:00, weight 0.38) and
after (2024-11-10 06:00:00, weight 0.62) in time
12:55:04 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:04 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:04 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:55:04 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:55:04 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:04 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:55:04 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:55:04 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-10 03:00:00 (before)
2024-11-10 06:00:00 (after)
12:55:04 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-10 03:00:00) in space (linearNDFast)
12:55:04 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:04 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-10 06:00:00) in space (linearNDFast)
12:55:04 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:04 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-10 03:00:00, weight 0.38) and
after (2024-11-10 06:00:00, weight 0.62) in time
12:55:04 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:04 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:04 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:04 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:55:04 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:55:04 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:55:04 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:55:04 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:04 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.719685 (min) 0.0487649 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.390768 (min) 0.1381 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: x_wind: -7.65455 (min) -1.4628 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: y_wind: -10.085 (min) -4.20047 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:04 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.486679, mean: 2.131346, max: 3.528152
12:55:04 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:55:04 DEBUG opendrift.models.physics_methods:1061: min: 3.800590, mean: 7.848492, max: 10.233006
12:55:04 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.800590, mean: 7.848492, max: 10.233006
12:55:04 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:55:04 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:04 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:55:04 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:55:04 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:55:04 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:55:04 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:55:04 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:55:04 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:55:04 DEBUG opendrift.models.physics_methods:1061: min: 3.800590, mean: 7.848492, max: 10.233006
12:55:04 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:55:04 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.008293, dN_50: 0.000651
12:55:04 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:55:04 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.051752585124629505
12:55:04 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:04 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:55:04 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:55:04 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1005 surface elements
12:55:04 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1004 surface elements
12:55:04 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1004 surface elements
12:55:04 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1005 surface elements
12:55:04 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1004 surface elements
12:55:04 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1003 surface elements
12:55:04 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1002 surface elements
12:55:04 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1001 surface elements
12:55:04 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 999 surface elements
12:55:04 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1000 surface elements
12:55:04 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1001 surface elements
12:55:04 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 999 surface elements
12:55:04 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 998 surface elements
12:55:04 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 997 surface elements
12:55:04 DEBUG opendrift.models.physics_methods:915: Advecting 1003 of 6000 elements above 0.100m with wind-sheared ocean current (0.026004 m/s - 0.341148 m/s)
12:55:04 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:55:04 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0017535503768980605 and 0.47908382173916075 m/s
12:55:04 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:55:04 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:55:04 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:04 INFO opendrift.models.basemodel:2038: 2024-11-10 05:51:18.915878 - step 90 of 120 - 6000 active elements (0 deactivated)
12:55:04 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:04 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:04 DEBUG opendrift.models.basemodel:2057: 34.5089784418426 <- latitude -> 35.13070094093084
12:55:04 DEBUG opendrift.models.basemodel:2062: 22.35319206515283 <- longitude -> 23.528322859476276
12:55:04 DEBUG opendrift.models.basemodel:2067: -74.11172899848576 <- z -> 0.0
12:55:04 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:04 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:04 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:04 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:55:04 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:04 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:04 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:04 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:04 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:04 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:04 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:55:04 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:04 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:04 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:04 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:04 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:55:04 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:55:04 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:04 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:55:04 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:55:04 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-10 03:00:00 (before)
2024-11-10 06:00:00 (after)
12:55:04 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-10 03:00:00) in space (linearNDFast)
12:55:04 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:04 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-10 06:00:00) in space (linearNDFast)
12:55:04 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:04 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-10 03:00:00, weight 0.05) and
after (2024-11-10 06:00:00, weight 0.95) in time
12:55:04 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:04 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:04 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:55:04 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:55:04 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:04 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:55:04 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:55:04 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-10 03:00:00 (before)
2024-11-10 06:00:00 (after)
12:55:04 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-10 03:00:00) in space (linearNDFast)
12:55:04 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:04 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-10 06:00:00) in space (linearNDFast)
12:55:04 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:04 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-10 03:00:00, weight 0.05) and
after (2024-11-10 06:00:00, weight 0.95) in time
12:55:04 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:04 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:04 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:04 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:55:04 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:55:04 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:55:04 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:55:04 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:04 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.682981 (min) 0.0353628 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.472438 (min) 0.1495 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: x_wind: -7.84527 (min) -1.49455 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: y_wind: -10.1672 (min) -4.08055 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:55:04 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:04 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.474616, mean: 2.203312, max: 3.566893
12:55:04 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:55:04 DEBUG opendrift.models.physics_methods:1061: min: 3.753190, mean: 7.983443, max: 10.289034
12:55:04 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.753190, mean: 7.983443, max: 10.289034
12:55:04 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:55:04 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:04 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:55:04 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:55:04 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:55:04 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:55:04 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:55:04 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:55:04 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:55:04 DEBUG opendrift.models.physics_methods:1061: min: 3.753190, mean: 7.983443, max: 10.289035
12:55:04 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:55:04 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.008475, dN_50: 0.000665
12:55:04 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:55:04 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.05232080846049499
12:55:04 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:04 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:55:04 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:55:04 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 998 surface elements
12:55:04 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 998 surface elements
12:55:04 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 997 surface elements
12:55:04 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 996 surface elements
12:55:04 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 995 surface elements
12:55:04 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 994 surface elements
12:55:04 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 992 surface elements
12:55:04 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 993 surface elements
12:55:04 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 991 surface elements
12:55:04 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 990 surface elements
12:55:04 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 989 surface elements
12:55:04 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 988 surface elements
12:55:04 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 988 surface elements
12:55:04 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 987 surface elements
12:55:04 DEBUG opendrift.models.physics_methods:915: Advecting 993 of 6000 elements above 0.100m with wind-sheared ocean current (0.005595 m/s - 0.338370 m/s)
12:55:04 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:55:04 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0031942751042075422 and 0.45174135874741206 m/s
12:55:04 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:55:04 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:55:04 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:04 INFO opendrift.models.basemodel:2038: 2024-11-10 06:51:18.915878 - step 91 of 120 - 6000 active elements (0 deactivated)
12:55:04 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:04 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:04 DEBUG opendrift.models.basemodel:2057: 34.49380976843931 <- latitude -> 35.12680646164118
12:55:04 DEBUG opendrift.models.basemodel:2062: 22.32532418742484 <- longitude -> 23.531461047876956
12:55:04 DEBUG opendrift.models.basemodel:2067: -72.66493448377227 <- z -> 0.0
12:55:04 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:04 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:04 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:04 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:55:04 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:04 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:04 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:04 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:04 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:04 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:04 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:55:04 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:04 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:04 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:04 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:04 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:55:04 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:55:04 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:04 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:04 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:55:04 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:55:04 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-10 06:00:00 (before)
2024-11-10 09:00:00 (after)
12:55:04 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
12:55:05 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
12:55:05 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:55:05 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:55:05 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:55:05 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
12:55:05 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 23x24x19) for time after (2024-11-10 09:00:00)
12:55:05 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-10 06:00:00) in space (linearNDFast)
12:55:05 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:05 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-10 09:00:00) in space (linearNDFast)
12:55:05 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:05 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-10 06:00:00, weight 0.71) and
after (2024-11-10 09:00:00, weight 0.29) in time
12:55:05 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:05 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:05 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:05 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:55:05 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:05 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:55:05 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:05 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:05 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:55:05 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:55:05 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-10 06:00:00 (before)
2024-11-10 09:00:00 (after)
12:55:05 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_wind to retrieve x_wind
12:55:08 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_wind to retrieve y_wind
12:55:11 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:55:11 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:55:11 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:55:11 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 720x361x1) for time after (2024-11-10 09:00:00)
12:55:11 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-10 06:00:00) in space (linearNDFast)
12:55:11 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:11 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-10 09:00:00) in space (linearNDFast)
12:55:11 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:11 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-10 06:00:00, weight 0.71) and
after (2024-11-10 09:00:00, weight 0.29) in time
12:55:11 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:11 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:11 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:11 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:11 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:55:11 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:55:11 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:55:11 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:55:11 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:11 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.762873 (min) 0.0602389 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.449179 (min) 0.112763 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: x_wind: -7.8112 (min) -1.32839 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: y_wind: -9.80448 (min) -3.43776 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:11 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.350127, mean: 2.058190, max: 3.386600
12:55:11 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:55:11 DEBUG opendrift.models.physics_methods:1061: min: 3.223609, mean: 7.696502, max: 10.025626
12:55:11 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.223609, mean: 7.696502, max: 10.025626
12:55:11 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:55:11 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:11 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:55:11 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:55:11 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:55:11 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:55:11 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:55:11 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:55:11 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:55:11 DEBUG opendrift.models.physics_methods:1061: min: 3.223609, mean: 7.696503, max: 10.025626
12:55:11 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:55:11 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.009022, dN_50: 0.000708
12:55:11 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:55:11 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.049676396407201825
12:55:11 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:11 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:55:11 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:55:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 987 surface elements
12:55:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 987 surface elements
12:55:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 986 surface elements
12:55:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 986 surface elements
12:55:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 985 surface elements
12:55:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 984 surface elements
12:55:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 985 surface elements
12:55:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 984 surface elements
12:55:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 983 surface elements
12:55:11 DEBUG opendrift.models.physics_methods:915: Advecting 988 of 6000 elements above 0.100m with wind-sheared ocean current (0.032145 m/s - 0.329633 m/s)
12:55:11 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:55:11 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0003736112535820404 and 0.4456414110334116 m/s
12:55:11 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:55:11 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:55:11 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:11 INFO opendrift.models.basemodel:2038: 2024-11-10 07:51:18.915878 - step 92 of 120 - 6000 active elements (0 deactivated)
12:55:11 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:11 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:11 DEBUG opendrift.models.basemodel:2057: 34.48504617343295 <- latitude -> 35.12184453512679
12:55:11 DEBUG opendrift.models.basemodel:2062: 22.286142019917058 <- longitude -> 23.528746820206894
12:55:11 DEBUG opendrift.models.basemodel:2067: -72.09345566034177 <- z -> 0.0
12:55:11 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:11 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:11 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:11 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:11 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:11 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:11 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:11 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:55:11 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:11 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:11 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:11 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:11 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:11 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:11 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:11 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:11 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:11 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:55:11 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:11 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:11 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:11 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:11 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:11 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:55:11 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:11 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:55:11 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:11 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:11 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:55:11 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:55:11 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-10 06:00:00 (before)
2024-11-10 09:00:00 (after)
12:55:11 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-10 06:00:00) in space (linearNDFast)
12:55:11 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:11 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-10 09:00:00) in space (linearNDFast)
12:55:11 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:11 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-10 06:00:00, weight 0.38) and
after (2024-11-10 09:00:00, weight 0.62) in time
12:55:11 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:11 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:11 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:11 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:55:11 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:11 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:55:11 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:11 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:11 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:55:11 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:55:11 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-10 06:00:00 (before)
2024-11-10 09:00:00 (after)
12:55:11 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-10 06:00:00) in space (linearNDFast)
12:55:11 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:11 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-10 09:00:00) in space (linearNDFast)
12:55:11 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:11 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-10 06:00:00, weight 0.38) and
after (2024-11-10 09:00:00, weight 0.62) in time
12:55:11 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:11 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:11 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:11 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:11 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:55:11 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:55:11 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:55:11 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:55:11 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:11 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.793377 (min) 0.029681 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.485055 (min) 0.0721125 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: x_wind: -7.73026 (min) -1.08095 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: y_wind: -9.36869 (min) -2.63127 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:11 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.199063, mean: 1.886799, max: 3.163230
12:55:11 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:55:11 DEBUG opendrift.models.physics_methods:1061: min: 2.430666, mean: 7.342065, max: 9.689358
12:55:11 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.430666, mean: 7.342065, max: 9.689358
12:55:11 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:55:11 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:11 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:55:11 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:55:11 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:55:11 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:55:11 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:55:11 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:55:11 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:55:11 DEBUG opendrift.models.physics_methods:1061: min: 2.430666, mean: 7.342065, max: 9.689359
12:55:11 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:55:11 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.009692, dN_50: 0.000761
12:55:11 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:55:11 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.046400176555287484
12:55:11 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:11 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:55:11 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:55:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 983 surface elements
12:55:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 982 surface elements
12:55:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 981 surface elements
12:55:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 985 surface elements
12:55:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 985 surface elements
12:55:11 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 984 surface elements
12:55:11 DEBUG opendrift.models.physics_methods:915: Advecting 988 of 6000 elements above 0.100m with wind-sheared ocean current (0.106360 m/s - 0.329527 m/s)
12:55:11 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:55:11 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0008931157718572477 and 0.40113677932116243 m/s
12:55:11 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:55:11 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:55:11 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:11 INFO opendrift.models.basemodel:2038: 2024-11-10 08:51:18.915878 - step 93 of 120 - 6000 active elements (0 deactivated)
12:55:11 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:11 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:11 DEBUG opendrift.models.basemodel:2057: 34.46779169768627 <- latitude -> 35.11996932585943
12:55:11 DEBUG opendrift.models.basemodel:2062: 22.25601978299968 <- longitude -> 23.526269610188017
12:55:11 DEBUG opendrift.models.basemodel:2067: -71.32051561846549 <- z -> 0.0
12:55:11 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:11 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:11 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:11 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:11 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:11 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:11 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:11 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:55:11 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:11 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:11 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:11 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:11 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:11 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:11 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:11 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:11 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:11 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:55:11 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:11 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:11 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:11 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:11 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:11 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:55:11 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:11 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:55:11 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:11 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:11 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:55:11 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:55:11 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-10 06:00:00 (before)
2024-11-10 09:00:00 (after)
12:55:11 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-10 06:00:00) in space (linearNDFast)
12:55:11 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:11 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-10 09:00:00) in space (linearNDFast)
12:55:11 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:11 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-10 06:00:00, weight 0.05) and
after (2024-11-10 09:00:00, weight 0.95) in time
12:55:11 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:11 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:11 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:11 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:55:11 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:11 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:55:11 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:11 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:11 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:55:11 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:55:11 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-10 06:00:00 (before)
2024-11-10 09:00:00 (after)
12:55:11 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-10 06:00:00) in space (linearNDFast)
12:55:11 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:11 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-10 09:00:00) in space (linearNDFast)
12:55:11 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:11 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-10 06:00:00, weight 0.05) and
after (2024-11-10 09:00:00, weight 0.95) in time
12:55:11 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:11 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:11 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:11 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:11 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:55:11 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:55:11 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:55:11 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:55:11 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:11 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.717392 (min) 0.0438734 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.484715 (min) 0.0555241 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: x_wind: -7.62183 (min) -0.962461 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: y_wind: -8.9274 (min) -1.88732 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:55:11 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:11 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.112411, mean: 1.726710, max: 3.003688
12:55:11 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:55:11 DEBUG opendrift.models.physics_methods:1061: min: 1.826564, mean: 6.992250, max: 9.441848
12:55:11 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.826564, mean: 6.992250, max: 9.441848
12:55:11 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:55:11 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:11 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:55:11 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:55:11 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:55:11 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:55:11 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:55:11 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:55:11 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:55:11 DEBUG opendrift.models.physics_methods:1061: min: 1.826564, mean: 6.992249, max: 9.441849
12:55:11 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:55:12 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.010439, dN_50: 0.000819
12:55:12 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:55:12 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.04406012730128656
12:55:12 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:12 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:55:12 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:55:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 987 surface elements
12:55:12 DEBUG opendrift.models.physics_methods:915: Advecting 994 of 6000 elements above 0.100m with wind-sheared ocean current (0.002707 m/s - 0.324581 m/s)
12:55:12 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:55:12 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0005524073059725985 and 0.43149785409241903 m/s
12:55:12 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:55:12 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:55:12 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:12 INFO opendrift.models.basemodel:2038: 2024-11-10 09:51:18.915878 - step 94 of 120 - 6000 active elements (0 deactivated)
12:55:12 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:12 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:12 DEBUG opendrift.models.basemodel:2057: 34.45657479799011 <- latitude -> 35.11464236197273
12:55:12 DEBUG opendrift.models.basemodel:2062: 22.228820278855657 <- longitude -> 23.52928153737726
12:55:12 DEBUG opendrift.models.basemodel:2067: -70.48179059131353 <- z -> 0.0
