Working with CSS Preprocessors
Many developers generate CSS style sheets using a CSS preprocessor, such as Sass, Less, or Stylus. Because the CSS files are generated, editing the CSS files directly is not as helpful.
For preprocessors that support CSS source maps, DevTools lets you live-edit your preprocessor source files in the Sources panel, and view the results without having to leave DevTools or refresh the page. When you inspect an element whose styles are provided by a generated CSS file, the Elements panel displays a link to the original source file, not the generated .css file.
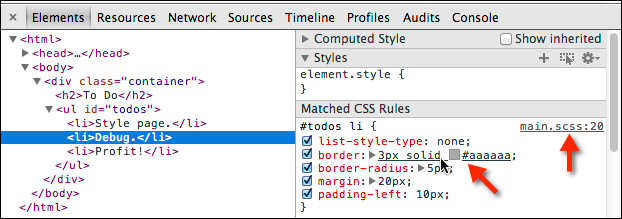
To jump to the source file:
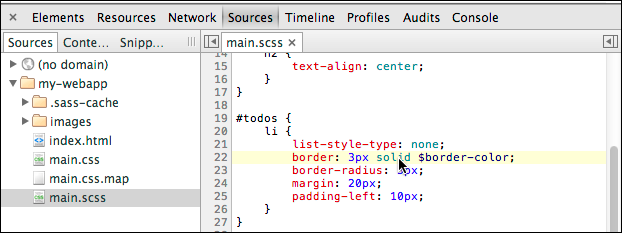
When you save changes to a CSS preprocessor file in DevTools, the CSS preprocessor should re-generate the CSS files. Then DevTools reloads the newly-generated CSS file.
Requirements
There are a few requirements to note when working with a CSS preprocessor:
To use this workflow, your CSS preprocessor must support CSS source maps, specifically the Source Map v3 proposal. The CSS source maps must be built along with the CSS files, so DevTools can map each CSS property to the correct location in the original source file (for example, .scss file).
For the DevTools to automatically reload styles when you change the source file, your preprocessor must be set up to regenerate CSS files whenever a source file changes. Otherwise, you must regenerate CSS files manually and reload the page to see your changes.
You must be accessing your site or app from a web server (not a file:// URL), and the server must serve the CSS files as well as the source maps (.css.map) and source files (.scss, etc.).
If you are not using the Workspaces feature, the web server must also supply the
Last-Modified
header. The Python SimpleHTTPServer
module provides this header by default.
You can start a web server to serve the contents of the current directory like this:
python -m SimpleHTTPServer
Enabling CSS source maps
CSS source maps are enabled by default. You can choose to enable automatic reloading of generated CSS files.
To enable CSS source maps and CSS reload:
Open DevTools Settings and click General.
Turn on Enable CSS source maps and Auto-reload generated CSS.
Using Sass with CSS source maps
To live-edit Sass files in Chrome you need to have the pre-release version of the Sass compiler, which is the only version that currently supports source map generation.
gem install sass -v '>=3.3.0alpha' --pre
Once Sass is installed, start the Sass compiler to watch for changes to your Sass source files and create source map files for each generated CSS file, for example:
sass --watch --sourcemap sass/styles.scss:styles.css
If you are using Compass, note that Compass doesn’t yet support the pre-release version of Sass, so you can’t use Sass debugging with Compass.
CSS preprocessor support
DevTools supports the Source Map Revision 3 proposal. This proposal is being implemented in several CSS preprocessors:
How CSS source maps work
For each CSS file it produces, the preprocessor generates a source map file (.map file) in addition to the compiled CSS. The source map file is a JSON file that defines a mapping between each generated CSS declaration and the corresponding line of the source file. Each CSS file contains an annotation that specifies the URL of its source map file, embedded in a special comment on the last line of the file:
/*# sourceMappingURL=<url> */
For instance, given an SCSS file named styles.scss:
$textSize: 26px; $fontColor: red; $bgColor: whitesmoke; h2 { font-size: $textSize; color: $fontColor; background: $bgColor; }
Sass generates a CSS file, styles.css, with the sourceMappingURL annotation:
h2 { font-size: 26px; color: red; background-color: whitesmoke; } /*# sourceMappingURL=styles.css.map */
Below is an example source map file:
{ "version": "3", "mappings":"AAKA,EAAG;EACC,SAAS,EANF,IAAI;EAOX,KAAK" "sources": ["sass/styles.scss"], "file": "styles.css" }
Resources
Many users have developed their own workflows using CSS preprocessors. See the following external articles for tutorials and alternate workflows.