Note
Go to the end to download the full example code.
Show dominating source
import os
from datetime import datetime
import xarray as xr
import opendrift
from opendrift.models.oceandrift import OceanDrift
of = 'test.nc'
Seed elements at 5 different locations/longitudes
lons = [4, 4.2, 4.3, 4.32, 4.6]
t = datetime.now()
o = OceanDrift(loglevel=20)
o.set_config('environment:constant:y_sea_water_velocity', .1)
for i, lon in enumerate(lons):
o.seed_elements(lon=lon, lat=60, radius=3000, number=2000, time=t, origin_marker_name='Lon %f' % lon)
o.run(steps=15, outfile=of)
17:14:50 INFO opendrift:509: OpenDriftSimulation initialised (version 1.13.0 / v1.13.0-15-g93fccf7)
17:14:50 INFO opendrift.models.basemodel.environment:206: Adding a dynamical landmask with max. priority based on assumed maximum speed of 2.0 m/s. Adding a customised landmask may be faster...
17:14:54 INFO opendrift.models.basemodel.environment:233: Fallback values will be used for the following variables which have no readers:
17:14:54 INFO opendrift.models.basemodel.environment:236: x_sea_water_velocity: 0.000000
17:14:54 INFO opendrift.models.basemodel.environment:236: sea_surface_height: 0.000000
17:14:54 INFO opendrift.models.basemodel.environment:236: x_wind: 0.000000
17:14:54 INFO opendrift.models.basemodel.environment:236: y_wind: 0.000000
17:14:54 INFO opendrift.models.basemodel.environment:236: upward_sea_water_velocity: 0.000000
17:14:54 INFO opendrift.models.basemodel.environment:236: ocean_vertical_diffusivity: 0.000000
17:14:54 INFO opendrift.models.basemodel.environment:236: sea_surface_wave_significant_height: 0.000000
17:14:54 INFO opendrift.models.basemodel.environment:236: sea_surface_wave_stokes_drift_x_velocity: 0.000000
17:14:54 INFO opendrift.models.basemodel.environment:236: sea_surface_wave_stokes_drift_y_velocity: 0.000000
17:14:54 INFO opendrift.models.basemodel.environment:236: sea_surface_wave_period_at_variance_spectral_density_maximum: 0.000000
17:14:54 INFO opendrift.models.basemodel.environment:236: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0.000000
17:14:54 INFO opendrift.models.basemodel.environment:236: sea_surface_swell_wave_to_direction: 0.000000
17:14:54 INFO opendrift.models.basemodel.environment:236: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0.000000
17:14:54 INFO opendrift.models.basemodel.environment:236: sea_surface_swell_wave_significant_height: 0.000000
17:14:54 INFO opendrift.models.basemodel.environment:236: sea_surface_wind_wave_to_direction: 0.000000
17:14:54 INFO opendrift.models.basemodel.environment:236: sea_surface_wind_wave_mean_period: 0.000000
17:14:54 INFO opendrift.models.basemodel.environment:236: sea_surface_wind_wave_significant_height: 0.000000
17:14:54 INFO opendrift.models.basemodel.environment:236: surface_downward_x_stress: 0.000000
17:14:54 INFO opendrift.models.basemodel.environment:236: surface_downward_y_stress: 0.000000
17:14:54 INFO opendrift.models.basemodel.environment:236: turbulent_kinetic_energy: 0.000000
17:14:54 INFO opendrift.models.basemodel.environment:236: turbulent_generic_length_scale: 0.000000
17:14:54 INFO opendrift.models.basemodel.environment:236: ocean_mixed_layer_thickness: 50.000000
17:14:54 INFO opendrift.models.basemodel.environment:236: sea_floor_depth_below_sea_level: 10000.000000
17:14:54 INFO opendrift:919: Using existing reader for land_binary_mask
17:14:54 INFO opendrift:930: All points are in ocean
17:14:54 INFO opendrift:2056: 2025-02-18 17:14:50.160183 - step 1 of 15 - 10000 active elements (0 deactivated)
17:14:55 INFO opendrift:2056: 2025-02-18 18:14:50.160183 - step 2 of 15 - 10000 active elements (0 deactivated)
17:14:55 INFO opendrift:2056: 2025-02-18 19:14:50.160183 - step 3 of 15 - 10000 active elements (0 deactivated)
17:14:55 INFO opendrift:2056: 2025-02-18 20:14:50.160183 - step 4 of 15 - 10000 active elements (0 deactivated)
17:14:55 INFO opendrift:2056: 2025-02-18 21:14:50.160183 - step 5 of 15 - 10000 active elements (0 deactivated)
17:14:55 INFO opendrift:2056: 2025-02-18 22:14:50.160183 - step 6 of 15 - 10000 active elements (0 deactivated)
17:14:55 INFO opendrift:2056: 2025-02-18 23:14:50.160183 - step 7 of 15 - 10000 active elements (0 deactivated)
17:14:56 INFO opendrift:2056: 2025-02-19 00:14:50.160183 - step 8 of 15 - 10000 active elements (0 deactivated)
17:14:56 INFO opendrift:2056: 2025-02-19 01:14:50.160183 - step 9 of 15 - 10000 active elements (0 deactivated)
17:14:56 INFO opendrift:2056: 2025-02-19 02:14:50.160183 - step 10 of 15 - 10000 active elements (0 deactivated)
17:14:56 INFO opendrift:2056: 2025-02-19 03:14:50.160183 - step 11 of 15 - 10000 active elements (0 deactivated)
17:14:56 INFO opendrift:2056: 2025-02-19 04:14:50.160183 - step 12 of 15 - 10000 active elements (0 deactivated)
17:14:56 INFO opendrift:2056: 2025-02-19 05:14:50.160183 - step 13 of 15 - 10000 active elements (0 deactivated)
17:14:56 INFO opendrift:2056: 2025-02-19 06:14:50.160183 - step 14 of 15 - 10000 active elements (0 deactivated)
17:14:56 INFO opendrift:2056: 2025-02-19 07:14:50.160183 - step 15 of 15 - 10000 active elements (0 deactivated)
Calculate spatial density of elements at 1500m grid spacing
d = o.get_histogram(pixelsize_m=1500, weights=None)
dom = d.argmax(dim='origin_marker', skipna=True)
dom = dom.where(d.sum(dim='origin_marker')>0)
dom.name = 'Dominating source'
17:14:58 INFO opendrift:3895: calculating for origin_marker 0...