12:55:12 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:12 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:12 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:12 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:12 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:12 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:12 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:12 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:55:12 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:12 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:12 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:12 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:12 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:12 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:12 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:12 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:12 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:12 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:55:12 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:12 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:12 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:12 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:12 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:12 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:55:12 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:12 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:55:12 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:12 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:12 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:55:12 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:55:12 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-10 09:00:00 (before)
2024-11-10 12:00:00 (after)
12:55:12 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
12:55:12 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
12:55:12 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:55:12 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:55:12 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:55:12 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 24x24x19) for time after (2024-11-10 12:00:00)
12:55:12 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-10 09:00:00) in space (linearNDFast)
12:55:12 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:12 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-10 12:00:00) in space (linearNDFast)
12:55:12 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:12 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-10 09:00:00, weight 0.71) and
after (2024-11-10 12:00:00, weight 0.29) in time
12:55:12 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:12 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:12 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:12 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:55:12 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:12 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:55:12 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:12 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:12 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:55:12 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:55:12 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-10 09:00:00 (before)
2024-11-10 12:00:00 (after)
12:55:12 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_wind to retrieve x_wind
12:55:15 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_wind to retrieve y_wind
12:55:19 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:55:19 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:55:19 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:55:19 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 720x361x1) for time after (2024-11-10 12:00:00)
12:55:19 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-10 09:00:00) in space (linearNDFast)
12:55:19 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:19 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-10 12:00:00) in space (linearNDFast)
12:55:19 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:19 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-10 09:00:00, weight 0.71) and
after (2024-11-10 12:00:00, weight 0.29) in time
12:55:19 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:19 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:19 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:19 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:19 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:55:19 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:55:19 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:55:19 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:55:19 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:19 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.802302 (min) 0.0520118 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.504016 (min) 0.0544833 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: x_wind: -7.64933 (min) -0.632423 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: y_wind: -8.82076 (min) -1.85832 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:19 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.094791, mean: 1.700827, max: 2.887310
12:55:19 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:55:19 DEBUG opendrift.models.physics_methods:1061: min: 1.677312, mean: 6.930352, max: 9.257129
12:55:19 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.677312, mean: 6.930352, max: 9.257129
12:55:19 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:55:19 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:19 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:55:19 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:55:19 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:55:19 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:55:19 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:55:19 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:55:19 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:55:19 DEBUG opendrift.models.physics_methods:1061: min: 1.677312, mean: 6.930352, max: 9.257130
12:55:19 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:55:19 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.010915, dN_50: 0.000857
12:55:19 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:55:19 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.04235317622092032
12:55:19 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:19 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:55:19 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:55:19 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 991 surface elements
12:55:19 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 990 surface elements
12:55:19 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 989 surface elements
12:55:19 DEBUG opendrift.models.physics_methods:915: Advecting 996 of 6000 elements above 0.100m with wind-sheared ocean current (0.017555 m/s - 0.317374 m/s)
12:55:19 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:55:19 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0022201257636422036 and 0.453444223813396 m/s
12:55:19 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:55:19 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:55:19 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:19 INFO opendrift.models.basemodel:2038: 2024-11-10 10:51:18.915878 - step 95 of 120 - 6000 active elements (0 deactivated)
12:55:19 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:19 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:19 DEBUG opendrift.models.basemodel:2057: 34.44876315428706 <- latitude -> 35.11950410597326
12:55:19 DEBUG opendrift.models.basemodel:2062: 22.18673136447132 <- longitude -> 23.5285359676512
12:55:19 DEBUG opendrift.models.basemodel:2067: -69.04493943540213 <- z -> 0.0
12:55:19 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:19 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:19 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:19 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:19 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:19 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:19 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:19 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:55:19 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:19 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:19 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:19 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:19 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:19 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:19 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:19 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:19 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:19 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:55:19 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:19 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:19 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:19 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:19 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:19 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:55:19 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:19 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:55:19 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:19 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:19 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:55:19 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:55:19 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-10 09:00:00 (before)
2024-11-10 12:00:00 (after)
12:55:19 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-10 09:00:00) in space (linearNDFast)
12:55:19 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:19 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-10 12:00:00) in space (linearNDFast)
12:55:19 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:19 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-10 09:00:00, weight 0.38) and
after (2024-11-10 12:00:00, weight 0.62) in time
12:55:19 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:19 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:19 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:19 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:55:19 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:19 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:55:19 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:19 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:19 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:55:19 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:55:19 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-10 09:00:00 (before)
2024-11-10 12:00:00 (after)
12:55:19 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-10 09:00:00) in space (linearNDFast)
12:55:19 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:19 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-10 12:00:00) in space (linearNDFast)
12:55:19 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:19 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-10 09:00:00, weight 0.38) and
after (2024-11-10 12:00:00, weight 0.62) in time
12:55:19 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:19 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:19 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:19 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:19 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:55:19 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:55:19 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:55:19 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:55:19 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:19 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.867236 (min) 0.0288759 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.46158 (min) 0.061886 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: x_wind: -7.63794 (min) -0.31538 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: y_wind: -8.76837 (min) -1.99274 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:19 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.100134, mean: 1.693923, max: 2.834849
12:55:19 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:55:19 DEBUG opendrift.models.physics_methods:1061: min: 1.723931, mean: 6.912154, max: 9.172645
12:55:19 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.723931, mean: 6.912154, max: 9.172645
12:55:19 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:55:19 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:19 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:55:19 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:55:19 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:55:19 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:55:19 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:55:19 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:55:19 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:55:19 DEBUG opendrift.models.physics_methods:1061: min: 1.723931, mean: 6.912154, max: 9.172645
12:55:19 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:55:19 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.011345, dN_50: 0.000890
12:55:19 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:55:19 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.04158371784401413
12:55:19 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:19 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:55:19 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:55:19 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 989 surface elements
12:55:19 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 992 surface elements
12:55:19 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 993 surface elements
12:55:19 DEBUG opendrift.models.physics_methods:915: Advecting 998 of 6000 elements above 0.100m with wind-sheared ocean current (0.003938 m/s - 0.307865 m/s)
12:55:19 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:55:19 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.002025634379548517 and 0.42067375800945606 m/s
12:55:19 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:55:19 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:55:19 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:19 INFO opendrift.models.basemodel:2038: 2024-11-10 11:51:18.915878 - step 96 of 120 - 6000 active elements (0 deactivated)
12:55:19 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:19 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:19 DEBUG opendrift.models.basemodel:2057: 34.44105105556925 <- latitude -> 35.118767803114
12:55:19 DEBUG opendrift.models.basemodel:2062: 22.15627652867928 <- longitude -> 23.520059022872857
12:55:19 DEBUG opendrift.models.basemodel:2067: -67.64919873093805 <- z -> 0.0
12:55:19 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:19 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:19 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:19 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:19 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:19 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:19 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:19 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:55:19 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:19 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:19 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:19 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:19 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:19 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:19 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:19 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:19 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:19 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:55:19 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:19 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:19 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:19 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:19 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:19 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:55:19 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:19 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:55:19 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:19 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:19 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:55:19 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:55:19 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-10 09:00:00 (before)
2024-11-10 12:00:00 (after)
12:55:19 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-10 09:00:00) in space (linearNDFast)
12:55:19 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:19 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-10 12:00:00) in space (linearNDFast)
12:55:19 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:19 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-10 09:00:00, weight 0.05) and
after (2024-11-10 12:00:00, weight 0.95) in time
12:55:19 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:19 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:19 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:19 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:55:19 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:19 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:55:19 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:19 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:19 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:55:19 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:55:19 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-10 09:00:00 (before)
2024-11-10 12:00:00 (after)
12:55:19 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-10 09:00:00) in space (linearNDFast)
12:55:19 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:19 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-10 12:00:00) in space (linearNDFast)
12:55:19 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:19 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-10 09:00:00, weight 0.05) and
after (2024-11-10 12:00:00, weight 0.95) in time
12:55:19 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:19 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:19 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:19 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:19 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:55:19 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:55:19 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:55:19 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:55:19 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:19 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.908406 (min) 0.0285714 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.432871 (min) 0.0904016 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: x_wind: -7.63368 (min) -0.000204332 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: y_wind: -8.71677 (min) -2.16471 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:55:19 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:19 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.115275, mean: 1.683398, max: 2.782815
12:55:19 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:55:19 DEBUG opendrift.models.physics_methods:1061: min: 1.849684, mean: 6.886919, max: 9.088071
12:55:19 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.849684, mean: 6.886919, max: 9.088071
12:55:19 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:55:19 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:19 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:55:19 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:55:19 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:55:19 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:55:19 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:55:19 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:55:19 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:55:19 DEBUG opendrift.models.physics_methods:1061: min: 1.849684, mean: 6.886919, max: 9.088071
12:55:19 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:55:19 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.011793, dN_50: 0.000926
12:55:19 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:55:19 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.04082051390774717
12:55:19 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:19 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:55:19 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:55:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 995 surface elements
12:55:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 997 surface elements
12:55:20 DEBUG opendrift.models.physics_methods:915: Advecting 1011 of 6000 elements above 0.100m with wind-sheared ocean current (0.012068 m/s - 0.311866 m/s)
12:55:20 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:55:20 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.005218131939962175 and 0.46212223535195446 m/s
12:55:20 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:55:20 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:55:20 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:20 INFO opendrift.models.basemodel:2038: 2024-11-10 12:51:18.915878 - step 97 of 120 - 6000 active elements (0 deactivated)
12:55:20 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:20 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:20 DEBUG opendrift.models.basemodel:2057: 34.43817257711331 <- latitude -> 35.1163305205381
12:55:20 DEBUG opendrift.models.basemodel:2062: 22.12863780124022 <- longitude -> 23.513501941518804
12:55:20 DEBUG opendrift.models.basemodel:2067: -66.88635019981938 <- z -> 0.0
12:55:20 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:20 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:20 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:20 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:20 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:20 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:20 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:20 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:55:20 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:20 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:20 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:20 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:20 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:20 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:20 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:20 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:20 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:20 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:55:20 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:20 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:20 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:20 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:20 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:20 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:55:20 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:20 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:55:20 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:20 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:20 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:55:20 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:55:20 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-10 12:00:00 (before)
2024-11-10 15:00:00 (after)
12:55:20 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
12:55:20 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
12:55:20 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:55:20 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:55:20 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:55:20 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 26x25x18) for time after (2024-11-10 15:00:00)
12:55:20 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-10 12:00:00) in space (linearNDFast)
12:55:20 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:20 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-10 15:00:00) in space (linearNDFast)
12:55:20 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:20 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-10 12:00:00, weight 0.71) and
after (2024-11-10 15:00:00, weight 0.29) in time
12:55:20 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:20 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:20 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:20 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:55:20 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:20 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:55:20 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:20 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:20 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:55:20 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:55:20 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-10 12:00:00 (before)
2024-11-10 15:00:00 (after)
12:55:20 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_wind to retrieve x_wind
12:55:23 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_wind to retrieve y_wind
12:55:26 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:55:26 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:55:26 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:55:26 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 720x361x1) for time after (2024-11-10 15:00:00)
12:55:26 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-10 12:00:00) in space (linearNDFast)
12:55:26 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:26 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-10 15:00:00) in space (linearNDFast)
12:55:26 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:26 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-10 12:00:00, weight 0.71) and
after (2024-11-10 15:00:00, weight 0.29) in time
12:55:26 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:26 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:26 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:26 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:26 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:55:26 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:55:26 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:55:26 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:55:26 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:26 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.924064 (min) -0.00645978 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.377139 (min) 0.159277 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: x_wind: -7.51474 (min) -0.181195 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: y_wind: -8.60102 (min) -1.30945 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:26 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.042988, mean: 1.627387, max: 2.717130
12:55:26 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:55:26 DEBUG opendrift.models.physics_methods:1061: min: 1.129548, mean: 6.758481, max: 8.980175
12:55:26 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.129548, mean: 6.758481, max: 8.980175
12:55:26 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:55:26 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:26 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:55:26 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:55:26 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:55:26 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:55:26 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:55:26 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:55:26 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:55:26 DEBUG opendrift.models.physics_methods:1061: min: 1.129548, mean: 6.758481, max: 8.980175
12:55:26 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:55:26 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.012383, dN_50: 0.000972
12:55:26 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:55:26 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.039857100884343695
12:55:26 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:26 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:55:26 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:55:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1005 surface elements
12:55:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1006 surface elements
12:55:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1005 surface elements
12:55:26 DEBUG opendrift.models.physics_methods:915: Advecting 1015 of 6000 elements above 0.100m with wind-sheared ocean current (0.003379 m/s - 0.305482 m/s)
12:55:26 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:55:26 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0027875798824675392 and 0.4313664508415794 m/s
12:55:26 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:55:26 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:55:26 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:26 INFO opendrift.models.basemodel:2038: 2024-11-10 13:51:18.915878 - step 98 of 120 - 6000 active elements (0 deactivated)
12:55:26 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:26 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:26 DEBUG opendrift.models.basemodel:2057: 34.42364555896448 <- latitude -> 35.11946237511937
12:55:26 DEBUG opendrift.models.basemodel:2062: 22.092888732677977 <- longitude -> 23.500282789667175
12:55:26 DEBUG opendrift.models.basemodel:2067: -66.13833714141967 <- z -> 0.0
12:55:26 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:26 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:26 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:26 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:26 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:26 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:26 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:26 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:55:26 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:26 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:26 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:26 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:26 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:26 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:26 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:26 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:26 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:26 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:55:26 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:26 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:26 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:26 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:26 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:26 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:55:26 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:26 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:55:26 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:26 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:26 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:55:26 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:55:26 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-10 12:00:00 (before)
2024-11-10 15:00:00 (after)
12:55:26 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-10 12:00:00) in space (linearNDFast)
12:55:26 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:26 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-10 15:00:00) in space (linearNDFast)
12:55:26 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:26 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-10 12:00:00, weight 0.38) and
after (2024-11-10 15:00:00, weight 0.62) in time
12:55:26 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:26 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:26 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:26 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:55:26 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:26 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:55:26 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:26 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:26 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:55:26 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:55:26 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-10 12:00:00 (before)
2024-11-10 15:00:00 (after)
12:55:26 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-10 12:00:00) in space (linearNDFast)
12:55:26 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:26 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-10 15:00:00) in space (linearNDFast)
12:55:26 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:26 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-10 12:00:00, weight 0.38) and
after (2024-11-10 15:00:00, weight 0.62) in time
12:55:26 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:26 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:26 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:26 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:26 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:55:26 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:55:26 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:55:26 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:55:26 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:26 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.859596 (min) -0.0280313 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.365814 (min) 0.289438 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: x_wind: -7.41025 (min) -0.349508 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: y_wind: -8.48824 (min) -0.253638 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:26 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.004588, mean: 1.568080, max: 2.629905
12:55:26 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:55:26 DEBUG opendrift.models.physics_methods:1061: min: 0.368996, mean: 6.619913, max: 8.834860
12:55:26 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 0.368996, mean: 6.619913, max: 8.834860
12:55:26 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:55:26 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:26 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:55:26 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:55:26 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:55:26 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:55:26 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:55:26 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:55:26 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:55:26 DEBUG opendrift.models.physics_methods:1061: min: 0.368996, mean: 6.619914, max: 8.834860
12:55:26 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:55:26 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.013023, dN_50: 0.001022
12:55:26 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:55:26 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.03857775046828911
12:55:26 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:26 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:55:26 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:55:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1006 surface elements
12:55:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1008 surface elements
12:55:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1007 surface elements
12:55:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1007 surface elements
12:55:26 DEBUG opendrift.models.physics_methods:915: Advecting 1010 of 6000 elements above 0.100m with wind-sheared ocean current (0.014286 m/s - 0.296204 m/s)
12:55:26 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:55:26 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0006340066027842817 and 0.44209196924331534 m/s
12:55:26 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:55:26 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:55:26 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:26 INFO opendrift.models.basemodel:2038: 2024-11-10 14:51:18.915878 - step 99 of 120 - 6000 active elements (0 deactivated)
12:55:26 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:26 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:26 DEBUG opendrift.models.basemodel:2057: 34.41217623507991 <- latitude -> 35.11443341920074
12:55:26 DEBUG opendrift.models.basemodel:2062: 22.06406181226759 <- longitude -> 23.49328280138753
12:55:26 DEBUG opendrift.models.basemodel:2067: -65.54301563055715 <- z -> 0.0
12:55:26 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:26 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:26 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:26 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:26 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:26 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:26 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:26 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:55:26 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:26 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:26 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:26 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:26 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:26 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:26 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:26 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:26 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:26 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:55:26 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:26 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:26 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:26 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:26 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:26 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:55:26 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:26 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:55:26 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:26 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:26 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:55:26 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:55:26 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-10 12:00:00 (before)
2024-11-10 15:00:00 (after)
12:55:26 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-10 12:00:00) in space (linearNDFast)
12:55:26 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:26 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-10 15:00:00) in space (linearNDFast)
12:55:26 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:26 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-10 12:00:00, weight 0.05) and
after (2024-11-10 15:00:00, weight 0.95) in time
12:55:26 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:26 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:26 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:26 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:55:26 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:26 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:55:26 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:26 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:26 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:55:26 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:55:26 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-10 12:00:00 (before)
2024-11-10 15:00:00 (after)
12:55:26 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-10 12:00:00) in space (linearNDFast)
12:55:26 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:26 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-10 15:00:00) in space (linearNDFast)
12:55:26 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:26 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-10 12:00:00, weight 0.05) and
after (2024-11-10 15:00:00, weight 0.95) in time
12:55:26 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:26 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:26 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:26 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:26 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:55:26 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:55:26 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:55:26 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:55:26 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:26 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.875174 (min) -0.0256512 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.350091 (min) 0.420863 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: x_wind: -7.34494 (min) -0.534078 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: y_wind: -8.38474 (min) 0.802439 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:55:26 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:26 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.022857, mean: 1.511574, max: 2.571601
12:55:26 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:55:26 DEBUG opendrift.models.physics_methods:1061: min: 0.823644, mean: 6.486728, max: 8.736377
12:55:26 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 0.823644, mean: 6.486728, max: 8.736377
12:55:26 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:55:26 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:26 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:55:26 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:55:26 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:55:26 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:55:26 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:55:26 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:55:26 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:55:26 DEBUG opendrift.models.physics_methods:1061: min: 0.823644, mean: 6.486729, max: 8.736377
12:55:26 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:55:26 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.013679, dN_50: 0.001074
12:55:26 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:55:26 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.037722584395813705
12:55:26 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:26 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:55:26 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:55:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1006 surface elements
12:55:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1006 surface elements
12:55:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1005 surface elements
12:55:26 DEBUG opendrift.models.physics_methods:915: Advecting 1012 of 6000 elements above 0.100m with wind-sheared ocean current (0.026347 m/s - 0.288006 m/s)
12:55:26 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:55:26 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0032562326131906514 and 0.4681391040911701 m/s
12:55:26 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:55:26 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:55:26 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:26 INFO opendrift.models.basemodel:2038: 2024-11-10 15:51:18.915878 - step 100 of 120 - 6000 active elements (0 deactivated)
12:55:26 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:26 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:26 DEBUG opendrift.models.basemodel:2057: 34.40493696744212 <- latitude -> 35.10596719371663
12:55:26 DEBUG opendrift.models.basemodel:2062: 22.039730347068932 <- longitude -> 23.492361365264678
12:55:26 DEBUG opendrift.models.basemodel:2067: -64.99845695662985 <- z -> 0.0
12:55:26 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:26 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:26 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:26 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:26 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:26 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:26 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:26 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:55:26 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:26 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:26 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:26 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:26 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:26 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:26 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:26 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:26 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:26 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:55:26 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:26 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:26 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:26 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:26 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:26 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:55:26 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:26 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:55:26 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:26 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:26 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:55:26 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:55:26 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-10 15:00:00 (before)
2024-11-10 18:00:00 (after)
12:55:26 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
12:55:27 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
12:55:27 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:55:27 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:55:27 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:55:27 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 26x25x18) for time after (2024-11-10 18:00:00)
12:55:27 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-10 15:00:00) in space (linearNDFast)
12:55:27 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:27 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-10 18:00:00) in space (linearNDFast)
12:55:27 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:27 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-10 15:00:00, weight 0.71) and
after (2024-11-10 18:00:00, weight 0.29) in time
12:55:27 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:27 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:27 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:27 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:55:27 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:27 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:55:27 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:27 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:27 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:55:27 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:55:27 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-10 15:00:00 (before)
2024-11-10 18:00:00 (after)
12:55:27 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_wind to retrieve x_wind
12:55:31 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_wind to retrieve y_wind
12:55:34 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:55:34 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:55:34 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:55:34 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 720x361x1) for time after (2024-11-10 18:00:00)
12:55:34 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-10 15:00:00) in space (linearNDFast)
12:55:34 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:34 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-10 18:00:00) in space (linearNDFast)
12:55:34 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:34 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-10 15:00:00, weight 0.71) and
after (2024-11-10 18:00:00, weight 0.29) in time
12:55:34 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:34 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:34 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:34 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:34 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:55:34 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:55:34 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:55:34 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:55:34 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:34 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.84494 (min) -0.0232696 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.335276 (min) 0.487737 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:905: x_wind: -7.25979 (min) -1.06235 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:905: y_wind: -7.90665 (min) 0.00350934 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:55:34 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:34 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.027763, mean: 1.458245, max: 2.457423
12:55:34 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:55:34 DEBUG opendrift.models.physics_methods:1061: min: 0.907749, mean: 6.352413, max: 8.540230
12:55:34 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 0.907749, mean: 6.352413, max: 8.540230
12:55:34 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:55:35 INFO opendrift.export.io_netcdf:121: Wrote 100 steps to file oil.nc
12:55:35 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:35 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:55:35 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:55:35 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:55:35 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:55:35 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:55:35 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:55:35 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:55:35 DEBUG opendrift.models.physics_methods:1061: min: 0.907749, mean: 6.352412, max: 8.540230
12:55:35 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:55:35 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.014421, dN_50: 0.001132
12:55:35 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:55:35 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.03604790679324428
12:55:35 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:35 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:55:35 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:55:35 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1009 surface elements
12:55:35 DEBUG opendrift.models.physics_methods:915: Advecting 1012 of 6000 elements above 0.100m with wind-sheared ocean current (0.094626 m/s - 0.273163 m/s)
12:55:35 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:55:35 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0012063472075475392 and 0.433497052307942 m/s
12:55:35 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:55:35 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:55:35 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:35 INFO opendrift.models.basemodel:2038: 2024-11-10 16:51:18.915878 - step 101 of 120 - 6000 active elements (0 deactivated)
12:55:35 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:35 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:35 DEBUG opendrift.models.basemodel:2057: 34.39044019674127 <- latitude -> 35.10128693104025
12:55:35 DEBUG opendrift.models.basemodel:2062: 22.01539384136946 <- longitude -> 23.493727726479868
12:55:35 DEBUG opendrift.models.basemodel:2067: -64.09463378882813 <- z -> 0.0
12:55:35 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:35 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:35 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:35 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:35 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:35 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:35 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:35 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:55:35 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:35 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:35 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:35 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:35 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:35 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:35 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:35 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:35 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:35 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:55:35 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:35 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:35 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:35 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:35 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:35 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:55:35 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:35 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:55:35 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:35 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:35 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:55:35 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:55:35 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-10 15:00:00 (before)
2024-11-10 18:00:00 (after)
12:55:35 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-10 15:00:00) in space (linearNDFast)
12:55:35 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:35 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-10 18:00:00) in space (linearNDFast)
12:55:35 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:35 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-10 15:00:00, weight 0.38) and
after (2024-11-10 18:00:00, weight 0.62) in time
12:55:35 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:35 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:35 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:35 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:55:35 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:35 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:55:35 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:35 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:35 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:55:35 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:55:35 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-10 15:00:00 (before)
2024-11-10 18:00:00 (after)
12:55:35 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-10 15:00:00) in space (linearNDFast)
12:55:35 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:35 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-10 18:00:00) in space (linearNDFast)
12:55:35 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:35 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-10 15:00:00, weight 0.38) and
after (2024-11-10 18:00:00, weight 0.62) in time
12:55:35 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:35 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:35 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:35 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:35 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:55:35 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:55:35 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:55:35 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:55:35 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:35 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.853288 (min) -0.0217745 (max)
12:55:35 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.3337 (min) 0.55505 (max)
12:55:35 DEBUG opendrift.models.basemodel.environment:905: x_wind: -7.11801 (min) -1.36062 (max)
12:55:35 DEBUG opendrift.models.basemodel.environment:905: y_wind: -7.37506 (min) -1.09747 (max)
12:55:35 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:55:35 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:55:35 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:55:35 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:55:35 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:55:35 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:55:35 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:55:35 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:55:35 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:55:35 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:55:35 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:55:35 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:55:35 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:55:35 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:55:35 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:35 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:55:35 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:35 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.096196, mean: 1.410182, max: 2.353004
12:55:35 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:55:35 DEBUG opendrift.models.physics_methods:1061: min: 1.689691, mean: 6.209508, max: 8.356818
12:55:35 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.689691, mean: 6.209508, max: 8.356818
12:55:35 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:55:35 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:35 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:55:35 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:55:35 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:55:35 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:55:35 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:55:35 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:55:35 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:55:35 DEBUG opendrift.models.physics_methods:1061: min: 1.689691, mean: 6.209508, max: 8.356818
12:55:35 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:55:35 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.015330, dN_50: 0.001203
12:55:35 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:55:35 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.034516362099959876
12:55:35 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:35 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:55:35 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:55:35 DEBUG opendrift.models.physics_methods:915: Advecting 1017 of 6000 elements above 0.100m with wind-sheared ocean current (0.003564 m/s - 0.277574 m/s)
12:55:35 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:55:35 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0013918111845223683 and 0.44933950370336645 m/s
12:55:35 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:55:35 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:55:35 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:35 INFO opendrift.models.basemodel:2038: 2024-11-10 17:51:18.915878 - step 102 of 120 - 6000 active elements (0 deactivated)
12:55:35 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:35 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:35 DEBUG opendrift.models.basemodel:2057: 34.38448696715361 <- latitude -> 35.10017982803408
12:55:35 DEBUG opendrift.models.basemodel:2062: 21.989611635324437 <- longitude -> 23.49677728857149
12:55:35 DEBUG opendrift.models.basemodel:2067: -63.410735114234384 <- z -> 0.0
12:55:35 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:35 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:35 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:35 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:35 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:35 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:35 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:35 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:55:35 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:35 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:35 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:35 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:35 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:35 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:35 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:35 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:35 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:35 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:55:35 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:35 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:35 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:35 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:35 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:35 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:55:35 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:35 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:55:35 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:35 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:35 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:55:35 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:55:35 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-10 15:00:00 (before)
2024-11-10 18:00:00 (after)
12:55:35 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-10 15:00:00) in space (linearNDFast)
12:55:35 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:35 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-10 18:00:00) in space (linearNDFast)
12:55:35 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:36 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-10 15:00:00, weight 0.05) and
after (2024-11-10 18:00:00, weight 0.95) in time
12:55:36 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:36 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:36 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:36 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:55:36 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:36 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:55:36 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:36 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:36 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:55:36 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:55:36 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-10 15:00:00 (before)
2024-11-10 18:00:00 (after)
12:55:36 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-10 15:00:00) in space (linearNDFast)
12:55:36 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:36 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-10 18:00:00) in space (linearNDFast)
12:55:36 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:36 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-10 15:00:00, weight 0.05) and
after (2024-11-10 18:00:00, weight 0.95) in time
12:55:36 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:36 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:36 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:36 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:36 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:55:36 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:55:36 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:55:36 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:55:36 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:36 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.848604 (min) -0.0211987 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.319535 (min) 0.578712 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:905: x_wind: -7.27904 (min) -0.959287 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:905: y_wind: -6.83021 (min) -1.68844 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:55:36 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:36 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.103753, mean: 1.369081, max: 2.290301
12:55:36 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:55:36 DEBUG opendrift.models.physics_methods:1061: min: 1.754807, mean: 6.064559, max: 8.244719
12:55:36 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.754807, mean: 6.064559, max: 8.244719
12:55:36 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:55:36 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:36 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:55:36 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:55:36 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:55:36 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:55:36 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:55:36 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:55:36 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:55:36 DEBUG opendrift.models.physics_methods:1061: min: 1.754807, mean: 6.064559, max: 8.244720
12:55:36 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:55:36 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.016445, dN_50: 0.001291
12:55:36 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:55:36 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.033596680802423964
12:55:36 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:36 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:55:36 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:55:36 DEBUG opendrift.models.physics_methods:915: Advecting 1029 of 6000 elements above 0.100m with wind-sheared ocean current (0.009439 m/s - 0.274968 m/s)
12:55:36 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:55:36 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.001498546767512832 and 0.5128868375072021 m/s
12:55:36 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:55:36 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:55:36 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:36 INFO opendrift.models.basemodel:2038: 2024-11-10 18:51:18.915878 - step 103 of 120 - 6000 active elements (0 deactivated)
12:55:36 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:36 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:36 DEBUG opendrift.models.basemodel:2057: 34.373880182529824 <- latitude -> 35.100960337898485
12:55:36 DEBUG opendrift.models.basemodel:2062: 21.968410640122258 <- longitude -> 23.493742210615828
12:55:36 DEBUG opendrift.models.basemodel:2067: -62.38355567017288 <- z -> 0.0
12:55:36 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:36 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:36 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:36 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:36 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:36 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:36 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:36 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:55:36 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:36 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:36 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:36 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:36 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:36 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:36 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:36 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:36 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:36 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:55:36 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:36 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:36 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:36 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:36 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:36 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:55:36 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:36 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:55:36 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:36 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:36 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:55:36 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:55:36 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-10 18:00:00 (before)
2024-11-10 21:00:00 (after)
12:55:36 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
12:55:36 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
12:55:36 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:55:36 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:55:36 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:55:36 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 27x26x18) for time after (2024-11-10 21:00:00)
12:55:36 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-10 18:00:00) in space (linearNDFast)
12:55:36 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:36 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-10 21:00:00) in space (linearNDFast)
12:55:36 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:36 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-10 18:00:00, weight 0.71) and
after (2024-11-10 21:00:00, weight 0.29) in time
12:55:36 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:36 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:36 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:36 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:55:36 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:36 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:55:36 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:36 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:36 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:55:36 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:55:36 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-10 18:00:00 (before)
2024-11-10 21:00:00 (after)
12:55:36 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_wind to retrieve x_wind
12:55:39 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_wind to retrieve y_wind
12:55:42 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:55:42 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:55:42 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:55:42 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 720x361x1) for time after (2024-11-10 21:00:00)
12:55:42 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-10 18:00:00) in space (linearNDFast)
12:55:42 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:42 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-10 21:00:00) in space (linearNDFast)
12:55:42 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:42 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-10 18:00:00, weight 0.71) and
after (2024-11-10 21:00:00, weight 0.29) in time
12:55:42 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:42 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:42 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:42 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:42 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:55:42 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:55:42 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:55:42 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:55:42 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:42 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.849585 (min) -0.027192 (max)
12:55:42 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.306907 (min) 0.525616 (max)
12:55:42 DEBUG opendrift.models.basemodel.environment:905: x_wind: -7.366 (min) -1.30495 (max)
12:55:42 DEBUG opendrift.models.basemodel.environment:905: y_wind: -5.95861 (min) -1.15172 (max)
12:55:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:55:42 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:55:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:55:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:55:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:55:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:55:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:55:42 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:55:42 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:55:42 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:55:42 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:55:42 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:55:42 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:55:42 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:55:42 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:42 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:55:42 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:42 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.082243, mean: 1.203550, max: 2.193215