17:14:58 INFO opendrift:3895: calculating for origin_marker 1...
17:14:58 INFO opendrift:3895: calculating for origin_marker 2...
17:14:58 INFO opendrift:3895: calculating for origin_marker 3...
17:14:58 INFO opendrift:3895: calculating for origin_marker 4...
Show which of the 5 sources are dominating within each grid cell
o.animation(background=dom, show_elements=False, bgalpha=1,
legend=list(o.origin_marker.values()), colorbar=False, vmin=0, vmax=4)
/opt/conda/envs/opendrift/lib/python3.11/site-packages/cartopy/mpl/geoaxes.py:1692: UserWarning: No data for colormapping provided via 'c'. Parameters 'cmap', 'vmin', 'vmax' will be ignored
result = super().scatter(*args, **kwargs)
17:15:14 INFO opendrift:4611: Saving animation to /root/project/docs/source/gallery/animations/example_dominating_0.gif...
17:15:26 INFO opendrift:3048: Time to make animation: 0:00:28.155143
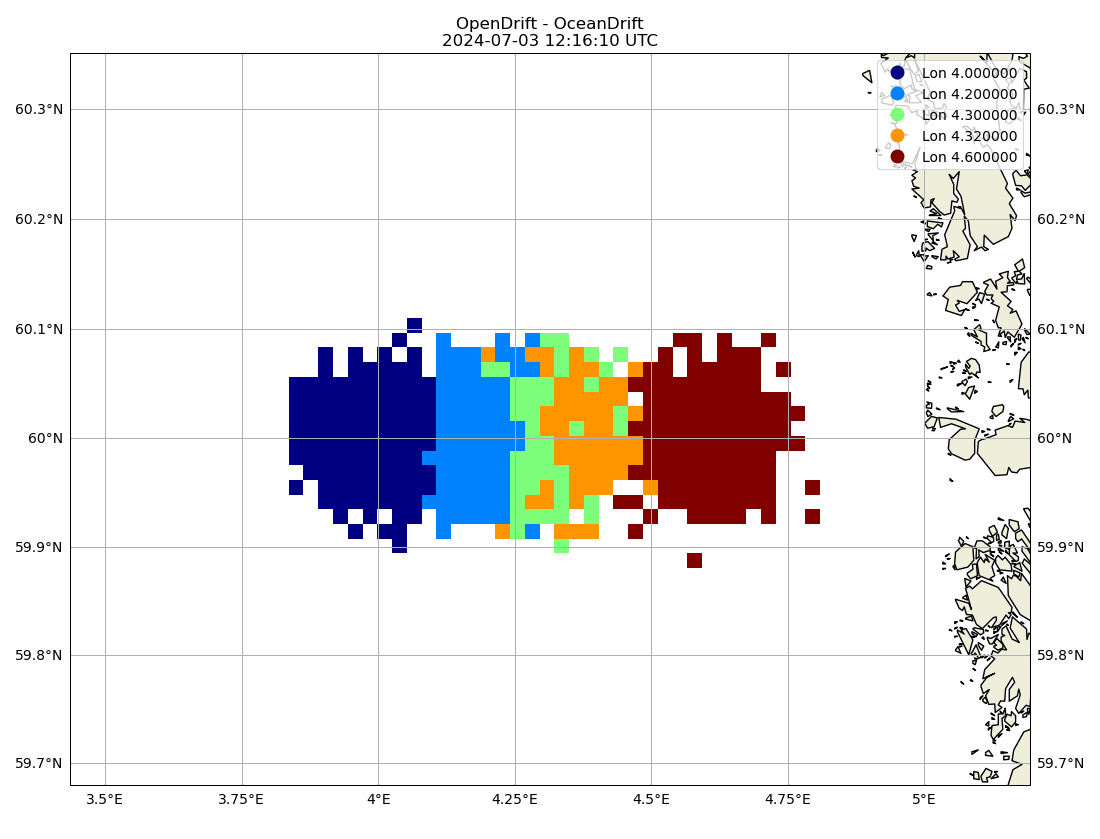
os.remove(of)
Total running time of the script: (0 minutes 41.238 seconds)