12:55:42 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:55:42 DEBUG opendrift.models.physics_methods:1061: min: 1.562349, mean: 5.682874, max: 8.068080
12:55:42 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.562349, mean: 5.682874, max: 8.068080
12:55:42 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:55:42 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:42 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:55:42 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:55:42 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:55:42 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:55:42 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:55:42 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:55:42 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:55:42 DEBUG opendrift.models.physics_methods:1061: min: 1.562349, mean: 5.682874, max: 8.068080
12:55:42 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:55:42 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.017659, dN_50: 0.001386
12:55:42 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:55:42 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.032172695565183394
12:55:42 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:42 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:55:42 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:55:42 DEBUG opendrift.models.physics_methods:915: Advecting 1033 of 6000 elements above 0.100m with wind-sheared ocean current (0.008580 m/s - 0.262466 m/s)
12:55:42 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:55:42 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.002504074671380457 and 0.45843017945001463 m/s
12:55:42 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:55:42 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:55:42 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:42 INFO opendrift.models.basemodel:2038: 2024-11-10 19:51:18.915878 - step 104 of 120 - 6000 active elements (0 deactivated)
12:55:42 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:42 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:42 DEBUG opendrift.models.basemodel:2057: 34.36876358549762 <- latitude -> 35.095936232148915
12:55:42 DEBUG opendrift.models.basemodel:2062: 21.942108088553788 <- longitude -> 23.48749706027115
12:55:42 DEBUG opendrift.models.basemodel:2067: -61.28581424132391 <- z -> 0.0
12:55:42 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:42 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:42 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:42 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:42 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:42 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:42 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:42 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:55:42 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:42 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:42 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:42 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:42 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:42 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:42 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:42 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:42 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:42 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:55:42 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:42 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:42 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:42 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:42 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:42 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:55:42 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:42 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:55:42 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:43 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:43 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:55:43 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:55:43 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-10 18:00:00 (before)
2024-11-10 21:00:00 (after)
12:55:43 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-10 18:00:00) in space (linearNDFast)
12:55:43 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:43 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-10 21:00:00) in space (linearNDFast)
12:55:43 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:43 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-10 18:00:00, weight 0.38) and
after (2024-11-10 21:00:00, weight 0.62) in time
12:55:43 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:43 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:43 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:43 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:55:43 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:43 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:55:43 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:43 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:43 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:55:43 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:55:43 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-10 18:00:00 (before)
2024-11-10 21:00:00 (after)
12:55:43 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-10 18:00:00) in space (linearNDFast)
12:55:43 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:43 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-10 21:00:00) in space (linearNDFast)
12:55:43 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:43 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-10 18:00:00, weight 0.38) and
after (2024-11-10 21:00:00, weight 0.62) in time
12:55:43 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:43 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:43 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:43 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:43 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:55:43 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:55:43 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:55:43 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:55:43 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:43 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.79736 (min) -0.0446211 (max)
12:55:43 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.260479 (min) 0.518676 (max)
12:55:43 DEBUG opendrift.models.basemodel.environment:905: x_wind: -7.57379 (min) -1.80842 (max)
12:55:43 DEBUG opendrift.models.basemodel.environment:905: y_wind: -5.61468 (min) -0.674321 (max)
12:55:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:55:43 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:55:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:55:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:55:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:55:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:55:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:55:43 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:55:43 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:55:43 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:55:43 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:55:43 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:55:43 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:55:43 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:55:43 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:43 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:55:43 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:43 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.099804, mean: 1.048971, max: 2.176264
12:55:43 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:55:43 DEBUG opendrift.models.physics_methods:1061: min: 1.721094, mean: 5.312751, max: 8.036841
12:55:43 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.721094, mean: 5.312751, max: 8.036841
12:55:43 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:55:43 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:43 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:55:43 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:55:43 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:55:43 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:55:43 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:55:43 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:55:43 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:55:43 DEBUG opendrift.models.physics_methods:1061: min: 1.721094, mean: 5.312751, max: 8.036841
12:55:43 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:55:43 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.018821, dN_50: 0.001477
12:55:43 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:55:43 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.031924069210122065
12:55:43 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:43 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:55:43 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:55:43 DEBUG opendrift.models.physics_methods:915: Advecting 1037 of 6000 elements above 0.100m with wind-sheared ocean current (0.029983 m/s - 0.262941 m/s)
12:55:43 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:55:43 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0009558359597159394 and 0.5089750825802459 m/s
12:55:43 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:55:43 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:55:43 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:43 INFO opendrift.models.basemodel:2038: 2024-11-10 20:51:18.915878 - step 105 of 120 - 6000 active elements (0 deactivated)
12:55:43 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:43 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:43 DEBUG opendrift.models.basemodel:2057: 34.36518374309291 <- latitude -> 35.092932565802585
12:55:43 DEBUG opendrift.models.basemodel:2062: 21.92647115146092 <- longitude -> 23.486566962454376
12:55:43 DEBUG opendrift.models.basemodel:2067: -60.13735243527756 <- z -> 0.0
12:55:43 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:43 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:43 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:43 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:43 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:43 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:43 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:43 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:55:43 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:43 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:43 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:43 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:43 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:43 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:43 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:43 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:43 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:43 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:55:43 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:43 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:43 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:43 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:43 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:43 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:55:43 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:43 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:55:43 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:43 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:43 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:55:43 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:55:43 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-10 18:00:00 (before)
2024-11-10 21:00:00 (after)
12:55:43 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-10 18:00:00) in space (linearNDFast)
12:55:43 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:43 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-10 21:00:00) in space (linearNDFast)
12:55:43 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:43 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-10 18:00:00, weight 0.05) and
after (2024-11-10 21:00:00, weight 0.95) in time
12:55:43 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:43 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:43 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:43 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:55:43 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:43 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:55:43 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:43 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:43 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:55:43 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:55:43 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-10 18:00:00 (before)
2024-11-10 21:00:00 (after)
12:55:43 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-10 18:00:00) in space (linearNDFast)
12:55:43 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:43 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-10 21:00:00) in space (linearNDFast)
12:55:43 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:43 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-10 18:00:00, weight 0.05) and
after (2024-11-10 21:00:00, weight 0.95) in time
12:55:43 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:43 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:43 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:43 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:43 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:55:43 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:55:43 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:55:43 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:55:43 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:43 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.840204 (min) -0.0241702 (max)
12:55:43 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.24222 (min) 0.529759 (max)
12:55:43 DEBUG opendrift.models.basemodel.environment:905: x_wind: -7.91406 (min) -2.18493 (max)
12:55:43 DEBUG opendrift.models.basemodel.environment:905: y_wind: -5.385 (min) -0.151359 (max)
12:55:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:55:43 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:55:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:55:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:55:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:55:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:55:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:55:43 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:55:43 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:55:43 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:55:43 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:55:43 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:55:43 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:55:43 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:55:43 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:43 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:55:43 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:43 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.139365, mean: 0.928814, max: 2.244365
12:55:43 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:55:43 DEBUG opendrift.models.physics_methods:1061: min: 2.033790, mean: 5.003381, max: 8.161620
12:55:43 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.033790, mean: 5.003381, max: 8.161620
12:55:43 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:55:43 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:43 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:55:43 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:55:43 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:55:43 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:55:43 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:55:43 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:55:43 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:55:43 DEBUG opendrift.models.physics_methods:1061: min: 2.033790, mean: 5.003381, max: 8.161620
12:55:43 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:55:43 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.019929, dN_50: 0.001564
12:55:43 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:55:43 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.03292292876463426
12:55:43 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:43 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:55:43 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:55:43 DEBUG opendrift.models.physics_methods:915: Advecting 1041 of 6000 elements above 0.100m with wind-sheared ocean current (0.053912 m/s - 0.265616 m/s)
12:55:43 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:55:43 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.00240026956778681 and 0.4808215351219167 m/s
12:55:43 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:55:43 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:55:43 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:43 INFO opendrift.models.basemodel:2038: 2024-11-10 21:51:18.915878 - step 106 of 120 - 6000 active elements (0 deactivated)
12:55:43 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:43 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:43 DEBUG opendrift.models.basemodel:2057: 34.35903805889601 <- latitude -> 35.09589914567513
12:55:43 DEBUG opendrift.models.basemodel:2062: 21.907911559263074 <- longitude -> 23.479411564770356
12:55:43 DEBUG opendrift.models.basemodel:2067: -58.99485312565706 <- z -> 0.0
12:55:43 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:43 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:43 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:43 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:43 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:43 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:43 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:43 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:55:43 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:43 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:43 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:43 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:43 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:43 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:43 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:43 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:43 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:43 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:55:43 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:43 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:43 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:43 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:43 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:43 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:55:43 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:43 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:55:43 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:43 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:43 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:55:43 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:55:43 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-10 21:00:00 (before)
2024-11-11 00:00:00 (after)
12:55:43 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
12:55:43 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
12:55:43 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:55:43 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:55:43 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:55:43 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 28x27x17) for time after (2024-11-11 00:00:00)
12:55:43 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-10 21:00:00) in space (linearNDFast)
12:55:43 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:43 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-11 00:00:00) in space (linearNDFast)
12:55:43 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:44 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-10 21:00:00, weight 0.71) and
after (2024-11-11 00:00:00, weight 0.29) in time
12:55:44 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:44 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:44 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:44 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:55:44 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:44 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:55:44 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:44 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:44 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:55:44 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:55:44 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-10 21:00:00 (before)
2024-11-11 00:00:00 (after)
12:55:44 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_wind to retrieve x_wind
12:55:46 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_wind to retrieve y_wind
12:55:49 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:55:49 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:55:49 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:55:49 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 720x361x1) for time after (2024-11-11 00:00:00)
12:55:49 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-10 21:00:00) in space (linearNDFast)
12:55:49 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:49 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-11 00:00:00) in space (linearNDFast)
12:55:49 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:49 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-10 21:00:00, weight 0.71) and
after (2024-11-11 00:00:00, weight 0.29) in time
12:55:49 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:49 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:49 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:49 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:49 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:55:49 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:55:49 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:55:49 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:55:49 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:49 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.850233 (min) -0.0120445 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.246355 (min) 0.510552 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: x_wind: -8.19785 (min) -2.40489 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: y_wind: -4.60372 (min) 0.0665671 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:49 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.163015, mean: 0.810552, max: 1.969097
12:55:49 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:55:49 DEBUG opendrift.models.physics_methods:1061: min: 2.199600, mean: 4.683445, max: 7.644747
12:55:49 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.199600, mean: 4.683445, max: 7.644747
12:55:49 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:55:49 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:49 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:55:49 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:55:49 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:55:49 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:55:49 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:55:49 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:55:49 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:55:49 DEBUG opendrift.models.physics_methods:1061: min: 2.199600, mean: 4.683445, max: 7.644747
12:55:49 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:55:49 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.021194, dN_50: 0.001663
12:55:49 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:55:49 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.02888548813477289
12:55:49 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:49 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:55:49 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:55:49 DEBUG opendrift.models.physics_methods:915: Advecting 1046 of 6000 elements above 0.100m with wind-sheared ocean current (0.031370 m/s - 0.244041 m/s)
12:55:49 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:55:49 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0009428015987188532 and 0.5026022427268693 m/s
12:55:49 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:55:49 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:55:49 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:49 INFO opendrift.models.basemodel:2038: 2024-11-10 22:51:18.915878 - step 107 of 120 - 6000 active elements (0 deactivated)
12:55:49 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:49 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:49 DEBUG opendrift.models.basemodel:2057: 34.35508749584171 <- latitude -> 35.09239476778522
12:55:49 DEBUG opendrift.models.basemodel:2062: 21.887408475406136 <- longitude -> 23.471399204198658
12:55:49 DEBUG opendrift.models.basemodel:2067: -58.14613057053764 <- z -> 0.0
12:55:49 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:49 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:49 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:49 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:49 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:49 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:49 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:49 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:55:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:49 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:49 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:49 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:49 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:49 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:49 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:49 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:49 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:49 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:55:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:49 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:49 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:49 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:49 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:49 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:55:49 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:49 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:55:49 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:49 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:49 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:55:49 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:55:49 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-10 21:00:00 (before)
2024-11-11 00:00:00 (after)
12:55:49 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-10 21:00:00) in space (linearNDFast)
12:55:49 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:49 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-11 00:00:00) in space (linearNDFast)
12:55:49 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:49 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-10 21:00:00, weight 0.38) and
after (2024-11-11 00:00:00, weight 0.62) in time
12:55:49 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:49 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:49 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:49 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:55:49 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:49 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:55:49 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:49 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:49 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:55:49 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:55:49 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-10 21:00:00 (before)
2024-11-11 00:00:00 (after)
12:55:49 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-10 21:00:00) in space (linearNDFast)
12:55:49 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:49 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-11 00:00:00) in space (linearNDFast)
12:55:49 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:49 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-10 21:00:00, weight 0.38) and
after (2024-11-11 00:00:00, weight 0.62) in time
12:55:49 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:49 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:49 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:49 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:49 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:55:49 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:55:49 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:55:49 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:55:49 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:49 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.79514 (min) 0.0209827 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.24655 (min) 0.50628 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: x_wind: -8.50474 (min) -2.61054 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.74735 (min) 0.318139 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:49 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.168003, mean: 0.703268, max: 1.978567
12:55:49 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:55:49 DEBUG opendrift.models.physics_methods:1061: min: 2.232998, mean: 4.380931, max: 7.663108
12:55:49 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.232998, mean: 4.380931, max: 7.663108
12:55:49 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:55:49 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:49 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:55:49 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:55:49 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:55:49 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:55:49 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:55:49 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:55:49 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:55:49 DEBUG opendrift.models.physics_methods:1061: min: 2.232998, mean: 4.380931, max: 7.663108
12:55:49 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:55:49 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.022469, dN_50: 0.001763
12:55:49 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:55:49 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.02902438854350132
12:55:49 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:49 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:55:49 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:55:49 DEBUG opendrift.models.physics_methods:915: Advecting 1054 of 6000 elements above 0.100m with wind-sheared ocean current (0.006812 m/s - 0.238455 m/s)
12:55:49 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:55:49 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.002611107654565998 and 0.4361354029880985 m/s
12:55:49 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:55:49 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:55:49 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:49 INFO opendrift.models.basemodel:2038: 2024-11-10 23:51:18.915878 - step 108 of 120 - 6000 active elements (0 deactivated)
12:55:49 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:49 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:49 DEBUG opendrift.models.basemodel:2057: 34.35663364926973 <- latitude -> 35.09295267408331
12:55:49 DEBUG opendrift.models.basemodel:2062: 21.87015039763085 <- longitude -> 23.47286332562209
12:55:49 DEBUG opendrift.models.basemodel:2067: -57.05088297994489 <- z -> 0.0
12:55:49 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:49 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:49 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:49 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:49 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:49 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:49 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:49 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:55:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:49 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:49 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:49 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:49 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:49 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:49 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:49 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:49 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:49 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:55:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:49 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:49 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:49 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:49 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:49 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:55:49 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:49 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:55:49 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:49 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:49 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:55:49 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:55:49 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-10 21:00:00 (before)
2024-11-11 00:00:00 (after)
12:55:49 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-10 21:00:00) in space (linearNDFast)
12:55:49 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:49 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-11 00:00:00) in space (linearNDFast)
12:55:49 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:49 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-10 21:00:00, weight 0.05) and
after (2024-11-11 00:00:00, weight 0.95) in time
12:55:49 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:49 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:49 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:49 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:55:49 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:49 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:55:49 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:49 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:49 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:55:49 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:55:49 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-10 21:00:00 (before)
2024-11-11 00:00:00 (after)
12:55:49 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-10 21:00:00) in space (linearNDFast)
12:55:49 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:49 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-11 00:00:00) in space (linearNDFast)
12:55:49 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:49 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-10 21:00:00, weight 0.05) and
after (2024-11-11 00:00:00, weight 0.95) in time
12:55:49 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:49 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:49 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:49 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:49 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:55:49 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:55:49 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:55:49 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:55:49 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:49 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.830135 (min) 0.0716045 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.278414 (min) 0.487765 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: x_wind: -8.783 (min) -2.74303 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.3082 (min) 0.855867 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:55:49 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:49 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.190357, mean: 0.614130, max: 2.075041
12:55:49 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:55:49 DEBUG opendrift.models.physics_methods:1061: min: 2.376920, mean: 4.119391, max: 7.847710
12:55:49 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.376920, mean: 4.119391, max: 7.847710
12:55:49 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:55:49 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:49 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:55:49 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:55:49 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:55:49 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:55:49 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:55:49 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:55:49 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:55:49 DEBUG opendrift.models.physics_methods:1061: min: 2.376920, mean: 4.119391, max: 7.847710
12:55:49 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:55:49 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.023663, dN_50: 0.001857
12:55:49 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:55:49 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.030439404616713586
12:55:49 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:49 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:55:49 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:55:49 DEBUG opendrift.models.physics_methods:915: Advecting 1057 of 6000 elements above 0.100m with wind-sheared ocean current (0.028517 m/s - 0.238308 m/s)
12:55:49 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:55:49 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0008336612351209821 and 0.46024731152655496 m/s
12:55:49 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:55:49 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:55:49 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:49 INFO opendrift.models.basemodel:2038: 2024-11-11 00:51:18.915878 - step 109 of 120 - 6000 active elements (0 deactivated)
12:55:49 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:49 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:49 DEBUG opendrift.models.basemodel:2057: 34.357828454081115 <- latitude -> 35.09100375443476
12:55:49 DEBUG opendrift.models.basemodel:2062: 21.850911159267923 <- longitude -> 23.456236488480886
12:55:49 DEBUG opendrift.models.basemodel:2067: -56.10591857887429 <- z -> 0.0
12:55:49 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:49 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:49 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:49 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:49 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:49 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:49 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:49 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:55:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:49 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:49 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:49 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:49 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:49 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:49 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:49 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:49 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:49 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:55:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:49 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:49 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:49 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:49 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:49 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:55:49 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:49 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:55:49 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:49 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:49 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:55:49 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:55:49 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-11 00:00:00 (before)
2024-11-11 03:00:00 (after)
12:55:49 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
12:55:50 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
12:55:50 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:55:50 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:55:50 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:55:50 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 28x27x17) for time after (2024-11-11 03:00:00)
12:55:50 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-11 00:00:00) in space (linearNDFast)
12:55:50 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:50 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-11 03:00:00) in space (linearNDFast)
12:55:50 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:50 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-11 00:00:00, weight 0.71) and
after (2024-11-11 03:00:00, weight 0.29) in time
12:55:50 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:50 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:50 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:50 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:55:50 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:50 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:55:50 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:50 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:50 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:55:50 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:55:50 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-11 00:00:00 (before)
2024-11-11 03:00:00 (after)
12:55:50 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_wind to retrieve x_wind
12:55:53 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_wind to retrieve y_wind
12:55:56 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:55:56 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:55:56 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:55:56 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 720x361x1) for time after (2024-11-11 03:00:00)
12:55:56 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-11 00:00:00) in space (linearNDFast)
12:55:56 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:56 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-11 03:00:00) in space (linearNDFast)
12:55:56 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:56 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-11 00:00:00, weight 0.71) and
after (2024-11-11 03:00:00, weight 0.29) in time
12:55:56 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:56 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:56 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:56 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:56 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:55:56 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:55:56 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:55:56 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:55:56 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:56 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.801179 (min) 0.0980221 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.263967 (min) 0.45955 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: x_wind: -8.65731 (min) -2.92774 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.60257 (min) 1.5972 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:56 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.239197, mean: 0.591887, max: 1.947899
12:55:56 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:55:56 DEBUG opendrift.models.physics_methods:1061: min: 2.664450, mean: 4.080710, max: 7.603485
12:55:56 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.664450, mean: 4.080710, max: 7.603485
12:55:56 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:55:56 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:56 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:55:56 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:55:56 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:55:56 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:55:56 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:55:56 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:55:56 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:55:56 DEBUG opendrift.models.physics_methods:1061: min: 2.664450, mean: 4.080709, max: 7.603486
12:55:56 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:55:56 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.024034, dN_50: 0.001886
12:55:56 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:55:56 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.028574567691969383
12:55:56 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:56 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:55:56 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:55:56 DEBUG opendrift.models.physics_methods:915: Advecting 1056 of 6000 elements above 0.100m with wind-sheared ocean current (0.058139 m/s - 0.226943 m/s)
12:55:56 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:55:56 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0017831224550114652 and 0.4948315270808111 m/s
12:55:56 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:55:56 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:55:56 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:56 INFO opendrift.models.basemodel:2038: 2024-11-11 01:51:18.915878 - step 110 of 120 - 6000 active elements (0 deactivated)
12:55:56 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:56 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:56 DEBUG opendrift.models.basemodel:2057: 34.35941662762224 <- latitude -> 35.09305291813402
12:55:56 DEBUG opendrift.models.basemodel:2062: 21.828134684764652 <- longitude -> 23.4477131647754
12:55:56 DEBUG opendrift.models.basemodel:2067: -55.30053085895778 <- z -> 0.0
12:55:56 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:56 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:56 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:56 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:56 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:56 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:56 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:56 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:55:56 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:56 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:56 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:56 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:56 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:56 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:56 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:56 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:56 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:56 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:55:56 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:56 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:56 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:56 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:56 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:56 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:55:56 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:56 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:55:56 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:56 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:56 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:55:56 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:55:56 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-11 00:00:00 (before)
2024-11-11 03:00:00 (after)
12:55:56 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-11 00:00:00) in space (linearNDFast)
12:55:56 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:56 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-11 03:00:00) in space (linearNDFast)
12:55:56 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:56 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-11 00:00:00, weight 0.38) and
after (2024-11-11 03:00:00, weight 0.62) in time
12:55:56 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:56 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:56 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:56 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:55:56 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:56 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:55:56 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:56 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:56 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:55:56 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:55:56 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-11 00:00:00 (before)
2024-11-11 03:00:00 (after)
12:55:56 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-11 00:00:00) in space (linearNDFast)
12:55:56 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:56 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-11 03:00:00) in space (linearNDFast)
12:55:56 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:56 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-11 00:00:00, weight 0.38) and
after (2024-11-11 03:00:00, weight 0.62) in time
12:55:56 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:56 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:56 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:56 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:56 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:55:56 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:55:56 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:55:56 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:55:56 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:56 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.824801 (min) 0.117122 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.248742 (min) 0.411135 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: x_wind: -8.52341 (min) -2.93974 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.49735 (min) 2.32494 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:56 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.264885, mean: 0.580050, max: 1.834844
12:55:56 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:55:56 DEBUG opendrift.models.physics_methods:1061: min: 2.803875, mean: 4.075240, max: 7.379537
12:55:56 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.803875, mean: 4.075240, max: 7.379537
12:55:56 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:55:56 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:56 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:55:56 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:55:56 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:55:56 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:55:56 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:55:56 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:55:56 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:55:56 DEBUG opendrift.models.physics_methods:1061: min: 2.803875, mean: 4.075240, max: 7.379537
12:55:56 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:55:56 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.024252, dN_50: 0.001903
12:55:56 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:55:56 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.026916359998896116
12:55:56 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:56 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:55:56 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:55:56 DEBUG opendrift.models.physics_methods:915: Advecting 1058 of 6000 elements above 0.100m with wind-sheared ocean current (0.032225 m/s - 0.215024 m/s)
12:55:56 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:55:56 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0016578149580066574 and 0.4569344530012448 m/s
12:55:56 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:55:56 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:55:56 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:56 INFO opendrift.models.basemodel:2038: 2024-11-11 02:51:18.915878 - step 111 of 120 - 6000 active elements (0 deactivated)
12:55:56 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:56 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:56 DEBUG opendrift.models.basemodel:2057: 34.35895592344188 <- latitude -> 35.105944049761064
12:55:56 DEBUG opendrift.models.basemodel:2062: 21.799266071384196 <- longitude -> 23.444134939458397
12:55:56 DEBUG opendrift.models.basemodel:2067: -54.56584408976128 <- z -> 0.0
12:55:56 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:56 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:56 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:56 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:56 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:56 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:56 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:56 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:55:56 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:56 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:56 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:56 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:56 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:56 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:56 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:56 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:56 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:56 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:55:56 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:56 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:56 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:56 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:56 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:56 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:55:56 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:56 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:55:56 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:56 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:56 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:55:56 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:55:56 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-11 00:00:00 (before)
2024-11-11 03:00:00 (after)
12:55:56 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-11 00:00:00) in space (linearNDFast)
12:55:56 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:56 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-11 03:00:00) in space (linearNDFast)
12:55:56 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:56 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-11 00:00:00, weight 0.05) and
after (2024-11-11 03:00:00, weight 0.95) in time
12:55:56 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:56 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:56 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:56 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:55:56 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:56 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:55:56 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:56 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:56 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:55:56 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:55:56 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-11 00:00:00 (before)
2024-11-11 03:00:00 (after)
12:55:56 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-11 00:00:00) in space (linearNDFast)
12:55:56 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:56 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-11 03:00:00) in space (linearNDFast)
12:55:56 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:56 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-11 00:00:00, weight 0.05) and
after (2024-11-11 03:00:00, weight 0.95) in time
12:55:56 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:56 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:56 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:55:56 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:55:56 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:55:56 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:55:56 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:55:56 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:55:56 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:55:56 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.861028 (min) 0.139147 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.266747 (min) 0.345671 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: x_wind: -8.43669 (min) -2.8938 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.60016 (min) 3.06799 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:55:56 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:55:56 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.289654, mean: 0.570781, max: 1.766468
12:55:56 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:55:56 DEBUG opendrift.models.physics_methods:1061: min: 2.932037, mean: 4.071914, max: 7.240732
12:55:56 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.932037, mean: 4.071914, max: 7.240732
12:55:56 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:55:56 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:55:56 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:55:56 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:55:56 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:55:56 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:55:56 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:55:56 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:55:56 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:55:56 DEBUG opendrift.models.physics_methods:1061: min: 2.932037, mean: 4.071914, max: 7.240732
12:55:56 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:55:56 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.024452, dN_50: 0.001919
12:55:56 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:55:56 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.025913474192292597
12:55:56 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:55:56 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:55:56 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:55:56 DEBUG opendrift.models.physics_methods:915: Advecting 1064 of 6000 elements above 0.100m with wind-sheared ocean current (0.004048 m/s - 0.214824 m/s)
12:55:56 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:55:56 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0031532199145495065 and 0.4342010919402262 m/s
12:55:56 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:55:56 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:55:56 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:55:56 INFO opendrift.models.basemodel:2038: 2024-11-11 03:51:18.915878 - step 112 of 120 - 6000 active elements (0 deactivated)
12:55:56 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:55:56 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:55:56 DEBUG opendrift.models.basemodel:2057: 34.36265306829391 <- latitude -> 35.1137711244975
12:55:56 DEBUG opendrift.models.basemodel:2062: 21.778448288273893 <- longitude -> 23.43847156642471
12:55:56 DEBUG opendrift.models.basemodel:2067: -53.520052599365115 <- z -> 0.0
12:55:56 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:55:56 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:56 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:55:56 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:56 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:55:56 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:56 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:56 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:55:56 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:56 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:56 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:56 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:56 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:55:56 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:56 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:55:56 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:56 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:56 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:55:56 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:55:56 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:55:56 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:56 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:56 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:56 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:55:56 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:56 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:55:56 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:56 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:56 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:55:56 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:55:56 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-11 03:00:00 (before)
2024-11-11 06:00:00 (after)
12:55:56 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
12:55:57 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
12:55:57 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:55:57 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:55:57 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:55:57 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 29x26x17) for time after (2024-11-11 06:00:00)
12:55:57 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-11 03:00:00) in space (linearNDFast)
12:55:57 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:57 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-11 06:00:00) in space (linearNDFast)
12:55:57 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:55:57 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-11 03:00:00, weight 0.71) and
after (2024-11-11 06:00:00, weight 0.29) in time
12:55:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:55:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:55:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:55:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:55:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:55:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:55:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:55:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:55:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:55:57 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:55:57 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-11 03:00:00 (before)
2024-11-11 06:00:00 (after)
12:55:57 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_wind to retrieve x_wind
12:56:00 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_wind to retrieve y_wind
12:56:03 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:56:03 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:56:03 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:56:03 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 720x361x1) for time after (2024-11-11 06:00:00)
12:56:03 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-11 03:00:00) in space (linearNDFast)
12:56:03 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:56:03 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-11 06:00:00) in space (linearNDFast)
12:56:03 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:56:03 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-11 03:00:00, weight 0.71) and
after (2024-11-11 06:00:00, weight 0.29) in time
12:56:03 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:56:03 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:56:03 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:56:03 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:56:03 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:56:03 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:56:03 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:56:03 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:56:03 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:56:03 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.854314 (min) 0.128006 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.266816 (min) 0.335133 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: x_wind: -8.16186 (min) -2.7279 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: y_wind: -1.85875 (min) 3.2058 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:56:03 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.317715, mean: 0.574554, max: 1.641531
12:56:03 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:56:03 DEBUG opendrift.models.physics_methods:1061: min: 3.070776, mean: 4.098193, max: 6.979979
12:56:03 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.070776, mean: 4.098193, max: 6.979979
12:56:03 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:56:03 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:56:03 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:56:03 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:56:03 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:56:03 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:56:03 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:56:03 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:56:03 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:56:03 DEBUG opendrift.models.physics_methods:1061: min: 3.070776, mean: 4.098193, max: 6.979980
12:56:03 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:56:03 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.024587, dN_50: 0.001930
12:56:03 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:56:03 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.0240809935972928
12:56:03 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:56:03 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:56:03 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:56:03 DEBUG opendrift.models.physics_methods:915: Advecting 1070 of 6000 elements above 0.100m with wind-sheared ocean current (0.016786 m/s - 0.206487 m/s)
12:56:03 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:56:03 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.002455117743429579 and 0.4157903382508061 m/s
12:56:03 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:56:03 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:56:03 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:56:03 INFO opendrift.models.basemodel:2038: 2024-11-11 04:51:18.915878 - step 113 of 120 - 6000 active elements (0 deactivated)
12:56:03 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:56:03 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:56:03 DEBUG opendrift.models.basemodel:2057: 34.35952533107222 <- latitude -> 35.118865054269854
12:56:03 DEBUG opendrift.models.basemodel:2062: 21.75727593583328 <- longitude -> 23.435011856880195
12:56:03 DEBUG opendrift.models.basemodel:2067: -52.915543699283496 <- z -> 0.0
12:56:03 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:56:03 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:56:03 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:56:03 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:56:03 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:56:03 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:56:03 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:56:03 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:56:03 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:56:03 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:56:03 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:56:03 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:56:03 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:56:03 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:56:03 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:56:03 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:56:03 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:56:03 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:56:03 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:56:03 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:56:03 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:56:03 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:56:03 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:56:03 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:56:03 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:56:03 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:56:03 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:56:03 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:56:03 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:56:03 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:56:03 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-11 03:00:00 (before)
2024-11-11 06:00:00 (after)
12:56:03 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-11 03:00:00) in space (linearNDFast)
12:56:03 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:56:03 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-11 06:00:00) in space (linearNDFast)
12:56:03 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:56:03 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-11 03:00:00, weight 0.38) and
after (2024-11-11 06:00:00, weight 0.62) in time
12:56:03 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:56:03 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:56:03 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:56:03 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:56:03 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:56:03 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:56:03 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:56:03 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:56:03 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:56:03 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:56:03 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-11 03:00:00 (before)
2024-11-11 06:00:00 (after)
12:56:03 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-11 03:00:00) in space (linearNDFast)
12:56:03 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:56:03 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-11 06:00:00) in space (linearNDFast)
12:56:03 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:56:03 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-11 03:00:00, weight 0.38) and
after (2024-11-11 06:00:00, weight 0.62) in time
12:56:03 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:56:03 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:56:03 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:56:03 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:56:03 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:56:03 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:56:03 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:56:03 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:56:03 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:56:03 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.774026 (min) 0.109655 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.274418 (min) 0.320659 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: x_wind: -7.83762 (min) -2.5251 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: y_wind: -1.01143 (min) 3.25641 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:56:03 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.356098, mean: 0.593721, max: 1.511466
12:56:03 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:56:03 DEBUG opendrift.models.physics_methods:1061: min: 3.250981, mean: 4.175600, max: 6.697747
12:56:03 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.250981, mean: 4.175600, max: 6.697747
12:56:03 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:56:03 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:56:03 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:56:03 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:56:03 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:56:03 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:56:03 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:56:03 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:56:03 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:56:03 DEBUG opendrift.models.physics_methods:1061: min: 3.250981, mean: 4.175600, max: 6.697747
12:56:03 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:56:03 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.024577, dN_50: 0.001929
12:56:03 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:56:03 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.022173286959497433
12:56:03 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:56:03 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:56:03 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:56:03 DEBUG opendrift.models.physics_methods:915: Advecting 1075 of 6000 elements above 0.100m with wind-sheared ocean current (0.006266 m/s - 0.198468 m/s)
12:56:03 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:56:03 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0022497978002803663 and 0.4435915815444184 m/s
12:56:03 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:56:03 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:56:03 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:56:03 INFO opendrift.models.basemodel:2038: 2024-11-11 05:51:18.915878 - step 114 of 120 - 6000 active elements (0 deactivated)
12:56:03 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:56:03 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:56:03 DEBUG opendrift.models.basemodel:2057: 34.35850290727684 <- latitude -> 35.129815157863376
12:56:03 DEBUG opendrift.models.basemodel:2062: 21.72967235780646 <- longitude -> 23.428059812000495
12:56:03 DEBUG opendrift.models.basemodel:2067: -51.907982948629474 <- z -> 0.0
12:56:03 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:56:03 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:56:03 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:56:03 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:56:03 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:56:03 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:56:03 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:56:03 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:56:03 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:56:03 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:56:03 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:56:03 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:56:03 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:56:03 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:56:03 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:56:03 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:56:03 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:56:03 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:56:03 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:56:03 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:56:03 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:56:03 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:56:03 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:56:03 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:56:03 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:56:03 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:56:03 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:56:03 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:56:03 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:56:03 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:56:03 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-11 03:00:00 (before)
2024-11-11 06:00:00 (after)
12:56:03 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-11 03:00:00) in space (linearNDFast)
12:56:03 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:56:03 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-11 06:00:00) in space (linearNDFast)
12:56:03 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:56:03 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-11 03:00:00, weight 0.05) and
after (2024-11-11 06:00:00, weight 0.95) in time
12:56:03 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:56:03 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:56:03 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:56:03 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:56:03 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:56:03 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:56:03 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:56:03 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:56:03 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:56:03 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:56:03 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-11 03:00:00 (before)
2024-11-11 06:00:00 (after)
12:56:03 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-11 03:00:00) in space (linearNDFast)
12:56:03 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:56:03 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-11 06:00:00) in space (linearNDFast)
12:56:03 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:56:03 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-11 03:00:00, weight 0.05) and
after (2024-11-11 06:00:00, weight 0.95) in time
12:56:03 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:56:03 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:56:03 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:56:03 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:56:03 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:56:03 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:56:03 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:56:03 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:56:03 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:56:03 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.824907 (min) 0.103228 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.276448 (min) 0.30541 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: x_wind: -7.53807 (min) -2.33168 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: y_wind: -0.722002 (min) 3.60742 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:56:03 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:56:03 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.415539, mean: 0.625991, max: 1.406780
12:56:03 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:56:03 DEBUG opendrift.models.physics_methods:1061: min: 3.511848, mean: 4.296440, max: 6.461639
12:56:03 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.511848, mean: 4.296440, max: 6.461639
12:56:03 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:56:03 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:56:03 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:56:03 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:56:03 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:56:03 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:56:03 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:56:03 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:56:03 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:56:03 DEBUG opendrift.models.physics_methods:1061: min: 3.511847, mean: 4.296440, max: 6.461638
12:56:03 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:56:03 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.024456, dN_50: 0.001919
12:56:03 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:56:03 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.020637830596602416
12:56:03 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:56:03 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:56:03 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:56:03 DEBUG opendrift.models.physics_methods:915: Advecting 1083 of 6000 elements above 0.100m with wind-sheared ocean current (0.039856 m/s - 0.189592 m/s)
12:56:03 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:56:03 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0009896160985744524 and 0.4257805741877193 m/s
12:56:04 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:56:04 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:56:04 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:56:04 INFO opendrift.models.basemodel:2038: 2024-11-11 06:51:18.915878 - step 115 of 120 - 6000 active elements (0 deactivated)
12:56:04 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:56:04 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:56:04 DEBUG opendrift.models.basemodel:2057: 34.360285820677305 <- latitude -> 35.143344261070766
12:56:04 DEBUG opendrift.models.basemodel:2062: 21.707881213889202 <- longitude -> 23.425413617102638
12:56:04 DEBUG opendrift.models.basemodel:2067: -50.901780205965565 <- z -> 0.0
12:56:04 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:56:04 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:56:04 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:56:04 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:56:04 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:56:04 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:56:04 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:56:04 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:56:04 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:56:04 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:56:04 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:56:04 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:56:04 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:56:04 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:56:04 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:56:04 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:56:04 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:56:04 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:56:04 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:56:04 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:56:04 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:56:04 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:56:04 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:56:04 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:56:04 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:56:04 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:56:04 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:56:04 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:56:04 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:56:04 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:56:04 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-11 06:00:00 (before)
2024-11-11 09:00:00 (after)
12:56:04 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
12:56:04 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
12:56:04 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:56:04 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:56:04 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:56:04 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 29x28x17) for time after (2024-11-11 09:00:00)
12:56:04 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-11 06:00:00) in space (linearNDFast)
12:56:04 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:56:04 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-11 09:00:00) in space (linearNDFast)
12:56:04 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:56:04 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-11 06:00:00, weight 0.71) and
after (2024-11-11 09:00:00, weight 0.29) in time
12:56:04 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:56:04 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:56:04 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:56:04 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:56:04 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:56:04 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:56:04 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:56:04 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:56:04 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:56:04 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:56:04 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-11 06:00:00 (before)
2024-11-11 09:00:00 (after)
12:56:04 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_wind to retrieve x_wind
12:56:08 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_wind to retrieve y_wind
12:56:11 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:56:11 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:56:11 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:56:11 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 720x361x1) for time after (2024-11-11 09:00:00)
12:56:11 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-11 06:00:00) in space (linearNDFast)
12:56:11 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:56:11 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-11 09:00:00) in space (linearNDFast)
12:56:11 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:56:11 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-11 06:00:00, weight 0.71) and
after (2024-11-11 09:00:00, weight 0.29) in time
12:56:11 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:56:11 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:56:11 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:56:11 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:56:11 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:56:11 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:56:11 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:56:11 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:56:11 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:56:11 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.821851 (min) 0.0693514 (max)
12:56:11 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.286825 (min) 0.345699 (max)
12:56:11 DEBUG opendrift.models.basemodel.environment:905: x_wind: -7.05676 (min) -2.32843 (max)
12:56:11 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0.101879 (min) 3.86167 (max)
12:56:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:56:11 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:56:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:56:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:56:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:56:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:56:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:56:11 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:56:11 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:56:11 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:56:11 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:56:11 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:56:11 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:56:11 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:56:11 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:56:11 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:56:11 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:56:11 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.449204, mean: 0.647591, max: 1.261842
12:56:11 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:56:11 DEBUG opendrift.models.physics_methods:1061: min: 3.651335, mean: 4.376558, max: 6.119726
12:56:11 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.651335, mean: 4.376558, max: 6.119726
12:56:11 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:56:11 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:56:11 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:56:11 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:56:11 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:56:11 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:56:11 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:56:11 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:56:11 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:56:11 DEBUG opendrift.models.physics_methods:1061: min: 3.651335, mean: 4.376558, max: 6.119727
12:56:11 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:56:11 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.024611, dN_50: 0.001931
12:56:11 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:56:11 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.0185119818320361
12:56:11 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:56:11 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:56:11 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:56:12 DEBUG opendrift.models.physics_methods:915: Advecting 1085 of 6000 elements above 0.100m with wind-sheared ocean current (0.018882 m/s - 0.171123 m/s)
12:56:12 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:56:12 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0006103068195744684 and 0.43305797711502986 m/s
12:56:12 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:56:12 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:56:12 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:56:12 INFO opendrift.models.basemodel:2038: 2024-11-11 07:51:18.915878 - step 116 of 120 - 6000 active elements (0 deactivated)
12:56:12 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:56:12 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:56:12 DEBUG opendrift.models.basemodel:2057: 34.36034137192979 <- latitude -> 35.152122194243546
12:56:12 DEBUG opendrift.models.basemodel:2062: 21.684924291884602 <- longitude -> 23.41638322894145
12:56:12 DEBUG opendrift.models.basemodel:2067: -50.6432438407247 <- z -> 0.0
12:56:12 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:56:12 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:56:12 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:56:12 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:56:12 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:56:12 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:56:12 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:56:12 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:56:12 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:56:12 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:56:12 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:56:12 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:56:12 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:56:12 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:56:12 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:56:12 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:56:12 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:56:12 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:56:12 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:56:12 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:56:12 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:56:12 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:56:12 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:56:12 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:56:12 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:56:12 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:56:12 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:56:12 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:56:12 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:56:12 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:56:12 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-11 06:00:00 (before)
2024-11-11 09:00:00 (after)
12:56:12 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-11 06:00:00) in space (linearNDFast)
12:56:12 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:56:12 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-11 09:00:00) in space (linearNDFast)
12:56:12 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:56:12 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-11 06:00:00, weight 0.38) and
after (2024-11-11 09:00:00, weight 0.62) in time
12:56:12 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:56:12 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:56:12 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:56:12 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:56:12 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:56:12 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:56:12 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:56:12 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:56:12 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:56:12 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:56:12 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-11 06:00:00 (before)
2024-11-11 09:00:00 (after)
12:56:12 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-11 06:00:00) in space (linearNDFast)
12:56:12 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:56:12 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-11 09:00:00) in space (linearNDFast)
12:56:12 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:56:12 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-11 06:00:00, weight 0.38) and
after (2024-11-11 09:00:00, weight 0.62) in time
12:56:12 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:56:12 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:56:12 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:56:12 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:56:12 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:56:12 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:56:12 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:56:12 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:56:12 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:56:12 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.851345 (min) 0.0261259 (max)
12:56:12 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.28282 (min) 0.384022 (max)
12:56:12 DEBUG opendrift.models.basemodel.environment:905: x_wind: -6.55016 (min) -2.36079 (max)
12:56:12 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0.578346 (min) 4.03865 (max)
12:56:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:56:12 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:56:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:56:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:56:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:56:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:56:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:56:12 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:56:12 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:56:12 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:56:12 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:56:12 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:56:12 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:56:12 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:56:12 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:56:12 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:56:12 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:56:12 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.470170, mean: 0.681271, max: 1.136578
12:56:12 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:56:12 DEBUG opendrift.models.physics_methods:1061: min: 3.735570, mean: 4.491394, max: 5.808035
12:56:12 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.735570, mean: 4.491394, max: 5.808035
12:56:12 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:56:12 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:56:12 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:56:12 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:56:12 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:56:12 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:56:12 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:56:12 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:56:12 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:56:12 DEBUG opendrift.models.physics_methods:1061: min: 3.735569, mean: 4.491394, max: 5.808035
12:56:12 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:56:12 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.024735, dN_50: 0.001941
12:56:12 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:56:12 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.016674708163727937
12:56:12 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:56:12 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:56:12 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:56:12 DEBUG opendrift.models.physics_methods:915: Advecting 1087 of 6000 elements above 0.100m with wind-sheared ocean current (0.000206 m/s - 0.168537 m/s)
12:56:12 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:56:12 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.002166793558045411 and 0.43894135414068536 m/s
12:56:12 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:56:12 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:56:12 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:56:12 INFO opendrift.models.basemodel:2038: 2024-11-11 08:51:18.915878 - step 117 of 120 - 6000 active elements (0 deactivated)
12:56:12 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:56:12 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:56:12 DEBUG opendrift.models.basemodel:2057: 34.36588492852661 <- latitude -> 35.16102187726866
12:56:12 DEBUG opendrift.models.basemodel:2062: 21.662970523781684 <- longitude -> 23.41377848104247
12:56:12 DEBUG opendrift.models.basemodel:2067: -49.14423373728795 <- z -> 0.0
12:56:12 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:56:12 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:56:12 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:56:12 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:56:12 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:56:12 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:56:12 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:56:12 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:56:12 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:56:12 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:56:12 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:56:12 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:56:12 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:56:12 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:56:12 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:56:12 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:56:12 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:56:12 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:56:12 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:56:12 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:56:12 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:56:12 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:56:12 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:56:12 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:56:12 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:56:12 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:56:12 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:56:12 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:56:12 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:56:12 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:56:12 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-11 06:00:00 (before)
2024-11-11 09:00:00 (after)
12:56:12 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-11 06:00:00) in space (linearNDFast)
12:56:12 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:56:12 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-11 09:00:00) in space (linearNDFast)
12:56:12 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:56:12 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-11 06:00:00, weight 0.05) and
after (2024-11-11 09:00:00, weight 0.95) in time
12:56:12 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:56:12 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:56:12 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:56:12 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:56:12 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:56:12 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:56:12 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:56:12 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:56:12 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:56:12 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:56:12 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-11 06:00:00 (before)
2024-11-11 09:00:00 (after)
12:56:12 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-11 06:00:00) in space (linearNDFast)
12:56:12 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:56:12 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-11 09:00:00) in space (linearNDFast)
12:56:12 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:56:12 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-11 06:00:00, weight 0.05) and
after (2024-11-11 09:00:00, weight 0.95) in time
12:56:12 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:56:12 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:56:12 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:56:12 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:56:12 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:56:12 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:56:12 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:56:12 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:56:12 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:56:12 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.861426 (min) 0.0260492 (max)
12:56:12 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.284134 (min) 0.404479 (max)
12:56:12 DEBUG opendrift.models.basemodel.environment:905: x_wind: -6.07742 (min) -2.36701 (max)
12:56:12 DEBUG opendrift.models.basemodel.environment:905: y_wind: 1.19026 (min) 4.22608 (max)
12:56:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:56:12 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:56:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:56:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:56:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:56:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:56:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:56:12 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:56:12 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:56:12 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:56:12 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:56:12 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:56:12 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:56:12 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:56:12 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:56:12 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:56:12 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:56:12 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.443022, mean: 0.731674, max: 1.046087
12:56:12 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:56:12 DEBUG opendrift.models.physics_methods:1061: min: 3.626119, mean: 4.654183, max: 5.572029
12:56:12 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.626119, mean: 4.654183, max: 5.572029
12:56:12 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:56:12 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:56:12 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:56:12 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:56:12 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:56:12 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:56:12 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:56:12 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:56:12 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:56:12 DEBUG opendrift.models.physics_methods:1061: min: 3.626119, mean: 4.654182, max: 5.572029
12:56:12 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:56:12 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.024766, dN_50: 0.001944
12:56:12 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:56:12 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.015347442879856269
12:56:12 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:56:12 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:56:12 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:56:12 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1083 surface elements
12:56:12 DEBUG opendrift.models.physics_methods:915: Advecting 1084 of 6000 elements above 0.100m with wind-sheared ocean current (0.001139 m/s - 0.174097 m/s)
12:56:12 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:56:12 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.001617745802806303 and 0.43838747934617933 m/s
12:56:12 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:56:12 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:56:12 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:56:12 INFO opendrift.models.basemodel:2038: 2024-11-11 09:51:18.915878 - step 118 of 120 - 6000 active elements (0 deactivated)
12:56:12 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:56:12 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:56:12 DEBUG opendrift.models.basemodel:2057: 34.372654288308745 <- latitude -> 35.17206737291213
12:56:12 DEBUG opendrift.models.basemodel:2062: 21.635227832152864 <- longitude -> 23.40666483423127
12:56:12 DEBUG opendrift.models.basemodel:2067: -48.684113832208126 <- z -> 0.0
12:56:12 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:56:12 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:56:12 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:56:12 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:56:12 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:56:12 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:56:12 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:56:12 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:56:12 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:56:12 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:56:12 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:56:12 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:56:12 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:56:12 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:56:12 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:56:12 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:56:12 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:56:12 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:56:12 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:56:12 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:56:12 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:56:12 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:56:12 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:56:12 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:56:12 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:56:12 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:56:12 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:56:12 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:56:12 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:56:12 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:56:12 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-11 09:00:00 (before)
2024-11-11 12:00:00 (after)
12:56:12 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
12:56:12 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
12:56:13 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:56:13 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
12:56:13 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
12:56:13 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
12:56:13 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 30x28x16) for time after (2024-11-11 12:00:00)
12:56:13 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-11 09:00:00) in space (linearNDFast)
12:56:13 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:56:13 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-11 12:00:00) in space (linearNDFast)
12:56:13 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:56:13 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-11 09:00:00, weight 0.71) and
after (2024-11-11 12:00:00, weight 0.29) in time
12:56:13 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:56:13 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:56:13 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:56:13 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:56:13 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:56:13 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:56:13 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:56:13 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:56:13 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:56:13 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:56:13 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-11 09:00:00 (before)
2024-11-11 12:00:00 (after)
12:56:13 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using eastward_wind to retrieve x_wind
12:56:16 DEBUG opendrift.readers.reader_netCDF_CF_generic:440: Using northward_wind to retrieve y_wind
12:56:18 DEBUG opendrift.readers.reader_netCDF_CF_generic:540: North is up, no rotation necessary
12:56:18 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
12:56:18 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
12:56:18 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 720x361x1) for time after (2024-11-11 12:00:00)
12:56:18 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-11 09:00:00) in space (linearNDFast)
12:56:18 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:56:18 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-11 12:00:00) in space (linearNDFast)
12:56:18 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:56:18 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-11 09:00:00, weight 0.71) and
after (2024-11-11 12:00:00, weight 0.29) in time
12:56:18 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:56:18 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:56:18 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:56:18 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:56:18 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:56:18 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:56:18 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:56:18 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:56:18 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:56:18 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.898663 (min) 0.000957355 (max)
12:56:18 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.262565 (min) 0.367308 (max)
12:56:18 DEBUG opendrift.models.basemodel.environment:905: x_wind: -5.92998 (min) -2.49489 (max)
12:56:18 DEBUG opendrift.models.basemodel.environment:905: y_wind: 1.0311 (min) 4.0845 (max)
12:56:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:56:18 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:56:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:56:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:56:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:56:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:56:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:56:18 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:56:18 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:56:18 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:56:18 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:56:18 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:56:18 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:56:18 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:56:18 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:56:18 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:56:18 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:56:18 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.461994, mean: 0.737424, max: 1.044534
12:56:18 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:56:18 DEBUG opendrift.models.physics_methods:1061: min: 3.702950, mean: 4.672093, max: 5.567893
12:56:18 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.702950, mean: 4.672093, max: 5.567893
12:56:18 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:56:18 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:56:18 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:56:18 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:56:18 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:56:18 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:56:18 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:56:18 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:56:18 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:56:18 DEBUG opendrift.models.physics_methods:1061: min: 3.702950, mean: 4.672092, max: 5.567893
12:56:18 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:56:18 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.025228, dN_50: 0.001980
12:56:18 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:56:18 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.015324669171113803
12:56:18 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:56:18 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:56:18 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:56:19 DEBUG opendrift.models.physics_methods:915: Advecting 1086 of 6000 elements above 0.100m with wind-sheared ocean current (0.007187 m/s - 0.176902 m/s)
12:56:19 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:56:19 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0008618147292253081 and 0.45196291556779145 m/s
12:56:19 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:56:19 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:56:19 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:56:19 INFO opendrift.models.basemodel:2038: 2024-11-11 10:51:18.915878 - step 119 of 120 - 6000 active elements (0 deactivated)
12:56:19 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:56:19 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:56:19 DEBUG opendrift.models.basemodel:2057: 34.36782011427233 <- latitude -> 35.18354211611749
12:56:19 DEBUG opendrift.models.basemodel:2062: 21.617344139097284 <- longitude -> 23.403909238011252
12:56:19 DEBUG opendrift.models.basemodel:2067: -47.53386623160524 <- z -> 0.0
12:56:19 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:56:19 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:56:19 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:56:19 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:56:19 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:56:19 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:56:19 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:56:19 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:56:19 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:56:19 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:56:19 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:56:19 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:56:19 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:56:19 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:56:19 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:56:19 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:56:19 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:56:19 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:56:19 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:56:19 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:56:19 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:56:19 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:56:19 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:56:19 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:56:19 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:56:19 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:56:19 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:56:19 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:56:19 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:56:19 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:56:19 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-11 09:00:00 (before)
2024-11-11 12:00:00 (after)
12:56:19 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-11 09:00:00) in space (linearNDFast)
12:56:19 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:56:19 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-11 12:00:00) in space (linearNDFast)
12:56:19 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:56:19 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-11 09:00:00, weight 0.38) and
after (2024-11-11 12:00:00, weight 0.62) in time
12:56:19 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:56:19 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:56:19 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:56:19 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:56:19 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:56:19 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:56:19 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:56:19 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:56:19 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:56:19 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:56:19 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-11 09:00:00 (before)
2024-11-11 12:00:00 (after)
12:56:19 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-11 09:00:00) in space (linearNDFast)
12:56:19 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:56:19 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-11 12:00:00) in space (linearNDFast)
12:56:19 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:56:19 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-11 09:00:00, weight 0.38) and
after (2024-11-11 12:00:00, weight 0.62) in time
12:56:19 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:56:19 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:56:19 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:56:19 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:56:19 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:56:19 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:56:19 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:56:19 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:56:19 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:56:19 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.897077 (min) 0.0183334 (max)
12:56:19 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.237928 (min) 0.412832 (max)
12:56:19 DEBUG opendrift.models.basemodel.environment:905: x_wind: -5.81301 (min) -2.63159 (max)
12:56:19 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0.808007 (min) 3.89723 (max)
12:56:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:56:19 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:56:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:56:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:56:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:56:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:56:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:56:19 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:56:19 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:56:19 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:56:19 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:56:19 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:56:19 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:56:19 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:56:19 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:56:19 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:56:19 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:56:19 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.493134, mean: 0.735160, max: 1.051730
12:56:19 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:56:19 DEBUG opendrift.models.physics_methods:1061: min: 3.825710, mean: 4.664591, max: 5.587039
12:56:19 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.825710, mean: 4.664591, max: 5.587039
12:56:19 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:56:19 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:56:19 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:56:19 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:56:19 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:56:19 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:56:19 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:56:19 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:56:19 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:56:19 DEBUG opendrift.models.physics_methods:1061: min: 3.825710, mean: 4.664591, max: 5.587039
12:56:19 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:56:19 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.025759, dN_50: 0.002022
12:56:19 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:56:19 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.015430217263919369
12:56:19 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:56:19 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:56:19 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:56:19 DEBUG opendrift.models.physics_methods:915: Advecting 1093 of 6000 elements above 0.100m with wind-sheared ocean current (0.010873 m/s - 0.178017 m/s)
12:56:19 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:56:19 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0016299246315648463 and 0.4430673946171603 m/s
12:56:19 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:56:19 DEBUG opendrift.models.basemodel:891: to be seeded: 0, already seeded 6000
12:56:19 DEBUG opendrift.models.basemodel:2037: ======================================================================
12:56:19 INFO opendrift.models.basemodel:2038: 2024-11-11 11:51:18.915878 - step 120 of 120 - 6000 active elements (0 deactivated)
12:56:19 DEBUG opendrift.models.basemodel:2044: 0 elements scheduled.
12:56:19 DEBUG opendrift.models.basemodel:2046: ======================================================================
12:56:19 DEBUG opendrift.models.basemodel:2057: 34.36085242795213 <- latitude -> 35.1936830216325
12:56:19 DEBUG opendrift.models.basemodel:2062: 21.60064481581765 <- longitude -> 23.40167673041313
12:56:19 DEBUG opendrift.models.basemodel:2067: -46.25284981604605 <- z -> 0.0
12:56:19 DEBUG opendrift.models.basemodel:2068: ---------------------------------
12:56:19 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:56:19 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
12:56:19 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:56:19 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
12:56:19 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:56:19 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:56:19 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 6000 elements
12:56:19 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:56:19 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:56:19 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:56:19 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:56:19 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:56:19 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:56:19 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:56:19 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:56:19 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:56:19 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:56:19 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:56:19 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:56:19 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:56:19 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:56:19 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:56:19 DEBUG opendrift.models.basemodel.environment:608: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
12:56:19 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:56:19 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
12:56:19 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:56:19 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:56:19 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
12:56:19 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:56:19 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-11 09:00:00 (before)
2024-11-11 12:00:00 (after)
12:56:19 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-11 09:00:00) in space (linearNDFast)
12:56:19 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:56:19 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-11 12:00:00) in space (linearNDFast)
12:56:19 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:56:19 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-11 09:00:00, weight 0.05) and
after (2024-11-11 12:00:00, weight 0.95) in time
12:56:19 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:56:19 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:56:19 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:56:19 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_wind', 'y_wind']
12:56:19 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:56:19 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
12:56:19 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:56:19 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:56:19 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
12:56:19 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
12:56:19 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-11-11 09:00:00 (before)
2024-11-11 12:00:00 (after)
12:56:19 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-11-11 09:00:00) in space (linearNDFast)
12:56:19 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:56:19 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-11-11 12:00:00) in space (linearNDFast)
12:56:19 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
12:56:19 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-11-11 09:00:00, weight 0.05) and
after (2024-11-11 12:00:00, weight 0.95) in time
12:56:19 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:56:19 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:56:19 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:56:19 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:56:19 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
12:56:19 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
12:56:19 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
12:56:19 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
12:56:19 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:56:19 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.836508 (min) 0.0492248 (max)
12:56:19 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.228613 (min) 0.480437 (max)
12:56:19 DEBUG opendrift.models.basemodel.environment:905: x_wind: -5.69035 (min) -2.75437 (max)
12:56:19 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0.51261 (min) 3.90873 (max)
12:56:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
12:56:19 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
12:56:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
12:56:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
12:56:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
12:56:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
12:56:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
12:56:19 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
12:56:19 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
12:56:19 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
12:56:19 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
12:56:19 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
12:56:19 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
12:56:19 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
12:56:19 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:56:19 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 20 (min) 20 (max)
12:56:19 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:56:19 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.486448, mean: 0.734575, max: 1.059335
12:56:19 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
12:56:19 DEBUG opendrift.models.physics_methods:1061: min: 3.799688, mean: 4.662091, max: 5.607202
12:56:19 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.799688, mean: 4.662091, max: 5.607202
12:56:19 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:56:19 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:56:19 DEBUG opendrift.models.basemodel:2109: Calling OpenOil.update()
12:56:19 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
12:56:19 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
12:56:19 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
12:56:19 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
12:56:19 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
12:56:19 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
12:56:19 DEBUG opendrift.models.physics_methods:1061: min: 3.799688, mean: 4.662091, max: 5.607202
12:56:19 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
12:56:19 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.026273, dN_50: 0.002062
12:56:19 DEBUG opendrift.models.oceandrift:521: Using diffusivity from Large1994 since model diffusivities not available
12:56:19 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.015541757139062239
12:56:19 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
12:56:19 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:environment
12:56:19 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
12:56:19 DEBUG opendrift.models.physics_methods:915: Advecting 1094 of 6000 elements above 0.100m with wind-sheared ocean current (0.085260 m/s - 0.179335 m/s)
12:56:19 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
12:56:19 DEBUG opendrift.models.basemodel:1672: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0017514011302482905 and 0.4319591425487651 m/s
12:56:19 DEBUG opendrift.models.basemodel:2124: 6000 active elements (0 deactivated)
12:56:19 DEBUG opendrift.models.basemodel:2153: Cleaning up
12:56:19 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
12:56:19 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
12:56:19 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
12:56:19 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
12:56:19 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
12:56:19 DEBUG opendrift.models.basemodel.environment:630: Data needed for 6000 elements
12:56:19 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 6000 elements
12:56:19 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
12:56:19 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
12:56:19 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
12:56:19 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
12:56:19 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
12:56:19 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
12:56:19 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
12:56:19 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
12:56:19 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
12:56:19 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
12:56:19 DEBUG opendrift.models.basemodel:2183: Writing and closing output file: oil.nc
12:56:19 INFO opendrift.export.io_netcdf:121: Wrote 21 steps to file oil.nc
12:56:20 DEBUG opendrift.export.io_netcdf:189: Making netCDF file CDM compliant with fixed dimensions
12:56:20 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:56:20 DEBUG opendrift.export.io_netcdf:294: Importing from oil.nc
/opt/conda/envs/opendrift/lib/python3.11/site-packages/numpy/ma/core.py:467: RuntimeWarning: invalid value encountered in cast
fill_value = np.array(fill_value, copy=False, dtype=ndtype)
12:56:22 DEBUG opendrift.models.basemodel:1710: No elements to deactivate
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:constant:x_sea_water_velocity -> None
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:fallback:x_sea_water_velocity -> None
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:constant:y_sea_water_velocity -> None
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:fallback:y_sea_water_velocity -> None
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:constant:x_wind -> None
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:fallback:x_wind -> None
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:constant:y_wind -> None
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:fallback:y_wind -> None
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:constant:sea_surface_height -> None
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:fallback:sea_surface_height -> 0
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:constant:upward_sea_water_velocity -> None
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:fallback:upward_sea_water_velocity -> 0
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:constant:sea_surface_wave_significant_height -> None
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:fallback:sea_surface_wave_significant_height -> 0
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:constant:sea_surface_wave_stokes_drift_x_velocity -> None
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:fallback:sea_surface_wave_stokes_drift_x_velocity -> 0
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:constant:sea_surface_wave_stokes_drift_y_velocity -> None
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:fallback:sea_surface_wave_stokes_drift_y_velocity -> 0
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:constant:sea_surface_wave_period_at_variance_spectral_density_maximum -> None
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:fallback:sea_surface_wave_period_at_variance_spectral_density_maximum -> 0
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:constant:sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment -> None
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:fallback:sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment -> 0
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:constant:sea_ice_area_fraction -> None
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:fallback:sea_ice_area_fraction -> 0
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:constant:sea_ice_x_velocity -> None
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:fallback:sea_ice_x_velocity -> 0
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:constant:sea_ice_y_velocity -> None
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:fallback:sea_ice_y_velocity -> 0
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:constant:sea_water_temperature -> None
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:fallback:sea_water_temperature -> 10
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:constant:sea_water_salinity -> None
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:fallback:sea_water_salinity -> 34
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:constant:sea_floor_depth_below_sea_level -> None
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:fallback:sea_floor_depth_below_sea_level -> 10000
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:constant:ocean_vertical_diffusivity -> None
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:fallback:ocean_vertical_diffusivity -> 0.02
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:constant:land_binary_mask -> None
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:fallback:land_binary_mask -> None
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:constant:ocean_mixed_layer_thickness -> 20.0
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:fallback:ocean_mixed_layer_thickness -> 50
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: general:use_auto_landmask -> True
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: drift:current_uncertainty -> 0.0
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: drift:current_uncertainty_uniform -> 0
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: drift:max_speed -> 1.3
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: readers:max_number_of_fails -> 1
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: general:simulation_name ->
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: general:coastline_action -> stranding
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: general:coastline_approximation_precision -> 0.001
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: general:time_step_minutes -> 60
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: general:time_step_output_minutes -> None
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: seed:ocean_only -> True
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: seed:number -> 1
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: drift:max_age_seconds -> None
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: drift:advection_scheme -> euler
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: drift:horizontal_diffusivity -> 20.0
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: drift:profiles_depth -> 50
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: drift:wind_uncertainty -> 0.0
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: drift:relative_wind -> False
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: drift:deactivate_north_of -> None
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: drift:deactivate_south_of -> None
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: drift:deactivate_east_of -> None
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: drift:deactivate_west_of -> None
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: seed:origin_marker -> 0
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: seed:z -> 0
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: seed:wind_drift_factor -> 0.03
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: seed:current_drift_factor -> 1
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: seed:terminal_velocity -> 0.0
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: seed:oil_film_thickness -> 0.001
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: drift:vertical_advection -> False
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: drift:vertical_mixing -> True
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: vertical_mixing:timestep -> 60
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: vertical_mixing:diffusivitymodel -> environment
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: vertical_mixing:background_diffusivity -> 1.2e-05
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: vertical_mixing:TSprofiles -> False
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: drift:wind_drift_depth -> 0.1
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: drift:stokes_drift -> True
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: drift:stokes_drift_profile -> Phillips
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: drift:use_tabularised_stokes_drift -> False
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: drift:tabularised_stokes_drift_fetch -> 25000
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: general:seafloor_action -> lift_to_seafloor
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: drift:truncate_ocean_model_below_m -> None
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: seed:seafloor -> False
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: seed:m3_per_hour -> 1
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: seed:droplet_size_distribution -> uniform
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: seed:droplet_diameter_mu -> 0.001
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: seed:droplet_diameter_sigma -> 0.0005
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: seed:droplet_diameter_min_subsea -> 0.0005
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: seed:droplet_diameter_max_subsea -> 0.005
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: processes:dispersion -> False
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: processes:evaporation -> True
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: processes:emulsification -> True
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: processes:biodegradation -> True
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: biodegradation:method -> half_time
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: processes:update_oilfilm_thickness -> False
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: wave_entrainment:droplet_size_distribution -> Johansen et al. (2015)
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: wave_entrainment:entrainment_rate -> Li et al. (2017)
12:56:22 DEBUG opendrift.export.io_netcdf:393: Setting imported config: seed:oil_type -> GENERIC MEDIUM CRUDE
12:56:22 DEBUG opendrift.models.basemodel:95: Changed mode from Mode.Result to Mode.Result
Plot and animate results
o.animation(color='z', markersize='mass_oil', markersize_scaling=80)
12:56:22 DEBUG opendrift.models.basemodel:2365: Setting up map: corners=None, fast=False, lscale=None
12:56:22 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:56:36 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:56:38 DEBUG opendrift.models.basemodel:3044: Saving animation..
12:56:38 INFO opendrift.models.basemodel:4613: Saving animation to /root/project/docs/source/gallery/animations/example_oilspill_seafloor_biodegradation_0.gif...
12:56:38 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:56:39 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:56:41 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:56:43 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:56:45 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:56:46 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:56:48 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:56:49 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:56:51 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:56:52 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:56:54 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:56:55 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:56:57 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:56:58 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:57:00 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:57:01 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:57:03 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:57:04 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:57:06 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:57:07 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:57:08 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:57:10 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:57:11 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:57:13 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:57:14 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:57:16 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:57:17 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:57:18 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:57:20 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:57:21 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:57:23 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:57:24 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:57:26 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:57:27 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:57:29 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:57:30 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:57:32 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:57:33 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:57:35 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:57:36 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:57:38 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:57:39 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:57:41 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:57:43 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:57:44 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:57:46 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:57:48 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:57:49 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:57:51 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:57:53 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:57:54 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:57:56 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:57:57 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:57:58 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:58:00 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:58:01 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:58:03 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:58:04 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:58:06 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:58:07 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:58:09 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:58:10 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:58:12 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:58:13 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:58:14 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:58:16 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:58:17 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:58:19 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:58:20 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:58:22 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:58:23 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:58:25 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:58:26 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:58:28 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:58:29 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:58:31 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:58:32 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:58:34 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:58:35 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:58:37 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:58:38 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:58:39 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:58:41 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:58:42 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:58:44 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:58:45 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:58:47 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:58:48 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:58:49 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:58:51 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:58:52 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:58:54 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:58:55 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:58:57 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:58:58 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:58:59 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:59:01 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:59:03 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:59:04 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:59:06 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:59:08 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:59:09 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:59:11 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:59:13 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:59:14 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:59:16 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:59:18 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:59:19 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:59:21 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:59:22 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:59:24 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:59:25 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:59:26 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:59:28 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:59:29 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:59:31 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:59:32 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:59:34 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:59:35 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:59:37 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:59:39 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:59:40 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:59:42 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:59:43 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:59:45 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:59:46 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:59:48 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:59:49 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:59:51 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:59:52 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:59:54 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:59:55 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:59:57 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
12:59:59 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:00:00 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:00:02 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:00:03 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:00:05 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:00:06 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:00:07 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:00:09 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:00:10 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:00:12 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:00:13 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:00:15 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:00:16 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:00:18 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:00:19 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:00:21 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:00:22 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:00:24 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:00:25 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:00:27 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:00:28 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:00:30 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:00:31 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:00:33 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:00:34 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:00:35 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:00:37 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:00:38 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:00:40 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:00:41 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:00:43 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:00:44 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:00:46 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:00:48 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:00:49 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:00:51 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:00:52 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:00:54 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:00:55 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:00:57 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:00:58 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:01:00 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:01:01 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:01:03 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:01:04 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:01:06 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:01:07 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:01:09 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:01:10 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:01:12 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:01:14 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:01:16 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:01:17 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:01:19 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:01:20 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:01:22 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:01:23 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:01:25 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:01:26 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:01:27 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:01:29 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:01:30 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:01:32 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:01:33 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:01:34 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:01:36 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:01:37 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:01:39 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:01:40 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:01:41 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:01:43 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:01:44 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:01:46 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:01:47 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:01:48 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:01:50 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:01:51 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:01:53 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:01:54 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:01:56 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:01:57 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:01:59 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:02:00 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:02:02 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:02:03 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:02:05 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:02:06 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:02:07 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:02:09 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:02:11 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:02:12 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:02:15 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:02:17 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:02:19 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:02:20 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:02:22 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:02:24 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:02:25 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:02:27 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:02:28 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:02:29 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:02:31 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:02:32 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:02:34 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:02:35 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:02:37 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:02:38 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:02:39 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:02:41 DEBUG opendrift.readers.reader_global_landmask:78: Adding GSHHG shapes from cartopy, scale: h, extent: (21.18811759948731, 24.018461608886714, 34.15508728027344, 35.399470520019534)..
13:02:44 DEBUG opendrift.models.basemodel:4651: MPLBACKEND = agg
13:02:44 DEBUG opendrift.models.basemodel:4652: DISPLAY = None
13:02:44 DEBUG opendrift.models.basemodel:4653: Time to save animation: 0:06:06.571157
13:02:44 INFO opendrift.models.basemodel:3037: Time to make animation: 0:06:22.285565
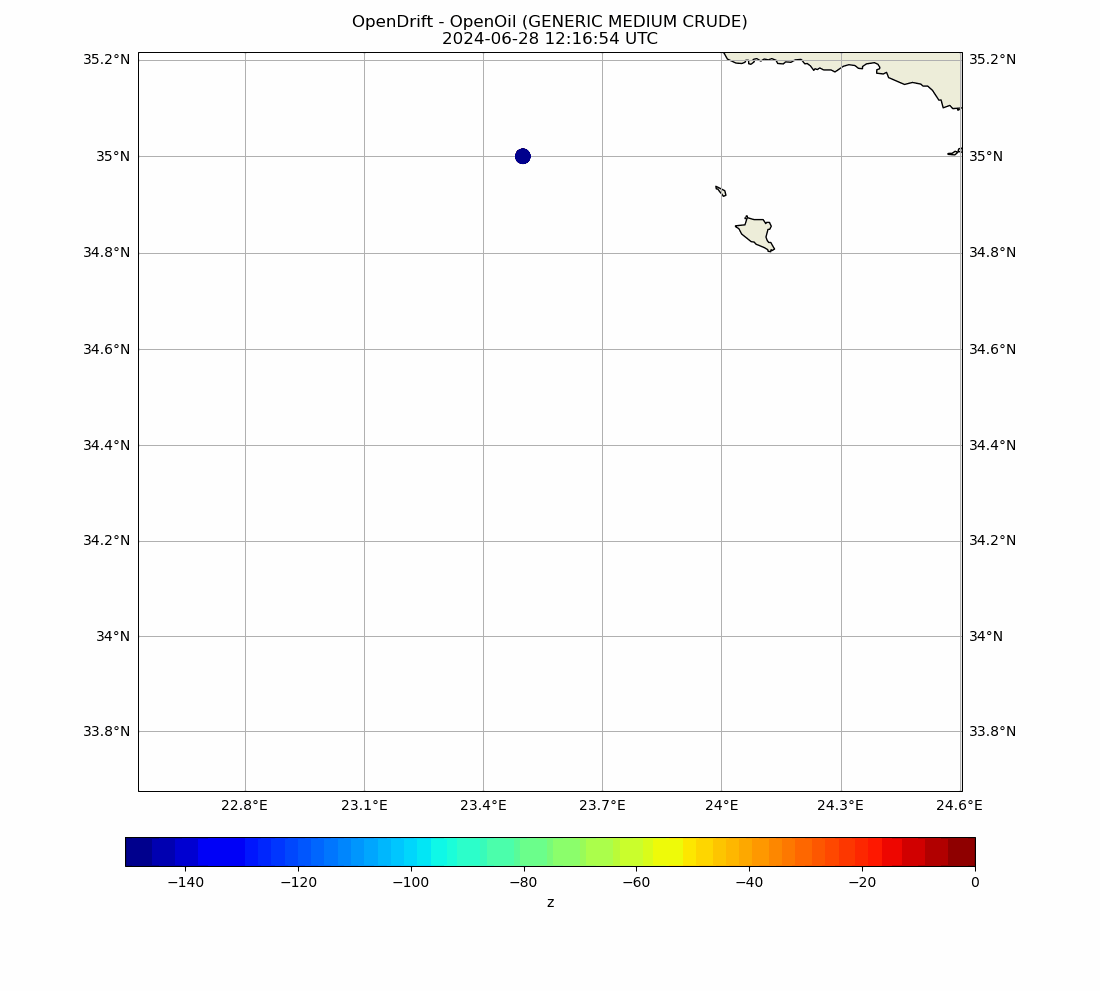
o.plot_oil_budget(show_watercontent_and_viscosity=False, show_wind_and_current=False)
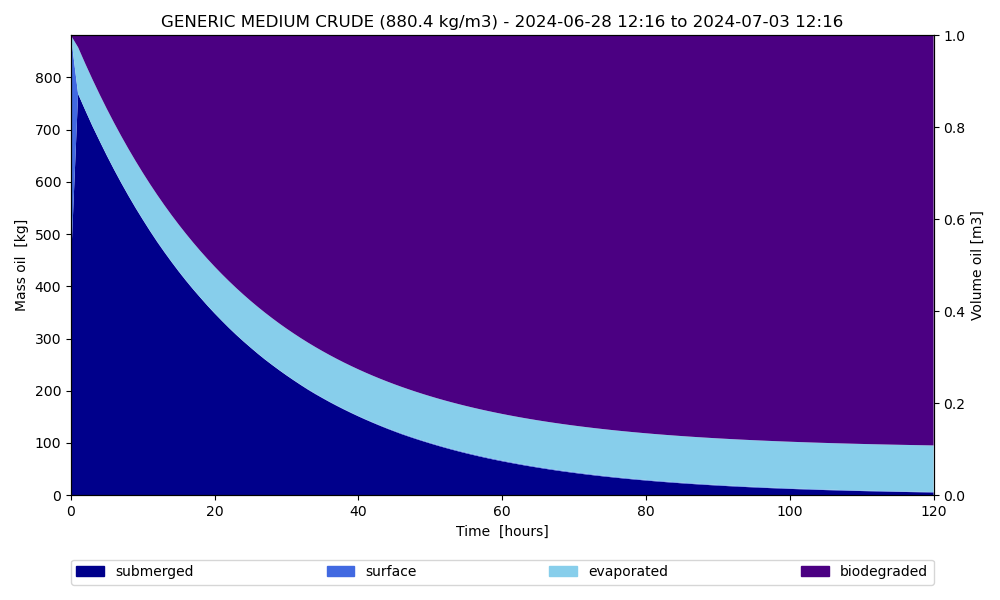
Custom oil budget plot
b = o.get_oil_budget()
import matplotlib.pyplot as plt
time, time_relative = o.get_time_array()
time = np.array([t.total_seconds() / 3600. for t in time_relative])
fig, ax = plt.subplots()
ax.plot(time, b['mass_submerged'], label='Submerged oil mass')
ax.plot(time, b['mass_surface'], label='Surface oil mass')
ax.plot(time, b['mass_biodegraded'], label='Biodegraded oil mass')
ax.set_title(f'{o.get_oil_name()}, {b["oil_density"].max():.2f} kg/m3')
plt.legend()
plt.xlabel('Time [hours]')
plt.ylabel('Mass oil [kg]')
plt.show()
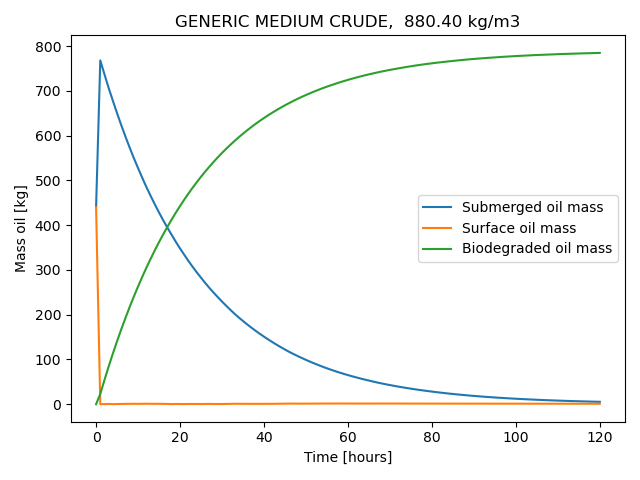
Animation of vertical behaviour
o.animation_profile(markersize='mass_oil', markersize_scaling=80, color='z', alpha=.5)
13:02:47 DEBUG opendrift.models.basemodel:3044: Saving animation..
13:02:47 INFO opendrift.models.basemodel:4613: Saving animation to /root/project/docs/source/gallery/animations/example_oilspill_seafloor_biodegradation_1.gif...
13:03:03 DEBUG opendrift.models.basemodel:4651: MPLBACKEND = agg
13:03:03 DEBUG opendrift.models.basemodel:4652: DISPLAY = None
13:03:03 DEBUG opendrift.models.basemodel:4653: Time to save animation: 0:00:15.858139
13:03:03 INFO opendrift.models.basemodel:3251: Time to make animation: 0:00:16.480210
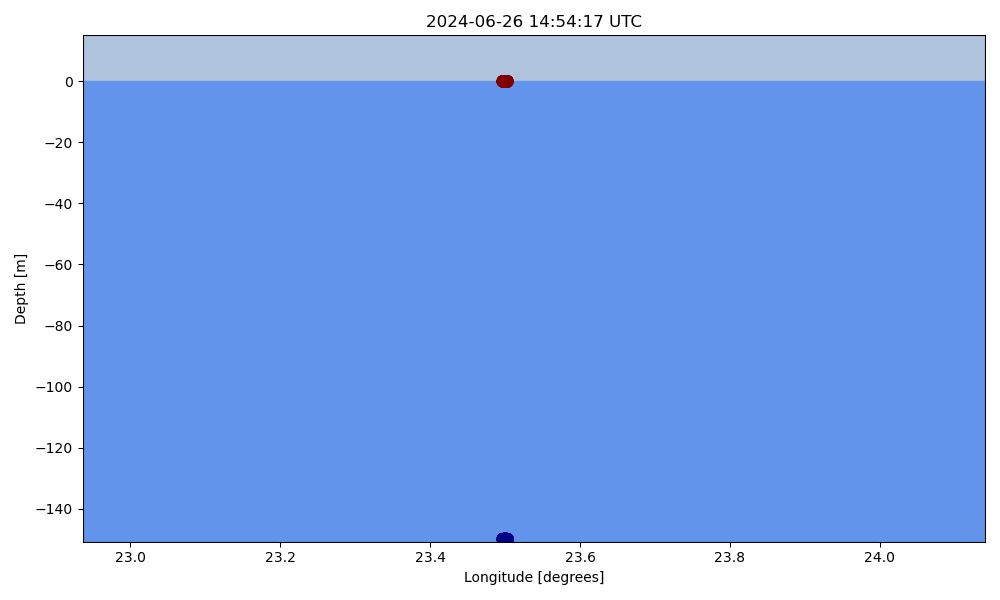
Total running time of the script: (11 minutes 52.417 seconds)